Mastering Data Structures and Algorithms: A Comprehensive Study Guide for Tech Enthusiasts
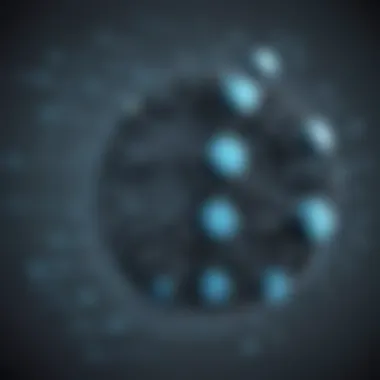
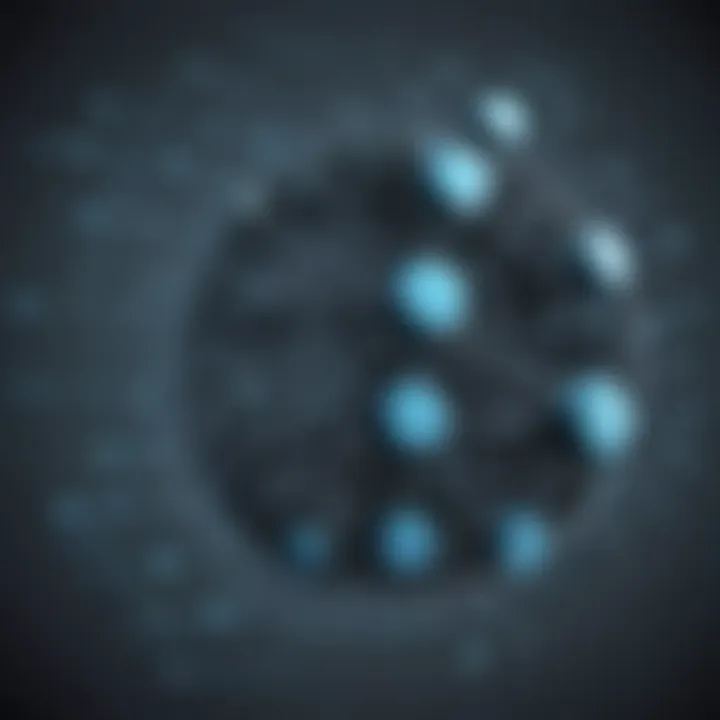
Overview of Data Structures and Algorithms
In this section, we lay the foundation for an in-depth exploration of data structures and algorithms. Understanding these concepts is pivotal for individuals in the tech realm, from novices to seasoned professionals. The significance of data structures and algorithms in the tech industry cannot be overstated; they form the backbone of computer science, enabling efficient problem-solving and complex system design. Exploring the history and evolution of these fundamental constructs provides insight into their relevance and practical applications in modern computing.
Fundamentals Unveiled
Delving into the fundamentals of data structures and algorithms unveils core principles that underpin their functionality. It is essential to grasp key terminologies like arrays, linked lists, stacks, queues, and tree structures to navigate the intricate world of data organization. Understanding basic concepts such as time complexity, space complexity, and algorithmic paradigms is crucial for developing a strong foundation in this domain.
Applications and Examples in Practice
Real-world applications and examples offer invaluable insights into the practical utility of data structures and algorithms. By examining case studies and hands-on projects, individuals can witness how these theoretical constructs translate into tangible solutions. Providing code snippets and implementation guidelines enhances comprehension, empowering learners to apply their knowledge in coding challenges and software development.
Advanced Concepts and Emerging Trends
Exploring advanced topics and emerging trends in data structures and algorithms sheds light on cutting-edge developments shaping the future of technology. From innovative techniques like dynamic programming and graph algorithms to the optimization of data storage and retrieval, staying abreast of the latest advancements is vital for staying ahead in this rapidly evolving field. Anticipating future prospects and upcoming trends enables professionals to adapt to the dynamic landscape of tech innovation.
Tips and Resources for Continued Learning
Equipping individuals with tips and resources for further learning is essential for their continuous growth and development in data structures and algorithms. Recommending books, online courses, and tools enhances the learning journey, providing avenues for deeper exploration and hands-on practice. By guiding learners towards valuable resources and practical applications, we empower them to expand their knowledge and proficiency in this critical domain.
Introduction to Data Structures and Algorithms
For tech enthusiasts, beginners, students, and professionals diving into the world of data structures and algorithms, understanding the fundamentals is paramount. In this section, we will unravel the core concepts that underpin this intricate domain. From the intricate details of data structures to the significance of algorithms, this guide aims to provide a robust foundation for anyone venturing into this field.
Understanding the Basics
Definition of Data Structures
Data structures form the backbone of any algorithmic solution, serving as the foundation upon which computational tasks are accomplished. Defined as the organization and storage format of data for efficient access and modification, data structures play a pivotal role in problem-solving scenarios. Their ability to streamline data management and retrieval processes makes them a popular choice among programmers. However, while data structures offer optimization benefits, they also come with trade-offs in terms of memory utilization and implementation intricacies.
Importance of Algorithms
Algorithms, on the other hand, are the blueprint for transforming data through a series of computational steps into a desired output. Their significance lies in their efficiency and effectiveness in solving complex problems with precision. By understanding algorithmic principles, programmers can devise optimized solutions and enhance code performance. Despite their advantages, algorithms may pose challenges in terms of scalability and adaptability to varying inputs.
Fundamental Concepts
Time Complexity
The time complexity of an algorithm quantifies the amount of time it takes to run as a function of the input size. It provides insights into the scalability and efficiency of the algorithm, crucial for selecting the most suitable approach to a problem. While lower time complexity signifies faster execution, optimizing time complexity often involves intricate design considerations and trade-offs between different strategies.
Space Complexity
In contrast, space complexity pertains to the amount of memory space required by an algorithm to solve a problem concerning the input size. Managing space complexity is vital for ensuring efficient memory utilization and preventing resource wastage. Balancing space complexity with time complexity forms a delicate interplay in algorithm design, necessitating thoughtful analysis and trade-off evaluations.
Big O Notation
Big O notation is a mathematical notation used to categorize the behavior of an algorithm based on its performance as the input size approaches infinity. By classifying algorithms into complexity classes, Big O notation facilitates comparisons and predictions of algorithmic efficiency across different scenarios. Understanding Big O notation enables programmers to make informed decisions regarding algorithm selection and optimization strategies.
Types of Data Structures
Arrays
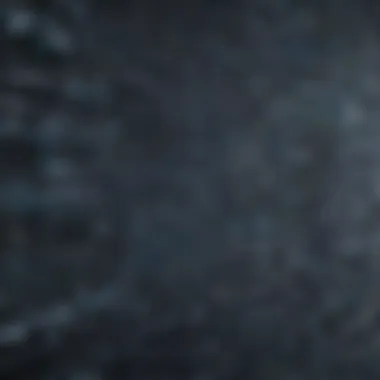
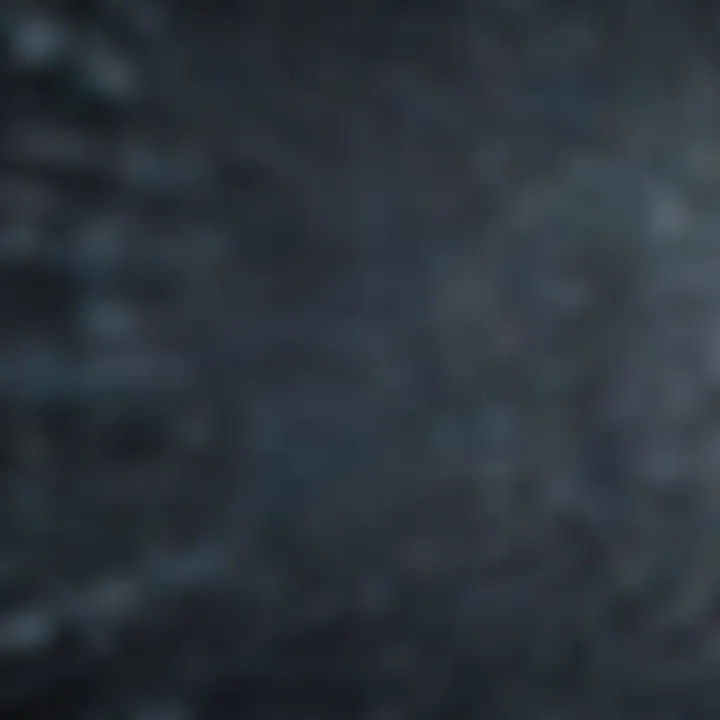
As one of the foundational data structures, arrays facilitate the storage of elements in contiguous memory locations, allowing for efficient random access to elements. Their simplicity and versatility make them suitable for various applications, from basic list operations to more complex data manipulations. However, the fixed size of arrays can constrain dynamic data management, requiring careful considerations in array usage.
Linked Lists
Unlike arrays, linked lists offer dynamic memory allocation and flexibility in data organization by connecting elements through pointers. This dynamic structure enables efficient insertion and deletion operations, ensuring optimal resource utilization. Nevertheless, the traversal overhead and lack of random access capabilities in linked lists may pose challenges in specific computational tasks.
Stacks
Stacks represent a fundamental data structure following the Last In, First Out (LIFO) principle, where elements are inserted and removed in a sequential manner. This behavioral characteristic makes stacks ideal for handling recursive function calls and tracking program state changes. While stacks offer simplicity and efficiency in certain scenarios, managing stack overflow and underflow conditions requires careful error handling.
Queues
Contrary to stacks, queues adhere to the First In, First Out (FIFO) ordering, ensuring that elements are processed in the order they were inserted. Queues find applications in scenarios requiring task scheduling and data processing sequences. However, the linear access pattern in queues may lead to performance bottlenecks in specific situations, emphasizing the need for queue optimization strategies.
Trees
Trees present hierarchical data structures comprising nodes connected by edges, enabling the representation of relationships between entities in a tree-like format. The versatility of trees makes them instrumental in modeling hierarchical data dependencies and facilitating quick search operations. Nonetheless, tree traversal complexities and maintenance overheads necessitate thoughtful tree design and manipulation strategies.
Graphs
Graphs offer a flexible structure for modeling complex relationships between entities through nodes and edges, allowing for the representation of diverse interconnected data scenarios. Their applicability spans a wide range of fields, from social network analysis to route optimization algorithms. Yet, handling the intricacies of graph traversal and ensuring optimal graph connectivity pose challenges that warrant careful algorithmic solutions.
Common Algorithms
Sorting Algorithms
Sorting algorithms are instrumental in arranging elements in a specified order, enhancing data search and retrieval processes. The selection of an appropriate sorting algorithm can significantly impact system performance and user experience. While sorting algorithms like quicksort offer efficient solutions for large datasets, considerations regarding stability and adaptability are crucial in algorithm selection.
Searching Algorithms
Searching algorithms enable the efficient retrieval of target elements within a data collection by systematically exploring relevant data subsets. Their effectiveness in locating specific items swiftly contributes to enhanced data processing speeds and user satisfaction. Nonetheless, optimizing searching algorithms for scalability and adaptability requires thoughtful design and implementation choices.
Dynamic Programming
Dynamic programming is a technique for solving complex problems by breaking them down into overlapping subproblems and storing computed solutions for future reference. This methodical approach streamlines problem-solving processes and reduces redundant computations, enhancing algorithm efficiency. However, deciphering optimal subproblem divisions and storage structures are key considerations in dynamic programming design.
Greedy Algorithms
Greedy algorithms aim to make locally optimal choices at each step of a problem with the objective of finding a global optimum. Their simplicity and efficiency in finding quick solutions make them appealing for various computational tasks. Yet, the inherent trade-off between local and global optimality requires careful algorithmic design to mitigate the risk of suboptimal outcomes.
Advanced Topics in Data Structures and Algorithms
In this section of our comprehensive guide on Data Structures and Algorithms, we delve into advanced topics that are crucial for a thorough understanding of this field. These topics go beyond the basics and provide a deeper insight into complex algorithms and sophisticated data structures. By studying Advanced Topics in Data Structures and Algorithms, readers can enhance their problem-solving abilities and analytical skills, making it indispensable for students and professionals alike.
Optimization Techniques
Divide and Conquer
Divide and Conquer is a fundamental algorithmic paradigm that involves breaking down a problem into smaller, more manageable subproblems, solving them recursively, and then combining the solutions to find the final result. This approach is key to many popular algorithms like Merge Sort and Quick Sort. The beauty of Divide and Conquer lies in its efficiency and scalability, allowing for faster computation of complex problems. However, its drawback includes increased memory consumption due to the recursive nature of the algorithm.
Memoization
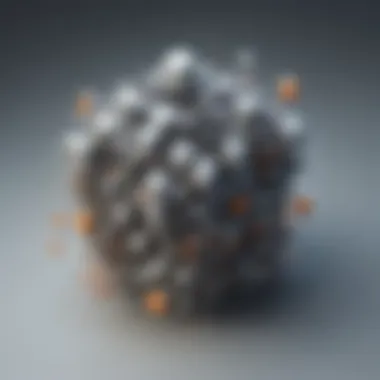
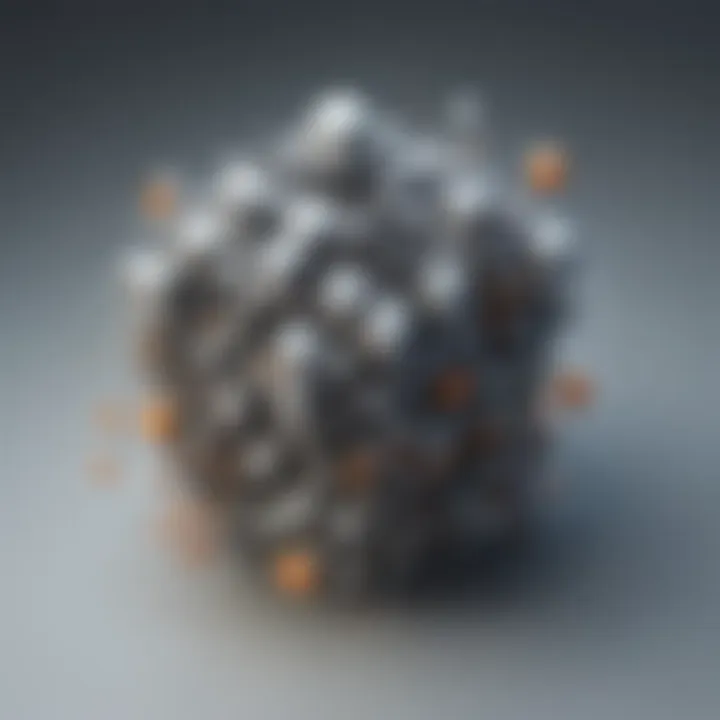
Memoization is a technique used to optimize recursive algorithms by storing previously computed results and retrieving them when needed. By memorizing the results of expensive function calls, Memoization reduces redundant computations, significantly improving the algorithm's performance. This approach is particularly beneficial for dynamic programming problems where subproblems are solved repeatedly, making Memoization a valuable tool in algorithmic optimization. Nonetheless, Memoization requires careful implementation to prevent memory overflow and ensure accuracy in results.
Backtracking
Backtracking is a systematic way to explore all possible solutions to a problem by incrementally building candidates and eliminating those that fail to satisfy the conditions. This method is commonly used in constraint satisfaction problems like the N-Queens puzzle and Sudoku. Backtracking offers a comprehensive search strategy but can be computationally expensive for problems with a large search space. Despite this drawback, Backtracking is essential for finding optimal solutions in various combinatorial problems, making it a versatile technique in algorithmic design.
Complex Data Structures
Within the realm of Data Structures and Algorithms, the study of Complex Data Structures delves into intricate and specialized data organization methods. Heaps, Hash Tables, Tries, and AVL Trees are advanced structures that provide efficient data storage and retrieval mechanisms for specific use cases. Understanding these structures is paramount for developing sophisticated algorithms and optimizing code efficiency in real-world applications.
Heaps
Heaps are binary trees that follow the heap property, where each parent node has a value greater than or equal to its children in a max heap, or less than or equal to its children in a min heap. This unique property enables efficient insertion, deletion, and extraction of the maximum or minimum element, making Heaps ideal for priority queue implementations. Although Heaps offer quick access to extremal elements, they may not perform as efficiently for arbitrary searches due to their tree structure.
Hash Tables
Hash Tables utilize a hash function to map keys to their corresponding values, facilitating constant-time average-case operations for basic data operations like insertions and lookups. This feature makes Hash Tables a popular choice for implementing dictionaries and databases where quick data retrieval is essential. However, collisions and load factor management are critical considerations in Hash Table design to ensure optimal performance and prevent degradation in search efficiency.
Tries
Tries are tree-like structures designed for efficient key search and prefix matching in dictionaries and word repositories. Each node in a Trie represents a common prefix and allows for fast retrieval of words based on partial matches. Tries excel in text processing applications where autocomplete and word prediction features are required. Despite their effectiveness in pattern matching, Tries may consume more memory compared to other data structures, especially for sparse datasets with limited common prefixes.
AVL Trees
AVL Trees are self-balancing binary search trees that maintain their height balance after each insertion or deletion operation. By ensuring that the height difference between the left and right subtrees does not exceed one, AVL Trees guarantee logarithmic time complexity for search, insertion, and deletion operations. This balanced property enhances the overall performance of AVL Trees in comparison to unbalanced binary search trees, making them an optimal choice for scenarios requiring consistent search efficiency. However, the need for balancing operations in AVL Trees can introduce additional overhead during dynamic data modifications.
Algorithm Design Patterns
Algorithm Design Patterns encapsulate reusable solutions to common algorithmic problems, offering developers ready-made strategies to address recurring challenges effectively. Greedy vs. Dynamic Programming, Sliding Window, and Two Pointers are prominent patterns that cater to different problem-solving paradigms, enabling efficient algorithm development and optimization.
Greedy vs. Dynamic Programming
Greedy algorithms make locally optimal choices with the hope of finding a global optimum, whereas Dynamic Programming breaks down problems into smaller subproblems and stores the results for future reference, avoiding redundant computations. The choice between Greedy and Dynamic Programming depends on the problem's characteristics, with Greedy algorithms excelling in simple, single-pass solutions and Dynamic Programming providing optimal solutions for more complex, overlapping subproblems. The trade-off lies in time complexity versus space complexity, with Greedy solutions often being faster but less accurate than Dynamic Programming approaches.
Sliding Window
Sliding Window is a technique used to efficiently process subarrays, substrings, or intervals of a given array or string. By maintaining a window of fixed size while iterating through the elements, Sliding Window algorithms update the window's boundaries based on specific conditions, allowing for constant-time operations in sliding unidirectional or bi-directional patterns. This approach is beneficial for problems requiring continuous subsequence computations, such as maximum sum subarray or longest substring without repeating characters. However, careful window management is crucial to ensure the algorithm's correctness and optimal performance.
Two Pointers
Two Pointers is a strategy that employs two iterators or indices moving towards each other to solve problems like array manipulation, linked list operations, or string processing. By manipulating pointers based on certain conditions, Two Pointers algorithms enable efficient scanning or comparison of elements in linear time complexity. This technique is particularly useful for problems involving array traversal, element pairing, or window aggregation. While Two Pointers offer a simple and intuitive approach to problem-solving, ensuring correct pointer movements and boundary conditions is essential to avoid errors and logical inconsistencies.
Practical Applications of Data Structures and Algorithms
Software Development
Optimizing Code Efficiency
Embarking on the intricate journey of [Optimizing Code Efficiency] is paramount within the scope of software development. This facet accentuates the essence of streamlining code to enhance performance and speed, a pivotal objective in the software engineering landscape. The core characteristic of [Optimizing Code Efficiency] lies in its efficacy in reducing processing time and resource utilization, thereby optimizing the overall functionality of software systems. Its widespread popularity stems from its ability to elevate productivity and user experience, making it a favored methodology in enhancing software performance. However, while the advantages of [Optimizing Code Efficiency] are pronounced, it is crucial to note that intricate optimization techniques may lead to increased complexity, potentially impacting code maintainability.
Problem-Solving Techniques
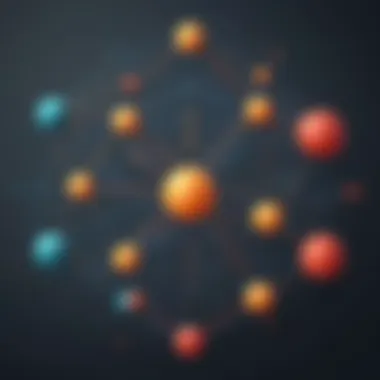
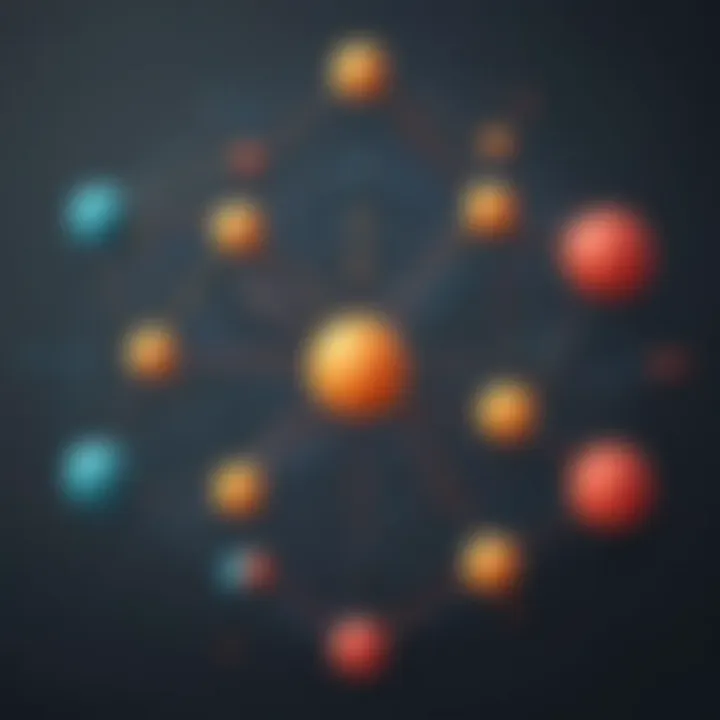
Diving into the intricacies of [Problem-Solving Techniques] elucidates the significance of employing structured methodologies to address complex software dilemmas effectively. The distinctive feature of [Problem-Solving Techniques] lies in its systematic approach towards dissecting and resolving intricate programming challenges, aiding developers in designing efficient and scalable solutions. Its prevalence as a go-to strategy in software development emanates from its capacity to foster logic and analytical thinking, essential components in devising robust and sustainable software architectures. While the benefits of [Problem-Solving Techniques] are multifaceted, one must remain cognizant of potential drawbacks, such as the time-intensive nature of employing elaborate problem-solving frameworks.
AI and Machine Learning
Optimization Algorithms
Unraveling the realm of [Optimization Algorithms] within the landscape of AI and Machine Learning unveils the pivotal role they play in enhancing predictive modeling and algorithmic efficiency. The key characteristic of [Optimization Algorithms] lies in their ability to iteratively refine and enhance models to achieve optimal performance metrics, making them indispensable in the data science domain. Their ubiquity and favor within this article stem from their capacity to expedite convergence towards accurate solutions, expediting the model development lifecycle. Nonetheless, despite their advantages, it is imperative to acknowledge potential limitations, including algorithm sensitivity to hyperparameters and model complexity.
Decision Trees
Exploring in-depth the intricacies of [Decision Trees] in AI and Machine Learning accentuates their significance as intuitive and interpretable tools for predictive modeling and classification tasks. The foundational characteristic of [Decision Trees] rests in their hierarchical structure that mimics human decision-making processes, simplifying complex data analyses into comprehensible decision paths. Their popularity and utility in this article are attributed to their ability to provide actionable insights and facilitate transparent decision-making within machine learning workflows. However, it is essential to recognize the trade-offs inherent in [Decision Trees], such as susceptibility to overfitting with noisy data.
Cybersecurity
Cryptography
Delving into the intricate domain of [Cryptography] within the purview of cybersecurity unveils its paramount role in safeguarding sensitive information and securing digital communications. The crux of [Cryptography] lies in its utilization of complex algorithms to encrypt and decrypt data, fortifying data integrity and confidentiality in communication channels. Its prominence in this article stems from its critical importance in thwarting cyber threats and ensuring data privacy across digital platforms. Despite its myriad advantages, aspects like algorithm vulnerability to sophisticated attacks underscore the perpetual cat-and-mouse game inherent in cybersecurity.
Secure Hash Algorithms
Navigating through the realm of [Secure Hash Algorithms] within cybersecurity illuminates their pivotal function in ensuring data integrity and authenticity through secure hashing mechanisms. The quintessential characteristic of [Secure Hash Algorithms] lies in their capability to generate unique hash values for data inputs, enabling verification and detection of tampering in digital transactions. Their preferred status within this article is underscored by their efficacy in fortifying digital signatures and preventing unauthorized alterations to transmitted data. Nevertheless, nuances like vulnerability to collision attacks remind practitioners to stay vigilant in enhancing algorithm resilience for robust cybersecurity frameworks.
Resources and Further Learning
In the realm of data structures and algorithms, the section on Resources and Further Learning holds paramount significance within this article. It serves as a guiding light for individuals eager to deepen their understanding and master the intricacies of this complex domain. By delving into additional resources and avenues for learning, readers can expand their knowledge horizons and cultivate a more profound comprehension of the subject matter. Furthermore, this segment not only facilitates skill enhancement but also enables continuous growth and development in the field of computer science.
Online Courses and Tutorials
Coursera
Coursera, known for its wide array of online courses, plays a pivotal role in augmenting the understanding of data structures and algorithms. Its structured modules and interactive learning experiences cater to diverse learning styles, making it an invaluable resource for learners of all levels. Coursera's emphasis on practical applications and real-world problem-solving scenarios equips individuals with tangible skills that are directly applicable in the professional realm, making it a preferred choice for enthusiasts seeking comprehensive knowledge in this domain.
edX
edX, a prominent platform for online education, contributes significantly to the overarching goals of enhancing proficiency in data structures and algorithms. With its curated courses and expert-led content, edX stands out as a reliable source of in-depth knowledge and skill development. Its flexibility and accessibility make it a favored option for individuals looking to upskill or transition into the field of software development, emphasizing a blend of theoretical concepts and practical implementation to maximize learning outcomes.
LeetCode
LeetCode, renowned for its challenging coding problems and algorithmic puzzles, serves as an indispensable tool for honing problem-solving skills in data structures and algorithms. The platform's focus on algorithmic thinking and efficiency drives individuals to enhance their logical reasoning and coding prowess. By tackling LeetCode's diverse range of algorithm challenges, learners can refine their problem-solving strategies and elevate their understanding of complex data structures, making it a popular choice among aspiring programmers seeking hands-on practice and application-oriented learning.
GeeksforGeeks
GeeksforGeeks, a treasure trove of programming resources and technical content, offers a wealth of information on data structures and algorithms. Its comprehensive tutorials, insightful articles, and practice problems cater to a broad audience, from novices to seasoned professionals, seeking to bolster their expertise in this domain. GeeksforGeeks' user-friendly interface and categorized content make it a preferred platform for individuals looking to enhance their problem-solving skills, understand algorithmic complexities, and stay updated with the latest industry trends, solidifying its place as a go-to resource for inquisitive minds.
Books and References
Introduction to Algorithms (CLRS)
The book 'Introduction to Algorithms (CLRS)' stands as a cornerstone in the realm of data structures and algorithms, offering an in-depth exploration of fundamental concepts and algorithmic techniques. Featuring comprehensive explanations and algorithm analyses, it provides readers with a robust foundation for understanding complex data structures and optimizing algorithmic performance. The book's lucid explanations and illustrative examples make it a preferred choice for individuals looking to delve into the intricate world of algorithms and data manipulation.
Data Structures and Algorithm Analysis in ++
The resource 'Data Structures and Algorithm Analysis in C++' serves as a comprehensive guide for individuals keen on mastering data structures and algorithmic principles using the C++ programming language. Through detailed explanations and practical examples, the book elucidates various data structures, their implementations, and the algorithmic paradigms associated with them. Its focus on C++ coding practices and efficiency analysis equips readers with the necessary tools to tackle algorithmic challenges and optimize code performance, making it an indispensable reference for developers and students alike.
Algorithms (Sedgewick)
The book 'Algorithms (Sedgewick)' elucidates intricate algorithmic concepts with clarity and precision, providing readers with a profound understanding of key algorithms and their applications. With a meticulous approach to algorithm design and analysis, the book equips individuals with a holistic view of algorithmic problem-solving and optimization techniques. Its emphasis on algorithmic paradigms and algorithm analysis fosters critical thinking and algorithmic creativity, making it a valuable resource for enthusiasts seeking to grasp the intricacies of algorithms and enhance their computational problem-solving skills.