Mastering Interfaces in Android Development
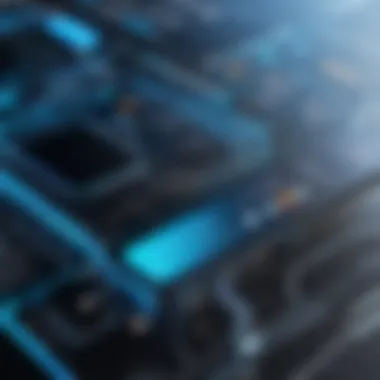
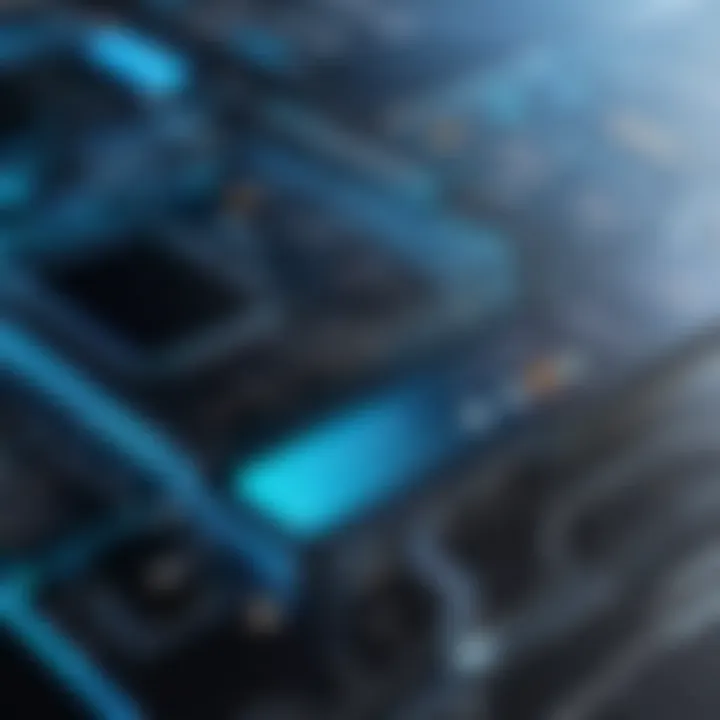
Overview of Topic
In the landscape of Android development, understanding how interfaces work can significantly enhance a programmer's ability to write cleaner, more maintainable code. At its core, an interface in programming defines a contract - a set of methods that a class agrees to implement. This structure fosters a level of abstraction that promotes modular design and effective reuse of components.
The significance of interfaces is hard to overstate. They are fundamental in creating scalable applications, especially where different modules communicate efficiently. In a rapidly changing tech environment, interfaces remain consistent, serving as a powerful tool to manage complexity in software development.
Historically, as programming languages evolved, so did the concept of interfaces. Early programming languages focused more on procedural programming, often leading to tightly coupled code. The introduction of object-oriented programming provided a shift towards more modular designs. With this evolution, interfaces emerged as key elements in object-oriented languages, enabling developers to define methods without dictating their implementation, thereby promoting flexibility.
Fundamentals Explained
Core Principles of Interfaces
At a basic level, an interface aligns with the core principle of polymorphism. It allows objects of different classes to be treated as objects of a common superclass. This is especially useful in scenarios where you may not know the exact types of objects you're working with at compile time.
Key Terminology
- Interface: A reference type in Java (and similar languages) that can contain only constants, method signatures, default methods, static methods, and nested types.
- Implementation: The process of writing the code for the methods defined in the interface.
- Abstract Class: A class that cannot be instantiated on its own and may contain abstract methods, but can also define behavior.
Basic Concepts
An interface typically consists of:
- Method Declarations: These are signatures without any body, defining what methods a class needs to implement.
- Multiple Interfaces: Unlike classes, an interface can extend multiple interfaces. This feature allows for a rich design without the pitfalls of multiple inheritance.
Practical Applications and Examples
In the real world, interfaces find use in various applications. For instance, in an e-commerce app, you might have an interface called . Different payment methods like , , and can all implement this interface. This design allows your application to handle payments without needing to know the specific classes:
When it's time to process a payment, the application just needs to call the method without worrying about the underlying implementation specifics.
Additionally, interfaces can provide hooks to allow plugins to enhance functionality without touching the core application, exemplifying code reusability and clean architecture.
Advanced Topics and Latest Trends
As technology advances, the traditional concept of interfaces is also evolving. New patterns emerge, like the use of Functional Interfaces, which enable usage with lambda expressions introduced in Java 8. This provides a powerful way for developers to write concise and readable code.
Cutting-edge Development: The trend now leans towards using interface segregation to promote focused interfaces with specific functionalities, which leads to better code comprehension and less clutter.
Future Prospects: Considering the rapid chalking of new programming paradigms, one cannot ignore the potential of interfaces in microservices architecture, providing clear communication channels between services.
Tips and Resources for Further Learning
To dive deeper into interfaces in Android development, consider exploring the following resources:
- Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma et al.
- Effective Java by Joshua Bloch
- Online courses on platforms like Coursera or Udacity that cover object-oriented programming.
For practical tools, IDEs like Android Studio provide excellent debugging and code management features, allowing you to test interface implementations easily.
As you continue your journey into Android development, remember that learning about interfaces is not just beneficial; itās essential for mastering the principles of clean and efficient coding.
Prelims to Interfaces
In the domain of Android development, interfaces serve as a fundamental construct that not only influences coding practices but also enhances the overall integrity of applications. The concept of interfaces allows developers to define contracts that specify behavior without dictating how that behavior should be implemented. This results in a layered approach to software architecture, encouraging modular design and bolstering code reusability. Importantly, as we step into the intricate world of Android programming, understanding interfaces becomes paramount to mastering many advanced design patterns and best practices.
Defining Interfaces in Programming
In programming, an interface is like a bridge connecting different components while hiding the underlying complexities from the user. It consists of a set of abstract methods that a class must implement to be classified as adhering to that interface. Imagine you have various forms of payment methodsācredit cards, PayPal, and cash. Rather than defining a unique set of features for each, an interface can specify necessary methods, like , while allowing each payment type to define how it operates internally.
This abstraction promotes adherence to the DRY (Don't Repeat Yourself) principle, where a single contract can serve multiple implementations. For instance, if a class implements the interface, it must provide its version of how a payment is processed, removing redundancy and streamlining future updates.
Importance of Interfaces in Android
In the fast-evolving landscape of Android applications, interfaces hold significant weight in ensuring that code remains clean and adaptable. The importance of well-defined interfaces cannot be overstated, particularly in an environment where user experiences can change rapidly, and applications must scale effectively.
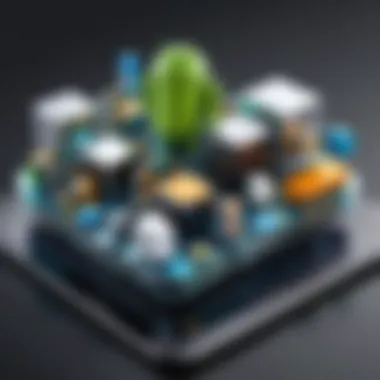
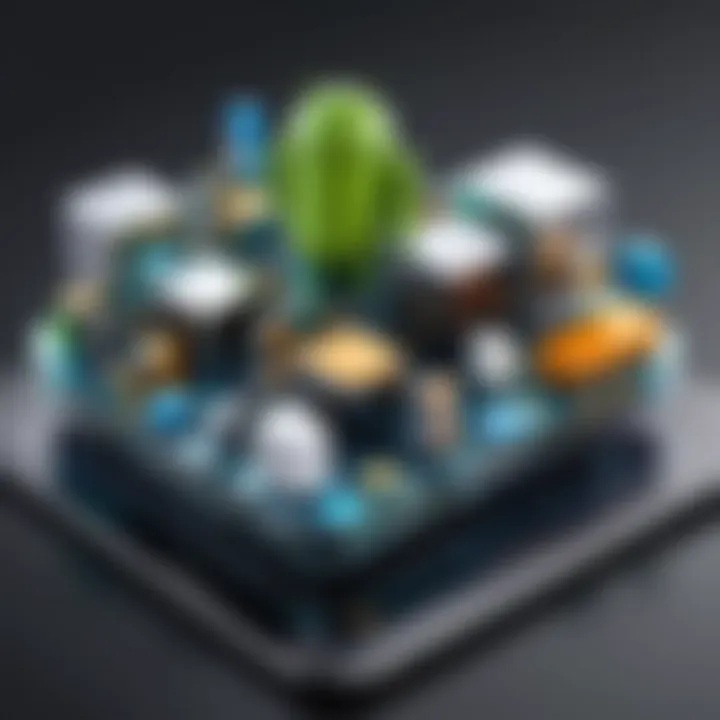
One notable benefit is modularity. By using interfaces, developers can break down functionality into separate pieces that are easier to manage and evolve over time. When features need to be adjusted or new capabilities need be added, they can simply create a new class that implements an existing interface without impacting the broader application structure.
Moreover, interfaces significantly enhance testability. They allow for the easy mocking of components during unit testing. For instance, if you're testing a user authentication system, you can create a mock object that adheres to the interface, ensuring that tests run quickly without needing a fully operational user management system.
"Interfaces are not just about connecting classes; they are about enhancing collaboration and fostering innovation in Android development."
Core Concepts of Interfaces
Understanding interfaces hinges on recognizing their critical role in programming, particularly within the Android ecosystem. Interfaces act as contracts. They lay down rules for classes that implement them, facilitating a structure around how classes communicate with each other. This not only enhances modularity but also enables a level of abstraction that makes the code easier to maintain and expand.
In Android development, interfaces help in separating the concerns within the codebase. By promoting a decoupled architecture, interfaces foster flexibility and facilitate easier testing. When developers write code with interfaces in mind, they create systems that can adapt and evolve without needing extensive rewrites. This adaptability is essential in todayās fast-moving tech landscape, where requirements can shift overnight and agile response is key.
Characteristics of Interfaces
Interfaces have several distinct characteristics that set them apart from classes:
- No State Limitation: Unlike classes, interfaces do not hold state. This means they can only declare methods, but not implement them. This ensures that any implementing class must provide functionality.
- Multiple Implementations: A single interface can be implemented by multiple classes, promoting code reuse and consistent method signatures across different types.
- Complete Abstraction: Interfaces provide a way to achieve complete abstraction for the methods they define. This allows programmers to interact with objects without needing to know their specific implementations.
- Default Methods: With the introduction of default methods in Java 8, interfaces can now have methods with a body. These default methods allow for some level of implementation within interfaces, enabling them to evolve without breaking existing code.
Types of Interfaces
Interfaces aren't just a one-size-fits-all concept. They come in various types, each serving a distinct purpose.
Standard Interfaces
Standard interfaces form the backbone of many Java applications, including those in Android. They are simply defined interfaces that include abstract methods that classes must implement. The main characteristic is their universal applicability across different scenarios.
This type is beneficial for developers as it provides a common structure for various classes, promoting consistency. One unique feature of standard interfaces is their ability to define callbacks, which are crucial in event-driven programming. However, maintaining clarity can become challenging if the interface grows too large, leading to a potential overload of methods that not every implementing class needs.
Marker Interfaces
Marker interfaces donāt have any methods at all. Think of it as a tag. They serve to convey metadata about a class. The most well-known example in Java would be . By simply implementing this interface, a developer indicates that instances of this class can be serialized, allowing them to be converted into byte streams.
Their primary advantage is simplicity. This distinct characteristic allows the developers to easily check capabilities without cluttering with method definitions. However, a potential downside is the reliance on convention, as there's no explicit method signifying the classās capabilities.
Functional Interfaces
Functional interfaces are interfaces designed with a single abstract method. This makes them particularly valuable in functional programming scenarios, especially in Androidās lambda expressions. Their key characteristic is the ability to facilitate functional programming, enabling behavior to be passed around as parameters.
These interfaces are beneficial for situations where concise code is desired, allowing for clearer, more readable expressions of intent. However, while they encourage brevity, developers must ensure they donāt sacrifice clarity for conciseness, as overly condensed lambda expressions can become difficult to understand.
Ultimately, understanding these core concepts of interfaces empowers developers to use them judiciously, paving the way for cleaner, more manageable, and scalable codebases.
Creating Interfaces in Android
Creating interfaces in Android plays a crucial role in enhancing the functionality and organization of code. By defining clear contracts, interfaces facilitate communication between disparate parts of an application without forcing tight coupling. This approach is essential in maintaining a clean architecture, where components interact while remaining independent. In a rapidly evolving environment like Android development, where updates and modifications to one part of the application shouldn't ripple through and break the entire system, interfaces help developers keep their code modular and flexible. When implementing a new feature or modifying existing code, interfaces ensure that the changes can be introduced with minimal friction.
Syntax and Declaration
The syntax for declaring an interface in Java, the primary programming language for Android, is rather straightforward. An interface can be defined using the keyword, followed by the interface name. Hereās a simple breakdown of how you would typically declare an interface:
In the above example, is an interface with two methods: and . Notice how methods in an interface do not have bodies. This absence of implementation means that any class choosing to implement this interface must provide the specific behavior for those methods.
When thinking about interface design, itās essential to focus on clarity. Each method should represent a single responsibility, thus adhering to the Single Responsibility Principle. By doing so, interfaces become easier to implement and maintain over time.
Implementing Interfaces
Implementing an interface is a vital step in utilizing its benefits within an Android project. A class, to adopt an interface, uses the keyword. Hereās how that looks in practice:
In this example, implements . Both methods defined in the interface are overridden, allowing to specify what happens when those are called. This not only gives flexibility but also promotes code reuse.
In many cases, you might find yourself implementing multiple interfaces in a single class. This can lead to a cleaner separation of concerns and, in turn, more manageable code overall. Itās worth noting that Java does not support multiple inheritance directly. However, interfaces provide a way to achieve a similar outcome through multiple interface implementations.
Practical Applications of Interfaces
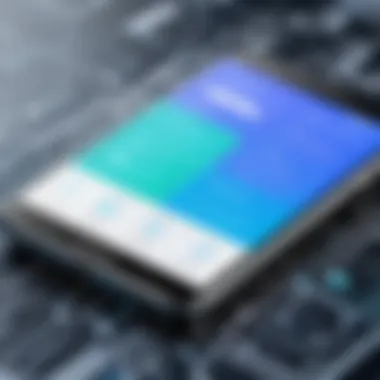
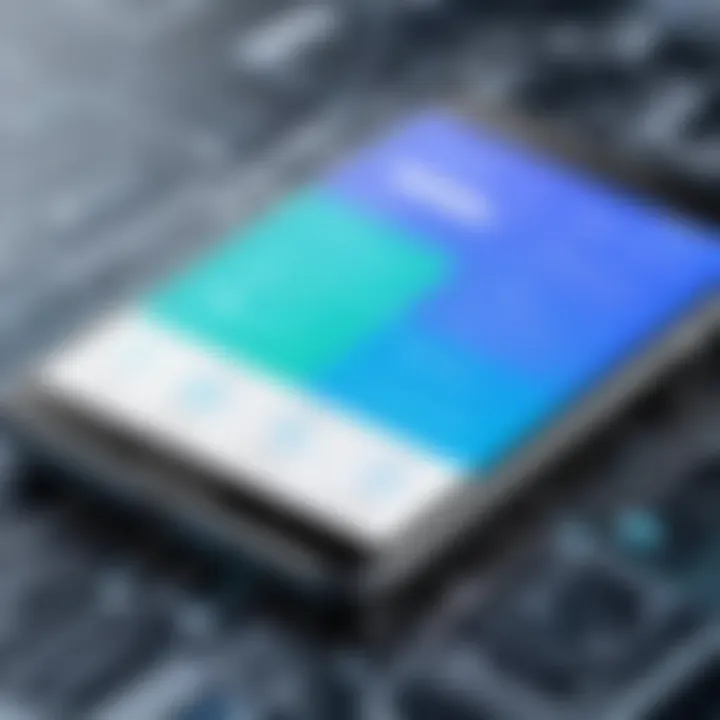
Interfaces in Android development serve not only as guidelines but also as powerful tools to enhance both the architecture and functionality of applications. When utilized correctly, these interfaces can significantly contribute to creating well-structured, efficient, and maintainable code. They encourage a modular approach, enabling developers to work on discrete components independently, while promoting interoperability between different parts of the code.
Using Interfaces for Event Handling
Event handling is at the heart of user interaction within an application. Without effective handling mechanisms, the user experience can fall flat. Here, interfaces shine brightly.
By implementing interfaces specifically designed for event handling, developers can decouple the event source from the event consumers. For instance, consider a simple scenario where a user clicks a button. Instead of embedding the click logic directly within the button's class or activity, an interface can be defined. This interface can be implemented by any listener class, allowing for various responses to the same event.
This approach not only promotes reuse of code but also enhances readability. Hereās a basic example:
Implementing this interface in an activity or fragment allows for different click behaviors without altering the source code of the button's class. This modularity makes event handling more dynamic, and it enables developers to build cleaner, more flexible architectures.
Design Patterns Utilizing Interfaces
Design patterns are proven solutions to recurring problems in software design. Interfaces are often integral to many design patterns, providing flexibility and promoting code reuse. Three notable patterns that leverage interfaces are the Observer Pattern, Strategy Pattern, and Decorator Pattern.
Observer Pattern
The Observer Pattern is a standout when it comes to making one part of an application responsive to changes in another. Essentially, this pattern establishes a subscription model, where one object (the subject) maintains a list of its dependents (observers) and notifies them of state changes automatically. This is especially beneficial in scenarios where the UI needs to reflect real-time data changes, like live sports scores or stock market updates.
A key characteristic of this pattern is its push model of data updates. In Android, implementing this pattern often utilizes interfaces, where the observers define how they want to be notified. For instance, one could have:
One of the unique features of the Observer Pattern is the decoupling it offers. This facilitates cleaner code and enhances maintainability, as adding new observers doesn't require changing the subject. However, a potential disadvantage is that it can lead to complexities in debugging due to potential interdependencies between various observers.
Strategy Pattern
The Strategy Pattern promotes the idea of defining a family of algorithms, encapsulating each one, and making them interchangeable. With interfaces at its core, this pattern allows one to select an algorithm at runtime based on specific conditions or user input.
A notable characteristic of the Strategy Pattern is its emphasis on separation of concerns. For example, in a payment processing scenario, one could create an interface like:
Implementing different payment methods as strategy classes (like CreditCardPayment or PayPalPayment) lets users choose how they want to pay without cluttering the process in a single class. The modular approach not only simplifies testing but also aligns perfectly with the open/closed principle, favoring extension over modification. However, a downside could be an increased number of classes, which might slightly complicate the codebase.
Decorator Pattern
The Decorator Pattern offers a way to extend functionality dynamically without modifying existing code. This pattern is exceptionally useful for adhering to the single responsibility principle because it allows adding behaviors to objects at runtime.
In a typical use case, suppose you have a basic coffee class:
Using a decorator interface, you can add features like milk or sugar. Each add-on conforms to the same interface, adding customization seamlessly:
This adds dynamism, making it easier to accommodate new features without disrupting the existing code. However, the downside is that it may lead to a complex structure of decorators, making it hard for newcomers to grasp.
Advantages of Interfaces
Interfaces in Android development hold key advantages that cannot be overstated. They fundamentally reshape the way developers structure their application logic and manage interactions within their code. As one moves deeper into the landscape of Android programming, understanding these benefits becomes pivotal. Interfaces foster a coding environment that is not only organized but also adapts gracefully to changes and evolving requirements.
Modularity and Flexibility
One of the foremost advantages of interfaces is the modularity they bring to code. When developers design an interface, they essentially lay down a contractādefining a set of methods that different classes can implement. This allows for a clear separation between the functionalities of different components. For instance, let's say you are developing a music player app. You might have an interface called , outlining methods like , , and . Multiple classes can then implement this interface in their unique ways, allowing for different behavior based on the media source, be it online streaming or local files.
"Modularity not only enhances flexibility but also ensures that changes do not ripple through the entire codebase."
In simple terms, if one part of your application changes, say, switching from local media to a network source, you only need to implement the interface differently in that context. Other parts of the code that use this interface remain untouched, preserving functionality and minimizing risks associated with bugs.
Enhanced Code Maintenance
Another significant benefit of utilizing interfaces in Android is the ease of code maintenance. Mr. Murphy of Murphy's Law once said, "Anything that can go wrong will go wrong." In the software world, this often rings true. However, having well-defined interfaces can mitigate this chaos to an extent.
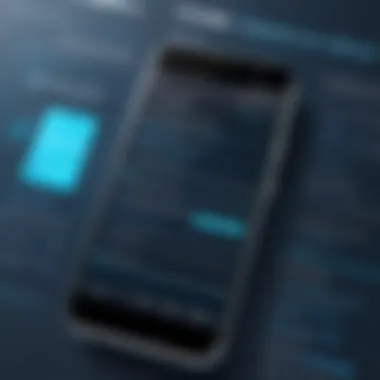
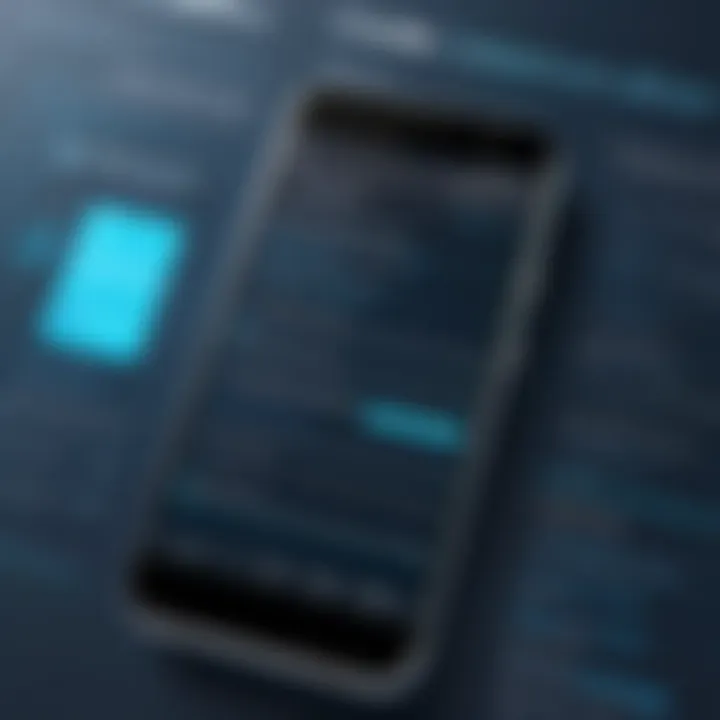
By promoting a structured approach, interfaces allow developers to pinpoint issues with greater precision. When a bug arises, trace-back becomes much simpler. You can check which implementation of an interface is responsible for a problem, rather than wading through an interconnected mess of classes. This makes fixing bugs faster and more effective, ultimately saving time and resources.
Moreover, refactoring becomes a less daunting task. Since classes implementing an interface must adhere to the defined methods, making changes in one class often doesnāt disrupt others utilizing the same interface.
Facilitating Testing and Mocking
Interfaces shine particularly in the realm of testing and mocking. In unit testing, it is crucial to isolate parts of the application to verify their behaviors. A common approach is to create mock implementations of your interfaces, allowing for the testing of specific components without the interconnected web of dependencies.
For instance, if you're testing a class responsible for playing media, you can create a mock that implements the interface. This way, you can simulate various scenariosālike media failure or successful playbackāwithout engaging with the actual media components. As a result, your tests can focus on specific functionalities, make them faster, and simplify their maintenance.
Common Challenges with Interfaces
Overusing Interfaces
It's tempting to think that more is better. However, when it comes to interfaces, this isnāt always the case. Overusing interfaces can lead to unnecessary complexity. For instance, in a project, a developer might create interfaces for every single class, intending to prepare for future use or flexibility. What happens, then, is that the codebase becomes cluttered with interfaces that don't add significant value.
Consider a scenario where a developer is working on an e-commerce app. Let's say they create several interfaces for handling payment methods - one for credit cards, another for PayPal, and yet another for gift cards. If these interfaces are barely distinguishable in functionality, it makes code harder to follow and maintain. Too many interfaces without clear, distinct roles can bog down the development process, as developers spend more time navigating between interfaces rather than focusing on actual implementation.
Therefore, it's crucial to strike a balance. Developers must evaluate the necessity of an interface for each component in their code. Are you using an interface to achieve polymorphism where it doesnāt really enhance your design? If yes, it's time to reconsider and simplify.
Interface Segregation Principle
The Interface Segregation Principle (ISP) is one of the five SOLID principles of object-oriented design. The ISP advises against forcing classes to implement interfaces they do not use. It's like inviting every cousin to a family gatheringāonly for them to sit at a table and stare at one another awkwardly. Similarly, forcing a class to implement methods that arenāt relevant leads to confusion and wasted effort.
For instance, imagine a media player app. If thereās a single interface that mandates methods for video playback, audio playback, and playlist management, and not all classes need all these functionalities, it could create issues.
By adhering to the ISP, developers can create smaller, more tailored interfaces that serve specific functionalities. For example, separate interfaces for VideoPlayer and AudioPlayer are more effective than one massive interface that forces all classes to accommodate every aspect. This adherence makes for cleaner code, simplifying both implementation and comprehension.
"Interfaces shouldn't be a catch-all solution but rather a tool for clear design."
The challenge, then, is to remain mindful of these principles while developing. Regular code reviews and team discussions can help in identifying when interfaces are unnecessarily utilized or when ISP could be better applied.
In summary, while interfaces serve many important roles in structuring Android applications, understanding the limitations and challenges of their use is essential to avoiding pitfalls that could complicate development.
Epilogue
In wrapping up this exploration of interfaces in Android development, it's vital to highlight their significant impact on creating robust applications. Interfaces serve as the backbone of a well-structured codebase, allowing developers to embrace modularity and ensure their applications remain flexible and maintainable. When one considers the architectural choices available, isolating the roles and interactions between various components through interfaces can lead to a cleaner, more comprehensible code structure.
The advantages of utilizing interfaces extend far beyond just reducing dependencies. They bolster collaboration amongst developers, inviting a clear separation of concerns which proves invaluable in large teams or projects. This clarity also aids in achieving effective code maintenance practices. With interfaces in place, future developers ā whether on the same team or new hires ā can grasp the functionality of code pieces without wading through intricate implementation details.
Moreover, understanding interfaces opens the door to several advanced design patterns used widely in software development, especially in Android. The realization that these building blocks encapsulate behavior without dictating how it's implemented grants immense freedom and power to a coder. This underscores the importance of interfaces in crafting an environment that supports innovation and experimentation.
"Clean code always looks like it was written by someone who cares." ā Robert C. Martin
Now, letās summarize the key points discussed and gaze into the future of interfaces in Android development.
References and Further Reading
When diving into the realm of Android development, References and Further Reading is the bread and butter for anyone looking to deepen their understanding of interfaces. Itās not just about writing code or creating applications; itās about gaining insights and sharpening your skills through a variety of resources that can illuminate complex concepts.
Having access to well-researched materials can enhance one's comprehension of the subject matter. Thereās a wealth of knowledge already out there, combining theories with practical applications. Whether you are a beginner trying to gain a foothold or a seasoned developer looking to refine your approach, tapping into the right resources is crucial. Here are some specific areas to focus on:
- Books and Articles: Books often offer in-depth explorations, structured sequences of learning that can help you grasp fundamental concepts progressively. Articles, on the other hand, usually provide quick insights, recent trends, and case studies that reflect the current industry practices. They can be more accessible for rapid learning and staying updated.
- Online Resources: The digital landscape is replete with interactive tools and online courses designed to cater to various learning styles. Websites and forums can provide immediate assistance when you're stuck, making it easier to troubleshoot problems or share ideas with peers. Furthermore, online community discussions can also educate you about industry standards and common practices.
This collaborative approachāto mix whatās found in books with the immediacy of online resourcesāencourages an informed yet practical learning experience tailored to modern development challenges.
"The essence of learning lies in the ability to apply knowledge within a real-world context."
Books and Articles
Books remain a cornerstone of structured education in programming. Many authors, experts in their field, have poured years of experience into volumes that demystify complex themes surrounding interfaces in Android development. Consider the following books:
- Effective Java by Joshua Bloch: This text introduces Java best practices and has a section dedicated to interfaces. It suggests guidelines that refine your understanding of how to implement them correctly.
- Android Programming: The Big Nerd Ranch Guide by Bill Phillips and Chris Stewart: This guide offers practical insights into Android development, including the use of interfaces. Its hands-on approach makes it easier to relate theoretical elements to code.
Subscribing to well-regarded programming magazines or journals adds another layer of knowledge that keeps you in sync with technical developments and design patterns.
Online Resources
In todayās age, education transcends traditional means, thanks to a plethora of online platforms. Some key online resources that can bolster your understanding of interfaces in Android include:
- Forums like Reddit: These platforms host vibrant communities where you can ask questions and get real-time answers from other developersājust remember, the quality of responses can vary.
- YouTube Tutorials: Many creators share valuable content that ranges from beginner-friendly explanations to advanced topics. You might discover engaging presentations that highlight the use of interfaces in real-world projects.
- Official Documentation and API References: Googleās official documentation is indispensable. It provides precise technical details alongside examples that are always beneficial to study.
Integrating various sources of information creates a dynamic educational experience. By continually working through recommended materials, one can enhance their command of interfaces in the Android ecosystem. Keep your curiosity alive and donāt shy away from exploring outside the conventional boundariesāeach reference can lead to surprising revelations.
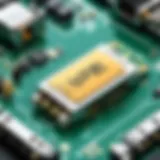
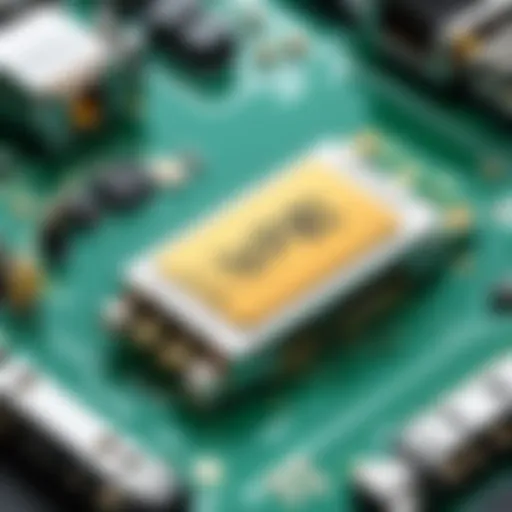