Mastering DataFrames in Python Pandas
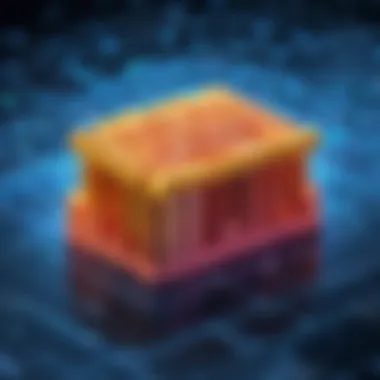
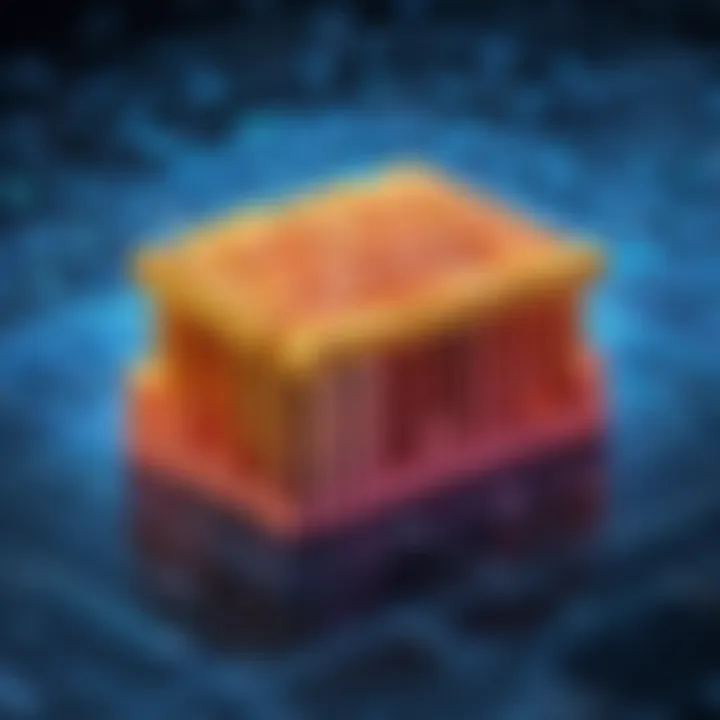
Overview of Topic
Foreword to DataFrames in Pandas
DataFrames are a central feature of the Pandas library in Python, widely used for data manipulation and analysis. With their user-friendly design, DataFrames allow users to work with structured data, akin to a table in a relational database. This section will explain the purpose of DataFrames, their design, and their role in data science and analytics.
Scope and Significance in the Tech Industry
As organizations increasingly rely on data-driven decision-making, understanding how to effectively manage and analyze large datasets becomes pivotal. In this context, DataFrames serve as a robust tool to facilitate data wrangling, transformation, and analysis in Python programming. Whether you are a novice programmer or a seasoned data scientist, mastering DataFrames can significantly enhance your analytics capabilities.
Brief History and Evolution
Pandas was developed in 2008 by Wes McKinney as an open-source library to meet the growing demand for data analysis tools in Python. Since then, it has evolved and expanded its features, now supporting numerous data operations. The DataFrame structure was inspired by data frames in R, but it has adapted to suit the versatility of Python. Today, Pandas remains one of the leading libraries for data analysis and manipulation.
Fundamentals Explained
Core Principles and Theories Related to DataFrames
A DataFrame is essentially a 2-dimensional labeled data structure, where data is aligned in a tabular format with rows and columns. This allows for both intuitive viewing and efficient data handling. Key principles involve indexing, selecting, and filtering data while maintaining performance and scalability.
Key Terminology and Definitions
Understanding a few key terms is fundamental to effectively using DataFrames.
- Series: A one-dimensional labeled array that can hold any data type.
- Index: A label that uniquely identifies each row in a DataFrame.
- Column: A label that identifies each variable or attribute in the data.
Basic Concepts and Foundational Knowledge
To work with DataFrames, familiarity with basic operations is necessary. This includes importing the Pandas library, loading data from various formats such as CSV and Excel, and performing essential functions like sorting and filtering.
Practical Applications and Examples
Real-World Case Studies and Applications
DataFrames have wide-ranging applications, from financial analysis to scientific research. For instance, a company may use DataFrames to analyze sales data, helping them identify trends and optimize marketing strategies. In research, scientists might utilize DataFrames to analyze experimental data, facilitating more informed conclusions.
Demonstrations and Hands-On Projects
Here is a simple example to demonstrate creating a DataFrame:
When you run this code, you will see a neatly organized table that showcases individual data points clearly.
Code Snippets and Implementation Guidelines
In addition to creating DataFrames, it’s important to know how to manipulate them. To filter rows based on conditions, one can use the following code:
Advanced Topics and Latest Trends
Cutting-Edge Developments in the Field
With the rise of big data, DataFrames are continually evolving. Recent advancements include enhanced performance for handling large datasets and integration with other libraries such as NumPy for scientific computing.
Advanced Techniques and Methodologies
Techniques such as pivoting, merging, and group-by operations allow for more complex analyses. Understanding these advanced functionalities enables users to derive deeper insights from their data.
Future Prospects and Upcoming Trends
As the landscape of data analysis evolves, so too will the tools that support it. DataFrames will likely continue to adapt, becoming even more integrated with machine learning and AI technologies to streamline analysis workflows.
Tips and Resources for Further Learning
Recommended Books, Courses, and Online Resources
To deepen your understanding of DataFrames, consider the following resources:
- "Python for Data Analysis" by Wes McKinney.
- Online courses on platforms like Coursera or Udacity that focus on data manipulation with Pandas.
Tools and Software for Practical Usage
Apart from Pandas, other tools can complement your work with DataFrames. Libraries like NumPy, Matplotlib, and Seaborn are beneficial for extended data analysis and visualization.
Building a strong foundation in Pandas DataFrames unlocks new possibilities in data science and analysis. Engaging with hands-on projects and continuous learning is vital in mastering the tool.
Preface to Python Pandas
Python Pandas is an essential library in the realm of data analysis. It provides tools for handling and analyzing data in a way that is both efficient and easily understandable. This article highlights the significance of mastering Python Pandas, particularly the DataFrame structure. Understanding this library equips individuals with the capabilities to manipulate complex data sets with ease.
The relevance of pandas cannot be understated. It simplifies various tasks, from data cleaning to statistical analyses. Its intuitive design allows for a smooth learning curve, making it accessible even to those new to programming. Moreover, with the explosion of data in various fields, the ability to analyze and extract insights from data has become more valuable.
Understanding the Basics
Before diving into advanced functionalities of pandas, it is vital to grasp the basic concepts. Pandas primarily offers two data structures: Series and DataFrame. A Series is essentially a one-dimensional labeled array, while a DataFrame is a two-dimensional data structure, similar to a table in a database or an Excel spreadsheet. It holds data in rows and columns, allowing for complex data handling tasks.
The syntax is designed to be straightforward. Operations such as filtering, merging, or transforming data can be performed with minimal lines of code. This efficiency is a key advantage when working with large data sets.
Importance in Data Analysis
Data analysis has evolved dramatically over the years, and Python Pandas plays a critical role in this evolution. By providing a user-friendly interface, it helps analysts and researchers transform raw data into actionable insights. The ability to quickly analyze data means faster decision-making processes, which is crucial in today's fast-paced world.
Pandas also offers robust support for data manipulation. For example, it allows users to handle missing data, perform group by operations, and aggregate data with ease. These functionalities are essential for accurately interpreting data.
Furthermore, pandas integrates seamlessly with other libraries such as NumPy and Matplotlib. This allows users to perform numerical computations and create visualizations without extensive boilerplate code. In essence, mastery over pandas can greatly enhance one's data analysis capabilities.
What is a DataFrame?
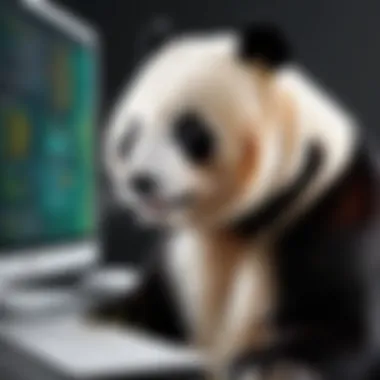
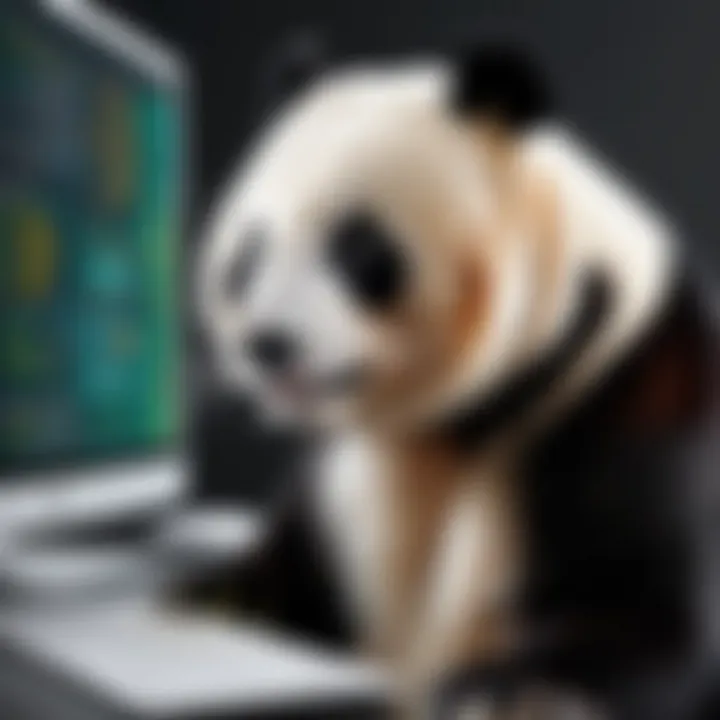
DataFrames are one of the core data structures in the Python Pandas library. Understanding what a DataFrame is, along with its structure and purpose, is essential for anyone interested in data analysis. The DataFrame provides a concise and efficient way to store and manipulate data in a tabular format, similar to a spreadsheet or SQL table. Given its versatility and power, DataFrames are invaluable for tasks like data wrangling, visualization, and statistical analysis. This section will clarify the concept of DataFrames and highlight their significance in data manipulation tasks.
Definition and Structure
A DataFrame is essentially a two-dimensional labeled data structure. It consists of rows and columns, making it approachable for users who are familiar with spreadsheet applications. The rows in a DataFrame represent observations, while the columns signify variables or features of those observations. Each column can have different data types, allowing the DataFrame to handle diverse datasets.
Typically, a DataFrame consists of:
- Rows: Each row corresponds to a single observation or data point.
- Columns: Each column represents a variable or a feature of the data. For example, in a DataFrame containing information about cars, columns could include make, model, year, and price.
- Index: The index is a unique identifier for each row, which allows for efficient data retrieval and manipulation. The index can be numerical, similar to a sequence or timestamp, or it can be set to any other variable that uniquely identifies the rows.
Here is an example of creating a simple DataFrame using Python code:
Executing this code gives a clear tabular view of data, making it intuitively easy to understand.
Key Characteristics
Several important characteristics define DataFrames, making them an attractive choice for data handling:
- Labeled Axes: Unlike traditional arrays, DataFrames use labels for rows and columns, which makes accessing and manipulating data clearer.
- Flexible Data Types: You can mix different data types within the same DataFrame. For example, one column might contain integers while another holds strings.
- Powerful Built-in Functions: Pandas offers various functions that facilitate data manipulation, such as filtering, sorting, and performing aggregate operations.
- Handling Missing Data: DataFrames come equipped with tools to identify and handle missing values, which is a common issue in real-world data sets.
- Integration with Other Libraries: DataFrames work well with libraries like NumPy for numerical operations and Matplotlib for visualization, enhancing their utility in data analysis workflows.
"DataFrames are critical for data manipulation and analysis in Python, acting as a bridge for integrating various data science processes."
Overall, grasping the definition and key characteristics of DataFrames is fundamental for anyone serious about pursuing data analysis with Pandas.
Creating a DataFrame
Creating a DataFrame is a fundamental step when working with the Pandas library in Python. The DataFrame serves as the primary structure for storing and manipulating data within the library. Understanding how to efficiently create DataFrames can greatly enhance your data analysis capabilities and workflow. The importance of this topic lies in its versatility and the various ways one can initialize a DataFrame, providing a significant advantage to those who rely on structured data.
Choosing the right method to create a DataFrame depends on the data sources available and your specific requirements for analysis. Whether gathering data from dictionaries, loading from CSV files, or creating from NumPy arrays, each method offers distinct benefits. Understanding these methods can help streamline your processes and maintain efficiency in data handling. Here, we will delve into three primary methods of initializing DataFrames in Pandas, each suited for different data contexts.
Initialization from Dict
Initializing a DataFrame from a dictionary is a simple yet effective method. In this approach, the dictionary keys typically represent column names, while the values can be lists or other data structures representing the data entries. This method is particularly useful when you already have data structured in key-value pairs.
For example:
This results in a DataFrame that organizes the data into a tabular format, making it easy to access and manipulate. Initialization from dict offers flexibility, as you can easily modify the data structure to include more columns or rows with minimal effort.
Loading from CSV
Loading a DataFrame from a CSV file is one of the most common practices in data analysis. CSV files are widely used for data storage due to their simplicity and human-readable format. The function is designed for this task, allowing you to import datasets efficiently.
For instance:
This code loads the CSV file into a DataFrame, preserving the structure of the original file. This approach is extremely useful for large datasets as it allows for quick access to data without manual entry. Additionally, parameters like , , and can be specified to customize how the data is read. Ensuring the data is correctly parsed during loading is crucial for accurate analysis.
Creating from Numpy Arrays
Creating a DataFrame from NumPy arrays offers an efficient method for users who are already working with numerical data. This method can be particularly useful when performing mathematical operations or simulations that generate structured numerical data.
To create a DataFrame from a NumPy array, you would use:
This syntax allows you to specify column names directly, enhancing readability. Creating a DataFrame in this manner is ideal when working with matrix-like data structures. Furthermore, once the DataFrame is established, Pandas' functionality can then be applied seamlessly.
In summary, the choice of method for creating a DataFrame can impact both the efficiency of your data analysis workflow and the ease of manipulation. Knowing how to initialize DataFrames effectively will allow for smoother transitions between data gathering and analysis. Understanding these techniques presents a solid foundation for leveraging the full power of Pandas in Python.
Exploring DataFrame Attributes
Understanding the attributes of a DataFrame is pivotal for leveraging its capabilities in data manipulation and analysis. Each attribute provides insight into the structure and the nature of the data held within the DataFrame. Knowing how to work with these attributes is essential for any user aiming to optimize their data workflows.
Indexing and Slicing
Indexing and slicing are fundamental operations when navigating through a DataFrame. This is how you access specific rows or columns of your data. Using loc and iloc methods in Pandas allows precise control over data selection.
- .loc is used for label-based indexing, which means you can use the row and column labels to retrieve data. For example:
df.loc[0, 'Column_name']# Grabs the first row of a specific column
The ability to slice your DataFrame enhances data manipulation tasks significantly. It’s vital for filtering data based on specific conditions or to extract subsets for further analysis.
Data Types
Understanding data types within a DataFrame is critical. Each column in a DataFrame can hold a different data type, such as integers, floats, or strings. Identifying these types - particularly if you aim to perform calculations - avoids errors and enhances performance.
You can check the data types using:
Be aware that mixing data types in a single column can lead to unexpected behavior, especially when filtering or using functions designed for specific types. Consistent data types improve analysis accuracy and reduce memory usage, which is crucial in handling large datasets.
Shape and Size
The shape and size of a DataFrame provide vital information about the dataset. The shape attribute returns a tuple representing the dimensionality of the DataFrame, offering counts of rows and columns. It can be accessed simply with:
This information allows you to quickly understand the scale of your dataset. Size, on the other hand, refers to the total number of elements, found using:
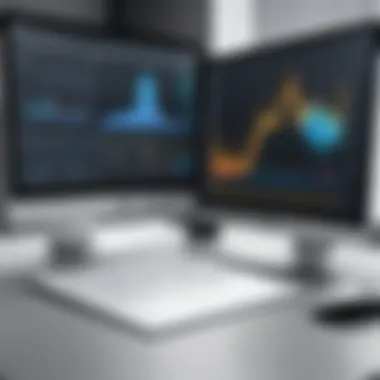
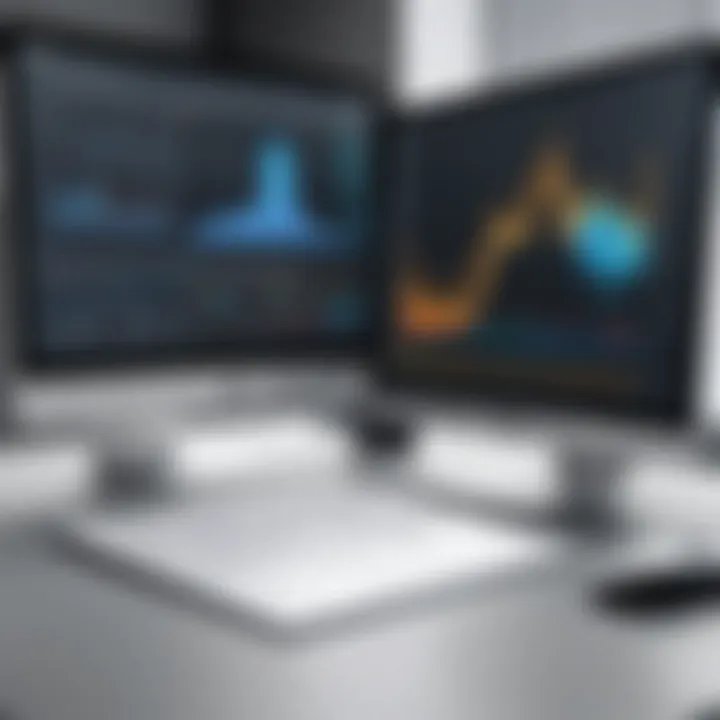
Knowing the shape and size is important for planning your data analysis strategy, whether you need to conduct operations on the entire dataset or focus on smaller segments. It sets the groundwork for understanding how to handle data effectively within the Pandas framework.
Data Manipulation Techniques
Data manipulation techniques are crucial in the context of DataFrames within the Pandas library. They allow users to efficiently manage, transform, and analyze data sets. The ability to filter, sort, and manage columns effectively is essential for data analysis tasks. When dealing with large volumes of data, these techniques enable simplification and enhanced clarity. Users can extract insights from raw data, ensure the integrity of their analyses, and save significant time.
Filtering Data
Filtering data in a DataFrame is the process of selecting rows based on specific criteria. This technique is vital for focusing on relevant information. For instance, if one is analyzing sales data, filtering can help isolate transactions from a particular region or time period.
To filter data, Pandas provides various methods. One frequently used method is boolean indexing. Users can create boolean conditions to access the rows they want. For example, if a DataFrame is named , one can filter it as follows:
Here, only the rows where sales exceed 5000 will be retained. This approach not only enhances data readability but also ensures the efficacy of decision-making processes. Furthermore, the filtering process is adaptable. Users can filter based on multiple conditions using the (and) or (or) operators.
Sorting and Reordering
Sorting and reordering data plays a significant role in data manipulation. This technique allows users to organize their data in a logical manner. It can be essential for recognizing patterns or relationships within the data. Sorting based on one or more columns helps reveal trends and discrepancies more clearly.
For example, to sort a DataFrame by sales figures in descending order, you can use the following command:
This method creates a new DataFrame sorted according to sales values. Users can also sort by multiple columns to create more complex data views. Sorting enhances the efficiency of data analysis by allowing clearer interpretations of results, ultimately guiding better business decisions.
Adding and Dropping Columns
The ability to add or drop columns is a fundamental aspect of data manipulation. Managing the structure of a DataFrame is critical to maintaining a clean and organized dataset. Users often encounter situations where they need to introduce new columns based on specific calculations or drop columns that are no longer necessary.
To add a new column, a simple assignment is often sufficient. For instance, if one needs to create a new column for profit based on sales and costs, the code would look something like this:
On the other hand, if a column is irrelevant, it can be dropped easily using the following command:
Adding and dropping columns allows for structured data that focuses on the essentials. It minimizes the complexity of DataFrames and improves data analysis. This practice leads to cleaner datasets and enhances clarity, making it easier to draw actionable insights.
Effective manipulation of DataFrames directly enhances the quality of data analysis. It transforms otherwise overwhelming datasets into manageable and insightful collections, critical for informed decision-making.
Aggregate Functions in Pandas
In data analysis, aggregate functions play a crucial role in summarizing and interpreting data efficiently. They condense large sets of data into meaningful statistics, which facilitates better insights and decision-making. Understanding aggregate functions in Python’s Pandas library is essential for anyone working with data analysis, as it presents a streamlined approach to quantitative analysis. Aggregate functions enable users to compute summaries for subsets of data, which can be instrumental in drawing conclusions based on trends and patterns.
Mean, Median, Mode
Mean, median, and mode are core statistics that serve different purposes for analyzing datasets.
- Mean is the average of all values in a dataset. It provides a sense of the overall trend but can be sensitive to outliers.
- Median is the middle value when the numbers are arranged in order. It is a better measure of central tendency when outliers may skew the data.
- Mode represents the most frequently occurring value in a dataset. It is particularly useful for categorical data or to understand common occurrences.
Using these three measures together gives a comprehensive view of the data's central characteristics. For instance, in a DataFrame containing income data, the mean may indicate general economic status, while the median may reveal income distribution fairness, and mode can show the most common salary range.
Group By Operations
Group By operations provide an effective way of aggregating data across different categories or groups. This allows for advanced analysis, such as segmenting data based on specific attributes. For example, a sales DataFrame might be grouped by region or product type to determine overall performance within those categories.
The process generally involves three steps:
- Splitting the data into groups based on certain conditions.
- Applying a function, such as sum or mean, to each group.
- Combining the results back into a DataFrame.
Example of using group by:
Through these operations, analysts can draw insightful conclusions about their data. For instance, understanding which region contributed the most to sales can influence strategic decisions for resource allocation. Aggregate functions and Group By operations form a powerful combination in the toolkit of data professionals, providing clarity and depth to complex datasets.
Handling Missing Data
In data analysis, handling missing data is crucial. Missing values can lead to distorted insights and misinformed decisions. Thus, recognizing and correctly addressing missing data becomes an essential skill for anyone working with DataFrames in Python’s Pandas library. Ignoring such values can impair the accuracy of the analysis, affect the performance of machine learning models, and lead to biased outcomes. A fundamental understanding of how to identify and manage missing values is step one toward effective data manipulation.
Identifying Missing Values
The first step in dealing with missing data is to identify where the gaps exist within your DataFrames. Pandas provides convenient tools to assist in this process. Using methods like and , one can easily pinpoint missing entries. Here’s a brief overview of how this works:
- : This method returns a DataFrame of the same shape where each cell contains a boolean value, indicating whether the original cell was missing.
- : Conversely, this method indicates the presence of data by returning True for non-missing entries.
Example usage:
Additionally, the method can provide an overall summary, including column data types and non-null count. This overview helps quickly assess the prevalence of missing data across the entire DataFrame. Such initial exploration lays the groundwork for further actions.
Imputation Techniques
After identifying missing data, one must decide how to address it. Imputation techniques refer to methods used to replace missing values with substituted data, allowing for a more complete dataset. These techniques fall broadly into three categories:
- Mean/Median/Mode Imputation: Replacing missing values with the mean, median, or mode of the respective column can be an effective method when the column contains numerical values. For example, replacing a missing entry in a numeric column with the column’s mean helps maintain overall data distribution.
- Forward/Backward Fill: This technique is used often in time-series data where it might make sense to carry forward or backward the last valid observation to replace missing values. The function in Pandas makes this straightforward:
- Interpolation: Interpolation estimates missing values based on surrounding data points. It can be particularly useful in time series data to maintain continuity.
While imputing, it is vital to consider the impact on data integrity and analysis goals. Each technique carries specific advantages and potential biases. Therefore, choosing an appropriate method depends on the data context, the nature of the missing values, and the intended analysis. Missteps in handling missing data can compromise subsequent analysis. Understanding the implications of each method is essential for accurate outcomes.
In summary, addressing missing data is not just a technical task but a critical decision-making process that influences the quality of analysis.
By effectively identifying and imputing missing values, analysts can ensure that their datasets remain robust and reliable.
Data Visualization with DataFrames
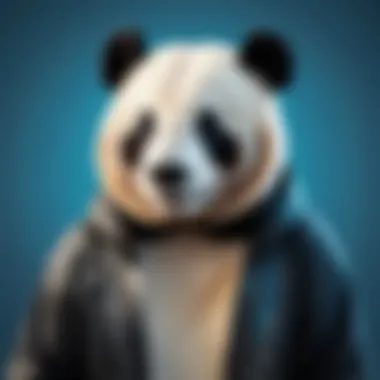
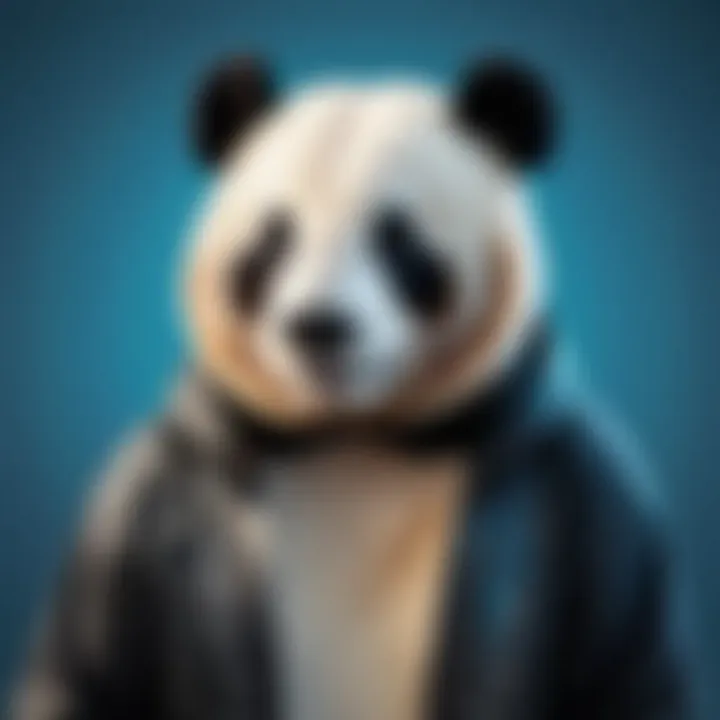
Data visualization plays a crucial role in the realm of data analysis. With the growing volume of data generated every day, clear visualization methods become vital for interpreting and communicating findings effectively. In the context of Python's Pandas DataFrames, visualization enables users to convert complex data sets into interpretable graphs and charts. This transformation helps in identifying patterns, trends, and insights that would otherwise be difficult to detect. The power of visualization is not just in presenting data but in making it understandable.
Benefits of Data Visualization:
- Simplification of Complex Data: Charts and graphs can distill dense information into digestible visuals.
- Identification of Trends: Visuals can quickly reveal trends over time, aiding decision-making processes.
- Enhanced Data Communication: A well-designed visual can convey a story or a finding more effectively than tables of numbers.
- Interactive Exploration: Tools that allow user interaction can empower deeper insights.
Visualization also requires careful consideration. Visual literacy is essential; users must understand how to interpret different types of graphs. They also need to select appropriate visual representations to avoid misconceptions. For example, bar charts are helpful when comparing quantities, while line graphs are suitable for showing trends.
Plotting Basics
To begin visualizing data within Pandas, one first needs a foundational understanding of plotting. Pandas provides a simple interface that is built on top of Matplotlib, a well-known plotting library in Python. Using Pandas, generating plots can be done in just a few lines of code, making it accessible for users at various levels.
The basic plot function in Pandas is . This function gives users an easy way to create different types of plots such as line plots, bar plots, histograms, and scatter plots. Here’s a simple example of how to create a line plot:
This code snippet will create a line graph showing the sales trend over the given year range. By tweaking parameters within , one can customize colors, line styles, and more to fit their presentation needs.
Integrating with Matplotlib
While Pandas has its own plotting capabilities, Matplotlib is an immensely powerful tool that extends these functionalities further. Integration between the two makes it possible to leverage Matplotlib's extensive feature set for creating intricate and polished visualizations.
To integrate Matplotlib with Pandas, one must first ensure that Matplotlib is installed in your Python environment. The primary advantage here is the ability to use Matplotlib functions for advanced customization. After plotting using Pandas, additional Matplotlib commands can be used to refine the visualization. For instance, adding gridlines or customizing tick labels enhances the visual. Here’s an example showing how to integrate:
In this example, one could see how additional Matplotlib features improve the visual presentation. Data visualization using Pandas DataFrames and Matplotlib thus becomes a powerful combination for understanding and showcasing data.
Exporting DataFrames
Exporting DataFrames is an essential process in data analysis, particularly when dealing with real-world applications. Understanding how to export data allows users to share information with others, integrate it into reports, or store it for future analysis. DataFrames, created with the Pandas library, are versatile and can be easily exported into various formats. The most common formats include CSV and Excel, each providing distinct advantages. In this section, we will discuss the methods and best practices for exporting DataFrames, ensuring that the data remains intact and usable for differing requirements.
Saving as CSV
CSV, which stands for Comma Separated Values, is a widely recognized format for data storage and transfer. One of the significant reasons to use CSV is its simplicity and compatibility. Most applications can open CSV files, making them ideal for data sharing.
To save a DataFrame as a CSV file in Pandas, the function is utilized. Below is a brief example of its usage:
In this example, we create a simple DataFrame with names and ages. The function converts it to a CSV file named output.csv, where the argument prevents Pandas from writing row labels. This aspect is often crucial for clarity and usability of shared data.
Benefits of Saving as CSV
- Simplicity: Easy to create and read.
- Universality: Supported by many applications.
- Lightweight: Ideal for large datasets without excessive metadata.
Exporting to Excel
Excel is another popular format for data storage, especially for users who require advanced features such as formatting or the ability to conduct complex calculations. Excel files provide better data organization and visual management compared to CSV files.
Pandas offers functionality to export DataFrames to Excel using the function. Here's an example to illustrate:
In this case, we export the same DataFrame to an Excel file named output.xlsx. Similar to CSV, the prevents adding row indices in the output file.
Key Considerations for Exporting to Excel
- Complex Capabilities: Allows formatting, charts, and formulas.
- Interactivity: Users can manipulate data directly in Excel.
- Multi-sheet Support: Can organize related DataFrames into one file with multiple sheets.
Through using these methods, one can effectively manage data export from Pandas DataFrames. Being able to export data seamlessly to formats like CSV and Excel is crucial for anyone engaged in data analysis. This knowledge not only promotes better data sharing but also enhances collaborative work across disciplines.
Best Practices for Working with DataFrames
Pandas DataFrames are powerful tools used in data analysis. To harness their potential fully, following best practices is crucial. These practices not only enhance the performance of your code but also ensure clarity and maintainability. For students, professionals, and those learning programming, understanding these practices can make a significant difference in the way data is handled and interpreted.
Performance Optimization Techniques
Optimizing performance when working with DataFrames is essential, particularly with larger datasets. Here are key techniques to consider:
- Use Vectorized Operations: Pandas is designed to work efficiently with its built-in operations. When possible, use these operations instead of traditional for-loops. For example, instead of iterating through rows, apply a function directly to a DataFrame.
- Leverage Built-in Methods: Functions like , , and are optimized for performance. Utilize them to perform complex data transformations efficiently.
- Consider Data Types: Be mindful of your data types. Using appropriate types, such as categoricals for text data, can lead to significant performance improvements.
- Memory Usage: Use to understand how much memory your DataFrame is using. This can help pinpoint inefficiencies and optimize the DataFrame’s structure.
Optimizing how you interact with Pandas DataFrames not just speeds up your computations but also prepares your projects for scaling in future.
Maintaining Clean Data
Clean data is the foundation for reliable analysis. Poor quality data can lead to incorrect conclusions. Here are practices to maintain cleanliness in DataFrames:
- Handle Missing Values: Identify missing values using methods such as or . Use techniques like imputation or dropping rows/columns based on your analysis needs.
- Consistent Formatting: Ensure that all textual data is consistently formatted. For example, unify cases (upper/lower) and remove extraneous white spaces.
- Validate Data Entries: Implement checks to validate data entries against known rules or formats. This can help catch anomalies early in the data processing pipeline.
- Document Changes: Keep track of any modifications made to the data. Documenting transformations clearly can help maintain integrity throughout the data analysis process.
By adhering to these practices, you not only improve the efficiency of your data operations but also enhance the reliability of the outcomes derived from your analyses. Maintaining a clean and optimized DataFrame is a road to insightful data analysis.
The End
In this section, we will underscore the importance of the conclusion in the broader context of the article. A well-crafted conclusion is not simply an end; it acts as the final argument underscoring the value and functioning of DataFrames within the Python Pandas library. It should instill a sense of closure while providing meaningful insights into the application of the content discussed.
The recap of key concepts facilitates a revision of essential knowledge. It reinforces what readers have learned about creating, manipulating, and optimizing DataFrames. The use of these techniques serves as groundwork for further explorations in the field of data analysis.
Moreover, considering future directions in data analysis opens up discussions about emerging trends and innovative practices. Readers can gain insights into how developments in technology influence data management and manipulation. This anticipatory view encourages ongoing learning, which is crucial in the ever-evolving field of data science.
In essence, the conclusion not only ties together the various sections of the article but also serves as a catalyst for further exploration and growth in the readers' understanding of Python Pandas and its application in real-world scenarios.
Recap of Key Concepts
Throughout this article, we explored numerous key concepts inherent to DataFrames in Python Pandas. Some of the highlights include:
- Definition and structure of a DataFrame.
- Creation techniques, including initializing from dictionaries and loading from CSV.
- Manipulation techniques such as filtering and sorting.
- Handling of missing data, ensuring that datasets remain robust.
- Visualization and exporting methods to enhance data interpretation and utility.
A solid grasp of these concepts lays the foundation for employing DataFrames effectively in various data tasks. Each element plays a vital role, equipping readers to tackle complex data analysis challenges with confidence.
Future Directions in Data Analysis
Looking ahead, the future of data analysis shows much promise. Several key developments may impact how we view DataFrames and data manipulation in general:
- Increased integration with artificial intelligence: This can enhance analytical capabilities.
- Real-time data processing: The demand for instant analysis requires continuous advancement in DataFrame functionalities.
- Emphasis on automation: Simplifying repetitive tasks through advanced libraries and features can save time and enhance productivity.
- Focus on data ethics and governance: As analysis becomes more widespread, understanding ethical implications remains fundamental.
By keeping abreast of these and other trends, readers can better prepare themselves for upcoming challenges and opportunities in the realm of data analysis. Continuous learning and adaptation ensure resilience in a field characterized by rapid changes.