Mastering Java Programming: A Comprehensive Guide for Tech Enthusiasts
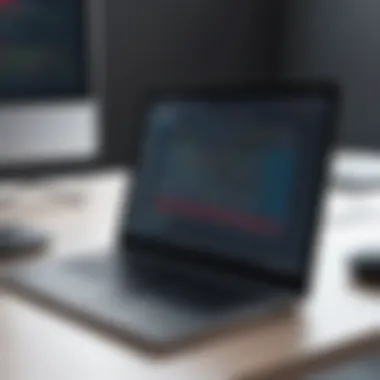
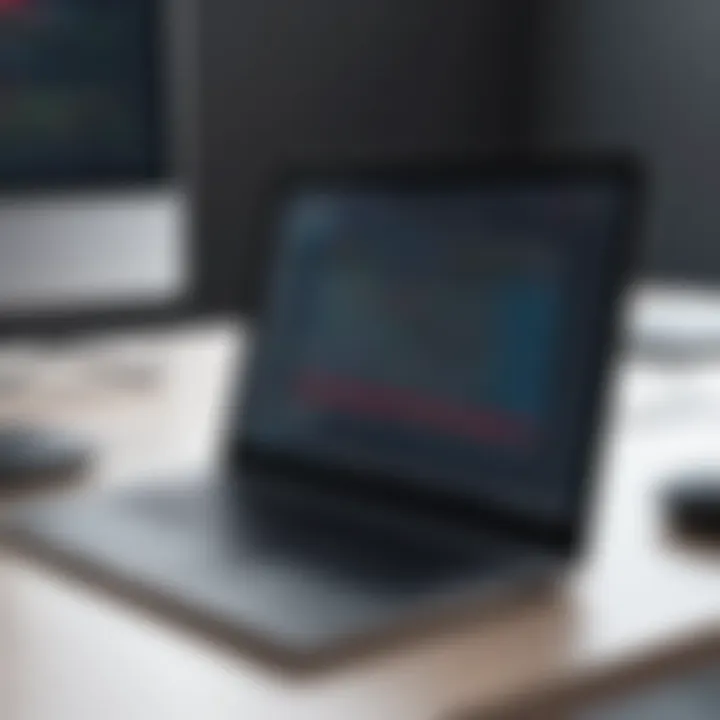
Overview of Java Programming
- Introduction to the Java programming language known for its versatility, portability, and robustness in the tech industry. Java has been a dominant player in the programming sphere due to its wide range of applications.
- Scope and significance: Exploring the vast scope of Java in software development, web applications, mobile applications, and enterprise solutions. Its significance lies in its object-oriented approach and platform independence.
- Brief history and evolution: Tracing the evolution of Java from its inception in the mid-1990s by Sun Microsystems to its current status as a widely used programming language with a strong community support and a rich ecosystem of libraries and frameworks.
Fundamentals of Java Programming
- Core principles and theories: Delving into the foundational principles of Java, including object-oriented programming, encapsulation, inheritance, and polymorphism. Understanding these core concepts is crucial for developing robust and scalable Java applications.
- Key terminology and definitions: Explaining essential terms such as classes, objects, methods, variables, and data types in Java. A firm grasp of these terms is necessary for proficient Java programming.
- Basic concepts and foundational knowledge: Covering basic concepts like control flow statements, loops, functions, and exception handling. These serve as the building blocks for more complex Java programming.
Practical Applications of Java Programming
- Real-world case studies and applications: Showcasing how Java is used in developing various applications, such as e-commerce platforms, financial systems, and data processing applications. Real-life examples provide insights into Java's versatility and applicability.
- Demonstrations and hands-on projects: Providing hands-on experience through practical projects like developing a simple GUI application, implementing data structures in Java, or creating a RESTful API. Practical exercises enhance understanding and mastery of Java programming.
- Code snippets and implementation guidelines: Offering code snippets for common Java tasks like file IO operations, string manipulation, and networking. Detailed implementation guidelines help in applying theoretical knowledge to practical scenarios.
Advanced Topics and Latest Trends in Java
- Cutting-edge developments in the field: Discussing advanced Java topics like multithreading, concurrency, Java virtual machine (JVM) internals, and performance optimization. Exploring these areas expands the horizons of Java programming beyond the basics.
- Advanced techniques and methodologies: Introducing advanced techniques such as design patterns, lambda expressions, stream API, and Java Persistence API (JPA). Mastering these concepts enhances code quality and developer productivity.
- Future prospects and upcoming trends: Looking ahead at the future of Java with emerging trends like modularization with Java 9 onwards, adoption of Kotlin for Android development, and advancements in cloud-native Java applications. Staying abreast of such trends is essential for staying competitive in the Java development landscape.
Tips and Resources for Further Learning
- Recommended books, courses, and online resources: Suggesting books like Effective Java by Joshua Bloch, online courses from platforms like Coursera and Udemy, and resources like Java documentation, Stack Overflow, and Java blogs. These resources aid in continuous learning and skill enhancement.
- Tools and software for practical usage: Listing essential tools for Java development such as Intelli J IDEA, Eclipse IDE, Maven for dependency management, and Git for version control. Leveraging these tools optimizes the development workflow and boosts productivity.
Introduction to Java Programming:
Java programming is a fundamental skill that every tech enthusiast, beginner, or professional should grasp. In the digital era, Java stands out for its versatility and applicability across various platforms, making it a cornerstone in the world of programming. Understanding Java programming opens up a realm of possibilities, from creating software applications to developing robust backend systems. This section provides a foundational understanding of Java programming, essential for anyone looking to embark on a journey into the realm of programming.
Overview of Java:
History and Evolution of Java:
The history and evolution of Java trace back to its inception in the mid-1990s by Sun Microsystems. Designed to be platform-independent, Java revolutionized the way software applications were developed. The key characteristic of Java lies in its 'write once, run anywhere' principle, allowing developers to write code that can be executed on various platforms without the need for recompilation. This feature makes Java a popular choice for this article as it emphasizes the importance of platform flexibility and ease of development. Despite its advantages, one notable disadvantage of Java's history and evolution is the occasional slower performance compared to natively compiled languages.
Features and Characteristics of Java:
Java boasts a slew of features and characteristics that differentiate it from other programming languages. Its simplicity, object-oriented structure, robustness, and security features are hallmarks that contribute to Java's enduring popularity. The key characteristic of Java's features is its extensive standard library that simplifies the development process and enhances productivity. This aids in reducing coding efforts, making it a highly beneficial language for developers of all levels. However, one disadvantage worth noting is Java's memory consumption, which can be higher compared to other programming languages. Despite this, the advantages of Java's features and characteristics far outweigh any drawbacks, making it an indispensable tool for developers.
Setting Up Java Development Environment:
Installing JDK:
Installing the Java Development Kit (JDK) is the first step in setting up the Java development environment. The JDK includes tools needed for Java programming, such as the compiler and debugger. By installing the JDK, developers gain access to the necessary components required to start writing, compiling, and executing Java code. The key characteristic of installing the JDK is its simplicity and cross-platform compatibility, making it a popular choice for developers across different operating systems. While the JDK offers numerous advantages, such as a vast set of tools and libraries, a potential disadvantage could be the disk space it consumes. Despite this, the advantages of the JDK outweigh any drawbacks, aligning with the goal of this article to provide a comprehensive Java programming guide.
Configuring IDE for Java Development:
Configuring an Integrated Development Environment (IDE) for Java development is crucial for enhancing the coding experience. IDEs like Eclipse or Intelli J IDEA offer features such as code autocompletion, debugging capabilities, and project management tools, streamlining the development process. The key characteristic of configuring an IDE is the increased productivity it brings to developers by providing a unified platform for coding and testing. This feature makes configuring an IDE a beneficial choice for this article as it emphasizes the importance of a conducive development environment. However, one potential disadvantage could be the learning curve associated with mastering all the features of a sophisticated IDE. Despite this, the advantages of configuring an IDE for Java development far outweigh any challenges, making it a valuable asset for Java programmers.
Writing Your First Java Program:
Creating a Basic Java Program:
Crafting a basic Java program is the gateway to mastering Java programming. By creating simple programs that display text or perform basic calculations, beginners can familiarize themselves with Java syntax and structure. The key characteristic of creating a basic Java program lies in understanding foundational concepts, such as variables, data types, and control structures. This simplicity makes creating a basic Java program a popular choice for introductory Java tutorials, offering a gentle introduction to programming paradigms. Despite its simplicity, a potential disadvantage could be the risk of developing rigid coding habits if beginners do not venture into more complex programming challenges. Nevertheless, the advantages of creating a basic Java program, such as developing problem-solving skills and logical thinking, make it a crucial step in the learning journey.
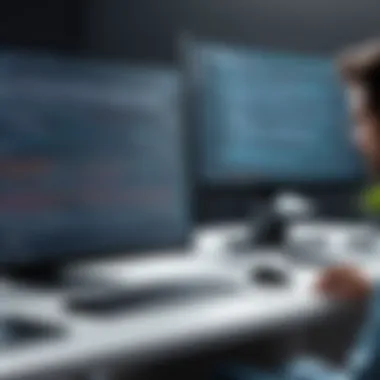
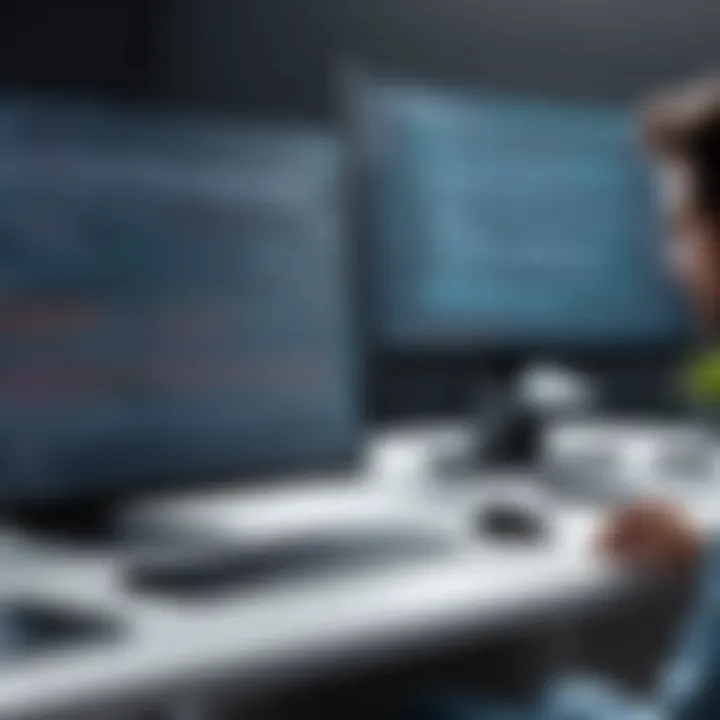
Compilation and Execution Process:
The compilation and execution process in Java involves translating human-readable code into machine-readable instructions and running the program on a Java Virtual Machine (JVM). Understanding how code is compiled and executed is essential for debugging and optimizing programs. The key characteristic of the compilation and execution process is its transparency, allowing developers to track errors and monitor program behavior efficiently. This transparency makes the compilation and execution process a beneficial choice for this article as it focuses on developing a robust understanding of Java programming fundamentals. However, a potential disadvantage could be the complexity of troubleshooting compilation or runtime errors, which may require advanced debugging skills. Despite this challenge, mastering the compilation and execution process is crucial for honing programming expertise and producing reliable Java applications.
Core Java Concepts
Core Java Concepts are the foundation of Java programming in this comprehensive guide. Understanding Data Types and Variables, Control Flow Statements, and Arrays and Collections are essential for mastering Java. These concepts serve as building blocks for developing robust and efficient Java programs, catering to both beginners and experienced developers.
Data Types and Variables
Primitive Data Types
Primitive Data Types play a crucial role in Java programming by defining the basic data types available in the language. They include int, double, char, and boolean, among others. Their simplicity and direct representation in memory make them efficient for performing operations and optimizing memory usage. However, their limitation lies in their fixed size, which can lead to potential overflow issues when handling large data sets.
Variables and Constants
Variables and Constants are fundamental elements in Java programming, enabling developers to store and manipulate data. Variables hold varying values during program execution, while constants maintain fixed values throughout the program. Their versatility allows for dynamic data handling and simplifies code maintenance by providing named references to values. However, improper variable declaration or excessive use of constants can impact code readability and maintenance, emphasizing the importance of proper variable management.
Control Flow Statements
Control Flow Statements dictate the flow of a Java program based on certain conditions and criteria. They include Conditional Statements and Loops, essential for implementing decision-making and repetitive tasks efficiently. Conditional Statements, such as if-else and switch-case, facilitate branching based on different conditions, enhancing program flexibility and responsiveness. Loops, including for, while, and do-while loops, enable iterative processes, reducing code redundancy and optimizing task execution. However, improper implementation of control flow statements can lead to logical errors and hinder program functionality, underscoring the need for precise logic and structure.
Conditional Statements
Conditional Statements in Java determine the flow of program execution based on specific conditions. By evaluating different scenarios, developers can control the program's behavior dynamically, allowing for complex decision-making processes. The versatility of conditional statements lies in their ability to handle multiple outcomes, enhancing code adaptability and responsiveness. However, nested or excessive conditional statements can complicate code readability and maintenance, necessitating strategic design and optimization for efficient program control.
Loops
Loops in Java are iterative structures that repeat a set of instructions until a certain condition is met. They provide a concise and efficient means of executing repetitive tasks, contributing to code modularity and reusability. Loops offer flexibility in handling varying data sets and streamlining computational processes, increasing program efficiency. Nonetheless, infinite loops or incorrectly defined loop conditions can lead to program crashes or performance issues, emphasizing the importance of accurate loop design and iteration management.
Arrays and Collections
Arrays and Collections are fundamental data structures in Java for storing and manipulating multiple values. Array Declaration and Initialization involve defining a fixed-size collection of elements, facilitating organized data storage and retrieval. Working with Array List and HashMap introduces dynamic data structures that enable flexible size management and efficient data access. Arrays are ideal for homogeneous data storage, while collections offer versatility in handling heterogeneous data types. However, improper array sizing or inefficient collection implementation can impact memory utilization and program performance, necessitating careful consideration and optimization strategies for effective data management.
Object-Oriented Programming in Java
Object-Oriented Programming (OOP) in Java serves as a fundamental pillar in this well-crafted guide on mastering Java programming. It plays a crucial role in organizing code, enhancing reusability, and promoting a structured approach to software development. By focusing on OOP concepts, readers delve into a paradigm that encapsulates data within objects, allowing for better control and modularity within their programs. Understanding OOP fosters the creation of efficient and scalable applications, making it an indispensable aspect of Java programming.
Classes and Objects
Defining Classes
In this section, the spotlight is on the essence of 'Defining Classes' within the realm of Java programming. This foundational concept lays the groundwork for creating blueprints that encapsulate data and behavior. The key characteristic of defining classes lies in its ability to define the structure of objects, incorporating attributes and methods essential for functionality. Such a practice promotes code organization and facilitates code reuse, making it a preferred choice for developers aiming for clean and structured codebases. Despite its benefits in promoting modularity and maintainability, the rigid structure of defining classes may pose challenges in scenarios requiring dynamic modifications.
Instantiating Objects
The process of 'Instantiating Objects' comes into play to bring classes to life within Java programs. By instantiating objects, developers create instances of classes, uniquely identifying isolated entities within the application. The characteristic feature of instantiating objects lies in its facilitation of individual instances with their own set of attributes and behaviors. This approach enhances code reusability and enables the management of multiple independent objects seamlessly. Choosing to instantiate objects reinforces the concept of object-oriented development, albeit at the cost of potentially higher memory consumption in projects requiring a multitude of instances.
Inheritance and Polymorphism
Concepts of Inheritance
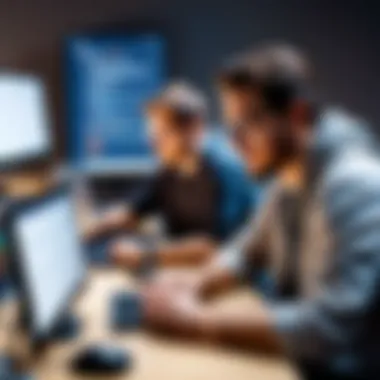
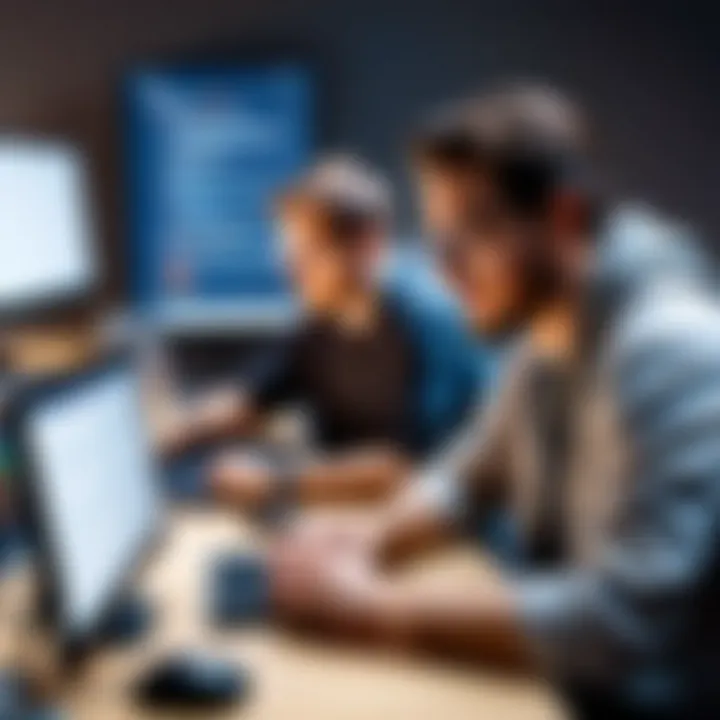
The concept of 'Inheritance' unfolds as a vital element within Java programming, enriching this guide with its scalability and code reusability benefits. Inheritance allows for the creation of hierarchical relationships between classes, paving the way for the extraction and extension of shared characteristics. The key characteristic of inheritance lies in its facilitation of the 'is-a' relationship, where subclasses inherit attributes and methods from superclasses. Embracing inheritance empowers developers to build upon existing code structures efficiently, promoting a DRY (Don't Repeat Yourself) approach to programming. However, an overreliance on inheritance can lead to overly complex class hierarchies, potentially hindering code maintainability.
Method Overriding
'Method Overriding' steps into the spotlight, emphasizing the flexibility and customization opportunities it offers within the realm of Java development. By overriding methods in subclasses, developers can tailor the behavior of inherited methods to suit specific requirements. The standout feature of method overriding lies in its ability to provide specialized implementations for methods inherited from parent classes, catering to diverse functionalities within the application. Opting for method overriding empowers developers to refine and adapt inherited behaviors, fostering code extensibility and tailor-made solutions. However, navigating multiple levels of method overriding can introduce complexities, requiring careful consideration to maintain code coherence and clarity.
Encapsulation and Abstraction
Encapsulation in Java
Encapsulation emerges as a cornerstone aspect in Java programming, contributing significantly to the integrity and security of codebases within this comprehensive guide. Encapsulation involves bundling data and methods within classes, shielding inner workings from external interference. The standout characteristic of encapsulation lies in its promotion of information hiding and access control, ensuring data privacy and maintaining code reliability. Embracing encapsulation enhances code maintainability and reduces dependencies, fostering modular and secure software development practices. Despite its advantages, overly stringent encapsulation can limit extensibility and hinder the interaction between components within the application.
Abstract Classes and Interfaces
The realm of 'Abstract Classes and Interfaces' offers a versatile approach to design and structure within Java programming, enriching this guide with its flexibility and contract-based implementations. Abstract classes and interfaces serve as blueprints for classes, defining methods and behaviors without implementation details. The key characteristic of abstract classes and interfaces lies in their promotion of design abstraction and contract adherence, providing a framework for consistent and structured class hierarchies. Integrating abstract classes and interfaces promotes code uniformity and enables multiple inheritance-like features through interfaces, fostering code adaptability and scalability. However, excessive abstraction through interfaces can lead to implementation complexities and potential obfuscation of code logic, requiring a balanced approach to design interfaces effectively.
Exception Handling and Multithreading
In the realm of Java programming, Exception Handling and Multithreading serve as indispensable components ensuring program stability and efficiency. Exception Handling is crucial for managing and resolving errors during program execution, averting abrupt crashes and ensuring graceful error handling. Multithreading, on the other hand, plays a pivotal role in improving performance by enabling concurrent execution of multiple threads within a program. Understanding these concepts is vital for developers aiming to create robust and efficient Java applications.
Handling Exceptions in Java
Try-Catch Blocks
Try-Catch Blocks are essential in Java programming for mitigating the impact of exceptions. By encapsulating potentially error-prone code within a Try block and providing instructions in the Catch block for handling exceptions, developers can prevent program disruptions. The key characteristic of Try-Catch Blocks lies in their ability to separate regular code from error-handling logic, promoting a clean and structured approach to managing exceptions. This makes Try-Catch Blocks a popular choice in Java programming for safeguarding the codebase and ensuring uninterrupted program flow. While Try-Catch Blocks excel in facilitating error management, they can introduce additional complexity to the codebase, requiring careful design and implementation to maintain code readability and efficiency.
Throw and Throws Keywords
In Java, the Throw and Throws Keywords play pivotal roles in exception propagation and handling. The Throw Keyword allows developers to explicitly raise and throw custom exceptions when certain conditions are met, facilitating precise error communication and resolution. On the other hand, the Throws Keyword indicates the possibility of an exception occurring within a method, necessitating proper exception handling by either propagating the exception up the call stack or handling it within the method. These keywords contribute significantly to Java programming by enhancing the clarity and maintainability of code, although improper usage may lead to code complexity and decreased readability.
Multithreading Concepts
Thread Creation
The concept of Thread Creation empowers developers to implement concurrent execution in Java programs, facilitating parallel processing and improved performance. By creating multiple threads to handle different tasks simultaneously, developers can optimize resource utilization and enhance program responsiveness. The key characteristic of Thread Creation lies in its ability to execute independent code paths concurrently, maximizing system efficiency and responsiveness. This makes Thread Creation a valuable choice for applications requiring enhanced performance and responsiveness. However, efficient thread management is essential to avoid resource conflicts and ensure seamless thread execution.
Synchronization and Deadlocks
Synchronization and Deadlocks are critical considerations in multithreaded Java programming. Synchronization ensures thread safety by coordinating access to shared resources, preventing data corruption and maintaining program integrity. Deadlocks, on the other hand, occur when multiple threads block each other's progress by holding resources and waiting for others. Understanding these concepts is paramount for developers to design robust and efficient multithreaded applications, striking a balance between concurrency and data consistency. While synchronization enhances data integrity and consistency, Deadlocks can lead to program stagnation and should be meticulously addressed to ensure uninterrupted program execution.
Advanced Java Features
In the realm of Java programming, delving into Advanced Java Features is a vital step towards honing one's expertise. These features serve as the building blocks for intricate software development and pave the way for creating robust applications. By understanding and implementing Advanced Java Features, developers can elevate their code efficiency, enhance application performance, and streamline intricate functionalities. With elements such as Generics, Annotations, Java IO, Networking, Frameworks, and Libraries, this section explores the advanced aspects that differentiate seasoned programmers from novices.
Generics and Annotations
Generics in Java
Generics in Java play a paramount role in object-oriented programming by offering a method to create generic classes, interfaces, and methods. The key characteristic of Generics lies in their ability to provide type-safety at compile time, ensuring code reliability and reusability. In this article, delving into Generics elucidates their capability to eliminate the need for type casting and enhance code readability. Despite their advantage of type-checking, Generics may pose challenges in dealing with primitive data types and enforcing constraints in certain scenarios.
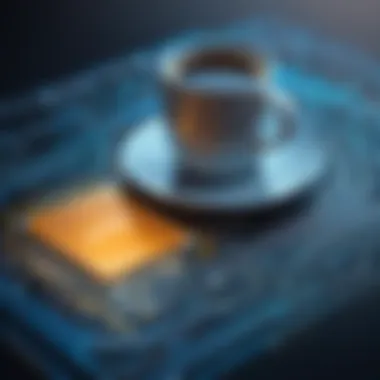
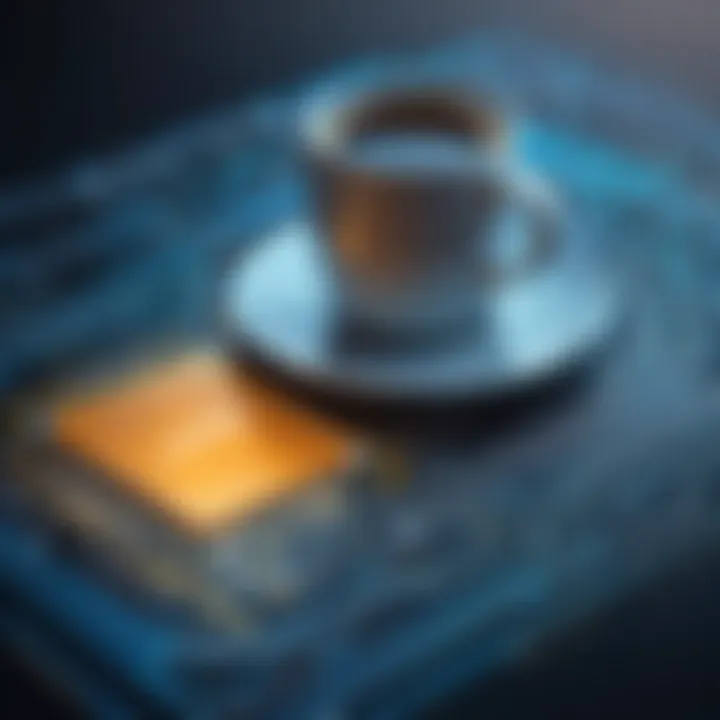
Annotation Types
Annotation Types in Java introduce metadata into the code, allowing developers to embed supplemental information for runtime processing. The distinct feature of Annotation Types is their ability to enable compile-time verification and runtime processing efficiencies, enhancing code organization and clarity. Discussing Annotation Types in this article sheds light on their versatility in marking declarations and enhancing code documentation. However, misuse or overuse of annotations can lead to code clutter and reduced readability, necessitating a judicious approach towards their implementation.
Java O and Networking
File Handling in Java
Efficient file handling is crucial in Java programming for tasks such as reading and writing data to files seamlessly. The key characteristic of File Handling in Java lies in its versatility to manipulate files, directories, and streams effectively. When integrating File Handling into applications, developers benefit from streamlined data storage mechanisms and simplified file operations. Exploring File Handling within this article unveils its utility in managing various file formats and facilitating data persistence. Nevertheless, improper file handling can lead to resource leaks and data corruption, emphasizing the importance of meticulous implementation.
Socket Programming
Socket Programming emerges as a fundamental aspect of networking in Java, enabling communication between devices over a network. The core characteristic of Socket Programming is its facilitation of reliable data transmission through sockets, fostering real-time interaction and data exchange. By delving into Socket Programming in this article, readers gain insights into establishing client-server connections and implementing network protocols. However, challenges such as handling multiple connections and network latency should be considered when leveraging Socket Programming for sophisticated applications.
Java Frameworks and Libraries
Introduction to Spring Framework
The Spring Framework stands out as a versatile tool for Java application development, with features such as inversion of control and aspect-oriented programming. A key characteristic of the Spring Framework is its modular structure, promoting code organization and scalability. Understanding the Spring Framework in this article showcases its capability in simplifying application configuration and fostering test-driven development. Despite its benefits, integrating the Spring Framework requires familiarity with its conventions and best practices to leverage its full potential.
Working with Hibernate ORM
Hibernate Object-Relational Mapping (ORM) simplifies database interaction in Java applications by mapping Java objects to database tables. The primary characteristic of Hibernate ORM lies in its capability to streamline data persistence and retrieval without writing complex SQL queries. Exploring Hibernate ORM within this article illuminates its role in enhancing database portability and abstraction. Nonetheless, aspects such as object-relational impedance mismatch and performance tuning should be considered to optimize the use of Hibernate ORM effectively.
Best Practices and Tips in Java Programming
When delving into the realm of Java programming, understanding and implementing best practices and tips is crucial for optimizing code efficiency and creating robust applications. This section focuses on highlighting key strategies that can elevate the quality of Java programs while facilitating smoother development processes. By adhering to best practices, developers can streamline their code, enhance performance, and minimize errors, ultimately leading to more maintainable and scalable software solutions.
Code Optimization Techniques
Efficient Memory Management
Efficient memory management plays a pivotal role in Java programming, ensuring optimal utilization of system resources and enhancing application performance. By efficiently managing memory allocation and deallocation, developers can prevent memory leaks and optimize the overall memory usage of their applications. The key characteristic of efficient memory management lies in enabling applications to run smoothly without excessive memory consumption, contributing to a seamless user experience. This approach is widely preferred in Java development for its ability to boost application speed, reduce overhead, and enhance system stability.
Reducing Code Complexity
Reducing code complexity is essential for simplifying development processes and enhancing code maintainability. By breaking down complex algorithms and structures into smaller, more manageable components, developers can improve code readability and scalability. The key characteristic of reducing code complexity is the ability to streamline program logic, making it easier to debug and modify. This practice is highly advantageous in Java programming as it promotes code reusability, increases efficiency, and reduces the likelihood of errors or bugs.
Debugging Java Programs
Using Debugging Tools
Utilizing debugging tools is fundamental in Java programming for identifying and resolving errors and malfunctions within code. These tools provide developers with valuable insights into program behavior, allowing them to diagnose and rectify issues efficiently. The key characteristic of using debugging tools is the ability to pinpoint specific areas of code that require attention, streamlining the debugging process. This practice is immensely beneficial in Java development for ensuring code reliability, improving software quality, and enhancing the overall debugging experience.
Handling Runtime Errors
Effectively handling runtime errors is crucial for maintaining the stability and performance of Java applications. By implementing robust error-handling mechanisms, developers can anticipate and manage unforeseen exceptions, preventing application crashes and data loss. The key characteristic of handling runtime errors lies in gracefully managing unexpected situations, ensuring application continuity and user satisfaction. This practice is essential in Java programming to promote application resilience, facilitate troubleshooting, and mitigate risks.
Performance Tuning Strategies
Improving Code Efficiency
Enhancing code efficiency is a cornerstone of performance tuning in Java programming, enabling applications to deliver optimal speed and responsiveness. By optimizing algorithms, reducing redundant operations, and enhancing data structures, developers can significantly improve the overall performance of their code. The key characteristic of improving code efficiency is the ability to minimize resource utilization while maximizing output, enhancing application speed and responsiveness. This practice is highly advantageous in Java development for boosting productivity, optimizing resource usage, and enabling scalability.
Monitoring Application Performance
Monitoring application performance is vital for evaluating system health, identifying bottlenecks, and optimizing resource allocation. By employing monitoring tools and performance metrics, developers can track system behavior in real-time, detect performance issues, and implement timely optimizations. The key characteristic of monitoring application performance is the ability to assess system performance and identify opportunities for enhancement, ensuring efficient resource allocation and optimal application functionality. This practice is essential in Java programming for proactive performance management, continuous improvement, and seamless scalability.