Mastering Swift Programming: An In-Depth Guide
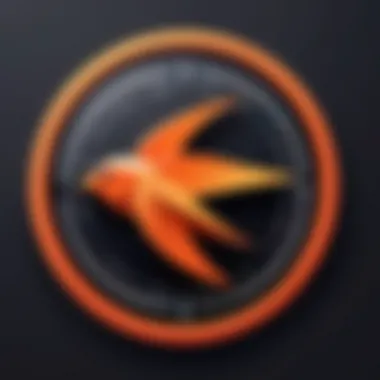
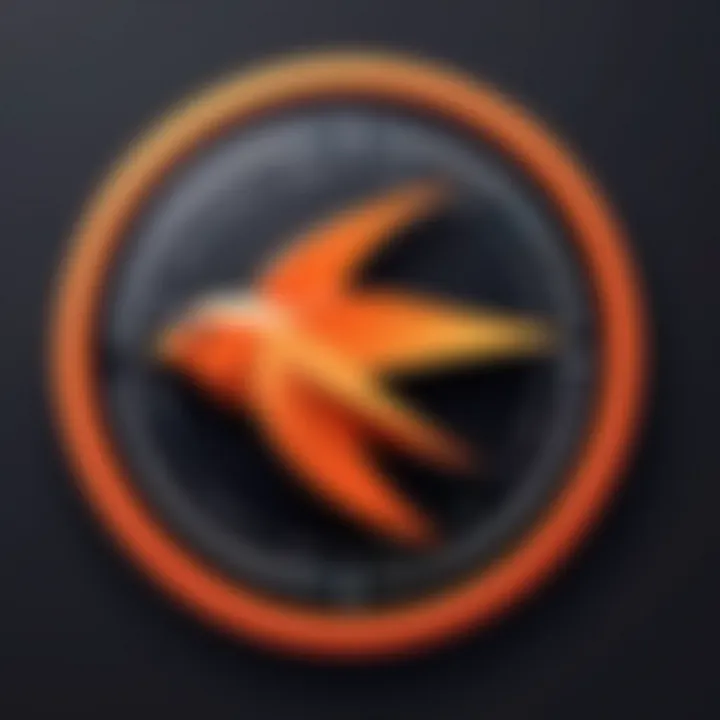
Overview of Topic
Swift programming has emerged as a powerful tool for developers, revolutionizing the landscape of software development. Its design is not merely a product of theoretical abstraction; its practical use in creating performant applications cannot be understated. Swift was introduced by Apple in 2014, targeting both iOS and macOS development, and since then, it has gained immense popularity among developers worldwide.
The scope of Swift extends beyond basic syntax. It incorporates modern programming paradigms and features, while its significance in the tech industry continues to grow. The need for high-quality apps that perform seamlessly on Apple devices drives the need for proficiency in this language.
Historically, Swift replaces Objective-C, which had held sway for a considerable period. As technology evolved, the limitations of Objective-C became apparent, leading to the development of Swift—aiming to enhance coder productivity and reduce application development times.
Fundamentals Explained
Understanding Swift begins with its core principles. These principles include safety, performance, and expressiveness. Swift aims to make code safer through optionals and type inference. By eliminating entire classes of common programming errors, it creates dependable environments for developers.
Key terminology in Swift includes:
- Optionals: Handle the absence of value, preventing runtime crashes.
- Closures: Self-contained blocks of functionality, akin to lambdas in other languages.
- Protocols: A blueprint of methods, properties, and other requirements for tasks or functionalities.
A solid grasp of these foundational pieces is essential for anyone aspiring to become proficient in Swift. Developers must familiarize themselves with these concepts to produce meaningful code.
Practical Applications and Examples
In practical scenarios, Swift demonstrates its versatility across various projects. For instance, many applications on the App Store owe their existence to Swift's ability to optimize performance while keeping code clean and understandable.
Sample project could involve creating a simple Todo app. The implementation can be done using Swift's UIKit framework for the interface design. Here is an example code snippet to illustrate how data can be managed:
The demonstrations of how Swift interacts with different frameworks expand its applicability. Hands-on projects like this can solidify understanding and showcase value in a tangible way.
Advanced Topics and Latest Trends
As with any technology, Swift continually evolves. The introduction of concurrency support in Swift 5.5 is a significant advancement. This feature allows for more efficient task management through structured concurrency.
Advanced methodologies now include utilizing SwiftUI for declarative UI programming. This trend emphasizes building interfaces with intuitive code, which is timely in today’s development landscape.
Looking forward, the Swift community is emphasizing more Swift server-side functionality as businesses increasingly look for solutions to build web applications efficiently in Swift. This trend hints at a bright future for language versatility in software development.
Tips and Resources for Further Learning
To enhance your knowledge and skills in Swift, consider exploring these resources:
- Books: "Swift Programming: The Big Nerd Ranch Guide” and “iOS Programming: The Big Nerd Ranch Guide" offer in-depth coverage.
- Online Courses: Platforms such as Coursera offer structured learning paths.
- Tools: Xcode is an essential tool for Swift development. It integrates code editing, debugging, and performance analysis seamlessly.
Additionally, engaging with community forums, like Reddit, can provide insight and practical advice from experienced developers. Collaboration can often lead to new ideas and solutions.
Learning Swift programming is not merely about syntax; it encapsulates a broader understanding of software development dynamics. As you take strides into this realm, remember that a solid foundation will always set the stage for advanced concepts and continuous improvement.
Preface to Swift Programming
Swift programming language emerges as a vital topic in the current tech landscape. The increasing demand for high-performance applications fuels its popularity among developers. Understanding Swift is essential for those who wish to develop applications for Apple's ecosystem, which includes iOS, macOS, watchOS, and tvOS. Additionally, Swift helps programmers write code that is safer and more efficient compared to many older languages. This efficiency can significantly enhance the development process, resulting in faster, more reliable applications.
History of Swift
Swift was introduced by Apple in 2014 as a modern programming language that prioritizes performance and safety. The development was led by Chris Lattner, who aimed to create a language that was easy to use and powerful enough for professional developers. Swift was designed to replace Objective-C, making it possible for developers to write code that is concise yet clear.
Since its introduction, Swift has rapidly evolved. Each new version brings enhanced features, improvements, and optimizations. With a strong emphasis on open-source development, the Swift community expands continuously, encouraging contributions from developers worldwide. The language itself is a synthesis of the best aspects of various programming languages, promoting an intuitive syntax that is accessible to newcomers.
Why Choose Swift?
Choosing Swift presents multiple benefits for developers:
- Safety: Swift incorporates type safety, which reduces runtime errors. This feature provides developers with greater tools for error detection early in the development process.
- Performance: Swift is designed for speed. It is compiled and optimized to leverage hardware, making applications run smoothly.
- Interactivity: Swift's Playgrounds provide an interactive coding environment that enhances learning and experimentation.
- Modern Syntax: The syntax is clean and modern, making it easier to read and write. This aspect appeals to both beginners and experienced programmers.
- Support and Community: Swift has a robust community and extensive resources, making it easier for learners to find help and share knowledge.
Ultimately, Swift provides a compelling option for developers looking to build modern applications across Apple's platforms. Its combination of safety, performance, and ease of use makes it a strong contender in the programming language arena, and understanding its core principles is essential for success.
Core Features of Swift
Swift programming language is designed with powerful and efficient features that stand at the core of its usage. Understanding these features is crucial for anyone looking to master Swift. They not only contribute to the language's overall functionality but also ensure that code is safer and easier to maintain. With a focus on performance and security, Swift brings several notable advantages to the programming landscape.
Type Safety
Type safety in Swift prevents many common errors by ensuring that type checks are performed at compile time. When you declare a variable, the compiler knows what type it is, and it checks type compatibility wherever that variable is used. This pro-active approach can significantly reduce runtime errors. For instance, if you try to assign a string to a variable intended for integers, Swift will generate a compile-time error, leading to less ambiguous code.
In addition, Swift’s type inference allows developers to write cleaner code without declaring types explicitly every time. The compiler can often infer the type from the context. This capability streamlines code while maintaining robustness. In complex applications, using type-safe features leads to better readability and a lower chance of bugs.
Optionals
One of Swift's unique features is optionals, which represent a variable that can have a value or can be . This concept addresses the common issue of null pointer exceptions seen in other programming languages. When a variable is defined as an optional, it explicitly tells the developer that the variable might not hold a value.
Optionals come into play especially during interactions with APIs, where data availability can be uncertain. To work with optionals, developers use optional binding or optional chaining. This not only makes the code safer but also more expressive. The developer is forced to handle the possibility of absence before proceeding, which ultimately improves code quality. By managing optional values properly, developers can create more resilient applications.
Memory Management
Swift employs Automatic Reference Counting (ARC) to manage memory, which efficiently tracks and manages the app’s memory usage. This ensures that the memory allocated to objects is freed when they are no longer needed, thus preventing memory leaks. Unlike manual memory management, ARC automates the process, allowing developers to focus on coding rather than dealing with complex memory allocation issues.
However, with ARC, it's crucial to be aware of strong and weak references. Having strong references between objects can lead to retain cycles, where two objects hold references to each other, preventing them from being freed. By using weak references appropriately, developers can counteract this problem and maintain a clean memory management system.
"Swift's architecture is designed to be fast, modern, and safe, allowing developers to create robust applications."
Understanding and leveraging these features are essential for anyone serious about programming in Swift.
Basic Syntax in Swift
Understanding the basic syntax in Swift is crucial for anyone venturing into programming with this language. This foundation enables developers to write clean, efficient, and maintainable code. Fluent command over syntax can lead to fewer errors and quicker debugging, ultimately leading to more successful project outcomes. Key components of Swift syntax include variables, constants, data types, and control flow statements. Together, they empower developers to create complex functionalities with relative ease.
Variables and Constants
In Swift, variables and constants represent fundamental building blocks for any program. Variables are used for storing values that can change during program execution, while constants hold values that remain unchanged. Keeping this distinction clear helps in managing data effectively.
To declare a variable, one can use the keyword:
Conversely, constants use the keyword:
The choice between variables and constants affects memory allocation and program reliability. It is typically a good practice to use constants whenever possible. This approach not only expresses intent clearly but also enhances performance due to less memory overhead.
Data Types
Swift supports a variety of data types which are essential for defining the kind of data stored in variables and constants. Some primary data types include:
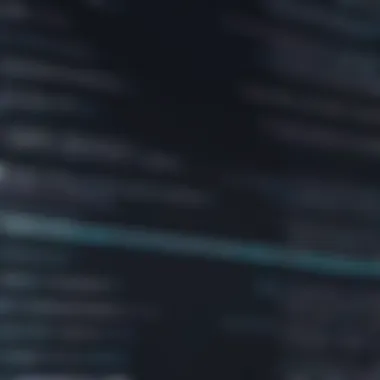
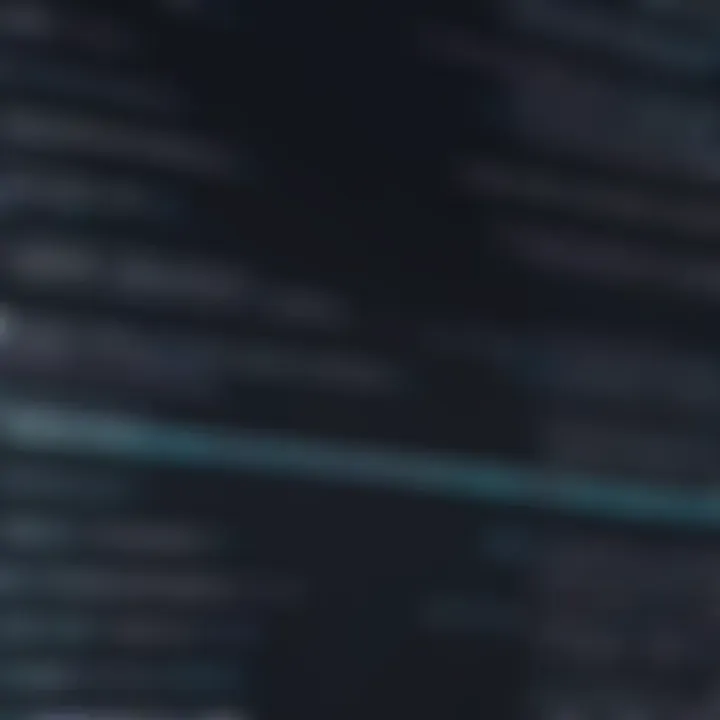
- Int: Represents integer values.
- Double: Represents floating-point numbers with precision.
- String: Represents sequences of characters.
- Bool: Represents Boolean values (true or false).
Understanding the appropriate data type for different situations allows for better memory usage and enhances code performance. Swift offers type inference, enabling developers to omit explicit type declarations when the compiler can infer the type from the assigned value.
Control Flow Statements
Control flow statements dictate the execution path of code, allowing for logic and decision-making in programs. In Swift, these are primarily categorized into conditionals and loops.
Conditionals include:
- statements
- statements
For example, an statement can look like this:
Loops allow for repetitive execution of code. Common looping structures are:
- loops
- loops
A loop example:
Control flow statements not only enhance functionality but also provide the means to handle complex logic in applications. Mastery of these components is essential for writing efficient Swift programs.
Advanced Features
The advanced features in Swift programming are essential for developers looking to maximize efficiency and control in their code. Understanding these features allows programmers to build more robust applications while maintaining clarity and performance. Closures, protocols, and generics are three critical components that not only enhance the functionality of Swift but also facilitate building scalable and maintainable software.
Closures
Closures in Swift serve as a self-contained block of functionality that can be passed around and used in your code. They are similar to functions but with the added flexibility of being anonymous. Closures can capture and store references to variables and constants from their surrounding context. This ability makes them particularly useful in scenarios where callbacks are needed, such as in asynchronous programming.
Some practical applications of closures include:
- Completion Handlers: Used to execute a block of code after an operation completes, essential in network requests.
- Functional Programming: Closures facilitate functional programming techniques, such as map, filter, and reduce, allowing for more concise code.
The syntax of a closure can be less verbose than a function, which is highly advantageous for readability.
Protocols
Protocols in Swift are a way to define a blueprint for methods, properties, and other requirements that suit a particular task or functionality. They provide a powerful mechanism for enabling multiple, distinct classes to implement shared functionality. This attribute of protocols promotes a clean and organized design.
Key benefits of using protocols include:
- Decoupling: They help to decouple code by allowing different classes to implement methods without a strict inheritance structure.
- Flexibility: They allow you to define a set of functionalities that different classes can adopt, enhancing code reuse and flexibility.
- Protocol-oriented programming: Swift encourages a protocol-oriented approach, pushing developers to write code with protocols rather than relying solely on class inheritance.
Protocols can also be extended to provide default implementations, further enhancing their utility.
Generics
Generics enable developers to write flexible and reusable code that can work with any type. This feature allows you to create functions and data types that can operate on different data types without sacrificing type safety. By using generics, developers can avoid code duplication and increase algorithm efficiency.
The key aspects of generics include:
- Type Parameters: You can define a function or type using type parameters, making them adaptable. For example, a function that sorts an array can be written once with generics instead of separately for each type of array.
- Improved Performance: With generics, the compiler can optimize code more efficiently by avoiding type casting, which may slow down performance.
- Safe Code: Generics maintain type safety, ensuring that the types align, which can reduce runtime errors.
By understanding these advanced features, developers can leverage the full potential of Swift, resulting in cleaner, more maintainable, and efficient code.
"Advanced features empower developers to create code that is not only powerful but also elegant."
Working with Object-Oriented Programming
Object-oriented programming, or OOP, forms a cornerstone of Swift’s design philosophy. It allows developers to organize code in a manner that mirrors real-world entities and their interactions. This structure fosters code reuse, scalability, and easier maintenance. In the context of Swift, OOP enables developers to build applications that are not only powerful but also elegantly structured.
Benefits of Object-Oriented Programming
- Encapsulation: This concept allows the bundling of data and methods that operate on that data within classes. It keeps the internal workings of a class hidden from the outside world, providing a clear interface for interaction.
- Abstraction: OOP allows developers to handle complexity by exposing only the relevant aspects of classes. This makes it simpler to manage large codebases by focusing on high-level interactions.
- Inheritance: Inheritance facilitates the creation of new classes that are based on existing ones, allowing for shared functionality and reducing the need for redundant code.
- Polymorphism: This allows methods to do different things based on the object it is acting upon, giving flexibility and extending the behavior of classes.
Understanding the principles of OOP is vital for effective Swift programming. By adopting these principles, developers can write clearer, more efficient code that is easier to navigate.
Classes and Structures
In Swift, both classes and structures are building blocks of OOP. They define blueprints for creating objects. While both can contain properties and methods, there are fundamental differences between them. Classes can inherit from other classes, while structures cannot. This means if you need to model a hierarchy or share behavior, classes are the preferred choice.
Classes are reference types, meaning when you assign a class instance to a variable, you are assigning a reference to that instance, not a copy. Changes made through one reference affect all other references. Structures, in contrast, are value types. Assigning a structure to a variable creates a new copy. This distinction is crucial for memory management and application behavior.
Quick Example of Classes
Inheritance
Inheritance in Swift allows a new class to inherit the properties and methods of an existing class, known as the superclass. This enables a hierarchy of classes, where derived classes can build on the behaviors of their parent classes. By using inheritance, Swift programmers can create a well-structured and reusable codebase.
For instance, consider a class called that inherits from . The class can have its own unique properties, such as the number of gears, while also accessing and extending the functionality of .
Code Snippet Illustrating Inheritance
In this example, inherits the functionality of but adds its own unique characteristics. The keyword indicates that we are providing a new implementation of the method.
Inheritance supports code mightiness and facilitates the management of large applications by reducing redundancy.
Error Handling in Swift
Error handling is crucial in Swift programming. It involves the aability to manage unexpected conditions that occur during runtime. This capability ensures that applications do not crash and can handle issues gracefully. In Swift, the framework provides various mechanisms to identify and manage errors effectively. Understanding error handling is essential for writing robust, maintainable, and user-friendly code.
There are several benefits to implementing error handling in your Swift code:
- Improved Stability: By gracefully managing errors, you prevent your app from crashing.
- Enhanced User Experience: Users receive informative messages rather than facing unexpected crashes.
- Simplified Debugging: Proper error handling allows developers to trace issues more efficiently.
Considerations when implementing error handling include understanding which types of errors to anticipate and how to respond to them. Training yourself to think about potential errors during the design phase of your application can save a lot of trouble later.
Using Try-Catch
Swift uses the mechanism to handle errors. In this approach, code that can produce an error is marked with the keyword. If an error occurs, it will be caught by a block. This separation makes it clear where the error might occur and facilitates handling it appropriately.
Here’s a simple example:
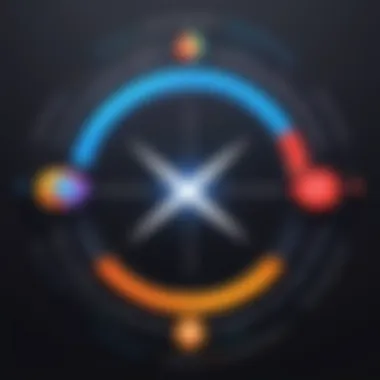
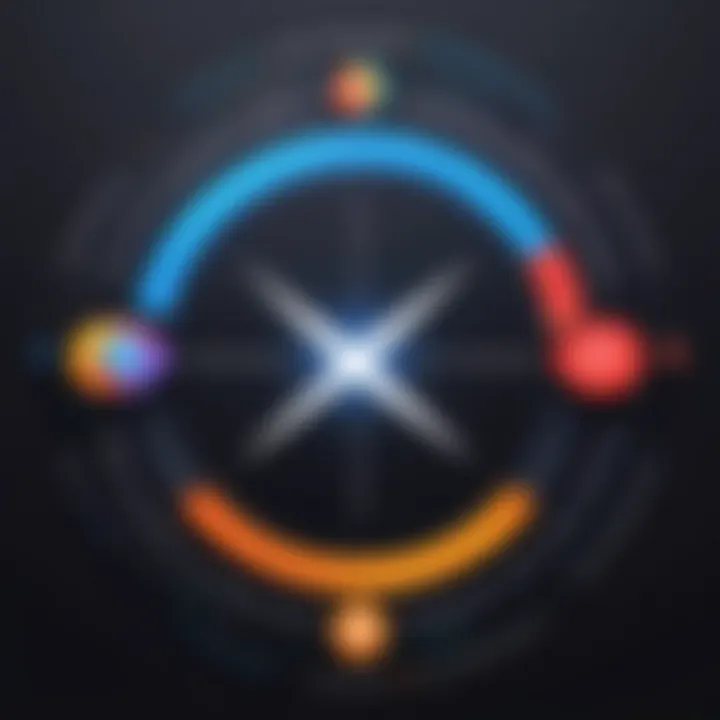
In this example, if throws an error, the program will not crash. Instead, it will handle the error in the block, allowing the program to continue running.
Throwing Errors
Throwing errors in Swift is straightforward. You define a function with the keyword, indicating that it may throw an error at runtime. This is part of Swift's method of making error handling explicit.
Creating custom error types can improve clarity and maintainability. You can define your own error enum to represent different error conditions. Follow the example below:
By using the keyword and creating your own error type, you enhance the capability of your Swift program to handle errors that may arise. Overall, proper error handling is an integral part of developing robust applications, ensuring a smoother development process and a better user experience.
Swift Programming Best Practices
Best practices in Swift programming are essential for creating maintainable, efficient, and error-free code. By adhering to these practices, programmers can enhance their productivity while minimizing bugs and issues. This section discusses the significance of such practices, focusing on code readability and testing/debugging methods.
Code Readability
Code readability is a crucial element in programming that directly impacts both individual developers and teams. Clear and concise code helps others understand the logic and structure quickly, facilitating collaboration and reduces the learning curve for new team members. In Swift, several strategies can be utilized to improve readability:
- Meaningful Names: Always use descriptive names for variables, functions, and classes. Avoid abbreviations that might confuse others.
- Consistent Formatting: Stick to a consistent coding style. This involves proper indentation, spacing, and alignment of code blocks. Swift uses the convention of four spaces for indentation.
- Comment Wisely: Use comments to explain complex or non-obvious parts of your code. However, avoid oversaturating code with comments, as this can clutter the readability.
- Limit Line Length: Ideally, keep lines under 80 characters. Longer lines can be difficult to read and understand.
Following these guidelines makes the code self-explanatory, reducing the time spent trying to figure it out. As a result, code reviews become more efficient and effective, leading to better software overall.
Testing and Debugging
Testing and debugging are vital parts of software development that ensure the code performs as intended and is free of errors. Swift offers robust tools and methodologies to aid in these processes. Key practices include:
- Unit Testing: Use unit tests to verify that individual components of your code behave as expected. XCTest is a framework provided by Apple for writing and running unit tests in Swift.
- Test-driven Development (TDD): Adopting TDD helps developers write code based on the tests they create first. This leads to better design decisions and reduces the amount of rework required.
- Debugging Tools: Xcode, Swift’s integrated development environment, includes powerful debugging tools that allow developers to set breakpoints, step through code, and inspect variables. Learn how to use these tools effectively.
- Error Reporting: Swift's error handling using , , and keywords helps in managing and responding to errors gracefully. Implementing a clear error handling strategy can prevent unexpected crashes.
By emphasizing testing and debugging, developers can catch bugs early in the development process, ultimately saving time and ensuring high-quality software.
In summary, prioritizing code readability and robust testing/debugging practices can significantly elevate the quality of Swift applications. By following these best practices, programmers can create effective solutions that are easier to maintain and enhance over time.
For more on best practices in coding, you can check the documentation at en.wikipedia.org.
Interoperability with Objective-C
Interoperability with Objective-C is a significant aspect of Swift programming as it allows developers to leverage existing code and libraries. This synergy is particularly relevant for mobile application development on Apple platforms, where many applications may have been initially written in Objective-C. Swift’s ability to interoperate with Objective-C eases the transition for developers familiar with the older language while still enabling the use of modern features.
Using Objective-C in Swift Projects
When integrating Objective-C into Swift projects, developers can include Objective-C classes directly within their Swift code. This can be achieved through the use of a bridging header. A bridging header file acts as a bridge between Objective-C and Swift, allowing Swift code to recognize and use Objective-C classes, constants, and functions.
- Create the Bridging Header: When you create a new Swift project in Xcode, it typically asks if you want to create a bridging header. If you select yes, Xcode generates a header file for you. This file will contain import statements for your Objective-C files.
- Import Objective-C Classes: In your bridging header, you need to import any Objective-C headers that you want to use in Swift. For example, if you have a class named , you will add this line to your bridging header:
- Accessing Objective-C Features in Swift: Once imported, you can create instances of Objective-C classes and call their methods directly in Swift code. This feature allows developers to take advantage of the vast libraries developed in Objective-C without needing to rewrite them in Swift.
Benefits and Challenges
The interoperability between Swift and Objective-C presents both benefits and challenges.
Benefits:
- Code Reusability: Developers can reuse existing Objective-C code, saving time and reducing redundant work.
- Fast Transition: It allows teams to transition gradually from Objective-C to Swift. Developers can incrementally refactor and modernize their codebase.
- Rich Ecosystem: Many libraries and frameworks are available in Objective-C, providing a wealth of resources that can be easily integrated into Swift applications.
Challenges:
- Compatibility Issues: Different type systems can cause compatibility problems. Developers need to be careful about how data is transferred between Swift and Objective-C, particularly with Swift’s optionals.
- Learning Curve: For those new to Swift or Objective-C, understanding the nuances of interoperability may pose a challenge.
- Runtime Overhead: Calling Objective-C methods from Swift can introduce some performance overhead compared to pure Swift calls.
"While interoperability can offer a significant advantage, it is crucial to understand both languages well to manage the complexities effectively."
Swift Development Environment
The Swift development environment plays a crucial role in the programming experience. It serves as the foundation for building applications using the Swift language. If the environment is not set up correctly, it can lead to frustrations that hinder one’s ability to write and test code effectively. Thus, understanding the development environment is essential for anyone looking to master Swift programming.
One of the primary components of the Swift development environment is Xcode. This integrated development environment (IDE) is developed by Apple. It includes a code editor, debugger, and interface builder, all in one package. Using Xcode enhances productivity by providing tools that support writing, testing, and debugging code seamlessly. This efficiency is invaluable, especially for newcomers who might struggle with configuring different tools individually.
Additional benefits of a well-organized development environment include:
- Immediate Feedback: With built-in testing functionalities, developers can run code snippets and receive feedback instantly.
- Code Suggestions: The IDE offers code completion suggestions that help users write correct syntax without having to memorize everything.
- Visual Interfaces: Xcode allows developers to design user interfaces visually, making the process more intuitive.
However, it is also important to consider some challenges associated with the Swift development environment. Compatibility with older software versions can sometimes cause issues, as Apple frequently updates its tools. Additionally, newcomers may experience a steep learning curve regarding all features of Xcode.
"A well-prepared development environment saves time and enhances learning, especially for those new to programming."
Having established the significance of the Swift development environment, the next step is properly setting up Xcode.
Xcode Setup
Setting up Xcode is straightforward, but attention to detail is key. To begin, you need a Mac computer, as Xcode is available exclusively on macOS. Here’s how to set it up:
- Download Xcode: Open the Mac App Store and search for Xcode. Click the download button to install it on your machine.
- Install Xcode: Once downloaded, the installation process will start automatically. Simply follow the on-screen prompts until it completes.
- Launch Xcode: Open Xcode from your applications folder. It may take a few moments to launch for the first time.
- Agree to Terms: Upon the first launch, you will likely be prompted to agree to the license terms provided by Apple.
- Test Functionality: Create a simple project to make sure everything is working correctly. This step ensures that no issues have cropped up during installation.
These steps should result in a fully configured development environment ready for coding.
Hello World Example
After successfully setting up Xcode, it’s time to write your first Swift program: a simple "Hello World" example. This will help reinforce your understanding of the basic syntax and functionality of Swift. To create this example:
- Open Xcode and select "Create a new Xcode project".
- Choose macOS as the platform and select the Command Line Tool template.
- Name your project, for instance, "HelloWorld", and make sure the language selected is Swift.
- Click on Next and specify a location to save your project.
- Once the project is created, navigate to the automatically generated file.
- Replace any existing code with the following line:
- Run the Program: Click on the play button in the Xcode toolbar or press Command + R on your keyboard.
You should now see the output "Hello, World!" in the console at the bottom of the Xcode window. This simple exercise demonstrates the fundamental approach to programming in Swift. It prepares you for more complex structures and algorithms to explore as you continue your programming journey.
The development environment, particularly through Xcode, is crucial for efficient coding. Familiarity with it enables better programming outcomes and a deeper understanding of Swift.
Applications of Swift Programming
Understanding the applications of Swift programming is critical for grasping its significance in today's tech ecosystem. Swift has emerged as a robust programming language widely used in various domains. This section explores the primary applications of Swift, specifically how it revolutionizes both iOS app development and server-side programming.
iOS App Development
Swift plays a pivotal role in iOS app development, becoming the preferred language for developers in the Apple ecosystem. Since its inception, Swift has been designed to optimize the performance and safety of applications. The following elements highlight why Swift is essential for iOS app development:
- Performance: Swift is compiled to native code which ensures high performance for applications. Developers find that apps written in Swift are not only faster but also more efficient in resource usage. This is crucial for mobile platforms where performance can directly impact user experience.
- User Interface (UI): Swift’s syntax allows developers to create expressive and concise UI code. It integrates seamlessly with Apple’s frameworks, such as UIKit and SwiftUI, enabling the creation of visually stunning applications. The high level of abstraction in Swift facilitates quick prototyping and iterating, which is essential in today's fast-paced development environments.
- Safety Features: Swift's type safety mitigates common programming errors. This feature reduces bugs that mostly stem from type mismatch, thus ensuring a smoother user experience in apps. Best practices encourage the use of optionals to handle the absence of a value. These safeguards contribute to the reliability of production applications.
- Rich Developer Ecosystem: The thriving community of Swift developers fosters collaboration and knowledge sharing. Many open-source resources and libraries are available, allowing new developers to find tools and frameworks that enhance their productivity. In addition, platforms like GitHub provide a wealth of Swift-based projects for study and integration into personal projects.
Server-Side Swift
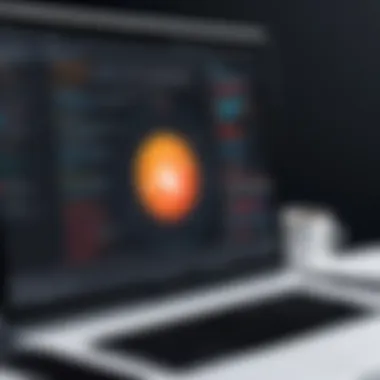
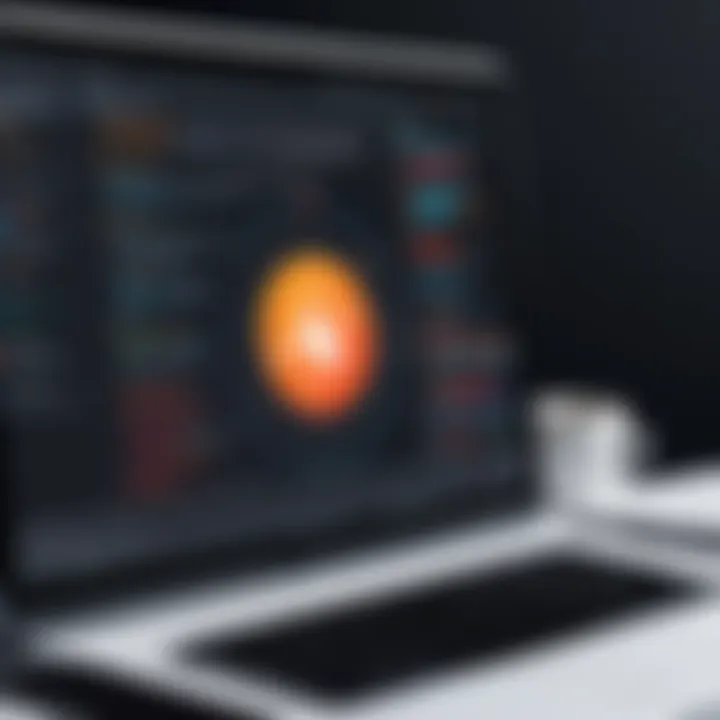
While Swift is popularly known for iOS app development, it has significantly made inroads into server-side programming. The framework SwiftNIO, for example, provides an asynchronous event-driven architecture for building web applications. Here are some considerations for server-side Swift:
- Performance and Scalability: As a compiled language, Swift offers superior performance compared to traditionally interpreted languages like Ruby or Python. This performance attribute is vital when handling large volumes of requests. Server-side applications written in Swift can efficiently manage resources, handling peaks in traffic without sacrificing response times.
- Unified Codebase: Leveraging Swift for both client-side and server-side development promotes a cleaner and more cohesive codebase. Developers can share libraries and logic between the client and server, reducing redundancy and increasing maintainability. This unique feature makes Swift an appealing choice for full-stack development.
- Growing Frameworks: The ecosystem of frameworks for server-side Swift is expanding, with options such as Vapor and Perfect. These frameworks provide robust tools required for building web applications. They offer functionalities for routing, middleware, and even database integration, allowing developers to create functional applications quickly and efficiently.
- Interoperability: With Swift's ability to work alongside other languages, organizations can gradually transition to Swift without needing to rewrite entire codebases. This interoperability allows teams to innovate while leveraging existing technologies.
Exploring Swift Package Manager
The Swift Package Manager (SPM) is a powerful tool that plays an essential role in the Swift programming ecosystem. As projects grow in complexity, managing dependencies can quickly become a challenging task. The Swift Package Manager provides a structured and efficient way to handle libraries and frameworks, ensuring that developers can focus on coding rather than the intricacies of their project's dependencies. By simplifying the process of adding, updating, and removing packages, SPM enhances the overall development experience.
Setting Up Packages
Setting up packages with the Swift Package Manager is a straightforward process. Understanding how to create and configure a package is crucial for any Swift developer. Here’s a step-by-step guide on how to get started:
- Create a Package Directory: Start by creating a new directory for your package.
- Initialize the Package: Run command in the terminal. This command sets up the package structure automatically.
- Modify : This file contains the package configuration. You can specify dependencies, targets, and other settings here.
- Add Source Files: Create a new folder called in your package directory where your Swift files will reside.
- Build Your Package: Use to compile your package.
With these steps, you will have a basic package set up and ready for development. The SPM takes care of the heavy lifting, simplifying the integration process.
Dependency Management
Dependency management is a critical aspect of software development, and SPM excels at this. It enables developers to define their project's dependencies easily, ensuring compatibility and resolving version conflicts. Here are some key points regarding dependency management with SPM:
- Adding Dependencies: You can add dependencies directly in the file by specifying the package name, repository URL, and branch or version.
- Versioning: SPM uses semantic versioning. You can specify the minimum and maximum version of a dependency to make sure your project works with compatible updates.
- Fetching Dependencies: The command fetches new versions of dependencies based on the rules you've specified in your file.
- Performance Optimization: SPM caches dependencies locally, speeding up the build process.
"The right dependency manager can drastically reduce complexities in software development."
In summary, the Swift Package Manager offers a robust solution for managing project dependencies. By following the appropriate steps to set up packages and understanding how to manage dependencies effectively, developers can streamline their workflow and minimize potential issues. Mastery of SPM is essential for anyone looking to excel in Swift programming.
Swift Coding Challenges
Coding challenges are an integral part of learning any programming language. They serve both as a means of testing one's knowledge and a way to apply theoretical concepts practically. In Swift programming, coding challenges present unique benefits and considerations. They help programmers fine-tune their skills while also encouraging critical thinking. By tackling various problems, beginners can gain confidence and a deeper understanding of the Swift language.
Engaging with coding challenges allows learners to discover the power of Swift's features, such as optionals, closures, and protocols. Moreover, these challenges often simulate real-world scenarios that developers face when building applications. Such practical exposure is invaluable.
Common Problems and Solutions
When engaging with Swift coding challenges, several common issues may emerge. Here are a few:
- Understanding Compiler Errors: New learners may find themselves stuck on compiler errors. These often arise due to syntax issues or type mismatches. Solution: Take time to read error messages carefully. They often provide hints.
- Managing Optionals: Swift's optional types can confuse newcomers. Not knowing how to unwrap an optional correctly can lead to runtime crashes. Solution: Understand the various unwrapping techniques, such as using or .
- Logic Errors: These occur when the program runs without crashing, but the output is not as expected. Solution: Debugging tools in Xcode can be tremendously helpful here. Step through your code to see where the logic diverges from your expectations.
- Performance Issues: Sometimes, code may work but run inefficiently. This can be especially true in cases involving large data processing. Solution: Learn about Swift's data structures and algorithms to optimize your code.
Coding challenges often include sample solutions. Analyzing these solutions can provide insights into better coding practices.
Resources for Practice
To improve skills through coding challenges, various resources are available.
- LeetCode: This platform offers a variety of coding problems, including those specifically in Swift. It is widely used by software developers for practice and interview preparation.
- HackerRank: Another excellent site for coding challenges in diverse domains, including Swift programming.
- Exercism.io: This site provides mentor-supported learning for Swift, helping coders at all levels.
- Codecademy: They offer practical courses in Swift that include multiple coding challenges to test knowledge.
- Books and Documentation: Reading documentation on Swift's official site and supplemental texts can provide context for the challenges faced. Additionally, community forums on platforms like Reddit and Stack Overflow can offer valuable advice and shared experiences.
Learning Swift through coding challenges is a strategy that can solidify understanding and enhance proficiency. Each problem solved is a step closer to mastery.
Future of Swift Programming
The future of Swift programming is a topic of significant interest and relevance in today’s fast-paced tech landscape. As the demand for high-performance applications rises, Swift's robust features and developer-friendly environment position it to play a crucial role in software development. Understanding the evolving paradigms, opportunities, and challenges associated with Swift is vital for anyone invested in programming. This section explores emerging trends in Swift and looks into the community and ecosystem that supports it.
Emerging Trends
The landscape of software development is continually changing, and Swift is no exception. As mobile app development thrives, Swift has gained popularity due to its seamless integration with iOS and macOS applications. One trend is the inclusion of Swift in server-side development. This shift allows developers to leverage Swift's performance and safety for backend services, broadening its usability beyond just mobile platforms.
Another trend is the growing focus on Swift's interoperability with other programming languages. With frameworks like Swift for TensorFlow, Swift is stepping into the realm of machine learning, enhancing its appeal to data scientists and engineers. In addition, Swift's emphasis on safety and performance makes it a strong candidate for emerging technologies, like Internet of Things (IoT) applications.
Furthermore, the introduction of SwiftUI marks a pivotal change. This declarative framework simplifies the process of building user interfaces, fostering rapid development and prototype testing. SwiftUI’s growth reflects a larger movement toward simplifying code management and enhancing user experience. As these trends evolve, they showcase Swift's adaptability and potential for the future.
Community and Ecosystem
A strong community backs Swift, providing resources and support that are indispensable for developers. The Swift Forums are a hub for discussion, allowing developers to share insights, seek guidance, and collaborate on projects. This engagement fosters a culture of open communication and continuous improvement.
Additionally, the abundance of educational resources available online plays a crucial role in building the Swift ecosystem. Platforms like Reddit and tech blogs offer a treasure trove of information for beginners and experienced programmers alike. Books and online courses provide structured learning paths that are focused on practical application of Swift.
Moreover, the support from organizations like Apple ensures that Swift continues to evolve. With regular updates and community-driven initiatives, the language remains relevant. Developers can expect new features that improve usability and performance through active participation in the Swift ecosystem.
"Emerging technologies and the community's enthusiasm indicate a bright future for Swift programming in various domains."
Community and Resources
In the realm of Swift programming, community and resources play a pivotal role in shaping both the learning experience and practical application. For both novices and seasoned developers, being part of a vibrant community can enhance understanding and foster collaboration. This section will delve into the benefits of engaging with fellow learners and professionals, as well as the significance of utilizing various educational materials.
Online Forums and Groups
Online forums and groups serve as essential platforms for Swift developers to connect and share insights. These spaces allow programmers to ask questions, discuss challenges, and exchange knowledge. Popular platforms include Reddit and Stack Overflow, where users can post queries and receive responses from experienced developers. The exchange of ideas in these forums contributes to a larger pool of collective knowledge, enriching the Swift community.
Participation in Facebook groups dedicated to Swift programming can also be highly advantageous. These groups often host discussions on recent developments, coding tips, and project showcases. Members can benefit from the camaraderie of likeminded individuals while gaining exposure to diverse perspectives in problem-solving.
Moreover, active involvement in such communities can lead to networking opportunities. Building connections may foster collaborations on projects or mentorship arrangements that guide emerging developers along their coding journey. Engaging with the community also keeps individuals abreast of industry trends and updates, ensuring their skills stay relevant.
Books and Documentation
Books and well-structured documentation are indispensable for mastering Swift programming. Comprehensive references not only cover the syntax but also provide deeper insights into best practices and design patterns. Relying on established literature can significantly enhance a learner's expertise.
Titles like "The Swift Programming Language" by Apple are foundational texts that offer a thorough overview. This resource is an official guide that covers the fundamentals and advanced features of Swift. Additionally, books like "Swift for Beginners" provide practical examples that are easy to follow for those new to programming.
Further, Apple's own documentation serves as a reliable resource. It outlines the various elements of Swift, making it a go-to reference for developers at all levels.
Closure
The importance of community and resources in Swift programming cannot be overstated. Engaging in online forums and utilizing quality literature enriches the learning experience. By actively participating in these venues, developers not only enhance their skills but also contribute to the continuous growth of the Swift ecosystem. This collaborative approach is vital for maintaining a competitive edge in a rapidly evolving tech landscape.
Epilogue
In this article, we explored various facets of Swift programming. The conclusion serves as a crucial element to synthesize the knowledge gained. It highlights the relevance of Swift in the current programming landscape. Swift has emerged as a preferred choice for iOS development, garnering attention for its safety features and performance.
Swift's popularity is propelled by its accessibility for beginners, while still being powerful enough to meet enterprise-level demands. This adaptability makes it essential for learners to embrace. Moreover, this language encourages best practices such as code readability and reliable error handling, key aspects that every programmer should prioritize.
A comprehensive conclusion also includes consideration of the trends affecting Swift programming, including community contributions and continuous updates from Apple. This ensures that it remains timely and aligned with modern development practices.
The culmination of this article encourages a reflective mindset towards the coding practices discussed. Integrating these practices helps develop more robust applications and enhances one's ability in coding and software development. Leveraging the skills acquired through this guide contributes positively to career growth in the tech industry.
"Continuous learning and adaptation define the successful programmer."
Summary of Key Takeaways
- Swift is an evolving programming language tailored for safety and performance.
- The language supports best practices that can lead to efficient and maintainable code.
- Its utilization in both iOS app development and server-side applications showcases its versatility.
- Understanding the community and resources available enhances the learning experience for new programmers.
- Engaging with online platforms, like Reddit, provides opportunities for knowledge exchange and support.
Next Steps for Learners
Learners who wish to deepen their knowledge of Swift should consider the following steps:
- Practice consistently: Engage with coding challenges to solidify understanding.
- Explore online courses that focus on advanced Swift concepts.
- Participate in community forums, such as those on Reddit or programming groups on Facebook to discuss and solve problems collaboratively.
- Read authoritative books and documentation to get different perspectives on Swift programming.
- Start building projects: Apply concepts learned by creating your own applications, progressively increasing complexity.
These steps will enable learners to transition from beginner to proficient Swift programmers, ultimately enhancing their skill set and career opportunities.