Mastering Domain Class Diagram Makers for Software Development
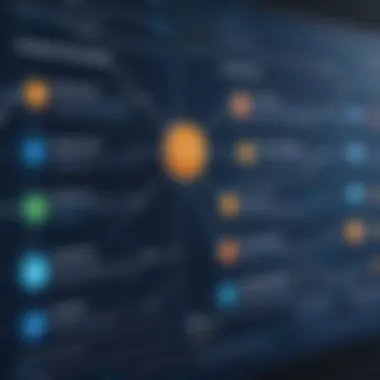
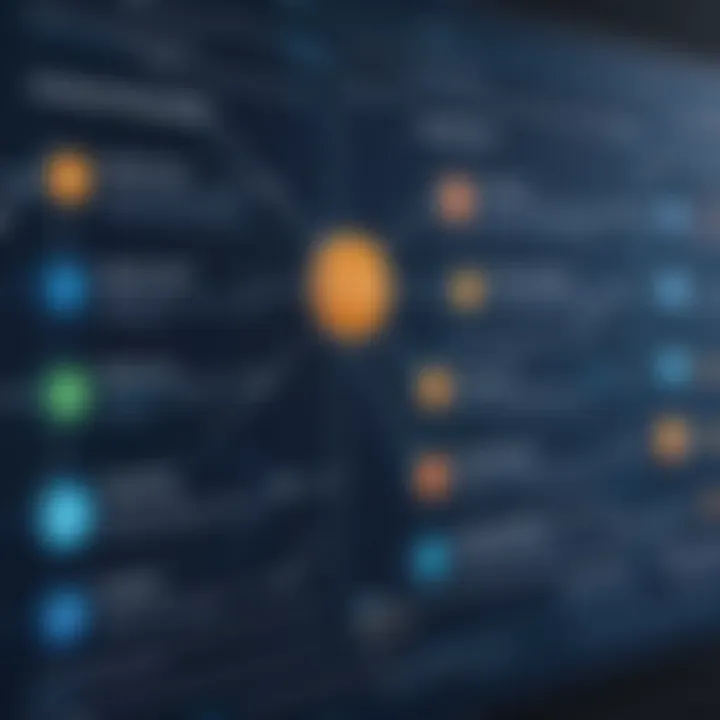
Overview of Topic
Preamble to the main concept covered
A domain class diagram is a visual representation of the classes in a system, revealing the relationships and constraints that exist within the domain of the application being developed. This type of diagram focuses on the semantics of the domain rather than its implementation. Understanding how to create effective domain class diagrams can greatly improve communication and understanding among stakeholders, developers, and designers.
Scope and significance in the tech industry
In the fast-paced tech industry, clear communication is essential. Domain class diagrams serve as a bridge between business requirements and technical design. They assist in modeling complex systems and facilitate better project management by providing a clear view of the system's structure.
Brief history and evolution
Domain modeling has its roots in object-oriented design. Over the years, as software development methodologies have evolved from structured programming to agile practices, the importance of blueprints such as domain class diagrams has grown. Tools for creating these diagrams have also advanced, moving from simple pencil-and-paper sketches to sophisticated software applications that allow for collaboration and integration with other development tools.
Fundamentals Explained
Core principles and theories related to the topic
The concept of domain modeling is founded on the idea of representing real-world entities and their interactions within a software environment. Each class in a domain class diagram represents a real-world concept with attributes and behaviors. Understanding entities, attributes, associations, and multiplicities are foundational to creating meaningful diagrams.
Key terminology and definitions
- Class: A template for creating objects, defining attributes and methods.
- Object: An instance of a class.
- Attribute: A property or characteristic of a class.
- Association: A link between classes indicating a relationship.
- Multiplicity: Describes how many instances of a class relate to instances of another class.
Basic concepts and foundational knowledge
Having a firm grasp of UML (Unified Modeling Language) is crucial in understanding domain class diagrams. It provides a standard way to visualize system design and simplifies communication between team members. Familiarity with basic principles of object-oriented programming also enhances the effectiveness of modeling with domain class diagrams.
Practical Applications and Examples
Real-world case studies and applications
Businesses from various sectors utilize domain class diagrams to model their systems. For instance, an e-commerce platform may represent classes for products, users, and orders. This helps design the structure and interactions within their software more efficiently.
Demonstrations and hands-on projects
Using a tool like Lucidchart, users can create a simple domain class diagram by identifying key classes and their relationships. Begin by listing core entities and then establish how these entities interact with each other through associations.
Code snippets and implementation guidelines
While the diagrams themselves are not code, they inform the coding process. Here's how a class might be represented in code after diagramming:
Advanced Topics and Latest Trends
Cutting-edge developments in the field
Modern software development emphasizes agility and iterative design. Domain-driven design (DDD) is a trending approach that enhances domain modeling through collaboration between technical and domain experts. Technologies like microservices also affect how diagrams are structured, as systems become more decentralized.
Advanced techniques and methodologies
Techniques such as event storming allow teams to generate domain models collaboratively. This is a workshop format where users discuss domain events and derive models from them. Using version control systems for diagrams is also gaining traction, expanding collaborative workflows.
Future prospects and upcoming trends
As artificial intelligence integrates with development tools and platforms, automated diagram generation might become more prevalent. These advancements could lead to more effective documentation and enhanced understanding of complex systems as they develop.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
- Books: "Domain-Driven Design" by Eric Evans.
- Courses: Relevant courses on platforms like Coursera or Udacity that focus on UML and software modeling.
- Websites: En.wikipedia.org and britannica.com provide valuable overviews and insights into UML.
Tools and software for practical usage
Many tools are available for creating domain class diagrams. Some notable ones include
- Visual Paradigm
- StarUML
- LucidChart
These tools simplify the process of creating and sharing diagrams, enhancing team collaboration.
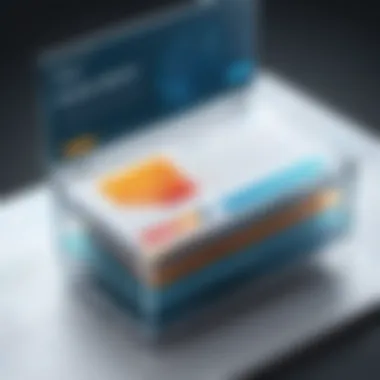
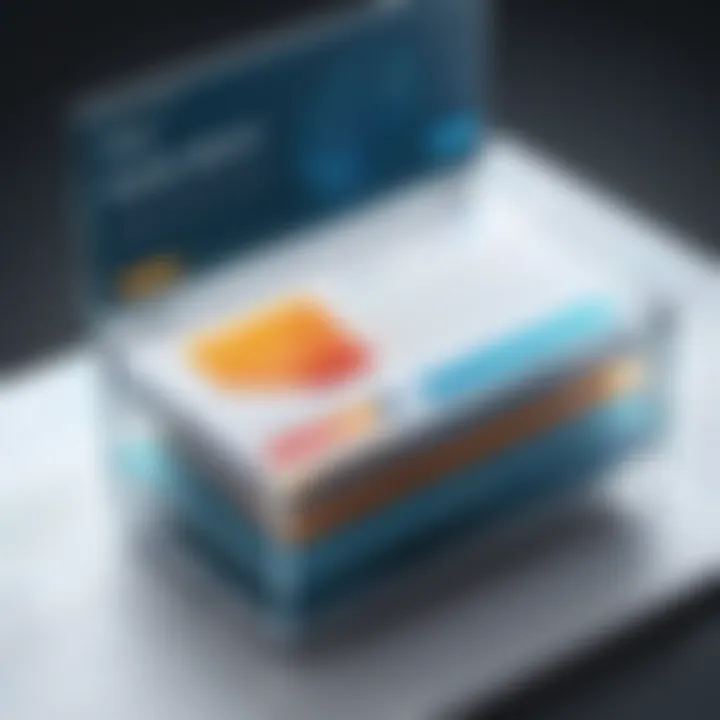
Understanding Domain Class Diagrams
Understanding domain class diagrams is vital for anyone involved in software development. These diagrams provide a structured representation of the system’s entities, their attributes, and the relationships that exist between them. This section aims to highlight why domain class diagrams are an essential skill for developers, architects, and stakeholders, offering insights into their key benefits and considerations.
Effective diagramming can majorly influence project outcomes. The primary purpose of a domain class diagram is to clarify requirements, helping stakeholders visualize the system's design and functionality. This reduces misunderstandings early in the development process, ultimately resulting in time and resource savings. Thus, familiarity with these diagrams enhances not only individual competency but also team collaboration.
Definition of Domain Class Diagrams
A domain class diagram is a type of static structure diagram that describes the structure of a system by showing its classes, attributes, and the relationships among those classes. Think of it as a blueprint that provides insight into how different parts of the software interact. Classes represent entities, while attributes define the characteristics of those entities. Relationships, such as associations, aggregations, and compositions, illustrate how classes connect.
This model serves as a foundation for further design, enabling developers to conceptualize the system without getting bogged down in technical details. According to Wikipedia, these diagrams are often utilized in the context of Object-Oriented Design, making them a crucial aspect of modern software development methodologies.
Importance in Software Development
The significance of domain class diagrams in software development cannot be overstated. They facilitate communication among various stakeholders, including software developers, project managers, and clients. Without a common visual language, it can be challenging to ensure that all parties share the same understanding of system requirements and design.
Benefits of Using Domain Class Diagrams:
- Visualization: Helps to make abstract concepts more concrete.
- Clarity: Reduces ambiguity regarding system design and architecture.
- Documentation: Serves as a reference point throughout the project lifecycle.
- Efficiency: Saves time in the development process by identifying issues early.
Moreover, maintaining a focus on domain class diagrams can lead to better software architecture and design practices. They ensure that the development process aligns with business objectives, fulfilling the needs of end-users efficiently.
In the field of software development, the ability to create and interpret domain class diagrams fosters a robust developmental framework. This strength can distinguish effective teams from less effective ones in a highly competitive landscape.
Core Concepts of Domain Class Diagrams
Understanding the core concepts of domain class diagrams is essential for anyone involved in modeling software systems. These concepts serve as the foundation for creating effective diagrams that not only convey information clearly but also assist in the development process.
Classes and Objects
Classes represent the blueprint for objects in a domain class diagram. They encapsulate attributes and methods relevant to the entities they represent. Objects, in turn, are instances of these classes, holding specific data and exhibiting behavior defined by their classes. This relationship between classes and objects is fundamental.
The significance of distinguishing between classes and objects cannot be understated. It allows developers to abstractly design their systems before diving into coding. For example, a class named could have various attributes, such as , , and . An object of might carry specific values, like , , and . By forming this clear separation, system complexity can be managed efficiently.
Moreover, classes can demonstrate inheritance, where one class derives from another, thus promoting code reuse. This concept also allows for polymorphism, where objects of different classes can be treated as objects of a common superclass. These principles enhance modularity and flexibility in software design.
Attributes and Methods
In domain class diagrams, attributes are characteristics of a class. They describe the properties of an object. For instance, in our class, relevant attributes may include , , and . Each attribute holds significant weight as it defines the state of its object.
On the other hand, methods represent the functionality provided by a class. They dictate what actions can be performed on the objects of that class. Continuing with the example, methods might include , , or . This delineation between attributes and methods aids in developing a clear understanding of the object-oriented design.
Understanding how to define attributes and methods is crucial not only for diagram makers but also for programmers. It allows for a more structured codebase and fosters easier maintenance. Properly documenting these components helps others in the team understand the functionality and intent of specific classes.
Relationships Between Classes
The interplay between classes is expressed through relationships in domain class diagrams. There are several types of relationships such as association, aggregation, and composition. Each type signifies how classes interact with each other.
- Association indicates a relationship where objects of one class are connected to objects of another. For example, a class can be associated with a class, indicating that a driver operates a car.
- Aggregation is a specialized form of association where a class can contain objects of another class, but those objects can exist independently. For instance, a can hold multiple , but the cars can exist without the garage.
- Composition, in contrast, signifies a strong relationship where the contained object cannot exist without its container. If the is deleted, all contained should also be deleted.
Understanding these relationships is imperative for anyone aiming to create insightful domain class diagrams. Taking the time to correctly map out how classes interact not only leads to clearer diagrams but also a more robust software architecture.
"A clear understanding of core concepts simplifies complex software design processes and encourages best practices in development."
By grasping these key aspects of classes, attributes, methods, and their relationships, you establish a solid starting point for effective domain modeling. As such, being adept in these concepts equips developers with enhanced agility in both design and implementation phases.
Tools for Creating Domain Class Diagrams
The creation of domain class diagrams is pivotal in the realm of software development. A quality diagram not only depicts the structure of a system but also elucidates how different components interact. Selecting the right tools is essential for achieving clarity and efficiency in this process. The tools available can offer varying features that cater to different needs and preferences, making it crucial to understand which ones to consider.
Overview of Popular Tools
There is a multitude of tools available for crafting domain class diagrams. A few notable options include:
- Lucidchart: This is a versatile diagramming application that supports real-time collaboration. It is user-friendly and offers a wide range of templates.
- Microsoft Visio: Known for its robust design functionalities, Visio is often favored by enterprises for its comprehensive features.
- Draw.io: A free-to-use platform, Draw.io allows easy diagram customization and is easily integrated with cloud storage services.
- StarUML: This tool is an open-source solution, providing support for various UML diagrams, including class diagrams. It offers a sleek interface tailored for software developers.
- PlantUML: A unique tool that uses plain text to create diagrams. This option appeals to programmers who prefer a code-based approach to diagramming.
Each of these tools has its pros and cons, making it essential to evaluate them against specific project requirements.
Features to Look for in Diagram Makers
When choosing a diagram maker, several features can significantly enhance your experience:
- User Interface: A clean and intuitive interface aids in reducing the learning curve, allowing users to focus on design rather than navigation.
- Collaboration Tools: For teams, the ability to collaborate in real-time is invaluable. Look for tools that enable multiple stakeholders to contribute simultaneously.
- Export Options: The capability to export diagrams in various formats (like PNG, SVG, or PDF) ensures that your work can be shared or integrated into presentations easily.
- Integration Capabilities: Tools that work well with other software development tools (e.g., GitHub, Jira) can enhance productivity by creating a seamless workflow.
- Template Library: A rich library of templates enables quicker diagram creation for common structures, saving time and effort.
Comparison of Leading Diagram Tools
To provide a clearer perspective, here is a brief comparison of the aforementioned tools based on key criteria:
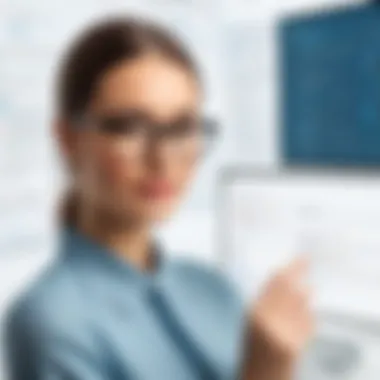
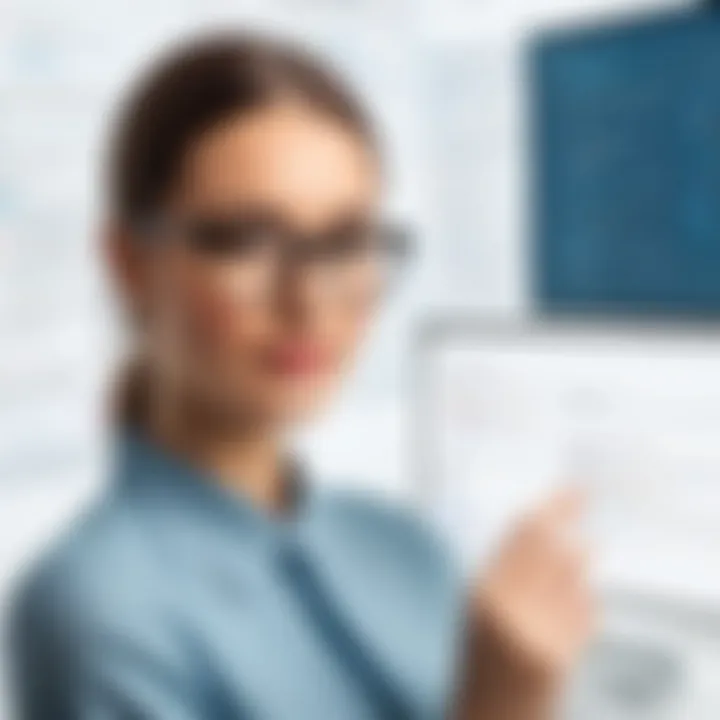
| Tool | Collaboration | User Interface | Price | Template Variety | | Lucidchart | Yes | Intuitive | Free with limitations | High | | Microsoft Visio | Yes | Comprehensive | Paid | Medium | | Draw.io | Yes | Simple | Free | Medium | | StarUML | No | User-friendly | Open Source | Low | | PlantUML | No | Code-based | Free | Low |
In summary, the ideal tool for creating domain class diagrams depends on the user’s specific needs, project constraints, and personal preferences. Taking the time to research and experiment with different options can lead to improved diagram quality and software development workflow.
Best Practices for Designing Domain Class Diagrams
Designing effective domain class diagrams is essential in software development. Best practices in this area can significantly enhance the clarity and utility of the diagrams. These practices ensure that diagrams are not only functional but also easy to understand. They serve as a guide for developers and stakeholders alike. In this section, we will explore three key practices: clarity and simplicity, modularity in design, and maintaining consistency.
Clarity and Simplicity
Clarity is paramount in any design work, especially in domain class diagrams. A clear diagram conveys information without unnecessary complexity. Simplicity allows stakeholders to understand the system at a glance. To achieve clarity, the following points should be considered:
- Avoid using too many classes and relationships. Instead, focus on the essential elements.
- Use succinct labels for classes and attributes. This minimizes potential confusion.
- Organize the diagram layout logically. Group related classes together for better comprehension.
In addition, removing extraneous details can simplify the diagram and make it more reader-friendly. Thus, clarity and simplicity are key to crafting effective domain class diagrams.
Modularity in Design
Modularity refers to breaking down a system into smaller, manageable parts. In the context of domain class diagrams, it means designing classes that can stand alone while still functioning within the larger system. This practice provides several benefits:
- Ease of Updates: Modifications in one module do not necessitate changes across the entire diagram.
- Reusability: Independent classes can be reused in different parts of the application or in future projects.
- Improved Focus: Designers can concentrate on specific features without losing sight of the overall architecture.
When designing for modularity, ensure that each class has a single responsibility. This practice helps maintain a clear structure and paves the way for scalable applications.
Maintaining Consistency
Consistency across diagrams fosters better communication among team members. Maintaining a standard notation and style reduces ambiguity. Key aspects include:
- Use of standard UML notations throughout the diagram. This avoids misinterpretation.
- Consistent naming conventions for classes, variables, and methods. This reduces cognitive load when reviewing diagrams.
- Adhering to a uniform layout style. This makes it easier to navigate and comprehend the diagram quickly.
By applying these principles, teams can enhance collaboration. Consistency allows for smooth transitions between different phases of software development, leading to more efficient project execution.
In summary, best practices in designing domain class diagrams significantly impact software development efficiency and communication. By prioritizing clarity, modularity, and consistency, designers create valuable tools that aid in understanding complex systems.
Role of Domain Class Diagrams in Software Architecture
Domain class diagrams serve as a foundational component in the realm of software architecture. Their primary purpose is to provide a clear visual representation of a system's structure, showcasing the various classes that will make up the software. This enables developers to effectively manage complexity, ensuring all components align with the intended requirements.
These diagrams help bridge the gap between abstract business requirements and concrete implementation. They illustrate how different classes relate to one another, which is crucial in identifying potential challenges early in the development process. By establishing this clear framework, domain class diagrams contribute significantly to the robustness of software design.
Effective use of domain class diagrams can lead to improved software quality and maintainability.
Linking Requirements to Implementation
Linking requirements to implementation is a vital aspect of software development, and domain class diagrams play an integral role in this process. At the onset of a project, stakeholders delineate their needs. It is the diagram that transforms these abstract ideas into systematic structures that developers can implement.
The diagram visually represents how business requirements map to actual classes and their interactions. Each class can reflect specific functionalities stipulated by requirements, showcasing attributes and methods pertinent to those needs. This alignment not only simplifies the implementation process but also validates that all requirements are accounted for.
Moreover, establishing this link enhances traceability. If changes in requirements occur, developers can quickly adjust the diagrams to ensure the implementation remains relevant. This adaptability is essential in today’s fast-paced development environments, where project scopes can evolve rapidly.
Facilitating Communication Among Stakeholders
Effective communication is a cornerstone in successful software development, and domain class diagrams are pivotal in this regard. They serve as a universal language that can be understood by both technical and non-technical stakeholders.
These diagrams facilitate discussions among team members, business analysts, and clients. They help clarify misunderstandings regarding system requirements, functionalities, and designs. By referencing a common visual tool, stakeholders can provide input, identify inconsistencies, and ensure everyone shares the same vision for the project.
In addition, domain class diagrams can act as training tools for new team members. The clarity and structure of these diagrams can quickly familiarize them with the existing architecture, reducing the learning curve. Thus, fostering a better collaborative environment where ideas can be exchanged freely and effectively.
Challenges in Creating Domain Class Diagrams
Creating domain class diagrams is essential for effective software development. However, this process comes with inherent challenges that can undermine the effectiveness of the diagrams. Understanding these challenges helps in overcoming obstacles and ensuring that the diagrams serve their intended purpose. This section explores two prominent challenges: overcomplicating designs and neglecting updates during development.
Overcomplicating Designs
One of the foremost issues in the creation of domain class diagrams is overcomplication. Developers often feel the need to represent every detail in a single diagram, which can lead to intricate designs that are difficult to comprehend. An overly complex diagram can obscure its foundational elements, making it challenging for stakeholders to grasp the system's structure or for new team members to onboard effectively.
To mitigate this issue, a more streamlined approach should be taken. Here are some strategies that can help:
- Focus on Core Concepts: Identify the key classes and relationships that form the backbone of the system. Avoid adding unnecessary classes that do not contribute to the primary objective.
- Limit Attributes: Include only essential attributes in each class. This simplifies the diagram, allowing more focus on the class interactions.
- Use Packages: Consider grouping related classes into packages. This encapsulation not only reduces clutter but also organizes the diagram logically.
By prioritizing simplicity and clarity, the design becomes more effective in conveying critical information.
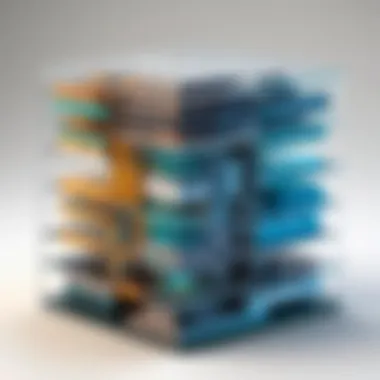
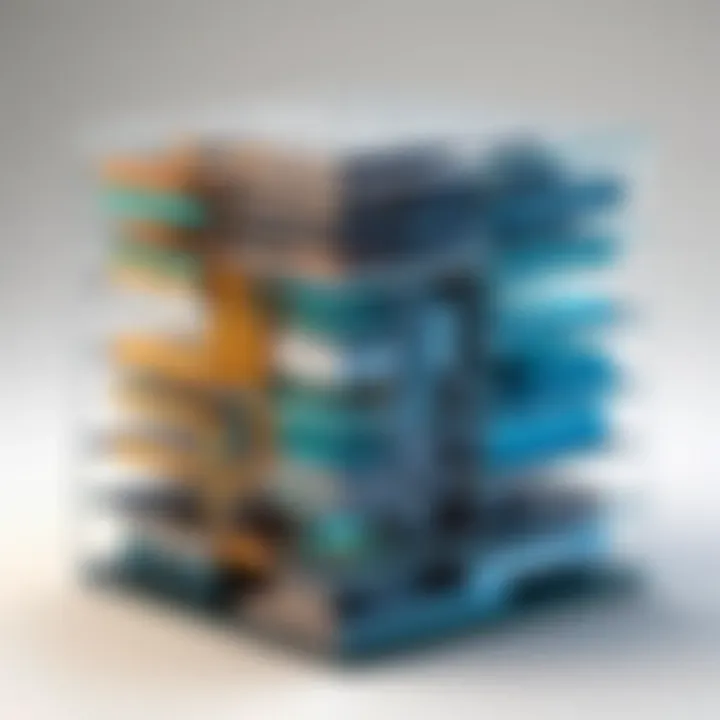
Neglecting Updates During Development
Another common challenge faced during the creation of domain class diagrams is the neglect of updates as the development progresses. Software projects often evolve, with requirements shifting and new details emerging. If the diagrams are not revised to reflect these changes, they risk becoming outdated and irrelevant. Outdated diagrams can lead to misunderstandings among team members and other stakeholders, which can affect project timelines and outcomes.
To address this challenge, consider the following actions:
- Regular Reviews: Schedule periodic reviews of the domain class diagrams. This practice ensures that the diagrams align with the current state of the project.
- Version Control: Implement a version control system for diagrams, akin to what is used with code. This keeps track of changes and ensures that everyone references the most current version.
- Collaboration Tools: Utilize tools that facilitate easy updates and allow for multiple team members to contribute simultaneously. This can help in keeping the diagrams updated while involving diverse perspectives.
Regular updates are crucial to ensure that domain class diagrams remain a relevant tool for communication and reference throughout the project lifecycle.
Addressing these challenges requires mindful planning and execution. Recognizing the importance of simplicity and regular updates can significantly enhance the utility of domain class diagrams.
Case Studies on Effective Use of Domain Class Diagrams
Domain class diagrams play a pivotal role in software development, transforming abstract ideas into structured concepts. This section explores the importance of case studies that highlight effective applications of domain class diagrams. Real-world examples allow us to grasp specific elements and benefits associated with their usage.
Real-World Applications
Examining case studies provides practical insights into how domain class diagrams facilitate the design process. For instance, consider the development of an e-commerce platform. Designers used domain class diagrams to accurately define relationships between various components such as products, customers, and orders. By visualizing these elements, teams could identify potential challenges. They improved upon the initial architecture swiftly, saving both time and resources.
Another example can be seen in the healthcare management system. Here, a clear representation of classes and their interactions helped ensure that critical data flows correctly between users, such as doctors and patients. Diagram makers allowed the project team to align the software functionalities with both user requirements and system constraints effectively.
Case studies enhance understanding of domain class diagrams by showcasing real-world implications, allowing developers to learn and adapt best practices.
Lessons Learned and Insights
From the aforementioned applications, several lessons emerge. For one, clarity in design is essential. Domain class diagrams that are overly complex lead to confusion among team members and stakeholders. Simplifying the diagrams can often make the relationships clearer and can enhance communication.
Moreover, stakeholder collaboration is crucial. Engaging different groups in discussions about the domain structure can lead to deeper insights. For instance, involving users during the design stages helps in capturing their requirements better, resulting in a more user-centered product.
- Regular update of diagrams helps in maintaining their relevance throughout the development life cycle.
- Keeping documentation aligned with domain class diagrams ensures that everyone has a shared understanding.
The Future of Domain Class Diagram Makers
The landscape of software development is continuously evolving. As businesses seek to become more agile and efficient, the tools that support software engineering must also adapt and improve. Domain class diagram makers are essential tools in this context, enabling developers to visualize system architecture, relationships, and functionalities in a clear manner. The future of these tools promises significant advancements, allowing teams to leverage increased capabilities and enhanced integrations.
Trends in Software Development
Recent years have ushered in several key trends in software development that will shape the future of domain class diagram makers. One of the most prominent trends is the shift towards agile methodologies. Agile development emphasizes iterative progress through small, manageable increments. Diagram makers will need to provide tools that support rapid changes and simplifications. This means offering features like drag-and-drop elements, easy adjustments to class relationships, and real-time collaboration tools.
Another significant trend is the adoption of DevOps practices. This integration of development and operations demands that software delivery is more streamlined and efficient. Domain class diagrams will be crucial in bridging gaps between teams. Enhanced features that facilitate sharing and accessing diagrams across various platforms will likely emerge. This would enable quicker feedback loops, ultimately leading to a higher quality of software.
Moreover, with the rise of microservices architecture, developers require ways to depict services and their interactions more effectively. Diagram makers must evolve to visualize these complex architectures, supporting not just individual classes but the overarching interaction patterns between services. Developers will look for tools that accommodate these changes in architecture seamlessly.
Integration with Other Technologies
The future of domain class diagram makers will also see an increased emphasis on integration with other technologies. This integration can enhance the overall efficiency and utility of these tools. For instance, integrating with cloud-based platforms can allow diagrams to become part of larger collaborative workflows. Members from different teams can access live updates, promoting a shared understanding of the project’s architecture.
Artificial Intelligence (AI) is another area that presents transformative opportunities for diagram makers. By incorporating AI, these tools can analyze existing diagrams and provide suggestions for improvements or identify potential issues in class relationships. This can save developers significant time and improve the accuracy of their designs.
Moreover, integration with API management tools allows for better alignment between documented diagrams and the actual implemented codebase. Teams can automatically generate diagrams from code, reducing manual work and ensuring that all stakeholders have the most up-to-date visualizations.
"The integration of domain class diagram makers with emerging technologies is not just beneficial; it is critical for staying competitive in software development."
Overall, the future of domain class diagram makers appears promising, with innovations aligning closely with the latest trends in software development and technology integration. It is likely that these tools will not only remain relevant but will also become indispensable in the software engineering landscape.
Closure
The conclusion serves as a vital section of this article, encapsulating the essence of what has been discussed regarding domain class diagram makers. It is not merely a summary; rather, it provides a platform for readers to reflect on the significance and applicability of the topic in real-world scenarios of software development.
Summarizing Key Takeaways
In this guide, key points have emerged that are crucial for understanding domain class diagrams:
- Definition and purpose: Domain class diagrams clarify the structure of a software system.
- Importance: They connect requirements to design, enhancing communication among stakeholders.
- Tools and techniques: Various tools like Lucidchart and Visual Paradigm provide essential features for effective diagram making.
- Best practices: Simplicity and modularity in design improve the usability and maintainability of diagrams.
- Challenges: Recognizing and addressing complexities can enhance the efficacy of domain class diagrams.
These takeaways highlight the fundamental role of domain class diagrams in bridging the gap between software requirements and implementation.
Encouraging Best Practices in Usage
Encouraging best practices in using domain class diagrams is paramount for achieving optimal results. Considerations include:
- Clarity: Create diagrams that are easy to understand, avoiding unnecessary complexity.
- Frequent Updates: Keep diagrams current with ongoing development, preventing discrepancies.
- Stakeholder Involvement: Engage team members to enhance comprehension and discover potential issues early in the design process.
By adhering to these practices, developers can maximize the effectiveness of their domain class diagrams, leading to improved software architecture and overall project success.
"Effective design is not about what's cool or trendy. It's about what's functional and clear."