Mastering SQL Subqueries: Structure and Applications
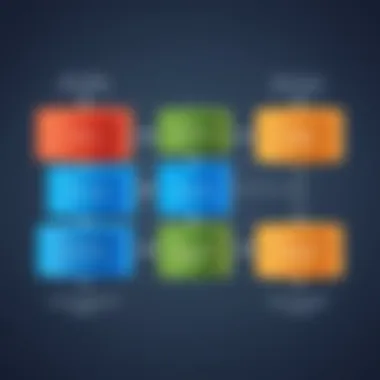
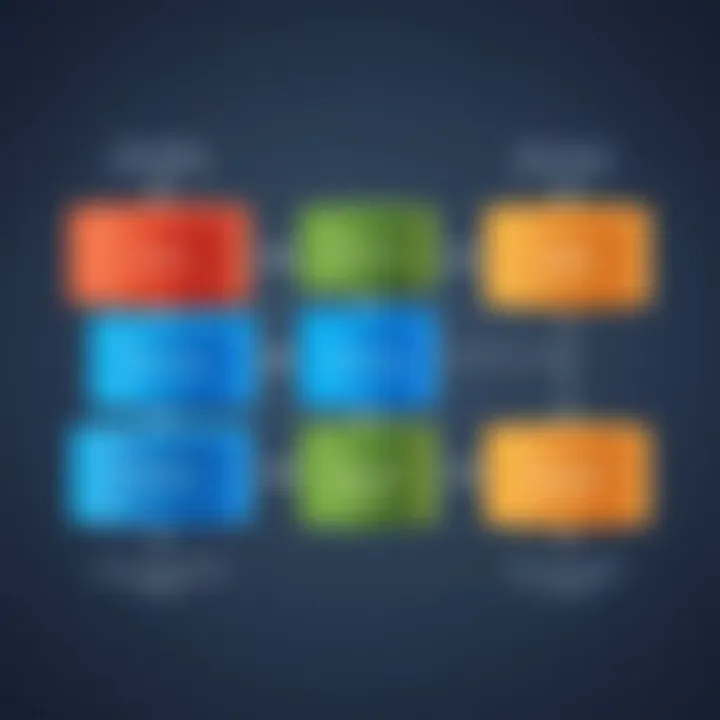
Overview of Topic
Preface to the Main Concept Covered
When it comes to SQL, understanding the power of subqueries is like knowing the secret handshake in a club. So, what's a subquery? In simplest terms, it's a query nested inside another query. This little gem allows you to break down complex data retrieval tasks into more manageable chunks, making it a cornerstone in advanced SQL programming.
Scope and Significance in the Tech Industry
The importance of mastering subqueries cannot be overstated. In a world overflowing with data, efficient data retrieval is paramount. Subqueries not only streamline processes but also enhance clarity in SQL statements. The ability to sort through vast troves of information swiftly places tech professionals a step ahead, whether they're diving into data analytics or developing sophisticated applications.
Brief History and Evolution
Subqueries have been around since the early days of SQL. Historically, they emerged to address the need for more flexible querying methods. Initially, developers crammed all their filters into a single statement, leading to unwieldy and hard-to-read code. As databases evolved, subqueries gave rise to more refined techniques, enabling cleaner code and increased productivity.
Fundamentals Explained
Core Principles and Theories Related to the Topic
At its heart, a subquery operates on the same principles as any SQL queryāit uses SELECT statements, often combined with WHERE clauses to filter data. The trick is in how these queries relate to one another. The outer query utilizes the results from the inner query, which can be executed separately.
Key Terminology and Definitions
Before diving deeper, let's clarify a few terms:
- Subquery: A SELECT statement nested within another query.
- Outer Query: The main query that encompasses the subquery.
- Inner Query: The query inside the outer query, often called a subquery.
Basic Concepts and Foundational Knowledge
Understanding the structure of subqueries forms the bedrock for their effective usage. They can be categorized as:
- Single-row subqueries: Returning a single value.
- Multiple-row subqueries: Returning multiple results, often used with operators like IN or EXISTS.
- Scalar subqueries: Which yield a single value and can be integrated directly into an expression.
Practical Applications and Examples
Real-World Case Studies and Applications
Imagine a scenario where you're managing an online bookstore. You might want to find all the books that have been ordered more than a certain number of times. A subquery here can simplify your search:
This query first identifies book IDs that frequently appear in orders and then retrieves the corresponding book titles.
Demonstrations and Hands-on Projects
To reinforce your understanding, consider implementing a small database project. Create two tables: one for courses and another for student enrollments. Write queries that use subqueries to find students who are enrolled in specific coursesāall done using various types of subqueries. This exercise drives home their utility.
Code Snippets and Implementation Guidelines
When using subqueries, remember that performance can take a hit. The more complex your subquery, the longer it might take to execute. Here's a tip: try to keep inner queries simple and focused. Always optimize for speed while maintaining readability.
Advanced Topics and Latest Trends
Cutting-edge Developments in the Field
As SQL continues to evolve, certain trends are shifting the landscape. For instance, with the rising prominence of NoSQL databases, there's an ongoing conversation about whether traditional subqueries will hold their ground or adapt to new paradigms.
Advanced Techniques and Methodologies
Advanced users often implement correlated subqueries, where the inner query references a value from the outer query. While powerful, they require careful management to avoid performance issues.
Future Prospects and Upcoming Trends
As database technologies grow, expect to see innovations that further optimize subquery processing. Techniques that enhance performance without sacrificing functionality will dominate, making the understanding of subqueries increasingly relevant.
Tips and Resources for Further Learning
Recommended Books, Courses, and Online Resources
- Books: "SQL Performance Explained" by Markus Winand - a detailed exploration of performance issues, including subqueries.
- Courses: Websites like Coursera and Udemy offer courses that tackle SQL including subquery techniques.
- Online Resources: Methods to explore concepts further include SQL Fiddle for hands-on practice, and sites like Reddit and Stack Overflow for community support.
Tools and Software for Practical Usage
For practical implementations, consider tools such as MySQL Workbench or PostgreSQL's pgAdmin. Utilize these platforms to write and test your SQL queries, sprinkling in subqueries as you go along.
Intro to SQL Subqueries
In the realm of database management, SQL subqueries are significant tools that empower users to execute complex queries with finesse. A subquery, simply put, is a query nested within another SQL statement. This nested structure allows for a more nuanced and refined approach to data retrieval, giving users the ability to filter more intelligently and aggregate with precision. Understanding how subqueries function is essential for anyone aiming to harness the full capabilities of SQL.
The importance of exploring SQL subqueries is underscored by the way they can simplify otherwise convoluted queries, promoting clarity and efficiency. For instance, when faced with a scenario that requires pulling data from multiple tables or performing calculations based on one dataset while referencing another, subqueries shine brightly. They enable SQL coders to encapsulate complex logic in a manageable format, making the overall code cleaner and easier to understand.
Beyond mere simplification, subqueries also enhance performance. When executed correctly, they can reduce the number of lines of code necessary, streamlining the process of data extraction. Yet, alongside their benefits, itās crucial to keep in mind certain considerations. Mismanagement of subqueries can lead to a performance lag, especially in large datasets, where each nested query can snowball into a larger problem.
By diving deep into the structure, types, and applications of subqueries, this article aims to equip students, budding programmers, and IT professionals with a holistic understanding. It sheds light on how to utilize SQL subqueries effectively, ensuring you can leverage them to boost your database querying skills.
"Understanding the role of subqueries is akin to holding a compass that points toward clarity in the vast wilderness of data."
Defining a Subquery
A subquery is essentially a query nested within another SQL query. This inner query is executed first, and its results are used by the outer query. In many cases, subqueries are encased in parentheses, allowing SQL to differentiate between the inner and outer operations. Understanding this definition is step one in grasping the greater context and application of these queries.
Subqueries can return a variety of data types, including single values, a list, or a complete table. The returned data can then be utilized for further operation or filtering in the main query. For example, if one needs to find all customers who have orders that exceed a certain amount, a subquery would first calculate that amount and then filter customers based on it.
The Role of Subqueries in SQL
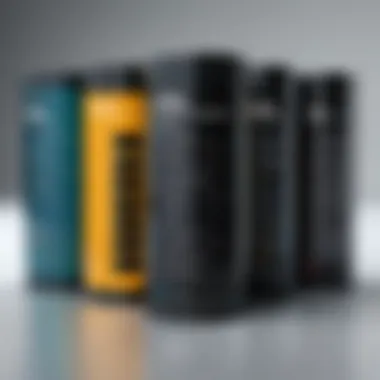
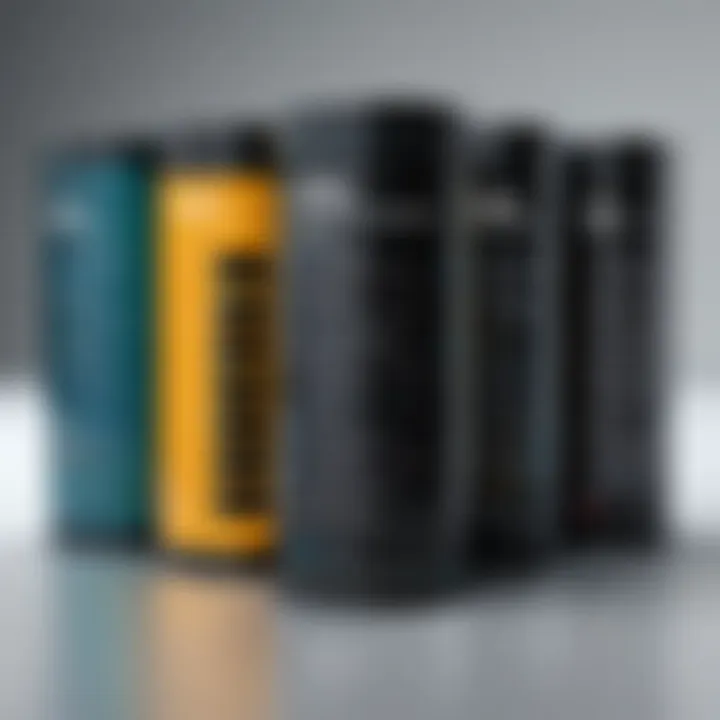
Subqueries play an essential role in the architecture of SQL statements, serving various functions across different segments of a query. Their versatility enables them to be used in WHERE clauses, FROM clauses, or even SELECT statementsāeach application serving a different purpose. For instance, when a subquery resides in the WHERE clause, it may help filter records based on conditions defined by the results of the subquery. This can be extremely useful for comparisons amidst datasets, allowing for refined control over the information retrieved.
Moreover, subqueries can be employed for aggregations. For example, nested queries can help in calculating aggregated values that can then be utilized to filter main result sets. This dual-functionality elevates the role of subqueries, proving them essential not merely as an option, but as a necessity in some complex queries.
To sum up, subqueries effectively refine data retrieval methods in SQL, providing intricate pathways to the information we seek. Understanding how to define and implement them correctly opens doors to more analytic and dynamic data management strategies.
Types of SQL Subqueries
Understanding the different types of SQL subqueries is key in mastering their implementation. Each type serves unique purposes and can affect the overall efficiency and accuracy of your queries. When dabbling in databases, knowing whether to use a single-row, multi-row, correlated, or non-correlated subquery can make all the difference in achieving a well-structured data retrieval process. Let's break down the main types and highlight their implications for SQL practice.
Single-Row vs. Multi-Row Subqueries
Single-row subqueries fetch exactly one row from the result set, while multi-row subqueries return multiple rows. This distinction is significant because it influences how you craft the SQL statement and the expectations of your results.
A single-row subquery is typically used when you need a specific value to filter your main query. For instance, if you are seeking the price of a product with a known product ID, a single-row subquery can directly yield that single act. Hereās a quick example:
This query will return the entire row for which the price matches that of product ID 5.
In contrast, multi-row subqueries shine when you require a set of values. Suppose you want to fetch information on all products with prices greater than those found in a certain category:
This will grab all products whose prices are in the list generated by the subquery targeting category ID 3.
Correlated Subqueries
Correlated subqueries stand apart as they reference columns from the outer query, creating a dependency between them. This means that the inner query must be executed for each row processed by the outer query, resulting in a less efficient but often necessary operation. In essence, for every single row of the outer query, the inner subquery runs.
Hereās an illustrative example:
This SQL statement pulls out the names of employees earning more than the average salary in their respective departments. It demonstrates how the correlated subquery uses the outer query's department identifier to compute average salaries dynamically.
Non-Correlated Subqueries
On the other hand, non-correlated subqueries operate independently of the outer query. They are executed once, and their results can be applied universally within an outer query. Because of this independence, they tend to be more efficient and straightforward to implement.
An example of a non-correlated subquery can be seen in the following query:
In this case, the inner subquery finds all departments located in New York, and the outer query retrieves the names of employees who belong to these departments.
Key Takeaway: Knowing the difference between these types of subqueries helps you choose the right approach. Depending on your needs, one type may be more suitable than another, impacting not just performance, but also the complexity of your SQL code.
Structure of a Subquery
Understanding the structure of a subquery is fundamental to effectively harnessing its power within SQL. Consider it the backbone that supports complex querying. When you understand the architecture, you can build queries that not only return the correct data, but also do so efficiently.
A subquery typically consists of a SELECT statement nested inside another SQL statement, often appearing in the main query's WHERE or SELECT clause. This layered approach allows for a more dynamically crafted query where results can be filtered or computed based on another set of results. With its ability to encapsulate a query, it provides modularity and a seemingly scalable solution, especially when handling intricate data retrieval requirements.
Basic Syntax of Subqueries
For those who are just stepping into the realm of SQL subqueries, hereās how the basic syntax breaks down:
In this snippet, the inner query, often called the child query, is executed first. The results are then fed into the outer query, known as the parent query. This sequential processing is essential to how subqueries operate, and knowing this hierarchy can help one foresee potential pitfalls, such as performance issues.
Placement of Subqueries in SQL Statements
Subqueries can be strategically placed in several locations within an SQL statement, but knowing where to put them can enhance both readability and performance. They primarily find homes in:
- SELECT Clause: This is where you use a subquery to calculate a value that is derived from a different dataset. For instance, calculating average sales per region can be neatly wrapped in a subquery that aggregates data from a related sales table.
- WHERE Clause: This placement is likely the most common use. You can filter results based on conditions that rely on results from another query. An example might be retrieving customers whose total purchase amount exceeds a set threshold.
- FROM Clause: Sometimes, you might want to treat the results of a subquery as a table. Placing a subquery in the FROM clause allows for a more complex dataset to be accessed directly within the main query, affording greater flexibility.
As an illustration:
In this example, the ad hoc view created through the subquery can be manipulated just like any other table.
"Subqueries offer a unique way to approach data, giving you the opportunity to think outside the conventional querying boxes."
Use Cases for SQL Subqueries
Understanding how to effectively utilize SQL subqueries can profoundly influence the way we approach data retrieval tasks. Subqueries provide a powerful mechanism for users to isolate data out of a larger dataset and derive meaningful insights from it. Through practical use cases, we can see how subqueries shine in various scenarios, allowing for more elegant, efficient, and maintainable SQL statements.
Data Filtering with Subqueries
Data filtering is one of the most straightforward yet impactful use cases for subqueries. When you want to extract specific records from a database that meet complex criteria, a subquery can come in handy. Rather than relying solely on a basic or multiple clauses, a subquery allows for a cleaner and more intuitive approach.
For instance, imagine you are managing a database for a retail store. You want to identify customers who have spent more than the average amount this year. A naive approach could involve calculating the average in one query and then using that value in a second query. However, you can achieve this in a single SQL statement using a subquery:
In this example, the subquery calculates the average spending of customers in the year 2023. The outer query then filters customers based on whether their spending exceeds this average. This not only streamlines the process but also keeps the code clean and avoids hardcoding of values.
Utilizing subqueries in data filtering provides several benefits:
- Enhanced clarity: The SQL statement succinctly expresses the intent, making it easier for others (or yourself in the future) to read and understand.
- Reduced redundancy: By calculating values within the subquery, you minimize repeated calculations and the potential for discrepancies in logic.
- Flexibility: If the average needs to change (e.g., based on a different year), only the subquery's logic needs modification, while the outer query remains intact.
When leveraging subqueries for data filtering, it's crucial to ensure your database is structured appropriately. Large datasets can slow down execution times, especially if the subquery runs repeatedly. Keeping performance in mind is key as you develop your queries.
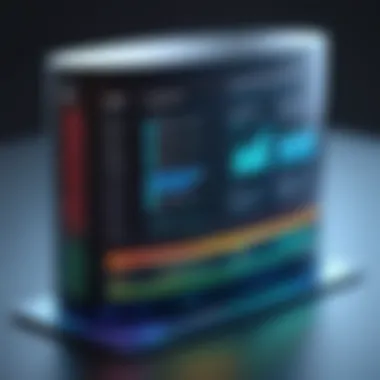
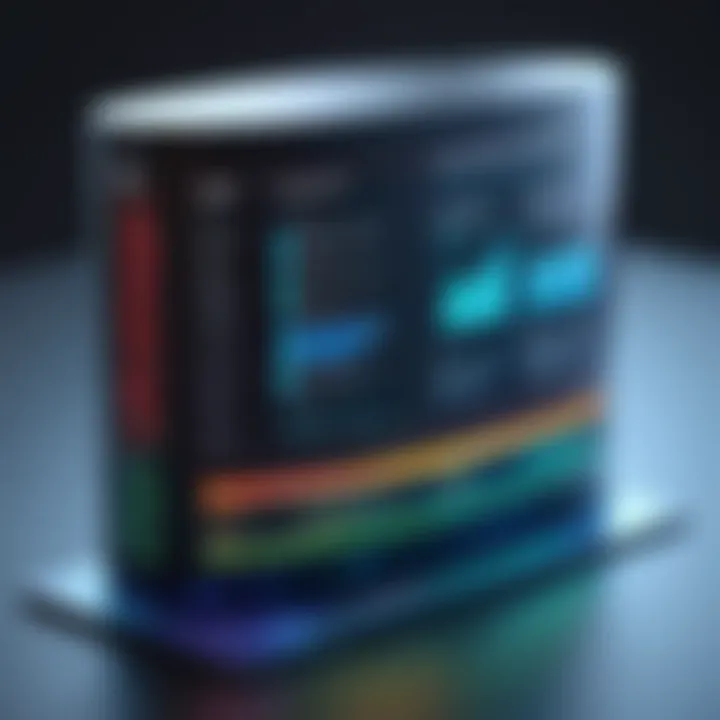
Aggregation and Subqueries
Aggregation is another common scenario where subqueries can provide significant advantages. When aggregate functions like , , or are combined with subqueries, you end up with a robust querying power capable of slicing and dicing your data in ways that basic SQL combinations struggle to achieve.
Consider you have a sales database and want to determine the total quantity sold for products above a certain threshold, say the average quantity sold. Instead of first calculating the average and then selecting products, we can embed the average calculation into the overall query:
In the example above, the subquery joins various layers of logic to calculate overall product sales while filtering out those that donāt meet the aggregate requirements. This method poses several benefits:
- Dynamic insights: Since the conditions for aggregation can be embedded within the same query, it allows real-time data verification without separate steps.
- Complex calculations: You can nest multiple layers of aggregation, enabling sophisticated insights only feasible through structured subqueries.
- Efficiency: Performing calculations in one query minimizes round trips to the database, which can be critical for performance in high-volume instances.
For data analysts and database administrators, employing subqueries in aggregation tasks ensures complex requirements can be met seamlessly, enhancing both the depth and quality of insights drawn from your data.
"In essence, effective SQL subquery use cases epitomize the marriage of clarity and complex querying ā a true mark of expertise in database management."
Performance Considerations
When dealing with SQL subqueries, understanding performance considerations is crucial. This section delves into how subqueries can influence query performance and offers insights into optimizing them for efficiency.
Impact on Query Performance
The influence of subqueries on overall performance canāt be shrugged off. When a subquery gets executed, the database engine may have to process that query separately before it can incorporate its results into the main query. This often leads to additional processing time, especially when dealing with large datasets.
In simpler terms, think of it like making a long detour on your road trip; you end up spending more fuel and time. The performance hit can be even more pronounced in cases where nested subqueries are involved, where one subquery relies on the results of another, creating layers of complexity.
Factors Influencing Performance
- Size of Dataset: The larger the dataset, the more time the database might take to fetch results from subqueries.
- Complexity of Subqueries: More complex queries can lead to inefficient execution plans.
- Indexes: Proper indexing can significantly mitigate performance issues.
- Database Design: Well-structured databases are naturally more efficient than poorly designed ones.
A key takeaway here is that while subqueries often make retrieval of specific data simpler, they can drain performance if not done smartly. It's essential to strike a balance between readability and efficiency.
Optimizing Subqueries for Efficiency
To enhance the performance of subqueries, several strategies can be employed. Each technique addresses the potential inefficiencies that come with using subqueries in SQL. Here are some optimization tips:
- Replace Subqueries with Joins: It's sometimes faster to use a join instead of a subquery, particularly when the subquery returns a large volume of data.
- Use EXISTS Instead of IN: When checking for the existence of records, prefer over . This often results in better performance, especially with larger data sets.
- Limit Results: Where possible, use in your subquery to minimize the number of returned rows.
- Avoid Nested Subqueries: Nested subqueries can dramatically increase execution time. If feasible, try to flatten them.
Effective optimization not only reduces execution time but also improves resource utilization.
Best Practices for Writing Subqueries
Creating effective SQL subqueries requires not just understanding syntax, but also awareness of common pitfalls and techniques that enhance clarity and performance. This section aims to illuminate some key best practices that can be applied when writing subqueries.
Common Mistakes to Avoid
When delving into subqueries, it's all too easy to step into pitfalls that can cause headaches down the road. Here are some common mistakes you will want to sidestep:
- Neglecting Performance: One of the most frequent blunders is overlooking the potential performance impact of subqueries. Overusing subqueries, especially in large datasets, can turn a quick query into a substantial drag. Always assess your subquery for efficient execution.
- Misplacing Subqueries: Placing a subquery in an inappropriate context can lead to nonsensical errors. For example, putting a multi-row subquery where only a single value is expected can cause issues. Always verify that the context aligns with the type of subquery youāre using.
- Ignoring Readability: In the rush of coding, some may forget about readability. Having overly complex subqueries can make maintenance a nightmare. Keeping your subqueries as straightforward as possible is vital for anyone who may work on the code laterāthis includes your future self!
- Not Using Aliases: It's advisable to use aliases for subqueries in order to simplify reference points, particularly in complicated queries. If you donāt, it can lead to confusion quickly.
- Failing to Test: Lastly, itās essential to test your subqueries independently before embedding them into larger SQL commands. This helps identify issues early and makes debugging much easier.
Improving Readability of Subqueries
An often-overlooked aspect of writing subqueries is ensuring that they are easy to read and understand. Effective readability does not just benefit you; it aids anyone else who might engage with your SQL scripts. Here are a few strategies:
- Use Whitespace: Make good use of whitespace in your SQL code. Proper indents and spaces can clarify the structure of your subqueries, making it easier to follow the logic.
- Descriptive Aliases: Utilize descriptive names for your aliases instead of cryptic short forms. For example, if your subquery calculates the total sales, name your alias something like rather than .
- Break Down Complex Queries: If a subquery is becoming unwieldy, consider breaking it down into smaller, manageable pieces. This can often be achieved through temporary tables or Common Table Expressions (CTEs), which allow for greater modularity and clarity.
- Commenting: Utilize comments to explain the intent behind your subqueries or any complex logic youāve implemented. While SQL is meant to be self-explanatory, a little context can go a long way, especially in intricate queries.
Always strive for clarity. A well-described subquery can save loads of time during troubleshooting.
Real-World Examples of Subqueries
Understanding how subqueries operate in practical situations is crucial for anyone looking to optimize their SQL skills. Subqueries not only help in data retrieval but also enhance the clarity and efficiency of SQL statements. They allow for a layered approach to data management, making it easier to handle complex queries which might otherwise become convoluted when using direct joins alone.
Subqueries can act as a powerful tool to fetch data based on conditions derived from other queries. This results in more organized code and can often lead to improved performance if utilized wisely. When tech professionals and students comprehend these real-world applications, they can effectively implement subqueries to solve intricate database problems, thus making them a vital part of their SQL toolkit.
Example One: Subquery in SELECT Statement
In this example, we examine how a subquery can be used within a statement to dynamically compute values. Letās say we have a database with a table named , containing individual employee records, including their salaries. If one wants to retrieve the names of employees who earn more than the average salary in the company, you might write:
Here, the subquery calculates the average salary and then serves as a filter for the main query. This approach is quite effective because it makes your query self-contained, clearly defining how the average was derived without any extra calculations.
Example Two: Subquery in WHERE Clause
Moving onward, using subqueries in the clause can help narrow down results by applying specific conditions. Consider you need to find customers who have placed orders greater than $500. Assuming we have a table and table, you might approach it like this:
In this case, the inner subquery retrieves all s that meet the order total condition, while the outer query uses that list to filter the names. This method streamlines the retrieval process, and itās clear how you derive your customer list based on their purchasing behavior, not just arbitrary numbers.
Example Three: Subquery in FROM Clause
Lastly, we can also utilize subqueries in the clause for intermediate calculations or simplified data handling. For instance, if we want to create a summary of average order totals by customer, we could do something like this:
In this case, the subquery creates an intermediate result set that can readily handle. This is particularly useful when working with complex datasets, enabling you not only to derive values like averages but also enhancing overall readability by abstracting lower-level computations.
Subqueries can greatly enhance your SQL capabilities, making your queries more efficient and easier to read.
Overall, these examples highlight the versatility of subqueries, showcasing their application across different clauses. They allow for abstraction and efficient data retrieval, which is essential in handling large datasets or complex queries.
Subqueries vs. Joins
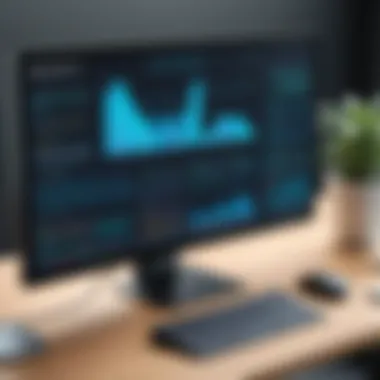
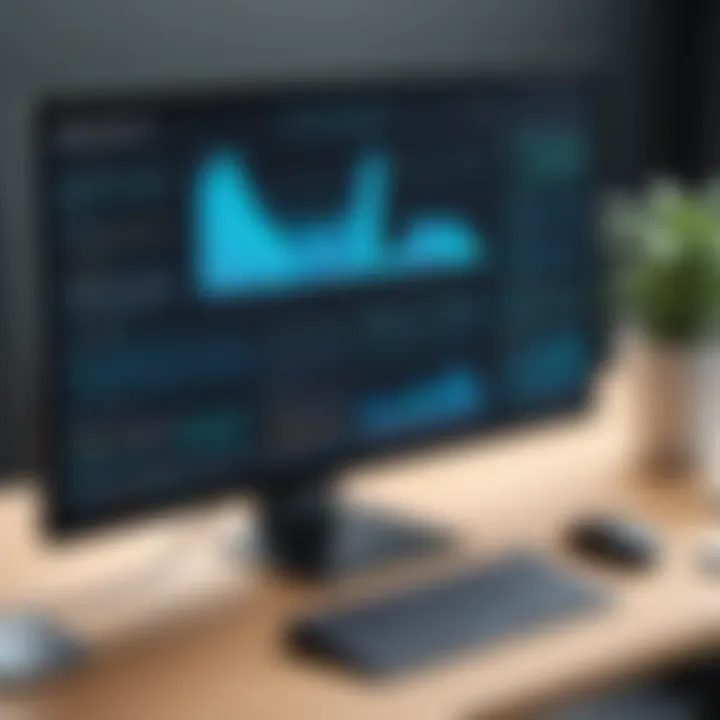
When navigating the murky waters of SQL, understanding the distinction between subqueries and joins can feel like comparing apples to oranges. Both approaches are pivotal in data retrieval, yet they function in ways that significantly influence query efficiency and complexity. Knowing when to leverage a subquery versus a join can mean the difference between a slick operation and a sluggish data-fetching experience. Often, developers may find themselves pondering which method to apply in their given scenarios, and it's not as straightforward as one might think.
Comparative Analysis
Subqueries and joins serve distinct purposes in SQL. While both allow you to fetch related data, their utilization often depends on the context of the data being requested.
- Subqueries, as discussed previously, query data from one table and use that data to filter or modify the query against another table. They are nested within the main query, often aiding in simplifying complex logic. A subquery can be ideal when you want to focus on a single piece of information before proceeding.
- Joins, on the other hand, combine rows from two or more tables based on related columns between them. They are more efficient when needing a bulk of data from different tables in a single go. For instance, if you want to combine customer orders from one table with customer details from another, a join can expediate this process without the need for nested queries.
To sum it up, while subqueries take a more step-by-step approach, joining tables is akin to gathering everything in one sweep. The choice between these methods can dictate not only how easy it is to write the query but how performant it turns out to be.
When to Use Each Approach
Determining when to implement a subquery instead of a joināor vice versaādepends largely on what you're trying to accomplish:
- Choose Subqueries when:
- Opt for Joins when:
- You require a single value from another table to filter your main query, such as fetching the average salary from an employee table to compare against individual salaries.
- The logic of your query benefits from a less cluttered syntax. This involves writing a clean, straightforward query without becoming tangled in multiple join conditions.
- The subquery returns a limited number of rows, especially if using it in a clause like WHERE or HAVING. Itās perfect, say, for checking if a project exists in a project table based on department ID.
- You need to retrieve complete datasets from multiple tables, especially in scenarios where there is a one-to-many or many-to-many relationship.
- Performance is critical, and you want to minimize the number of database calls. Joins can handle larger records much quicker since they operate on sets of rows rather than relying on nested data retrieval.
- Data integrity is a concern, and you need comprehensive visibility into related records, such as having customer transaction histories alongside customer demographics.
In the end, whether you fetch data using subqueries or joins, considering the specific context and requirements of there's paramount. As you evolve in your SQL journey, these decisions will sharpen your skills, enabling you to write refined SQL queries like a seasoned pro.
To align with best practices, always think about maintainability and readability of your queries. Your future self will be thankful you did.
Advanced Techniques Involving Subqueries
Understanding advanced techniques involving subqueries is essential for unlocking the full potential of SQL. These techniques allow for more complex queries, enabling elaborate data manipulations and analysis that are often required in today's database-driven environments. The ability to harness subqueries in advanced contexts, like recursive structures or stored procedures, can significantly enhance both the efficiency and clarity of your SQL code. In essence, mastering these techniques equips tech professionals with powerful tools that streamlines data handling, ultimately boosting productivity and performance.
Using Subqueries for Recursive Queries
When it comes to databases, recursion isnāt just a fancy term tossed around in programming classes; itās a practical and powerful method to solve hierarchical data problems. A recursive subquery essentially calls upon itself to navigate through various layers of results. This is extremely handy when dealing with data that is inherently nested, such as organizational charts or category trees.
For example, consider a database holding employee information, where each employee has a designated manager. To fill out all levels of hierarchy, you can utilize a recursive subquery to fetch all employees under a specific manager:
This subquery starts by selecting top-level employees (those without a manager) and then continues to include their direct reports, effectively building a complete hierarchy. This approach minimizes the number of queries, presenting a clear method to handle complex data structures efficiently. Using recursive subqueries can lead to cleaner, less error-prone queries compared to multiple joins or union clauses.
Leveraging Subqueries in Stored Procedures
Stored procedures are the backbone of many database applications, providing a way to encapsulate and manage complexity through reusable scripts. Subqueries play an invaluable role in enhancing stored procedures, allowing for dynamic and adaptable queries tailored to various scenarios.
Incorporating subqueries in stored procedures offers several unique advantages:
- Dynamic Data Retrieval: Subqueries can adjust the data fetched based on parameters, making stored procedures flexible and context-sensitive.
- Simplifying Code Maintenance: By enclosing subquery logic within stored procedures, you avoid repetitiveness and reduce the chances of errors when changes are needed.
- Improved Readability: Complex logic can be neatly nested within subqueries, leading to cleaner and more understandable code.
Hereās a practical example of employing a subquery within a stored procedure:
In this case, the stored procedure retrieves all employees who report to a specific manager. The subquery streamlines the process, showing exactly which employees fall under the designated manager without complicating the primary code logic. Moreover, leveraging subqueries in stored procedures not only optimizes performance but also enables developers to maintain clean, effective, and simpler codebases.
Troubleshooting Common Issues
In the world of SQL subqueries, things donāt always go according to plan. You might write a query that seems straightforward at first, but an unexpected outcome can lead to frustration. Troubleshooting common issues related to subqueries is essential for multiple reasons. It helps ensure that your SQL commands execute properly, yields accurate data, and minimizes wasted time spent on queries that don't work as intended. Understanding how to diagnose errors and debug your queries can set you apart from the crowdāa necessity for students, IT professionals, and budding programmers alike.
Diagnosing Subquery Errors
When a subquery throws you for a loop, diagnosing the error is the first step to finding a solution. One aspect to consider is the overall structure and syntax of your query. Subqueries can often lead to error messages or results that simply donāt make sense due to common pitfalls like misplaced parentheses, incorrect data types, or missing keywords. For instance, if you have a subquery that returns unexpected results, it could stem from flawed logic or a misunderstanding of how the subquery is integrated with the outer query.
Here are a few steps to help you diagnose subquery errors:
- Verify Syntax: Always start with a line-by-line review of your SQL syntax. Ensure that commands, parameters, and functions are correctly specified.
- Use Simplified Queries: Before executing the entire SQL command, simplify it to its core components. Test the subquery independently to see if it returns the expected results.
- Check Data Types: Ensure that the data types in your subquery match those expected during comparisons and joins. Mismatches can lead to runtime errors.
- Review Execution Plan: Utilize the execution plan feature of your SQL database to understand how your query is being processed.
A little meticulousness goes a long way, and recognizing where things go wrong can save you hours of headache.
Debugging Techniques for Subqueries
Once you've diagnosed the error, the next step is effectively debugging the subquery. This process might seem daunting initially, but it doesnāt have to be. Here are some handy techniques that can help you clear up issues and fine-tune your subqueries:
- Use Common Table Expressions (CTE): Instead of placing a subquery directly into the main query, consider using a CTE for clarity. This separation helps simplify complex relationships, making it easier to spot errors.
- Logging Intermediate Results: When debugging, log the results of intermediate steps. It could involve temporarily inserting logs into the database to track values returned by your subquery. This technique helps visualize how data traverses through your queries, making it easier to identify anomalies.
- Test for Nulls: Always check how your subqueries handle null values, as they can significantly affect query outcome.
- Break Down Complex Queries: Segment complex queries incrementally, confirming each part is functioning before combining them.
"A stitch in time saves nine; addressing errors early prevents cascading issues later."
By applying these troubleshooting tactics, you not only solve immediate problems but also build a deeper understanding of how SQL subqueries work. With practice, diagnosing and debugging will become second nature, helping you write more effective SQL queries.
Future of SQL Subqueries
The future of SQL subqueries is not just a mere footnote in database discussions; itās a vital piece of the evolution in how we manage data. With the increasing complexity of data systems, the role that subqueries play is becoming more pronounced. As applications grow and data requirements shift, relying on efficient data retrieval methods is essential. Subqueries, being a potent tool in SQL, allow developers to dive deep into data analysis without convoluting the main query. This helps in not only simplifying query structure but also enhancing overall performance.
As technology advances, the integration of SQL subqueries in various database management solutions will become paramount. Itās important to understand the emerging trends associated with subqueries, particularly how they interact with new database frameworks and methodologies. The ability to adapt to these trends will determine the efficiency of data handling in future applications.
Emerging Trends in Database Management
In today's fast-paced tech landscape, several trends are shaping the way we think about database management and specifically, subqueries. Here are some significant elements:
- Increased Use of Cloud Databases: As businesses transition to cloud computing, database management systems are evolving too. Subqueries are optimized in many cloud platforms for enhanced scaling and performance.
- Focus on Data Privacy: With regulations like GDPR influencing data management strategies, subqueries help in accessing user-specific data without compromising privacy, paving the way for compliant and responsible data handling.
- Integration with Big Data Technologies: As the volume of data grows, subqueries can interact more seamlessly with big data tools (like Apache Spark). This integration enables massive datasets to be queried using familiar SQL syntax while maintaining efficiency.
These trends signify that subqueries will not fade away; rather, they'll evolve, enhancing their role in modern database management.
The Evolution of SQL Subqueries in New Systems
Database systems are often perceived as static constructs. However, they are malleable, transforming in tandem with user needs and technological advancements. The evolution of SQL subqueries can be observed through several lenses:
- Improved Optimization Algorithms: New systems are integrating sophisticated optimization techniques that enable subqueries to execute faster and more efficiently. This makes it feasible to handle large datasets without a hitch.
- Increased User-Friendliness: As more people venture into SQL, database systems are focusing on making subqueries easier to write and debug. Features like visual query builders help users construct subqueries intuitively.
- Support for NoSQL Environments: Even in non-relational database systems, the concept of subqueries is gaining traction. Elements similar to SQL subqueries are being incorporated into NoSQL databases, allowing users to perform complex data retrieval tasks without sacrificing performance.
In summary, the future of SQL subqueries is bright, as they adapt and integrate with emerging trends and technologies. Recognizing these developments will allow tech enthusiasts and professionals to leverage subqueries as powerful assets in their data analysis toolkit, ensuring they stay ahead in an ever-evolving digital landscape.