Learn Python Programming for Free: An In-Depth Guide
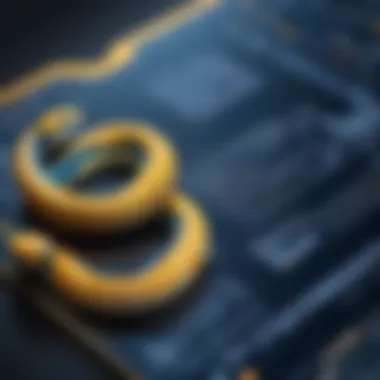
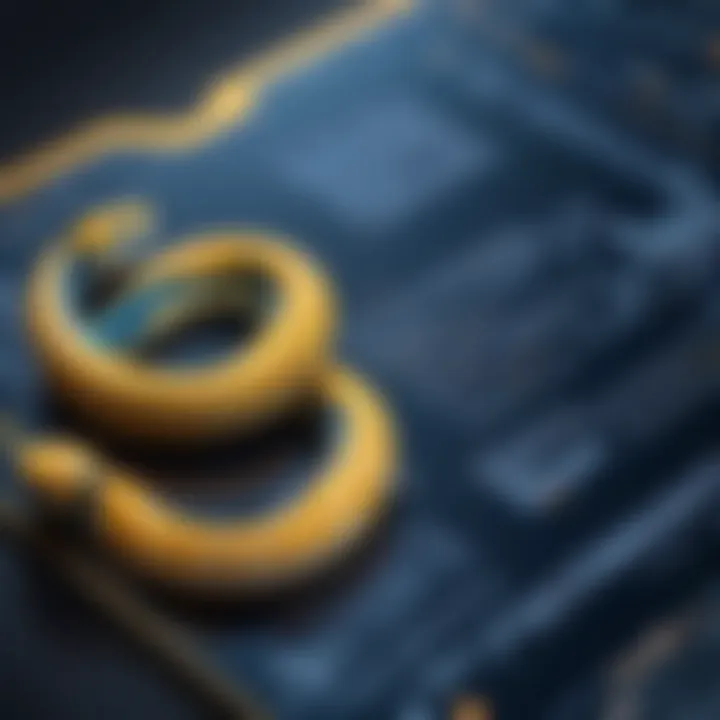
Overview of Topic
Learning Python programming is becoming increasingly relevant in today's technology-focused world. Organizations across industries look for skilled programmers who can efficiently solve problems and create solutions. Python, owing to its versatility and simplicity, has emerged as a preferred choice for beginners and experienced developers alike.
The significance of Python in the tech industry cannot be overstated. It is widely used in data science, web development, artificial intelligence, and more. This guide aims to explore free resources available for learning Python, thus making it accessible for anyone who wishes to embark on this programming journey.
Historically, Python was created by Guido van Rossum and first released in 1991. Its unique syntax emphasizes readability and ease of learning, which contributes to its popularity among new coders. Over time, Python has evolved, gaining support from numerous libraries and frameworks that extend its capabilities.
Fundamentals Explained
To grasp Python programming, one must understand some core principles.
Core Principles
Python follows the principles of object-oriented programming, which allows for the structuring of software in a modular way. This can make complex systems easier to manage and develop.
Key Terminology
- Variable: A storage location for data that can change during execution.
- Function: A block of code that performs a specific task and can be reused.
- Loop: A programming structure that repeats a block of code as long as a condition is met.
These terms form the building blocks of understanding Python coding. New learners should familiarize themselves with these concepts to solidify their foundational knowledge.
Practical Applications and Examples
Python's practical applications are vast and varied.
Real-world Case Studies
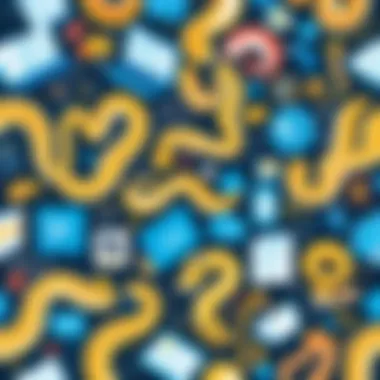
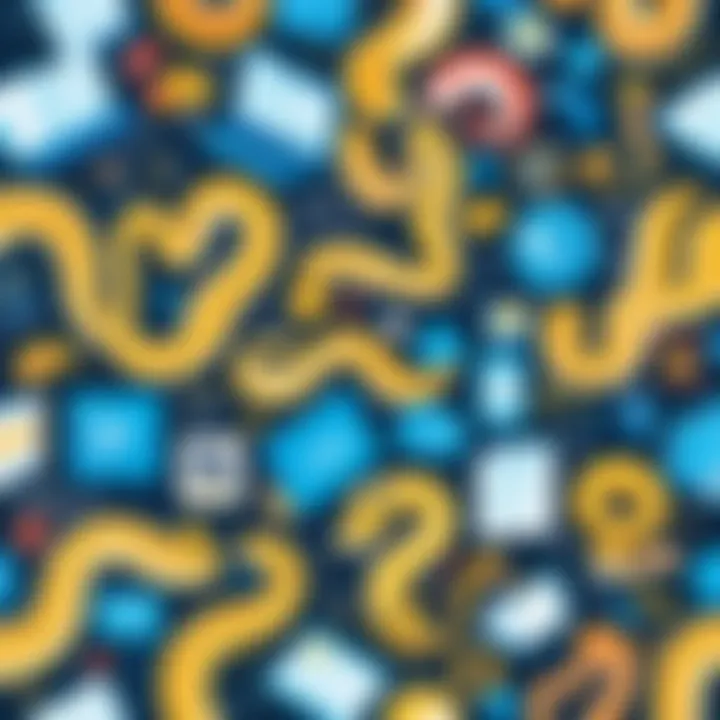
- Web Development: Frameworks like Django enable developers to create robust websites.
- Data Analysis: Libraries such as Pandas and NumPy help analyze large datasets efficiently.
- Machine Learning: Python’s simplicity allows for quick development of machine learning models with tools like TensorFlow.
Demonstrations and Hands-on Projects
Engaging in practical projects enhances learning. Starting with small projects, like a simple calculator or a to-do list application, can provide immediate feedback and boost confidence.
Code Snippet
Here's a simple Python function that adds two numbers:
This function, when invoked, returns the sum of and , illustrating basic function creation.
Advanced Topics and Latest Trends
Python continues to grow and evolve. Some advanced topics to consider include:
- Asynchronous Programming: This allows for more efficient code execution and better performance in I/O operations.
- Web Scraping: Used to extract data from websites, a skill increasingly necessary in data analysis.
- Blockchain Technology: Python is often used to develop blockchain applications, a field that is gaining traction.
Keeping abreast of these trends ensures that learners remain relevant in a competitive market.
Tips and Resources for Further Learning
A wealth of resources exist for those looking to deepen their understanding of Python:
- Books: "Automate the Boring Stuff with Python" offers practical examples for beginners.
- Online Courses: Websites like Coursera and edX provide free insights into Python programming.
- Communities: Engaging with groups on platforms like Reddit and Stack Overflow can provide support and additional learning opportunities.
Recommended Tools
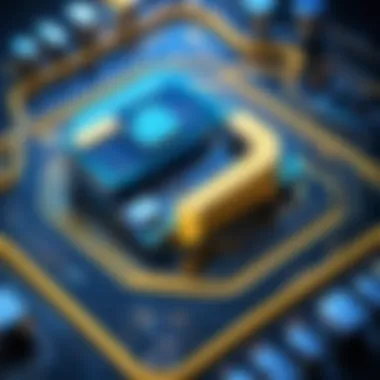
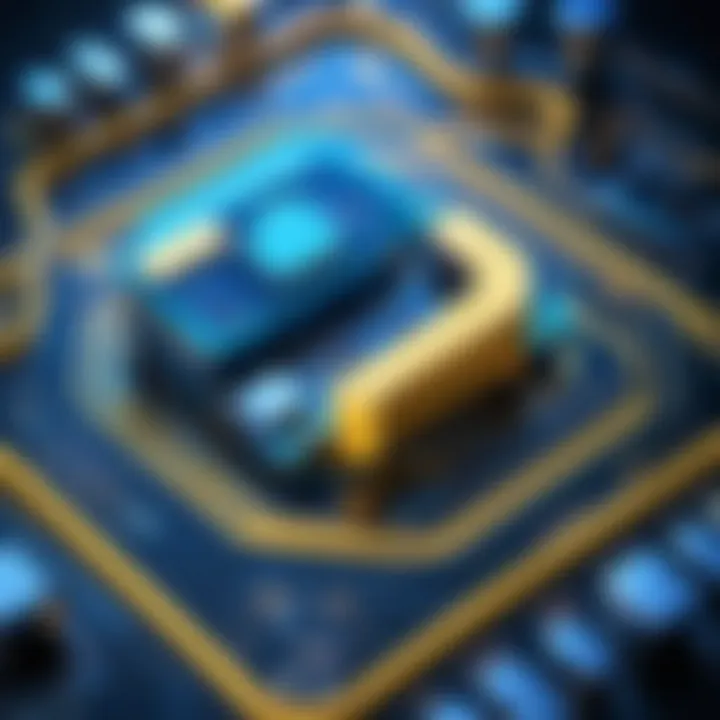
- IDLE: Python's built-in Integrated Development and Learning Environment.
- Jupyter Notebook: A popular tool for coding, especially for data science projects.
Learning Python need not be an expensive endeavor. By utilizing the vast array of free resources available, anyone can start their programming journey effectively.
Intro to Python Programming
Understanding Python programming is crucial for anyone seeking to delve into the world of software development or data analysis. This section introduces the fundamental aspects of Python, setting the foundation for further exploration of the language. As the use of Python continues to grow in various industries, particularly in data science and web development, it becomes evident why it is essential to learn this programming language.
Python is not just a programming language but an enabler of creativity and innovation. Its syntax and structure are designed to be intuitive, allowing learners to focus more on problem-solving rather than grappling with complex coding issues. Here, we explore what Python is and why it holds great significance in the modern tech landscape.
What is Python?
Python is a high-level, interpreted programming language created by Guido van Rossum and first released in 1991. Its design philosophy emphasizes code readability and simplicity. Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming.
One of the defining features of Python is its extensive standard library, which supports many common programming tasks, such as string manipulation, data serialization, and file handling. Additionally, the language’s versatility makes it ideal for various applications ranging from web development to machine learning. The syntax is clean, using indentation to define code blocks, which is different from many other programming languages. This can make Python more accessible to beginners.
Importance of Learning Python
The significance of learning Python cannot be overstated. Here are several reasons why individuals should consider investing their time in mastering it:
- Widely Used: Python is one of the most popular programming languages today. It is used by companies like Google, Facebook, and Netflix, showcasing its versatility and reliability.
- Job Opportunities: As industries increasingly adopt data-driven decision-making, the demand for Python skills has surged. Many tech job postings now list Python as a key requirement.
- Community and Resources: The Python community is extensive and welcoming, providing abundant resources—from tutorials to forums—where learners can seek help and share knowledge.
- Frameworks and Libraries: Python offers powerful frameworks, such as Django for web development and Pandas for data analysis, which can significantly accelerate development processes.
In summary, learning Python opens doors to numerous opportunities in tech and beyond, making it an essential skill for anyone interested in programming. As this guide unfolds, it will provide a roadmap for acquiring Python knowledge effectively and without cost.
Understanding the Basics of Python
The foundational knowledge of Python programming is critical for anyone seeking to master the language. Without understanding the basics, advanced topics can seem overwhelming. Python is designed with a simple and clear syntax, which allows newcomers to grasp programming concepts more rapidly compared to languages like C++ or Java. This section will highlight key components necessary for effective learning.
Python Syntax and Semantics
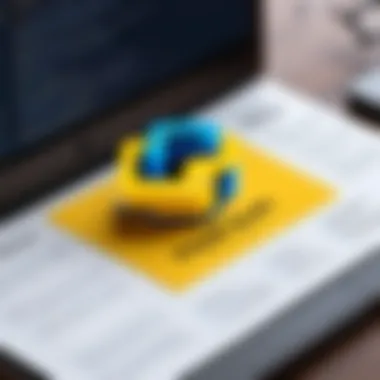
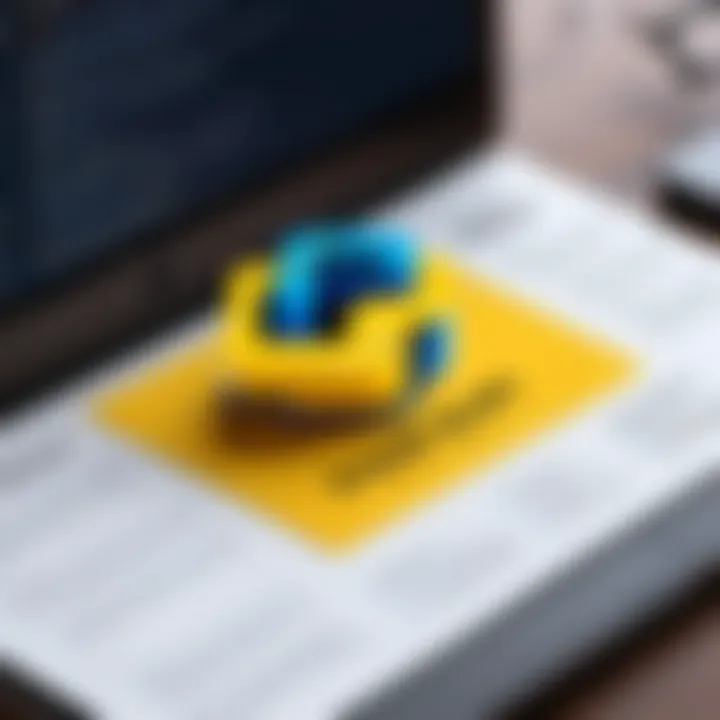
Python's syntax is the set of rules that defines the combinations of symbols that are considered to be correctly structured programs. One aspect of Python's syntax that sets it apart is its emphasis on readability. For instance, Python uses indentation to denote block structures instead of braces or keywords, which helps maintain clarity. This makes it easier for beginners to follow and understand code.
Key elements of Python syntax include:
- Variables: These are used to store data. Naming conventions in Python are straightforward, allowing alphanumeric characters and underscores. Thus, a valid variable name could be .
- Comments: Python allows the use of comments to annotate your code. Comments begin with a hashtag (#) and are ignored during execution.
- Functions: Functions are defined using the keyword. A simple function could look like this:
This focus on a clean syntax not only aids learning but also fosters good programming practices as learners become accustomed to writing clear and understandable code.
Data Types and Variables
Understanding data types is essential because they define what kind of data your variable can hold. Python has several built-in data types, including:
- Integers: Whole numbers, such as 1, 2, 3.
- Floats: Decimal numbers, like 1.5 or 3.14.
- Strings: Sequences of characters, enclosed in quotation marks. For example, is a string.
- Booleans: Represents one of two values: or .
These types can be defined implicitly. For instance:
Knowing data types is crucial for data handling and manipulation. It ensures that operations you apply to data will produce the expected results. Students should practice defining various data types and using them in simple programs.
Control Structures in Python
Control structures allow for decision-making in your code. Python includes several types of control structures:
- Conditional Statements: Use , , and to execute different blocks of code based on certain conditions. For example:
- Loops: Python supports and loops, which are helpful for repeated execution of code blocks. A simple loop example is:
for i in range(5):# Prints numbers from 0 to 4 print(i)