Mastering C# Basics: Your Complete Guide to Programming
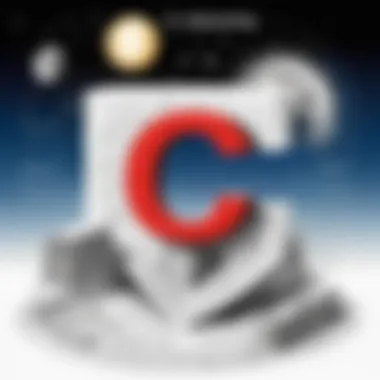
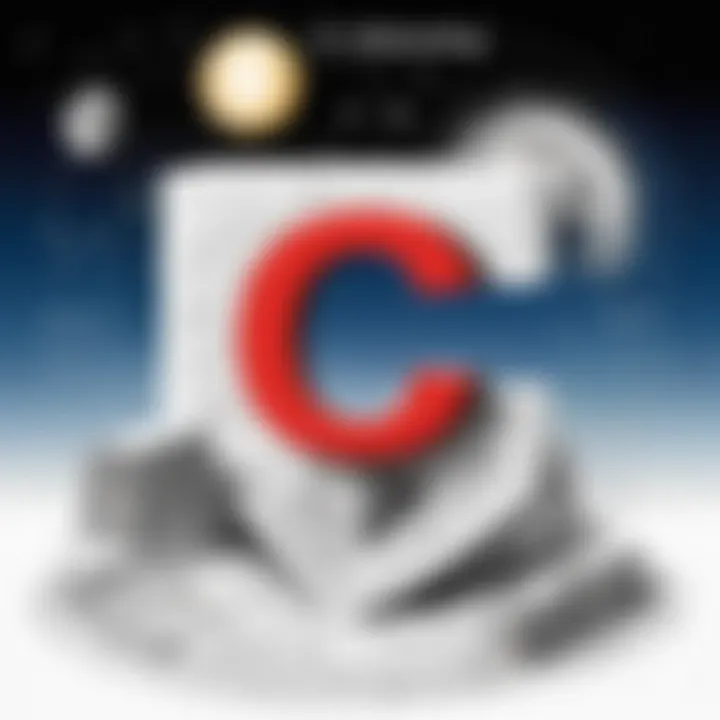
Overview of Topic
C# has come a long way since its inception in the early 2000s. As an object-oriented programming language developed by Microsoft, it plays a vital role in the .NET ecosystem. Whether you're building desktop applications, web services, or mobile applications, mastering C opens doors to countless opportunities in software development. The relevance of C in todayâs tech landscape cannot be overstated â itâs a staple in enterprise environments, providing developers with robust tools to create scalable applications.
A blend of simplicity and power, C# combines the accessibility of high-level languages with the efficiency of low-level programming. This guide will provide a birdâs eye view of C#, breaking down the core concepts, showcasing its features, and emphasizing its practical applications.
Fundamentals Explained
At its core, C# revolves around a few key principles that any developer must grasp. The language is built around the concept of objects, promoting encapsulation, inheritance, and polymorphism. Understanding these principles allows you to design and implement complex systems without getting entangled in excessive complexity.
Key Terminology
- Class: A blueprint for creating objects, encapsulating data and functions.
- Object: An instance of a class containing specific data.
- Method: A function defined within a class, performing actions on objects.
These concepts create the foundational knowledge necessary for effective programming in C#. Itâs like having the right tools in your toolbox; knowing what they are and how they work is crucial for any successful project.
Practical Applications and Examples
C# isnât just theoretical; itâs widely used in real-world applications. From developing web applications using ASP.NET to creating games with Unity, the possibilities are vast. Hereâs a quick look at how C makes an impact in the industry:
- Web Development: With ASP.NET, developers can build dynamic web applications, leveraging the power of C#.
- Game Development: Unity, one of the most popular game engines, relies heavily on C# for scripting â offering interactive and immersive user experiences.
- Desktop Applications: Windows Forms and WPF enable the creation of robust desktop applications that meet user needs effectively.
Code Snippet Example
Here's a simple example that introduces the basic structure of a C# class:
This code illustrates a basic C# class that writes "Hello, World!" to the console. It's fundamental but effective in demonstrating how C operates.
Advanced Topics and Latest Trends
As C# evolves, developers should keep a lookout for the latest trends and advanced techniques. Some noteworthy advancements include:
- Asynchronous Programming: With the async and await keywords, handling operations without blocking the main thread has become easier and more efficient.
- Language Integrated Query (LINQ): This feature allows you to write SQL-style queries directly in C#, improving data handling and manipulation.
Staying informed about these advancements is key to leveraging C# effectively.
Tips and Resources for Further Learning
Expanding your knowledge base can prove invaluable in mastering C#. Here are some resources worth checking out:
- Books:
- C# in Depth by Jon Skeet.
- Pro C# 9 by Andrew Troelsen and Philip Japikse.
- Online Courses: Websites like Pluralsight and Udemy offer comprehensive courses on C#.
- Tools: Visual Studio is ideal for C# development, providing a rich set of features for coding and debugging.
Investing time into these resources will aid in transforming your C# skills from basic understanding to advanced expertise.
"The best time to learn a programming language is when you have a project in mind that can utilize it."
By taking the time to grasp the fundamentals, engage in practical applications, and seek out continued learning opportunities, anyone can master C# and apply it in various tech scenarios.
Prologue to
C# is a versatile and powerful programming language widely used in software development today. Its importance cannot be overstated; C serves as a cornerstone in the realm of modern programming, particularly for those who venture into Windows applications, game development, and web services. As you embark on your coding journey, understanding the fundamentals of C sets the stage for mastering various technological frameworks and programming paradigms. This section introduces you to the essence of C#, emphasizing its ease of use, safety features, and extensive libraries that help developers create robust applications efficiently.
What is #?
C# (pronounced "C-sharp") is a high-level, object-oriented programming language developed by Microsoft in the early 2000s. Designed to be simple and expressive, C
facilitates the development of a wide range of applicationsâfrom desktop to mobile, web, and even enterprise-level software.
It is built on the .NET framework, which provides a comprehensive environment for building, deploying, and running applications. One significant advantage of C# is its strong type-checking, which catches many errors at compile time rather than at runtime, helping developers maintain clean and bug-free code.
Developing in C# allows for:
- Cross-platform compatibility: C# runs on multiple operating systems via .NET Core, letting you build apps for Windows, macOS, and Linux.
- Rich library support: C# users benefit from vast libraries and APIs that simplify many programming tasks.
- Strong community support: The growing C# community means a wealth of resources, forums, and libraries are available for developers.
History and Evolution
C# was launched by Microsoft in a bid to create a language that merged the best features of various programming languages available at the time, such as C++, Java, and Delphi. The first version, C 1.0, was introduced in 2000 alongside the .NET framework. Since then, C has undergone several transformations to accommodate the evolving landscape of software development.
- C# 1.0 (2000): The initial release established C as a modern programming language suitable for application development within the .NET framework.
- C# 2.0 (2005): Introduced generics, anonymous methods, and iterators, expanding its usability significantly.
- C# 3.0 (2007): Brought language-integrated query (LINQ) capabilities, allowing for enhanced data manipulation.
- C# 5.0 (2012): Added support for asynchronous programming with the introduction of the async and await keywords.
- C# 9.0 (2020): Further enhanced expression-bodied members, records, and more to simplify object-oriented programming.
As C# continues to evolve, new features are consistently added, reflecting its commitment to adapting to modern software needs and developer preferences.
"C# is not just a programming language; it's a tool that allows you to turn ideas into reality rapidly and efficiently."
Through this historical lens, itâs clear that C# is more than just another language; it's a dynamic platform that continually shapes the future of software development.
Setting Up the Development Environment
Setting up the development environment is a cornerstone of programming in C#. A solid environment not only streamlines your workflow but also enhances productivity. When getting started with C#, having the right tools and configurations ensures that you can focus more on coding than troubleshooting setup issues. Let's unravel the steps necessary for a smooth start.
Installing Visual Studio
When it comes to developing in C#, Visual Studio stands out as the go-to Integrated Development Environment (IDE). Itâs packed with features that simplify the coding process, making it accessible for beginners while being robust enough for seasoned developers.
- Download Visual Studio: To kick things off, head over to the official Visual Studio website and download the community edition, which is free and offers all the basic functionalities you'll need.
- Installation Process: Once you've got the installer, double-click to launch it. During installation, youâll be presented with options for different workloads. For C# development, select ".NET desktop development". This selection will pave the way for building Windows applications and services using C#.
- Completing the Setup: After choosing your workloads, complete the installation process. Expect it to take some time, depending on your internet speed and system performance. Once done, youâll want to launch Visual Studio and familiarize yourself with the interface.
- Check for Updates: Donât forget to check for updates after installation. This keeps your tools up-to-date with the latest features and bug fixes, which can make a world of difference in your development experience.
"A well-configured environment can save you headaches and hours of debugging."
Visual Studio's robust debugging capabilities and code completion support signify a step into a broader and more efficient development world.
Configuring Project Settings
After installing Visual Studio, the next step is configuring project settings to ensure that everything aligns with your development goals.
- Creating a New Project: Start Visual Studio and click on 'Create a new project'. Here youâll find templates tailored for various project types like Console Apps, Windows Forms, etc. Choose the one that fits your needs best, say a Console Application for most learning scenarios.
- Selecting Framework Versions: Pay attention to the framework version you choose. C# runs on .NET Core and .NET Framework. For new projects, it's advisable to lean towards .NET Core as it supports cross-platform development, giving you flexibility.
- Project Properties: Once the project is created, navigate to the 'Project' menu and select 'Properties'. Here, you can configure various settings:
- NuGet Package Manager: While not strictly a setting, familiarize yourself with the NuGet Package Manager. This is where you can add additional libraries to your project, which can enhance functionality significantly without reinventing the wheel.
- Setting Up Version Control: Although a bit tangential, consider integrating version control, such as Git, especially for larger projects. This practice can safeguard your code and facilitate collaboration.
- Build Configuration: Choose between Debug and Release modes. Debug mode provides detailed error messages during development, while Release mode optimizes the program for end-users.
- Target Framework: Ensure that your target framework aligns with the libraries you plan to use. This is crucial for compatibility.
- Assembly Name and Root Namespace: These identifiers can be crucial for larger projects, helping you maintain clarity.
Taking the time to set up the environment correctly can lead to a smoother coding experience. The earlier in your programming journey you establish a solid foundation, the easier it will be to handle complexities down the line.
Understanding
Syntax
In the world of programming, syntax is akin to grammar in a spoken language. Just as correct grammar is essential for effective communication, understanding syntax is crucial for writing code that not only works but is also readable and maintainable. C# syntax defines how various elements of the language are arranged and how commands, functions, and variables interact. By grasping the basics of C syntax, developers can better navigate through the language's features, avoid common pitfalls, and write cleaner code. Furthermore, a solid foundation in syntax allows programmers to engage with more advanced concepts, as many of these build upon basic structural elements.
Basic Structure of a
Program
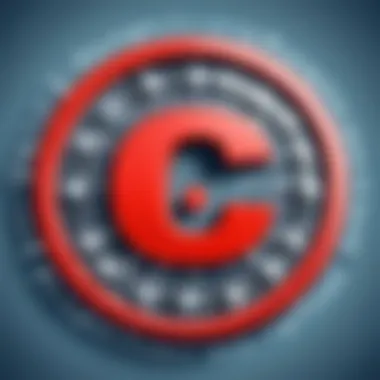
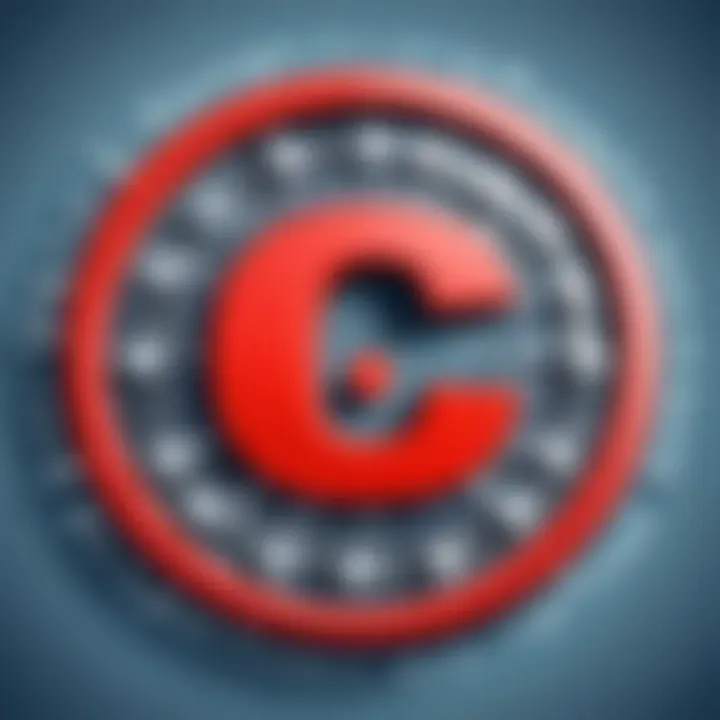
Every C# program has a basic structure that remains consistent regardless of the complexity of the application being developed. To illustrate:
- ``
In this snippet, the first line imports the System namespace, which includes essential classes and methods, such as Console. The defines the main class of the application. The method serves as the entry point for execution. The curly braces delineate blocks of code, ensuring clarity in the programâs structure. This fundamental architecture lays the groundwork for any C# application and showcases the importance of understanding how each piece interacts within the overall framework.
Data Types and Variables
Data types serve as the building blocks for defining variables in C#. Understanding these is essential because variables hold data that your program manipulates. Each variable must have a defined data type, indicating what kind of data it can store.
Value Types
Value types in C# include types such as , , and . One of their defining features is that they store data directly. For instance:
These types offer efficient memory usage because they are stored directly on the stack, providing better performance than reference types. However, a notable aspect of value types is that when they are assigned to a new variable, a separate copy is created. This characteristic often results in a cleaner, more predictable behavior, especially in cases where mutable state is not a concern. The focus on value types is particularly relevant for beginners, as they reinforce core programming concepts without introducing unnecessary complexity.
Reference Types
Reference types differ significantly from value types. They include classes, arrays, and strings. Unlike value types, reference types store references to their data rather than the data itself. For example:
When you create a reference type, itâs stored in the heap, which can lead to more efficient use of memory for larger data structures. However, this also means that when you assign one reference type variable to another, both will point to the same object in memory. This can lead to unintended side effects if not carefully managed. Understanding reference types is essential for working with more complex data structures and sophisticated applications in C#.
"Understanding the nuances of data types is key to mastering the language and ensuring optimal performance in your applications."
In this section, we have explored the importance of syntax and examined the two primary categories of data types. Each contributes to the larger goal of developing applications that are not only functional but also maintainable and efficient. As you delve deeper into C#, this foundational knowledge will be indispensable in your journey to becoming a proficient developer.
Control Flow Statements
In the realm of programming, control flow statements are pivotal. They mold the flow of execution and allow developers to dictate how different blocks of code interact. Imagine driving down a road, but instead of a clear path, you face intersections and detours; thatâs what control flow essentially addresses. Without these statements, a program operates like a car with no steering wheelâgoing nowhere fast.
The significance of mastering control flow is underscored when one contemplates decision-making in programs. They enable conditional execution and iterative processes, which are essential for dynamic and responsive applications. Right from simple programs to complex systems, control flow statements help in making a program behave intelligently.
Moreover, their understanding not only contributes to building functional applications but also promotes better debugging techniques, ensuring that errors or logic mistakes can be swiftly patched without unraveling the entire workings of the code.
If and Switch Statements
At the heart of decision-making in C# are the if and switch statements. They allow your code to take different paths based on certain conditions, and choosing between them often depends on the scenario at hand.
The if statement is straightforwardâif a condition is true, execute the code block within. This is akin to making a choice at a crossroads; if the signs say one way is safe, you choose that path. Hereâs a practical example of its use:
This simple code checks the temperature and prints a message accordingly.
On the other hand, the switch statement shines when you have multiple potential outcomes based on a variable. Picture it like a vending machine; you input a selection, and it dispenses the corresponding product. It makes the code cleaner and more readable when you're checking a single variable against several values:
Both statements are fundamental, yet they serve specific roles. Knowing when to use one over the other can simplify the writing process and make the code more efficient.
Loops: For, While, Do-While
When it comes to repetition in programming, loops are invaluable. They allow you to run a block of code multiple times without rewriting itâa true time-saver. There are several types of loops in C#, among which for, while, and do-while are the most common.
The for loop is like a well-oiled machine, great for scenarios where you know how many times you want to iterate. For example:
This code snippet will print numbers from 0 to 9. Itâs concise and effective for scenarios like iterating over arrays or lists.
While loops, on the other hand, are more flexible. They run as long as a specified condition remains true. Itâs like an open invitation; as long as guests keep coming, the party continues. For instance:
A do-while loop is similar to a while loop, but it guarantees that the block of code inside it will run at least once, even if the condition is false on the first run. Itâs as if youâre saying, "Iâll at least open the door once, regardless of whether anyone is out there."
Functions and Methods
Functions and methods play a crucial role in C# programming. These fundamental building blocks help organize code, making it more manageable and efficient. By breaking down complex problems into smaller, reusable pieces, you can significantly enhance your code quality and maintainability. Additionally, understanding these concepts is essential for anyone looking to write clean code and develop applications that are easy to comprehend and modify.
Defining Methods
In C#, methods are blocks of code designed to perform a specific task. They allow developers to encapsulate logic, making it easier to reuse and debug. When defining a method, it's vital to specify several key components:
- Return Type: This indicates what the method will output, whether it's an integer, string, or even a custom object.
- Method Name: It's essential to pick a name that reveals the purpose of the method, giving users an idea of what it does without needing to read the whole implementation.
- Parameters: These are input values that the method requires to execute. They allow you to pass different data into the method without altering its structure.
Here's a simple example of a method definition in C#:
In this snippet, takes two integers as parameters and returns their sum. By defining such methods, you clarify what each part of your application does, making it easier for others (or future you) to understand the code.
Method Overloading
Method overloading is tricky yet highly beneficial. It allows multiple methods to coexist with the same name but different parameters. This feature is advantageous because it lets developers keep intuitive naming without creating confusion.
Imagine you need addition functionality for both integers and floating-point numbers. Instead of creating distinct methods like and , you can leverage method overloading like this:
Both methods carry the same name but accept different types of inputs, providing versatility while maintaining clarity.
Key Point: Method overloading enhances code readability and reduces clutter, promoting a cleaner coding practice.
In essence, mastering functions and methods, along with their nuances, such as overloading, opens doors to efficient coding practices, laying the groundwork for advanced programming techniques. Focus on these concepts, and you'll find that writing and maintaining code becomes a more seamless experience.
Object-Oriented Programming in
Object-Oriented Programming (OOP) is a paradigim fundamental to C#. Its significance in this programming language can't be overstated. It allows developers to create programs that are modular, reusable, and easier to maintain. By breaking down software into smaller, manageable pieces (commonly known as objects), OOP simplifies complex systems, enabling more efficient coding practices.
There are several key concepts that form the backbone of OOP in C#. These include encapsulation, inheritance, and polymorphism. This foundational knowledge empowers developers to deepen their C# skills effectively.
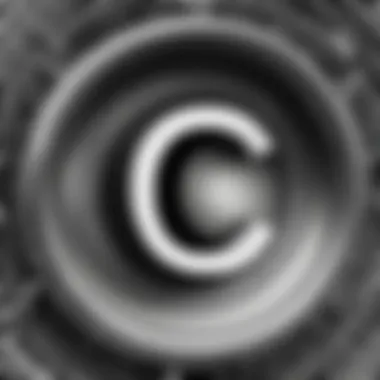
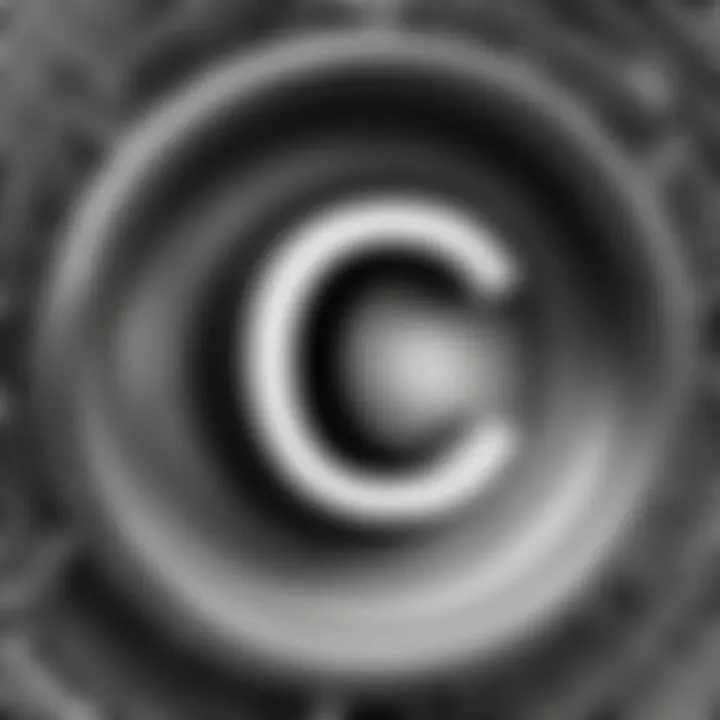
Key benefits of embracing OOP include:
- Modularity: Small chunks of code can be easy to manage and test.
- Reusability: Once a class is defined, it can be reused throughout the application without modification.
- Flexibility and scalability: New features can be added with a minimal impact on existing code.
OOP steers clear of monolithic design, focusing instead on object interactions and state management. This not only promotes cleaner code but also allows for easier debugging, which is crucial as projects grow in size.
Classes and Objects
Classes are blueprints for objects, defining the structure and behaviors that the objects created from the class will possess. In C#, a class serves as a data type that encapsulates related functionality and data. An object, on the other hand, is an instance of a class.
In practical terms, when you define a class in C#, you create templates for various properties and methods. For instance:
This example shows a simple Car class defining color and model properties, as well as a method to drive.
When you instantiate this class, you create an object that represents a specific car, allowing individual characteristics and actions to be assigned and performed.
Inheritance and Polymorphism
Inheritance allows a new class, known as a derived class, to inherit properties and methods from an existing class, referred to as a base class. This promotes code reuse and can simplify complex systems. For instance, consider a class hierarchy where is a base class, and and are derived classes. A Car could inherit from Vehicle, sharing certain attributes while still maintaining its unique properties.
Polymorphism further enhances the functionality of inheritance. It allows methods to do different things based on the object that it is acting upon, despite having the same name. This can be very beneficial for keeping code organized and easy to understand. A classic example in C# is method overriding, where a derived class provides a specific implementation for a base class method. Hereâs an illustrative example:
When you call the method on an instance of Car or Truck, it executes the specific implementation relevant for that type.
Error Handling and Exceptions
When programming in C#, dealing with errors is not just an incidental task; itâs a central piece of the development puzzle. Errors can quietly lurk behind the scenes, causing your applications to halt or behave unexpectedly. Therefore, error handling is not merely a good practiceâit's essential for creating robust, user-friendly software. Effective error handling enables the developer to address issues proactively and maintain control of the execution flow, ultimately leading to a better user experience.
Handling exceptions effectively allows you to catch and manage errors without crashing your application. A good grasp of error handling opens up a clearer path for your programming endeavors. Itâs about anticipating potential problems and having a strategy to deal with them. In this section, we will delve into understanding exceptions and the various mechanisms in C# to deal with them, specifically focusing on the try, catch, and finally constructs.
Understanding Exceptions
An exception in C# is an event that occurs during the execution of a program, disrupting the normal flow of instructions. Think of exceptions as those pesky roadblocks that can throw a wrench into your perfectly planned route. When used properly, exceptions enable you to handle errors gracefully rather than allowing your program to crash or exhibit erratic behavior.
There are various types of exceptions in C#. Some common ones include:
- System.Exception: The base class for all exceptions. Every exception derives from this class.
- System.ArgumentNullException: Thrown when a null reference is passed to a method that does not accept it.
- System.InvalidOperationException: Indicates that a method call is invalid for the object's current state.
Understanding these exceptions and when they might occur is critical in building error handling strategies in your applications. It's not just about preventing crashes; itâs about managing potential faults without compromising the integrity of your software.
Using Try, Catch, Finally
Now that we have a solid understanding of what exceptions are, letâs dive into how to handle them using the , , and blocks in C#. These constructs provide a structured way to manage exceptions and ensure your code can respond accordingly.
- Try Block: The code that might produce an exception is placed inside the try block. If an exception occurs, it's thrown, and the control is immediately passed to the catch block, if one is present.
- Catch Block: It follows the try block and is used to catch exceptions that occur within the try block. You can have multiple catch blocks to handle different types of exceptions in distinct ways, which allows flexibility in your error management strategy.
- Finally Block: This block is optional and is executed after the try/catch blocks, regardless of whether an exception was thrown or caught. Itâs a great place to put clean-up code, like closing files or releasing resources, ensuring that these actions occur whether or not an error occurs during processing.
Using these blocks wisely can make your code resilient. Here's an example of how they all fit together:
In this snippet, attempting to access an invalid index triggers an , which is caught and handled gracefully, while the finally block always executes, confirming the completion of the execution flow.
By mastering these elements of error handling, you can elevate the quality and reliability of your C# applications, making them not just functional but resilient.
Working with Collections
In the world of programming, collections serve as the backbone for organizing and managing data. When we talk about working with collections in C#, weâre referring to the various types of data structures that allow us to store, manipulate, and retrieve data efficiently. Grasping this concept is crucial not only for writing effective programs but for ensuring that they run smoothly and deliver desired outcomes without hiccups.
Collections in C# range from simple structures like arrays to more complex ones like dictionaries. Each has its own advantages and use cases, which can greatly influence the performance and maintainability of your code. Understanding how to choose the right type of collection and utilize it effectively can save you a world of trouble down the line. A common mistake is to resort to arrays for everything, but sometimes using a list or dictionary can be a more fitting choice.
Arrays and Lists
Arrays are the bread and butter of data storage in C#. They provide a fixed-size, indexed collection of elements. Think of an array as a row of boxes where each box has a label (index) ranging from zero to the length of the array minus one. This makes data retrieval straightforward, but the fixed size can also be a limitation. Once you declare an array, you can't change its size.
Consider the following example:
In scenarios where you need to manipulate data dynamically, lists shine. Lists are part of the namespace and offer more flexibility. You can add, remove, and search elements without worrying about the underlying size constraints that come with arrays. If youâre unsure of the number of items youâll be working with, lists are generally the way to go.
For instance:
The key takeaway here is knowing when to use an array versus a list. Arrays might offer better performance for fixed-size collections, while lists provide dynamic capabilities that can save you from a lot of grief.
Dictionaries and Sets
When it comes to handling key-value pairs, dictionaries are the champions. They provide a way to map unique keys to specific values, allowing for quick lookups. It's comparable to looking up a word in a dictionaryâyou have a term (the key), and you want to find its meaning (the value).
Hereâs how you might use a dictionary in C#:
Using a dictionary is particularly beneficial when you need rapid access to data without scanning through a collection. However, ensure that the keys you choose are unique to avoid conflicts, which can lead to unwanted results.
On the other hand, sets are collections that store unique elements without worrying about their order. They come in handy particularly when you're only interested in the presence or absence of an item and want to eliminate duplicates. Hereâs a quick example:
In this case, irrespective of how many times you try to add "Apple", it remains listed only once. This trait of sets proves extremely valuable in situations where data integrity is essential.
The choice of collection directly influences your programâs efficiency. Picking the right one can save you time and prevent headaches.
Understanding how to work with collections in C# gives you the tools to handle data effectively, optimize code performance, and ultimately write more effective programs. Each collection type serves a specific purpose, so knowing their strengths and weaknesses is key to mastering the language.
LINQ: Language Integrated Query
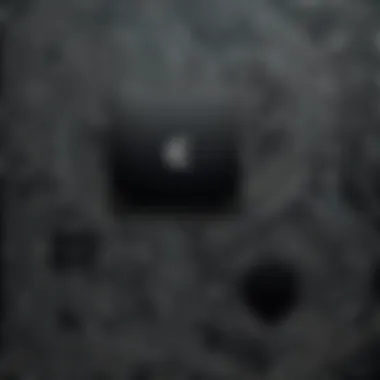
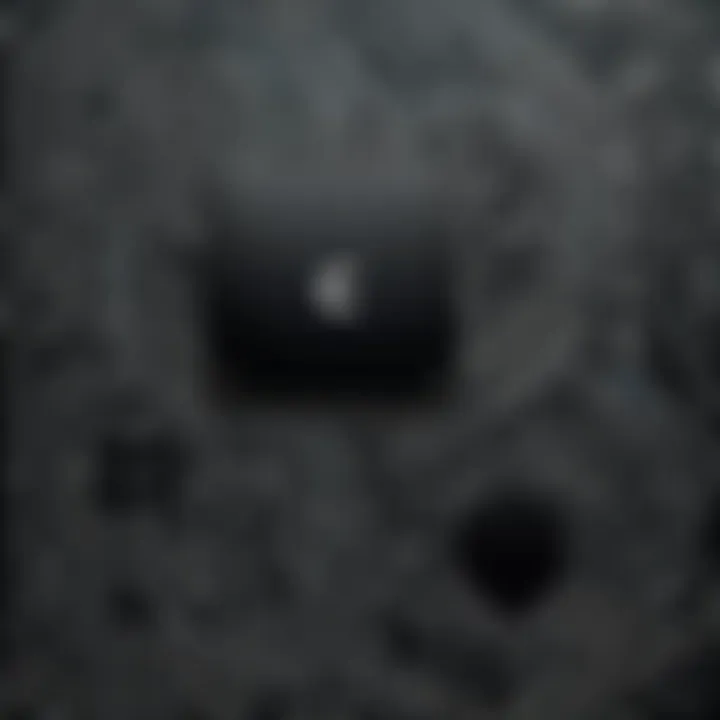
Language Integrated Query, or LINQ, is a powerful feature in C# that simplifies data manipulation and retrieval. It allows developers to write database queries directly within their C code, presenting an ideal fusion of programming and querying capabilities. Understanding LINQ is crucial for anyone looking to work with dataâbe it databases, collections, or XML. It streamlines data access methods, reduces code complexity, and enhances maintenance, making it a staple tool in the programmer's toolkit.
Prolusion to LINQ
LINQ was introduced in .NET Framework 3.5 as part of an effort to standardize data access across different types of data sources. Its syntax is straightforward and can seamlessly blend into C# language constructs, which brings several advantages:
- Readable Code: LINQ enables clear and expressive syntax. Queries can be written like C# code, utilizing its structure, which improves readability. Instead of wrestling with complex SQL statements embedded in C#, you can write queries that are self-explanatory.
- Strongly Typed Queries: LINQ is integrated with C#, thus offering compile-time checking. This means errors are caught early during development rather than at runtime, which is a common nuisance with traditional SQL queries.
- Versatile Data Sources: LINQ can be used to query diverse data sources including arrays, lists, XML, and SQL databases. This flexibility means developers can use a single method to work across various types of data.
As programmers get familiar with LINQ, they often find it not just an efficient way to handle data, but also an enhancement to their programming style. It allows a seamless shift from procedural to a declarative approach, which can be a refreshing change for many.
LINQ Queries in
Writing a LINQ query can often feel akin to reading a bookâclear and direct. There are two main forms of LINQ queries: Query Syntax and Method Syntax.
Query Syntax
Query syntax resembles SQL, making it easy for those familiar with database queries to adapt. Here's an example of filtering a list of numbers:
This code declares a list of numbers, retrieves only even numbers from the list and displays them. The SQL-like style can be particularly welcoming for someone transitioning from SQL to C#.
Method Syntax
In contrast, method syntax relies on method calls, which grants a bit more flexibility.
Both approaches yield the same result yet provide programmers the choice based on their preference. Furthermore, LINQ allows cascading methods, so multiple operations can be applied seamlessly, creating a flow that feels intuitive.
Important Note: Using LINQ effectively means understanding its performance implications. While LINQ is powerful, overusing it for extensive operations on large data sets can lead to performance bottlenecks.
By mastering LINQ, developers can significantly enhance their productivity, streamline their code, and even address more complex data challenges with ease. As you delve deeper into C#, embracing LINQ will surely become one of your biggest strengths.
File /O Operations
File I/O (Input/Output) operations are essential in C# programming, enabling interactions with the file system. Understanding how to read from and write to files allows developers to manage data efficiently and is fundamental for applications that require persistence. File I/O not only helps in storing user-generated data but also plays a significant role in processing information from external sources. As software solutions become increasingly data-driven, mastering these operations is crucial across various domains.
Reading from Files
Reading files is a common task that is necessary for any application that relies on external data. Whether itâs configuration settings, user data, or logs, C# provides several ways to accomplish this. The namespace is your best friend here; it contains classes like that facilitate easy file access.
To read a text file, you might do something like this:
This code succinctly explains how to open a file, read it line by line, and display its contents. The use of ensures that the file is closed properly after operations are complete, which is a good practice for resource management.
There are several critical considerations when reading files:
- Encoding Issues: Different files may use various encodings (like UTF-8 or ASCII). Always ensure that you read the file using the correct encoding to avoid misinterpretation of data.
- File Availability: Always check whether the file exists before attempting to read, to prevent runtime exceptions. You can do this with the method.
- Error Handling: Implementing robust error handling while dealing with file I/O ensures that your application can gracefully recover from issues like missing files or insufficient permissions.
Writing to Files
Writing to files in C# is just as important as reading. Developers often need to log activities, save user input, or back up data to a file. The class, also found in the namespace, makes this task seamless.
You might write data to a file like this:
This example demonstrates the basic process of writing to a file, ensuring that the writer is disposed of properly to free resources.
When writing data, consider these aspects:
- Overwriting vs Appending: Decide whether you want to overwrite existing data or append new data to the file. You can specify this in the constructor by using the second parameter.
- Buffering: Writing data is often buffered for performance reasons. There may be a slight delay before data is physically written to the disk.
- Permissions: Ensure that your application has the necessary file permissions to avoid write failures, especially if it's interacting with system-level folders.
Proper file management is a cornerstone of effective C# programming, ensuring seamless data handling and user experience.
Best Practices and Common Pitfalls
The journey of mastering C# is not just about learning the guidelines of the language but also about recognizing the habits that can either bolster your coding prowess or lead you down a troublesome path. Navigating these best practices and common pitfalls can make a significant difference in your development experience. Adopting solid coding practices means writing cleaner code thatâs easier to understand and maintain. In contrast, falling into traps can result in unnecessary debugging sessions that waste both time and energy.
Code Readability and Maintenance
A cornerstone of effective programming is ensuring that your code is readable. Readable code acts like a well-drawn map, guiding othersâand future youâthrough the logic without much fuss. Here are some pointers for improving code readability in C#:
- Use Meaningful Names: Variables, classes, and methods should have descriptive names. For instance, instead of naming a variable , consider something like . This can save time for anyone reading the code down the line, including yourself.
- Consistent Formatting: Adhere to a consistent indentation style and use spaces to separate logical units in your code. This will help in easily understanding the structure and flow of your program.
- Comment Purposefully: While comments are essential, overusing them can clutter your code. Use comments to explain the 'why' behind complex logic, not the 'what' which should be clearly inferred from the code itself.
"Readability counts. Thereâs no excuse for writing code thatâs so intricate that it requires a manual to understand."
In practice, you can see the effect of these tips in an example:
This example is straightforward, maintains clear logic, and uses descriptive naming.
Avoiding Common Errors
Every coder, no matter how seasoned, has stumbled at some point. Knowing common pitfalls can save you from perpetually stepping in the same puddle. Here are several recurring errors new C# programmers often face:
- Null Reference Exceptions: These happen when you try to use an object that isn't actually instantiated. Always check that objects are not null before accessing their members.
- Off-by-One Errors: Especially common in loops, these occur when your loop condition mishandles boundaries. An extra iteration can lead to bugs that are tricky to track down.
- Ignoring Exception Handling: Itâs tempting to bypass error catching, thinking your code is perfect. In C#, not wrapping risky operations in try-catch blocks can lead to crashes â and a headache when tracking down bugs.
- Not Using Annotations: Attributes like or help in building better code by providing additional context. Ignoring these can lead to confusion.
By being aware of these issues, you can sharpen your skills and write C# scripts that run smoothly. Embracing best practices and steering clear of common pitfalls will help you build not just any software, but quality software!
Epilogue and Next Steps
In the fast-paced world of technology, mastering programming languages like C# becomes imperative. This section wraps up what we have explored and sets the stage for your continued journey in the realm of software development. Understanding the structure, syntax, and the paradigms of C not only enhances your coding skills but also fosters a mindset of problem-solving, which is critical in any tech field.
Recap of Key Concepts
Throughout this guide, we have delved into several essential elements of C#. Letâs take a moment to summarize these key concepts:
- Foundation of C# Programming: We began by grasping what C is and its historical significance. The foundation sets the tone for everything that follows.
- Development Setup: Setting up a development environment with Visual Studio was vital. Configuring project settings ensured that your coding experience is smooth and efficient.
- Basic Syntax and Control Flow: Understanding the basic structure, data types, and control flow statements lays the groundwork for any programming undertaking. This knowledge is crucial to developing logical and functional code.
- Methods and OOP Principles: Learning about functions and the object-oriented programming model allowed us to appreciate the elegance of C#. The concepts of classes, inheritance, and polymorphism are cornerstones in modern programming.
- Error Management and Collections: Knowing how to handle exceptions and working with collections, such as arrays, lists, dictionaries, and sets, is vital for writing robust applications.
- Exploring LINQ: The introduction to Language Integrated Query (LINQ) opened doors to powerful data manipulation capabilities within C#, allowing for more fluid and efficient coding.
- File Operations: We explored file I/O operations, understanding how to read and write files helped solidify practical application skills.
- Best Practices: It is paramount to emphasize code readability and maintenance while highlighting common pitfalls to avoid.
While this guide provides a comprehensive overview, the journey in mastering C# is far from over.
Resources for Further Learning
To keep advancing your C# skills, consider these helpful resources:
- Microsoft Documentation: The official documentation offers a wealth of knowledge on C# and the .NET framework. Itâs always up-to-date with the latest features.
- Wikipedia - C#: A good place to gain historical context and an overview of the language. C Sharp
- Reddit Communities: Engage with other learners and professionals in dedicated C# subreddits. The community offers invaluable insights and support. Reddit
- Online Courses: Platforms like Coursera, Udemy, and Pluralsight offer extensive courses that cover C# in various depths. These can provide structured learning tailored to your needs.
- Books: Consider titles like "C# in Depth" by Jon Skeet for a deep dive into advanced topics.
By continuing to explore and practice, you will not only solidify your knowledge but also position yourself as a capable developer ready to tackle real-world challenges. Remember, programming is as much about persistence and curiosity as it is about technical skills.
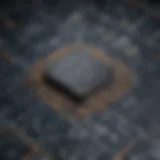
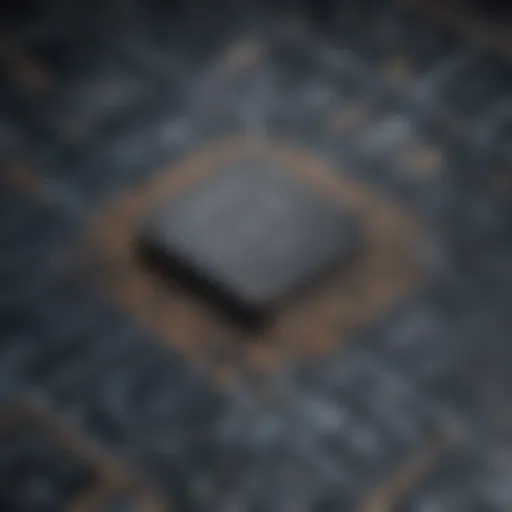