Mastering Python Debugger: A Complete Guide
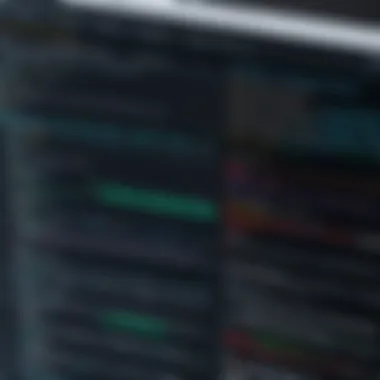
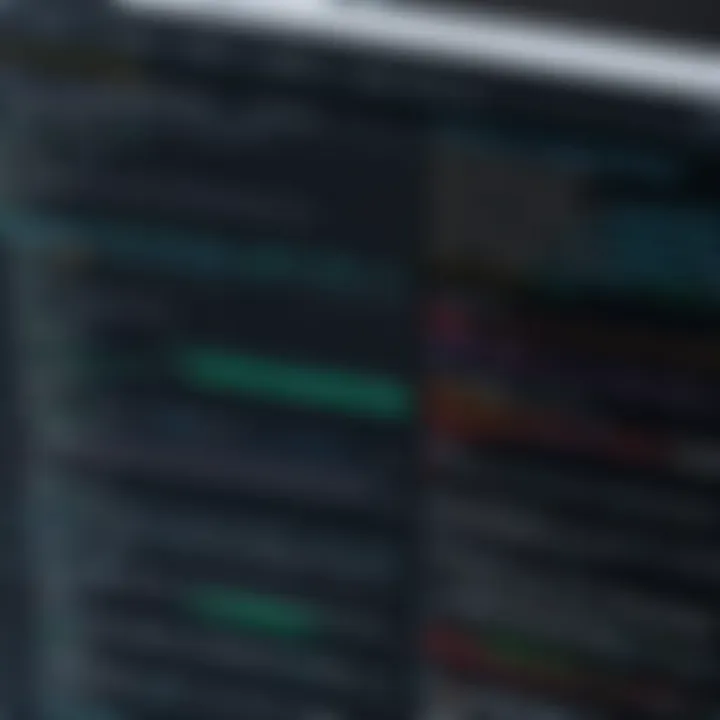
Overview of Topic
Debugging, often seen as a chore by many, stands as a critical component in the software development cycle. Think of it as the behind-the-scenes hero that ensures your code runs smoothly. A debugger is a tool that helps to track down and resolve issues or bugs in a program. In the realm of Python, mastering the debugger can transform how developers approach problem-solving, essentially heightening their coding maturity.
The importance of debugging in today's tech landscape cannot be overstated. Python's versatility, simplicity, and widespread adoption in fields such as data science, web development, and automation make it an essential language to learn. As organizations increasingly rely on Python-based software solutions, understanding how to effectively debug can set a programmer apart from the crowd.
Historically, debugging has evolved remarkably. The roots trace back to the early days of computing when a physical moth was famously removed from a computer, thus coining the term "debugging." Over the decades, the process has transformed from simple print statements to advanced tools like integrated development environments (IDEs) with sophisticated debuggers built-in.
Fundamentals Explained
Before diving deeper into how Python Debugger operates, itâs essential to grasp some foundational concepts. Debugging involves systematically identifying, isolating, and correcting problems in code. The most effective debugging strategies lean on a systematic approach rather than mere guesswork.
Important terminology includes:
- Breakpoint: A deliberate stopping point in the code to monitor variable states or flow control.
- Step Over/Step Into: Functions that let programmers execute code line-by-line for thorough examination of behavior.
- Stack Trace: A report outlining active stack frames during execution at a specific point of error, providing context to the failure.
Developers should feel comfortable working with key Python concepts, like functions, loops, and conditional statements, before tackling debugging strategies. Knowing how these elements fit together is crucial for effectively employing debugging tools.
Practical Applications and Examples
Applying your debugging skills can significantly enhance your programming competencies. For instance, a common real-world challenge is the infamous "off-by-one error". This occurs frequently in loops where the loop counter may exceed or not reach established boundaries. By employing a debugger, programmers can set breakpoints to observe variable changes during execution and effectively pinpoint these subtle errors.
In practice, letâs look at a simple example:
Here, if the expectation is to sum the numbers 0 through 4, setting a breakpoint at allows you to monitor how the variable updates with each iteration. This immediate observation can clarify whether your calculations are on the right track.
Case Study
Consider a scenario in a web application utilizing frameworks like Flask and Django. When a crucial feature fails to respond or throws an error, efficient use of Python Debugger can help locate the exact line of problematic code swiftly. This not only saves time but reduces frustration, as developers can visualize where things went awry almost instantly.
Advanced Topics and Latest Trends
As Python continues to grow in popularity, so does the complexity of the projects being developed. New debugging tools that integrate Artificial Intelligence (AI) are emerging to assist developers in spotting and fixing bugs. This next-gen debugging empowers developers to not just find errors but learn from patterns in past mistakes.
Moreover, the integration of debugging tools with version control systems like Git is reshaping how teams collaborate on projects. Understanding how to debug collaboratively is becoming just as crucial as individual debugging skills. Upcoming trends indicate a shift towards interdisciplinary team dynamics, combining builders and testers more closely, fostering a culture of quality code from the start.
Tips and Resources for Further Learning
For those keen to elevate their debugging skills, several resources stand out:
- Books: "Python Crash Course" by Eric Matthes offers practical projects to sharpen your skills.
- Online Courses: Platforms like Coursera and Udemy provide tailored Python courses focusing on debugging techniques.
- Communities: Engaging with communities on sites such as Reddit can provide real-world scenarios and solutions for debugging in Python.
Additionally, utilizing tools like PyCharm or Visual Studio Code can streamline your debugging processâeach featuring built-in debuggers that enhance the coding experience.
Debugging in Python, when approached effectively, not only simplifies resolving issues but promotes a deeper understanding of programming as a whole. With the foundational knowledge laid out, one can delve into the nuances of each tool and practice methods that will elevate their programming mastery.
Understanding Python Debugger
Debugging is the backbone of programming, acting as the lighthouse guiding developers through the treacherous waters of code errors. A deep grasp of the Python debugger is not just beneficialâit's often essential for anyone serious about writing robust Python applications. This section will thoroughly dissect not only what makes Python's debugging tools stand out but also how they can transform your coding practices.
Overview of Debugging in Programming
Debugging in programming generally refers to the methods used to identify and fix errors or bugs in code. Picture it as a detective work, where each line of code tells a story. When the narrative doesn't flow, that's when the bugs rear their ugly heads. The debugging journey starts with locating the culpritâwhether itâs a logical flaw, syntax error, or even an intricate issue buried deep within a loop.
With Python, the landscape of debugging has a structure that accommodates both simplicity and complexity. The language's readability allows newcomers to pick it up with relative ease, but as you dive deeper, the need for effective debugging becomes evident. For example, when programmers hit a snag, like an unexpected output or a crash, the debugging process allows them to step back, reassess, and ultimately solve the riddle.
"A good programmer is not someone who just writes code well; it's someone who knows how to debug it effectively."
Purpose and Importance of Debugging
The purpose of debugging goes beyond merely getting rid of errors. It's fundamentally about improving code: making it more efficient, readable, and resilient. In an era where software runs the show, ensuring that applications work as intended is not just a concern, it's a necessity. When developers invest time in debugging, theyâre investing in the longevity and maintainability of their code.
Here are some key points regarding the importance of debugging:
- Cost-Effective: Finding and resolving bugs early can significantly reduce the costs associated with deployment failures or system outages.
- User Satisfaction: A smoother experience for users is often achieved through properly debugged applications. A robust program is likely to garner positive feedback.
- Deepens Understanding: Debugging forces developers to dissect their logic and choices. This leads to a stronger command over programming skills and typically fosters a better overall design philosophy.
- Easier Collaboration: Clear, debugged code can benefit teams working on projects together, reducing the friction often caused by misunderstandings in shared codebases.
In summary, understanding the mechanics of the Python debugger not only equips you with the necessary skills to identify and fix problems but also sharpens your overall proficiency as a programmer. Recognizing the nuances of debugging can be a game changer, leading to cleaner, more effective code that stands the test of time.
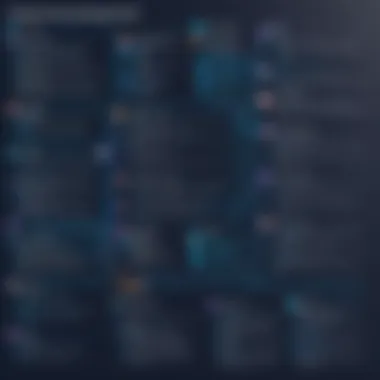
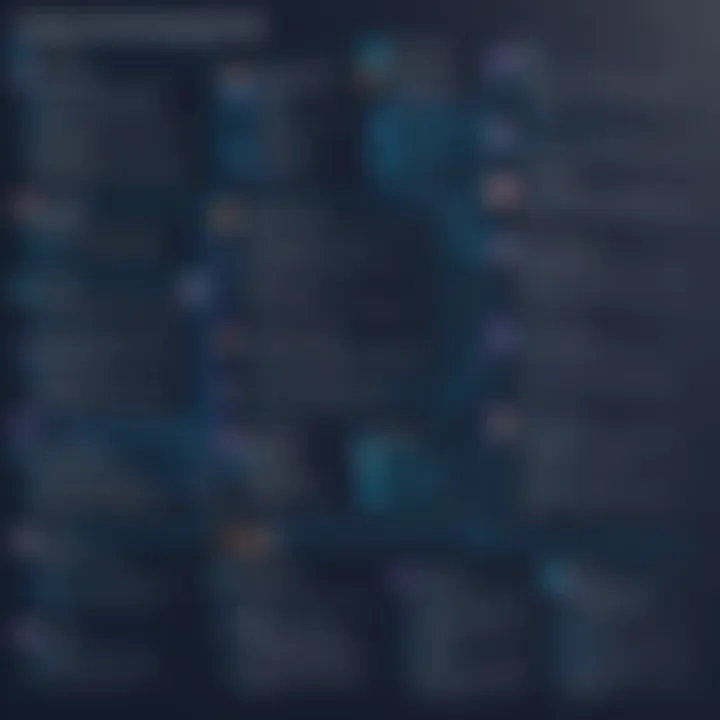
Prelims to Python Debugger
In the world of coding, errors can sneak in like uninvited guests at a party. The importance of debugging cannot be overstated, especially when it comes to programming languages like Python. This section lays the groundwork for the concepts, tools, and techniques associated with Python Debugger, setting the stage for a more solid understanding of how to effectively identify and rectify errors in your code.
When you think about it, debugging is more than just fixing mistakes; itâs a vital part of the programming life cycle. It helps you understand the code youâve written, and it opens doors to learning and improving as a developer. If youâre a student or an IT professional, grasping how the Python Debugger operates is a key element in honing your skills.
What Is Python Debugger?
Python Debugger, often referred to as pdb, is a built-in tool that allows developers to debug their Python programs systematically. Think of pdb as a magnifying glass for your code, enabling you to inspect every line and variable to spot issues lurking beneath the surface. It can be wielded in a command-line interface, making it both powerful and versatile.
The essence of pdb lies in its ability to set breakpoints, step through code, and inspect variables. When an application runs, the Debugger catches problems before they snowball into a major headache. You can think of it as running a detective agency for your code, piecing together clues and conducting thorough investigations to resolve issues.
Key Features of Python Debugger
The functionality offered by Python Debugger is designed to streamline the debugging process. Here are some of its key features:
- Step Execution: This allows you to run your code line by line, which is crucial when you want to understand how different parts of your code interact with each other.
- Breakpoints: You can set these at any line in your code, temporarily halting execution at that point. This gives you a chance to check the current state of your program.
- Variable Inspection: It allows you to observe the values of variables, which can be the difference between a simple bug and a more complex issue.
- Stack Traces: When an error occurs, you can view the call stack, which helps in determining where things went awry.
Utilizing these features effectively not only enhances your debugging experience but also improves your overall coding strategy. Increased familiarity with the Python Debugger is likely to yield better code quality and, ultimately, a smoother development process.
Common Debugging Concepts
Debugging is an inevitable part of programming, not just a mere afterthought. Itâs like learning to ride a bike; you might fall at first, but you improve as you go. In this section, we will explore the pivotal components of debugging. Understanding common debugging concepts is important because they lay the groundwork for successful troubleshooting and code refinement. Each of these elements contributes to a more effective debugging process, allowing programmers to catch errors swiftly and gain profound insights into their code.
Breakpoints and Their Functionality
Breakpoints serve as the unsung heroes in the debugging process. They mark specific lines of code where execution should pause, allowing the developer to scrutinize the state of the application at that moment. Think of breakpoints as train stops along a journey; they let you evaluate how well your train is running before it zips off again.
Utilizing breakpoints effectively can indeed save you hours of frustration. Hereâs why theyâre crucial:
- Pacing the Execution: Instead of watching an entire program run in one go, stopping at critical junctures gives you a chance to assess how the logic is unfolding.
- Variable Inspection: When execution halts at a breakpoint, developers can examine variable values in real-time. This reveals if the values align with expectations or if theyâre misbehaving.
- Tracing Issues: If a bug pops up, setting a breakpoint just before the error occurs can provide insights into why it happened. Itâs like shining a flashlight on a dark corner to spot whatâs lurking there.
You can set breakpoints in many Python debugging tools, like pdb (the built-in Python Debugger). For example:
Step-by-Step Execution Techniques
Running your program line by line can unveil the inner workings of your application. This approach promotes an in-depth understanding of each operation and is a valuable technique in a debuggerâs toolkit. By stepping through your code, you can observe how variables change and how functions get calledâessentially tracing the path taken by data.
Hereâs why this method proves useful:
- Methodically Analyze Logic: Each line of code is executed one at a time, making it easier to pinpoint where things start to go awry.
- Immediate Feedback: Seeing the output or state of the program right after executing a line can reveal unexpected behaviors on-the-fly.
- Enhanced Control: This technique provides granular control over the execution flow, as you can decide to step over or into functions depending on what you wish to inspect.
You might employ this technique in various debugging environments, utilizing commands like , , or , which guide how your program navigates between lines.
Inspecting Variables and Their Values
When debugging, variable inspection is akin to examining artifacts at an archaeological dig. Each variable holds clues about the functionality of your code and its ultimate success or failure. Understanding the values stored in your variables at various points helps in making sense of the overall behavior of the application.
Hereâs how variable examination enhances debugging:
- Clarifying Assumptions: Developers might assume a value is one thing but find out itâs different. Inspecting allows you to confirm or refute these assumptions decisively.
- Understanding Flow: Knowing variable states at different execution stages paints a clearer picture of the programâs flow, enabling better decision-making on fixes or optimizations.
- Spotting Erroneous Modifications: If a variableâs value seems to change unexpectedly, closer inspection may reveal where your program diverges from intended behavior.
Python debuggers like pdb allow you to print or evaluate variable values seamlessly:
Setting Up Python Debugger
Setting up a Python debugger is a key milestone for anyone looking to enhance their coding skills. Debugging isnât just about fixing bugs; itâs also about understanding your code better. In this section, weâll dive into the vital components of integrating a debugger into your Python environment and configuring it for maximum productivity.
Integrating Debugger in a Python Environment
Integrating a Python debugger into your coding environment makes all the difference when tackling code issues efficiently. The first step is to ensure you have the right tools at your disposal. Python provides built-in options, such as , which can be utilized directly from the command line. You can start by importing it into your script and calling it as follows:
This one line will allow you to pause execution at the point of interest, enabling you to inspect variables and step through the code as needed. Alternatively, many Integrated Development Environments (IDEs) like PyCharm, Visual Studio Code, and IDLE offer more advanced features and user interfaces for debugging.
Things to Consider:

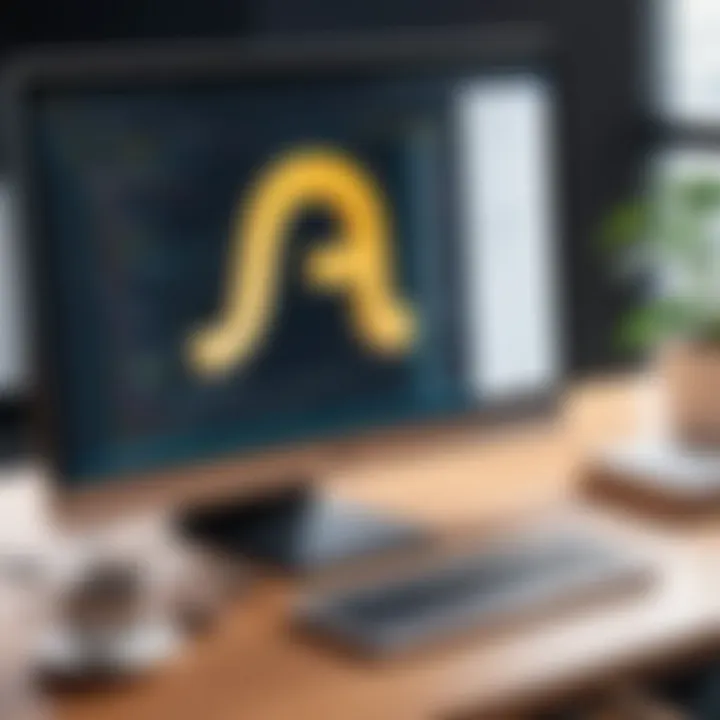
- Ensure your Python version is compatible with the debugger you choose.
- Familiarize yourself with the debugger's specific commands and functionalities.
- Opt for a development environment that supports direct debugger integration to make the entire process seamless.
Configuring Debugger Settings for Optimal Use
Once the debugger is integrated, the next step is to configure its settings to fit your development style. Generally, it involves customizing breakpoints, choosing how code execution should proceed, and adjusting variable inspections.
- Breakpoint Configuration: You might be inclined to set breakpoints at multiple line numbers but remember, too many can clutter your view. Focus on key points.
- Adjusting Execution Flow: Depending on the complexity of your application, it could be beneficial to specify whether you want to step into functions or just run them directly.
- Variable Viewing: Some setups allow you to view changes in variable states in real-time, which can be tremendously helpful. Make sure to enable this feature if available.
"Configuring your debugger can drastically reduce trial-and-error time, making your coding journey smoother and more productive."
By fine-tuning these settings to your requirements, you can establish an efficient debugging workflow that not only spots bugs but also enhances your understanding of the code. Debugging can be a critical part of development; a well-set-up environment is akin to having a reliable compass when navigating through complex code.
Break down tasks and remember that getting your debugger set up properly pays dividends down the line, as each effective solution brings you closer to mastering Python programming.
Types of Debuggers in Python
Debugging is an essential skill for any programmer, and knowing about the different types of debuggers available in Python can be a game changer. Understanding these tools is crucial because they can significantly improve the efficiency and effectiveness of your debugging efforts. When tackling all sorts of programming challenges, having the right debugger for the task at hand can make life a lot easier. Let's peel back the layers and scrutinize two main categories of debuggers in Python: the built-in debugging tools and the third-party libraries.
Built-in Debugging Tools in Python
Python offers several built-in debugging tools that can be quite handy. Among these, the most prominent is the PDB (Python Debugger). This tool is a part of the standard library and allows you to set breakpoints in your program, inspect variables, and step through your code line by line.
Key Features of PDB:
- Interactive Debugging: PDB runs in the command line, allowing users to execute commands directly and interactively.
- Breakpoint Management: You can set breakpoints at specific lines to pause execution and investigate the state of your program, which can save you from going down a rabbit hole of confusion.
- Variable Inspection: With commands like (print) and , you can quickly look at variable values and data types, helping pinpoint where things may have gone wrong.
Utilizing these built-in tools means not having to rely on external libraries, making them a go-to option for many. Theyâre integrated right into Python and suited for straightforward debugging tasks.
Third-Party Debugging Libraries
Besides the in-house tools, Python has a rich ecosystem of third-party debugging libraries that can enhance the debugging experience. Two noteworthy mentions are pudb and pydevd.
- pudb: This library provides a full-screen, console-based visual debugger. It's designed for those who prefer a more visual approach as it displays stack frames and variables in a clearer manner.
- pydevd: As part of the PyDev project, this debugger is particularly useful if you're using IDEs like Eclipse or PyCharm. It provides powerful debugging features and seamlessly integrates with these environments.
When choosing between built-in and third-party tools, consider factors like project requirements, personal preference, and the specific bugs you encounter. Each debugger has its strengths and weaknesses, and being informed about these can vastly improve your ability to troubleshoot effectively.
"Understanding the right tools for the job is half the battle in programming. Choose wisely."
In summary, whether you opt for Python's built-in tools or delve into external libraries, each type of debugger serves a unique purpose. By mastering their functionalities, you can navigate the often rocky road of programming with greater confidence and finesse.
Debugging Strategies and Best Practices
Effective debugging goes far beyond just identifying and correcting errors; it requires a structured approach that maximizes efficiency and reduces frustration. Developing sound debugging strategies and practices plays an integral role in both individual and collaborative programming environments. This section emphasizes specific elements necessary for creating a seamless debugging experience, outlines the tangible benefits of a methodical approach, and discusses important considerations that can help to streamline your workflow.
Creating Efficient Debugging Workflows
An efficient debugging workflow can save programmers significant time and effort. A systematic approach generally involves defining a sequence of steps that ensure nothing is overlooked. Hereâs how to create an effective debugging workflow:
- Outline the Problem: Start by documenting what the problem is. Clearly state what the expected outcome should be and whatâs currently happening. This provides a reference point.
- Reproduce the Issue: Consistently reproduce the error to understand under what conditions it appears. Knowing when and why an issue occurs is crucial.
- Isolate the Section of Code: Determine the part of the codebase that may be contributing to the problem. Narrowing it down makes it easier to focus your debugging efforts.
- Test Hypotheses: Formulate hypotheses about what might be going wrong and test them. If you suspect a variableâs value is incorrect, print it out or use a debugger to single-step through the code.
- Use Version Control: Version control systems like Git can be invaluable. Being able to compare changes or revert to a previous version can often highlight what introduced the bug.
- Document Findings: As you debug, note what youâve discovered. This can be exceedingly useful not just for you but for others who may face similar challenges.
Utilizing these steps not only refines your process but also contributes to a culture of thoroughness in coding practices.
Common Pitfalls to Avoid in Debugging
While there are many effective strategies for debugging, there are also several pitfalls that developers frequently encounter. Avoiding these common mistakes can make the process much smoother:
- Over-Reliance on Print Statements: While they can be helpful, relying solely on print statements can lead to cluttered code and may not provide sufficient information about the programâs state. Use a debugger to step through your code instead.
- Ignoring Compiler/Interpreter Feedback: Many programmers overlook warnings and messages generated by tools. It is vital to listen to these signals as they often indicate what's wrong or what needs addressing.
- Skipping Tests: Neglecting to run tests can also come back to bite developers later. Unit tests should not be an afterthought; theyâre good indicators of the solidity of your code.
Remember: An ounce of prevention is worth a pound of cure.
- Getting Stuck in One Mindset: If you find yourself solving a problem with the same approach repeatedly, it might be time to consider alternative methods or even to consult someone else about it. Fresh perspectives can often lead to solutions that were right under your nose.
By steering clear of these common pitfalls, programmers can enhance their debugging effectiveness and foster a more accurate coding environment.
In summary, embedding debugging strategies and best practices into your development routine ensures a smoother coding journey. Focusing on efficient workflows and being aware of common traps allows programmers to tackle bugs proactively rather than reactively. This engagement not only benefits individual projects but enhances overall team productivity, getting you closer to your development goals.
Debugging in Complex Systems
Debugging in complex systems can feel like navigating a labyrinth. The intricacy of software that handles multiple processes or threads often obstructs visibility, making it a challenge to pinpoint exact problems. As projects grow and integrate various components, the probabilities of bugs increase exponentially. Recognizing how to manage these complexities is pivotal for effective development.
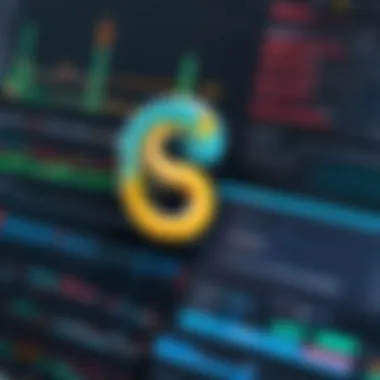
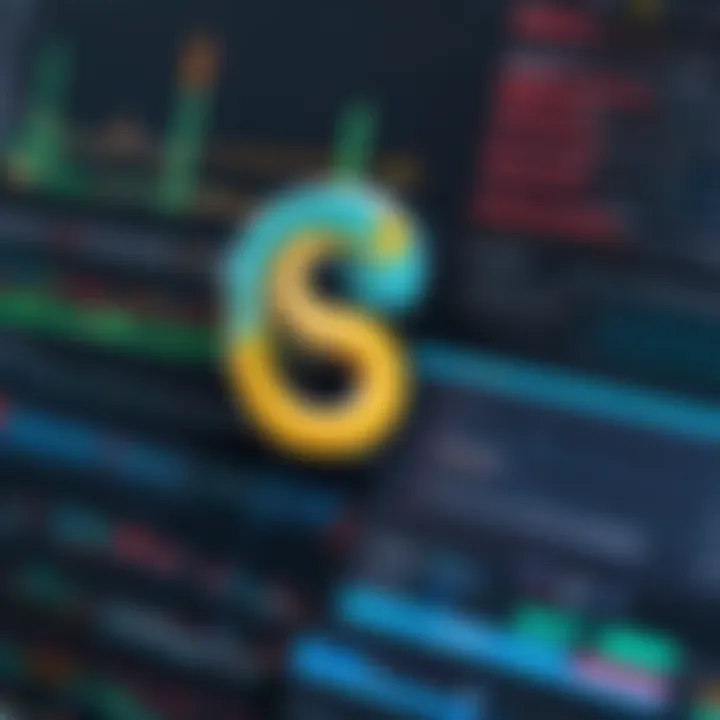
The importance of debugging in such systems cannot be overstated. When youâre dealing with numerous interdependent parts, a failure in one area can trigger a domino effectâcausing unexpected issues elsewhere. Thus, mastering debugging techniques becomes crucial not just for finding bugs but for preventing them in the first place. Here, weâll zero in on two key areas of focus: managing exceptions and tackling multithreading issues.
Handling Exceptions and Errors
In programming, exceptions represent errors that disrupt normal flow. Understanding how to handle these exceptions efficiently is a huge part of debugging. Imagine youâre working on a project that interfaces with an external API; if an unexpected response format is returned, it could result in your application crashing. Handling exceptions allows your code to continue running despite these errors.
First, identify common exceptions using your debugger. Here are actionable strategies:
- Use Try-Except Blocks: This structure helps in controlling what happens when an error arises. For example:
- Log Errors: Instead of just printing exceptions, logging them can be beneficial. Use Python's built-in logging module to keep a record of what went wrong without cluttering the console.
- Be Specific: Catch specific exceptions rather than using a generic Exception. This helps pinpoint errors without glancing over valid ones.
Adopting these practices allows developers not only to debug effectively but also to build more robust applications that handle errors gracefully.
Debugging Multithreaded Applications
Multithreading introduces even more complexity to your debugging efforts. When multiple threads run concurrently, bugs can manifest in elusive ways, like race conditionsâwhere the order of operations affects the outcome. To tackle these challenges, it's vital to approach debugging with a systematic mindset.
Consider the following:
- Isolate Thread Behavior: During debugging sessions, run single-threaded versions of your application first. This can help confirm whether an issue resides in logic or multithreading.
- Utilize Thread-Safe Practices: Ensure that data shared between threads is protected, using locks or other synchronization mechanisms.
- Debugging Tools: Use tools like PyCharm or Visual Studio Code which provide suitable debugging capabilities, including thread state visualization. With these tools, you can see what each thread is doing in real-time.
To underline the importance of adeptly debugging multithreaded applications, remember the adage:
"A watched pot never boils."
In this context, it reminds us that without proper observation of thread activity, issues may simmer beneath the surface, waiting to cause significant problems.
By prioritizing these debug strategies, developers can create clean, reliable systems, even amidst complexity.
Real-World Debugging Examples
Debugging isnât just an abstract practice; itâs a vital skill that finds its footing in real-world applications. When youâre neck-deep in code, dealing with actual issues that affect users or systems, thatâs where the rubber meets the road. Understanding how to navigate the debugging process can save countless hours of frustration and improve the overall performance of applications.
Real-world debugging examples serve several purposes. They not only illustrate common issues developers might face but also highlight effective strategies for resolution. This section will delve into practical cases, the nuts and bolts of debugging a web application, and the most frequent bugs programmers stumble upon, presenting solutions that have been tried and tested.
Case Study: Debugging a Web Application
To bring the concept of debugging to life, letâs consider a typical scenario involving a web application, such as an e-commerce platform. Imagine a user trying to add a product to their cart but hitting a snag where the cart fails to update. This glitch could stem from various issuesâmaybe itâs a front-end problem, or perhaps thereâs an underlying server issue that needs addressing.
Using a debugger, the development team can pinpoint exactly where things are going awry. They might set breakpoints at pivotal locationsâsay, when the add-to-cart function is initiated. By stepping through the process, they can inspect variables at each stage to find out whether the product ID is correctly passed and whether the API is responding as expected.
Throughout the debugging process, the team could leverage built-in logging tools to capture real-time data about the applicationâs behavior. A solid approach could blend both visual debugging tools and console logs for comprehensive insights.
Common Bugs and Their Resolutions
As experienced developers know, bugs come in all shapes and sizes. Addressing each one requires a sharp eye for detail and a systematic approach. Some of the most prevalent issues include:
- NullReferenceException: This arises when a code attempts to access an object that isnât initialized. A good resolution here is to ensure all objects are properly instantiated before use.
- Infinite Loops: These can cause an application to hang. A meticulous review of loop conditions can help identify where the exit criteria were missed.
- Incorrect Data Types: For instance, attempting to perform a mathematical operation on a string type can confuse most debuggers. Adjusting the data type appropriately fixes this problem.
When tackling these bugs, a developer can start by reproducing the issue, employing the debugger to both observe variable states and see how they change over time. Proper documentation of each step taken can also provide a valuable resource in future debugging efforts.
Always remember, understanding the context of a bug within the broader application can lead to quicker and more effective resolutions.
In sum, the art of debugging isnât merely about fixing what's brokenâit's about fostering a mindset of problem-solving and continuous improvement. As Python developers hone their debugging skills in real-world contexts, they not only become more proficient in their craft but also contribute to better-functioning, more reliable software.
Future Trends in Debugging Tools
As the landscape of software development evolves, debugging practices are undergoing significant transformations. These changes are not just a matter of convenience but are becoming crucial for managing increasingly complex software systems. Understanding future trends in debugging tools can equip programmers with the necessary skills to stay ahead of the curve.
Integrating Artificial Intelligence in Debugging
Artificial Intelligence (AI) is making strong inroads in the development of debugging tools. The potential benefits are vast, ranging from automating mundane tasks to providing predictive analysis of bugs. For instance, imagine a scenario where an AI system analyzes the codebase and identifies common patterns in previous errors. Such a system could preemptively notify developers of potential issues before they ever arise.
Some key advantages include:
- Automated Bug Detection: AI can analyze large amounts of code much faster than a human could, flagging potential issues based on learned patterns.
- Enhanced Code Quality: AI-assisted debugging can improve the quality of code by suggesting optimizations and finding vulnerabilities that might be missed otherwise.
- Continuous Learning: AI tools can continuously learn from new code submissions, adapting their understanding of what constitutes a bug in different contexts.
However, this integration isn't without challenges. Data privacy and the reliability of AI suggestions are concerns that need addressing. Developers must also be trained to interpret AI outputs, not merely rely on them.
Emerging Debugging Technologies
The tools available for debugging are becoming more sophisticated by harnessing advancements in technology. Here are a few trends worth noting:
- Real-time Collaboration: Tools that enable teams to debug issues in real time are gaining traction. They allow multiple developers to work on the same bug, which can accelerate resolution times and enhance collective learning.
- Cloud-based Debugging: Cloud environments enable developers to replicate production settings more easily. This technology helps in debugging complex issues that might be hard to reproduce locally. Code can be tested in virtualized environments that mimic the actual deployment.
- Visual Debugging Interfaces: User-friendly interfaces are becoming a staple. These tools help developers visualize code execution paths and variable states, making it easier to pinpoint where things go awry. Instead of sifting through lines upon lines of code, a graphical representation can often reveal insights more directly.
The advancement in debugging technologies will significantly reshape how developers approach problem-solving, ultimately leading to more robust software and efficient workflows.
Keeping abreast of these trends will not only enhance debugging efficiency but also improve overall project outcomes. As the complex systems we build become more reliable, so too does our confidence in the debugging tools we choose.