Mastering the React CI/CD Pipeline for Developers
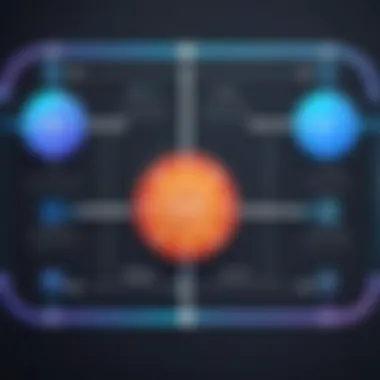
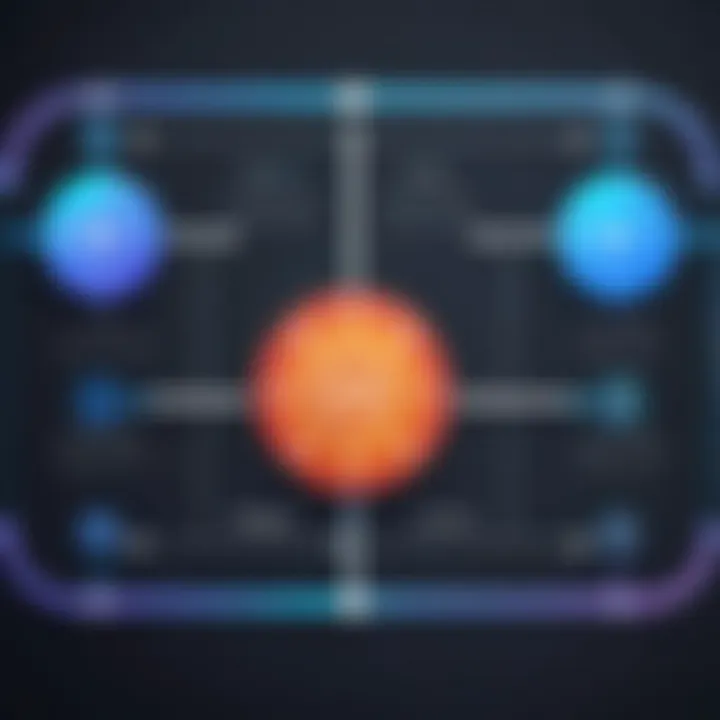
Overview of Topic
Understanding the CI/CD pipeline specific to React can greatly enhance the efficiency of your software development process. CI, or Continuous Integration, is the practice of merging all developer working copies to a shared mainline several times a day. CD stands for Continuous Deployment or Continuous Delivery, depending on the context, and relates to the automated deployment of code to production environments. This article explores the significance of these practices in modern tech, delving into their evolution and relevance.
The integration of CI/CD practices began to take shape as applications became more complex and the need for rapid delivery increased. React, a library developed by Facebook, emerged as a dominant tool for building user interfaces, aligning perfectly with CI/CD strategies by allowing for modular and reusable components.
Fundamentals Explained
To grasp the React CI/CD pipeline, understanding some core principles is essential. Continuous Integration emphasizes regular code integration into a shared repository, which helps catch bugs early and reduces integration problems. Continuous Deployment aims at ensuring that changes can be deployed to production environments efficiently and without manual intervention.
Key terminology includes:
- Build: The process of converting source code into a standalone form that can be run on a different computer.
- Test: A mechanism to check if the application behaves as expected.
- Deployment: The act of making your application available for users.
Understanding these components is critical to implementing a successful pipeline in your React projects.
Practical Applications and Examples
The practical application of React in a CI/CD framework can be illustrated through real-world case studies. Many organizations, like Facebook, utilize continuous deployment to enable faster releases. When you write a component in React, it is tested in various stages of the pipeline.
For example, one might set up a GitHub Actions workflow that contains several steps to build, test, and deploy a React application automatically. Here is a simplified example of what this might look like in a workflow file:
This illustrates a basic setup to get your application from code to deployment, integrating testing thoroughly along the way.
Advanced Topics and Latest Trends
In the realm of CI/CD, there are various advanced techniques and emerging trends. For instance, the use of microservices in combination with React can lead to a more robust architecture that enhances efficiency. Tools like Docker are becoming critical in this space as they allow for consistent environments from development through production.
Additionally, the rise of serverless architecture is influencing how applications are deployed. Serverless platforms allow developers to run code without managing servers, aligning perfectly with CI/CD methodologies to optimize workflows.
Tips and Resources for Further Learning
For those looking to expand their understanding of the React CI/CD pipeline, several resources can be of assistance:
- Books: "Continuous Delivery: Reliable Software Releases through Build, Test, and Deployment Automation" by Jez Humble and David Farley.
- Online Courses: Platforms like Coursera and Udemy offer courses focusing on CI/CD practices.
- Communities: Engaging in discussions on platforms like Reddit can provide insights from industry professionals. Additionally, visiting websites such as Wikipedia or Britannica can give historical context and definitions.
By leveraging these resources, developers can continue to grow and refine their CI/CD practices within React, ensuring that they remain at the cutting edge of software development.
Preamble to / in React
In the realm of modern software development, the integration of Continuous Integration and Continuous Deployment (CI/CD) systems into workflows is invaluable. React, a popular JavaScript library, benefits greatly from these methodologies. CI/CD transformation is not merely a trend; it is swiftly becoming a necessary facet of any successful development strategy. Utilizing CI/CD enables teams to gain efficiency and agility when developing applications, which is essential for React developers facing tight deadlines.
Defining /
Continuous Integration (CI) is a development practice that encourages developers to frequently merge their code changes into a central repository. Each integration is verified by an automated build and automated tests, allowing teams to detect problems early. Continuous Deployment (CD) builds upon this foundation by automatically deploying all code changes to production after the build stage. This combination allows for rapid release cycles and a more streamlined development process.
Importance of / for React Development
For React development, implementing a CI/CD pipeline can lead to significant benefits:
- Early Error Detection: Frequent integrations lead to fewer integration issues. It becomes easier to identify and fix errors, reducing the complexity involved in debugging.
- Faster Release Cycles: Automation in deployment minimizes waiting time for developers. New features and bug fixes can reach users more swiftly, maintaining momentum in an agile environment.
- Improved Collaboration: CI/CD fosters a culture of collaboration among team members. With clear visibility of changes and automated processes, all contributors can stay aligned.
- Consistency and Reliability: Automation ensures that the deployment process is repeatable, leading to consistent application behavior and a reliable user experience.
"Implementing a CI/CD pipeline is not simply an enhancement; it is essential for efficiently managing projects in today’s fast-paced development landscape."
Understanding the significance of CI/CD in React development equips teams to optimize their workflows and improve productivity. By recognizing the foundation of CI/CD along with its tangible advantages, developers can position themselves to advance not only their applications but their overall programming proficiency.
Core Concepts of Continuous Integration
Continuous Integration, often abbreviated as CI, is a critical aspect in the realm of software development. In the context of React, CI facilitates a seamless development flow, enabling developers to integrate code into a shared repository frequently. This concept's core benefits revolve around enhancing collaboration, improving quality, and accelerating the release cycle.
One of the main advantages of adopting CI is the early detection of integration issues. With regular code commits, conflicts are identified promptly. This allows developers to address problems immediately, rather than noticing them later in the development cycle when they may become more complex and time-consuming to fix.
Build Automation
Build automation refers to the process of compiling source code into executable code without requiring extensive manual input. In a CI environment, automated builds are triggered with each code commit. This creates a consistent environment that mimics production settings, aiding developers in verifying the integrity of their code changes quickly.
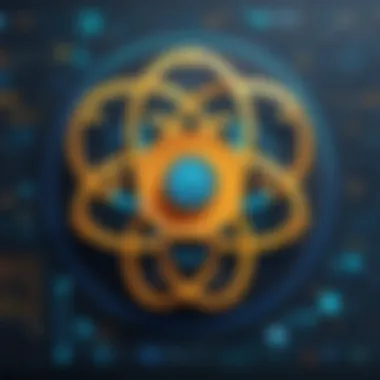
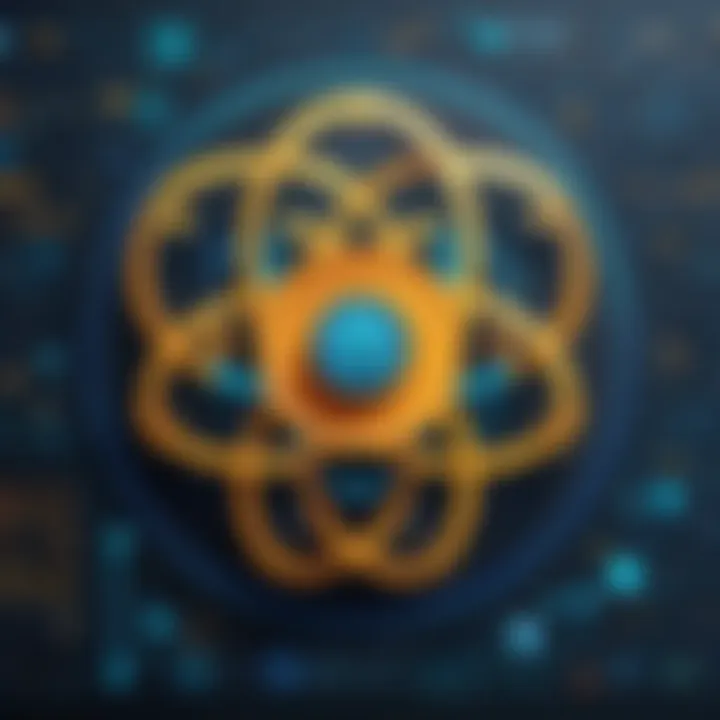
The setup of build automation can involve various tools such as Jenkins or GitHub Actions. These tools are designed to detect changes in the codebase and initiate builds automatically. Adding build automation to the CI process enhances productivity by saving time, ensuring that code can be tested and integrated without manual interference. This ensures that potentially faulty code is identified early before progressing to deployment.
Moreover, automated builds allow for reproducible builds, offering a standardized process for all developers. Consistency across builds can reduce unexpected behavior when code is moved between environments. This becomes especially critical in collaborative projects where multiple developers might be working on different features simultaneously.
Automated Testing
Automated testing is another fundamental pillar of Continuous Integration. This encompasses various testing methodologies, including unit testing and end-to-end testing. By running automated tests with every build, developers can validate code changes against predefined criteria, ensuring that changes do not introduce new bugs or regressions.
The integration of automated testing tools, such as Jest for unit tests in React applications, enhances reliability. These tools provide immediate feedback on the impact of code changes, promoting a culture of quality. In addition, automated tests are less error-prone compared to manual testing, especially as the codebase grows.
It's essential to remember that establishing a robust suite of automated tests requires an initial investment of time and effort. However, over time, the return on investment becomes apparent as the team spends less time on debugging during later stages of development and more time on creating new features.
In summary, the core concepts of Continuous Integration—build automation and automated testing—are indispensable for effective React development. They not only improve code quality but also streamline the development process, making it crucial for maintaining agility in today’s fast-paced software landscape.
"Continuous Integration is not just about tools; it is a mindset that advocates for frequent integration, leading to better productivity and higher quality products."
By understanding and implementing these core concepts, developers can create a resilient CI pipeline that significantly enhances their workflow while minimizing the risk of integration-related issues.
Understanding Continuous Deployment
Continuous Deployment (CD) is a vital phase in the software development lifecycle, particularly in the context of React applications. It refers to the automated release of software changes to production, ensuring that every modification that passes the automated tests can be deployed as soon as it is ready. This practice offers several benefits and considerations that influence how development teams handle updates, manage releases, and maintain their applications.
Deployment Strategies
When discussing deployment strategies, it is crucial to understand the various approaches developers can choose. These strategies dictate the path a new application version takes from development to production. Some common deployment strategies in React CI/CD include:
- Blue-Green Deployment: This strategy involves two identical environments. One environment runs the current application version while the other hosts the new version. After testing the new version, traffic is switched to the updated version, minimizing downtime.
- Canary Releases: This approach allows developers to roll out changes to a small subset of users before a full-scale launch. By monitoring the new version's performance, teams can identify issues without affecting the majority of users.
- Rolling Updates: This method updates the application in phases. A small number of servers are updated at a time, allowing users to experience the new features while other servers still run the older version.
Each strategy has its own pros and cons concerning risk management, user experience, and operational complexity. Companies must evaluate their specific needs to determine which approach aligns best with their operational goals.
Rollback Mechanisms
Effective rollback mechanisms are equally important in a continuous deployment environment. Despite thorough testing, issues may arise once the new version goes live. A rollback mechanism allows teams to revert to a previous stable version quickly. Some key aspects of rollback mechanisms include:
- Automated Rollbacks: Implementing automated triggers that can revert to a previous version upon detecting failures can save precious time and reduce manual overhead.
- Version Control: Using tools like Git enhances rollback capabilities by keeping a history of all changes. This can facilitate seamless transitions back to stable states without losing significant work.
- Testing Rollback Procedures: Practicing rollback scenarios is essential. Teams should routinely test their rollback plans to confirm they can return to a working state smoothly and with no disruption to their services.
"A robust rollback mechanism not only provides safety nets but also builds confidence in rapid deployment practices."
Understanding Continuous Deployment is not just about tools or processes; it's a strategic mindset that prioritizes stability and reliability while enabling innovation. Adoption of effective deployment strategies, along with solid rollback mechanisms, can mitigate risk and enhance the overall quality of a React application's lifecycle.
Tools and Frameworks for / with React
In the context of React development, selecting appropriate tools and frameworks for CI/CD is crucial. These tools enhance the development experience by automating processes that involve integration, testing, and deployment. They thus enable teams to deliver software faster and with higher quality. The right tools can save time and reduce errors, making the development lifecycle more efficient.
Version Control Systems
Version control systems (VCS) play an integral role in CI/CD workflows. They allow developers to track changes in code, collaborate effectively, and revert to previous versions if necessary. Using version control is essential for managing project histories and facilitating team collaboration.
Popular systems such as Git are widely adopted in the React ecosystem. These systems offer branching and merging capabilities, enabling multiple developers to work concurrently without conflicts. Moreover, VCS can integrate with CI/CD platforms to automate build processes whenever code is pushed or merged. This seamless integration enhances the CI/CD pipeline significantly.
/ Platforms
CI/CD platforms are essential in automating the integration and deployment processes. They provide a framework where code changes can be tested and deployed automatically. Here are some well-regarded CI/CD platforms:
GitHub Actions
GitHub Actions is a powerful tool that allows developers to automate their workflows directly in GitHub repositories. A key characteristic of GitHub Actions is its tight integration with GitHub, providing an efficient way to trigger actions based on repository events. This is a beneficial choice for those already using GitHub for version control.
A unique feature of GitHub Actions is the ability to define custom workflows that can include multiple steps, such as building the application or running tests. This allows for a highly customizable CI/CD pipeline. One potential disadvantage is that complex workflows may require a steeper learning curve for newcomers.
CircleCI
CircleCI is another popular CI/CD platform known for its ease of use and flexible configuration. It offers a key characteristic of parallel execution, enabling multiple jobs to run simultaneously, which can significantly reduce build times. This can be a valuable asset in a fast-paced development environment.
CircleCI allows for easy integration with various version control systems and supports Docker natively. However, teams may find that CircleCI's free tier has limitations in concurrency and build minutes, necessitating a careful consideration of costs as projects scale.
Travis
Travis CI is well-known for its simplicity and integration with GitHub. One strong point of Travis CI is its straightforward configuration via a file. This approach allows developers to define the build environment with ease. It is a beneficial choice for open-source projects, as Travis CI offers free usage for public repositories.
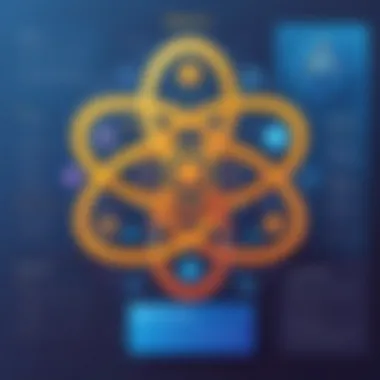
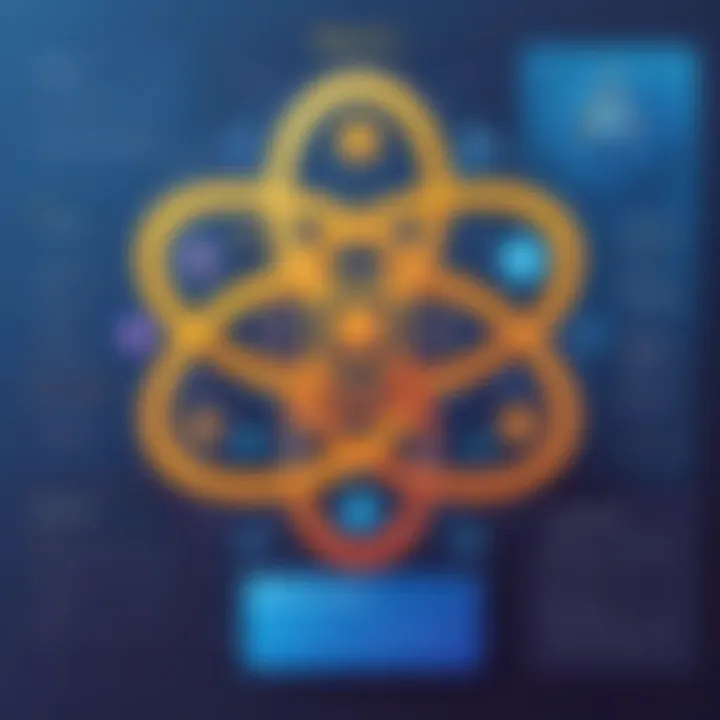
A unique feature of Travis CI is its ability to support multiple languages, including JavaScript, Ruby, and Python. However, some users report that it can be slower compared to other platforms when handling larger codebases or complex builds.
Containerization Tools
Containerization is another fundamental aspect of modern CI/CD. By encapsulating applications in containers, developers ensure consistency across different environments. Two widely used containerization tools are Docker and Kubernetes.
Docker
Docker simplifies the process of deploying applications in containers. One key characteristic of Docker is its ability to create a consistent development environment, eliminating discrepancies between development and production. This is especially valuable in a React CI/CD pipeline.
Docker's unique feature is its lightweight containers, which can run anywhere Docker is installed. This flexibility is a significant advantage, but managing multiple containers can become complex as projects grow.
Kubernetes
Kubernetes is an orchestration tool for managing containerized applications. It offers robust scaling and automated deployment features. A key characteristic of Kubernetes is its ability to manage many containers across clusters, optimizing resource usage and improving reliability.
A unique feature of Kubernetes is its support for self-healing. It automatically replaces and reschedules containers that fail, enhancing uptime. However, the learning curve can be steep due to its complexity and extensive feature set.
Setting Up a React / Pipeline
Setting up a React CI/CD pipeline is critical for ensuring a streamlined workflow in modern software development. A well-configured CI/CD pipeline automates processes that increase efficiency in the development lifecycle. This section will break down essential elements that contribute to effective pipeline setup. The benefits include reduced integration issues, quicker feedback loops, and more reliable deployments. However, there are important considerations to keep in mind, such as selecting appropriate tools and appropriately scaling the environment.
Creating the Initial Setup
Establishing your initial setup requires a few straightforward steps. First, ensure you have a version control system in place, typically Git. Repositories can be hosted on platforms like GitHub. After the setup of your Git repository, a basic project structure will be established. Begin by creating the React application using Create React App or another template if you have specific needs. This initial groundwork will set the stage for further configurations.
Configuring the Environment
Once the initial setup is in place, the next step is configuring the Continuous Integration (CI) environment. This process usually involves choosing a CI service such as CircleCI or GitHub Actions. Configuring the CI environment often includes defining workflows and setting up environments where the application code will run during testing.
Key considerations include:
- Environment Variables: These are crucial for managing secrets and configuration parameters. Store them securely in your CI tool rather than hardcoding them in your repository.
- Build Scripts: Create scripts that will validate your code. A common practice is to run scripts such as or to generate a production-ready application.
- Static Code Analysis: Integrate tools such as ESLint to enforce code quality before the main testing phase occurs.
Implementing Automated Testing
Automated testing is a vital component of the CI/CD pipeline. It helps ensure that each code change works as intended. Testing can come in many forms, including unit tests and integration tests.
You might integrate libraries such as Jest or React Testing Library for efficient validation of components.
Key steps include:
- Writing Test Cases: Ensure all components have corresponding tests that cover critical functionality.
- Running Tests in CI: Configure the CI tool to run tests automatically when new code is pushed to the repository.
- Test Reports: Make sure the CI platform provides meaningful reports on test results so that developers can easily troubleshoot failures.
In essence, setting up a React CI/CD pipeline involves careful consideration and planning. Effective execution brings clarity to the development process and enhances the overall quality of outputs.
Best Practices for React /
Implementing a CI/CD pipeline in React projects is complex. However, following certain best practices can greatly enhance the process. These practices ensure that the development workflow is efficient, maintainable, and capable of delivering high-quality software.
Maintaining Code Quality
Code quality is paramount in any development process. In the context of React CI/CD, it becomes even more critical due to the increasing complexity of applications. Maintaining high code quality involves several strategies:
- Static Code Analysis: Tools like ESLint and Prettier can help enforce coding standards. They allow developers to catch errors and stylistic issues before the code is merged.
- Code Reviews: Integrating code review processes into the CI pipeline ensures that multiple eyes evaluate changes. This practice not only identifies bugs but also promotes knowledge sharing within the team.
- Test-Driven Development (TDD): Writing tests beforehand can guide the development process, making it easier to maintain code quality while evolving features. This ensures that the code base remains robust against future changes.
By prioritizing code quality, developers can minimize bugs and improve the overall health of their projects.
Optimizing Build Times
Long build times can severely hinder productivity. To improve performance, consider the following:
- Incremental Builds: Use incremental builds to compile only the changed parts of an application. This saves time and resources, making the CI process more efficient.
- Caching Strategies: Implement caching for dependencies and build artifacts. Tools like GitHub Actions and CircleCI offer caching mechanisms that can significantly reduce build times.
- Parallel Execution: Configure your CI/CD tools to run tests or builds in parallel. This takes advantage of modern multi-core processors, reducing the overall time needed for CI tasks.
Reducing build times not only saves developer hours but also enhances the feedback loop, allowing for quicker iterations.
Effective Monitoring Strategies
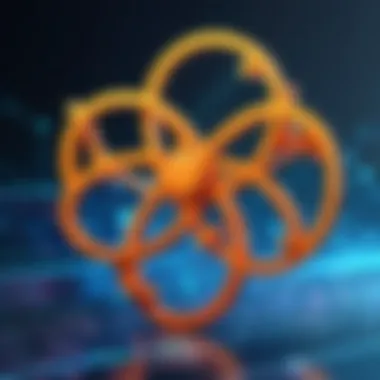
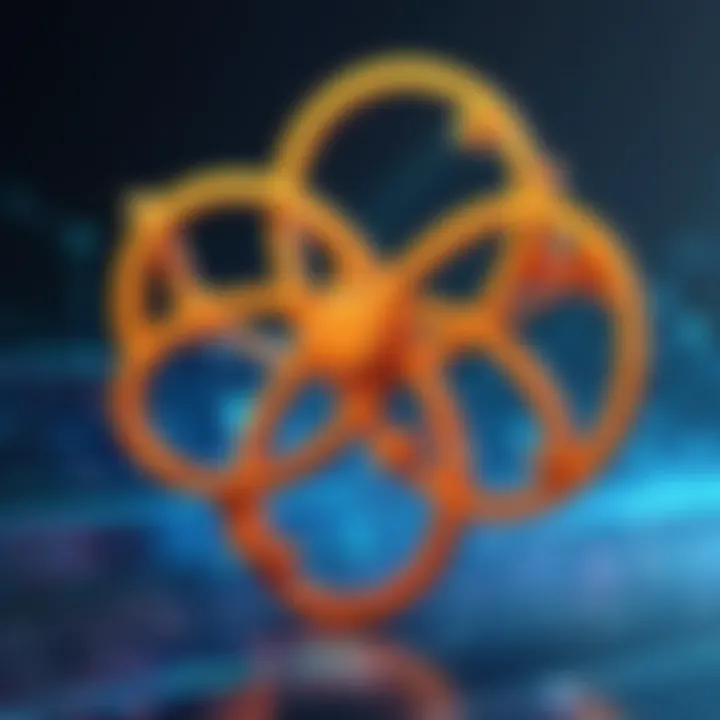
Monitoring the CI/CD pipeline is essential for proactive maintenance and ensuring the smooth operation of deployments. Effective monitoring can include:
- Log Management: Utilize centralized logging solutions to track errors and performance metrics across your pipeline. This provides insights into failures and bottlenecks.
- Automated Alerts: Set up alerts for failures or performance issues. Tools like Slack or email notifications can notify the team immediately when there’s a problem.
- Performance Metrics: Establish metrics to evaluate the performance of your application post-deployment. Monitoring key performance indicators like load times and error rates can help optimize the user experience.
An effective monitoring strategy not only identifies problems early but also ensures a reliable user experience post-release.
"A well-implemented CI/CD pipeline can significantly decrease the time-to-market for React applications while maintaining exceptional software quality."
Common Challenges in React /
The implementation of CI/CD practices in React development is not without its obstacles. Recognizing these challenges is crucial for developers and teams aiming to optimize their software development processes. In this section, we will explore common issues that arise in the context of React CI/CD, focusing on integration issues, dependency management, and scalability concerns. Addressing these aspects is key to enhancing the overall efficiency and reliability of your development pipeline.
Integration Issues
Integration issues often manifest when different components of the system are brought together. In the CI/CD pipeline, this typically includes the integration of various tools and services. When developing with React, developers frequently face scenarios where the React application does not sufficiently align with other components of the tech stack. This misalignment can lead to problems such as build failures or runtime errors.
Integration testing becomes essential in these cases. Conducting thorough tests can help in identifying discrepancies early in the development cycle. One effective strategy is the use of mock services to simulate backend interactions during integrations. By embracing this practice, developers can create a more cohesive environment where integrations occur smoothly.
Dependency Management
In any React project, managing dependencies is a significant concern. As projects grow, the number of dependencies often increases. Each dependency may have its own set of requirements, which can lead to version conflicts. These conflicts can cause unexpected behavior during the CI/CD process, leading to failed builds. The risk of breaking changes in updates from third-party libraries adds further complexity.
Adopting a solid strategy for dependency management is vital. Tools like npm or Yarn can aid in effectively managing these dependencies. Regularly auditing dependencies for vulnerabilities and ensuring compatibility across different versions is essential. Being proactive about dependency updates can help in avoiding issues before they arise.
Scalability Concerns
Scalability is another challenge that React developers may encounter in CI/CD pipelines. As applications scale, the volume of code and the number of tests can increase massively. This growth can result in longer build times and slower deployment processes, which may hinder development velocity. In a rapidly evolving tech landscape, slow pipelines can create competitive disadvantages.
To combat scalability issues, teams might explore techniques such as distributed builds or parallel testing. These methods can speed up the CI/CD process by allowing various tests or builds to run concurrently. Additionally, leveraging cloud-based CI/CD solutions often provides the necessary infrastructure to scale effectively.
"Addressing common challenges in React CI/CD is vital for maintaining a fluid development cycle. Both the teams and applications benefit from robust management strategies."
Future Trends in / for React
Continuous Integration and Continuous Deployment, or CI/CD, are key components of modern software development, especially in React projects. Keeping up with future trends in CI/CD is essential for developers to maintain competitiveness and adaptability. Understanding these trends not only helps in optimizing development workflows but also in ensuring the security and quality of applications. The forthcoming technological advancements will significantly influence how developers build, test, and deploy their React applications.
Emerging Technologies
New technologies are regularly emerging that can reshape the CI/CD landscape for React applications. One notable trend is the increasing use of serverless architecture. Serverless solutions allow developers to focus more on coding while the cloud manages server provisioning, scaling, and management. This technology aligns well with CI/CD practices, as it minimizes the backend configurations necessary for deployment pipelines.
Another area of development is the rise of microservices. This approach allows React applications to be broken into smaller services that are developed independently. Each service can have its own CI/CD pipeline, enhancing efficiency and maintaining separation of concerns.
In addition, GitOps has started to gain attention as a CI/CD practice that uses Git as a single source of truth for declarative infrastructure and applications. Changes are made through Git resources and automatically applied to the environment. This practice increases transparency and provides an auditable trail of changes made in the deployment process.
The Role of AI in /
AI is beginning to play a significant role in automating CI/CD processes. By leveraging machine learning algorithms, developers can analyze patterns in code changes, predict failures, and optimize build pipelines. One of the immediate benefits of AI integration is the enhanced ability to conduct automated testing. AI can intelligently determine which tests to run based on the nature of the recent code changes, efficiently pinpointing potential problem areas.
Furthermore, AI can assist in enhancing code quality through intelligent code reviews. It can analyze past projects for best practices and flag deviations from coding standards in real-time, thus improving overall code maintainability.
This automation saves time and increases confidence in deployed applications, reducing risks associated with human error.
In terms of deployment strategies, AI can facilitate the decision-making process on whether to promote code from staging to production. By evaluating various metrics, AI can predict the impact of deployments, helping teams deploy with better certainty.
In summary, staying informed about these emerging technologies and the impact of AI in CI/CD processes is crucial for React developers. As the industry evolves, embracing these trends will lead to more efficient, secure, and maintainable software development practices.
Finale
In the realm of software development, particularly in React projects, understanding CI/CD pipelines is essential. The conclusion of this article encapsulates the core ideas and provides insights into the importance of these practices. Implementing a CI/CD pipeline enhances the development process significantly. It fosters agility and encourages faster feedback cycles. The ability to automate testing and deployment processes translates to improved reliability and efficiency.
Summary of Key Points
- Continuous Integration streamlines the building and testing of changes, ensuring that errors are detected early.
- Continuous Deployment enables seamless updates without significant downtime, improving user experience.
- Utilizing various tools like GitHub Actions and Docker facilitates the automation of workflow.
- Adhering to best practices ensures that the CI/CD pipeline remains efficient and maintainable.
- Recognizing common challenges such as integration issues and dependency management is vital for successful implementation.
These elements collectively underline the advantages of CI/CD in improving the React development lifecycle. Successfully integrating these practices can lead to higher code quality and more robust applications.
Final Thoughts on React /
Embracing CI/CD in React development is not just a trend; it is becoming a standard. The adoption of these practices provides developers with a structured approach to manage their projects effectively. Moreover, as technologies evolve, the landscape of CI/CD will also transform, with artificial intelligence playing a more prominent role in optimization and automation.
The necessity of being adaptive is clear. Developers should stay updated on emerging tools and methodologies to maximize the benefits of CI/CD. Thus, by focusing on effective integration and maintenance of CI/CD pipelines, organizations can deliver high-quality software that meets their users' needs promptly and efficiently.
"A well-implemented CI/CD pipeline doesn’t just bring value in terms of speed; it enhances quality and reduces friction in deploying software."
In summary, the continued exploration and improvement of CI/CD practices will lead to a more efficient, responsive development process in the React ecosystem.