Mastering Express Applications: A Complete Guide
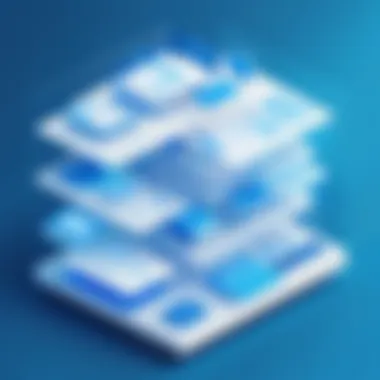
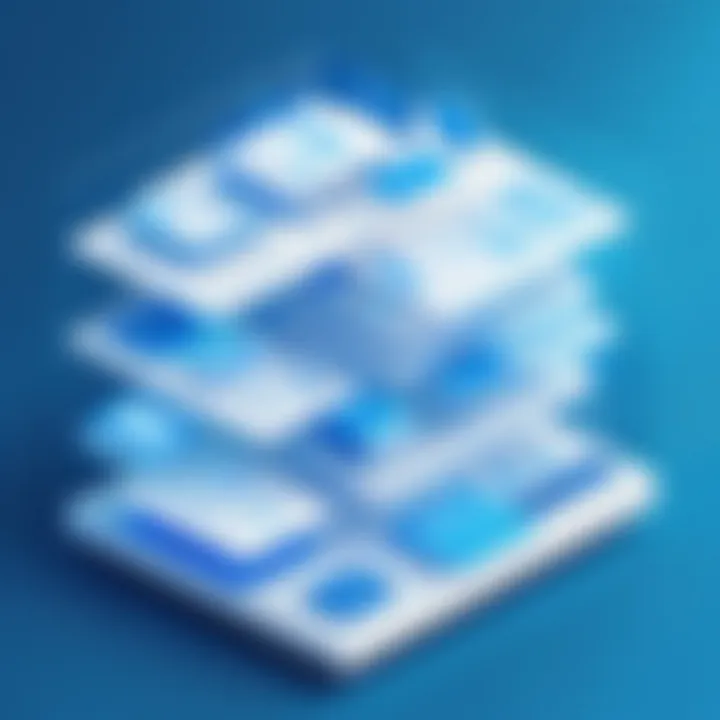
Overview of Topic
Understanding Express applications is fundamental for anyone delving into web development with Node.js. Express is a minimalist framework that provides the essential tools for building robust web applications. Its significance in the tech industry is notable, especially considering the increasing demand for responsive and scalable web solutions.
Express is not just a framework; it is an ecosystem, bridging the gap between raw Node.js capabilities and the intricate requirements of modern web applications. It simplifies the development process, enabling developers to focus on their applicationโs core functionality rather than boilerplate code.
Brief History and Evolution
Express was created by TJ Holowaychuk and released in 2010. Over time, it has undergone various updates to meet evolving web standards and user needs. Its popularity has led to a vibrant community, providing a wealth of middleware and plugins to enhance its functionality. Today, Express remains a cornerstone for many developers, powering countless applications across different domains.
Fundamentals Explained
The core principles of Express revolve around simplicity, flexibility, and performance. Understanding these principles is crucial for maximizing the framework's potential.
Key Terminology and Definitions
- Middleware: Functions that execute during the request-response cycle, enabling developers to add custom functionality at various stages of a request.
- Routing: The mechanism by which incoming requests are matched to corresponding handler functions.
- Request and Response Objects: Core objects in Express that facilitate communication between the client and server.
A foundational knowledge of these concepts allows developers to effectively navigate and utilize the framework's capabilities.
Practical Applications and Examples
Express offers extensive practical applications. To illustrate, consider building a simple RESTful API for managing tasks. This provides a real-world scenario to understand how Express facilitates web application development.
This code snippet demonstrates setting up a basic server and defining a route. The practical implementation helps solidify theoretical aspects.
Advanced Topics and Latest Trends
In recent years, advancements in Express continue to evolve. With features like asynchronous middleware and improved error handling, Express remains relevant. Understanding these advanced techniques can elevate a developer's skill set.
Future Prospects
As web technologies advance, so does the framework itself. The integration of modern JavaScript features, such as async/await, enhances the ability to write cleaner and more efficient code. Keeping abreast of these trends is essential.
Tips and Resources for Further Learning
To further your knowledge, consider diving into dedicated resources:
- Books: "Express in Action" by Evan Hahn.
- Courses: Platforms like Codecademy offer interactive tutorials.
- Online Resources: Websites like Wikipedia and Reddit provide forums for discussion and learning.
Taking advantage of these materials will support continuous learning and skill improvement in Express application development.
Prelude to Express Application Framework
In the landscape of web development, understanding the Express application framework is vital. Express serves as a minimalist framework for Node.js, providing essential features to build web applications efficiently. Its design allows developers to create robust server-side applications that can handle a variety of tasks. This article will explore the framework's architecture, middleware capabilities, routing, and much more. As developers increasingly seek to streamline their applications, being familiar with Express becomes not just beneficial but necessary.
Overview of Express Framework
Express.js is a web application framework designed for Node.js. Its primary goal is to simplify the process of building web applications by providing a set of tools and best practices. Notably, it enables the creation of dynamic web applications with ease due to its minimalistic approach. Express encapsulates the complexities of handling HTTP requests and responses, making it easier for developers to focus on functionality rather than boilerplate code.
Developers can define routes, manage middleware, and handle requests without delving deeply into the underlying HTTP protocol. Express provides methods to create APIs and render views, thus making it versatile. The flexibility of Express allows it to be used for anything from small projects to large-scale enterprise applications.
Significance in Web Development
The significance of Express in web development cannot be understated. It has gained popularity for several reasons:
- Simplicity: With its straightforward and unopinionated structure, Express allows developers to start quickly and be productive from the get-go.
- Middleware Support: Express utilizes middleware to handle requests, allowing seamless integration of additional functionality, such as authentication or error handling.
- Community and Ecosystem: A vibrant community supports Express, resulting in a rich ecosystem of plugins and middleware, enhancing its capabilities.
- Performance: Express is designed to be lightweight, enabling great performance in handling multiple connections concurrently.
- Flexibility: Developers can structure their applications as they see fit, using either a RESTful approach or more traditional web-server methods.
"Express.js empowers developers to build applications with minimal friction, allowing them to focus on building core features without the overhead of complex frameworks."
Core Concepts of Express
Understanding the core concepts of Express is essential for developers aiming to build effective web applications. The framework's architecture integrates well with JavaScript, making it an ideal choice for developers familiar with the language. By grasping these concepts, readers will unlock the full potential of Express, allowing for more efficient development and better application performance.
Understanding Middleware
Defining Middleware
Middleware is a key feature in Express. It acts as a bridge between the HTTP request and the response. In simple terms, it processes requests before reaching the final route handler. One significant characteristic is its modularity. This allows developers to use third-party middleware, or create their own to tailor the applicationโs behavior. The benefits of using middleware include improved code organization and functionality, which contribute significantly to the robustness of applications.
Common Use Cases
Middleware can be leveraged in numerous ways. It handles tasks like authentication, logging, and error processing efficiently. Each of these tasks is vital for any web application, as they help manage user requests effectively. The key feature here is the ability to stack multiple middleware functions. This stacking allows for streamlined processing of requests in a clear order. However, managing many middleware components can complicate debugging if not documented properly.
Creating Custom Middleware
Creating custom middleware offers flexibility for specific application needs. Developers can write middleware to parse request data, validate user input, or even manage sessions. One important aspect of custom middleware is that it can focus on a single responsibility, keeping the code modular. The advantage of this custom approach is the enhancement of application functionality without affecting the overall architecture. However, it demands a good understanding of the middleware lifecycle in Express.
Routing Mechanism
Basic Routing
Routing is a foundational element of Express. It determines how the application responds to client requests. The basic routing mechanism uses methods like , , etc., to define routes easily. This simplicity is one reason many developers prefer Express for rapid application development. It allows for quick setup and minimal overhead. However, without careful management, route conflicts can arise, leading to confusion in request handling.
Route Parameters
Route parameters are dynamic segments of a URL and provide a way to capture values. For example, allows the application to extract the user ID from the request. This key characteristic enables the building of RESTful APIs efficiently. However, the flexibility comes with a caution; mismanagement of parameters can lead to security vulnerabilities and logical errors if not validated properly.
Route Handling
Effective route handling ensures that each request is managed correctly. Express allows developers to define separate functions for different routes. Each function can process the request according to the application's needs. The benefit of this is clear, as it improves maintainability and readability. Handling various routes with clarity is a hallmark of good application architecture. Still, it requires diligent management to ensure that route handlers do not become overwhelmingly complex or interdependent.
Setting Up Your Express Environment

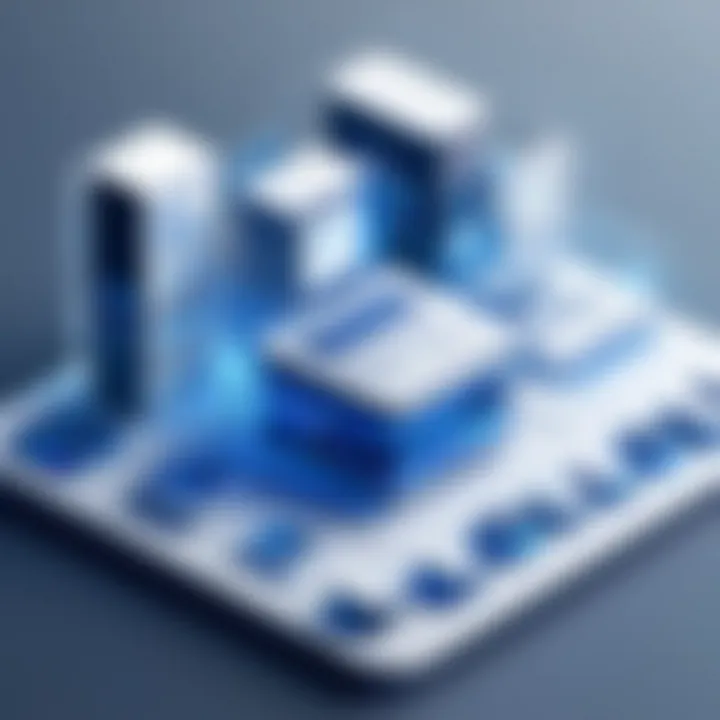
Setting up the environment for Express applications is crucial. This foundation determines how effectively developers can create and manage web applications. An organized and efficient setup ensures that the application runs smoothly and allows easy implementation of features. It streamlines the development process and minimizes issues related to environment compatibility and dependencies.
Prerequisites for Installation
Before diving into the installation of Express, certain prerequisites must be met. First, Node.js needs to be installed. Node.js is the underlying runtime for Express. You can download it from the official Node.js website. Along with Node.js, npm (Node Package Manager) is also installed automatically. npm is essential for managing packages. It allows the easy installation of Express and other necessary libraries.
Additionally, a text editor is required. Popular choices among developers include Visual Studio Code, Sublime Text, and Atom. Each of these editors provides features that can enhance coding efficiency, such as syntax highlighting and debugging tools.
Another important requirement is having a basic understanding of JavaScript. Since Express is built on JavaScript, knowledge of the language will aid in comprehension and facilitate a smoother development experience.
Installation Steps
Once the prerequisites are fulfilled, the installation of Express can commence. The following steps outline the process:
- Open Terminal or Command Prompt: Depending on the operating system in use, open the appropriate command line interface.
- Create a New Directory: It is best practice to create a folder for your Express project. Use the command:Replace with your desired application name.
- Initialize a New Node.js Project: This step sets up a package.json file, which keeps track of the project's dependencies. Run:The flag automatically accepts all default settings.
- Install Express: Now, install Express by running:This command downloads and installs the Express framework, adding it to the project's dependencies in the package.json file.
- Create the Main Application File: Create a new file called or . This will be the main entry point of your Express application. You can do it using a text editor or by running:
- Start the Application: Open and set up a simple server. Here is a basic example:
- Run the Application: Finally, run the application using:Navigate to http://localhost:3000 in a web browser. You should see "Hello World!" displayed.
By following these steps, the Express environment is set up effectively. This allows for further exploration of the framework and the development of more complex applications.
Building a Simple Express Application
Building a simple Express application is fundamental for understanding the functionalities and structure of the Express framework. Express serves as a flexible Node.js framework that provides a robust set of features to build web and mobile applications. Emphasizing this topic is crucial as it gives developers a hands-on perspective on how to utilize Express in real-world projects.
This section highlights the step-by-step process of creating your first application along with understanding its structure. It will not only simplify the learning path for beginners but also enhance the skills of those familiar with Node.js. By diving into the mechanics of Express, developers can appreciate the low overhead and ease of integration it offers.
Creating Your First App
To create your first Express application, a few basic steps must be followed. First, ensure that you have Node.js installed. With Node.js, you can easily set up an Express project which simplifies the process of developing server-side software.
- Initialize your project: Run the following command in your terminal to create a new directory and navigate to it:
- Create a package.json file: This file is essential for managing dependencies, scripts, and project information. Execute this command:
- Install Express: Using npm, install the Express package with this command:
- Creating server.js file: Now, you can create a file named which will serve as the main entry point to your application. The following code creates a simple server:
- Running your application: In your command line, execute the following command:
Upon running this command, your simple Express application will be accessible at , displaying 'Hello World!'. This illustrates how easy it is to set up an application using Express.
Understanding the App Structure
Understanding the app structure is essential to effectively maintain and expand your application. The application developed above consists of a single file and is perfect for demonstration. Nevertheless, as applications grow, a structured and organized approach is necessary.
An Express application generally follows this structure:
- app.js/server.js: The main application file.
- routes/: Contains all route definitions.
- views/: Template files for rendering web pages.
- public/: Static files such as CSS and JavaScript.
- node_modules/: Contains all npm packages.
- package.json: Dependency management file.
Reflecting on this structure provides clarity in terms of file organization. Furthermore, it minimizes confusion and augments collaboration among team members. Mastering the files and their purposes will facilitate better project management.
Building a simple Express application provides great insight into the operational mechanics of this framework. Such foundational knowledge prepares developers for more advanced concepts in web development.
Enhancing Your Express Application
Enhancing your Express application goes beyond initial setup and basic functionality. It involves incorporating elements that improve user experience, boost application performance, and facilitate future scalability. In the fast-evolving tech environment, ensuring your application meets user expectations is vital. Integrating tools such as template engines and static file serving can significantly enhance the overall effectiveness of your application.
Implementing Template Engines
Template engines are crucial in rendering dynamic content. They allow developers to create HTML pages with ease and maintain a clear separation of logic from presentation. Two popular template engines in Express applications are EJS and Pug. Understanding their features helps in making an informed choice based on project needs.
EJS
EJS (Embedded JavaScript Templating) is a simple yet powerful templating solution. It allows for embedding JavaScript code directly into HTML, enabling developers to create dynamic web pages efficiently.
A key characteristic of EJS is its syntax, which is similar to HTML. This makes it beginner-friendly, especially for those already familiar with traditional web development. Its ability to include partials and layouts supports the DRY (Don't Repeat Yourself) principle.
Moreover, one of the unique features of EJS is its partial rendering system. Developers can define common elements like headers or footers in separate files and include them in their main templates. This reduces redundancy and enhances maintainability. However, EJS does not enforce strict indentation rules, which can occasionally lead to inconsistent formatting.
Pug
Pug, formerly known as Jade, sets itself apart with its clean, minimalistic syntax. It reduces HTML boilerplate, enabling developers to write less code to achieve the same result. This makes it a favorable choice for those looking for succinctness in their markup.
Pug's key characteristic lies in its output efficiency. With its indentation-based structure, it provides clear nesting, making it visually intuitive. This can be particularly advantageous for maintaining complex views. One notable feature of Pug is the ability to use mixins, offering enhanced flexibility in creating reusable components.
However, the learning curve can be steep for those accustomed to traditional HTML. The unique syntax might be challenging for beginners at first, leading to initial confusion.
Static File Serving
Static file serving is another fundamental feature that enhances Express applications. It allows developers to serve static assets like images, stylesheets, and client-side JavaScript files efficiently. By correctly serving these resources, applications can deliver a smoother experience.
To enable static file serving in Express, you can use the built-in middleware. It's simple to implement:
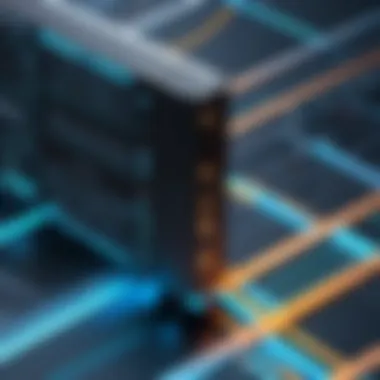
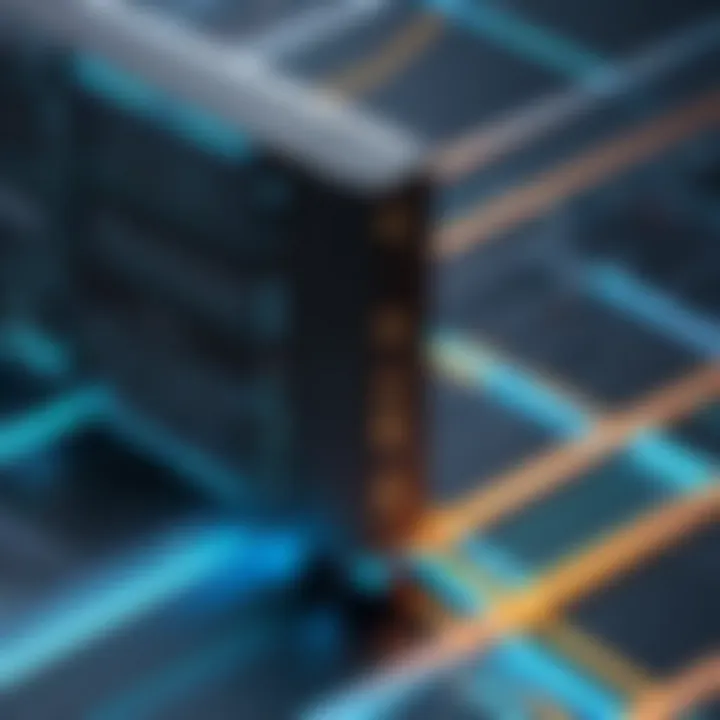
In this example, all files within the directory become accessible to users. Properly configuring static file serving can improve load times for your users, as these files can be cached by browsers.
Data Handling in Express
Data handling is a significant part of web development when using the Express framework. The ability to receive, process, and respond to various data formats is essential for building interactive applications. This section deals with some critical aspects of data handling in Express, notably focusing on how to work with JSON and manage form data. Understanding these elements can directly impact application performance and user experience.
Working with JSON
JSON (JavaScript Object Notation) is crucial for data exchange in web applications. Express simplifies JSON parsing, making it seamless for developers to handle incoming JSON requests. When an Express server receives a JSON request, it is vital to extract and process the data properly.
- Ease of Integration: Express supports JSON natively using middleware, allowing for easy integration into routes.
- Performance: Improved performance can be seen when using JSON, due to its lightweight nature compared to other formats.
- Compatibility: JSON is well-supported across various programming languages and platforms, enhancing interoperability.
To enable JSON handling in an Express app, the following middleware is often used:
This enables the application to parse JSON bodies and lets developers access data through .
Handling Form Data
Form data is another common type of data that web applications manage. In Express, handling form submissions typically involves parsing URL-encoded data and plain text in addition to JSON. There are some essential aspects to consider when working with this type of data.
Using body-parser
Body-parser is a middleware module for parsing the body of incoming requests in various formats. It is particularly useful for working with form data. The main advantage of body-parser is its simplicity and effectiveness. For example, to handle URL-encoded data, you would use:
This allows developers to easily access form fields via . Its popularity stems from the ability to configure it based on project requirements. However, using body-parser also has its downsides. Adding more middleware to your application can lead to performance overhead, so it should be used judiciously.
Form Validation
Form validation ensures that the data submitted through forms meets specific criteria before processing. This is essential for maintaining data integrity and security. Using libraries such as is common practice in the Express ecosystem.
- Prevention of Invalid Data: Form validation effectively prevents the submission of invalid or harmful data.
- User Experience: Providing immediate feedback enhances the user experience, leading to cleaner interactions.
- Customization: Validation can be tailored to specific fields, allowing for detailed error messages.
While form validation is beneficial, its implementation can increase development time. Balancing thorough validation with a straightforward user experience is crucial.
"Effective data handling forms the foundation of a solid web application. With Express, developers can streamline this process through middleware."
Error Handling in Express Applications
Error handling is a vital aspect of building robust Express applications. In a production environment, unhandled errors can lead to server crashes, poor user experience, and a loss of data integrity. Understanding how to manage errors effectively is crucial for any developer working with Express. This section aims to elucidate the significance of error handling, discuss common errors encountered in Express applications, and guide you in creating custom error handlers.
Common Errors in Express
In the development of Express applications, several common errors may arise. Recognizing these errors is the first step to implementing effective error handling strategies. Some of the most frequent issues include:
- 404 Not Found: This error occurs when a requested resource is not available. For example, if the user tries to access a route that does not exist, the server will return this error.
- 500 Internal Server Error: This is a generic error message indicating that something has gone wrong on the server. It could stem from various issues, such as database connectivity problems or uncaught exceptions in your code.
- Validation Errors: These happen when user input does not meet certain criteria. For instance, if a form requires an email address, and the user provides an invalid format, a validation error will occur.
- Database Errors: Issues connecting to databases can lead to errors. Improper queries or unhandled promises might also cause these problems.
Being aware of these common errors is essential. Dealing with them promptly can save significant time and resources.
Creating Custom Error Handlers
Creating custom error handlers in Express allows for a tailored error management strategy that meets your application's specific needs. Hereโs how to set up a basic custom error handler:
- Define the Error Handling Middleware: Middleware functions are essential in Express. You can create a custom error handler middleware that takes four arguments: , , , and .
- Implement in Your Application: Place the custom error handler after all your route definitions, to catch any errors that occur in the application.
- Customize the Response: Instead of providing a generic error message, tailor your response according to the error type. For example, return different status codes for validation errors versus server errors.
- Log the Errors: Itโs crucial to log errors for debugging purposes. Use a logging library like Winston or Morgan for better logging management.
By implementing a custom error handler, your application will be more maintainable and user-friendly. Proper error management leads to better performance and a positive experience for users.
"Good error handling is as important as writing good code. It reflects the professionalism of developers."
Testing and Debugging Express Applications
Testing and debugging are crucial components of any software development lifecycle. In the context of Express applications, these practices ensure reliability, maintainability, and scalable performance. As developers create applications, they often encounter bugs or unexpected behaviors. Hence, adopting a structured approach to testing and debugging is vital. It not only accelerates the development process but also enhances code quality.
Benefits of Testing:
- Early Detection of Bugs: By integrating testing into the development process, developers can identify potential errors early on, reducing the cost of fixing them later.
- Code Confidence: Comprehensive tests provide confidence that the code functions as intended, enabling safer refactoring and feature additions.
- Documentation: Tests act as a form of documentation, illustrating how different parts of the application should behave, aiding both current developers and future contributors.
Considerations:
- It is essential to use the right testing frameworks to optimize productivity.
- Testing should cover unit tests, integration tests, and end-to-end scenarios to ensure complete coverage of application functionalities.
Overall, thorough testing and debugging practices foster robust Express applications that can handle real-world challenges efficiently, making these practices indispensable.
Unit Testing with Mocha
Unit testing focuses on validating individual units of code. In Express applications, this process ensures that each route, middleware, and function behaves correctly. Mocha is a popular JavaScript test framework that facilitates unit testing.
To set up unit testing with Mocha, follow these steps:
- Installation: First, install Mocha using npm:
- Creating Test Files: Create a directory, often named , where test files will reside.
- Writing Tests: Use the following format to write a basic test:
- Running Tests: Execute tests with the following command:
Using Mocha allows developers to create clear and maintainable tests that are essential for building reliable Express applications.

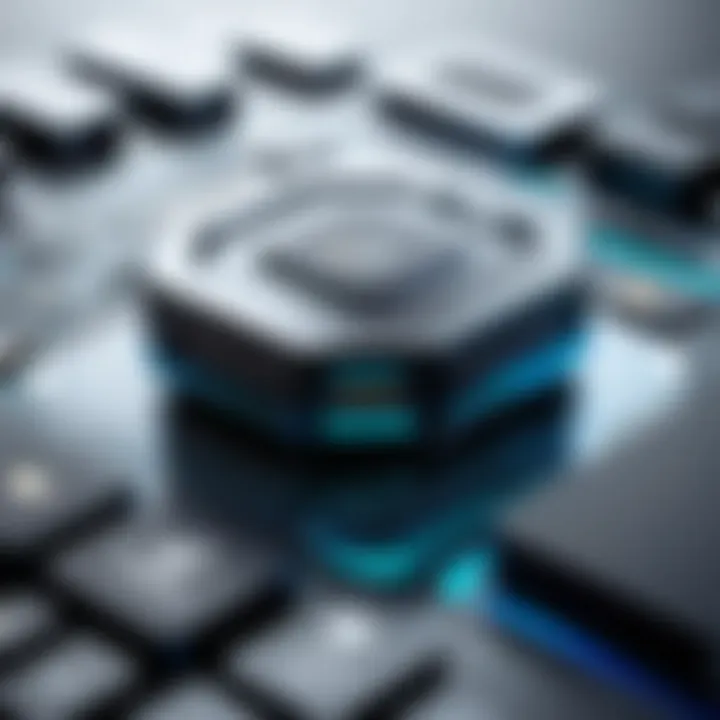
Integration Testing
Integration testing focuses on verifying the interactions between different components of an application. In Express, this includes testing how various middleware, routes, and database connections work together.
For integration testing in Express, developers often use tools like Supertest along with Mocha. Supertest allows developers to simulate HTTP requests to their application. Here are steps to set up integration testing:
- Installation: Add Supertest to your project:
- Creating Test Cases: Integrate Supertest to test your routes, for example:
- Running Integration Tests: Execute them just like unit tests:
Integration tests are vital for ensuring that all components of an Express application are communicating correctly and functioning together as expected, thereby providing a more complete coverage of potential issues that may arise in real-world usage.
Advanced Express Concepts
Advanced Express concepts are vital for building scalable and efficient web applications. Understanding these concepts helps developers leverage Express to its full potential. This section will explore how Express integrates with databases and implements authenticationโa crucial aspect of securing web applications.
Using Express with MongoDB
Integrating Express with MongoDB allows developers to manage data effectively in their applications. MongoDB is a NoSQL database, making it flexible and ideal for handling unstructured data. With Express serving as the web framework, the combination provides a powerful solution for modern web development.
Benefits of using MongoDB include:
- Scalability: Can handle large volumes of data without a fixed schema.
- Performance: Quick data retrieval as it stores data in JSON-like documents.
- Ease of Use: Simple API that integrates well with JavaScript.
To connect Express with MongoDB, developers generally use libraries like Mongoose. Mongoose facilitates schema creation and data manipulation in a seamless way. Here is a basic example of setting up a connection:
This snippet demonstrates a typical connection setup. By utilizing MongoDB with Express, developers can build applications that capture, store, and manage user data dynamically.
Implementing Authentication
Authentication is crucial for protecting sensitive user information. In an Express application, implementing secure authentication mechanisms enhances user trust and maintains data integrity. Two prominent methods for implementing authentication are Passport.js and JWT (JSON Web Tokens).
Using Passport.js
Passport.js is a middleware that simplifies the authentication process in Node.js applications. Its design allows easy integration of various authentication strategies, such as OAuth, Local, or OpenID. One key characteristic of Passport.js is its modular approach. This means developers can select specific strategies to fit their needs.
A primary benefit of Passport.js is its extensive documentation and community support, which enhances its usability. The unique feature of Passport.js is its ability to allow users to remain logged in, providing a smooth user experience. However, challenges include the necessity of managing sessions securely, which may require additional development time.
JWT Authentication
JWT Authentication operates by generating a token that contains user details. This token is sent with every request, allowing for stateless authentication. A primary advantage of using JWT is its performance. Since it does not require session storage on the server-side, JWT can handle a higher load of users without affecting performance.
The unique feature of JWT includes its ability to validate tokens easily using a secret key. However, there are disadvantages too. If the token is compromised, it might allow unauthorized access until it expires. Therefore, careful management of token expiration times is needed.
By understanding and implementing advanced Express concepts, developers can create robust, secure, and scalable applications that meet user needs effectively.
Deployment of Express Applications
Deploying an Express application is a crucial phase in its lifecycle. This stage emphasizes transforming a locally developed application into a fully realized web service accessible to users across the internet. Understanding deployment is vital not only for ensuring the application runs smoothly but also for maintaining its reliability over time. Here, we will consider several essential elements, benefits, and considerations regarding the deployment of Express applications.
When deployment is handled well, the application benefits from improved performance, scalability, and security. These qualities are critical as they determine how effectively the application can serve users. Developers must address issues such as environment configuration, server management, and application scaling. Moreover, choosing the right hosting options can significantly impact the success of the deployment.
Preparing for Production
Before deploying your Express application, you need to prepare it for production environments. This preparation involves several steps:
- Configuration: Ensure that environment variables are set properly. These variables can include API keys, database connection strings, and other sensitive data.
- Logging and Monitoring: Implement logging for various events and errors. Effective monitoring tools allow developers to track application performance and user activity.
- Optimize Performance: Use tools to minify your assets and enable caching. This step can greatly enhance load times and reduce server response time.
- Security Measures: Apply security best practices like HTTPS, data validation, and sanitation to protect against attacks.
With these aspects in mind, your application stands a much better chance of functioning effectively in a live setting.
Common Hosting Options
Choosing the right hosting service is essential. Two popular choices for deploying Express applications are Heroku and DigitalOcean.
Heroku
Heroku is a platform that simplifies the deployment process for developers. One of the key characteristics of Heroku is its ease of use. The platform follows a simple model where developers push their code using Git, and it automatically handles the deployment process. This aspect makes Heroku a beneficial choice for those looking to deploy applications quickly without worrying too much about the underlying infrastructure.
However, one unique feature is the Dynos concept, which refers to the lightweight containers that run your applications. While Heroku's marketplace offers various add-ons for database, monitoring, and more, it can be costly for larger applications due to its pricing model based on resource usage.
DigitalOcean
DigitalOcean is known for providing a more hands-on experience compared to Heroku. The platform is characterized by its simplicity and flexibility, which appeals to developers familiar with managing their own servers. One significant advantage of DigitalOcean is the ability to deploy on Droplets, which are virtual machines tailored to specific computational needs.
This flexibility allows precise control over the environment and resources. However, the requirement for server management knowledge can be a disadvantage for beginners. Users must also consider monitoring and scaling configurations, which may require additional setup time.
Future of Express Framework
The future of the Express framework is vital to understand for those engaging with web development using Node.js. With the rapid growth in web technologies, keeping pace with the evolving demands of developers is crucial. Express has emerged as a key tool, providing simplicity and flexibility. It is essential to explore what lies ahead for this framework, its upcoming features, and the evolving community that supports it.
Upcoming Features
As Express continues to develop, several features are expected to enhance its utility:
- Improved Performance: The development team is focused on optimizing the performance of the framework, making it more robust for high-traffic applications.
- TypeScript Support: With the rise in popularity of TypeScript, it is anticipated that official TypeScript support will be further integrated, facilitating a smoother experience for developers who prefer typed languages.
- Better Middleware Management: Enhancements to how middleware is managed and integrated will likely simplify application structure and reduce overhead.
- Enhanced Routing Capabilities: Future releases may focus on advanced routing options to support complex application structures more effectively.
These anticipated improvements are geared toward maximizing developer productivity while bolstering application performance.
Community and Ecosystem
The Express community plays a significant role in its evolution. A thriving community ensures that users have access to a variety of resources and support. Key aspects of the Express ecosystem include:
- Active Contributions: Numerous contributors are involved in the ongoing development of Express. Their collaborative efforts lead to continuous improvements and innovations.
- Extensive Documentation: The community maintains comprehensive documentation, making it easier for both new and experienced developers to understand the framework.
- Plugins and Middleware: A wide array of third-party middleware options greatly enhance the capabilities of Express applications. This diversity empowers developers to easily extend functionality based on project needs.
- Support Channels: Online platforms such as Reddit and dedicated forums offer spaces for users to discuss issues, share solutions, and explore new features.
"The spirit of collaboration defines the success of open-source frameworks like Express. Continuous improvement is not just a goal, but a shared journey for all developers involved." - Express Community Insights