Mastering JavaScript Skills Tests: A Deep Dive
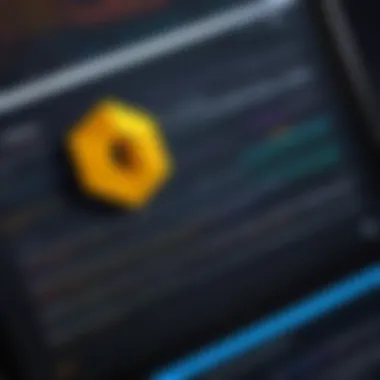
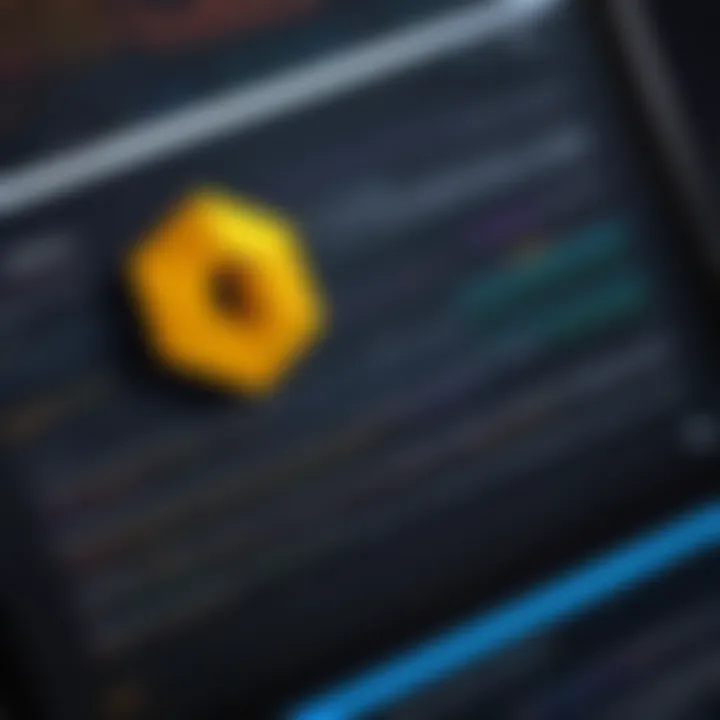
Overview of Topic
The examination of JavaScript skills tests is increasingly vital in today's tech landscape. As organizations continuously seek proficient developers, knowing how to effectively assess these skills has become crucial. From junior developers to experienced professionals, JavaScript skills tests serve as an evaluative tool, ensuring candidates possess the necessary competencies to contribute effectively in a work environment.
The significance of these tests lies not only in their ability to identify skill levels but also in fostering a culture of continuous learning among developers. As the programming languages and technologies evolve, so do the expectations placed on developers. An understanding of key methodologies in creating these tests is essential.
Historically, JavaScript has undergone a significant evolution. From its inception as a simple scripting language to its current status as a fundamental pillar of web development, its associated skill assessments must reflect these changes. Adapting the tests to current industry standards ensures that they remain relevant and effective.
Fundamentals Explained
It's important to establish some core principles and terminology connected to JavaScript skills tests. Understanding these elements provides a solid foundation.
Core Principles:
- Assessment Validity: Ensuring that tests measure what they claim to measure.
- Reliability: Consistency of assessments across various test iterations.
- Fairness: Equitable testing environments that don't favor certain groups over others.
Some key terms include:
- Code Quality: Refers to the effectiveness of written code, including readability and maintainability.
- Test Coverage: Indicates the percentage of the codebase tested by automated tests.
- Frameworks: Tools like Jest or Mocha utilized for testing JavaScript applications.
Focusing on these principles contributes significantly to developing competent JavaScript programmers who can navigate the complexities of modern development.
Practical Applications and Examples
Examining real-world applications of JavaScript skills tests can illustrate their practical significance. Companies like Google and Facebook use custom-built coding challenges during their hiring processes. Their approaches often involve hands-on tasks that mimic actual programming scenarios.
For instance, candidates might be tasked to build a simple web application using React or Node.js. This provides insight into their real coding abilities.
Implementation summary:
- Create a functional web app
- Apply necessary JavaScript libraries
- Optimize code for performance
This demonstration of skills ensures candidates can apply theoretical knowledge to practical tasks.
Advanced Topics and Latest Trends
The realm of JavaScript skills testing is dynamic, with shifts influenced by emerging technologies. Notable trends include the integration of machine learning in assessment processes. Tools like Codility and HackerRank now offer platforms where candidates tackle algorithm problems that are graded automatically.
Another fascinating trend is the use of remote coding assessments. The lifting of geographical boundaries means organizations can tap into global talent pools. Candidates may need to complete timed tests in specific environments using tools such as Zoom or collaborative coding platforms.
Understanding these advanced techniques can greatly improve both the testing experience and the overall quality of assessments.
Tips and Resources for Further Learning
For those seeking to enhance their JavaScript tests or their own skills, several resources are worth exploring.
- Books:
- Online Courses:
- Tools:
- JavaScript: The Good Parts by Douglas Crockford
- Eloquent JavaScript by Marijn Haverbeke
- FreeCodeCamp
- Codecademy
- ESLint for coding standards
- Jest for testing frameworks
Utilizing these mechanics can significantly improve understanding and application of JavaScript skills testing.
Prologue to JavaScript Skills Tests
JavaScript skills tests are essential in the tech industry, bridging the gap between theoretical knowledge and real-world application. They serve multiple purposes: evaluating a programmer's understanding of JavaScript, ensuring competency in coding, and providing employers a reliable means of assessing candidates. In an era where technology evolves rapidly, the importance of these tests cannot be overstated. They offer a structured approach to gauge skills and identify gaps in knowledge, ensuring that both new and seasoned developers meet the ever-increasing demands of the field.
Purpose and Utility
The primary goal of JavaScript skills tests is to measure a candidate's coding proficiency. These tests focus on various aspects of the programming language, including syntax, functions, and problem-solving abilities. Evaluators can gain insights into a programmer’s ability to apply their knowledge effectively. Evaluating candidates through skills tests helps organizations streamline their hiring process. Furthermore, they foster a clearer understanding of where individuals need improvement, allowing for targeted learning experiences. Skills tests also benefit job seekers, as passing these assessments can boost their employability and increase confidence in their own capabilities.
Historical Context
JavaScript has undergone significant evolution since its inception in 1995. Initially designed as a simple scripting language for enhancing web pages, it has grown into a robust tool for both client-side and server-side development. As JavaScript gained popularity, so did the necessity for evaluating programming skills.
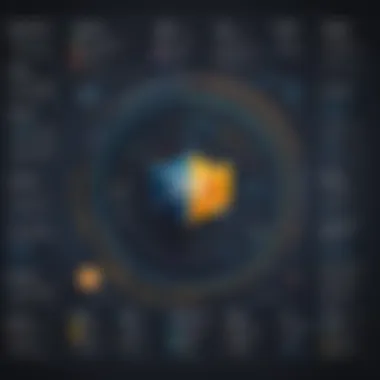
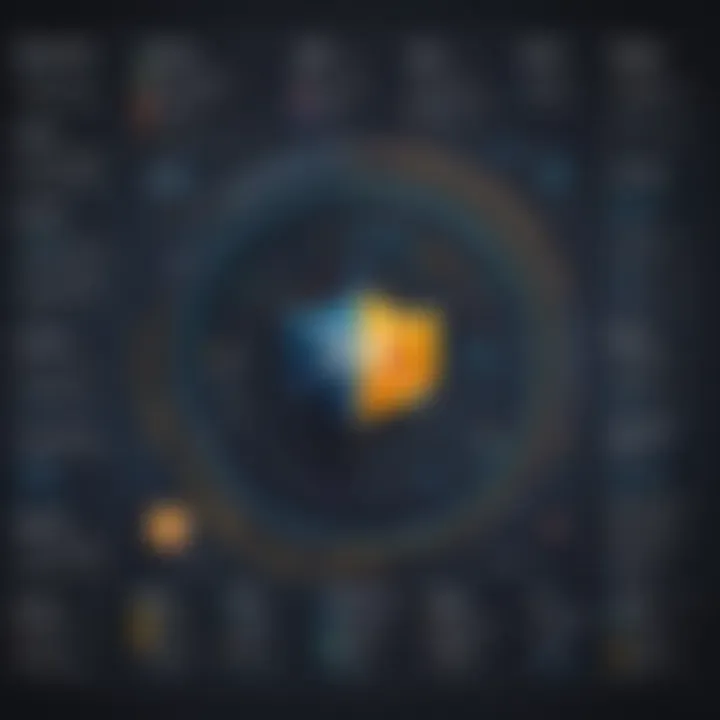
In the early 2000s, organizations began implementing basic coding tests, primarily focusing on syntax and simple algorithm challenges. However, as the landscape of web development evolved with frameworks like Angular and React, the need for more comprehensive assessments emerged. Today, tests not only evaluate core JavaScript knowledge but also encompass advanced topics such as Promises and Async Programming, Closures and Scopes, and Modules.
With the rise of online platforms, skills assessment has become more accessible. Companies like HackerRank and LeetCode have made it feasible for candidates to practice coding challenges. This historical trajectory illustrates the increasing complexity and sophistication of JavaScript skills tests, establishing them as a fundamental aspect of the hiring process in the tech industry.
The Essential Skills to Assess
Assessing JavaScript skills involves identifying core competencies that define one’s proficiency in this versatile programming language. Understanding these essential skills is vital as they provide the foundation upon which developers can build more complex applications and solutions. When evaluating a candidate's or one’s own skills, focusing on both core concepts and advanced topics become crucial. This comprehensive examination presents a clear pathway for skill enhancement.
A well-rounded assessment evaluates a myriad of elements. Benefits of such focused testing include improved hiring processes and better outcomes in learning. These skills help in distinguishing candidates who possess not only theoretical knowledge but also practical application abilities. It allows hiring managers to pinpoint those who can adapt to real-world challenges effectively. Ultimately, attention to essential skills sharpens the overall competency within teams, leading to innovative solutions and efficient problem-solving.
Core JavaScript Concepts
Core JavaScript concepts form the backbone of any robust testing strategy. Proficiency in these fundamentals is non-negotiable for any aspiring developer. Key areas include understanding data types, control structures, functions, and error handling. Familiarity with these elements ensures that a developer can navigate through basic scripting smoothly and troubleshoot common issues efficiently.
Advanced Topics
Advanced topics demonstrate a deeper knowledge of JavaScript and its ecosystem. Competency in these subjects signifies a readiness to tackle sophisticated projects that require a detailed understanding of asynchronous programming and modular design.
Promises and Async Programming
Promises and async programming represent a key aspect of modern JavaScript development. They enable non-blocking code execution, crucial for developing efficient web applications. A fundamental characteristic of promises is their ability to handle asynchronous operations neatly. This enables developers to write cleaner, more manageable code.
However, one must understand the potential pitfalls. Incorrect implementation can lead to callback hell or complex nesting, which can make code harder to read and maintain.
Closures and Scopes
Closures and scopes are pivotal in understanding how JavaScript manages variables and memory. A major feature of closures is their ability to maintain access to outer variable scope, enabling data encapsulation. This characteristic is beneficial in building functions that require private data, enhancing security and module design.
However, closures can also lead to unintended side effects if not managed correctly, resulting in memory leaks or performance issues in large applications. This necessitates a careful approach to using closures effectively.
Modules and Bundling
Defining modules in JavaScript allows developers to break applications into manageable pieces. The bundling process further streamlines the delivery of these modules, enhancing application load times and performance. One defining feature of modules is their isolation, which prevents variable clashes and promotes better organization.
Yet, improper use of modules can lead to overly complex dependencies or code that is difficult to scale. Balancing modular design with readability is essential for a sustainable codebase.
Frameworks and Libraries
Frameworks and libraries significantly enhance productivity by providing pre-built components and structures. Each has unique strengths that appeal to different programming needs.
React
React is a popular library for building user interfaces. Its component-based architecture allows for reusable code, reducing redundancy. One of the key characteristics of React is its virtual DOM, which optimizes rendering and improves performance. However, its learning curve can be steep for beginners.
Vue
Vue is another framework that emphasizes simplicity and adaptability. Its progressive structure means developers can scale applications with ease. A notable feature of Vue is its two-way data binding, which streamlines state management. However, reliance on certain patterns can lead to an inflexible code structure in larger projects.
Node.js
Node.js extends JavaScript's capabilities to server-side programming. This allows developers to use a single language across the stack, enhancing efficiency. Its event-driven architecture is particularly well-suited for I/O-heavy applications. However, managing callbacks in Node.js can lead to convoluted code if not handled properly.
Understanding these essential skills empowers both candidates and testers to engage meaningfully in the rapidly evolving landscape of JavaScript development.
Designing Effective Skills Tests
Designing effective skills tests is a cornerstone of evaluating JavaScript knowledge and expertise. This topic is critical because proper assessments can shape the abilities of developers. A well-designed test not only gauges the technical skills but also reflects how candidates apply their knowledge in real-world scenarios. By focusing on various elements, one can create a meaningful evaluation process.
Good tests must consider different methods of assessment. For example, a blend of theoretical questions and practical tasks can provide a clearer picture of a candidate's capabilities. The incorporation of diverse formats can cater to different learning styles, which enhances the overall effectiveness of the test. Additionally, creating tests that avoid common pitfalls ensures that they truly represent the skills necessary for success in JavaScript development.
Test Types and Formats
Multiple Choice Questions
Multiple Choice Questions (MCQs) serve as a practical tool for quickly assessing knowledge. They present a succinct method of testing candidates on core concepts of JavaScript. A notable characteristic is their speed; testers can evaluate many candidates in a short amount of time. This approach minimizes the subjectivity that can be present in other formats, providing clear right or wrong answers.
However, MCQs can also have limitations. They may not assess a candidate's ability to apply their knowledge in practical situations. This drawback can lead to overestimating a candidate's true skills, particularly if they excel in memorization rather than application.

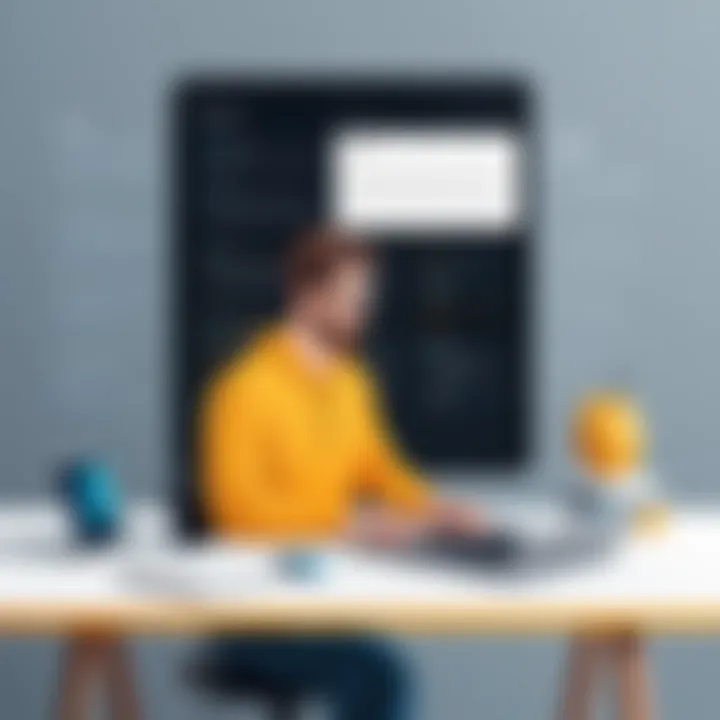
Technical Assessments
Technical assessments go one step further by requiring candidates to demonstrate their skills in a hands-on manner. This type of evaluation is beneficial because it provides insight into how candidates approach problems and solve them in real time. Candidates are usually given tasks that mimic real development challenges, providing a practical context for their skills.
While this format is advantageous, it also comes with its challenges. The assessments can be time-consuming to execute and evaluate. In addition, the setting may not fully represent a candidate's typical working environment, which can affect their performance.
Project-Based Evaluations
Project-Based Evaluations are often regarded as the most comprehensive assessment method. This approach allows candidates to work on a project that utilizes various JavaScript concepts, demonstrating their ability to integrate knowledge into a larger context. A major characteristic is that it gives a real-life assessment of a candidate's end-to-end skills, from coding to debugging to deploying.
Despite its strengths, this type of evaluation requires significant time and resources. It may be difficult to standardize the assessment criteria, which can introduce bias and complicate comparison among candidates. Furthermore, projects can vary greatly in scope, making it challenging to evaluate all candidates on the same level.
Balancing Theory and Practice
The principle of balancing theory and practice in JavaScript skills tests is crucial for a thorough evaluation process. Theory provides candidates with foundational knowledge, while practical skills illustrate their ability to implement concepts effectively. Tests should be designed to integrate both elements seamlessly. A candidate who understands the theory behind asynchronous programming but cannot effectively implement it in code will not be suited for many real-world challenges.
In summary, creating effective skills tests involves a thoughtful mix of test types. By recognizing the benefits and drawbacks of each category, one can design assessments that foster a comprehensive understanding of JavaScript. It is also essential to ensure that candidates can apply their knowledge in practical scenarios, preparing them for the complexities of the tech industry.
Common Pitfalls in Testing
When designing JavaScript skills tests, recognizing common pitfalls is crucial. A well-constructed test effectively distinguishes between varying levels of understanding and competency. Avoiding missteps not only improves the evaluation process but also enhances the candidates' experience. This section explores two major pitfalls: ambiguous questions and an overemphasis on syntax.
Ambiguous Questions
Ambiguity in testing can lead to confusion and misinterpretation among candidates. Questions that lack clarity may leave the test-taker unsure of what is being asked. This uncertainty can affect performance and shift the focus away from assessing true programming skills.
To create clear questions, it is essential to be precise and direct in wording. For example, instead of asking, "How does a function work?", a more effective question could be, "Explain the difference between a regular function and an arrow function in JavaScript." This specificity directs the candidate’s attention to key differences, encouraging critical thought.
Here are some strategies to avoid ambiguous questions:
- Use straightforward language.
- Define technical terms when necessary.
- Provide context where it might be needed.
By adopting these practices, testers can ensure clarity in their questions, thus enhancing the evaluation process significantly.
Overemphasis on Syntax
Focusing too heavily on syntax can detract from a more holistic assessment of a candidate's skills. While understanding the nuances of JavaScript syntax is important, it should not be the sole focus of a skills test. Concentrating on syntax alone can lead to surface-level evaluations that may overlook deeper competencies, such as problem-solving and logic.
Moreover, tests centered around syntax can lead to rote memorization rather than genuine learning. For instance, asking candidates to write code snippets from memory might show their recall abilities but does not effectively measure their practical understanding.
Instead, consider these approaches:
- Incorporate situational questions that require applying concepts.
- Use real-world scenarios to assess how candidates solve problems.
- Balance syntax-based questions with conceptual challenges.
In summary, both ambiguous questions and an overemphasis on syntax are common pitfalls in JavaScript skills testing. Addressing these issues can significantly enhance the quality and effectiveness of assessments, ultimately benefiting both candidates and evaluators.
Resources for JavaScript Knowledge Enhancement
Understanding JavaScript and mastering its various elements is fundamental for anyone in the tech industry. Resources for knowledge enhancement serve as essential tools that guide learners and professionals towards a deeper comprehension of JavaScript. The right resources not only improve coding skills but also keep one updated with the latest trends and best practices in the language. In this section, we look at online platforms, courses, and noteworthy publications that are highly regarded in the realm of JavaScript education.
Online Platforms and Courses
Learning JavaScript can be more engaging and effective when using established online platforms. Here are three of the most useful ones:
MDN Web Docs
MDN Web Docs stands out as a comprehensive resource in the programming community. It is curated by Mozilla and provides thorough documentation on web standards, including JavaScript. The key characteristic of MDN is its extensive coverage of both basic and advanced topics. It is a highly beneficial choice because it not only guides learners through JavaScript syntax but also explains the practical implications of using different JavaScript features.
One unique feature of MDN is its interactive examples, which allow users to modify code snippets and see results in real-time. This advantage helps users understand concepts more intuitively. However, some users have mentioned that the documentation can feel a bit overwhelming due to its depth and breadth.
freeCodeCamp
freeCodeCamp offers a hands-on approach to learning JavaScript through coding challenges and projects. Its main allure lies in its structured learning paths, which guide learners from basics to more complex topics efficiently. Many learners appreciate freeCodeCamp for its community aspect, where they can engage with others eager to learn JavaScript.
One unique benefit of freeCodeCamp is that it encourages users to build projects for non-profit organizations, providing real-world experience. However, some might find the platform's self-paced nature challenging if they require more structured guidance.
Codecademy
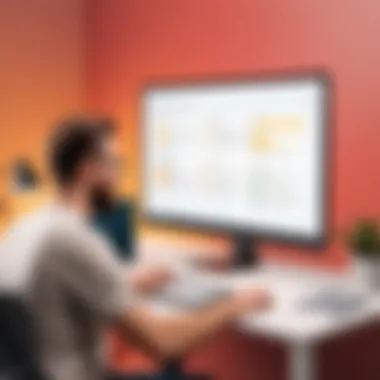
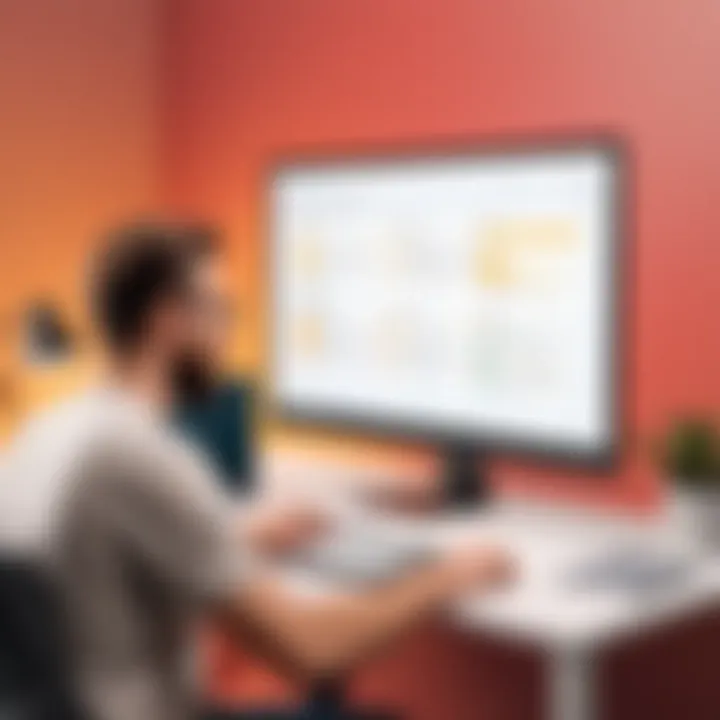
Codecademy is another popular platform that provides interactive coding lessons in JavaScript. Its essential feature is simulated coding environments, allowing users to write code directly in the browser. This immediate feedback mechanism enhances the learning experience by identifying errors quickly.
Codecademy is favorable for its engaging user interface and gamified approach to learning, making it appealing for beginners. On the downside, some users feel that the subscription cost for premium content can be a barrier, limiting access to additional valuable resources.
Books and Publications
In addition to online platforms, books and publications play a crucial role in enhancing JavaScript knowledge. Here are three noteworthy titles:
Eloquent JavaScript
Eloquent JavaScript is a celebrated book that offers a deep dive into the language. Its main contribution lies in its ability to blend theory with practical exercises. The book’s strength is its rich explanations of JavaScript concepts alongside well-designed code examples.
This book is regarded as beneficial for self-learners as it reinforces learning through practice. A unique feature of Eloquent JavaScript is that it is freely available online, although some readers point out that it may be challenging for absolute beginners due to its dense content.
JavaScript: The Good Parts
JavaScript: The Good Parts is a classic in the programming world. Authored by Douglas Crockford, this book emphasizes the functional aspects of JavaScript and explores why certain features are preferable. Its key characteristic is its focus on understanding the language's strengths rather than merely its syntax.
This book is a beneficial choice because it distills JavaScript into its most powerful elements. A unique feature is how it encourages readers to approach problems in more elegant ways. However, some readers have noted that it may feel dated and lack context for contemporary JavaScript development practices.
Learn JavaScript VISUALLY
Learn JavaScript VISUALLY is aimed at visual learners. This book employs graphics and illustrations to explain concepts clearly. Its main contribution is in making complex topics accessible through visual representation, which can be helpful for those who struggle with traditional text-heavy resources.
The key characteristic of this book is its contrasting approach to teaching, which makes it a popular choice among beginners and those with different learning styles. A unique feature is its storytelling format, which might be considered a refreshing change. However, it may not cover all topics in-depth, thus potential readers should consider it as a supplementary resource rather than a stand-alone guide.
Evaluating Test Results
Evaluating the results of JavaScript skills tests is crucial in determining a candidate's proficiency and identifying areas for improvement. Scores obtained from these assessments serve as a reflection of the understanding of JavaScript concepts, syntax, and practical applications. Proper evaluation ensures that individuals are not only assessed accurately but also provided a roadmap for future learning.
The results can reveal trends in an individual's performance and highlight specific strengths and weaknesses. Understanding these nuances can guide educators and employers in tailoring learning paths or professional development programs. This process plays a vital role in the tech industry as it directly influences recruitment decisions, team dynamics, and project success.
By implementing structured evaluation techniques, testers can provide candidates with a comprehensive picture of their skills, which enables ongoing development. An emphasis on continuous improvement is an essential aspect of any modern assessment strategy.
Interpreting Scores
Interpreting the scores from JavaScript skills tests requires a nuanced approach. Raw scores are often just one component of the evaluation. Context is critical; factors such as the difficulty level of questions, the candidate’s chosen learning path, and even the testing environment can impact results. It is valuable to understand how high a score is in relation to the benchmark set for the specific role or learning goal.
Metrics such as percentile ranks can also be utilized. A candidate scoring in the 90th percentile indicates a strong grasp of the material relative to peers. It’s also important to compare results against the desired competency levels required for specific roles. Overall, scores should be seen not only as an assessment of knowledge but as a launchpad for discussing future opportunities and areas of development.
Providing Constructive Feedback
Providing constructive feedback post-evaluation is essential for fostering an environment of growth. Feedback should be specific, actionable, and tailored to the individual's performance. Highlighting not only what was done well but also where improvements are necessary helps candidates understand their learning trajectory.
Some strategies for effective feedback include:
- Highlighting Strengths: Identify and affirm areas where the candidate performed well. This boosts confidence and recognizes effort.
- Addressing Weaknesses: Clearly outline areas needing improvement and recommend resources or strategies to strengthen those skills.
- Setting Goals: Create short-term and long-term goals for skill enhancement or mastery, aiding in focused learning.
- Encouraging Questions: Allow candidates to ask questions about their performance. This helps clarify misunderstandings and promotes curiosity.
Constructive feedback should be a two-way conversation, inviting candidates to engage with their learning journey.
"The goal of feedback is not merely to inform of shortcomings, but to illuminate the path for future growth."
This approach leads to a more engaged learner, who is better prepared to move forward with their JavaScript skills.
Culmination and Future Directions
The domain of JavaScript skills tests is continually evolving amid shifting trends in technology and programming fundamentals. Recognizing the importance of conclusion and future directions allows us to delve into key considerations that influence how testing is conducted and interpreted.
The Evolution of Skills Testing
Historically, skills testing in programming primarily revolved around rote learning and basic syntax recognition. However, this method has shifted towards evaluating practical skills that resonate more with real-world applications. Modern testing frameworks prioritize problem-solving abilities, code structure, and the developer’s approach to debugging. As organizations evolve in their approach to software development, testing methodologies have followed suit, demanding a more nuanced understanding of a candidate's capabilities.
The introduction of automated testing tools, coding bootcamps, and online platforms like MDN Web Docs, freeCodeCamp, and Codecademy marks a significant step forward. These resources shift the focus from pure memorization to the application of concepts. According to recent trends, assessments now incorporate projects, collaborative coding environments, and peer reviews to provide a holistic view of an individual’s skill set. This shift ensures relevance in a fast-paced tech landscape.
"The most essential skill for a programmer is not just knowing the right answers, but knowing how to find them."
— Anonymous
Continued Skill Development
For both candidates and organizations, continuous skill development remains paramount. The fast advancements in technology necessitate an ongoing commitment to learning. Professionals are encouraged to regularly update their knowledge through courses, seminars, and community engagement. This approach not only enhances individual talents but also fortifies the overall competency framework within an organization.
Understanding emerging technologies and methodologies, such as new JavaScript frameworks like React and Vue, is vital for maintaining relevance. Additionally, participation in developer communities, such as those on Reddit or tech-focused forums, can provide essential support and insight into contemporary challenges faced in the field.
The landscape of JavaScript and web development will continue to change, prompting the need for agile learning methods that cater to both evolving technologies and industry demands. Engagement with current literature, adopting a mindset of lifelong learning, and embracing mentorship are instrumental in sustaining growth.
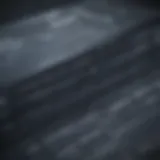
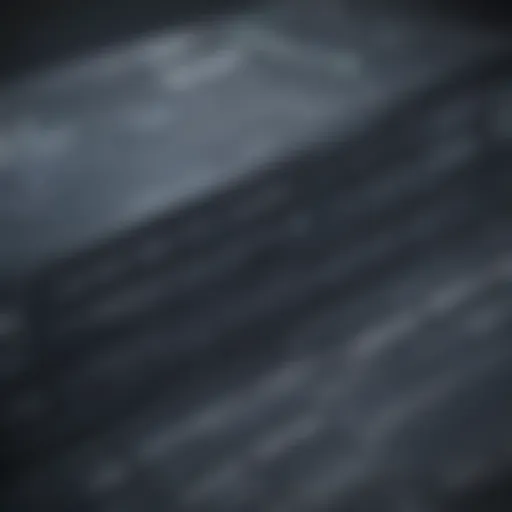