Mastering Java Programming: Essential Concepts Explained
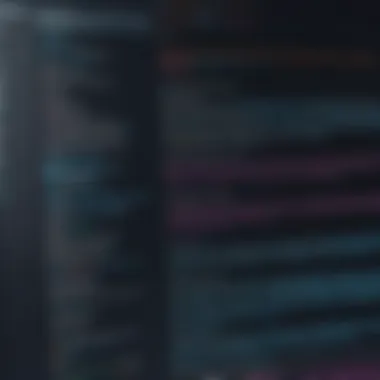
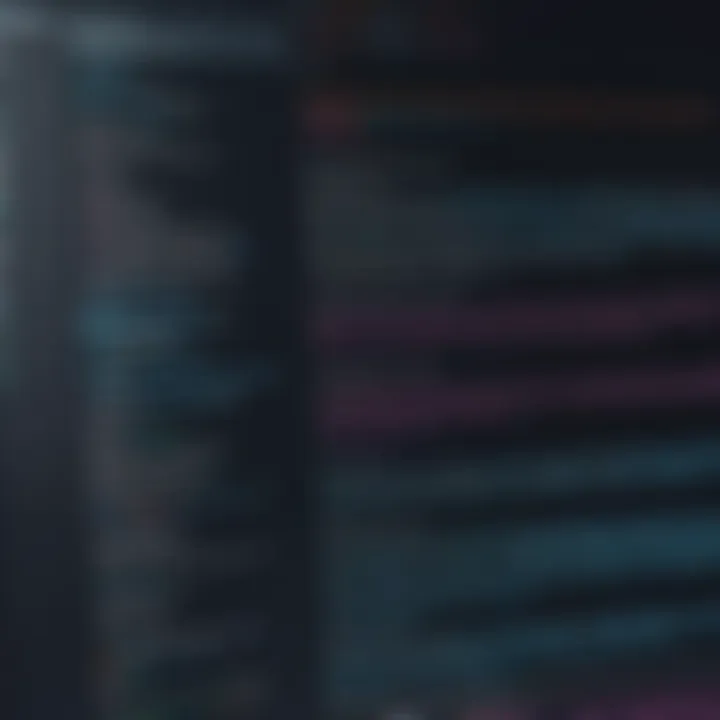
Overview of Topic
Introduction to the main concept covered
Java is a powerful and versatile programming language that has become a staple in software development. With its platform independence and robustness, it serves as a critical foundation for many applications ranging from mobile apps to large-scale enterprise systems. This guide aims to lay bare the essentials of Java programming, ensuring you grasp the core concepts needed to embark on your coding journey.
Scope and significance in the tech industry
The relevance of Java in todayâs tech industry cannot be overstated. According to stats, Java remains among the top three most used programming languages worldwide, making it a primary choice for backend development, web applications, and Android programming. Given its strong presence, understanding Java opens countless doors in a competitive job market.
Brief history and evolution
Java was developed by Sun Microsystems in the mid-1990s, with the primary goal of creating a language that could run on any device without the need for complex recompilation. Over the years, Java has evolved significantly, from early versions focusing on applets to the modern iterations which emphasize scalability and security, shaping the way we think about software design today.
Fundamentals Explained
Core principles and theories related to the topic
At the heart of Java programming lies the concept of object-oriented programming (OOP). OOP is built around four primary principles: inheritance, encapsulation, polymorphism, and abstraction. Each of these principles helps in creating more manageable and reusable code, which is essential when dealing with complex systems.
Key terminology and definitions
To navigate the Java programming landscape effectively, it is crucial to familiarize yourself with basic terms:
- Class: A blueprint for creating objects, defining attributes and methods.
- Object: An instance of a class, representing a real-world entity.
- Method: A function defined within a class to perform specific tasks.
- Variable: A container for storing data values.
Basic concepts and foundational knowledge
Learning Java is about understanding its syntax and core structures. Java uses a C-like syntax, which is relatively straightforward but requires precise attention to detail. The main method is the entry point of any Java application, and itâs where execution begins. Understanding the syntax rules, such as how to define variables, how to use loops, and how to implement control structures will set the groundwork for all future programming efforts.
Practical Applications and Examples
Real-world case studies and applications
Java finds applications in numerous real-world scenarios, from fostering mobile applications on Android to powering backend services for major companies like Google and Netflix. Moreover, financial institutions often rely on Java due to its security features, ensuring that sensitive transactions are handled with care.
Demonstrations and hands-on projects
A practical approach helps consolidate the theoretical knowledge gained. Start by creating simple console applications that may include a calculator or a to-do list manager. These projects will shed light on handling user input, controlling flow with decisions and loops, as well as effectively utilizing classes and objects.
Code snippets and implementation guidelines
Hereâs a basic code snippet to illustrate a simple Java program that prints "Hello, World!":
This code defines a class named and runs the method to display the string on console.
Advanced Topics and Latest Trends
Cutting-edge developments in the field
In recent years, features like lambda expressions and streams have transformed Java programming. These features enable more concise code and facilitate functional-style programming, letting developers handle complex data manipulations with ease.
Advanced techniques and methodologies
As you progress, familiarize yourself with concepts such as multithreading, which allows concurrent processing and enhances application performance. Another noteworthy area is Java frameworks, such as Spring and Hibernate, designed for simplifying the development of enterprise-level applications.
Future prospects and upcoming trends
With ongoing advancements in cloud computing and big data, proficiency in Java remains vital. The language continues to adapt to the evolving tech landscape. Learning about microservices and their interactions with cloud platforms will give aspiring developers an edge.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
To deepen your understanding, several resources can be invaluable:
- "Effective Java" by Joshua Bloch
- "Head First Java" by Kathy Sierra and Bert Bates
- Online platforms like Codecademy and Udemy provide structured courses tailored for beginners.
Tools and software for practical usage
An integrated development environment (IDE) is crucial for programming efficiently. Popular options like Eclipse and IntelliJ IDEA offer robust features for editing, debugging, and running Java applications, significantly enhancing your coding experience.
Foreword to Java Programming
Java has become a cornerstone in the realm of programming languages over the years, proving itself to be more than just a passing fad. It's crucial to grasp the significance of introducing Java at this stage, as it opens up a door to endless possibilities for budding developers and seasoned professionals alike.
The realm of Java programming is not merely about writing lines of code. It's about understanding the logic behind the programs and how they interact with the real world. From mobile applications to enterprise systems, knowing Java can pave the way for various career opportunities. Plus, its object-oriented nature simplifies complex problems by breaking them down into manageable chunks.
As we embark on this journey through the fundamentals of Java programming, consider the following key elements:
- Versatility: Java can run on any device with the Java Virtual Machine (JVM), making it platform-independent.
- Strong Community Support: A vast community surrounds Java, ensuring that learners can find help and resources with ease, spanning forums like Reddit and countless online tutorials.
- Robust Libraries and Frameworks: Java boasts a multitude of libraries and frameworks that accelerate development, allowing programmers to focus on creating innovative solutions rather than reinventing the wheel.
Taking the plunge into Java programming might seem daunting, but it offers extensive benefits that far outweigh the challenges. Remember, the journey of a thousand lines of code begins with just one. This guide will walk you through the essentials, helping you build a strong foundation in this powerful language.
"Java is to JavaScript what car is to carpet." â Chris Heilmann
Through the course of this article, various aspects will be uncovered, such as what exactly Java entails, its historical evolution, and the key features that set it apart from other programming languages. By the end, you will possess a rounded understanding that arms you with the knowledge necessary to embark on your Java programming journey.
Setting Up the Java Development Environment
Setting up the Java Development Environment is a pivotal step in your journey as a Java programmer. The importance of having a proper environment cannot be overstated, as it directly influences your productivity and the overall experience you have while coding. A well-configured environment not only allows you to write and test your code efficiently but also helps in troubleshooting and understanding the fundamentals of Java programming more deeply.
Installing Java Development Kit (JDK)
The Java Development Kit, commonly referred to as JDK, is essential for anyone looking to develop applications in Java. It provides the necessary tools to create, compile, and run Java programs. Installing the JDK is straightforward:
- Download the JDK: Head over to the official Oracle website or OpenJDK to download the version that suits your needs. Ensure that you choose the right architecture, either x64 or x86, based on your machine.
- Run the Installer: Once downloaded, run the installer. During installation, it may be advisable to ensure the option for setting up the environment variables for you is checked, although it can be done manually later.
- Set Environment Variables: To compile Java from the command line, you'll need to set your environment variable pointing to the folder where the JDK is installed. Additionally, you'll want to update the variable to include the folder within your JDK installation. This allows you to run Java commands from any terminal window.
After installation, you can confirm that everything is set up correctly by opening a command prompt and typing:
If successful, this command will display the installed version of Java.
Choosing an Integrated Development Environment (IDE)
The right Integrated Development Environment can make a world of difference in your programming journey. An IDE streamlines the development process by providing features like code completion, syntax highlighting, and debugging tools.
Some popular choices among Java developers include:
- Eclipse: Known for its powerful features and extensibility through plugins.
- IntelliJ IDEA: Often praised for its smart code completion and other advanced features. A large portion of professionals in the field choose this due to its efficiency.
- NetBeans: A user-friendly IDE that is ideal for beginners and includes features out of the box, supporting various languages besides Java.
When selecting an IDE, consider factors such as your familiarity with the tool, the features you need, and community support. Most importantly, choosing one that aligns with your learning style will enhance your coding experience significantly.
Configuring Your Environment
Once you have installed the JDK and chosen your IDE, itâs time to configure your environment for optimal development. This includes setting preferences and integrating version control systems, which are key elements in modern software development.
- Configuring the IDE: Each IDE has different settings. Take the time to explore them, customizing themes, formatting options, and the project structure to match your preference.
- Integrating Version Control: Familiarizing yourself with version control systems like Git can be beneficial. Integrate Git with your IDE for easier source code management. This allows for collaborative work and helps keep track of the changes in your codebase.
- Testing Your Setup: After everything is set, writing a simple "Hello, World!" program can help ensure your environment is correctly configured. Here's how:
When you run this program, if it outputs "Hello, World!", you've successfully set up your development environment.
"A good environment is the foundation of productive coding; it shapes how we write, run, and debug our programs."
Setting up your Java development environment may seem like a tedious task initially, but it serves as a roadmap for your learning. With everything in place, you can focus on what truly mattersâcoding and honing your skills.
Basic Syntax and Structure of a Java Program
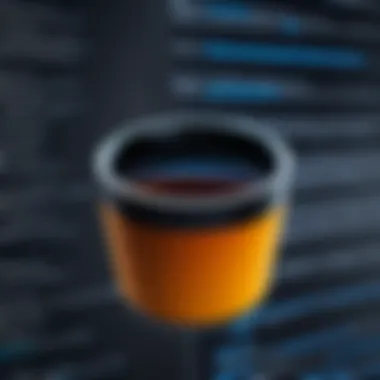
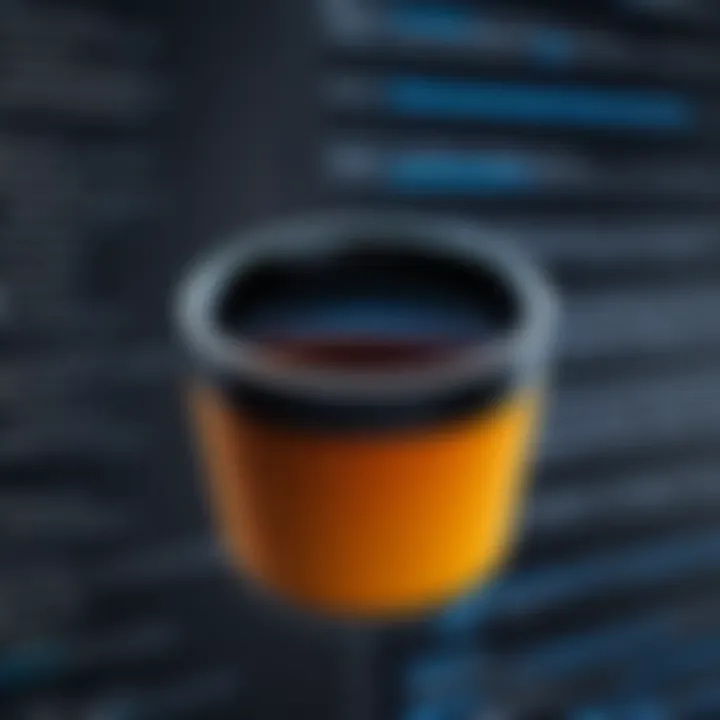
When diving into Java programming, grasping the basic syntax and structure of a Java program is crucial. This foundational knowledge acts like the bedrock for understanding more complex concepts later on. If you envision Java as a language, the syntax comprises the grammar and vocabulary you need to communicate effectively with the Java Virtual Machine, or JVM. Without a firm grasp of the basics, one's journey in programming might feel like navigating through foggy watersâunclear and disorienting.
Understanding the Main Method
Every Java application must have a method. This is the entry point of any standalone Java program. Itâs here where the execution begins. The signature of the main method looks like this:
Let's unpack that. The keywords and serve important roles. means that the method can be accessed from anywhere, while indicates that this method belongs to the class, rather than to any specific instance of the class. The specifies that this method doesn't return any value. Finally, the parameter comes into play, allowing data to be passed into the method from the command line.
In simpler terms, think of the main method like the front door of a houseâeveryone must go through it to get inside. If this door isn't included, your home (or program, in this case) won't function.
Comments in Java Code
In programming, comments are akin to whispering helpful hints to future readers of your code. They donât affect the program itself but enhance understanding. In Java, comments can be single-line or multi-line.
- Single-line comments start with and apply to the rest of the line.
- Multi-line comments are surrounded by and , allowing multiple lines to be commented out.
Example:
Utilizing comments judiciously can streamline your code and facilitate collaboration with others. A well-commented code base allows fellow codersâor even your future selfâto easily grasp what the original programmer intended. Ignoring comments will make one's code more akin to a puzzle with missing pieces.
Compiling and Running a Java Program
Compiling and running a Java program is like preparing a dish and then serving it. First, you need to compile the program to check for errors and create a .class file which the JVM understands. The command to do this is:
Next, to execute the compiled program, you would type:
Letâs break this down further:
- is the Java Compiler that transforms your source code (.java file) into bytecode (.class file).
- runs the bytecode generated by the compiler.
The difference between compiling and running is significant. Compiling checks for syntax errors and creates an executable file, whereas running carries out the program's instructions.
The entire process ensures that what you programmed behaves as intended, much like one double-checking a recipe before serving.
Understanding these facets of Java syntax lays a solid groundwork for future exploration of programming constructs. As you build more complex applications, you will find that mastering the basics can save a lot of time and headaches down the line.
Data Types and Variables
Data types and variables are the backbone of any programming language, and Java does not stray from this essential truth. Understanding how data is categorized and stored, and how to manage this data effectively aids in writing efficient and robust programs. This section will disentangle the concepts of primitive data types, how to declare and initialize variables, and the significance of variable scope and lifetime. Each concept plays a vital role in handling information and crafting responses from your Java programs.
Primitive Data Types
In Java, primitive data types are the most basic forms of data that can be used in programming. Unlike objects, these types don't carry any methods; rather, they hold simple values. Java has eight primitive data types:
- byte: 8-bit signed integer, suitable for saving memory in large arrays.
- short: 16-bit signed integer.
- int: 32-bit signed integer, a widely used data type for number storage.
- long: 64-bit signed integer for a wider numerical range.
- float: single-precision 32-bit floating point for decimal numbers.
- double: double-precision 64-bit floating point, used when more precision is needed.
- char: single 16-bit Unicode character.
- boolean: represents only two values: or .
Each type serves a unique purpose. Choosing the right one is not just about convenience; it's also about optimizing memory and performance. For instance, using where a could suffice wastes memory on larger arrays, a critical consideration in large-scale applications. Always think twice about it!
Declaring and Initializing Variables
To work with variables in Java, you must first declare them, specifying their data type. A variable declaration tells the compiler about the variable's name and type. Here's the simple syntax for variable declaration:
Once declared, you can choose to initialize it at the same time:
Proper initialization is essential as it helps to avoid compilation errors. If you try to utilize a variable without initializing it, you will hit a roadblock. Java will raise an error, as the variable is regarded as uninitialized. To prevent confusion, always initialize your variables when you declare them.
Variable Scope and Lifetime
Understanding variable scope and lifetime is crucial for efficient programming. In Java, the scope of a variable determines where it can be accessed within your code, while lifetime refers to how long the variable maintains its value.
There are generally four scopes for variables in Java:
- Local variables: These are declared within a method and can only be accessed inside that method. Their lifetime is tied to that method being called.
- Instance variables: Found within a class but outside any method, these variables are unique to an instance of a class and exist as long as the object exists.
- Static variables: Declared with the keyword, these belong to the class rather than any object instance. They exist for the duration of the program.
- Parameters: Variables passed into methods. Their scope is limited to the method they're declared in.
Understanding these scopes can prevent unexpected behaviors in your programs, such as variable shadowing or unintended modifications. Keep track of where and how your variables are defined.
"A variableâs scope can always be a good friend, helping to keep things organized. And its lifetime can be a bit of a double-edged sword, so handle it with care!"
By mastering data types and variables, you're laying the groundwork needed to engage effectively with more complex topics in Java programming. Remember, successful programming comes down to managing data smartly.
Control Structures in Java
Control structures are a vital aspect of programming in Java, shaping the way your code makes decisions, repeats tasks, and manages complex flows. They are the building blocks that allow a computer to make choices based on conditions, turning a simple sequence of instructions into a dynamic and interactive program.
The relevance of control structures cannot be understated. When writing applications, you're often faced with scenarios that demand conditional logicâlike checking data validity or informing the user based on input. Indeed, these structures are what help make Java a powerful and flexible language. They not only enable program control in terms of logic and behavior but also contribute to the clarity and maintainability of your code.
Conditional Statements
Conditional statements permit programs to branch out, performing different actions based on whether a condition is true or false. Essentially, this is where the decision-making occurs.
Key Types of Conditional Statements:
- if statements: The simplest form to execute a code block if a condition is true.
- if-else statements: Offers a fallback, executing one block of code if trueâotherwise, it executes another.
- else if ladders: Enables multiple conditions to be checked in sequence.
Example of a basic statement:
In this snippet, the program checks the value of . If it's greater than or equal to 18, it prints that the user is an adult; otherwise, it states they are not. Such conditions add functionality to your applications, making them responsive to various scenarios.
A nuanced use of these statements can influence the application's behavior, allowing it to adapt to user inputs dynamically.
Loops and Iteration
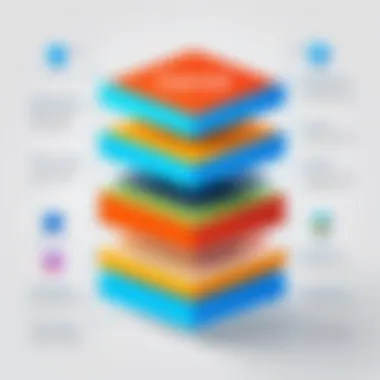
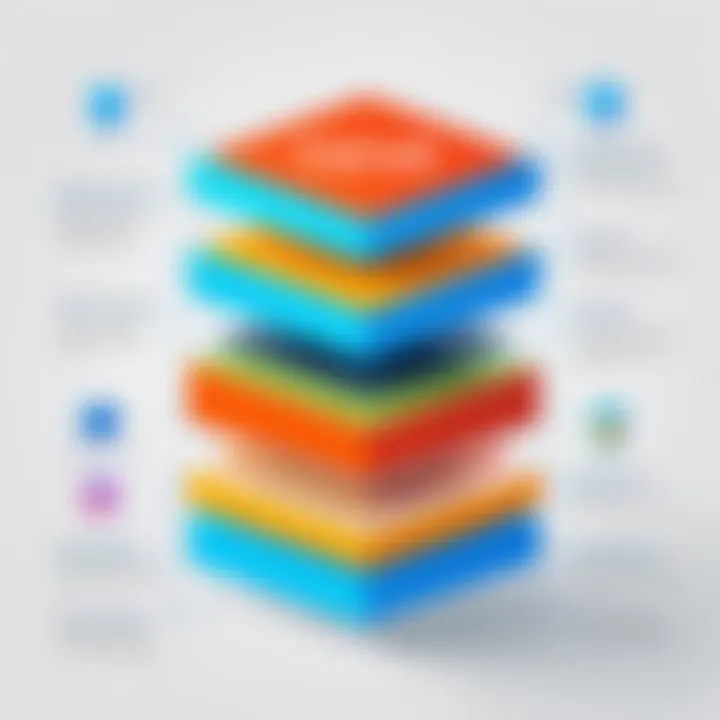
Loops are another critical control structure in Java. They allow a programmer to repeat a set of instructions until a specific condition is met. This is particularly useful for tasks that involve repetitive actions, such as processing items in a data collection.
Types of Loops:
- for loop: Best for scenarios when the number of iterations is known beforehand.
- while loop: Useful when the number of iterations isn't clear and depends on a condition.
- do-while loop: Similar to a while loop, but ensures the code block executes at least once.
Here's a simple example using a loop:
In this instance, the program iterates five times, printing the iteration count. This featureâs strong point lies in its ability to handle repetitive tasks elegantly, helping maintain clean and succinct code.
Moreover, proper understanding of loops can lead to efficient algorithm implementations, saving resources and processing time.
Switch Case Statement
The switch case statement is an alternative to multiple statements, especially when dealing with discrete values like integers or strings. Itâs typically more readable and can be easier to manage when handling numerous conditions.
How it Works:
A switch statement evaluates a variable, matching it against various cases. If a match is found, it runs the corresponding block of code and, if necessary, exits the structure.
Example:
In this example, the program looks at the value of , finds the match, and prints Wednesday. Failure to use could lead to fall-through behavior, causing subsequent case statements to run unintentionally.
Ultimately, control structures in Javaâconditional statements, loops, and switch case statementsâempower developers to create logic that mimics real-world decision-making. By mastering these structures, you not only improve your coding skills but also enhance the user experience by making applications more functional and engaging.
"Control structures are not just tools; they are the language of logic that translates ideas into executable steps."
Through understanding and applying these concepts, you build a foundation where complex applications can evolve.
Object-Oriented Programming Concepts
In the world of programming, the concept of Object-Oriented Programming (OOP) stands as a pillar that supports the creation of robust and scalable software systems. Understanding OOP is not just a step; itâs like finding the hidden map that leads to effective coding practices. Java, as a language designed with OOP principles, helps programmers articulate their ideas through models that reflect real-world entities. This approach enhances code reusability, maintainability, and organization, allowing developers to build systems that are easier to understand and modify over time.
OOP revolves around four primary concepts: classes, inheritance, polymorphism, encapsulation, and abstraction. Getting a grip on these ideas is crucial for anyone stepping into the world of Java. They allow developers to structure their program logically, making it more relatable and intuitive.
Letâs dive deeper into these concepts, starting with the very building blocks of OOP:
Classes and Objects
At the heart of OOP lies the notion of classes and objects. A class can be thought of as a blueprint for creating objects. It defines the properties (attributes) and behaviors (methods) that the created objects will possess. For example, you might have a class called . This class would have attributes like , , and , as well as methods like and .
Objects, on the other hand, are the instances of classes. When you create an object from a class, you are effectively saying, "Letâs build a specific using the general characteristics defined in the class."
This succinctly illustrates how you can use classes and objects to encapsulate data and functions related to specific things in your program. The beauty of this approach is that as you build your system, you can create many objects from the same class, sharing its properties and functions while being distinct entities.
Inheritance and Polymorphism
Inheritance is a powerful feature of OOP because it allows one class to inherit properties and behaviors from another class. This not only saves time but also promotes code reusability. For instance, if you have a class called , you might derive a subclass called . The class can inherit all the features of the , while also adding unique attributes specific to cars.
Polymorphism magnifies the power of inheritance. It allows one interface to be used for a general class of actions. The exact action is determined by the exact nature of the situation. For example, a method named might behave differently based on whether itâs called on a , , or object.
The code shows how calling from a type reference can lead to different behaviors depending on the object type. Itâs an elegance of design that keeps programs dynamic and flexible.
Encapsulation and Abstraction
Encapsulation is sticking all data (variables) in one place along with the methods that manipulate those data. Itâs like wrapping something up in a box, keeping it safe and sound while ensuring that the necessary access points are there for anyone who needs it. In Java, this is typically achieved using access modifiers like , , and . By restricting direct access to some of an objectâs components, you can prevent unintended interference and misuse of the methods and data.
Abstraction, on the opposite side, focuses on hiding the complexity while exposing only the essential features. Think of it as an interface that lets users interact with some functions without needing to know the intricate details of the implementation. You might use abstract classes or interfaces in Java to achieve this.
"In OOP, each class, method, and object tells a part of the story, shaping the software as a masterpiece of design and engineering."
For further exploration of Javaâs OOP characteristics, consider checking resources at Wikipedia) or engaging in discussions at Reddit.
Functions and Methods
Understanding functions and methods within the Java programming language is paramount for anyone looking to write efficient and maintainable code. This section will dig deep into the concepts surrounding these fundamental building blocks. Functions and methods help in breaking down complex programming tasks into manageable parts, allowing programmers to write code that is clean and easy to debug. Moreover, they promote code reusability, enabling developers to call upon existing methods rather than rewriting code each time they need a particular functionality.
Defining Methods in Java
In Java, a method is a block of code designed to perform a specific task. When defining methods, you need to specify four key components: the access modifier, the return type, the method name, and parameters if applicable. Hereâs a basic structure of a method:
Take for example a simple method that calculates the square of a number:
By being clear about how you define methods, you bolster not just your code's eloquence but also facilitate easier maintenance in the long run.
Method Overloading
One of the more intriguing features of Java is method overloading, which allows multiple methods to have the same name but different parameters within the same class. The critical factor is the method signature, which comprises the method's name and the parameter list. This feature enriches the language and adds flexibility to your code.
For example, you might have two overloaded methods:
In this way, depending on the types of arguments passed in, Java can determine which method to invoke. It makes for a more intuitive and streamlined programming experience, and it significantly enhances readability.
Return Values and Parameters
Every method in Java is defined by its ability to return a value or not, the return type playing a key role in this functionality. If your method intends to return a value, as shown previously, it must declare the return type. Conversely, if no value is returned, you'll define the return type as .
Parameters, on the other hand, serve as input to methods, acting as variables that hold values while the method executes. A method can have zero or more parameters, making them versatile and adaptable to various scenarios.
When it comes to calling a method, ensure the arguments you provide match the type and number of parameters defined. An example:
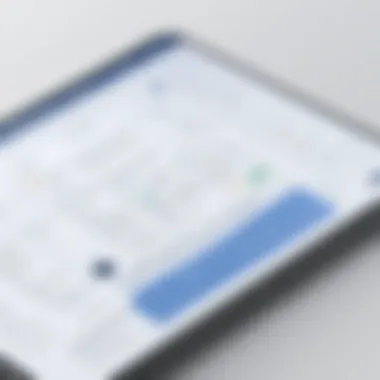
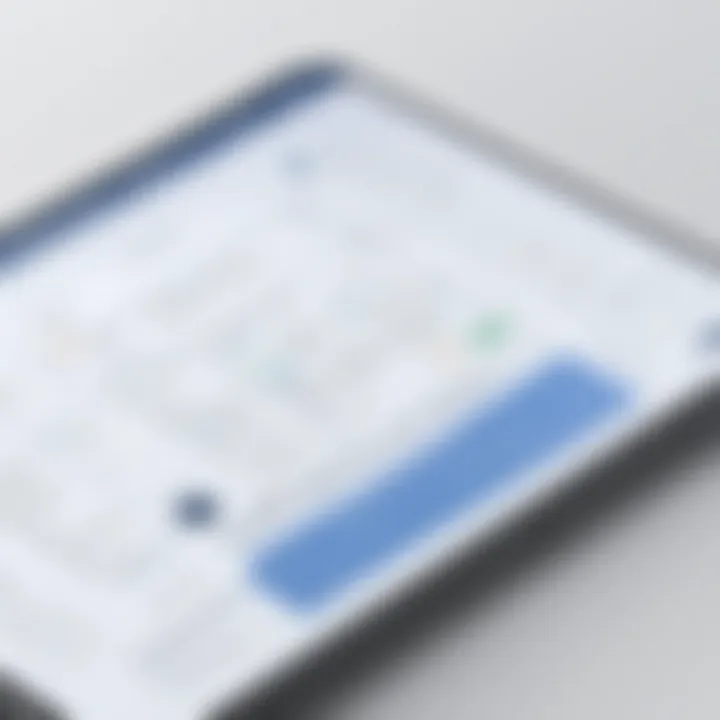
This line calls the method with as an argument, demonstrating how values flow into methods and what becomes of them afterward.
As methods encapsulate code for specific functionalities, they promote a clear separation of concernsâkeeping things tidy in your code and allowing for easier testing and modification later on.
In summary, mastering functions and methods in Java not only empowers you with the knowledge needed to tackle programming challenges efficiently, but it also helps in enhancing code readability and maintainability. Keep practicing with different examples to internalize these concepts, and you'll find they become second nature as you delve deeper into Java programming.
Error Handling and Debugging Techniques
Error handling and debugging are crucial components in the life cycle of software development, especially when it comes to Java programming. Any seasoned programmer will tell you that no code is flawless on the first run. Mistakes, often called bugs, can crop up anywhere, from the simplest conditional statements to the more complex methods of object-oriented design. Thus, understanding how to effectively manage errors and troubleshoot issues is paramount for developers who wish to create robust applications.
In this section of our guide, we delve into the significance of error handling and debugging. With Java being a statically typed language, errors can frequently arise when data types conflict, leading to unexpected behavior. Knowing how to handle scenarios where things don't go as planned can save both time and resources.
Understanding Exceptions
In Java, exceptions are events that disrupt the normal flow of a program. When something goes wrongâlike trying to divide a number by zero or referencing an object that doesn't existâJava generates an exception. Essentially, an exception is like a red flag that alerts the program (and the programmer) that something's amiss.
Dealing with exceptions is a fundamental skill. Here are a few categories to keep in mind:
- Checked Exceptions: These are exceptions that the compiler forces you to handle, as they are checked during compile time. For instance, trying to read a non-existent file would trigger a .
- Unchecked Exceptions: These include runtime exceptions like , which the compiler does not require you to catch. It's crucial to use good practices to avoid them altogether.
By understanding exceptions, you can better anticipate the bumps in the road that might come up while coding, enabling you to write cleaner, more reliable Java programs.
Using Try-Catch Blocks
One of the most effective techniques for handling exceptions in Java is the use of try-catch blocks. This is where you try to execute a block of code, and if an exception occurs, control is transferred to a catch block. Itâs a structured way to manage the unpredictability of programming and maintain program stability.
Here's how it works:
In this example, instead of crashing the program when it hits a division by zero, the catch block captures the exception and provides a user-friendly message. This not only aids in debugging but also enriches the user's experience by preventing abrupt program terminations.
Benefits of using try-catch:
- Gracefully handle exceptions without crashing.
- Enhance user experience by providing informative error messages.
- Maintain flow in an application even when unexpected errors occur.
Debugging Strategies
Debugging is a systematic process for identifying and removing errors in your code. Itâs an essential skill that every developer must hone to effectively maintain and enhance applications. Here are several strategies that can aid in efficient debugging:
- Print Statements: A classic method, but donât underestimate a few well-placed print statements to trace variable values and program flow.
- Integrated Debugger Tools: Most modern IDEs, like Eclipse and IntelliJ IDEA, come equipped with built-in debuggers. These tools let you run your program step-by-step, inspect variables, and evaluate expressions!
- Unit Testing: Prepare your code for unit testing using frameworks like JUnit. These tests can both find bugs and ensure that recent changes donât break existing functionality.
- Code Reviews: Having a second pair of eyes can often catch issues you might miss yourself. Pair programming or regular code reviews can enhance code quality.
Debugging is like being the detective in a crime movie where you are also the murderer.
For more on exceptions and debugging practices, check out the Java Exceptions documentation for a deeper dive into the subject.
Building a Simple Java Application
Creating a simple Java application is a fulfilling exercise that encapsulates the theoretical knowledge one has accumulated. It is not just about writing the code; it encompasses planning, designing, coding, testing, and finally deploying the application. Each step serves as a critical building block that allows a developer to understand the bigger picture behind Java programming. This section emphasizes the importance of grasping the entire software development lifecycle, which ultimately equips one with the skills necessary to tackle complex projects.
Planning Your Application
Before you dive headfirst into writing code, careful planning is paramount. Planning your Java application can save you from future headaches, guiding you through potential pitfalls. Consider the following aspects during the planning phase:
- Identify the Purpose: What does your application aim to achieve? Whether itâs a simple calculator or a to-do list manager, defining its purpose will help you narrow down the requirements and features.
- User Requirements: Who will use your application? Gather feedback from potential users; understanding their needs can greatly influence the design and functionality.
- Create a Flowchart: Visualize how different components interact. Flowcharts help in determining the flow of control within the application, making it easier to outline its structure.
Having identified these elements, you can proceed with a clear vision. Itâs almost like having a roadmap before embarking on a journey.
Writing the Code
Once planning is solidified, itâs time to put pen to paperâwell, fingers to keyboard. Writing the code involves implementing what was outlined during the planning stage. Here are some essential points to keep in mind:
- Start Small: Begin with the main features. Incremental development, rather than trying to build everything at once, helps in managing complexity.
- Follow Good Coding Practices: Code readability canât be overstated. Use meaningful variable and method names. Keep your code organized to ensure others (or you in the future) can understand it quickly.
- Use Version Control: Employ Git or another version control system. This provides a safety net for changes and allows you to track progress.
Hereâs an example snippet:
This simple calculator program demonstrates fundamental Java syntaxâdefining a class, utilizing the main method, and basic arithmetic.
Testing and Deployment
Testing is where you ensure everything is working as expected. Itâs essential to identify and fix bugs before your application goes live. Consider these steps:
- Unit Testing: Test each part of your application independently. This ensures that individual components function properly.
- Integration Testing: Once each unit has been tested, see how they work together. This phase checks for interface issues among components.
- User Acceptance Testing (UAT): Get feedback from actual users. They may discover flaws or suggest improvements you hadn't considered.
Deployment is wrapping it all up. Once the testing phase is completed, you can share your application with the intended audience. However, prepare for maintenance. Users might face issues or seek features that were never planned. Being responsive to this feedback helps you grow and improve your application.
"All software at some point returns to shadows, but through planning, coding, testing, and more, we can shine a light on our applications in the users' hands."
In summary, building a simple Java application not only reinforces your programming skills, but it also ingrains discipline and best practices that are vital for a successful developer. With every application you create, you cultivate a stronger grasp of Java, which lays the foundation for tackling more complex projects in the future.
End and Next Steps in Java Programming
Wrapping up your journey through Java programming fundamentals, itâs crucial to reflect on the significance this section carries. This conclusion isnât merely a closing statement; rather, it serves as a pivotal point to consolidate your learning and to guide you toward the next stages of your programming endeavor. Coming out of this guide, you have been equipped with a rich arsenal of knowledge â from the very basics of Java syntax to the intricate concepts of object-oriented programming. These building blocks are not just academic; they are what you'll leverage in real-world applications.
Recap of Key Concepts
In revisiting the essential elements discussed, hereâs a succinct overview:
- Basic Syntax: You learned how to structure a Java program and the importance of the main method, which is the entry point for any Java application.
- Control Structures: Grasping conditional statements and loops has better armed you to make decisions in your code.
- Object-Oriented Programming: Understanding classes, objects, inheritance, and encapsulation laid the groundwork for efficient code organization.
- Error Handling: You know how to anticipate issues in your code using exceptions and try-catch blocks, which is invaluable for maintaining code stability.
- Building Applications: Having practical experience through the simple Java application project gave life to the theoretical concepts.
These core themes are a treasure trove of tools to carry forward. The more you practice, the more fluent you'll be in Java programming.
Resources for Further Learning
Now, whatâs next? Thereâs a vast ocean of knowledge beyond what youâve covered. Here are some excellent resources that can provide further insight and skill enhancement:
- Java Documentation: The official and comprehensive resource for all Java libraries.
- Codecademy Java Course: An interactive platform that can help solidify your understanding with hands-on tasks.
- Coursera Java Courses: Offers numerous courses from esteemed universities and institutions.
- Stack Overflow: Engage with a community of developers where questions can be raised and solutions shared.
- Reddit: A vibrant place to connect with other learners and professionals to share experiences and seek advice.
Encouragement to Continue Practice
It's essential to remember that learning programming is a continuous journey. Theoretical knowledge takes you only so far. Begin to tackle personal projects, contribute to open-source applications, or perhaps delve into programming challenges on platforms like LeetCode or HackerRank.
Hereâs the key takeaway: Don't allow any setbacks to discourage you. Each error encountered is a stepping stone to mastery. Maintaining curiosity and a hunger for problem-solving will serve you well in the tech world. Keep coding, keep exploring, and most importantly, enjoy the creative process of programming. Remember, with every line of code, you're not just writing; youâre creating something valuable.
"The only limit to your impact is your imagination and commitment."
As you venture forth, understanding that programming is as much about creativity as it is about logic will unlock countless opportunities. The future of tech, with Java as your tool, is wide open.