Mastering React Redux: A Complete Guide for 2021
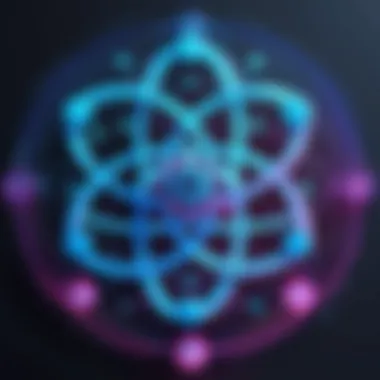
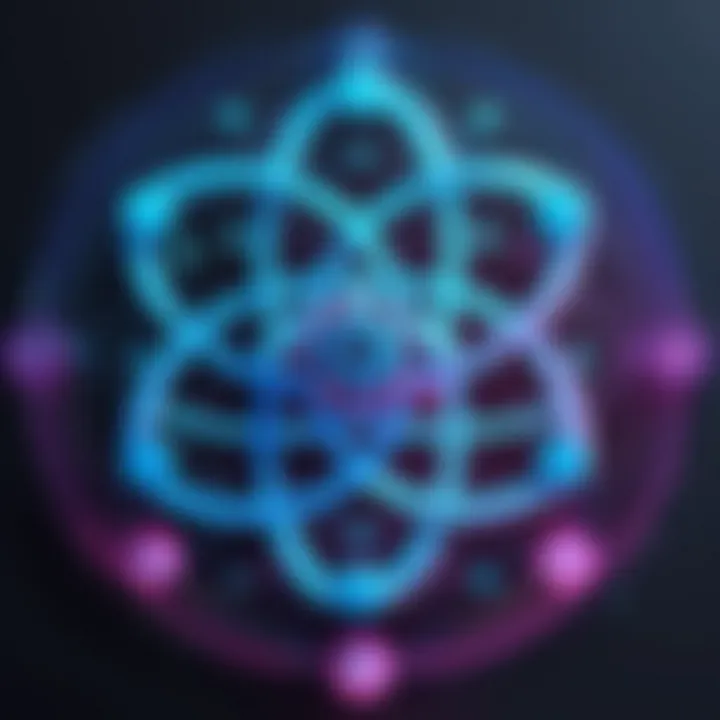
Overview of Topic
React Redux has become a cornerstone in the JavaScript development community, particularly for those working with modern web applications. At its core, React Redux simplifies the way state is managed in applications built with React, allowing developers to maintain a clear separation of concerns between UI components and the application's data.
The significance of employing modern state management techniques can't be emphasized enough. As applications grow in complexity, managing state effectively becomes a challenge. Redux offers a predictable state container, making it easier to manage interactions across different parts of an app.
Historically, Redux emerged from the desire to address the shortcomings in managing state in React. Initially gaining traction around 2015, it has evolved significantly since its inception. The rise of functional programming concepts within JavaScript has propelled its adoption, with functional components and hooks being amongst the many advancements.
Fundamentals Explained
Understanding the foundation of React Redux is essential. The core principles revolve around the idea of a single source of truth—a central store that holds the entire state of your application. Here's a brief outline of key terminology:
- Store: The single object that contains the state.
- Actions: These are plain JavaScript objects that represent an intention to change the state.
- Reducers: Functions that specify how the state changes in response to actions.
By grasping these basic concepts, developers put themselves in a strong position to handle more complex situations that arise in state management.
Practical Applications and Examples
Let's look through the lens of practical applications. Imagine an e-commerce site. Items in a shopping cart, user authentication, and product listings—all are examples of state that needs to be managed. By employing Redux, developers can ensure that changes in any one part of the application update the necessary components without a hitch.
For instance, if a user adds a product to their shopping basket, that action triggers an action that Redux can intercept, adjust the state accordingly, and then propagate those changes to the React components that display the cart.
Here’s a simplified code snippet to illustrate how an action might look:
This action could be dispatched to the store, signaling that the cart's state should update. This design keeps everything neat and organized.
Advanced Topics and Latest Trends
As we venture into more advanced terrain, several trends emerge. The integration of middleware is one such advancement. Tools like Redux Thunk or Redux Saga help manage complex asynchronous logic in your applications. They serve as a bridge between action creators and the moment actions get dispatched, making it easier to handle side effects like API calls.
Looking ahead, React and Redux continue to adapt. The rising popularity of hooks is not to be ignored. Hooks can complement Redux, offering a new way to access the state and actions within functional components, yielding a more streamlined coding paradigm.
Tips and Resources for Further Learning
For those eager to extend their knowledge, various resources stand ready to assist. A few recommendations could include:
- Books: "Learning React" by Alex Banks and Eve Porcello
- Courses: FreeCodeCamp and Codecademy offer interactive lessons.
- Online Forums: Reddit's r/reactjs and Stack Overflow discussions can dive deep, and community insights go a long way.
Additionally, tools like Redux DevTools can provide invaluable insights and debugging capabilities for applications implemented with Redux.
In summary, React Redux isn’t just a framework but rather a comprehensive solution for state management in dynamic JavaScript applications. As developers dig deeper, a world of possibilities unfolds before them. By keeping pace with the advanced topics and resources available, one can harness the full potential of Redux to create highly interactive user experiences.
Prologue to React Redux
In the rapidly evolving world of web development, understanding the interplay between React and Redux has become paramount. This section lays the groundwork for grasping how these two technologies can work in harmony to create dynamic web applications. Essentially, React is all about building user interfaces, enabling developers to create reusable UI components. But as applications scale up, managing state becomes a headache. That’s where Redux comes into play, providing a central store for a predictable state container.
Understanding the Basics of React
Let’s kick things off by talking about React. At its core, React simplifies the development of user interfaces by breaking down complex UIs into smaller, manageable components. Imagine a well-stocked toolbox; each tool (or component) serves a distinct purpose, allowing a more organized approach to development.
React operates on a declarative paradigm, which means developers describe what the UI should look like, and React handles the rendering. This approach not only speeds up the process but also makes debugging a lot easier. When a component's state changes, React re-renders only the part of the UI that needs updating.
When you’re deep into component hierarchies, though, sharing data between them can be quite the puzzle. That’s where Redux steps in to make life easier.
What is Redux?
Now, let’s dig into Redux. Simply put, Redux is a state management tool designed to keep your application’s state in sync. It allows for a single source of truth—the store—where the entire state of your application resides. It’s like the grand central station of your data: it receives, manages, and dispatches any action related to application state.
Redux follows three core principles:
- Single Source of Truth: The state of your application is stored in a single object. This makes it easy to track changes over time and helps in debugging.
- State is Read-Only: The only way to change the state is to emit an action, which ensures that state changes are traceable and predictable.
- Changes are Made with Pure Functions: To specify how the state updates in response to an action, Redux uses reducers—functions that take the previous state and an action and return the next state.
By adhering to these principles, Redux provides a robust infrastructure that enhances the reliability of complex applications.
The Importance of State Management
State management might sound dry, but it's one of the most critical aspects of modern web applications. If you think about it, every interactive app needs a way to handle user input, API responses, and UI states. Without effective state management, you end up with tangled code that’s harder to maintain and debug.
Using Redux empowers developers to:
- Maintain Predictability: When actions are dispatched, the flow of data is clear, and debugging becomes straightforward.
- Improve Scalability: As applications grow, managing state through Redux’s structured framework makes adding new features or altering existing ones far easier.
- Enhance Performance: React's virtual DOM works seamlessly with Redux, ensuring that only the necessary parts of the UI are updated, improving the app's performance.
“The beauty of a well-structured state management solution is that it can cut down on chaos and confusion, making developing a large application an almost manageable task.”
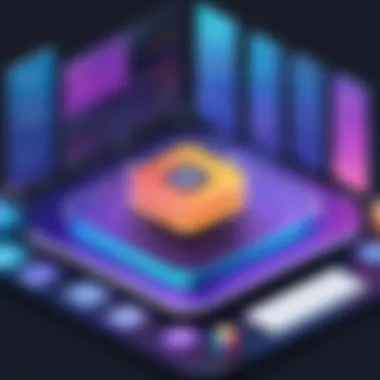
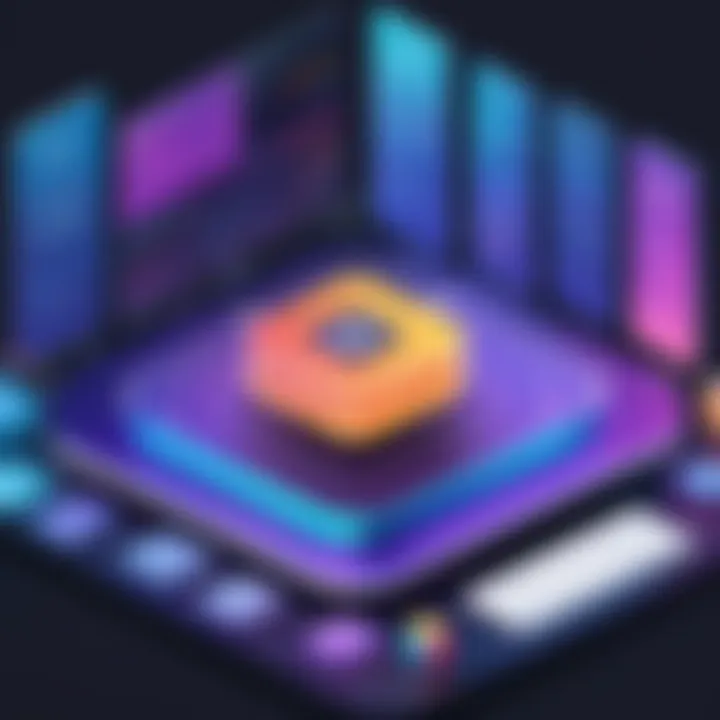
Setting Up Your Development Environment
Setting up your development environment is a crucial component when embarking on a journey with React Redux. This stage is not merely about installing software; it lays the groundwork for a seamless development experience. A well-configured environment allows developers to leverage tools efficiently, which can significantly boost productivity and minimize roadblocks. In this section, we’ll delve into the fundamental steps, tools, and considerations to ensure that you are equipped to integrate React with Redux effectively.
Prerequisites for React Redux
Before jumping into the deep end, a few prerequisites can smoothen the path ahead. First and foremost, having a basic understanding of JavaScript is essential. No one expects you to know it inside out, but familiarity with ES6 features, such as arrow functions and destructuring, will go a long way. Next, a grasp of React concepts can be quite handy. If you're not already comfortable with components, props, and state, consider brushing up on them. These existing skills will form the backbone of your Redux integration.
Installing Node.js and NPM
To start, you’ll need Node.js and NPM (Node Package Manager). Node.js allows you to run JavaScript on the server side, and NPM is a package manager that simplifies the installation of libraries and tools.
- Download Node.js: Head over to nodejs.org and download the latest version compatible with your operating system. The installation process is straightforward, just follow the prompted instructions.
- Verify Install: Once installed, open your terminal or command prompt and run:This will display the versions of Node.js and NPM, confirming that you’ve got everything set up correctly.
Creating a New React Application
With Node.js and NPM in place, the next step is creating your new React application. The easiest way to do this is by using , a command-line tool that sets up a new React project with all the boilerplate for you. Here’s how:
- Open the Terminal: Navigate to the directory where you wish your application to reside.
- Run the Command: Type the following command:Replace with your desired project name. This command automatically pulls the necessary packages and sets up your project’s structure.
- Navigate into Your App: Once installed, change into your newly created project directory:
- Start the Development Server: You can test it out by starting the local development server:This should open your browser with a fresh React application running.
Having set up your development environment, you are now better equipped to dive deeper into integrating and implementing Redux within your new React application.
"The right tools can make all the difference between a dream and reality in software development."
In summary, taking the time to set up a robust development environment creates a solid foundation for learning and using React Redux effectively. Do not skip this step; it pays dividends in terms of efficiency and ease of learning.
Integrating Redux with React
Integrating Redux with React is pivotal for building applications that require efficient state management. As applications grow in complexity, managing the global state becomes crucial. Redux provides a structured way to maintain the application state while offering components a clear pathway to access or update that state. This partnership allows developers to reduce bugs and improve code maintainability. Furthermore, it enhances the user experience by ensuring that the UI remains in sync with the application’s state.
Installing Redux and React-Redux
To kick off, installing the necessary packages is your first step toward integrating Redux with React. You’ll need both Redux and its official React bindings, React-Redux. Here's how to do that:
- Open your terminal or command prompt.
- Navigate to your React project directory.
- Use NPM to install Redux and React-Redux with the following command:
These packages are essential for creating a Redux store and connecting it to your React components. Once installed, you are well on your way to harnessing the power of Redux in your React application.
Creating a Redux Store
Now that you have Redux set up, the next logical step is creating a Redux store. A store holds the state of your application, primarily composed of a JavaScript object. This store can then be shared across your React components. To create a store, you can follow these steps:
- First, import the function from Redux in your code:
- You will also need to create a reducer, which is a function that determines how the state changes in response to actions. For simplicity, you'll have something like this:
- With the reducer in place, you can now create the store:
By following these steps, you’ve built a Redux store that holds your application’s state.
Connecting Components to the Redux Store
What’s important now is connecting your React components to this store. The component from React-Redux comes into play here. It makes the Redux store available to any nested components that need to access the Redux store. Here’s how to do it:
- Import the from React-Redux:
- Wrap your application with the and pass in your store:
- In , you can now use the function to interact with the Redux store. Import the function:
- Then, define your mapStateToProps and mapDispatchToProps functions, and connect them to your component:
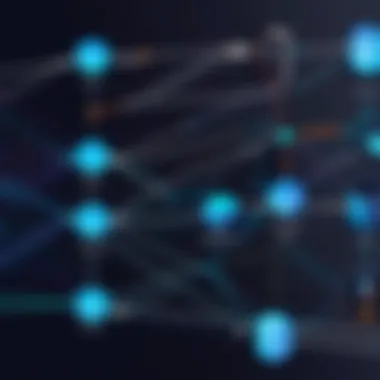
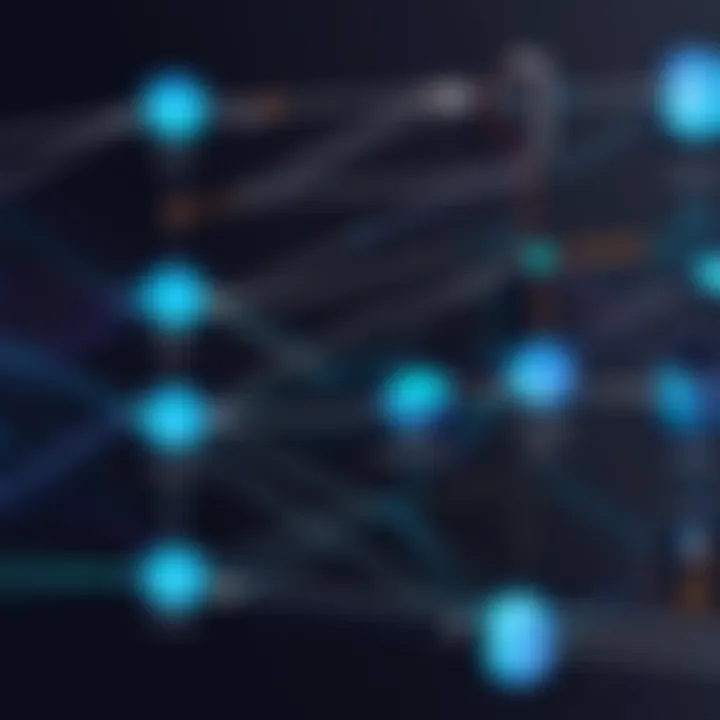
By completing these steps, you've linked your component to the Redux store, allowing it to read from the state and dispatch actions to update it.
Using Redux with React can feel complex initially but trust the process. Well structured state management makes everything easier down the road!
For further reading about Redux and its benefits, check out Wikipedia or Britannica.
Stay informed, and you’ll find that as your Redux skills sharpen, your productivity and code quality will soar.
Core Concepts of Redux
Understanding the core concepts of Redux is not just an academic exercise; it’s fundamental for realizing the full power of state management in React applications. Redux offers a predictable state container that adheres to principles like single source of truth, immutability, and unidirectional data flow. Each concept plays a vital role in achieving a robust architecture for your applications.
Actions and Action Creators
At the heart of any Redux application lies actions. An action in Redux is simply a plain JavaScript object that describes a change in state. It must have a property, typically defined as a constant. This identifier helps to inform the reducers about what kind of update they are expected to handle. Action creators, on the other hand, are functions that create these actions. They allow developers to encapsulate the action logic and streamline the process of dispatching actions to the store.
For instance:
Creating separate action creators for different actions keeps your code organized and enhances maintainability.
Reducers and Their Role
Once an action is dispatched, the next component in the Redux flow is the reducer. Reducers are functions that take the current state and the action as arguments and return the next state. The nature of reducers—being pure functions—ensures that state changes don't happen directly but rather through defined pathways.
For example, a simple todo reducer might look like this:
Here, the reducer listens for the action and appends a new todo to the list. This method of organizing state changes keeps things predictbale and easier to debug
Understanding Middleware
Middleware in Redux acts as a bridge between the dispatching of an action and the moment it reaches the reducer. It's particularly useful for logging actions, performing asynchronous operations, and handling side effects within your application. Middleware can significantly enhance your application's capabilities.
A popular choice for middleware is Redux Thunk, which allows action creators to return a funtion instead of an action. This makes it possible to create actions that perform async calls, like fetching data from an API:
Important: Middleware can modify the behavior of the dispatching process, thus giving you added control over how actions are processed.
Through these core concepts, Redux creates a structure that maximizes not only the management of state but also the potential for scalability and efficiency in your React applications. Each element—actions, reducers, and middleware—works in tandem to guarantee a smooth flow of data, which is crucial for any engaging user experience.
Best Practices for Using React Redux
When working with React Redux, adhering to best practices is not just a suggestion; it’s essential. This foundational framework has a robust architecture that demands thoughtful consideration in its application. Implementing effective strategies can drastically enhance the maintainability and scalability of your applications, allowing for smoother development experiences.
Organizing Redux Code Structure
A well-organized code structure is the backbone of any project built with Redux. If your code resembles a tangled mess, you’re in for a world of hurt when debugging and expanding features. Here are some key points to consider when structuring your Redux code:
- Directory Layout: It’s wise to keep your files tidy. Some developers follow a feature-based structure, where files relevant to a particular feature reside together. Others prefer a type-based structure, where actions, reducers, and components are grouped by type. Both styles have their merits. Choose one that aligns with your project’s size and complexity.
- Module Independence: Aim to keep your modules decoupled. Each action and reducer should focus on a specific concern, making it easier to manage and reason about changes.
- Consistent Naming Conventions: Stick to clear and consistent naming conventions. A general guideline is to name actions as verbs and reducers as nouns, which makes it easy to navigate your code.
In essence, tidy code and a clear structure lead to efficient development and lower cognitive overhead, keeping your sanity intact!
Avoiding Common Pitfalls
When setting out on your Redux journey, there are plenty of traps that can ensnare the unwary. Here’s a brief rundown of common pitfalls to steer clear from:
- State Mutability: Never mutate the state directly. Instead, always return new objects from reducers. Remember, Redux thrives on immutable state management.
- Overusing Redux: Not every piece of data needs to go into Redux. Local component state can sometimes be simpler and more effective, especially for data that doesn’t require global visibility.
- Ignoring Performance: If your application harbors a vast number of components subscribing to the same state, performances can take a nosedive. Use tools like and to mitigate unnecessary renders.
By recognizing these pitfalls early on, developers can launch their applications without facing avoidable headaches down the road.
Testing Redux Applications
Testing Redux applications is crucial in ensuring that your application functions as intended without any hiccups. Here’s how to go about it:
- Unit Tests for Reducers: Start with reducers, which are pure functions. This makes them easier to test. Input your state and actions, and expect a documented output.
- Action Testing: Similarly, test your actions. They should create the expected action objects. Use tools like Jest or Mocha to automate these tests.
- Integration Testing: Lastly, test how your components interact with the Redux store. Libraries like Enzyme or React Testing Library will help you simulate user events and assert outcomes.
Testing gives you confidence that your application will perform in line with your expectations, reducing the chaos in a production environment.
By adhering to these principles, you can use Redux effectively, allowing for a stronger foundation in your React applications. As you grow in your understanding, these best practices will form the cornerstones of your development toolkit.
Advanced Redux Patterns
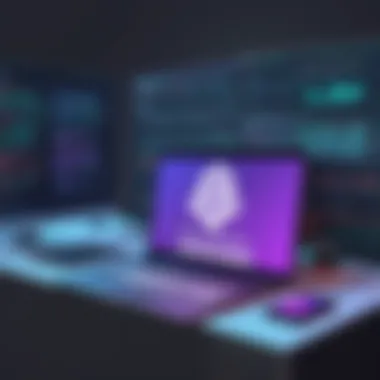
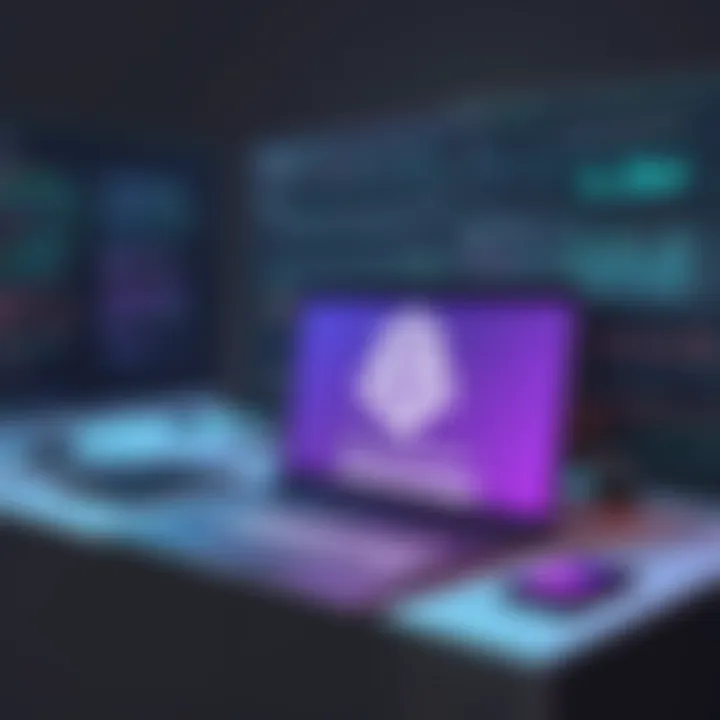
Understanding the landscape of Advanced Redux Patterns is crucial for developers seeking to maximize the capabilities of Redux in their applications. These patterns not only simplify complex state management tasks but also enhance the overall maintainability and scalability of your code. Remember, just like a well-tended garden, a clean and organized state management system can yield fruitful results in the long run.
Redux Thunk and Async Actions
When it comes to handling asynchronous actions in your Redux store, Redux Thunk is a game changer. Essentially, Thunk is a middleware that allows you to write action creators that return a function instead of an action. This can be particularly powerful when you need to perform side effects, such as fetching data from an API. For example:
Here, the function can dispatch multiple actions throughout its lifecycle, meaning you can manage loading states as well as errors—keeping your UI in sync with your data fetching process. This pattern helps keep your components cleaner and focused solely on rendering logic.
Using Redux Toolkit
With the introduction of Redux Toolkit, Redux has become more approachable and less cumbersome. It provides a set of tools that streamline the configuration and management of your store. The Toolkit encompasses utilities like , which allows you to create reducers and actions in a more concise manner. Here’s a quick example:
By simplifying the syntax needed to define reducers and actions, Redux Toolkit helps prevent boilerplate code, making your Redux code cleaner and easier to understand.
Integrating with React Router
One major consideration when developing React applications is how to manage routing alongside your Redux store. Integrating React Router with Redux may seem daunting, but it’s quite manageable. By keeping your routing state within Redux, you enable a single source of truth for your application's state.
For instance, you could set up an action to navigate to a new route:
In this snippet, both the Redux store and the browser history are updated, ensuring coherent application behavior. It keeps the logic centralized, avoids scattergun approaches, and enables cleaner testing.
"With great power comes great responsibility." The power brought by Advanced Redux Patterns requires responsibility in implementation. Use them wisely to prevent overcomplicated code.
By diving into these advanced patterns, you position yourself not only to master Redux but to create applications that are robust, scalable, and efficient. As with any tool, the true mastery comes with practice and understanding of when and how to apply these principles.
Debugging and Profiling Redux Applications
Debugging and profiling are often overshadowed by the excitement of building applications, yet they hold a key position in software development, particularly in the realm of Redux. When issues arise in applications, having a robust debugging strategy empowers developers to pinpoint problems swiftly, improving both productivity and application reliability.
Redux applications can become complex, especially as more features and state management intricacies are introduced. This complexity makes it all too easy for bugs to slip through the cracks. Thus, understanding how to effectively debug and profile Redux applications is not just beneficial, it's essential. Not only does it enhance the development process, but it also leads to a more seamless user experience.
Utilizing Redux DevTools
One of the most invaluable tools for Redux developers is Redux DevTools. This browser extension provides a powerful suite for observing how actions affect the state of your application in real-time. Setting it up is quite straightforward. Once integrated, Redux DevTools offers the following benefits:
- Time-travel debugging: This feature allows you to go back and forth in your application’s state. If you make an error or want to see how your state evolves, you can jump through different states without needing to refresh the entire application.
- State monitoring: Developers can inspect the current state and dispatched actions, making it easier to understand the flow of data.
- Action logging: Each action that is sent to the Redux store can be logged, giving insights into what changes occurred when.
To get started with Redux DevTools, you can initialize it in your store configuration like so:
Once set up, you can view interactions and behaviors easily, enabling a better understanding of both your application and Redux’s inner workings.
Common Debugging Techniques
Even with tools at your disposal, good old-fashioned debugging practices can’t be overlooked. Here are some common techniques that can enhance your debugging workflow:
- Console logging: Don’t underestimate the power of logging. Insert statements strategically throughout your code to track the flow of data and identify where things might be going awry.
- Breakpoints: Set breakpoints in your code to pause execution at certain points. This lets you inspect the variables and state at specific times, which is invaluable for understanding context.
- Redux middleware: Implementing middleware like Redux Logger helps provide a clear overview of dispatched actions and the resulting state changes. This insight can make it easier to spot unexpected updates.
Though these techniques are straightforward, their impact can be substantial. Keep in mind that debugging is a skill that improves with practice. The more you engage with these techniques, the more intuitive they become, ultimately smoothing out the development process.
Debugging is not just about fixing bugs. It's about understanding your application and improving it.
Understanding the art and science of debugging in Redux can significantly affect your development experience and outcomes. Each of these tools and techniques shines a light into the often murky waters of application behavior, ensuring that you're not just building applications, but also creating scalable, maintainable code that stands the test of time.
Culmination and Further Resources
Understanding React Redux is not merely about knowing how to set it up and getting the code to work. Instead, it embodies a broader understanding of state management pertinent to React applications and user experiences. This article has laid out a roadmap, guiding developers through the complexities and intricacies of React Redux. While practical implementation is crucial, it’s the underlying principles that drive effective applications.
As we reflect on the essential elements covered, embracing these core concepts enables developers to harness the true potential of React and Redux in tandem. Knowing the structure of actions, reducers, and middleware is pivotal, but it's essential to grasp how they interconnect, enriching user interfaces with seamless state transitions. This linkage not only aids in building performant applications but also prepares developers for scaling as demands on their projects grow.
Further, best practices discussed earlier serve as a scaffolding, supporting developers in organizing code efficiently, thus mitigating errors and enhancing maintainability. Taking time to focus on debugging and profiling ensures that the application is optimized and responsive, providing an improved experience for the end user. Therefore, moving beyond just implementation and focusing on these practices helps developers remain adaptable in a field that fast-evolves.
Recap of Key Concepts
- State Management: The control of how data flows through an application is at the heart of what Redux offers. Understanding how to manage global state effectively can pave the way for a more robust application.
- Actions and Reducers: Actions are the payloads of information that send data from your application to your Redux store. Reducers specify how the application's state changes in response to actions.
- Middleware: Middleware in Redux acts like a bridge between dispatching an action and the moment it reaches the reducer. Middlewares, like Redux Thunk, enable asynchronous actions.
By synthesizing these concepts into your development workflow, you not only strengthen your coding capabilities but also create applications that are modular, testable, and maintainable.
Recommended Additional Readings
- Getting Started with Redux - A guide that explains all the fundamental aspects of Redux, perfect for those looking to dive deeper.
- React Redux Documentation - It provides comprehensive information on how to integrate Redux seamlessly with your React applications.
- Redux Toolkit - Simplifying Redux development with good practices.
- Understanding Redux Thunk - A deep dive into managing asynchronous actions in Redux, enhancing your skills further.
These resources equip developers with the knowledge and skills necessary for mastering React Redux. As technology advances, committing to continued learning in this domain will ensure you remain at the cutting edge, adeptly navigating challenges that come your way.