Mastering While Loops in Python: Complete Overview
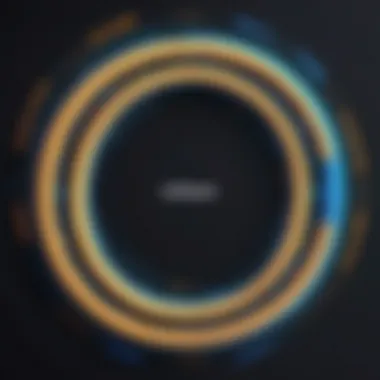
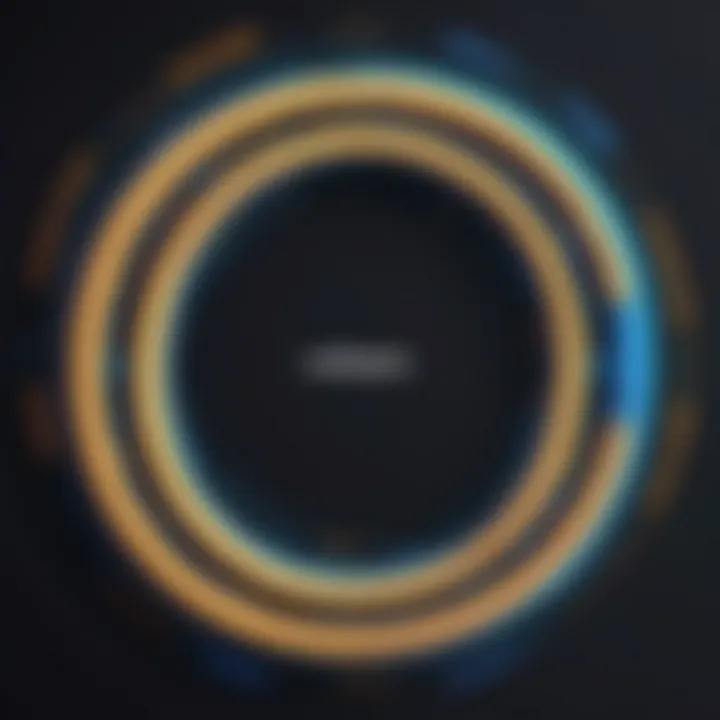
Overview of Topic
While loops are a fundamental feature of Python programming that allow for repeated execution of a block of code as long as a specified condition holds true. Understanding while loops is essential for any programmer, especially those seeking to enhance their control flow skills. In the tech industry, while loops have a significant role in automating processes, managing tasks that need repeated evaluation, and controlling the logic of programs thoroughly.
Historically, control flow mechanisms like while loops have evolved alongside programming languages. As languages matured, so did structures to facilitate efficient coding practices. Python, known for its readability and simplicity, has popularized the use of such loops, enabling both novice and experienced programmers to implement logical flows effectively.
Fundamentals Explained
When discussing while loops, several core principles are essential to grasp.
Core Principles:
- A while loop runs as long as its condition evaluates to true.
- Inside the loop, code can modify variables that participate in the loop's condition, leading eventually to false and terminating the loop.
Key Terminology:
- Condition: Expression evaluated before each iteration of the loop.
- Iteration: A single execution of the block within the while loop.
Basic Concept: The while loop begins with the keyword , followed by the condition. When the while loop is used effectively, it allows for significant control over program execution.
Practical Applications and Examples
In real-world scenarios, while loops find various applications. For instance, they can be utilized in processing user input until a valid response is fetched. Hereβs a simple demonstration of a while loop:
This code snippet demonstrates how to increment a count until it reaches five. As seen, the loop condition remains satisfied until count equals five.
While loops can also manage more complex tasks such as data validation, file processing, or even implementing algorithms like the Fibonacci sequence.
Advanced Topics and Latest Trends
Recently, the usage of while loops has expanded in data science and machine learning. For example, they're often employed in iterative algorithms where modeling often requires stepping through various data representations multiple times. Understanding the nuances of using while loops in these contexts requires more advanced techniques and sampling methodologies.
Future prospects indicate that as machine learning models become more sophisticated, the ability to efficiently harness loops will remain critical, particularly in handling large datasets and optimizing algorithms.
Tips and Resources for Further Learning
To strengthen your understanding of while loops, consider the following resources:
- Books: "Automate the Boring Stuff with Python" by Al Sweigart provides practical examples involving loops.
- Online Courses: Platforms like Coursera and edX offer courses in Python that include sections on control flow.
- Community Forums: Websites like Reddit have active programming communities ready to assist.
- Reference Sites: For in-depth theoretical understanding, consult sources like Wikipedia and Britannica.
Learning about while loops equips programmers with essential skills needed to effectively handle repetitive tasks, making it a core component of programming expertise.
Intro to Loops in Python
In the realm of programming, the utility of loops cannot be overstated. They provide a fundamental structure that allows for the repetition of code until a certain condition is met. This enhances efficiency and automation when working with data. Understanding loops is essential for anyone looking to master Python, as it forms the backbone of control flow.
Loops streamline the process of executing a block of code multiple times. They prevent redundancy in programming, making code more concise and maintainable. With loops, developers can write less and achieve more. Specifically, Python offers two primary types of loops: for loops and while loops, each serving unique purposes depending on the context.
When discussing loops, it is valuable to consider the scenarios in which they are most effective. For example, while loops shine in situations where the number of iterations is unknown upfront. Their flexibility allows programmers to accommodate varying inputs and conditions efficiently. In contrast, for loops are optimal for iterating over defined sequences, such as lists or ranges, providing structure when working with known quantities.
This guide aims to illuminate the importance of loops in Python programming, offering insights into their syntax, applications, and some pitfalls to avoid. By delving deeper into the characteristics of each loop type, the reader will gain a robust understanding of how to implement and control flow effectively in Python.
Understanding the While Loop
The while loop is a crucial construct in Python programming, playing a significant role in enabling iterative processes. Understanding how while loops function allows programmers to manage control flow effectively within their code. This section emphasizes the relevance of while loops, highlighting their flexibility and power in various programming scenarios. Through comprehension of this topic, readers can apply these loops to solve problems efficiently, making their code more dynamic.
Syntax of a While Loop
The syntax of a while loop in Python is straightforward. It consists of the keyword followed by a condition, a colon, and a block of code indented underneath. The structure can be visualized as follows:
In this syntax:
- The is a boolean expression. This means it can be either true or false.
- The block of code will execute repeatedly as long as the condition evaluates to true. Once the condition is false, the loop ends.
Knowing this syntax is essential: it forms the basis of how while loops operate in practice. By effectively using this structure, programmers can harness the full potential of the while loop.
Basic Structure of a While Loop
The basic structure of a while loop consists of three main components: initialization, condition checking, and updating. Each component plays an integral part in the loop's operation, ensuring it runs correctly and terminates as expected.
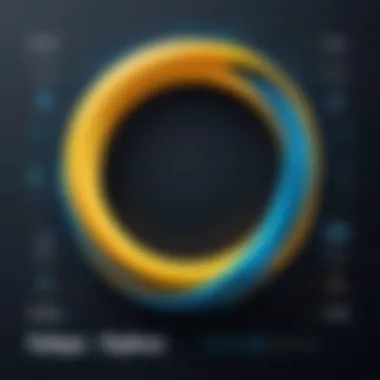
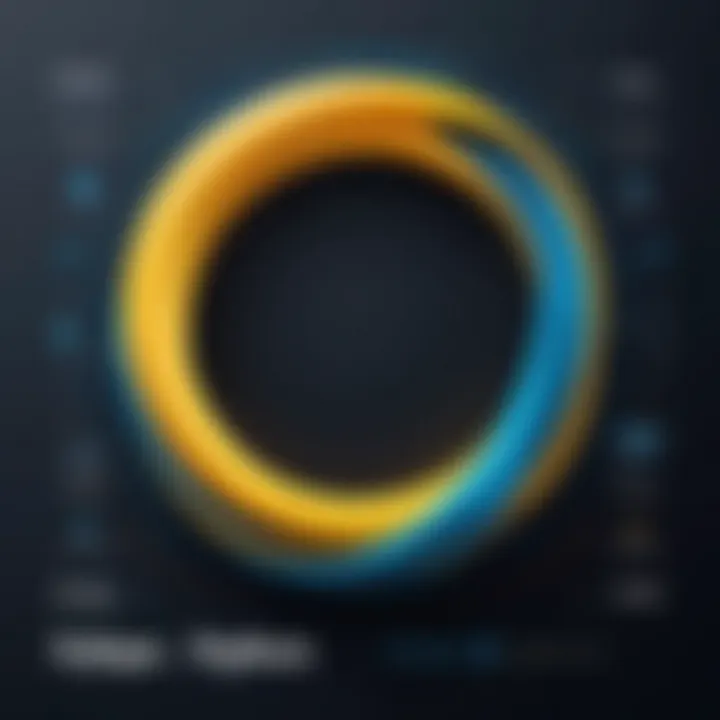
- Initialization: This step usually occurs before the loop. Here, you set the initial state of the variable that will be tested in the condition.
- Condition Checking: When the while loop starts, Python checks the specified condition. If the condition is true, the code in the block executes.
- Updating: To prevent infinite loops, it is vital to update the variable used in the condition within the loop, ensuring that it will eventually evaluate to false.
An exemplary while loop might look like this:
In this example, the loop initializes to five, checks if it is less than five, prints the current value, and increments it. This cycle continues until reaches five, at which point the condition becomes false, and the loop terminates.
Understanding these elements forms a foundation for using while loops effectively in Python programming.
Control Flow and While Loops
Understanding control flow is critical when working with while loops in Python. Control flow dictates the order in which individual statements, instructions, or function calls are executed in a program. A while loop enables programmers to repeat a block of code as long as a specified condition remains true. This flexibility is what makes while loops a powerful tool for controlling the flow of a program.
The significance of control flow becomes evident in situations where the exact number of iterations is not predetermined. Unlike for loops, which iterate over a sequence or range, while loops adapt dynamically based on a condition, providing greater control to the programmer. This adaptability allows for responsive programming, accommodating varying inputs or states, and is especially useful in scenarios such as user input handling or iterative calculations.
When using while loops, understanding the condition being evaluated is crucial. The loop will continue executing until the condition becomes false. Therefore, the formulation of this condition directly influences the effectiveness and efficiency of the loop. The programmer must ensure that the condition will eventually be false to prevent infinite loops, which can hang or crash a program.
> Key Takeaway: Control flow determines how and when code executes; while loops utilize this concept for iteration until specific conditions change.
In the following sections, we will further explore how control flow operates within while loops and the role of boolean expressions in defining the loop's behavior.
How Control Flow Works
Control flow in while loops begins with an initial evaluation of the loop's condition. Before executing the loop's body, Python checks this condition. If it returns true, the statements within the loop are executed. After executing the statements, the condition is evaluated again before the next iteration. This process repeats until the condition evaluates to false.
This mechanism underscores the importance of properly defining exit conditions. An example of control flow in a while loop is shown below:
In this code, the loop prints the value of as long as it is less than 5. Once reaches 5, the condition becomes false and the loop exits.
When designing a while loop, programmers must keep the following considerations in mind:
- Initial Condition: Establish a clear starting point, often found before the loop begins.
- Exit Condition: Ensure that there is a definitive point at which the loop will stop.
- Increment/Decrement Mechanism: Modify the control variable within the loop body to avoid infinite iterations.
A well-defined control flow is pivotal in achieving efficient and functional code, driving the loop to completion without unnecessary stumbling blocks.
Boolean Expressions in While Loops
Boolean expressions serve as the foundation for the conditions evaluated in while loops. In Python, any expression that returns a boolean value (True or False) can dictate the execution of a while loop. Understanding how to construct effective boolean conditions is essential for harnessing the full power of while loops.
For instance, a basic boolean expression can compare two integers, like so:
This loop continues until is no longer greater than 0, demonstrating a simple yet effective boolean condition at work.
In practice, boolean expressions can become more complex, incorporating logical operators such as , , and . For example:
Here, the loop runs as long as is greater than 0 and less than 15. This not only showcases the versatility of boolean expressions but also emphasizes the need to design conditions that can adapt to various input scenarios.
In summary, control flow and boolean expressions are integral to using while loops effectively in Python. These concepts help define how loops operate, ensuring that they execute correctly and efficiently.
Practical Applications of While Loops
The practical applications of while loops in Python are broad and varied, making them essential for many programming tasks. Understanding these uses allows for greater flexibility and efficiency in coding. While loops facilitate iteration under specific conditions, which can be more dynamically controlled compared to the fixed nature of for loops. This adaptability can be crucial when handling unpredictable outcomes, such as user inputs or processing streams of data.
Common Use Cases
User Input Handling
User input handling is one of the prominent areas where while loops shine. Unlike a for loop that iterates a predefined number of times, a while loop continues until a specific condition is met. This characteristic makes it ideal for scenarios where the number of iterations is not known beforehand. For instance, when creating a program that asks for user input until valid data is given, a while loop efficiently manages the process.
The primary benefit of this approach is its ability to maintain user engagement until the desired input is received. However, it also has the disadvantage of potentially leading to infinite loops if exit conditions are not properly defined.
Data Processing
Data processing also significantly benefits from while loops. They allow for continuous processing of data, making them an efficient choice for applications where data is received in real time or where the total quantity cannot be predetermined. For example, a program that processes data packets until the end of the stream can effectively utilize a while loop.
The flexibility to stop processing based on certain conditions, such as reaching a specific packet or encountering an error, underscores the popularity of this method in real-time applications. The down side includes the necessity for robust exit conditions to prevent unintentional infinite execution, which can halt further application functionality.
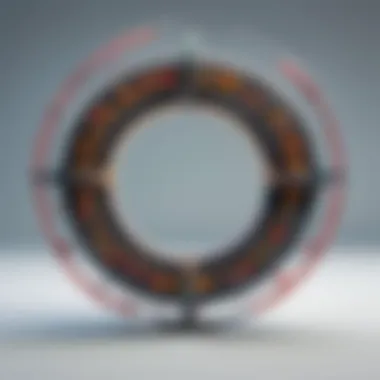
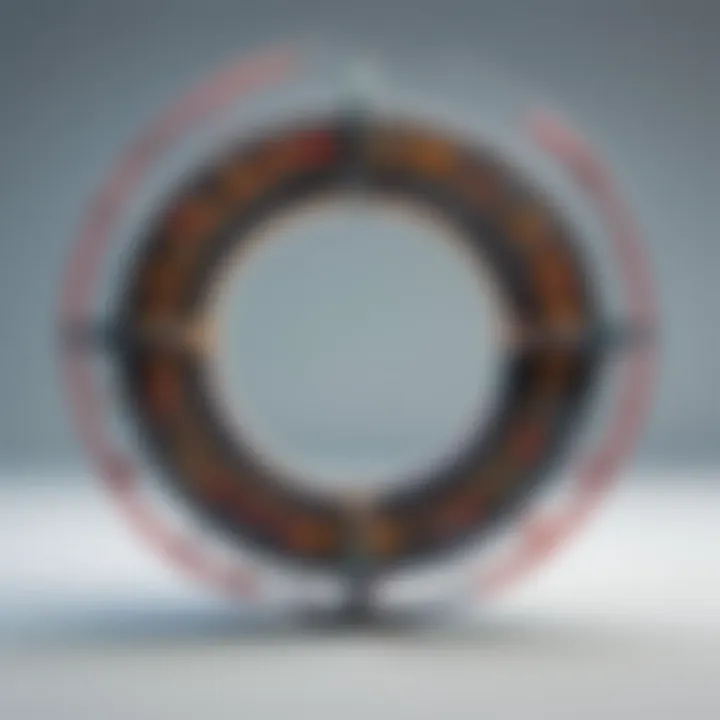
Iterative Calculations
Iterations involving calculations are another area where while loops prove advantageous. This is particularly true in scenarios where results determine the necessity for further calculations. For instance, when calculating factorial of a number, a while loop can iterate until it reaches the conclusion:
The iterative nature of calculations in while loops allows for adjustments based on intermediate results, enhancing the program's adaptability. However, accumulated errors over iterative steps can lead to incorrect results if not carefully managed.
Real-World Examples
Real-world applications of while loops illustrate their functionality across various domains. For instance, in web scraping, a while loop can sustain data extraction until all desired information has been gathered. In automation scripts, while loops facilitate continuous checks of system states or user conditions, ensuring dynamic response to changing environments. These tangible implementations emphasize how while loops are not only theoretical constructs but powerful tools in practical programming scenarios.
While loops offer a unique capability to handle situations that require continuous monitoring and iteration until a condition is satisfied.
Error Handling and Debugging
Error handling and debugging are critical aspects of programming in Python, particularly regarding while loops. While loops can lead to complex flow control situations, making it essential to identify and address errors effectively. Errors can arise at any stage of the while loop's execution, jeopardizing the reliability of software applications. Thus, understanding how to handle potential pitfalls is fundamental for anyone looking to master while loops.
When dealing with while loops, programmers face specific errors that can disrupt the flow of code. Recognizing these common issues can prevent frustration and wasted time during development. Furthermore, debugging strategies enhance oneβs ability to trace and fix problems efficiently, ultimately leading to more robust code.
Common Errors in While Loops
Infinite Loops
Infinite loops are one of the most notorious issues in programming with while loops. An infinite loop occurs when the condition in the while statement always evaluates to true, causing the loop to execute indefinitely. This can lead to system resources being consumed unnecessarily, as the program does not progress to termination. The key characteristic of an infinite loop is that it lacks an appropriate exit strategy, rendering it a potential hazard for programmers.
The discussion of infinite loops is especially relevant in this article because they highlight the importance of carefully evaluating loop conditions. A beneficial way to address infinite loops is through the principle of code logic. Employing a counter or a break condition allows programmers to control the loop and prevent it from running ad infinitum. However, identifying the correct exit condition can sometimes be challenging, particularly in more complex scenarios.
Unmet Exit Conditions
Unmet exit conditions represent another common error that arises in while loops. This error occurs when the condition required to terminate the loop is never satisfied. As a result, the program may hang or behave unexpectedly. This situation is often related to incorrect implementation of termination logic in the loop. The key characteristic of unmet exit conditions is that they can arise from user inputs or external data that do not match the expectations set within the loop.
Addressing unmet exit conditions involves careful design of the loop's control flow. Ensuring that user inputs or variables are accurately assessed before they affect the loopβs continuation is crucial. While addressing this aspect, programmers can also check conditions logically corresponding to real-world requirements and constraints. This practice leads to more predictable applications and ultimately enhances the user experience.
Strategies for Debugging
Debugging while loops requires a systematic approach. Here are some effective strategies:
- Print Statements: Using print statements can help track the values of variables and conditions as the loop progresses. This simple method can clarify how the loop executes and where it may fail.
- Using Debugging Tools: Tools such as Python's built-in debugger or IDEs like PyCharm can provide a controlled environment to step through the code, inspect variables, and understand how control flows within the while loop.
- Code Review: Engaging in peer code reviews often uncovers overlooked logic paths and exit conditions, allowing for fresh perspectives and solutions to potential problems.
By combining these strategies, programmers can continually refine their skill set regarding error handling and debugging while loops. Mastering these elements not only improves the reliability of code but also fosters a deeper understanding of control flow within Python.
Optimization Techniques
Optimization techniques are vital in mastering while loops in Python. These techniques not only enhance the efficiency of the code but also help in avoiding common pitfalls such as infinite loops. Understanding these techniques can lead to better performance and more maintainable code.
Improving Efficiency
The efficiency of while loops can be improved through various strategies. The first approach is to minimize the number of operations within the loop. Each iteration should ideally perform only essential calculations. Additionally, consider using data structures that provide faster access or modification times. For example, utilizing lists or dictionaries judiciously can reduce the time complexities of certain operations.
Moreover, it is essential to ensure that the exit condition is clearly defined and attainable. This avoids unnecessary iterations that can degrade performance. By dropping the number of computations and clarifying exit criteria, developers can significantly enhance the processing speed of their loops.
When to Avoid While Loops
While loops are not always the ideal choice for iterative processes. There are specific scenarios where alternative loops, especially for loops, may provide more benefits. It is important to know when to opt for different structures.
When to Use For Loops Instead
For loops can be preferred when the number of iterations is predetermined. Their syntax simplifies looping over a sequence or collection, making them easier to read and maintain. This characteristic often makes for loops a popular choice in situations where the total number of cases is known ahead of time.
For loops also implicitly manage the loop counter. This minimizes the chance of errors related to incrementing or resetting the counter, which can lead to infinite loops if handled incorrectly in while loops.
Considerations for Performance
When assessing performance, consideration of the context and complexity of the task at hand is essential. While loops may be less performant when manipulating large datasets or executing complex tasks. In such cases, optimized for loops can provide consistent performance and reduce memory usage. Additionally, if the loop requires extensive operations, rewriting it in a functional programming style using map or filter functions might yield better results.
By being aware of these performance considerations, programmers can choose the most suitable looping structure based on the requirements of their specific tasks.
"Choosing the right loop structure is critical for developing efficient algorithms. Always evaluate the task before implementing any loop."
In summary, optimization techniques for while loops are significant in crafting high-performance Python programs. Understanding when to utilize these techniques can result in cleaner, faster, and more reliable code.
Advanced Concepts
Understanding advanced concepts in while loops not only enriches one's programming toolkit but also improves code efficiency and maintainability. While the basic structure of while loops is fundamental, exploring nested loops and their interaction with other control structures expands the versatility of how we can control the flow of our programs. Exploring these advanced topics empowers the programmer, allowing for greater complexity in data handling and process control.

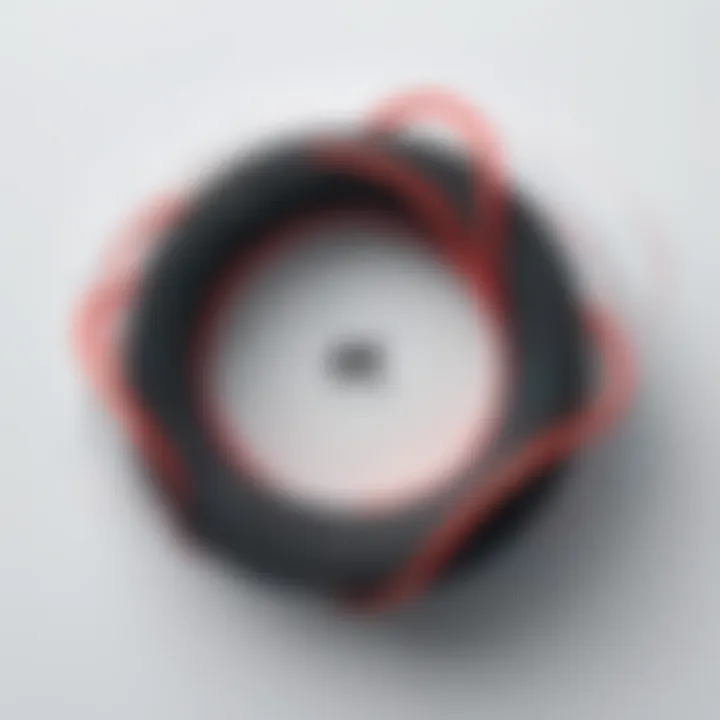
Nested While Loops
Nested while loops occur when one while loop is placed inside another. This can be particularly useful for multi-dimensional data structures, such as arrays or matrices. For instance, if you want to iterate through a grid, a nested while loop allows you to go through each row, and within that loop, you can iterate through each column. This use of nested loops is a powerful feature of the while loop, offering flexibility in how complex data is processed.
When using nested while loops, care must be taken to manage the exit conditions for each loop. Improper handling may lead to infinite loops, which can be difficult to debug. Additionally, readability becomes a concern; thus, it is important to keep the logic clear to avoid confusion for anyone who may read your code later.
Combining While Loops with Other Control Structures
If Statements
If statements are one of the most common control structures that can enhance the functionality of while loops. By integrating if statements within a while loop, a programmer can introduce conditional logic that dictates how the loop behaves based on certain criteria. For example, you could use an if statement to check specific conditions that determine whether to continue the iteration or to break out of the loop.
A key characteristic of using if statements in while loops is that it allows for dynamic decision-making. This means that the program can make real-time decisions based on variables that might change during execution. This integration can lead to more efficient code, as iterations can be skipped if certain conditions are met, enhancing performance in scenarios where specific data does not need to be processed.
However, one disadvantage might be that it can complicate the loop's logic, especially for beginners. It is vital to keep the conditions organized and straightforward to avoid confusion.
Break and Continue Statements
The break and continue statements are crucial for controlling the flow of while loops effectively. The break statement allows a programmer to exit the loop prematurely based on specified conditions. This can be particularly useful in scenarios where you've reached the desired outcome or have encountered an error condition that necessitates halting further execution.
On the other hand, the continue statement is used to skip the current iteration and proceed to the next one. This becomes beneficial when a specific condition is met that requires the loop to ignore certain data but continue processing others.
Both statements add efficiency and clarity to the code. They enable more intricate behaviors within looping structures, allowing for fine-tuned control over how the program runs and when to halt or skip specific iterations. The key feature of these statements is their ability to manipulate the flow without convoluting the overall structure of the loop, yet, they may lead to less intuitive code if overused or misused, particularly in complex loops.
In summary, mastering advanced concepts like nested while loops and control structures allows programmers to write more efficient and flexible code.
Understanding and implementing these techniques broadens a programmer's capabilities and prepares them for real-world programming challenges.
Comparative Analysis: While Loops vs. For Loops
In the realm of programming with Python, the choice between while loops and for loops often sparks substantial debate. Understanding when to employ each type of loop is essential for efficient coding. While loops are designed for scenarios where the number of iterations is not predetermined, in contrast, for loops are ideally suited for situations where the number of iterations is known.
A significant consideration is performance. While loops can run indefinitely if not designed correctly, causing potential inefficiencies in a program. On the other hand, for loops operate within a defined range or collection, thus ensuring that they will not exceed their limits inadvertently. Each loop has its specific advantages, which make them suitable for distinct situations.
For instance, while loops shine in conditions where the iteration depends on a dynamic situation, such as reading user input until a correct entry is made.
Performance Comparison
When we analyze performance, for loops have a clear advantage in scenarios where the number of iterations is defined beforehand. Since the loop iterates over a specified range or a list, Python can optimize the loop execution effectively. This is particularly evident in larger data sets where predictability plays a role in execution speed.
In contrast, while loops possess a level of flexibility that allows them to be more adaptable in certain contexts. However, their performance might degrade in instances of poor structure, particularly with infinite loops. For loops are generally more efficient since they remove the need for continuous condition checking once the loop's bounds have been established.
For example, consider the following code snippets for clarity:
In terms of resource management, while loops demand careful attention to prevent potential infinite iterations, which can lead to crashes or unresponsive programs. Conversely, for loops, when implemented correctly, provide a more straightforward execution pathway.
When to Use Each Loop
Choosing between a while loop and a for loop boils down to the specific requirements of your program. Here are key factors to consider:
- Use a while loop when:
- Use a for loop when:
- The number of iterations is uncertain.
- You need to wait for external conditions to change (like user input).
- You face situations requiring repeated execution until certain criteria are met.
- The number of iterations is known ahead of time.
- You want to iterate through fixed data structures (e.g., lists, tuples, or strings).
- The task involves processing elements in a collection one by one.
Understanding these nuances helps programmers make informed decisions regarding loop usage. Each loop has its place in the programming toolkit, and discerning their strengths can lead to more efficient and readable code.
Epilogue
In this article, we have delved into the significance of while loops in Python, examining their structure, practical uses, and potential optimizations. Understanding while loops is crucial for anyone looking to enhance their programming skills. They allow for iterative processes that can adjust based on real-time conditions, making them versatile and powerful.
Key elements highlighted include the syntax of a while loop, its control flow, and common pitfalls associated with improper use. For instance, recognizing the risk of creating infinite loops is fundamental to avoiding runtime errors that can lead to performance issues. Moreover, the discussion around optimization techniques helps programmers choose the right tool for the job, whether that's a while loop or an alternative like a for loop.
Reflecting on these components allows Python developers to utilize while loops more effectively in various scenarios. It fosters a deeper understanding of how control flow operates, enabling them to write cleaner and more efficient code.
"Mastering while loops not only improves code quality but enhances overall programming proficiency."
As you consider your programming journey, remember that while loops are an essential component of Python. They can serve as building blocks for complex algorithms and solutions. Ultimately, grasping this concept expands your capabilities in software development, data processing, and automation tasks.
Summary of Key Points
- Definition and Importance: While loops facilitate repeated execution based on conditions.
- Control Flow: Properly managing control flow is vital to avoid errors, such as infinite loops.
- Practical Applications: These loops are used in user input handling, data processing, and iterative calculations.
- Optimization: Understanding when to utilize while loops versus for loops can impact performance significantly.
- Real-World Examples: The practical implementation of while loops has been demonstrated in various contexts.
Encouragement for Further Learning
Consider exploring additional resources to deepen your understanding of while loops and their alternatives. Websites like Wikipedia and Britannica provide comprehensive insights into control flows in programming.
Engaging with communities on platforms like Reddit can offer valuable peer support and learning opportunities. Interacting with fellow learners and professionals can expose you to innovative approaches and methodologies that enhance your knowledge.