Mastering Swift: A Comprehensive Guide

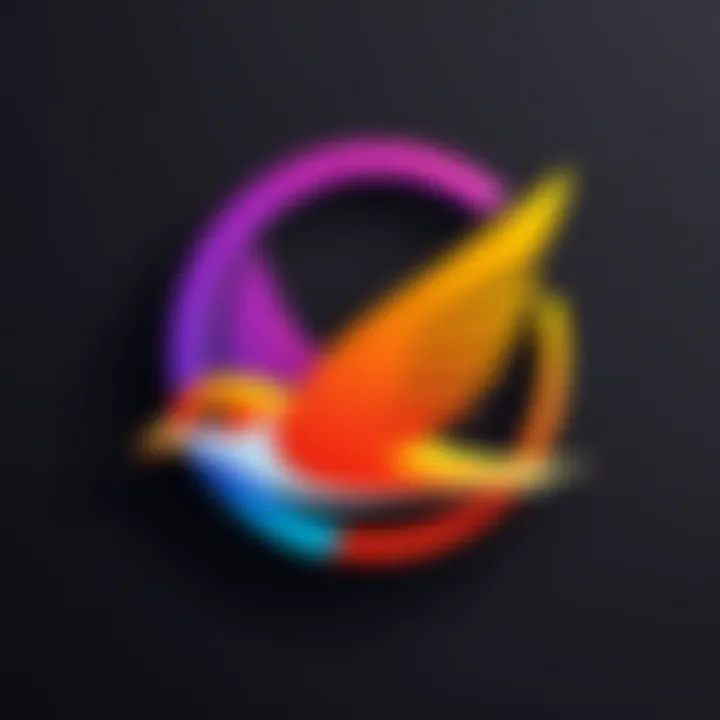
Overview of Topic
Preface to the main concept covered
Swift is a programming language developed by Apple Inc. It's primarily used for creating applications for iOS and macOS. The language is designed to be fast, modern, and powerful, allowing for a greater degree of flexibility and performance than many of its predecessors. Its clean syntax makes it accessible for newcomers, while also catering to the complexities demanded by experienced developers.
Scope and significance in the tech industry
Swift has gained significant traction in the tech industry. Its relevance is fueled by the growth of Apple's ecosystem, making it vital for developers interested in mobile app development. Swift provides robust features and tools for building apps that not only meet but exceed user expectations. As businesses increasingly move towards mobile solutions, understanding Swift becomes crucial.
Brief history and evolution
Introduced in 2014, Swift aimed to replace Objective-C, which had been the foundation of iOS development for years. Swift's concise syntax and safety features were revolutionary at that time. Over the years, continuous improvements have been made, including the introduction of new libraries, frameworks, and support for server-side development. Its open-source nature has also fostered a vibrant community, accelerating its evolution and adoption.
Fundamentals Explained
Core principles and theories related to the topic
At its core, Swift is built on principles of simplicity, performance, and safety. It emphasizes clarity, with a syntax that reduces the chance of errors during development. Swift includes features such as optionals, generics, and closures, which help manage complexity in large codebases.
Key terminology and definitions
- Optionals: A powerful feature that handles the absence of values, preventing runtime crashes.
- Closures: Self-contained blocks of functionality that can be passed around and used in your code.
- Generics: A way to write flexible, reusable functions and types that work with any type.
Basic concepts and foundational knowledge
New learners should grasp the following concepts to build a solid foundation in Swift:
- Variables and Constants
- Data Types (Strings, Integers, Arrays, Dictionaries)
- Control Flow (If Statements, Loops)
- Functions and Methods
- Classes and Structures
Practical Applications and Examples
Real-world case studies and applications
Many apps in the App Store are built using Swift. High-profile applications such as Airbnb and LinkedIn utilize Swift to deliver seamless user experiences. In addition, numerous startups and large enterprises prefer Swift due to its efficiency and performance metrics.
Demonstrations and hands-on projects
Engaging with Swift requires practical experience. A common starting project is developing a ToDo list app, which encompasses a variety of essential skills such as UI design, database management, and user interaction handling.
Code snippets and implementation guidelines
Here’s a basic example of a function in Swift that calculates the area of a rectangle:
Advanced Topics and Latest Trends
Cutting-edge developments in the field
As Swift evolves, it incorporates modern programming paradigms. The introduction of SwiftUI allows developers to create user interfaces declaratively, streamlining the app development process. Moreover, Swift's interoperability with Python and JavaScript opens new avenues for integration.
Advanced techniques and methodologies
Developers should explore advanced topics such as:
- Protocol-oriented programming
- Functional programming concepts
- Concurrency and Multithreading in Swift
Future prospects and upcoming trends
The language is positioned for continued relevance. As Swift adapts to new technologies like augmented reality and machine learning, its scope will expand, encouraging developers to innovate within the Apple ecosystem.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
For those eager to dive deeper, consider the following resources:
- "The Swift Programming Language" (Apple’s official guide)
- Online courses on platforms like Coursera and Udemy
- Swift forums on Reddit and Stack Overflow for community support
Tools and software for practical usage
To get started with Swift, use tools such as:
- Xcode: The official Integrated Development Environment (IDE) for macOS.
- Playgrounds: For experimenting and prototyping code snippets quickly.
Learning Swift can be both exciting and rewarding. With its rich ecosystem, understanding Swift offers a pathway to engaging with modern technology and building applications that resonate with today's users.
Prelude to Swift
Learning Swift is vital for anyone interested in Apple’s platforms, particularly iOS and macOS development. Swift offers modern programming tools while maintaining performance and safety. Understanding Swift help programmers create efficient and reliable applications. This section intends to elucidate the significance of Swift for developers today.
The Evolution of Swift
Swift was introduced by Apple in 2014. It was developed to overcome limitations of Objective-C, which was the previous primary language for Apple app development. Swift combines the best ideas from many programming languages and implements an open-source model. This evolution has made it easier for developers to adopt and use the language as it grows.
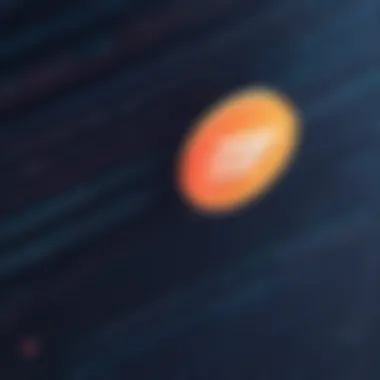
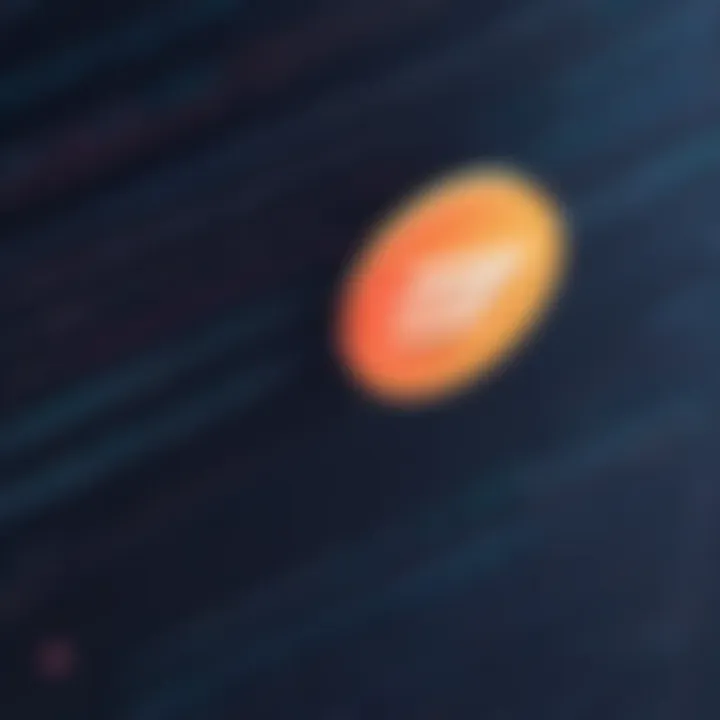
The design of Swift emphasizes performance and efficiency. By incorporating features like type inference and functional programming, it provides a more progressive programming experience. Over the years, Swift has undergone several updates, with each version bringing enhancements to syntax, performance, and interoperability. This adaptive nature makes Swift a preferred choice for developers.
Why Swift? An Overview
Swift's relevance cannot be overstated. Here are the compelling reasons to learn Swift:
- Safety: Swift’s strong type system and error handling mechanisms contribute to robust applications.
- Performance: Swift programs often run faster than those written in Objective-C due to optimized back-end code.
- Readability: The clean and expressive syntax allows developers to read and write code more efficiently, reducing development time.
- Interoperability: Swift works seamlessly with existing Objective-C code, allowing developers to integrate new functionality without completely rewriting applications.
- Community and Resources: A vigorous community support exists, providing tutorials, libraries, and frameworks that enhance learning and development.
Swift’s benefits make it an attractive language for both beginners and seasoned developers aiming to build sophisticated applications across Apple’s ecosystem.
Setting Up the Environment
Establishing a solid development environment is crucial for successful programming in Swift. It ensures that you have all the necessary tools and configurations in place to maximize your productivity. This section focuses on key aspects of setting up your Swift environment and the benefits that come with it.
The first step in becoming proficient with Swift is equipping your computer with Xcode. This integrated development environment (IDE) not only offers a space to write code, but it also provides helpful features like syntax highlighting, debugging tools, and a user-friendly interface. Without a proper setup, you might face hurdles that can impede your learning process.
Installing Xcode
Installing Xcode is a straightforward process, essential for any iOS or macOS developer. Xcode serves as the primary IDE for Swift development and offers all the tools needed to write, test, and debug your code. To begin, follow these steps:
- Open the Mac App Store.
- Search for "Xcode" in the search bar. Make sure you download the official version.
- Click on "Get" or "Install" to start the download. Xcode is a large app, so it might take some time depending on your internet speed.
- Once installed, open Xcode. You might need to accept the license agreement.
After installation, it's wise to check for updates. Apple frequently improves Xcode with new features and bug fixes. You can update directly from the Mac App Store.
Configuring Your First Project
Once Xcode is installed, it's time to start your first project. This step is significant, not just for practical experience, but also for familiarizing yourself with Xcode's interface and functionalities. To configure your project, follow these detailed steps:
- Open Xcode and select "Create a new Xcode project."
- Choose the project template. For beginners, the "iOS App" template is generally the best choice.
- Set a product name and select a team. If you're an individual developer, your personal account will work.
- Choose the programming language as Swift.
- Select SwiftUI or UIKit as your interface. SwiftUI is more modern, but UIKit has been widely used.
- Set the project directory where you wish to save your files, and then click "Create."
As you navigate through the project setup, familiarize yourself with the various sections of Xcode, such as the Navigator on the left, the Editor in the center, and the Inspector on the right. Understanding these components will enhance your coding efficiency.
"A well-set development environment can lead to fewer distractions and better focus on coding."
Through these initial steps, you pave the way for a productive journey in Swift development. By taking the time to properly set up your environment, you reduce the learning curve and enable smoother progress as you delve deeper into Swift programming.
Swift Syntax Basics
Understanding Swift syntax is crucial for anyone looking to develop applications using this programming language. Swift's syntax is clean and expressive, making it easier for both new and seasoned programmers to write maintainable and readable code. Knowing the basics of Swift syntax lays the foundation for more complex programming concepts. In this section, we will explore variables and constants, data types, and control flow constructs. Each subsection will highlight the relevance and utility of these elements in practical applications.
Variables and Constants
In Swift, variables and constants are fundamental components. A variable is a named memory location that can hold a value which can change during the program's execution. Conversely, a constant is defined with the keyword and indicates a value that cannot be modified once set.
Using variables and constants effectively can lead to better memory management and prevent unintentional changes to values.
Key Points:
- Variables are declared using the keyword. For example,
- Constants are declared with . For example,
- Use constants for values that remain unchanged during execution.
- Utilizing the right type can enhance code readability.
Data Types Overview
Data types are essential in programming as they define the kind of data a variable can hold. Swift is strongly typed, meaning that every variable must be declared with a type. This reduces errors that can occur during runtime.
Swift provides various built-in data types including:
- Int for integers.
- Double for floating-point numbers.
- String for textual data.
- Bool for true/false values.
Understanding data types assists in selecting the appropriate one for your needs, optimizing memory usage, and ensuring your code runs smoothly. Misusing data types can lead to type errors, which can be tedious to debug.
Control Flow Constructs
Control flow constructs allow for decision-making within your code. They enable you to execute different code paths based on specific conditions. In Swift, common control flow constructs include , , , and .
Overview of Control Flow Constructs:
- If statements execute code blocks based on boolean conditions. Here’s an example:
- Switch statements provide a cleaner way to handle multiple conditions compared to multiple statements.
- For loops iterate over collections.
- While loops execute as long as a specified condition evaluates to true.
Object-Oriented Programming with Swift
Object-oriented programming (OOP) is a paradigm that enables developers to design software in a more organized way. In Swift, OOP principles play a crucial role in building scalable and maintainable applications. Understanding these principles helps developers to encapsulate, reuse, and manage code efficiently.
When you work with Swift, the focus on objects allows a program to mimic real-world relationships. This approach offers several benefits, including improved code readability, enhanced maintainability, and easier debugging. Moreover, Swift’s support for OOP can help streamline applications by promoting modular design, which can be crucial in large projects.
As you delve into Swift, you will encounter diverse elements like classes, structures, inheritance, and protocols, which are central to OOP. Each component serves a specific purpose and contributes to the overall functionality of your code. Hence, a solid grasp of OOP concepts is necessary for anyone looking to master Swift.
Classes and Structures
In Swift, both classes and structures are fundamental building blocks of OOP. They allow you to create complex data types that can encapsulate both data and functionality. Classes are reference types, while structures are value types, which leads to different behaviour when it comes to memory management and assignment.
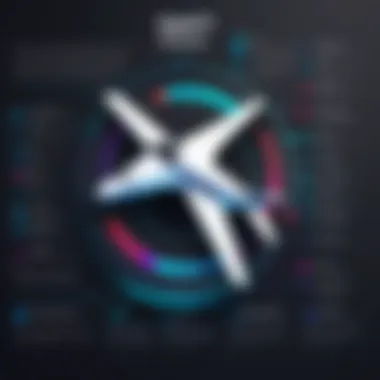
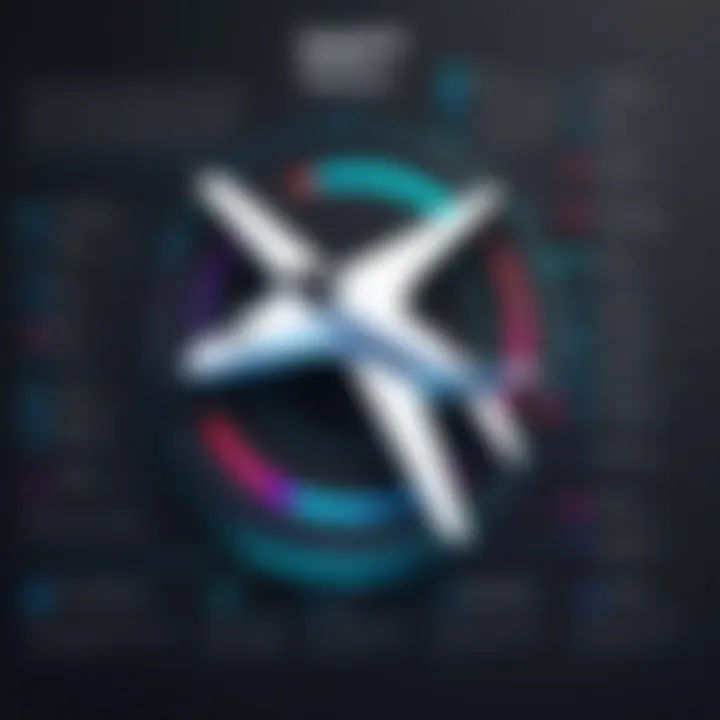
- Classes allow for inheritance, meaning that you can create new classes based on existing ones. This feature promotes code reuse and the creation of more specialized classes with minimal effort.
- Structures, on the other hand, make copies when they are assigned or passed. This behaviour is especially important when immutability is desired.
Both classes and structures can have properties, methods, and initializers. Understanding when to use one over the other is crucial. Generally, if you need reference semantics, opt for classes. If you are looking for value semantics, structures are your go-to.
Inheritance and Protocols
Inheritance is a powerful feature of OOP that allows a class to inherit properties and methods from another class. In Swift, this means that you can create a base class and extend it into subclasses. This facilitates code reuse and improves the organization of your application.
- Inheritance in Swift allows subclasses to override or extend functionalities while maintaining the integrity of the base class. This concept is especially useful when you are dealing with a hierarchy of related classes.
Protocols, however, introduce a different level of abstraction. A protocol defines a blueprint of methods and properties that can be adopted by any class or structure. This is critical for promoting coding standards and ensuring that different types conform to a consistent interface.
"Protocols are ideal for defining shared behaviours among disparate types, making them particularly valuable in large projects."
In summary, mastering OOP in Swift allows developers to create more robust and dynamic applications. By understanding classes, structures, inheritance, and protocols, you position yourself to leverage the full power of Swift’s object-oriented features.
Exploring Swift's Advanced Features
Exploring advanced features in Swift is crucial for those who seek to elevate their coding skills and make the most of the language's capabilities. These features are not just enhancements; they significantly affect code readability, efficiency, and maintainability. Knowing how to effectively utilize advanced Swift topics can lead to cleaner code and solutions that are more powerful and flexible. In this section, we will analyze topics like closures, error handling, and generics—all fundamental elements for crafting robust and scalable applications.
Swift Closures
Closures are a core concept in Swift, serving as self-contained blocks of functionality that can be passed around and used in your code. They can capture and store references to any variables and constants from the surrounding context in which they are defined. This is often referred to as capturing values. The importance of closures in Swift comes from their versatility; they can replace function pointers, and they can be used as completion handlers in asynchronous functions, making them a critical instrument for handling tasks like network requests.
To define a closure, you can use a syntax similar to function definitions but without a name. Here's a simple example:
This illustrates the ease with which you can define and use closures. Utilizing closures can lead to more succinct and expressive code, particularly in callback functions or when using higher-order functions like , , and , enhancing both readability and functionality.
Error Handling Techniques
Error handling is a fundamental aspect of programming in Swift. The language provides a powerful error handling model that involves throwing and catching errors. This allows developers to write more resilient code by managing exceptional conditions gracefully instead of allowing the application to crash. Understanding these techniques is vital for developing reliable software applications.
Swift uses the syntax for error handling. When an error is thrown, it can be caught and dealt with accordingly. Here’s a short example:
This example shows how developers can manage errors effectively, enhancing the robustness of their applications. When done correctly, error handling makes the application more user-friendly, as users can receive clear messages indicating what went wrong and what actions to take.
Generics in Swift
Generics are another advanced feature that significantly boosts the flexibility and reusability of your code. They allow you to write functions and data types that can work with any type, subject to certain restrictions. This capability is crucial for reducing code duplication and enhancing type safety while maintaining performance.
Using generics can lead to cleaner and more maintainable code. For example, you might define a generic function as follows:
This function can swap two values of any type, not limited to just integers or strings. Utilizing generics, you can create flexible data structures like stacks and queues while ensuring type safety. This feature pushes Swift beyond basic type declarations, allowing for more dynamic and reusable code constructs.
In summary, mastering these advanced features in Swift—closures, error handling, and generics—is essential for building efficient, secure, and robust applications. With a strong grasp on these concepts, developers can take full advantage of Swift's capabilities, paving the way for a smoother and more productive development process.
Swift for iOS Development
Swift is integral to iOS development. It is a robust language designed specifically for building applications on Apple's platforms. The significance of Swift in this context cannot be overstated. It allows developers to write safer and more reliable code. With Swift, developers can leverage modern programming concepts such as optionals, generics, and functional programming patterns. These features not only enhance code quality, but they also streamline the development process.
One of the outstanding benefits of using Swift in iOS development is its performance. Swift is faster than its predecessor, Objective-C, making applications run more efficiently. This speed is crucial, as users expect seamless interactions from apps. Additionally, Swift's concise syntax reduces boilerplate code, allowing developers to focus on defining functionality rather than structure.
Another important consideration is Swift's safety features. Swift incorporates error handling and type checking, which significantly minimizes bugs during development. This focus on safety aligns with Apple's commitment to delivering premium user experiences.
In this section, we will explore three vital aspects of iOS development with Swift: the Model-View-Controller design pattern, user interface design, and networking capabilities. Each of these areas plays a critical role in creating a well-rounded application that meets user needs and expectations.
Understanding the Design Pattern
The Model-View-Controller (MVC) design pattern is a software architectural pattern that separates application logic into three interconnected components. This structure facilitates organized code and enhances the maintainability of applications.
- Model: Represents the data and the business logic of the application.
- View: Displays the data and sends user commands to the controller.
- Controller: Acts as an intermediary between the model and view, modifying the model in response to user input.
This separation of concerns is pivotal, especially in iOS development, where user interactions are frequent. Developers can focus on individual components without affecting the entire application. Understanding MVC empowers developers to create scalable and maintainable code as they build their iOS applications.
Working with User Interfaces
Creating user interfaces in iOS involves utilizing technologies like UIKit and SwiftUI. UIKit provides a comprehensive framework of prebuilt components that developers can use to construct interfaces. These components range from buttons and labels to tables and collection views.
On the other hand, SwiftUI, introduced recently, adopts a declarative syntax. This allows developers to describe their UI in a clear and straightforward manner. It automatically updates the interface when data changes, improving efficiency during development.
When designing user interfaces, considering usability and user experience is vital. Swift allows developers to create engaging and intuitive designs. Best practices include:
- Keeping layouts simple and consistent.
- Using color schemes and typography thoughtfully.
- Ensuring that accessibility features are integrated for inclusivity.
Networking with Swift
Networking in Swift is essential for applications that require data exchange with web services. Swift offers several libraries capable of handling networking tasks. One of the most commonly used is URLSession, which provides an API for downloading and uploading data to and from the internet.
When dealing with web services, it is essential to manage data formats effectively. JSON is widely used for data interchange, and Swift's native protocol streamlines this process. Developers can convert data between JSON and Swift objects seamlessly.
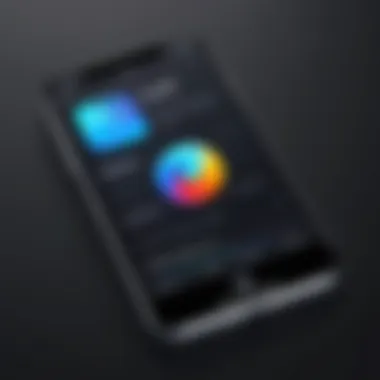
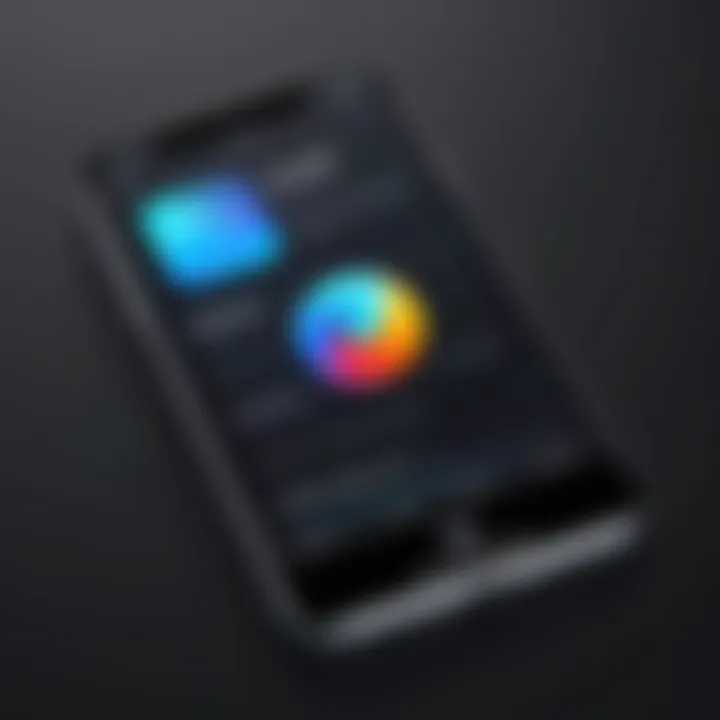
Here are some key practices for networking in Swift:
- Handle network errors gracefully.
- Use background tasks to maintain app performance during data fetches.
- Secure sensitive user information using best practices for API calls, such as HTTPS.
In summary, mastering Swift for iOS development opens doors to creating high-quality applications. Understanding important concepts like MVC, user interface design, and networking capabilities are essential for anyone looking to excel in this field.
"Swift provides the tools to transform your ideas into remarkable apps tailored for millions of users."
By harnessing these capabilities, developers can deliver efficient, user-friendly applications that make a meaningful impact.
Testing and Debugging in Swift
Testing and debugging are crucial elements of software development, particularly in the context of Swift, Apple's programming language. Developers must ensure that their code is not only functional but also efficient and maintainable. Testing allows developers to identify issues before deployment, while debugging aids in resolving those issues as they arise. This section addresses both practices in detail, giving insight into their respective importance in Swift development.
Effective testing contributes to overall software quality, fostering reliability in applications. By implementing testing strategies, developers can be confident in their code's ability to perform as intended. Debugging, on the other hand, is an iterative process that helps refine code and improve robustness.
Unit Testing Basics
Unit testing is a method where individual components of a program are tested in isolation to ensure they operate correctly. In Swift, the XCTest framework is commonly used for this purpose. Understanding unit testing can dramatically improve code quality and enhance developer productivity.
Key advantages of unit testing include:
- Early Bug Detection: Finding and fixing bugs during the development stage prevents issues from escalating.
- Refactor Confidence: Developers can safely refactor code, knowing that tests will catch any resulting errors.
- Documentation: Unit tests serve as a form of documentation, illustrating how various components are supposed to interact.
- Regression Prevention: When code changes, unit tests help prevent regressions by verifying that existing functionality remains intact.
A simple example of a unit test in Swift using XCTest may look like this:
This code showcases a straightforward unit test checking the addition operation. The process of writing such tests encourages clear thinking and clarity of purpose among developers.
Debugging Techniques
Debugging is a critical skill that every developer must cultivate. Swift offers several robust tools and techniques for debugging, which can help developers trace and identify errors effectively.
Some prominent debugging techniques include:
- Using Breakpoints: Developers can set breakpoints to pause code execution. This allows for examination of variable states and program flow at specific points.
- Print Statements: A simple yet effective debugging tool. By adding print statements, developers can inspect variable values dynamically during a run.
- View Debugger: In Xcode, the View Debugger aids in visualizing UI layouts and interactions, making it easier to understand how different components interact.
- Memory Graph Debugger: This tool helps visualize memory usage and identify memory leaks, essential for optimizing applications.
"Debugging is like being the detective in a crime movie where you are also the murderer."
Employing these techniques ensures that developers remain acutely aware of their code’s function and potential faults.
Ultimately, mastering testing and debugging within Swift empowers developers to deliver high-quality applications. They can approach projects with greater assurance, knowing their code has been rigorously validated. Developing these practices early in one’s career provides a valuable foundation for ongoing success in software development.
Deployment and Distribution
When it comes to software development, understanding deployment and distribution is essential. Swift developers must consider how to take their applications from a local environment to the end-users. Deployment refers to the procedures for preparing and uploading applications so they can run in production. Distribution is about making those applications accessible to users. Together, these components ensure that your work reaches its intended audience.
Effective deployment and distribution enhance application accessibility. For instance, while developing an iOS app in Swift, the primary avenue for distribution is the App Store. This path involves specific steps that demand careful attention. A successful release not only showcases your technical skills but also reflects professionalism.
App Store Submission Process
Submitting your app to the App Store can be daunting. This process consists of several critical steps that should not be overlooked.
- Preparing Your App: Ensure that the app meets Apple's guidelines and requirements. This includes functionality, user interface, and performance standards.
- Testing: Before submission, thorough testing is essential. Utilize Xcode's tools to test for bugs and ensure a seamless user experience.
- Creating an App Store Connect Account: You need this account to manage your app's submission. Once registered, you can navigate through the platform's interface.
- Filling Out Metadata: This includes providing app details like title, description, and keywords. This information plays a key role in app discoverability.
- Submitting for Review: After fulfilling all requirements and ensuring your app meets all standards, submit it for Apple's review. Note that this review process can vary in duration. If the app gets rejected, you'll need to address the issues and resubmit accordingly.
In this phase, attention to detail is paramount. Ignoring guidelines can lead to rejection and delay. Therefore, familiarize yourself with the latest App Store Review Guidelines to enhance your chances of approval.
Version Control with Git
Version control is an indispensable tool for any developer. Git, as a popular version control system, provides a way to track changes in your codebase, enable collaboration, and safeguard your work.
- Tracking Changes: Git allows you to record modifications to your Swift code. This feature helps you go back and review or restore previous versions if necessary.
- Collaborating with Others: When working in teams, Git makes it easier to collaborate. Multiple developers can work on different aspects of an app, merging changes seamlessly.
- Branching and Merging: You can create branches for new features or bug fixes without affecting the main codebase. Once a feature is stable, it can be merged back into the main branch.
- Remote Repository Management: Git integrates well with platforms like GitHub. Through these, you can host your code online, allowing for backup and collaboration.
Culmination and Resources
In any programming journey, the conclusion serves as a pivotal junction, synthesizing experiences and knowledge acquired. This section addresses the importance of wrapping up your learning in Swift and highlights key resources that can sustain and extend this educational journey.
The Importance of Concluding Your Learning
A well-articulated conclusion reinforces the concepts learned. Swift is an evolving language, and recognizing its dynamics is crucial. By summarizing your insights, you solidify your understanding and prepare yourself for more complex tasks. Consider the following:
- Reflection: Taking time to reflect on what you have learned helps reinforce knowledge. List the main topics covered, such as Swift syntax, object-oriented principles, and advanced features.
- Assessment: Evaluate your skills against real-world applications. Are you ready to implement what you have learned? Identify gaps by testing yourself or taking on small projects.
- Future Learning: In the tech world, learning never stops. Conclude with a plan for continuous development. Prioritize new features released by Apple and emerging best practices within the Swift community.
Continuing Education in Swift
Swift, as a language, is continually evolving. Staying updated is essential. Engage in ongoing education to keep your knowledge relevant. Here are some avenues:
- Online Forums: Join communities on platforms like Reddit to discuss recent changes and share insights. Participate in conversations and contribute where you can.
- Workshops and Meetups: Look for local or online events. These opportunities to meet fellow developers are invaluable for networking and learning.
- Documentation: Regularly consult the official Swift documentation. It is a comprehensive resource that offers up-to-date information on the language.
Recommended Books and Online Courses
Invest in high-quality learning materials for deeper understanding. Here are some noteworthy recommendations:
- Books:
- Online Courses:
- "Swift Programming: The Big Nerd Ranch Guide" offers a practical approach to learning Swift, with hands-on exercises.
- "The Swift Programming Language" by Apple is an excellent reference and learning resource from the creators of Swift.
- Website like Coursera provides courses tailored for all skill levels. Check out courses focused on iOS development using Swift.
- Udacity offers a nanodegree in iOS development that incorporates Swift.
Always choose resources that resonate with your learning style.
"Staying engaged in learning is the key to mastering Swift and using it effectively in applications."