Mastering C++: A Comprehensive Application Development Guide
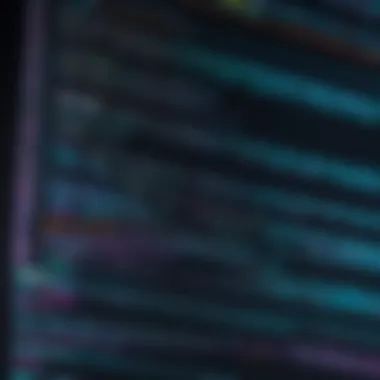
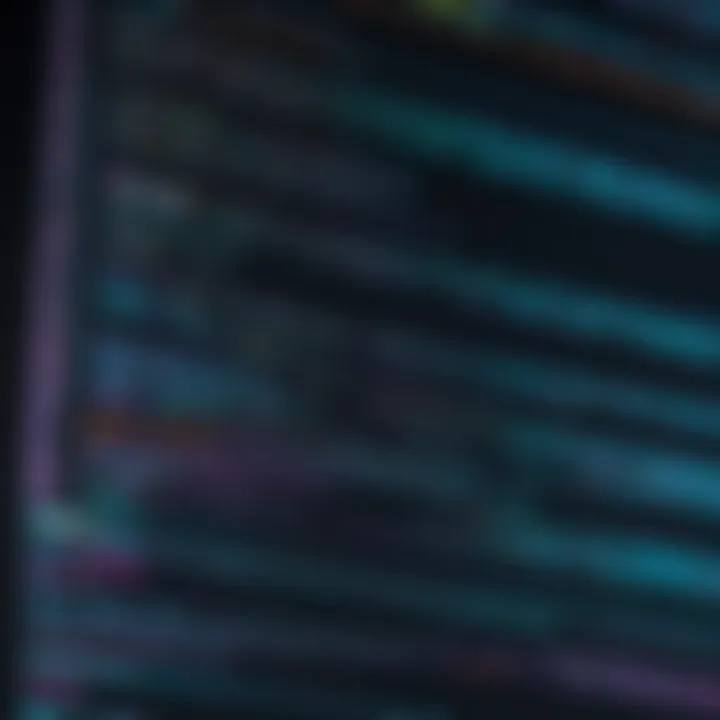
Overview of Topic
Intro to the Main Concept Covered
C++ is a robust programming language that is pivotal in software development. It offers a balanced blend of high-level and low-level features, making it suitable for varied applications, from system software to game development. This guide explores the nuances of developing applications with C++, focusing on essential tools and practices required for successful programming.
Scope and Significance in the Tech Industry
The C++ language is integral in several technological domains. It powers operating systems, game engines, and applications demanding performance efficiency. Developers often choose C++ for its control over system resources and its ability to utilize complex algorithms effectively.
Brief History and Evolution
C++ was developed by Bjarne Stroustrup at Bell Labs in the early 1980s as an enhancement of the C language. Over the years, it has undergone numerous revisions, each adding new features such as object-oriented programming, templates, and the Standard Template Library (STL). Understanding its evolution gives insight into its current capabilities and applications.
Fundamentals Explained
Core Principles and Theories Related to the Topic
C++ is built around several core principles. These include encapsulation, inheritance, and polymorphism. These concepts facilitate cleaner code architecture, which is crucial as projects scale. Understanding these fundamentals enables programmers to create more maintainable and reusable code.
Key Terminology and Definitions
Some key terms related to C++ development include:
- Object-Oriented Programming (OOP): A programming paradigm centered around objects, which combine data and functionality.
- Class: A blueprint for objects, encapsulating data and methods.
- Template: A mechanism that allows functions or classes to operate with generic types.
Basic Concepts and Foundational Knowledge
Before diving into C++ application development, one should grasp variables, data types, operators, and control structures. These elements form the building blocks for more complex functionality, serving as the groundwork for application logic.
Practical Applications and Examples
Real-World Case Studies and Applications
C++ is widely recognized for applications in high-performance games and complex simulations. For example, game engines like Unreal Engine utilize C++ for their flexibility and speed, demonstrating the language's capabilities in demanding environments.
Demonstrations and Hands-On Projects
Engaging in small projects can deepen understanding. A simple project like a console-based calculator can help illustrate how to implement functions, loops, and data types effectively in C++. This hands-on approach solidifies foundational concepts.
Code Snippets and Implementation Guidelines
Here's a basic code snippet to illustrate a simple class in C++:
This code defines a class with a method that outputs text to the console.
Advanced Topics and Latest Trends
Cutting-Edge Developments in the Field
The field of C++ development continues to evolve. Features from recent ISO revisions introduce concepts like coroutines and modules, further enhancing the language's performance and usability.
Advanced Techniques and Methodologies
Advanced developers often employ design patterns such as Singleton and Observer to tackle complex problems more elegantly. Familiarity with these patterns elevates a programmer's toolkit.
Future Prospects and Upcoming Trends
As applications grow in complexity, the demand for languages that offer both performance and ease of use remains. C++ is adapting by integrating modern features, ensuring it remains relevant in the software development landscape.
Tips and Resources for Further Learning
Recommended Books, Courses, and Online Resources
Investing time in quality resources can greatly enhance understanding. Books like "The C++ Programming Language" by Bjarne Stroustrup provide detailed insights, while platforms like Coursera offer structured courses.
Tools and Software for Practical Usage
Some essential tools for C++ development include:
- Visual Studio: A comprehensive IDE for Windows.
- CLion: A powerful cross-platform C++ IDE.
Learning C++ requires patience and practice. Start simple and build complexity gradually.
Engaging in communities such as Reddit and specialized forums can provide support and different perspectives as you progress in your journey of mastering C++.
Prologue to ++ for Application Development
C++ is a powerful language for application development. Its versatility allows developers to create software that runs on various platforms. This section introduces C++ and its significance in the programming landscape. Understanding C++ is crucial as it serves as a foundation for many modern languages and technologies. Learning C++ can enhance one's coding skills and open opportunities in software development, game development, and systems programming.
What is ++?
C++ is a high-level programming language that combines features of both low-level and high-level languages. It was developed by Bjarne Stroustrup at Bell Labs in the early 1980s as an enhancement of the C programming language. C++ supports procedural programming, object-oriented programming, and generic programming, making it flexible and adaptable for various applications. The language is known for its performance and efficiency, making it popular among developers who require control over system resources.
Historical Context of ++
The development of C++ began in 1979. Stroustrup aimed to add object-oriented features to the C language. In 1985, the first edition of "The C++ Programming Language" was published, introducing the language to a wider audience. Over the years, C++ has evolved through several standards, including C++98, C++03, C++11, C++14, C++17, and the most recent C++20, each adding new features and improvements. This history marks C++ as a language that adapts and remains relevant in the technology landscape.
++ Applications in Modern Tech
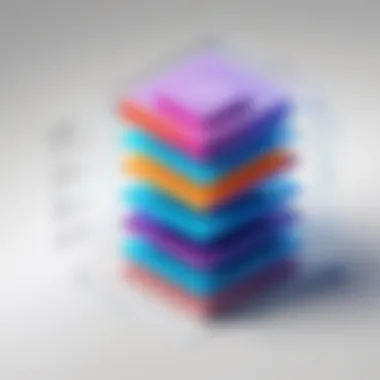
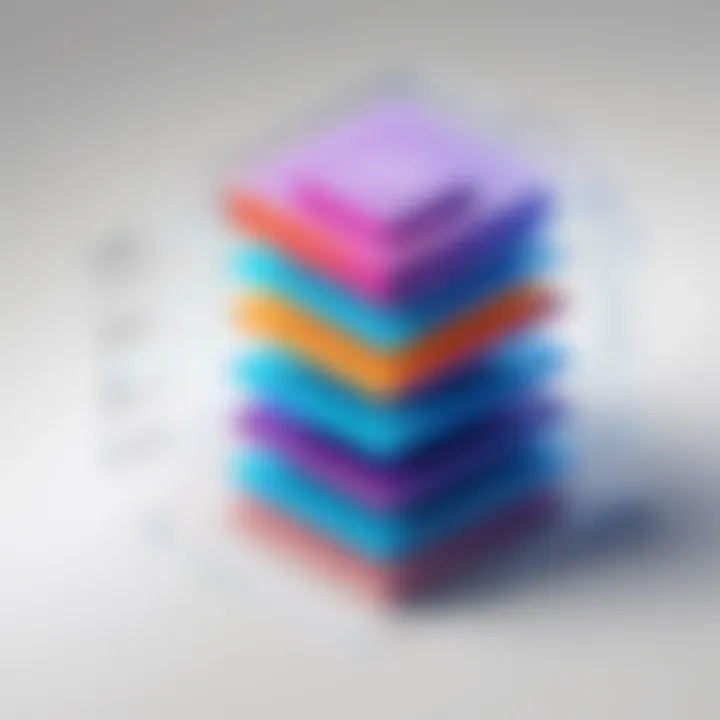
C++ has found its application in various domains including:
- System Software: Operating systems use C++ for its performance. Examples include parts of Windows and Unix.
- Game Development: Many game engines, like Unreal Engine, utilize C++. This is due to the need for high-performance graphics and real-time processing.
- Embedded Systems: C++ is widely used in programming embedded systems, where resource management is critical.
- Finance: High-frequency trading systems often use C++ because of its speed and efficiency.
- Graphical User Interfaces: Libraries like Qt allow developers to create rich GUI applications with C++.
Understanding C++ and its applications can significantly aid a developer's career. Its importance in modern technology continues to grow, making it a valuable skill for both beginners and experienced programmers.
Setting Up Your Development Environment
Setting up your development environment is crucial for efficient C++ application development. This step impacts how smoothly you can write, test, and debug your code. An ideal setup provides the right tools and resources that enhance productivity, leading to improved code quality. It is not just about having a C++ compiler but also configuring integrated development environments (IDEs) to fit your coding style and needs. Such considerations help avoid unnecessary frustration as you delve into programming tasks.
Installing a ++ Compiler
The first step in setting up your environment involves installing a C++ compiler. A compiler transforms your C++ code into executable programs, making it a fundamental element for application development. Popular options include GCC, Clang, and Microsoft's Visual C++. Each of these compilers has its own strengths. For instance, GCC is known for its extensive support across various platforms, while Visual C++ is particularly beneficial for Windows development.
Consider your operating system and the specific features you might need. A compatible compiler ensures you can access all standard and advanced functionalities of C++. Installation processes can vary. It’s essential to follow the official guides for each compiler to ensure a successful setup.
Choosing an Integrated Development Environment (IDE)
An IDE is more than just a code editor; it is a complete software suite that facilitates the development process. Choosing the right IDE can streamline your workflow, enhancing overall efficiency. An effective IDE includes features like intelligent code completion, integrated debugging, and project management.
Popular IDEs for ++
Some popular IDEs for C++ include Microsoft Visual Studio, Code::Blocks, and JetBrains CLion. Visual Studio is a robust choice that integrates seamlessly with Windows platforms. It offers a range of tools for debugging and testing, making it a favorite among professionals. Code::Blocks, on the other hand, is known for its simplicity and is widely appreciated by beginners. JetBrains CLion provides a modern interface along with advanced features like refactoring tools and version control.
Each of these IDEs caters to different user preferences and requirements. For instance, Visual Studio might be overkill for someone working on small projects, while Code::Blocks offers a more straightforward approach.
Configuring Your IDE
Once you’ve chosen an IDE, the next step is configuration. This process involves setting up project settings, adjusting themes, and personalizing shortcuts according to your demand. Effective configuration can greatly improve how you interact with the IDE, making you more productive. Key configurations include specifying compiler paths, enabling code linting, and even setting up version control systems.
A well-configured IDE will often facilitate debugging, allowing you to identify issues more quickly. For example, you can set breakpoints in the code, which pause execution, offering insights into variable states and flow of control.
Tip: Spend time exploring your IDE’s settings. Customizing your environment can significantly streamline your coding process and enhance your comfort.
In summary, setting up your development environment effectively is key to successful C++ application development. By choosing the right compiler and IDE, you prepare the ground for a more productive coding experience.
Key Concepts in ++ Programming
Understanding key concepts in C++ programming is vital for anyone seeking to develop applications using this powerful language. C++ offers a rich set of features that enable developers to write efficient and maintainable code. The importance of these concepts lies not only in their theoretical knowledge but also in their practical application.
One core element is the distinction between data types and variables. They allow developers to define what kind of data is handled and how much memory is allocated. Mastering control structures, including conditional statements and loops, is crucial as they dictate the flow of execution in programs. Functions and scope further enable modular programming and code reuse, enhancing the clarity and maintainability of the code.
Data Types and Variables
Data types in C++ establish the type of data a variable can hold. Variables, on the other hand, act as containers that store this data. Common data types include integers, floats, doubles, chars, and strings. Each type comes with its own range and storage requirements. Understanding these data types is not just foundational; it leads to efficient memory management and optimal performance in applications.
Developers are expected to choose appropriate data types based on the requirements of the application. For instance, if precise decimal representation is needed, using or is preferable as compared to . Variables allow programmers to store and manipulate data dynamically. Defining variables correctly ensures that the program adheres to the specified data type constraints, which reduces errors during execution.
Control Structures
Control structures are essential in determining the flow of execution in a C++ program. They include conditional statements and loops, which are fundamental for decision-making and repetition.
Conditional Statements
Conditional statements, such as , , and , provide a mechanism to execute different blocks of code based on certain conditions. This aspect is crucial as it allows the program to make decisions at runtime, improving its adaptability to various inputs. The key characteristic of conditional statements is their ability to branch execution paths in a straightforward manner.
This choice is popular because they enable developers to write clean and readable code. The unique feature of conditional statements lies in their flexibility. Developers can use complex conditions to handle multiple scenarios efficiently. However, nested conditional statements can lead to complexity and should be used judiciously to maintain code clarity.
Loops
Loops facilitate the repeated execution of a block of code until a specific condition is met. C++ supports several types of loops, including , , and . The main characteristic of loops is their efficiency in running a sequence of statements multiple times without needing to rewrite code. This repetition is beneficial when processing data collections or performing operations that require multiple iterations.
The unique feature of loops is their control over the number of iterations. For instance, a loop is well-suited for a predetermined number of iterations, while a loop is ideal for processes that depend on a dynamic condition. However, poorly implemented loops can lead to infinite iterations, causing programs to run indefinitely.
Functions and Scope
Functions in C++ break programs into self-contained modules, allowing for code reuse. When a function is defined, it can be invoked from multiple locations within the program, reducing redundancy. Understanding scope is crucial in this context. It refers to the region within the code where a variable or function is accessible. C++ distinguishes between global and local scope, impacting how data flows through the program.
Mastering functions and their scope enables structured programming. It enhances separation of concerns, where different functionalities can be isolated and modified independently. This aspect is particularly useful for debugging and modifying applications over time.
Object-Oriented Programming in ++
Object-Oriented Programming, commonly known as OOP, is a fundamental programming paradigm integral to the design and implementation of C++ applications. OOP emphasizes the use of "objects" as the primary means of structuring software. In C++, OOP provides a robust framework for managing complex systems by encapsulating code in reusable units and fostering better organization. This approach enhances modularity and clarity, allowing developers to focus on the specific characteristics and behaviors of objects, rather than merely the procedures that manipulate them.
Classes and Objects
In C++, classes serve as blueprints for creating objects. A class defines a type of object that includes attributes and methods. Attributes represent data, while methods define behavior. Creating an object from a class involves instantiating it, which allocates memory for its data members. The following is an example of a simple class definition:
In this example, the class has two attributes: and , and one method , which outputs the car's information. Objects are created by instantiating the class, for example:
This demonstrates how objects utilize the class structure to maintain data and perform operations.
Inheritance and Polymorphism
Inheritance allows a class to derive properties and behaviors from another class, creating a hierarchical structure. This feature promotes code reuse and simplifies maintenance. For instance, suppose we have a base class, , from which and classes can inherit:
In this case, both and have access to the method from the class. This exemplifies code reuse and the DRY (Don't Repeat Yourself) principle.
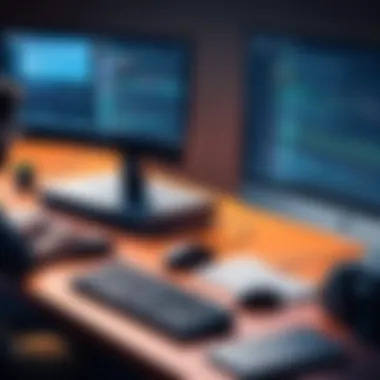
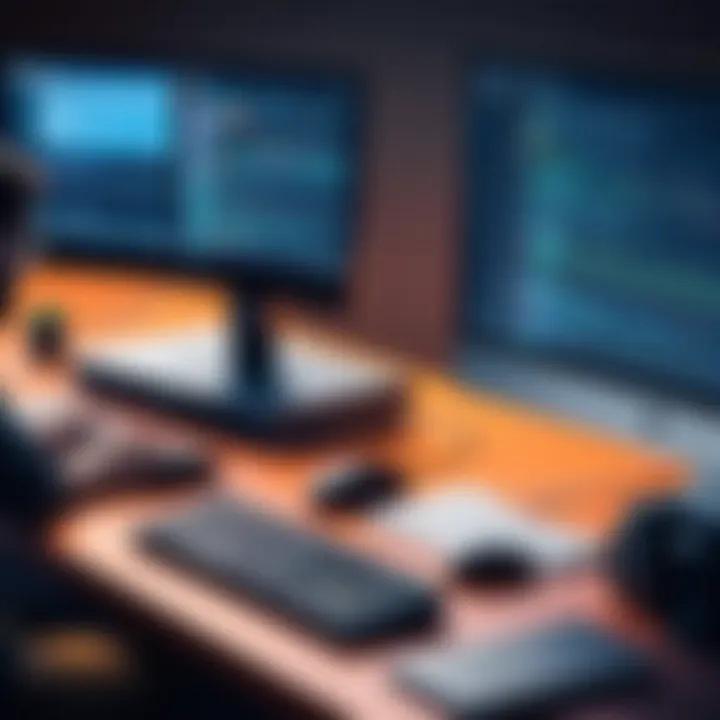
Polymorphism enables treating objects of different classes through a common interface. This often involves method overriding, where a derived class implements a method differently than its base class. This flexibility facilitates a cleaner design in applications where different behaviors are necessary without altering the broader architecture.
Encapsulation and Abstraction
Encapsulation is the concept of wrapping data and methods within a single unit, restricting direct access to some of the object's components. This practice ensures that the internal representation remains hidden from the outside. For instance, private data members can only be accessed through public methods:
From this code snippet, the of cannot be directly manipulated outside the class. This safeguard highlights the importance of controlled access to an object's state.
Abstraction involves simplifying complex systems by modeling classes based on essential characteristics, avoiding unnecessary details. It allows for focusing on relevant aspects while hiding implementation specifics. This principle ultimately fosters more maintainable and understandable code.
"Object-Oriented Programming excels in managing complexity through abstraction, encapsulation, inheritance, and polymorphism."
In summary, Object-Oriented Programming in C++ is an essential concept that structures code effectively, promoting reuse and clarity. Understanding these principles equips developers with the tools necessary to create scalable and maintainable applications.
Essential Libraries and Frameworks
In the realm of C++ application development, libraries and frameworks play a pivotal role. They act as extensions of the core programming language, providing pre-written code to simplify complex tasks, enhance functionality, and improve overall efficiency. By utilizing these tools, developers can significantly speed up their workflow and focus on writing unique components of their applications instead of repetitive code.
Choosing the right libraries and frameworks is essential. It can influence everything from application performance to the ease of maintenance. With a vast array available, developers must understand the benefits and limitations of each option to make informed decisions that align with their project needs.
Standard Template Library (STL)
The Standard Template Library (STL) is one of the cornerstones of C++ programming. It provides a collection of data structures and algorithms designed to improve efficiency and performance. The primary components of STL include containers, iterators, algorithms, and function objects.
Benefits of Using STL:
- Efficiency: STL provides ready-to-use templates, reducing the time needed for coding and debugging.
- Flexibility: The library supports various data structures, such as vectors, lists, and maps, allowing developers to choose the best fit for their needs.
- Performance: STL is optimized for performance, which can lead to faster execution of algorithms compared to manually implemented ones.
Utilizing STL not only allows developers to follow best practices in C++ but also helps in creating portable and maintainable code structures.
Boost Libraries
Boost libraries are widely recognized for their extensive collection of portable libraries. They enhance C++ functionality and offer solutions for issues not covered by the STL. With over 80 libraries available, Boost covers various aspects, including linear algebra, multithreading, and asynchronous programming.
Key Aspects of Boost:
- Modularity: Developers can choose specific libraries from Boost without needing to integrate the entire collection into their projects.
- Community Support: Boost has a strong community that continuously contributes to its development, ensuring libraries are up-to-date and resilient to bugs.
- Ease of Integration: Using Boost alongside existing C++ code is usually straightforward, allowing for gradual adoption in projects.
Boost libraries frequently serve as a base for new features in upcoming C++ standards, demonstrating their importance in the evolution of the language.
Qt Framework for GUI Applications
When it comes to developing graphical user interface (GUI) applications, the Qt framework stands out as a leading choice among C++ developers. It provides tools to create cross-platform applications that have a native feel on different operating systems.
Advantages of Using Qt:
- Cross-Platform Development: Applications developed with Qt can run on multiple platforms with minimal changes, saving development time and costs.
- Rich UI Elements: Qt comes with a comprehensive set of widgets and tools for creating responsive and aesthetically pleasing UIs.
- Signal and Slot Mechanism: This unique feature in Qt allows easy communication between objects, making it simpler to manage user interactions and events within the application.
For developers looking to create robust, user-friendly applications, Qt is an invaluable resource that combines the power of C++ with a versatile GUI toolkit.
Best Practices in ++ Development
The importance of adhering to best practices in C++ development cannot be overstated. These practices not only enhance the quality of the code but also contribute to the maintainability, readability, and efficiency of applications. When developers follow best practices, they help ensure that the codebase is more understandable for others and even for themselves in the future.
Good coding habits lead to fewer bugs, easier troubleshooting, and quicker onboarding for new team members. Moreover, an application that is built with careful attention to best practices has a better chance of scaling effectively as project requirements evolve. Below, we detail some key areas that contribute to successful C++ development processes.
Coding Standards and Style Guidelines
Coding standards in C++ represent a set of rules for writing code that increases consistency and readability. These standards can cover naming conventions, the structure of files, and the formatting of code itself. Following established style guidelines makes it easier for multiple developers to collaborate on a project. Some widely recognized guidelines include:
- Google C++ Style Guide: Focuses on readability and consistency.
- LLVM Coding Standards: Provides rules specifically useful for C++ projects.
- C++ Core Guidelines: Offers principles and practices for modern C++ programming.
When teams agree on a specific style, it reduces cognitive load. Developers don't need to spend time deciphering less familiar code styles. This consistency ultimately enhances the entire development workflow.
Error Handling and Debugging Techniques
Effective error handling is crucial for creating robust C++ applications. Using try-catch blocks and exception handling can greatly improve how programs respond to unexpected events. It is essential to document error handling strategies clearly. The following techniques are essential:
- Use of Exceptions: C++ allows developers to throw exceptions in scenarios that would normally cause a program to terminate unexpectedly.
- Log Critical Issues: Implement logging mechanisms to capture errors and warnings, helping to pinpoint issues during debugging.
- Validating Inputs: Before manipulation, inputs should be verified. This practice can prevent runtime errors and improve reliability.
Incorporating these practices can lead to quicker diagnosis of issues and better overall software stability. Good debugging techniques include using tools such as gdb or Valgrind to analyze program behavior.
Performance Optimization Strategies
Performance is a critical factor in C++ application development. Optimized code runs faster and is capable of handling larger processes. Key strategies include:
- Efficient Use of Memory: Using smart pointers (like std::unique_ptr) can help eliminate memory leaks and enhance performance.
- Algorithm Optimization: Selecting the right algorithm for the task at hand can greatly improve efficiency. For example, using std::sort over a simple bubble sort.
- Profiling Tools: Utilizing tools like gprof or perf to analyze performance bottlenecks helps developers focus their optimization efforts where they are most needed.
By addressing these strategies, developers can achieve significant performance gains without compromising code clarity or maintainability.
Ultimately, best practices in C++ development empower developers to produce high-quality, maintainable, and efficient software applications. Investing time in these practices pays dividends in the long run.
Testing and Quality Assurance for ++ Applications
Testing and quality assurance are foundational elements in the development of reliable C++ applications. This section explores the significance of establishing rigorous testing mechanisms and quality assurance practices to ensure that applications function smoothly and meet the desired specifications. The complexity of modern software necessitates well-structured testing systems to identify and fix defects early in the development cycle.
The benefits of robust testing include higher code quality, increased user satisfaction, and reduced long-term costs associated with maintaining software. By prioritizing testing and quality assurance, developers can detect issues before they reach the production environment, ultimately leading to fewer real-world failures. It is essential to understand that testing is not merely a final step, but an integral part of the entire development process.
Unit Testing Frameworks
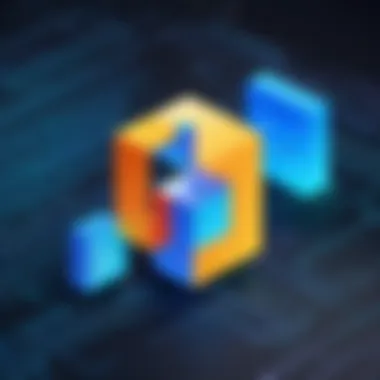
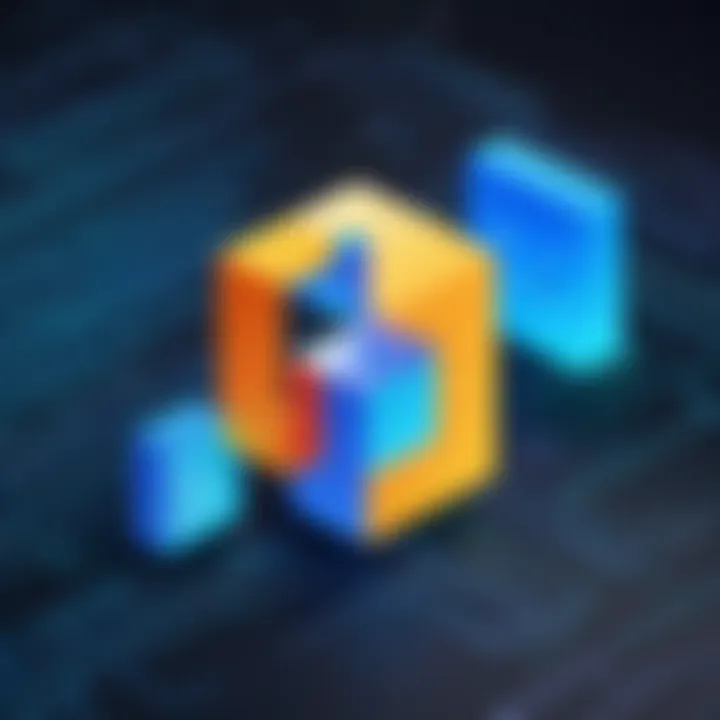
Unit testing focuses on verifying the smallest parts of an application, typically individual functions or methods. Implementing unit tests helps developers catch bugs early and facilitates code refactoring without fear of breaking functionality. Popular C++ unit testing frameworks include Google Test and Catc. These frameworks provide features such as test runners, assertions, and fixtures, making it easier to write and manage unit tests.
Here are some key reasons to adopt unit testing in C++ development:
- Early Bug Detection: Finding and fixing issues during development is significantly cheaper than after deployment.
- Simplified Debugging: When a test fails, it quickly indicates where the problem lies.
- Code Maintainability: Well-tested code is easier to modify as changes can be validated through existing unit tests.
Integration Testing Approaches
Integration testing is the process of combining individual units or components of an application and testing them as a group. This crucial phase helps identify issues that arise when different components interact. In C++, it can be adapted to verify the connections between libraries, APIs, and modules.
Common approaches to integration testing in C++ include:
- Top-Down Integration: Testing begins at the top level of the application and integrates lower-level modules progressively.
- Bottom-Up Integration: Testing starts with lower-level components and gradually integrates upper-level components.
- Big Bang Integration: All or most of the components are integrated simultaneously and then tested at once, although this can complicate debugging efforts.
Integrating components is vital. If components perform well in isolation but fail when combined, the application may not function as intended.
Continuous Integration and Deployment
Continuous integration (CI) and continuous deployment (CD) are practices that promote frequent code changes and automated testing. CI ensures that every code change made by developers is immediately tested, while CD automates the deployment of applications after successful test passes.
Utilizing CI/CD in C++ development brings multiple advantages:
- Automatic Testing: Every change triggers automated tests, ensuring that the application remains functional.
- Faster Release Cycles: Frequent integrations lead to quicker delivery of features and fixes to users.
- Enhanced Collaboration: CI/CD practices streamline teamwork, allowing developers to work concurrently with less risk of integration conflicts.
To implement successful CI/CD pipelines, developers can use tools such as Jenkins or Travis CI. These tools help manage builds, run automated tests, and deploy code efficiently.
"Quality is never an accident; it is always the result of intelligent effort."
– John Ruskin
Deployment of ++ Applications
Deployment is a vital stage in the journey of any software application. It refers to the process of making an application operational for users. In the context of C++ applications, deployment encompasses several specific activities, from building executables to distributing them effectively. Understanding the intricacies of deployment ensures that your application not only functions as intended but also reaches the target audience efficiently.
The significance of deployment lies in its role as the final bridge between development and user interaction. Even the most meticulously crafted C++ application can falter if its deployment is mishandled. Thus, grasping the nuances of how to deploy C++ applications can save substantial time and resource in the long run.
"The best code on earth still needs proper deployment."
Building Executables and Libraries
Creating executables and libraries in C++ is the first step toward deployment. This process involves compiling your source code into a format that the machine can execute. In most cases, this results in an executable file, which users interact with directly. Here are some key points to note during this process:
- Compiler Flags: Make sure to select the proper compiler flags when compiling your C++ project. This impacts optimization, debugging symbols, and warnings.
- Static vs Dynamic Libraries: You can build static libraries, which are included in the executable, or dynamic libraries (also referred to as shared libraries), which are separate files that the executable links to at runtime. Choose based on your application’s requirements and potential for reuse.
- Cross-Compilation: If you plan to run your C++ application on different platforms, consider cross-compiling. This allows you to build executables for multiple systems without needing to switch environments constantly.
Distribution Strategies
Once the executable and libraries are ready, the next step is distribution. Selecting the right distribution strategy is crucial to reach users effectively. Here are several approaches:
- Direct Downloads: Offering direct downloads from your website is still a prevalent method. Ensure that users have clear instructions on how to install the software.
- Package Managers: For applications meant for Linux users, consider distributing through package managers like APT or RPM. This helps users install and update your software more easily.
- App Stores: If relevant, distribute your application through platforms like Microsoft Store or Apple App Store. This can broaden your reach but may involve stringent guidelines.
- Version Control Systems: For developers or technical users, you might use GitHub or GitLab to provide access to your codebase. Make sure to include detailed instructions in a readme file for ease of use.
Effective deployment strategies consider not only how to build and distribute but also how to support users post-deployment. Having a solid plan for updates, patches, and user feedback can enhance the overall success of your C++ application in the marketplace.
Future Trends in ++ Development
In the ever-evolving landscape of technology, C++ continues to hold its ground as a critical language for application development. The importance of discussing future trends in C++ development cannot be overstated. As developers seek to create more efficient, robust, and scalable applications, understanding these trends becomes essential. The exploration of emerging features in recent C++ standards and evolving use cases reveals how C++ is adapting to meet modern demands.
Emerging Features in Recent ++ Standards
Recent C++ standards, such as C++11, C++14, C++17, and the upcoming C++20, have introduced significant features that increase the language's capabilities. Here are some key features that have emerged:
- Automatic Type Deduction: with the introduction of , developers can benefit from less verbose code while maintaining strong type safety.
- Lambda Expressions: these allow for inline function definitions, essential for functional programming styles, making it easier to write clean and efficient code.
- Smart Pointers: features such as and help in managing memory automatically, reducing the risk of memory leaks.
- Concurrency Support: Improvements like and the introduction of thread libraries enable developers to write parallel code more effectively.
As C++ evolves, such features significantly enhance productivity and code safety, solidifying its relevance in high-performance software development. With every new standard, C++ becomes more user-friendly and aligned with contemporary programming practices.
Evolving Use Cases and Market Demand
The demand for C++ skills in the job market remains high, reflecting its versatile application range. Notable use cases that illustrate this can include:
- Game Development: Engines like Unreal Engine utilize C++ for high-performance graphics rendering and optimal control over hardware. Its efficiency is crucial here.
- Systems Programming: Operating systems, embedded systems, and real-time systems often rely on C++ due to its ability to directly manipulate hardware and memory.
- Finance and Embedded Systems: C++ is widely adopted in industries where performance and real-time processing are central, such as financial trading platforms and automotive software.
The ongoing evolution in these areas calls for developers skilled in modern C++ features to keep pace with industry requirements. Firms are increasingly seeking professionals who can leverage the latest language enhancements to craft sophisticated applications.
"The future of C++ greatly hinges on how well the community embraces the novel standards while addressing performance, safety, and usability."
As we look forward, keeping abreast of these trends within C++ development will not only elevate one's skills but also enhance employability in a competitive tech landscape. Understanding these emerging features and use cases is key to harnessing the full potential of C++ in modern application development.
End
The conclusion serves as the final synthesis of the entire article, encapsulating the journey through C++ application development. It is important because it allows readers to reflect on everything they have learned. This section highlights the significance of the previous topics and reaffirms key concepts presented throughout the guide, ensuring that critical information is crystallized in the minds of the readers.
A well-structured conclusion helps to reinforce the main ideas and encourages further exploration of the C++ language. It also emphasizes the evolving nature of technology and programming, making it clear that mastering C++ provides a solid foundation not just for immediate project goals but also for future endeavors in software development.
Considerations related to the conclusion should include how C++ fits uniquely in the programming landscape. Emphasizing its versatility, performance, and wide industry support can foster a greater appreciation among novices and seasoned programmers alike. Furthermore, the final thoughts should encourage readers to apply what they have learned, seek out projects that challenge their skills, and remain engaged with the ongoing developments in C++.
"Programming is not about what you know; it’s about what you can create."
Recap of Key Points
In this article, we have traversed several critical aspects of C++ application development. Here is a concise recap:
- C++ Overview: Understand the foundational elements of C++ and its historical evolution.
- Environment Setup: Learn how to set up your development environment, including compilers and IDEs.
- Key Concepts: Explore the fundamental programming concepts, such as data types, control structures, and functions.
- Object-Oriented Programming: Investigate principles like inheritance, polymorphism, encapsulation, and abstraction.
- Essential Libraries: Familiarize with libraries like the Standard Template Library and frameworks like Qt for GUI applications.
- Best Practices: Acknowledge practices that enhance code quality, such as error handling, debugging, and performance optimization.
- Testing and Quality Assurance: Understand various testing methodologies and the significance of quality assurance in development.
- Deployment: Grasp the essentials of building executables and strategies for effective application distribution.
- Future Trends: Stay informed about emerging trends, features in C++ standards, and changing market demands.
Further Resources for ++ Learning
For readers looking to delve further into C++ or programming in general, several valuable resources are available:
- Wikipedia - C++ offers a comprehensive history and overview of the language.
- Britannica - Computer Programming provides insights into programming fundamentals and various languages.
- Reddit - Learn Programming features discussions and resources shared by learners and experienced programmers.
- Online courses on platforms such as edX or Coursera can offer structured learning environments.
Additionally, joining communities, forums, or even local meetups can enhance the learning experience and provide support during coding endeavors.