Mastering Selenium with Python for Web Automation
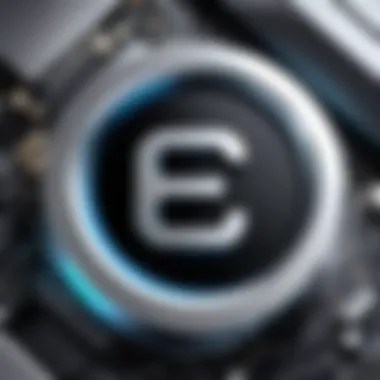
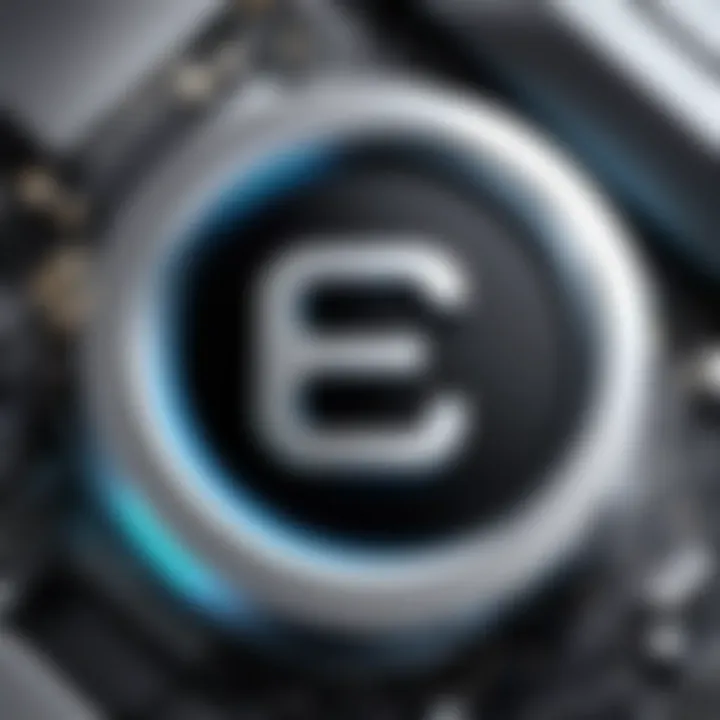
Overview of Topic
Preamble to the main concept covered
Selenium is a powerful tool for automating web applications. It allows users to control a browser programmatically, enabling various tasks such as automated testing or web scraping. When combined with Python, Selenium becomes even more efficient due to Python's simplicity and versatility. This guide seeks to provide an all-encompassing resource for understanding the integration of Selenium and Python, focusing on practical applications and usage.
Scope and significance in the tech industry
The significance of automation in today's tech landscape cannot be understated. Developers and testers consistently need tools to improve workflow efficiency. Selenium, being open-source and adaptable, has gained a strong following. Python's popularity in data science, web development, and automation further solidifies the relevance of this combination. Many organizations depend on automated solutions for their daily operations.
Brief history and evolution
Selenium originated in 2004, created by Jason Huggins initially to automate web applications for testing purposes. Since then, it has undergone substantial development, evolving into a suite of tools. This includes Selenium WebDriver, which provides a programming interface for various browsers. Python, introduced in 1991, has also grown from a simple programming language to a dominant force in various fields, making its synergy with Selenium a natural progression.
Fundamentals Explained
Core principles and theories related to the topic
At its core, Selenium operates on a few fundamental principles. It interacts with web elements through a browser, mimicking user actions such as clicking, typing, or scrolling. Automation scripts written in Python can encapsulate these actions, making testing or data extraction efficient. Understanding the application of these principles is essential for anyone delving into web automation.
Key terminology and definitions
To navigate Selenium effectively, certain terms should be clear:
- WebDriver: The component that drives the browser to perform actions.
- Locator: A method to find HTML elements on a web page (e.g., ID, XPath).
- Element: A single interactive component on a webpage.
Basic concepts and foundational knowledge
For someone new to Selenium and Python, grasping the basic setup is critical. You will need to install the Selenium module using pip. Additionally, ensuring you have a compatible web browser and its corresponding WebDriver is essential for successful automation.
Practical Applications and Examples
Real-world case studies and applications
Selenium finds application in several domains. For instance, online retailers often use it for automated testing of their applications, ensuring user experience remains consistent. Similarly, researchers utilize Selenium for web scraping to gather data from websites efficiently.
Demonstrations and hands-on projects
A simple demonstration would involve creating a Python script to automate logging into a web application. You can use a code block like this:
Code snippets and implementation guidelines
When implementing Selenium, it’s crucial to follow best practices for writing clear and maintainable code. This includes using descriptive names for functions and adhering to coding standards within Python. Additionally, implementing error handling will ensure your automation can recover from unexpected issues.
Advanced Topics and Latest Trends
Cutting-edge developments in the field
As technology advances, so does Selenium. Newer versions provide enhanced support for modern web applications, with features like better integration with headless browsers. This can lead to faster execution times, a crucial benefit for large-scale applications.
Advanced techniques and methodologies
In addition to basic automation, advanced users can leverage Selenium with frameworks like TestNG or pytest for robust testing solutions. Users can also integrate Selenium with continuous integration tools, like Jenkins, to streamline deployment processes.
Future prospects and upcoming trends
The future of Selenium appears bright. With ongoing development and enhancements, it is likely to remain a pillar in the automation community. Additionally, closely following trends in artificial intelligence may reveal new ways to incorporate Selenium with intelligent decision-making capabilities.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
To deepen your understanding, consider exploring comprehensive books like "Selenium with Python". Online courses, available on platforms like Coursera or Udemy, also offer valuable insights and practical projects.
Tools and software for practical usage
Beyond Python and Selenium, tools such as Jupyter Notebook can facilitate experimentation with code snippets. Additionally, browser extension tools for web development can aid in identifying elements to interact with Selenium.
"Understanding and mastering Selenium with Python is a stepping stone towards becoming proficient in web automation and testing."
By diving into the nuances of this combination, individuals can significantly improve their capabilities and streamline processes in various fields.
Prelude to Selenium
Selenium stands out as a vital tool in the realm of web automation. Its significance revolves around its ability to mimic human interactions with web browsers. It offers developers and testers a seamless method to automate web applications for diverse purposes, including testing, scraping, and monitoring. Understanding Selenium is essential for any programmer looking to enhance their automation skills using Python. The integration of Selenium with Python results in a powerful combination that maximizes the efficiency of web-driven tasks.
Overview of Web Automation
Web automation refers to the processes by which applications can automate repetitive tasks that users typically perform in a web browser. These tasks can involve filling out forms, clicking buttons, or even scraping data from websites. The importance of web automation lies in its capacity to eliminate mundane tasks, saving time and minimizing human error. Automation not only increases productivity but also ensures consistency across various operations.
- Benefits of Web Automation:
- Increases efficiency by performing actions faster than a human user.
- Reduces the risk of errors that can come from manual input.
- Valuable for tasks like regression testing, where repeated testing is essential for quality assurance.
- Helps in data extraction for analytics and reporting.
Selenium provides a robust framework to achieve these automation tasks effortlessly. With Python’s simplicity, user-friendly syntax, and extensive libraries, users can script complex automation scenarios with ease.
History and Evolution of Selenium
Selenium has evolved significantly since its inception in 2004. Initially created by Jason Huggins, its primary purpose was to aid in testing web applications. Over the years, Selenium matured and expanded its capabilities, adapting to the evolving web landscape.
- Key Milestones:
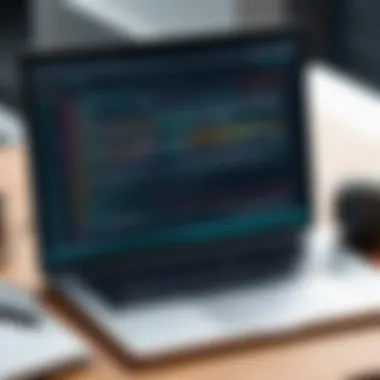
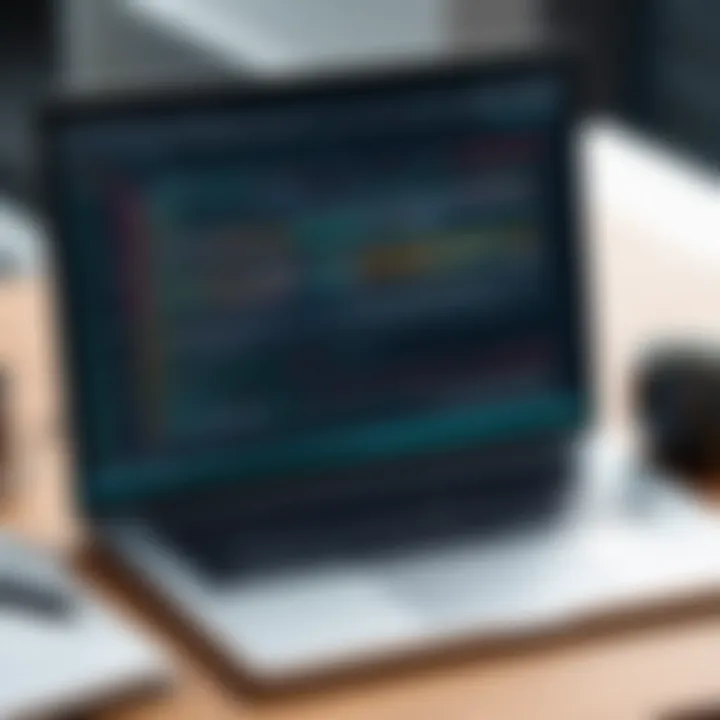
- 2004: The inception of Selenium as a JavaScript library.
- 2006: Launch of Selenium Remote Control (RC), enabling remote test execution.
- 2008: Introduction of Selenium WebDriver, which set a new standard for browser automation.
- 2011: Selenium became a part of the Software Testing Automation Framework (STAF), which enhanced its testing capabilities.
This evolution marks Selenium’s journey from a basic tool to a comprehensive framework that supports multiple programming languages, including Python, Java, and Ruby. The active community and regular updates ensure that Selenium stays relevant, addressing new challenges as they arise in web development and testing.
Getting Started with Selenium
Getting started with Selenium is crucial for anyone looking to automate web interactions. This section addresses essential facets of effectively utilizing Selenium with Python, ensuring a solid foundation. Understanding the requirements and installation process helps users streamline their automation tasks. Gathering this groundwork allows developers to focus on creating robust scripts rather than getting bogged down in initial setup challenges.
System Requirements
Before diving into the installation of Selenium, it's important to consider the system requirements. Ensuring your system meets these criteria will provide a smoother experience. Here are key elements to consider:
- Operating System Compatibility: Selenium is compatible with various operating systems, including Windows, macOS, and Linux.
- Browser Support: Make sure your browser version is supported by the Selenium WebDriver you intend to use. Common choices include Google Chrome, Mozilla Firefox, and Safari.
- Python Version: Selenium requires Python 3.6 or higher for compatibility with the latest features and security updates. Ensuring you are using an updated version of Python is vital.
Meeting these requirements will help avoid potential issues when running automation scripts.
Installation of Selenium
Installing Selenium is a straightforward process, especially when using Python's package manager, pip. The installation allows users to easily incorporate Selenium into their development environment. Here’s how to go about it:
Using pip
Using pip to install Selenium is popular due to its simplicity and efficiency. Pip automates the installation of packages, making it a convenient choice. The key characteristics that make pip appealing include:
- Rapid Installation: You can install Selenium with a simple command in a command line interface, greatly reducing setup time.
- Package Management: Pip manages dependencies effectively. If Selenium requires other packages, pip installs them automatically, simplifying the process significantly.
To install Selenium using pip, run the following command in your terminal:
Despite its advantages, there are considerations to note. Users must ensure that pip itself is updated, as an outdated version may lead to installation errors.
Setting up a Virtual Environment
Setting up a virtual environment is a best practice when working with Python projects, including those using Selenium. This approach isolates project-specific dependencies, preventing conflicts with other projects. A few notable points about using virtual environments include:
- Dependency Management: Each project can have its unique libraries without interference. This is crucial for maintaining different versions of packages.
- Simplified Collaboration: Developers can share their projects without worrying about discrepancies in libraries and tools used by team members.
To create a virtual environment, you can use the following commands:
While creating a virtual environment adds an extra step in setup, the benefits often outweigh this minor inconvenience. It fosters cleaner, more organized development practices.
In summary, establishing a clear understanding of system requirements and the installation process paves the way for effectively using Selenium with Python. By following the guidelines in this section, beginners can lay a strong groundwork for successful web automation projects.
Selenium WebDriver Basics
Selenium WebDriver serves as a fundamental backbone of automated web interactions. Its role in automating browsers is pivotal as this is where most automation scripts begin. Understanding WebDriver Basics is crucial because it sets the ground for how to effectively write automation scripts that achieve desired outcomes. The WebDriver interface allows testers and developers to communicate with web elements, run scripts, and validate user interface behavior. This interaction becomes paramount in both web scraping and automated testing, as it affords a level of control and precision.
Furthermore, familiarity with WebDriver Basics fosters a stronger grasp of how to navigate challenges associated with web automation. A well-structured approach in understanding this topic can significantly influence performance and reliability of automation tasks.
Understanding WebDriver Architecture
The architecture of Selenium WebDriver is comprised of several key components that interact with each other to facilitate browser automation. At its core, WebDriver establishes a communications link between your test scripts and the browser you intend to control.
- Browser Driver: Each web browser has a specific driver. For example, there are ChromeDriver for Google Chrome, GeckoDriver for Mozilla Firefox, and IEDriver for Internet Explorer. Each driver translates commands from your scripts into actions that the browser can execute.
- WebDriver Protocol: This serves as a bridge allowing the communication of commands and responses. It adheres to the W3C WebDriver specification, ensuring consistency across various browser platforms.
- Client Libraries: These libraries support various programming languages, including Python, enabling the development of test scripts. This means developers can utilize Selenium’s capabilities in a familiar programming context.
In essence, understanding this architecture is fundamental to resolving any issues that may arise and to optimizing the automation process.
Starting a WebDriver Session
Initiating a WebDriver session is a straightforward task but critical for the success of any automated testing or web scraping task. To start a session, you need to instantiate a WebDriver object that will control the browser.
To launch a browser, you can follow these basic steps:
- Import the necessary libraries: Ensure that you have imported Selenium's WebDriver module, as it contains the essential components.
- Create an instance of a browser: You instantiate a WebDriver corresponding to the desired browser.
driver = webdriver.Chrome()# for Chrome
driver = webdriver.Firefox()# for Firefox
Each of these steps is critical in establishing and executing scripts. The session will run until you explicitly close the browser using . A properly opened and terminated session will ensure resource management and session integrity.
Starting a WebDriver session is the first step in automating any task.Testing and web scraping requires precision and establishing a connection to the browser is foundational.
Interacting with Web Elements
Interacting with web elements is a crucial aspect of working with Selenium and Python. This involves not only identifying elements on a web page but also interacting with them through various actions. Such actions can include clicking buttons, entering text, or selecting options from dropdowns. Understanding how to effectively interact with these elements enhances the capability of automated scripts, allowing for more complex and useful automation tasks. Moreover, accurately locating and interacting with elements helps in achieving the objectives of web automation more efficiently.
Locating Elements
Locating elements on a web page is the foundation of interaction. Selenium provides several strategies that allow users to pinpoint specific elements and perform actions on them. Each method has its own characteristics and use cases, which makes understanding them vital for effective automation.
By
Locating elements by ID is one of the simplest methods in Selenium. The ID attribute in an HTML element is unique within a page. This uniqueness makes it an efficient way to identify elements. Using IDs often leads to faster performance as it allows for quicker lookups in the Document Object Model (DOM). However, while IDs are reliable, the challenge may arise when web pages do not appropriately use unique IDs. They are a popular choice for many due to the speed and simplicity they offer. In short, IDs provide a direct path to an element, making them beneficial.
By Name
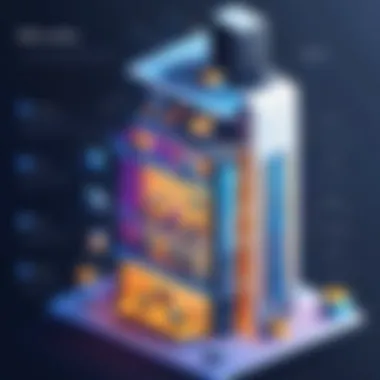
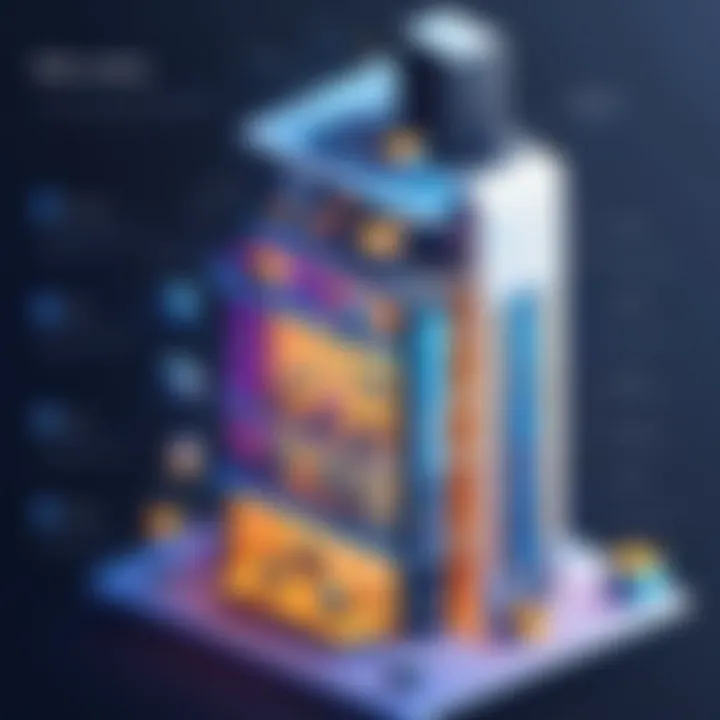
The name attribute is another way to locate elements. Many form elements, like input fields, have a name attribute. This method works well when multiple elements share the same class or ID but differ by other attributes. While useful, this method can sometimes lead to ambiguity if multiple elements share the same name. Thus, the effectiveness of the strategy hinges on the structure of the HTML document. Overall, using names can be a beneficial choice for sorting through form elements.
By XPath
XPath provides a powerful way to locate elements through their position in the HTML structure. This method allows navigation through the hierarchical structure of the document. It is highly flexible and can be used to identify elements based on attributes, text, or even relationships among elements. However, XPath can be slower than ID and Name locators. Additionally, constructing valid XPath expressions can be intricate, adding to the complexity. Yet, its flexibility makes it a valuable tool for more complicated locating scenarios.
By CSS Selector
CSS selectors offer another method for locating elements. They are based on the styling of the elements and provide a way to select elements based on their CSS classes, IDs, attributes, and more. CSS selectors can combine multiple selectors to create a single search expression, which makes it a powerful option. Its versatility allows for selecting elements in various ways. However, constructing complex CSS selectors might be challenging for less experienced users. While CSS selectors are very expressive, care must be taken to ensure accuracy in their use.
Performing Actions on Elements
Once elements are located, performing actions on them is the next step. This may involve clicking buttons, entering text, or selecting values from dropdown menus. Each action is vital to drive web applications through automation.
Clicking Buttons
Clicking buttons is a fundamental action when interacting with web elements. This action directly triggers functionalities within web applications, from submitting forms to opening new pages. Buttons on web pages are primarily input elements, making this action straightforward. However, there may be situations where buttons are non-standard or implemented using JavaScript, complicating the interaction. Despite this, understanding how to click buttons deliberately ensures a smooth flow in automation tasks.
Entering Text
Entering text into input fields is a common requirement in web automation. This may involve filling out forms or search bars. The process involves locating an input element and sending keys to it. The ability to automate text entry can save significant time and reduce human error. It is essential to ensure that the field is ready to accept input; otherwise, errors may occur. Overall, text entry can be seen as a beneficial action when it comes to simulating user behavior.
Selecting Dropdowns
Selecting options from dropdowns is another essential action in web interaction. Many web applications incorporate dropdowns for selections. The process involves locating the dropdown element and making the appropriate selection. The Selenium library provides straightforward tools for handling dropdowns. However, it’s crucial to confirm that the element is interactable at the time of selection. Managing dropdowns effectively can considerably enhance the automation workflow, allowing for seamless user experience simulation.
Selenium for Web Scraping
Web scraping is a crucial technique for data collection, allowing users to extract information from websites quickly and efficiently. Using Selenium with Python enhances this process by providing tools to automate interactions with web pages, which can be particularly useful when dealing with dynamic content that standard scraping libraries may struggle to handle. Selenium is capable of controlling a web browser, which enables it to mimic human actions. This capability makes it a valuable asset for web scraping tasks.
Understanding Web Scraping
Web scraping refers to the automatic collection of information from the internet. It is widely used in various sectors, including market research, price comparison, and data analysis. The fundamental concept relies on making HTTP requests to fetch web pages and parsing the content to extract the desired data. However, traditional scraping methods using libraries like Beautiful Soup may have limitations when it comes to handling JavaScript-generated content. This is where Selenium shines since it can interact with a fully rendered page, giving it an advantage over static scraping techniques. It allows users to not only scrape the text but also interact with web elements like buttons and forms.
Practical Web Scraping Example
A practical example of web scraping using Selenium involves setting up a script to extract data from an online store. This process typically begins with configuring the environment and writing the script to navigate and locate elements on the page.
Setting Up a Scraping Script
Setting up a scraping script is a fundamental step in automating web data extraction. The process involves defining the target website and identifying the specific elements to extract. One key characteristic of a scraping script is its ability to open the browser, navigate to a desired URL, and wait for the required elements to load. This ability to wait improves the efficiency and effectiveness of data collection.
Using Python with Selenium offers considerable flexibility for building such scripts. A simple setup can start from importing necessary libraries, like Selenium's WebDriver, and initializing browser settings. For instance:
This code snippet represents the basic structure to launch a browser and access a webpage. The advantages of such scripting include the ability to automate repetitive tasks and the flexibility to extract various data elements with minimal manual intervention. This is a beneficial approach for both novices and experienced programmers.
Handling JavaScript-Rendered Content
Handling JavaScript-rendered content is essential for scraping modern web applications where data is often loaded asynchronously. Regular scraping tools may return incomplete data if they try to access elements before they are fully rendered on the page. Selenium effectively overcomes this issue by waiting for specific elements to become available.
A unique feature of this approach allows automation of the browsing experience as if a user were interacting with the browser. As a result, it provides a comprehensive view of the webpage content even when JavaScript generates elements dynamically. One significant advantage is flexibility because as websites evolve, Selenium scripts can often be adjusted only slightly to accommodate new structures.
"Selenium is not just a tool for automated testing; its capabilities lend themselves well to web scraping, especially as web technologies advance."
Automated Testing with Selenium
Automated testing is a critical component in software development, especially in web applications. Using Selenium with Python offers powerful tools that simplify the testing process. The ability to automate browser actions not only saves time but also enhances the reliability of the testing process. Automated tests help to identify defects earlier in the development phase, ensure consistency across different testing environments, and allow for the repeated execution of tests with minimal effort.
Automated testing with Selenium addresses various challenges. One significant benefit is reducing human error in the testing process. When testing is automated, the tests can be executed the same way every time, leading to more trustworthy results. Additionally, test scripts can be run whenever necessary, providing immediate feedback on new code changes. This immediacy aids quick iterations and reduces downtime.
Another important aspect is scalability. Automated tests are much more scalable than manual tests. As applications grow, the number of functionalities can increase significantly. Automated tests can easily be adapted to cover new features, which is often a labor-intensive task when done manually. Such adaptability is crucial in today’s fast-paced development environments where updates are frequent.
Importance of Automated Testing
Automated testing plays a pivotal role in maintaining software quality. It allows teams to execute comprehensive tests across various scenarios that would otherwise be impossible or too time-consuming for manual testing. This plays a larger part in continuous integration and deployment processes, where changes are made frequently and need to be validated quickly.
Team collaboration is greatly enhanced through automated testing as well. Developers and QA engineers can create a seamless workflow where all parties are kept informed about test results. This transparency encourages collaboration and improves the overall quality of the product, as everyone can see how changes affect the application.
Writing and Running Tests
Writing automated tests with Selenium involves a structured approach to ensure efficiency and effectiveness. Leveraging frameworks plays a significant role in enhancing the testing process, as does the methodology behind creating test cases.
Using Test Frameworks
Integrating Selenium with test frameworks like pytest or unittest is a strategic way to manage and organize tests. Test frameworks streamline the process and encourage best practices in writing tests. They come with features like test discovery, fixture management, and reporting.
A key characteristic of these test frameworks is their ability to structure tests clearly, which enhances readability and maintainability. This organization becomes particularly beneficial for larger projects where multiple tests might exist. Additionally, these frameworks often provide built-in assertions, saving time and reducing errors.
The unique feature of test frameworks is their flexibility in executing tests in different ways. For instance, tests can be run in sequences or parallel, depending on what is most efficient. However, some drawbacks include the potential learning curve associated with each framework. New users may find it challenging to adapt initially, but the benefits often outweigh the disadvantages once familiarized.
Creating Test Cases
Creating test cases is an essential aspect of automated testing. A well-defined test case serves as a guide to ensure that the functionality being tested behaves as expected. Good test cases are easy to understand and maintain. They help in making sure tests cover all the necessary scenarios.
One key characteristic of creating test cases is that they should be specific, measurable, and traceable. This specificity allows testers to know exactly what they're validating, simplifying debugging when necessary. A beneficial approach to creating test cases is to start with a clear requirement or user story.
The unique feature of this approach lies in its systematic nature. Each test case can be linked back to specific requirements, aiding in comprehensive coverage. However, challenges may arise if new requirements emerge after the test cases have been defined. This can result in outdated test cases unless they are reviewed and revised regularly. Keeping the test cases updated is paramount to maintaining their effectiveness.
Handling Waits and Delays
In the realm of web automation with Selenium, the management of waits and delays proves to be critical. The dynamic nature of web applications can often lead to unforeseen circumstances where elements take time to load. Users may find themselves waiting for elements to appear or become interactive. When scripts execute too soon, it can result in errors or incomplete actions. This necessitates a deep understanding of how to implement waits effectively.
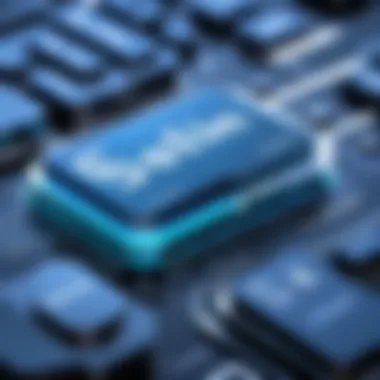
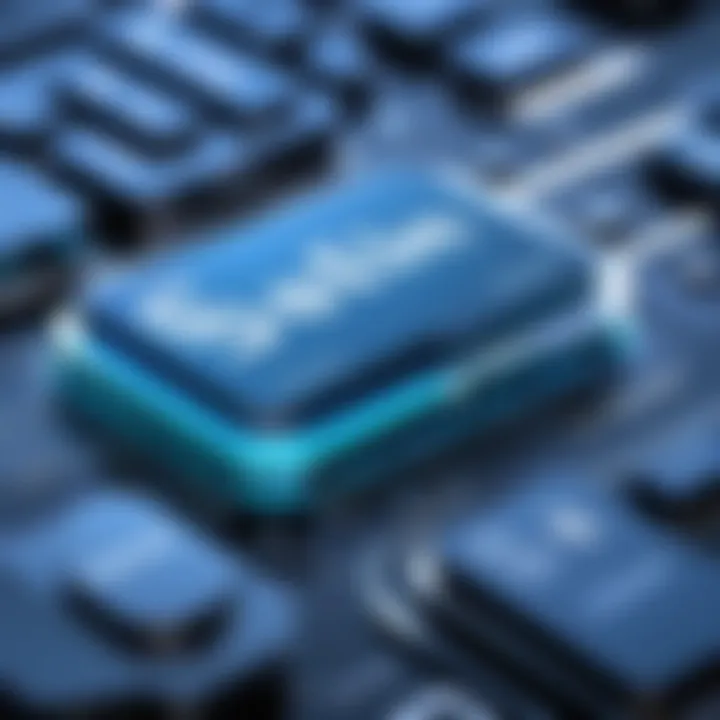
Implicit and Explicit Waits
Selenium offers two primary types of waits: implicit and explicit. Implicit waits set a universal time frame for the WebDriver to look for elements before throwing an error. This approach is straightforward and minimizes the potential for failures due to loading times. When using implicit waits, all elements will allow for the same amount of pause irrespective of their location or loading characteristics.
On the other hand, explicit waits provide a more granular control. This type allows the developer to define specific conditions to wait for a particular element to appear or be clickable. For example, one may specify that the WebDriver should wait until a specific button is clickable before proceeding. This is achieved using the class in conjunction with .
The choice between implicit and explicit waits often depends on the complexity of the web application and the specific needs of the automation task. Implicit waits can simplify the coding process in simpler setups, whereas explicit waits shine in more dynamic scenarios.
Best Practices for Implementing Waits
Implementing waits effectively enhances robust interaction with web elements. Here are best practices to consider:
- Avoid Mixing Waits: Stick to either implicit or explicit waits in a session to prevent unforeseen results. Mixing the two can lead to confusing issues where some waits may override others.
- Optimal Timing: Adjust your waiting periods according to the conditions of the target web application. A longer timeout may be appropriate for slower sites, while quicker response sites can use shorter waits.
- Use Explicit Waits Judiciously: Explicit waits offer flexibility, but excessive use can slow down overall test performance. Apply them only where necessary.
- Apply Wait Conditions Efficiently: Clearly define the conditions under which to wait. This may include waiting for visibility, clickability, or text presence within elements.
- Testing on Different Network Speeds: Evaluate the behavior of your waits under varying network conditions. This ensures that your waits are indeed effective across different scenarios.
"Effective waits can mean the difference between a reliable script and one riddled with errors."
By adhering to these practices, one can mitigate common issues related to web timing, paving the way for smoother interactions and more reliable test results.
Advanced Selenium Features
Advanced Selenium features are vital for developers and testers striving for a deeper mastery of web automation. These features enhance the capabilities of Selenium, enabling users to address specific challenges and integrate more complex interactions within their automation scripts. By understanding how to effectively utilize these advanced options, users can improve their testing and scraping processes, making them more robust and adaptable to varied scenarios.
Executing JavaScript with Selenium
Executing JavaScript with Selenium allows users to directly interact with the webpage's Document Object Model (DOM). This capability is crucial when engaging with dynamic content that traditional methods may not easily manipulate.
JavaScript can be executed via the method in Selenium. This method enables you to run any arbitrary JavaScript code in the context of the currently selected window or frame. For instance, if you want to fetch specific data or trigger events, this method becomes particularly useful. Here is how you might execute simple JavaScript:
This snippet demonstrates fetching the title of a webpage. The ability to manipulate JavaScript expands the functionality of Selenium significantly, allowing for direct control over elements and value retrieval when standard Selenium commands fall short.
Handling Alerts and Alerts Management
Alerts are common in web applications, often used to convey important information or gather input from users. Selenium provides features to handle these alerts effectively. Recognizing the types of alerts—simple alerts, confirmation dialogs, and prompt dialogs—is crucial for proper management.
To interact with an alert, users typically employ the feature. This allows users to gain access to the alert and perform actions such as accepting or dismissing it. Here’s how it can be done:
Alerts can interrupt the flow of your script, so ensure they are handled gracefully to avoid unexpected behavior in your tests.
By mastering these advanced features, users can ensure their applications are both flexible and responsive to user interactions, which is essential in today’s interactive web environment.
Challenges and Limitations
In web automation, understanding challenges and limitations is crucial. This knowledge helps users make informed decisions when utilizing Selenium in Python. Various issues may arise during implementation, affecting the accuracy and efficiency of automation tasks. Therefore, recognizing these elements allows for preemptive measures and effective solutions.
Common Challenges in Web Automation
- Dynamic Content: Many websites use AJAX and JavaScript to load content dynamically. This can make locating elements more complex. The code may run before the elements are fully loaded, leading to errors.
- Element Identification: Websites can alter their structure without warning. This can result in selectors becoming outdated. Ensuring elements are correctly identified is an ongoing challenge for developers.
- Browser Compatibility: Different browsers may behave inconsistently. While Selenium aims to standardize interactions, scripting may require additional adjustments for specific browsers. Testing across various platforms adds time to the development cycle.
- Rate Limiting and Captchas: Websites employ security measures to prevent automated access. Features like CAPTCHA can hinder automation efforts and require manual intervention. This can disrupt workflows and reduce efficiency. Acknowledge that overcoming these barriers often demands creative and technical solutions.
- Resource Heavy Operations: Running automated tests can consume significant system resources. Performance may degrade if too many instances run simultaneously. Managing resources efficiently is vital to maintain smooth operation.
"Understanding the challenges in web automation is the first step towards successful implementation."
Mitigating Limitations of Selenium
Several strategies can help in mitigating Selenium limitations. Adopting best practices is crucial for enhancing the stability and reliability of automation.
- Utilizing Waits: Implementing implicit and explicit waits can resolve issues related to dynamic elements. This practice allows scripts to pause until all necessary elements are present before proceeding.
- Robust Selectors: Avoid relying on fragile selectors. Instead, focus on using unique identifiers like IDs. If possible, use XPath or CSS selectors that are less likely to change.
- Cross-Browser Testing: Utilize tools like BrowserStack or Sauce Labs for automated cross-browser testing. This assists in identifying compatibility issues early in the development process.
- Handling CAPTCHA: Consider using third-party services or libraries that assist in solving CAPTCHA challenges when testing. These can streamline workflows without manual intervention.
- Optimizing Resource Use: Schedule automated tests during off-peak hours to leverage available resources better. You can also limit the number of concurrent sessions to distribute load efficiently.
By addressing challenges and employing mitigation strategies, users can harness the full potential of Selenium in Python. Adopting these practices ultimately leads to better results and improved productivity.
Best Practices for Using Selenium
In the realm of web automation, implementing best practices when using Selenium with Python is crucial. These practices not only enhance the performance and maintainability of the automation scripts but also ensure that users can efficiently debug and scale their projects. For those new to this framework or seasoned developers, adhering to these standards can save time and reduce frustration in the long run.
Optimizing Performance
Performance is a critical aspect of web automation. Slow scripts can hinder productivity and lead to frustration. Here are several strategies to optimize performance in Selenium:
- Minimize Page Load Times: Configure browser settings to disable images, and ads and use headless mode if visual output is not necessary. This helps in loading pages faster and reduces the overall run time.
- Use Implicit and Explicit Waits Wisely: While waiting for elements to load is essential, mismanagement can lead to time wastage. Implicit waits set a default wait time for all elements, while explicit waits can handle specific conditions. Use them appropriately to balance efficiency and reliability.
- Limit Browser Instances: Opening multiple browser instances simultaneously increases resource usage. Consider consolidating tests or using tools for parallel execution.
- Headless Browsing: Running tests in headless mode (without a graphical user interface) can significantly improve execution speed. This is especially useful in continuous integration environments.
Always profile and monitor script performance to identify bottlenecks. Tools such as Python's built-in module can help assess specific parts of your script.
Maintaining Code Quality
Maintaining code quality is essential for long-term project success. High-quality code is easier to read, debug, and enhance. Below are techniques to keep your Selenium scripts well-organized and efficient:
- Use Version Control: Implement Git to track changes, collaborate efficiently, and manage different versions of your code. This allows for easy rollback in case of errors.
- Follow Coding Conventions: Adhere to Python's PEP 8 style guide. Consistent formatting improves readability and makes it easier for others to understand your code.
- Write Modular Code: Break your scripts into modular functions. Each function should handle one specific aspect of the automation process, making your code reusable and easier to test.
- Documentation and Comments: Regularly document your code. Clear comments explaining your logic help others (and future you) understand the reasoning behind your decisions.
Implementing these practices will not only elevate the quality of your automation projects but also foster a robust coding environment. Following these principles ensures that you can adapt your codebase as requirements evolve without incurring technical debt.
"Good practices in programming are the cornerstone of any successful project. Without them, even the best tools can lead to chaos."
In summary, committing to best practices while using Selenium not only facilitates smoother automation but also strengthens the foundation for future development.
Culmination
In this article, we have explored the significant aspects of using Selenium with Python for web automation. The relevance of this topic extends beyond just basic understanding; it encapsulates a comprehensive suite of tools and techniques that can be harnessed by developers to create automated solutions.
The benefits of leveraging Selenium with Python are numerous. First, it provides robust capabilities for web scraping, allowing users to extract data efficiently from various websites. This is particularly valuable in industries where data collection is essential for decision making. Second, automated testing becomes streamlined, leading to increased productivity and accuracy. This automation not only saves time but also helps in maintaining high-quality standards in software development.
Considerations for implementing Selenium effectively include understanding its architecture and being aware of potential limitations. As discussed in previous sections, mastering waits and delays, handling alerts, and optimizing performance are crucial steps in successful integration.
"Automation can drastically improve the efficiency and reliability of processes."
Finally, it is essential to focus on best practices and maintaining code quality. This will ensure that the solutions developed are scalable and maintainable over time. Keeping abreast with updates in Selenium and Python will further enhance the potential of web automation efforts.
In sum, the journey of mastering Selenium with Python is not just an exercise in technical skill; it is a pathway towards innovation in automation that can yield substantial dividends in various fields.