Mastering JavaScript Operators: Key Insights and Uses

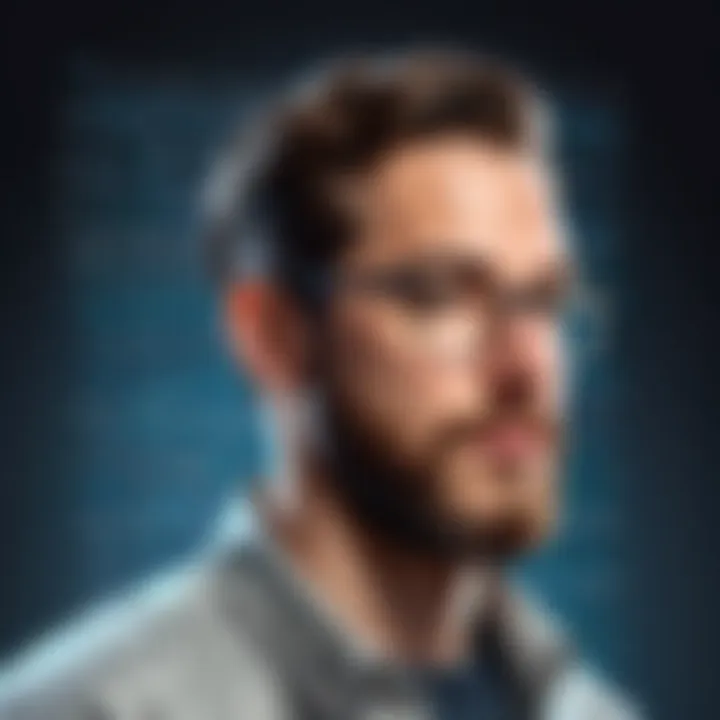
Overview of Topic
JavaScript, a cornerstone of web development, operates primarily through a series of operators. Understanding these operators is fundamental for anyone looking to write efficient and effective code. This article explores the various types of operators within JavaScript, focusing on their functions and applications.
The significant need for clarity around operators stems from how they directly impact the efficiency and readability of your code. If not used properly, one can easily lead to bugs or unexpected behaviors which can be frustrating, especially for newer developers. Therefore, grasping these concepts not only enhances coding skills but also serves as a ticket to deeper waters in programming.
Preamble to the main concept covered
Operators in JavaScript are key components that allow manipulation of values and variables. At its core, an operator performs actions like addition, comparison, or logical operations. With a robust arsenal of operators at one's disposal, a programmer can control the flow of data, making choices based on certain conditions.
Scope and significance in the tech industry
The tech industry thrives on code performance. Whether it’s for web applications, mobile apps, or other software, understanding how operators enhance or hinder performance is invaluable. With JavaScript being a popular choice for front-end development, a solid grasp of operators becomes increasingly relevant as projects grow in complexity.
Brief history and evolution
JavaScript has come a long way since its inception. Originally created in 1995 to enhance web pages, it has evolved into a language capable of building entire applications. Several versions have emerged, with significant improvements, and operators have adapted along the way. The introduction of ES6 brought many new features, expanding the capabilities of operators and their use cases.
Fundamentals Explained
To appreciate how operators function, one must first understand the core principles.
Core principles and theories related to the topic
- Types of Operators: JavaScript primarily categorizes operators into several types, such as Arithmetic, Logical, Bitwise, and Comparison Operators. Each serves unique purposes with specific syntax.
- Operator Precedence: Not all operators execute in the same order. Understanding precedence is crucial for evaluating expressions correctly.
Key terminology and definitions
- Operator: A symbol that tells the interpreter to perform a specific mathematical, relational, or logical operation.
- Operand: The values or variables that an operator works with.
- Expression: A combination of variables, operators, and values that evaluates to a single value.
Basic concepts and foundational knowledge
At the foundation lies the simplest arithmetic operators like , , , and . These perform basic calculations. Logical operators such as , , and manipulate boolean values, essential for controlling flow in code. Understanding these basics sets the stage for exploring more advanced applications of operators in larger projects.
Practical Applications and Examples
Real-world usage of operators is where theory meets practice.
Real-world case studies and applications
Consider a simple login validation system. When the user enters credentials, logical operators can determine accessibility based on truth values. For instance, using checks if both conditions are met before granting access.
Demonstrations and hands-on projects
You could even create a calculator that utilizes arithmetic operators for basic functions:
Code snippets and implementation guidelines
Experimenting is vital. Try out different operators by implementing small functions in various scenarios. Don't shy away from creating mini-projects like quizzes to reinforce logic through conditionals and comparisons.
Advanced Topics and Latest Trends
The landscape of JavaScript is dynamic, with techniques continually evolving.
Cutting-edge developments in the field
One must consider modern frameworks like React, which utilize operators creatively for state management. The introduction of optional chaining () and nullish coalescing () in recent versions serves as prime examples of how operators are adapted for improved efficiency.
Advanced techniques and methodologies
Higher-order functions are increasingly common. They often leverage operators for more profound implications, allowing developers to pass functions as arguments.
Future prospects and upcoming trends
As web standards grow, we can anticipate more sophisticated operators that facilitate complex data manipulations, particularly in handling asynchronous operations and reducing boilerplate code.
Tips and Resources for Further Learning
Deepening understanding is essential. Here are a few resources to consider:
- Books: "You Don’t Know JS" series provides insight into JavaScript fundamentals.
- Courses: Platforms like Codecademy and freeCodeCamp offer comprehensive JavaScript courses.
- Tools and software for practical usage: Explore integrated development environments like Visual Studio Code for a rich, supportive coding experience.
As you venture further into JavaScript, remember that mastering operators is a stepping stone in your programming journey. By constantly experimenting and learning, you can elevate your coding prowess to new heights.
Foreword to Operators in JavaScript
In the realm of JavaScript programming, operators serve as the backbone of virtually every action within the code. Understanding operators is crucial, as they enable the manipulation of data, facilitate logic flow, and bolster functionality in applications. In essence, operators guide how expressions evaluate and how data interacts, playing a pivotal role in coding efficiency and clarity.
Definition of Operators
To grasp the concept of operators in JavaScript, it helps to start with a simple definition. Operators are symbols or keywords that perform operations on one or more operands. These operands can be values, variables, or expressions. Each operator is designed to accomplish a specific type of task, which can range from arithmetic calculations to logical comparisons.
For instance, the operator adds two numbers together. The kind of operation performed is determined by the operator being used. Here’s an illustrative example:
In the above snippet, and are operands, and is the operator. Operators can be as straightforward as this arithmetic one, or they can become quite complex, depending on the type of operation needed and the nature of the data.
Importance of Operators in Programming
The significance of operators in programming cannot be overstated. They provide the necessary functionality for performing computations, making decisions, and controlling the flow of execution within programs. Here are a few key aspects to consider:
- Logical Operations: Operators form the core of logical reasoning in code, allowing programmers to create conditional statements that govern application behavior. For example, using logical operators like (AND) or (OR) enables nuanced decisions based on multiple criteria.
- Data Manipulation: Many programs revolve around data, and operators are essential for manipulating this data. Arithmetic operators help in calculations, while assignment operators allow you to store values in variables effectively.
- Comparative Assessments: Comparison operators are crucial for evaluating conditions, which impacts decisions in programming. They help to test the equality or inequality of values, often serving as the foundation for control structures such as if-else statements.
To highlight their importance:
Operators in JavaScript are the tools that shape the logic and functionality of a program. Without them, many programming tasks would become cumbersome and inefficient.
In summary, recognizing how operators work and understanding their multitude of functions is vital for anyone looking to delve deeper into JavaScript. Their ability to transform raw data into functional outputs makes operators not only relevant but indispensable in programming.
Types of Operators
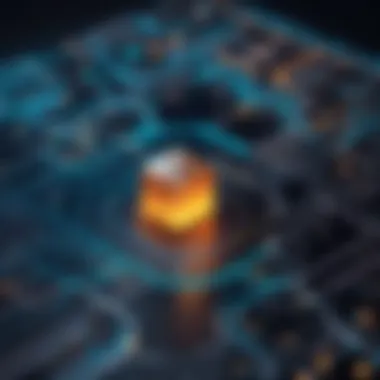
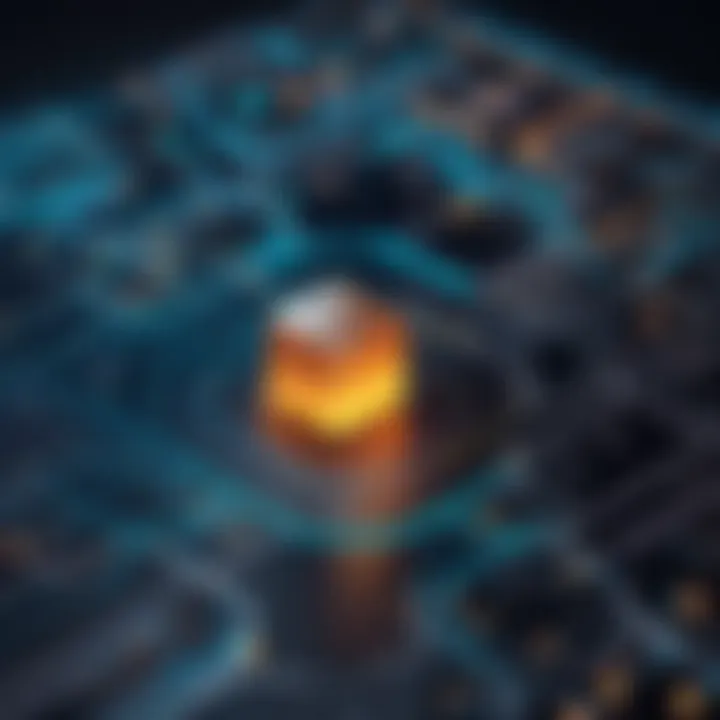
Understanding the different types of operators in JavaScript is crucial for any programmer. Operators serve as the building blocks for creating expressions, managing data manipulation, and controlling the flow of a program. By mastering these tools, one can write clearer, more efficient code while reducing the likelihood of errors. Let's explore each type in detail.
Arithmetic Operators
Overview and Syntax
Arithmetic operators are fundamental to performing mathematical calculations in JavaScript. These include operations such as addition, subtraction, multiplication, and division. Their syntax is straightforward, making it easy for developers to implement them in their code. For instance, the addition operator can combine numbers, while the subtraction operator removes one value from another.
A key characteristic of arithmetic operators is their ability to handle both integers and floating-point numbers. This versatility allows for a wide range of calculations in various scenarios, from simple financial applications to complex scientific computations. However, while they’re common and intuitive, they come with occasional pitfalls. For example, JavaScript can suffer from imprecise calculations when dealing with floating points, which requires careful attention.
Common Use Cases
Arithmetic operators are ubiquitous in programming. They can be seen in scenarios like calculating totals in e-commerce systems, determining averages in statistical simulations, or even in game score calculations. Using these operators effectively can save time and simplify code, which is why they are so popular among developers.
Consider a budget calculator that sums user expenses:
This line succinctly sums values with arithmetic operators. Despite their reliability, one must remember their limitations with large integers or special cases – like division by zero.
Limitations and Considerations
While arithmetic operators are powerful, they are not without their concerns. As mentioned, floating-point arithmetic can lead to unexpected results. For instance, the result of might not equal , highlighting a peculiar behavior that could confuse newcomers.
It’s also essential to consider operator precedence. When mixing different operators, JavaScript follows specific rules on which operators to evaluate first. Misunderstanding these rules can lead to incorrect results. To avoid such issues, use parentheses thoughtfully to ensure clarity in your expressions.
Comparison Operators
Equality vs. Strict Equality
Comparison operators are designed to compare two values, returning a boolean result. While the equality operator checks for value equality, the strict equality operator goes a step further by considering both value and type. This distinction is vital as it helps avoid problematic type coercion in JavaScript, where variables can change types unexpectedly.
For instance, using on the string "0" and the number will return , while will yield . Such subtlety provides a direct insight into data types and minimizes bugs within code. Adopting the strict equality approach often leads to cleaner and more reliable comparisons.
Comparison in Conditional Statements
When it comes to controlling the flow of a program, comparison operators shine in conditional statements. They help dictate the logic based on varying conditions, impacting functions, loops, and more. For example, in an if statement:
This code block showcases how a strict comparison can guide program execution. By understanding how different comparison methods work, programmers can write better logic, which is crucial for creating responsive applications.
Best Practices
Working with comparison operators comes with its set of best practices. The first step is always to use strict equality whenever possible to avoid unintended results due to type conversion. Another suggestion is to avoid chaining equality checks, as this can obfuscate the intended logic. Instead, use explicit comparisons for clarity.
Synthetic checks—such as —should be clear and easy to read, reducing cognitive load for anyone revisiting the code.
Logical Operators
AND, OR, and NOT Operations
Logical operators allow combining multiple boolean expressions and return a boolean value based on the logical operation. The most common includes (AND), (OR), and (NOT). They are essential in forming complex conditions in logic statements.
For instance, using AND means all conditions must be true:
Conversely, OR checks if at least one condition is true, which broadens possible outcomes. These operators elevate the sophistication of JavaScript code and ensure that programs can handle diverse scenarios efficiently.
Applications in Conditionals
Logical operators find their primary applications in conditional statements, allowing developers to craft intricate decision-making pathways. They can enhance user interactivity and response mechanisms as well. By leveraging logical constructs, program behavior can adapt to numerous circumstances dynamically, which is especially useful in web applications, where user actions dictate results.
For example, validating user input requires a blend of logical checks to ascertain expected criteria before processing. Logical operators facilitate this while providing power and simplicity to code design.
Potential Pitfalls
Despite their usefulness, logical operators can lead to confusion if misapplied. Short-circuiting behavior, for instance, might cause unexpected results when conditions are chained. If the first condition of an AND statement evaluates to false, JavaScript does not evaluate the second one, which can inadvertently hide bugs or affect functionality.
Being aware of these nuances and applying best practices will help avoid common traps that even seasoned developers can fall into.
Bitwise Operators
Bit Manipulation Techniques
Bitwise operators manipulate the binary representation of numbers, allowing for efficient data handling at a low level. This is less common in standard programming but crucial in performance-critical applications such as gaming or system programming. For instance, the bitwise AND operator checks each bit of the binary representation of two numbers.
These techniques are central to optimizing performance, especially when dealing with large numbers of elements in arrays or managing flags. However, they require a solid understanding of binary arithmetic, which can be a steep learning curve for new developers.
Use Cases in Performance Optimization
Utilizing bitwise operators can yield performance gains in specific contexts. For example, they can replace certain arithmetic checks with faster operations. When toggling specific bits in a set of flags, such as modulating settings in games, bitwise operators deliver quick evaluations that standard operators cannot match.
However, developers must gauge whether the added complexity is justified against the performance benefits, as this approach can obscure readability. When performance is paramount, such as in tight loops or high-frequency calculations, bitwise operations become a valuable tool in the developer's arsenal.
Common Misunderstandings
Many new developers perceive bitwise operations as unnecessary intricacies, yet grasping their functionality has the potential to unveil significant advantages. Misunderstandings often arise from associating these operators solely with low-level programming where their application is more visible.
A vital aspect to clarify is that although bitwise operators can outperform arithmetic operators in specific scenarios, their lack of versatility in handling standard mathematical calculations can limit their use cases. Therefore, educational focus should balance the theoretical knowledge and practical applications of bitwise operators.
Assignment Operators
Basic Assignment vs. Compound Assignment
Assignment operators serve a critical role in variable management. The basic assignment operator is straightforward—simply placing a value into a variable. However, compound assignment operators like , , , and condense operations, allowing for a more concise syntax in code.
For example:
This highlights how compound assignment can simplify code and improve readability without sacrificing clarity. Still, developers must remain aware of the scope and lifecycle of variables during their manipulation.
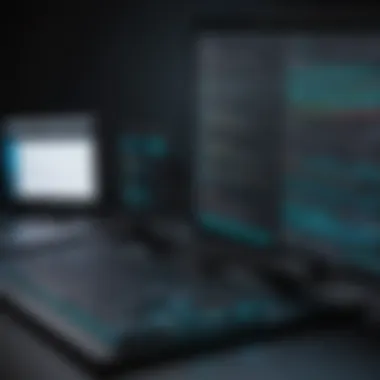
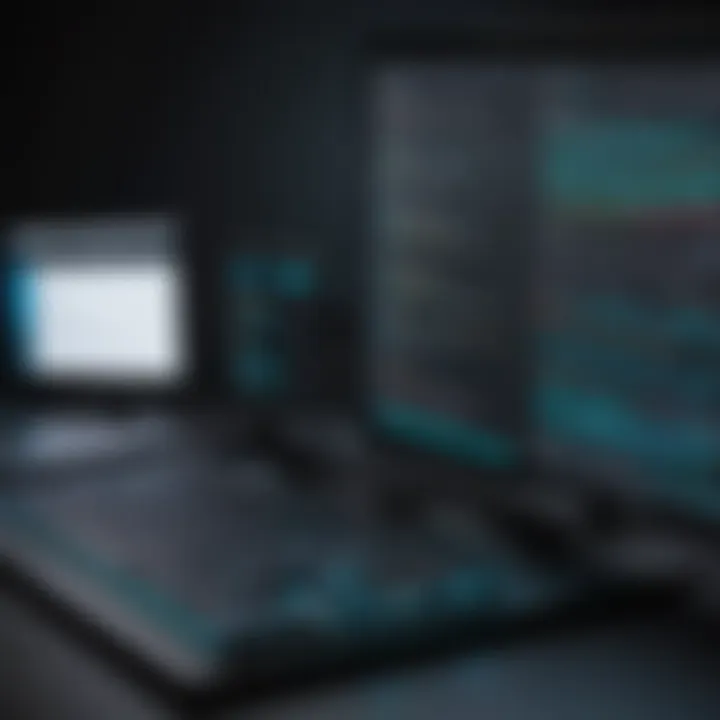
Understanding the Shorthand Notation
Shorthand notation for assignment operations provides clarity and conciseness. As seen in the previous example, combining assignment with arithmetic aids swift coding without compromising understanding. It’s beneficial as long as the developer grasps the underlying operation occurring in the background.
The shorthand can lead to errors when misapplied or when the programmer overlooks the order of operations, so ensuring the intended logic is paramount.
Best Practices for Clarity
When using assignment operators, clarity should be the top priority. Overusing complex compound statements can muddy code readability. It’s generally advisable to use base assignments for complex functions or loops, avoiding shorthand that may confuse future you.
A good rule of thumb is to review assignments for intention—if a statement can be misunderstood, rewriting it may save headaches later. Consistent variable naming also assists in mitigating confusion and enhances overall code legibility.
Ternary Operators
Syntax and Structure
Ternary operators provide a streamlined approach for conditional expressions. Their syntax is formatted as , resulting in compact conditional logic that remains clear. Ternary operators act as a shortcut for statements, which can enhance code brevity and readability.
Using these operators wisely allows for elegant solutions in situations where simple decisions are needed. However, over-reliance on ternary logic can cause code to quickly become convoluted; therefore, paying attention to context is vital.
Use Cases in Conditional Logic
Ternary operators frequently lend themselves to uncomplicated decision-making processes. They shine in UI development, where rapid evaluations determine which elements to render. For instance, toggling visibility of a component based on user login status can easily employ a ternary operation:
This promotes concise, efficient code while maintaining clarity of function. Nonetheless, using them in more complex conditions may lead to issues with readability.
Considerations for Readability
Although ternary operators simplify syntax, they should not replace clear structuring of logic. Nested ternaries can quickly become confusing and diminish readability. It’s prudent to use them sparingly or only in straightforward scenarios to ensure others can swiftly understand the intent behind the code.
The general recommendation remains that clarity trumps brevity in programming—do not sacrifice your reader's understanding just for a quick line of code.
Advanced Operator Concepts
Understanding Advanced Operator Concepts in JavaScript is imperative for any programmer aiming to master the craft. These concepts lay the groundwork for writing efficient, optimized code and for utilizing operators effectively in various programming scenarios. As the programming landscape continually evolves, a firm grasp of advanced operator principles can yield tangible benefits in both debugging and enhancing code functionality. Moreover, these insights allow for a finer control over data types and logical structures, driving home the significance of being adept in this area.
Operator Precedence
Understanding Operator Hierarchy
Grasping the nuances of Operator Hierarchy is crucial as it dictates the order in which operations are performed in an expression. This is particularly useful when multiple operators are present and one needs to predict how the JavaScript engine interprets their intent. A favorable aspect of understanding this hierarchy is its ability to prevent unexpected results in calculations and logical evaluations.
For instance, in the expression , the multiplication will be computed first due to its higher precedence compared to addition. As such, recognizing this can save a developer from falling into the trap of logic errors or miscalculations. The uniqueness of this concept lies in its universal applicability across different programming languages, reinforcing its significance.
Practical Examples
To comprehend the practical significance of Operator Precedence, consider the following example:
The evaluation starts with the multiplication, resulting in , further simplifying to . Without understanding the operator precedence, a developer might incorrectly anticipate , leading to a wrong conclusion.
Such examples underline the practicality of mastering this concept. Notably, programming operates on logic, and leaps in understanding can bolster a coder's confidence and code accuracy.
Common Issues and Resolutions
While understanding operator precedence is beneficial, it certainly comes with its own set of challenges. A common issue many developers encounter is neglecting parentheses, leading to misinterpretations of operator hierarchy. For example:
In this case, the brackets clearly dictate that multiplication's outcome should be prioritized, ensuring a clean evaluation.
To resolve such issues, one should practice consistently and implement clear coding styles that emphasize clarity. A not-so-hidden perk is that proper usage of parentheses, despite seeming like extra work, actually enhances the readability and maintainability of code, making it easier for you or someone else to manage.
Type Coercion
Implicit vs. Explicit Coercion
The concept of Implicit vs. Explicit Coercion touches upon how values can change types in JavaScript operations. Implicit coercion occurs when JavaScript automatically converts types to fit the operation context, while explicit coercion is explicitly defined by the programmer using conversion functions like or .
Recognizing this distinction clarifies how data types interact and helps in detecting potential issues before they arise. Implicit coercion can lead to surprising behaviors:
Here, the number is coerced into a string, demonstrating how JavaScript handles operations seamlessly but without the programmer's consent.
In contrast, being deliberate through explicit coercion can prevent surprises:
This precise control over data types ensures that programmers write intention-based code.
Examples and Edge Cases
Concerning Examples and Edge Cases, the unpredictability of type coercion can manifest in some intriguing contradictions. Consider comparing strings and numbers:
In this case, the equality operator allows type conversion, while does not. Understanding these edge cases is essential in forming precise, robust comparisons. It leads to more predictable code that can seamlessly navigate various data scenarios and ensures better performance.
Impact on Comparisons
Discerning the Impact on Comparisons through coercion styles is fundamental for avoiding bugs in applications. Type Coercion can introduce faulty logic if developers do not pay attention to types while comparing:
In this snippet, although is a falsy value, misinterpretation of coercion can occur if were mis-declared. Thus, it’s crucial for developers to be well-acquainted with coercion impacts, afin preventing logical errors that can derail functionality.
Short-Circuit Evaluation
Definition and Mechanism
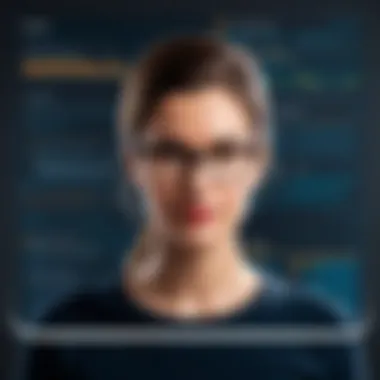
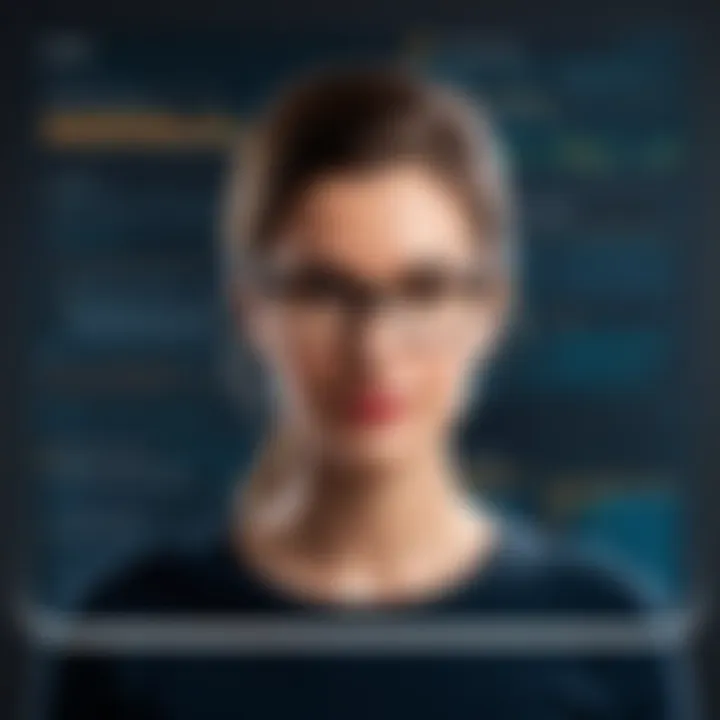
Short-Circuit Evaluation is an interesting behavior where logical operations stop evaluating once the overall result is guaranteed. For example, in the logical OR operation, if the first operand evaluates to true, the second operand is not evaluated, as the overall statement cannot be false regardless of subsequent values. This characteristic can significantly enhance performance and reduce computational expenditure. Using this effectively allows for more streamlined code execution, as unnecessary computations are inherently avoided.
Applications in Programming Logic
The practical applications of Short-Circuit Evaluation come into play frequently in conditional structures. A common way this is utilized is in controlling the flow of operations. For instance:
Here, the check for being works perfectly, without further computations made on a non-existent user object. This sparse technique not only heightens efficiency but also keeps code cleaner.
Challenges and Best Practices
Despite its advantages, Short-Circuit Evaluation can be tricky. One potential challenge is the misuse of this concept, wherein developers fail to recognize how evaluations halt. It’s wise to recognize that if an expression's side effects rely on evaluation beyond the short-circuit, results may be unpredictable. A typical best practice is to ensure side effects can occur independently of logical operations, enhancing clarity.
In summary, the deeper a programmer delves into advanced operator concepts like operator precedence, type coercion, and short-circuit evaluation, the more they enhance their ability to write clean, efficient code. These principles serve not just as theoretical insights but as solid foundations that can influence the daily coding tasks and result in superior programming outcomes.
Common Mistakes with Operators
Understanding operators in JavaScript is crucial, but it’s just as vital to recognize how they can be misused. Mistakes with operators can lead to unexpected behavior in your code, which may result in debugging that feels like a game of whack-a-mole. The pitfalls can be subtle, particularly for those learning programming. By being aware of common mistakes, you can enhance your programming skills, ensuring your code is not just functional but also efficient and clean.
Confusion Between == and ===
In JavaScript, there’s often a tussle between the equality operators: and . The first checks for value equality but does not consider data types. That means, when you compare different types, JavaScript will try to convert one to the other before making the comparison. For example:
On the flip side, the strict equality operator checks both value and type. When using it, if the types don’t match, even if the values do, you’ll end up with a . This means:
It's easy to see why beginners might stumble here. Using might seem convenient, but it can bite you later when you expect certain behavior. To avoid confusion, it’s recommended to use consistently unless you have a specific reason to do otherwise. Remember, clarity in your comparisons leads to fewer headaches down the line.
Misuse of Logical Operators
Logical operators — , , and — can be a source of frustration for coders. A common blunder is mixing them up during conditions. For instance, consider using without realizing both conditions must be true for an action to proceed. If you're not careful, your logic might short-circuit your intent.
This can also lead to unintended outcomes in function calls or event handlers. For example:
When you mess with logical operators, you can overlook essential aspects of your program's flow. What might feel like a minor detail can spiral into a significant bug. It's wise to take a step back and think through your logic before solidifying it in code. Double-check this area to save time debugging later.
Neglecting Operator Precedence
Operator precedence defines the order in which different operations are performed. Misunderstanding how precedence works can lead to unexpected results. For instance, suppose you have:
You might have assumed it worked as you read it, resulting in arithmetic errors. A common practice to avoid this confusion is to use parentheses. When you aren't sure about operator precedence, wrapping expressions in parentheses can clarify your intent and the sequences of operations, making your code more legible:
Always be aware of which operators have higher precedence in JavaScript, and when in doubt, don’t hesitate to use parentheses. This not only ensures your logical groups are correct but also keeps your code cleaner. Good practices in clarity now can save debugging woes later.
Understanding these key mistakes helps sharpen your coding skills and enhances the quality of your JavaScript projects. With attention to detail, you can transform potential pitfalls into learning points, paving the way for more robust programming.
Practical Applications of Operators
Understanding how operators work within JavaScript is crucial for writing effective code. Their importance cannot be overstated, particularly in practical applications where real-world scenarios come into play. Whether it's performing calculations, making decisions within your code, or handling data manipulation, operators are the building blocks that enable these operations.
When employing operators in your coding endeavors, one needs to consider several factors:
- Efficiency: Well-placed operators can significantly reduce both the execution time and the complexity of your code.
- Readability: Code should not just be functional, but also comprehensible. Using operators effectively and appropriately can enhance the clarity of your logic.
- Maintainability: Code that utilizes operators well is typically easier to maintain over time. New developers can quickly grasp the logic without sifting through convoluted statements.
"Operators are like tools in a toolbox. Each serves a unique purpose, and knowing how and when to use them can make a world of difference."
Using Operators in Function Logic
Operators play a vital role in function logic. For instance, when it comes to calculating parameters or returning specific outcomes based on conditions, operators streamline the processes.
Take a look at this simple function:
In this example, the operator combines values, while the operator performs multiplication. Without operators, deriving a total price would be much more cumbersome. Through operators, you can handle complex calculations seamlessly and efficiently.
Moreover, operators can help in simplifying code using functional programming practices. For instance, you can combine logic with the use of higher-order functions, allowing operators to create neater and more expressive code.
Operators in Conditional Rendering
Conditional rendering is where operators truly shine. They determine what gets displayed in your application based on specific conditions. This is particularly useful in front-end development, where UI elements often depend on the state of the application.
Consider a scenario where you want to display a welcome message based on a user's login status:
Here, the ternary operator acts as a shortcut for an statement. It checks if the exists and then decides which message to render. This can make your code cleaner and more intuitive, allowing for immediate comprehension of flow.
In summary, harnessing the practical applications of operators in your coding toolkit is indispensable. It leads to efficient, clean, and maintainable code, which, ultimately, contributes to better programming practices and outputs.
End
In this article, we have journeyed through the core principalities of operators within JavaScript, which have undoubtedly carved their niche in programming. Understanding these operators is not just an academic endeavor; it's an essential skill for anyone wishing to write efficient and effective code. As we’ve outlined, the various categories—arithmetic, comparison, logical, bitwise, and assignment—each wield their own particular significance, unraveling multiple layers of functionality within the language.
Summary of Key Points
To recap, here are some of the vital aspects we've covered:
- Types of Operators: Each type of operator serves a distinct purpose—from performing basic math with arithmetic operators to making logical decisions with logical operators.
- Advanced Concepts: Topics like operator precedence and type coercion play a critical role in how JavaScript evaluates expressions and executes code. Understanding these can save developers from headaches later on.
- Common Mistakes: We talked about common pitfalls around operators. Recognizing these mistakes, like confusing with , is crucial for crafting reliable solutions.
- Practical Applications: Finally, applying operators in real-world scenarios enhances both code performance and readability.
Encouragement for Continued Learning
The world of JavaScript and its operators is vast and ever-evolving. It's vital to keep an inquisitive mind. Delve deeper into more advanced concepts such as closures and higher-order functions; these often interplay with how operators function. Furthermore, explore forums like Reddit, where many developers share real-world examples and solutions.
It's also worthwhile to experiment with code snippets in environments like JSFiddle or CodePen. Hands-on practice will solidify the understanding of these operators. Stay curious, enlightened, and don't hesitate to revisit the basics even as you explore advanced topics—there's always something new to learn!
"In programming, every moment spent debugging will pay off tenfold with understanding and proficiency."
Developing as a programmer is a lifelong journey, and understanding operators is merely a stepping stone in mastering the multifaceted JavaScript language. Keep learning!