Managing Node.js Versions in AWS Environments
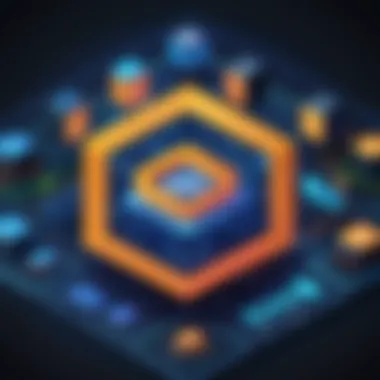
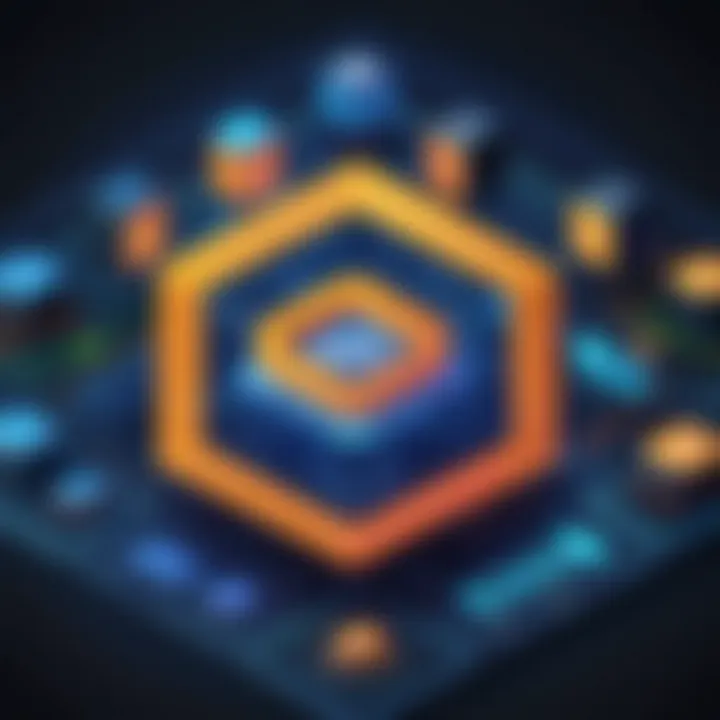
Overview of Topic
Node.js has transformed the landscape of server-side programming. With its asynchronous, non-blocking architecture, it allows developers to build dynamic and scalable applications efficiently. However, managing Node.js versions in various environments—especially in cloud settings like AWS—presents a unique set of challenges worth exploring.
Understanding how to navigate these challenges is crucial for developers looking to leverage AWS's capabilities effectively. The tech industry increasingly sees companies migrate to cloud solutions, and with Node.js's growing popularity, mastering version management is not just beneficial, but essential.
Preamble to the Main Concept Covered
At its core, this article dives deep into the intricacies of how to handle different Node.js versions in AWS. We will elucidate why proper management is vital for application stability and performance. You'll find that not all applications run smoothly with the same Node.js version. Picking the right version can impact everything from security to the efficiency of your application.
Scope and Significance in the Tech Industry
As Node.js continues to evolve, so too does the importance of keeping pace with version updates. This is particularly pertinent within AWS environments, which frequently undergo updates and introduce new features that may or may not support earlier Node.js versions.
In the past few years, the tech industry has seen a rise in the number of cloud-native applications, and having a strong grasp on version control helps ensure these applications utilize the latest features while maintaining compatibility with existing systems.
Brief History and Evolution
Node.js was first introduced in 2009. Over the years, it has seen a steady stream of versions, each adding new functionalities and improvements. AWS, as a leading cloud provider, has continuously adapted its services to support the latest versions of Node.js, providing developers with tools like AWS Elastic Beanstalk and AWS Lambda for deployment. Keeping updated on Node.js versions within AWS environments provides a comprehensive framework for modern web application development.
Fundamentals Explained
To grasp the nuances of Node.js version management, it’s crucial to understand some of the foundational concepts surrounding it.
Core Principles and Theories Related to the Topic
Node.js operates on a V8 JavaScript engine, which translates JavaScript into machine code. This allows Node.js to execute code without the need for a browser, making it particularly well-suited for server-side applications. The non-blocking nature means that other operations aren’t waiting on I/O operations, allowing asynchronous programming to shine.
Key Terminology and Definitions
- Node.js Versioning: Refers to the practice of maintaining and potentially switching between different Node.js iterations.
- LTS (Long-Term Support): The versions that get the longest support and are stable over time, recommended for production applications.
- Semantic Versioning: A versioning scheme that uses a format of MAJOR.MINOR.PATCH to signal backwards compatibility.
Basic Concepts and Foundational Knowledge
When developing in Node.js, it's imperative to understand the distinction between the different types of versions, especially when deploying in AWS. For instance, the differences between LTS and current releases can affect application performance and security.
Practical Applications and Examples
Understanding Node.js version management can lead to more reliable production environments. Here are some practical applications:
Real-World Case Studies and Applications
Consider a scenario where a startup is deploying a microservices architecture. Using Docker on AWS allows teams to specify their Node.js version per container, ensuring each microservice functions with the appropriate dependencies without conflict.
Demonstrations and Hands-On Projects
Imagine you want to use AWS Lambda for a serverless application. You can specify the Node.js runtime version in your function configuration. This helps avoid incompatibilities, especially when running older libraries that expect earlier Node.js versions.
Code Snippets and Implementation Guidelines
Here’s how you might specify a Node.js version in an AWS Lambda deployment:
This snippet illustrates how to set a specific Node.js version while deploying a Lambda function. Consistently defining the Node.js version helps ensure the environments mirror development setups.
Advanced Topics and Latest Trends
With technology continually advancing, staying abreast of the newest developments in Node.js is important.
Cutting-Edge Developments in the Field
Recent updates have focused on performance optimizations and improved support for HTTP/2, enhancing the capabilities of Node.js in handling concurrent connections—a boon for microservices frameworks.
Advanced Techniques and Methodologies
Utilizing tools like nvm (Node Version Manager) allows for rapid version switching on local development environments while ensuring that your production stacks remain stable and predictable.
Future Prospects and Upcoming Trends
Looking ahead, the integration of TypeScript with Node.js is gaining traction, which may alter how we think about version management. This trend demands that developers remain adaptable and knowledgeable about how these languages and frameworks interact.
Tips and Resources for Further Learning
Staying informed about Node.js and AWS best practices can facilitate smoother deployments. Here are some recommendations:
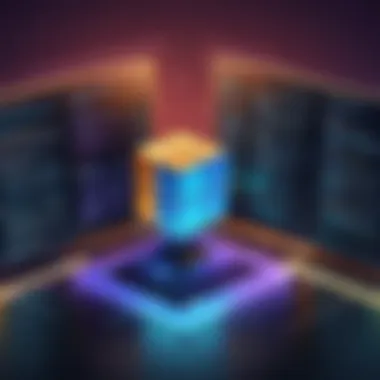
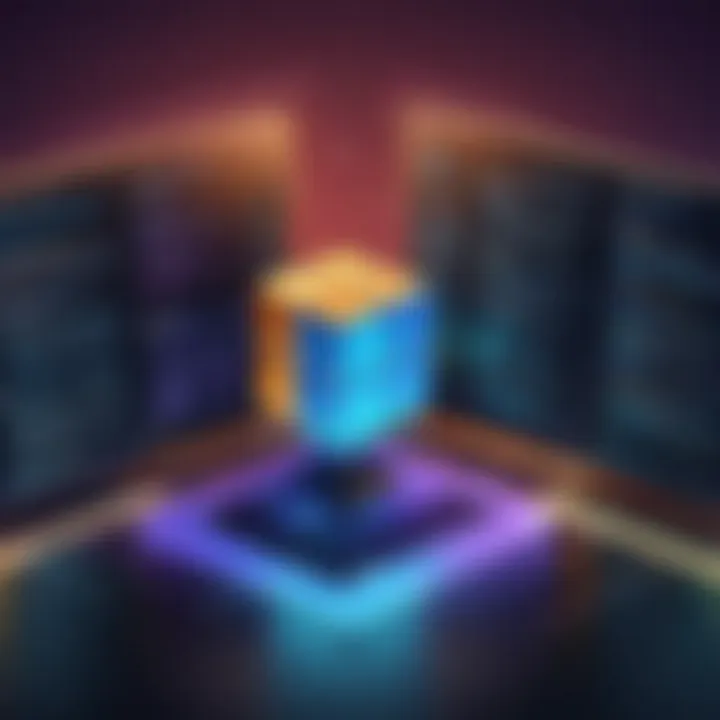
Recommended Books, Courses, and Online Resources
- Node.js Design Patterns by Mario Casciaro is a comprehensive guide to design principles around Node.js.
- Free courses on Coursera and Udemy cover everything from basics to advanced deployment strategies in AWS.
Tools and Software for Practical Usage
- AWS SDK for JavaScript can streamline your development and deployment of Node.js applications on AWS.
- nvm provides a simple way to manage Node.js versions locally.
By mastering these tools and practices, developers will gain the upper hand in creating applications that not only work flawlessly but are also robust and scalable in AWS environments.
Prologue to Node.js
Node.js has rapidly become a cornerstone in the world of web development and server-side programming, largely due to its non-blocking, event-driven architecture. This section seeks to lay a solid foundation for understanding Node.js, focusing on its significance in today’s tech landscape. As cloud computing continues to evolve, incorporating platforms like AWS, the demand for efficient, scalable applications has skyrocketed. Node.js perfectly fits the bill, offering unique advantages that can help developers optimize their workflows and enhance application performance.
In this article, we will explore various aspects of Node.js while shedding light on its relevance particularly within AWS environments. We will discuss how different versions of Node.js can affect application functionality and performance, ensuring that the reader grasps key elements of version control.
Overview of Node.js
Node.js is essentially a JavaScript runtime built on Chrome's V8 engine. What makes it exceptional is its ability to execute JavaScript code on the server side, allowing developers to create dynamic web applications that manage a high number of concurrent requests with ease. Leveraging an event-driven architecture, Node.js operates on a single-threaded model, employing asynchronous programming techniques that are particularly valuable in high-load scenarios.
One of the standout benefits of using Node.js is its extensive npm ecosystem, which hosts countless libraries and utilities designed to accelerate development processes. This popularity means that finding community support and additional resources is fairly easy, which can be an incredible boost for those venturing into the realm of server-side programming.
Key Features of Node.js
Node.js boasts a multitude of features that contribute to its stunning rise in popularity:
- Asynchronous and Event-Driven: Most operations in Node.js are non-blocking, making it highly efficient for handling a variety of tasks that would otherwise slow down traditional server environments.
- Single Language: Developers can use JavaScript on both the frontend and backend, streamlining the development process and eliminating context switching between languages.
- Microservices Architecture: Node.js is well-suited for developing microservices, which makes it easier to create and scale applications in modular fashion.
- Rich Ecosystem: The npm registry is vast. Developers have access to a plethora of modules that can be utilized to extend functionality quickly and effortlessly.
- Robust Community: Node.js has garnered a vibrant community that fosters collaboration and support, which is essential for problem-solving and knowledge sharing.
"In a world driven by technology, Node.js stands out as a transformative force, shaping our online experiences by ensuring seamless interactions and speedy server responses."
Understanding these core principles underpinning Node.js will guide readers as we delve into its role within AWS, how to manage versions effectively, and the best practices surrounding this powerful framework.
The Role of Node.js in AWS
Node.js has become a pivotal player in the realm of cloud computing, especially within Amazon Web Services (AWS). Its significance stems from multiple factors that cater to both developers and organizations seeking efficiency and scalability. As an event-driven, non-blocking I/O model, Node.js shines in handling concurrent requests. This aspect aligns remarkably well with the scalability demands of cloud environments.
Integration with AWS services enhances Node.js' capabilities further. The seamless connection to AWS's storage, computing, and database services allows for a flexible architecture, supporting various applications, from microservices to serverless frameworks.
With AWS, Node.js enables rapid deployment and iteration of applications. This speed is essential in competitive markets where time-to-market can be a make-or-break factor. Furthermore, the broad ecosystem of tools provided by AWS, such as Lambda, facilitates developers in creating solutions that are not only powerful but also easily maintainable.
Integrating Node.js with AWS Services
Merely deploying a Node.js application on AWS is one side of the coin; the real magic happens when these applications interact with AWS services. For instance, by utilizing AWS Lambda, you can run your Node.js code in response to specific events without having to manage servers. This allows developers to focus on writing code rather than dealing with infrastructure management.
When integrating Node.js with AWS S3, applications can handle file storage and retrieval seamlessly. This creates opportunities for applications that require media uploads or complex data handling without a hitch. Similarly, using AWS DynamoDB, a NoSQL database service, can provide rapid data access for Node.js applications, lending itself well to applications needing high throughput and low latency.
In addition, tools like AWS API Gateway work hand-in-hand with Node.js to create robust APIs that are essential for microservices architectures. All of these integrations highlight a fast and effective workflow, allowing for continuous development and deployment.
Common AWS Use Cases for Node.js
Node.js has carved out its niche within AWS by catering to various practical applications. Below are some prominent use cases:
- Real-Time Applications: With Node.js’ non-blocking architecture, it’s well-suited for real-time web applications like chat applications and collaboration tools. The ability to scale horizontally makes it a favorite among developers.
- Microservices Architecture: Node.js supports a microservice architecture, allowing applications to be broken into smaller, manageable services. This promotes better resource management and faster deployment.
- Serverless Computing: Node.js shines in serverless applications using AWS Lambda. Developers can create applications that only use resources as needed, significantly reducing costs.
- Data-intensive Applications: Any application that processes large amounts of data, such as APIs calling multiple external services, can benefit from Node.js’ performance capabilities.
Node.js Version Management
Managing Node.js versions is crucial in maintaining efficient application performance and ensuring compatibility across different systems. In cloud environments like AWS, where deployments can happen at breakneck speed, understanding how to manage these versions becomes even more pertinent. Version management covers everything from keeping your software updated, to ensuring that the right version of Node.js is running in the right environment. Without a solid grasp of this, developers may run into problems, such as bugs caused by incompatible versions or security vulnerabilities due to outdated code.
Understanding Node.js Versions
Node.js’s vibrant ecosystem is built on its diverse versions. Every version can offer a different set of functionalities, some may include cool features while others might work to correct bugs haunting previous releases. When setting out to manage Node.js versions, it's important to first understand the semantic versioning system in place. This system uses a format of MAJOR.MINOR.PATCH.
- MAJOR: Typically, any time you make incompatible API changes, increment the MAJOR version.
- MINOR: When you add functionality in a backward-compatible manner, you can increment the MINOR version.
- PATCH: If you make backward-compatible bug fixes, then you raise the PATCH level.
Recognizing which version you need for your specific application can aid in making informed decisions when deploying to AWS, ensuring the deployment runs smoothly without unexpected hiccups.
LTS vs. Current Releases
When working with Node.js, one must be aware of the distinction between Long Term Support (LTS) versions and current releases. LTS versions are like the evergreen trees of the software world; they promise stability and an extended support period, allowing developers to build and run applications without the constant need to upgrade. On the flip side, current releases provide the latest features, but they may introduce unexpected bugs or instability given their newer nature.
Key points to consider when deciding between the two:
- Stability vs. Features: If your application relies on stability, go for LTS. If it needs cutting-edge functionality, consider current.
- Deployment Frequency: For environments that require rapid deployment, sticking with LTS might prevent unexpected failures in the production environment.
- Support Timeline: Always check how long the support lasts for a particular version. The LTS versions are typically supported for 30 months, providing peace of mind.
Version Compatibility Issues
As software evolves, not all versions are created equal. Version compatibility can be a sticky wicket, especially when APIs change or dependencies shift. Different Node.js versions may not always align seamlessly, leading to a host of potential issues.
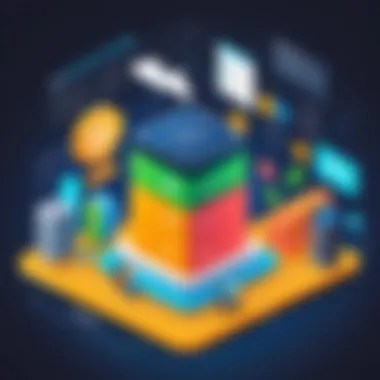
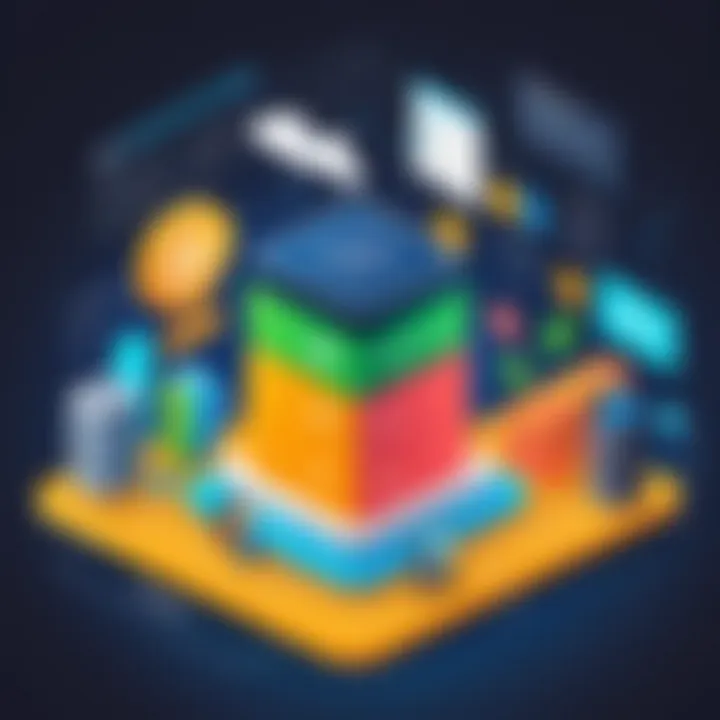
Here are some common compatibility challenges you might face:
- Library Dependencies: Some libraries may only support specific versions of Node.js, which can leave your application stranded if a critical library hasn't updated.
- API Changes: Functions or modules may be deprecated in a newer version. This means existing functionality in your application could break unexpectedly.
- Environment Differences: Even if you're running the same version across different environments, subtle differences in configurations can lead to discrepancies that are hard to debug.
To mitigate these issues, it's beneficial to establish a robust testing framework and to create dependency maps that reflect your application's architecture. Regularly check for updates and always read release notes before upgrading, to stay informed and prepare for any potential breakage.
"Keeping your Node.js versions in check isn't just a task, it's an ongoing dialogue between your code and the ecosystem it's a part of."
Methods for Managing Node.js Versions in AWS
Managing Node.js versions in AWS is not merely a technical requirement; it’s a strategic advantage. As applications develop and expand, having control over the Node.js version in use can prevent significant downtime. In AWS environments, diverse deployment methods exist, each presenting unique opportunities and considerations for proper version management. It's crucial to select the right approach based on the application’s needs, anticipated growth, and the team's expertise.
Using AWS Lambda
AWS Lambda provides a serverless architecture, allowing developers to run code in response to events without provisioning or managing servers. This simplicity is an appealing aspect, especially for applications that require consistent and scalable execution. With Lambda, you can specify the version of Node.js your function should run.
What’s vital to note here is Lambda's support for both current and LTS versions. The flexibility lets developers choose cutting-edge features or stick to a stable release as per their project’s demands.
"Choosing the right Node.js version in AWS Lambda can enhance performance and security, tailored precisely to your application's lifecycle."
However, Lambda has some constraints regarding memory and execution time which can affect larger applications. Developers must optimize their functions and confirm that dependencies are compatible with the selected Node.js version.
Deploying with Elastic Beanstalk
Elastic Beanstalk is another service that simplifies deploying and managing applications. It handles the deployment, from capacity provisioning to load balancing, allowing developers to focus on the application code. When deploying a Node.js application using Elastic Beanstalk, selecting the Node.js version during the environment configuration is key. This management ensures that as you release new features, you're harmonizing with the most suitable Node.js version.
The environment tiers (like web server environments) support multiple Node.js versions. This can create a smoother upgrade path or even allow for A/B testing with different versions running in separate environments. Yet, it's essential to monitor changes to dependencies and assess how they interact with different Node.js versions. Regular updates to the Elastic Beanstalk environment also help in staying aligned with security and performance enhancements.
Utilizing EC2 Instances
EC2 instances offer the highest level of flexibility in deploying Node.js applications on AWS. With EC2, developers can configure the server's environment precisely, including the installation of specific Node.js versions. This hands-on approach empowers teams to run various instances with different Node.js versions simultaneously. For instance, a development team might be testing for compatibility with an upcoming Node.js release while keeping their production environment on a stable version.
In this case, dockerization is often a wise move. By containerizing the Node.js application, developers ensure that the code runs consistently across every node because the environment remains the same regardless of where it’s deployed. The nodes can have different Node.js versions while using the same container image, providing both versatility and stability. Moreover, the AWS Marketplace houses many ready-to-use images that come almost configured for Node.js.
In summary, understanding which AWS service suits your version management needs is imperative. Whether you favor the versatility of EC2, the efficiency of Lambda, or the simplicity of Elastic Beanstalk, each brings its own set of capabilities for managing Node.js versions effectively. By keenly selecting your approach, you can ensure that your applications run smoothly and efficiently, blending functionality with performance.
Best Practices for Node.js Version Management
Managing Node.js versions effectively in AWS environments is not just a nicety; it’s a necessity. With the rapid evolution of Node.js, keeping your projects up-to-date is vital for security, performance, and compatibility. Adhering to best practices in version management can save time, reduce headaches, and ensure a smooth development process.
Regular Version Updates
One of the cornerstones of effective Node.js version management is regular updates. Regularly updating to the latest Long Term Support (LTS) versions helps you gain the latest features and security improvements. The Node.js community puts significant effort into maintaining these versions, which are proven to be stable and secure.
- Security Patches: By staying current, your applications are less likely to be vulnerable to known exploits. Not keeping up can leave gaping holes that malicious actors can exploit.
- Performance Enhancements: With every version, improvements are made that can enhance the performance of your application. Sometimes, those enhancements can lead to significant reductions in runtime or memory usage.
- Feature Integration: New APIs and features introduced in newer versions might provide straightforward solutions to problems your application faces today.
It's advisable to set a reminder or schedule a review every few months to evaluate your Node.js version. A simple command like executed in your terminal can help you check your current version.
Testing for Compatibility
Testing for compatibility can be quite a chore, but it can’t be overlooked. Each new release might introduce subtle changes that could affect your code. Here are some strategies to ensure compatibility:
- Unit Testing: Implement robust unit tests that cover a wide variety of use cases. This helps catch issues that arise from version changes.
- Dependency Management: Check whether your project dependencies are compatible with the new version of Node.js. Sometimes, third-party modules may lag behind the latest release.
- Staging Environment: Before deploying to production, it’s wise to deploy your updated application in a staging environment that mimics production as closely as possible. This helps to identify unexpected breaks caused by the updated Node.js environment.
A notable practice here is using tools like Jest or Mocha for JavaScript testing. They can automate this process, ensuring your application runs smoothly across different Node.js versions.
Automating Version Control
Automation can take a load off your shoulders. The more you can automate your version control process, the less chance there will be for human error. Consider the following approaches:
- Version Managers: Tools like Node Version Manager (NVM) can simplify switching between Node.js versions easily on your local machine.
- Continuous Integration/Continuous Deployment (CI/CD): Implementing a CI/CD pipeline can automate testing every time you commit code. Tools like Jenkins or GitHub Actions can run tests against various Node.js versions, ensuring that your code remains compatible.
- Docker: Using Docker containers allows you to specify which Node.js version your application runs on, providing a consistent environment from development to production.
"Automation isn't just a trend; it's the backbone of modern software development. Adopting it now can streamline your Node.js management strategies and save resources in the long run."
In summary, employing these practices can significantly optimize how you manage Node.js versions within your AWS environments. Getting into the habit of regular updates, thorough testing, and leveraging automation will pave the way for a smoother development experience and superior application performance.
Tools for Managing Node.js Versions
Managing Node.js versions effectively is a crucial aspect of deploying applications in AWS environments. With various tools available, developers can handle version control seamlessly, optimizing their cloud deployments. Let’s delve into three prominent tools that aid in managing Node.js versions, each with specific features and benefits tailored to different use cases.
Node Version Manager (NVM)
Node Version Manager, commonly referred to as NVM, provides developers with an efficient way to install and manage multiple Node.js versions on the same machine. This tool is vital for testing applications across different Node.js versions without the headache of manual installations.
Benefits of using NVM include:
- Flexibility: Developers can switch between Node.js versions easily, enabling them to test their code against different environments.
- User-Specific Installation: Each user can have their local versions of Node.js without system-wide changes, avoiding potential conflicts.
- Simple Commands: NVM operates through straightforward command-line interactions. For example, to install a specific version, one might execute:
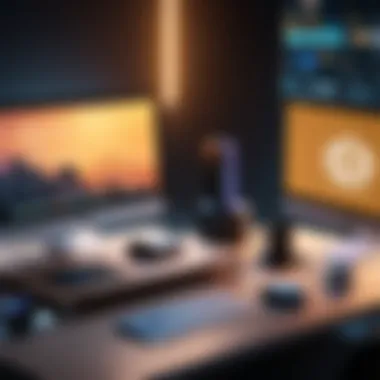
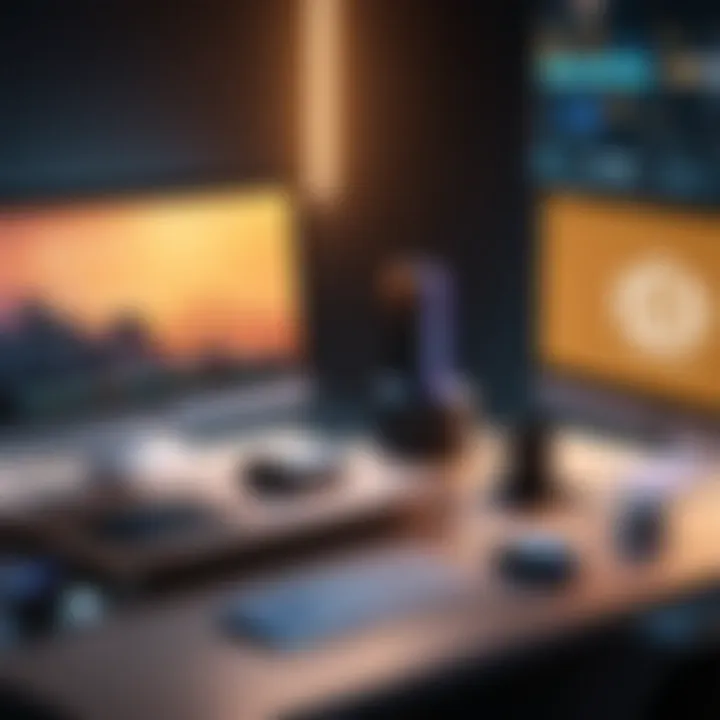
This command is especially handy when working in collaborative environments where different team members may rely on varying features and fixes across versions.
Docker for Node.js Applications
Docker revolutionizes the way applications are packaged and deployed. By using containerization, developers encapsulate Node.js applications along with their dependencies and specific version requirements. This approach ensures consistency across environments, whether it’s development, testing, or production.
Key advantages of Docker include:
- Isolation: Applications run independently within their containers, preventing conflicts that can arise from shared libraries or dependencies.
- Environment Consistency: The same Docker image can be deployed across various platforms such as AWS EC2 and ECS, ensuring no surprises during deployment.
- Scalability: Docker simplifies scaling, allowing applications to handle varying workloads efficiently.
For many programmers, Docker's allure lies in easy version control alongside comprehensive application deployment. Using a Dockerfile, developers can specify the Node.js version like this:
In this scenario, Node.js will be installed in an Alpine image, keeping the size light while ensuring the application runs as intended.
AWS Cloud Development Kit (CDK)
The AWS Cloud Development Kit allows developers to define cloud infrastructure using familiar programming languages, including TypeScript and JavaScript. This toolkit enables the automation of app deployment, allowing for specified Node.js versions through declarative infrastructure as code.
AWS CDK offers several benefits:
- Infrastructure as Code: Using programming languages lets teams manage infrastructure alongside application code, improving cohesion and reducing errors.
- Flexibility in Node.js Version Selection: With CDK, developers can specify the Node.js runtime version directly in their application’s configuration, accommodating changing requirements efficiently.
- Easily Shareable Constructs: Teams can build constructs that encapsulate complex cloud architecture, which can then be reused across various projects.
When defining a Lambda function in AWS CDK, the code version can be set as shown:
This streamlined code impart flexibility, enhancing development efficiency alongside reducing operational risks.
By utilizing appropriate tools for managing Node.js versions, developers ensure smoother deployments and more maintainable applications in AWS environments.
Case Studies of Node.js in AWS
When delving into the relationship between Node.js and Amazon Web Services (AWS), it becomes clear that examining real-world case studies can provide invaluable insights into the practical applications and implications of various Node.js versions. Case studies serve not only to highlight success stories but also provide cautionary tales that illustrate potential challenges and pitfalls. By analyzing how different organizations leverage Node.js in tandem with AWS, we can glean important lessons about scalability, performance, and version management.
Real-world examples showcase how Node.js can excel in cloud environments, addressing needs ranging from rapid application development to efficient API services. As we explore this section, it is crucial to consider specific elements such as the architecture choices made by companies, the Node.js features utilized, and the overall impact on performance and user experience.
Notably, case studies reveal a range of benefits:
- Proven Techniques: Learning from successes can highlight effective strategies for implementing Node.js across AWS services.
- Adaptation to Challenges: Recognizing common pain points can offer a roadmap to avoid similar issues.
- Innovation and Growth: Successful deployments often serve as benchmarks, encouraging innovation and adaptation in technology choices.
Equipped with these insights, companies can make informed decisions regarding their own Node.js applications hosted on AWS. This section aims to draw out those lessons, providing a rich tapestry of knowledge that benefits not just developers but also project managers and decision-makers alike.
Successful Deployments
In the realm of technology, nothing speaks louder than successful deployments. Organizations harnessing the power of Node.js in AWS have showcased how the combination can facilitate robust performance and rapid scaling. For instance, Netflix, a pioneer in streaming services, adopted Node.js to manage their high-traffic delivery systems. One notable feature of this deployment was their ability to handle vast concurrent connections with minimal latency, significantly enhancing user experience during peak times.
Something similar can be seen in LinkedIn’s use of Node.js for mobile backend development. After transitioning from Ruby on Rails to Node.js, LinkedIn reported a twice-as-fast response time and a notable decrease in server resource consumption. The adoption of specific Node.js versions ensured compatibility with AWS tools, streamlining their development pipeline and supporting their scaling requirements.
Beyond just technical performance, these successes underline the importance of aligning Node.js version selections with project goals and cloud services employed. For teams exploring deployment strategies, focusing on:
- Performance Metrics: Continuous monitoring can flag bottlenecks early on.
- User Experience: Fast response and minimal downtime should be paramount.
- Scalable Architecture: Ensure that applications can grow without major overhauls.
Common Pitfalls to Avoid
While the journey of deploying Node.js applications in the AWS ecosystem can lead to outstanding success, it is littered with challenges that can impede progress. It’s important to arm oneself with knowledge about common pitfalls that organizations have encountered.
One frequently noted issue is improper version management. Companies sometimes leapfrog Node.js versions which can cause compatibility headaches, especially with libraries and frameworks that depend on specific features or bug fixes. This can become a major source of frustration when working in environments that require consistent uptime.
Another significant pitfall involves neglecting extensive testing before deployment. In a rush to seize market opportunities, some teams skip key testing phases, leading to unexpected failures post-launch. It’s essential to:
- Conduct Thorough Compatibility Checks: Ensure that all dependencies work well with selected Node.js versions.
- Implement Automatic Testing: Unit tests and integration tests can catch issues early in the development process.
- Collaborate on Documentation: Keeping detailed records of various deployments assists future teams in understanding configuration changes and decisions made.
Closure
In wrapping up our exploration of Node.js versions within Amazon Web Services (AWS), it's crucial to underscore several key elements. Managing Node.js versions is not merely a technicality; it’s a vital practice that can significantly influence application performance and reliability. A systematic approach to version management helps avoid conflicts and ensures that applications maintain operational integrity, especially when dealing with frequent updates and potential deprecations.
The Future of Node.js in AWS
The trajectory of Node.js in the AWS ecosystem appears bright and full of promise. As cloud computing continues to evolve, so too does the role of Node.js. The framework’s non-blocking architecture and its ability to handle numerous simultaneous connections bode well for scalable cloud applications.
With the rise of microservices architectures, Node.js lends itself well to modular development, which can streamline updates and improve maintainability. Moreover, the continued integration of newer features and enhancements in Node.js, such as improved performance through the V8 engine updates, positions it as a powerhouse for cloud-native applications. It’s plausible that we might see increased support in AWS services tailored for Node.js, simplifying deployment and scaling operations. To capitalize on these developments, developers should stay informed about the latest updates and actively engage with the community through forums like Reddit.
Final Thoughts on Version Management
Ultimately, effective version management is about foreseeing potential issues and being proactive in addressing them. In the world of programming, where change is the only constant, having the right Node.js version can protect your project from unforeseen bugs and security vulnerabilities. The benefits of regular updates, comprehensive testing, and the use of helpful tools like Node Version Manager (NVM) cannot be overstated.
To navigate the complexities of AWS and Node.js seamlessly, developers must prioritize compatibility and integrate version management into their deployment strategies. Failing to do so may result in inefficiencies that could ripple through the entire application lifecycle.
By employing best practices and leveraging the right tools, the management of Node.js versions does not have to be an insurmountable challenge but can instead become a smooth aspect of your cloud development process.
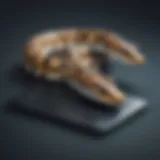
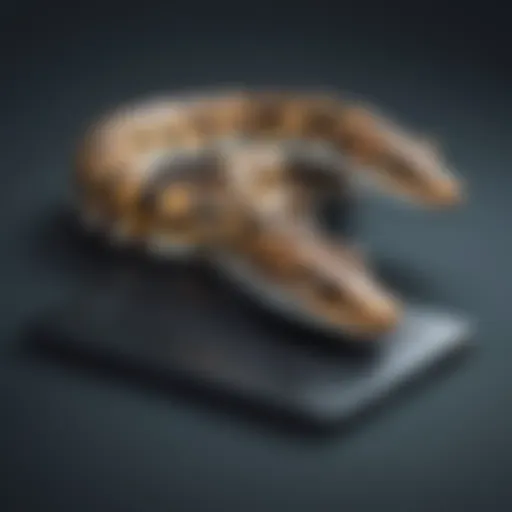