Mastering Java Unit Testing Efficiency: Advanced Strategies Revealed
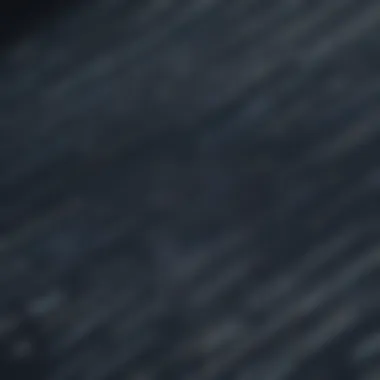
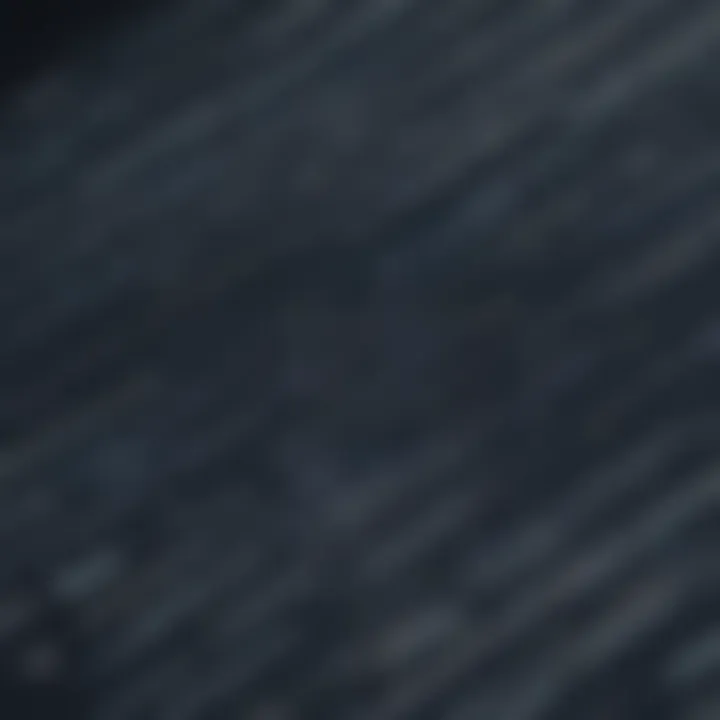
Overview of Topic
Java unit testing is a vital aspect of software development, aiming to enhance efficiency and reliability in Java applications. Understanding the core principles and advanced strategies of unit testing is crucial for tech enthusiasts, professionals, and beginners alike. This section will delve into the significance of unit testing in the tech industry, its evolution over time, and the key concepts that form the foundation of effective testing methodologies.
Fundamentals Explained
To grasp the essence of Java unit testing, one must familiarize themselves with its core principles and theories. Key terminologies like test cases, test suites, and assertions play a pivotal role in ensuring the quality and functionality of software. Delving into basic concepts such as test-driven development (TDD) and behavior-driven development (BDD) will provide a solid foundation for implementing robust testing practices in Java programming.
Practical Applications and Examples
Real-world case studies and hands-on projects serve as valuable learning tools for Java unit testing. By exploring code snippets and implementation guidelines, readers can witness the practical application of testing techniques in action. Demonstrations of how to write effective test cases, conduct test coverage analysis, and automate testing processes offer insights into efficient testing practices tailored for Java applications.
Advanced Topics and Latest Trends
The field of Java unit testing is constantly evolving, with the emergence of cutting-edge developments and advanced methodologies. Exploring topics like microservices testing, continuous integrationcontinuous deployment (CICD) pipelines, and cloud-based testing environments unravels the latest trends in the realm of software testing. Understanding these advancements equips professionals with the knowledge to stay ahead in the competitive tech landscape.
Tips and Resources for Further Learning
For those looking to deepen their understanding of Java unit testing, a plethora of resources are available. Recommended books, online courses, and tools like JUnit and Mockito offer avenues for practical learning and skill enhancement. By leveraging these resources, individuals can not only refine their testing abilities but also stay abreast of the latest innovations in Java testing practices.
1. Introduction to Java Unit Testing
Java unit testing is a critical aspect of software development, ensuring the reliability and functionality of Java applications. Understanding the principles and practices of unit testing is imperative for developers looking to create robust and error-free code. In this article, we delve into the realm of Java unit testing, exploring advanced strategies to enhance efficiency and effectiveness, catering to tech enthusiasts, professionals, and beginners.
2. Understanding Unit Testing
The Importance of Unit Testing
Unit testing plays a pivotal role in software development by allowing developers to test individual units of code in isolation, ensuring that each component functions as intended. The key characteristic of unit testing lies in its ability to identify bugs and errors early in the development process, thus reducing the overall cost of debugging and maintenance. The unique feature of unit testing is its capacity to validate the correctness of code logic, improving code quality and system dependability.
Basic Concepts of Unit Testing
Basic concepts of unit testing encompass writing test cases to validate individual units of code, executing them independently to ensure accuracy and reliability. The fundamental aspect of unit testing lies in creating reliable and repeatable tests that can be automated for continuous integration and deployment. The unique feature of basic unit testing concepts is their emphasis on isolating specific code components for targeted testing, leading to improved code coverage and fault detection.
Benefits of Unit Testing in Software Development
Unit testing brings several advantages to the software development process, including early bug detection, faster debugging cycles, and improved software quality. By testing individual units of code in isolation, developers can identify and rectify issues promptly, reducing the likelihood of bugs reaching production environments. The unique feature of unit testing's contribution to software development is its role in promoting robust, maintainable, and scalable codebases, enhancing long-term project success.
3. Setting Up Java Environment for Unit Testing
Configuring IDE for Unit Testing
Configuring an Integrated Development Environment (IDE) for unit testing is essential for streamlining the testing process and enhancing developer productivity. By integrating unit testing frameworks within the IDE, developers can seamlessly write, execute, and analyze test cases within a familiar environment. The key characteristic of IDE configuration for unit testing is its ability to provide a centralized platform for managing test suites and results, simplifying the testing workflow.
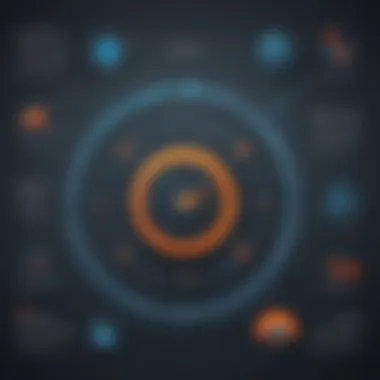
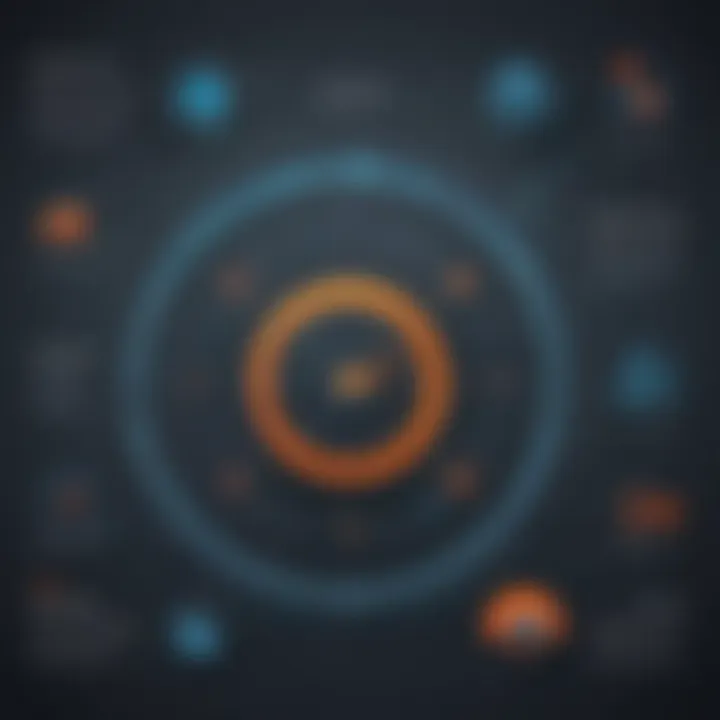
Integrating Testing Frameworks in Java Projects
Integrating testing frameworks, such as JUnit or Test NG, in Java projects is crucial for automating the execution of test cases and generating comprehensive test reports. These frameworks offer a wide range of assertion methods and annotations to simplify the testing process and facilitate accurate result verification. The unique feature of integrating testing frameworks is their contribution to establishing a structured and organized approach to unit testing, enhancing code readability and test maintainability.
Installing Necessary Dependencies for Unit Testing
Installing necessary dependencies, such as testing libraries and plugins, is vital to enable seamless integration of testing frameworks and tools in Java projects. These dependencies provide essential functionalities, such as mocking, assertion, and test execution, to support efficient and effective unit testing. The key characteristic of installing dependencies is to ensure compatibility with the chosen testing frameworks and maintain consistency across different project environments.
4. Best Practices in Java Unit Testing
Naming Conventions for Test Methods
Following consistent and descriptive naming conventions for test methods is essential for enhancing code readability and test documentation. By adopting a standardized naming format, developers can easily identify the purpose and scope of each test case, promoting better test management and collaboration. The unique feature of naming conventions lies in their ability to communicate the intent and expected outcomes of test methods, facilitating efficient test execution and result analysis.
Isolating Unit Tests for Independence
Isolating unit tests from external dependencies and interactions is critical for ensuring reliable and reproducible test results. By minimizing dependencies on external systems or resources, developers can control the test environment and focus solely on the behavior of the unit under examination. The key characteristic of isolating unit tests is to promote test independence, reduce test flakiness, and enhance test reliability and determinism.
Utilizing Mocking Frameworks for External Dependencies
Utilizing mocking frameworks, such as Mockito, for simulating external dependencies and behaviors is essential for testing components in isolation. Mocking frameworks enable developers to create mock objects that mimic the behavior of real components, facilitating controlled and predictable testing scenarios. The unique feature of mocking frameworks is their versatility in simplifying test setup, verifying method calls, and handling complex dependencies, enhancing the efficiency and effectiveness of unit testing practices.
Advanced Techniques in Java Unit Testing
In the realm of Java unit testing, the focus shifts to advanced techniques aiming to enhance efficiency and effectiveness. Understanding these techniques is crucial for developers, as they pave the way for robust test cases that ensure software reliability. Advanced Techniques in Java Unit Testing play a pivotal role in streamlining the testing process, reducing bugs, and enhancing the overall quality of Java applications. By delving into these intricacies, developers can elevate their testing capabilities and produce more stable and robust software solutions.
Test Driven Development (TDD)
Principles of TDD
The Principles of Test Driven Development (TDD) form the core foundation of automated testing practices in Java programming. One of the distinctive aspects of TDD is its iterative nature, where developers write test cases before the actual code implementation. This method not only ensures comprehensive test coverage but also fosters a deep understanding of the software requirements. The Principles of TDD promote a disciplined approach to software development by prompting developers to focus on small, testable increments, leading to more maintainable and resilient code.
Implementing TDD in Java Projects
Implementing TDD in Java Projects involves aligning the development process with the test-first approach. By adhering to TDD practices, developers can create robust, bug-free code that meets the specified requirements. The key characteristic of Implementing TDD in Java Projects lies in its ability to promote code quality and reduce the debug cycle durations. Although adopting TDD may initially seem time-consuming, the long-term benefits include faster development cycles, improved code quality, and enhanced software maintainability.
Benefits of TDD in Unit Testing
The Benefits of Test Driven Development (TDD) in Unit Testing are manifold. By following TDD practices, developers can detect defects early in the development cycle, leading to significant cost savings. TDD encourages modular and loosely coupled code structures, making enhancements and modifications easier. Moreover, the iterative nature of TDD ensures continuous feedback, resulting in higher code quality and overall software reliability.
Parameterized Tests
Creating Parameterized Test Cases in Java
Creating Parameterized Test Cases in Java involves generating a set of inputs and their expected outputs to run multiple test variations efficiently. Parameterized testing allows developers to verify the behavior of a method against different input values, enhancing test coverage. The main advantage of this approach is its ability to streamline test case creation and execution, minimizing redundancy and maximizing efficiency in the testing process.
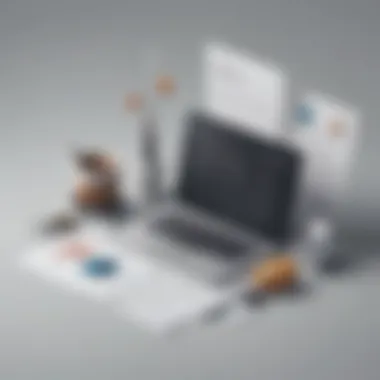
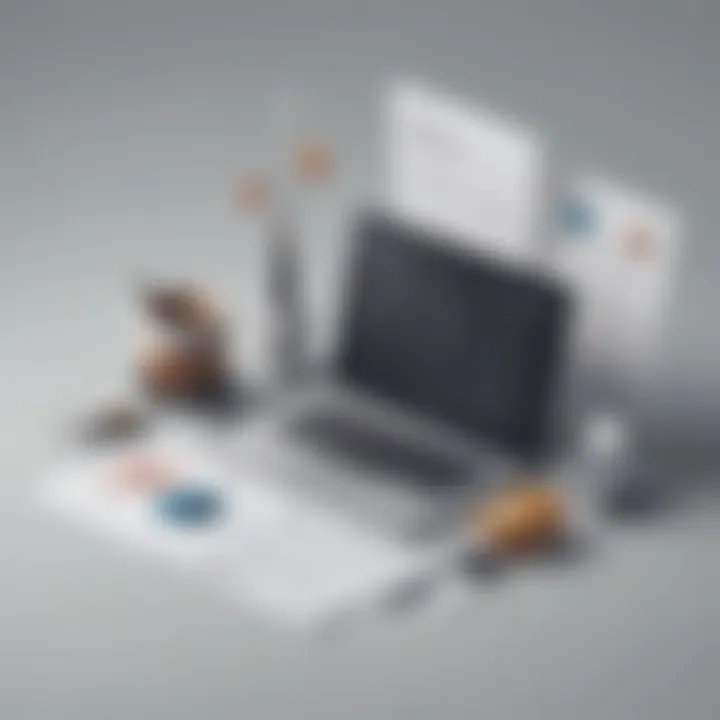
Testing Multiple Scenarios with Parameterized Tests
Testing Multiple Scenarios with Parameterized Tests enables developers to evaluate various test conditions using a single test method. This method proves to be highly efficient, especially when multiple scenarios need to be tested simultaneously. By organizing test data into manageable sets, developers can ensure comprehensive test coverage while maintaining test clarity and conciseness.
Enhancing Code Coverage with Parameterized Tests
Enhancing Code Coverage with Parameterized Tests is crucial for achieving thorough testing of Java applications. By leveraging parameterized tests, developers can enhance code coverage by systematically testing different input combinations. This approach not only improves the robustness of the test suite but also helps identify edge cases and potential vulnerabilities within the codebase.
Mockito Framework for Mocking
Introduction to Mockito
The Introduction to Mockito brings forth a powerful tool for Java developers to address dependencies during unit testing. Mockito facilitates the creation of mock objects that mimic the behavior of complex components, enabling isolated testing of individual units. The key characteristic of Mockito lies in its flexibility and ease of use, making it a popular choice for simplifying unit test development in Java projects.
Mocking Objects and Behaviors with Mockito
Mocking Objects and Behaviors with Mockito involves creating mock objects that emulate the interactions and responses of real Java components. This practice enables developers to isolate specific units for testing without requiring the entire application to run. The unique feature of Mockito lies in its ability to simulate complex scenarios effortlessly, allowing developers to focus on testing the logic of individual components effectively.
Verifying Method Calls and Interactions in Unit Tests
Verifying Method Calls and Interactions in Unit Tests using Mockito ensures that the expected methods are invoked correctly during unit testing. This verification process validates the interactions between different components within the software, ensuring proper communication and functionality. By accurately verifying method calls and interactions, developers can enhance the reliability and robustness of their unit tests, thereby improving the overall quality of Java applications.
Optimizing Java Unit Testing Workflow
In the realm of Java unit testing, optimizing the workflow plays a pivotal role in ensuring the efficiency and efficacy of the testing process. By focusing on streamlining various aspects of unit testing, such as automation, code coverage, and test suite management, developers can enhance the overall quality of their software products. The importance of this topic lies in its ability to significantly impact the reliability and stability of Java applications by identifying and rectifying potential issues early in the development cycle.
Continuous Integration for Unit Tests
Automating Unit Testing with Pipelines
Automating unit testing through CICD pipelines is a crucial aspect of ensuring swift and consistent testing procedures in Java projects. This automation minimizes manual errors, accelerates the testing process, and facilitates seamless integration of new code changes. By automating unit testing, developers can achieve a continuous feedback loop that enhances the overall software quality and optimizes the development workflow. One of the key characteristics of automating unit testing with CICD pipelines is its ability to provide rapid feedback on code changes, allowing developers to detect and fix issues promptly. While this approach offers efficiency and reliability, it requires a considerable initial investment in setting up the pipelines and maintaining them throughout the project lifecycle.
Integrating Testing Reports in Build Processes
Integrating testing reports in build processes empowers developers to gain insights into the test results and the quality of their code base. These reports highlight areas of improvement, code coverage statistics, and test case performance, enabling teams to make informed decisions regarding code enhancements and bug fixes. A key characteristic of this integration is the visibility it provides into the testing outcomes, allowing for continuous monitoring of the project's health and identifying potential bottlenecks early on. While this practice is essential for ensuring code quality, it may require additional resources and time for setting up and maintaining the reporting infrastructure effectively.
Ensuring Consistent Test Execution with
Ensuring consistent test execution with CI ensures that unit tests are run systematically and reliably throughout the development lifecycle. By enforcing standardized testing protocols and automated workflows, developers can minimize test failures, optimize resource utilization, and enhance the overall testing efficiency. A crucial characteristic of this approach is its ability to create a consistent testing rhythm that aligns with the iterative nature of agile development methodologies. While consistent test execution offers predictability and reliability in the testing process, it demands meticulous attention to detail in configuring the CI environments and monitoring test outputs for potential deviations.
Code Coverage Analysis
Understanding Code Coverage Metrics
Understanding code coverage metrics is essential for evaluating the comprehensiveness of test suites and identifying areas of code that lack sufficient testing. This analysis helps developers assess the effectiveness of their testing strategies, measure the quality of their test cases, and prioritize areas for improvement. A key characteristic of code coverage metrics is their ability to quantify the extent to which different parts of the codebase are tested, enabling teams to focus on enhancing the coverage of critical functionalities. While this analytical approach offers valuable insights into test quality, it requires careful interpretation and continuous refinement to ensure meaningful improvements in the testing process.
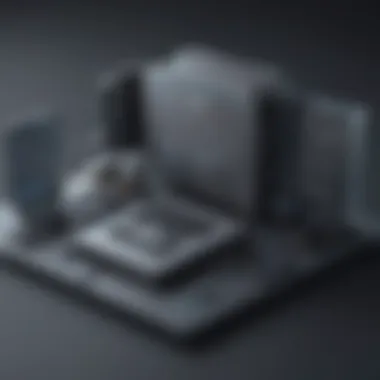
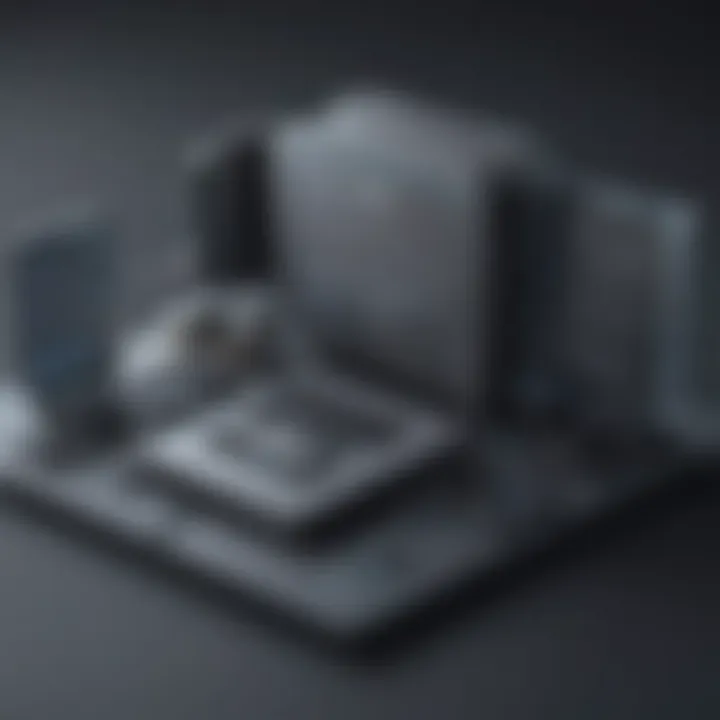
Improving Code Coverage in Java Projects
Improving code coverage in Java projects involves enhancing the scope and depth of test cases to increase the overall quality and reliability of the software. By targeting under-tested code segments and edge cases, developers can fortify their test suites and identify potential vulnerabilities or bugs early on. A key characteristic of this improvement process is its impact on code quality and robustness, as higher coverage often correlates with lower defect rates and improved software stability. While improving code coverage is a valuable practice for ensuring comprehensive testing, it necessitates strategic planning, thorough test case design, and continuous monitoring to maintain and enhance coverage levels effectively.
Tools for Analyzing Code Coverage
Utilizing tools for analyzing code coverage streamlines the assessment of test effectiveness and facilitates data-driven decisions for optimizing testing strategies. These tools offer detailed reports on code coverage metrics, highlighting gaps in test coverage and suggesting areas for improvement. A key characteristic of code coverage analysis tools is their ability to provide actionable insights for enhancing testing methodologies and focusing resources on critical code components. While these tools are valuable for improving test quality, they often require integration into the development environment and ongoing calibration to align with the project's testing goals.
Test Suite Optimization
Organizing Test Suites for Efficiency
Organizing test suites for efficiency involves structuring test cases in a logical and systematic manner to expedite test execution and maintenance. By categorizing tests based on functionalities, components, or modules, developers can enhance the readability, reusability, and scalability of their test suites. A key characteristic of this optimization approach is its impact on testing productivity and the ease of managing and scaling test suites as the project evolves. While organizing test suites offers advantages in streamlining test processes, it requires careful planning, documentation, and periodic reviews to ensure optimal organization and consistency across test cases.
Reducing Redundancy in Test Cases
Reducing redundancy in test cases is crucial for optimizing testing efforts, enhancing maintenance efficiency, and avoiding unnecessary duplication of test scenarios. By identifying and eliminating duplicate or overlapping test cases, developers can streamline the testing process, reduce execution times, and maintain the integrity of test suite outcomes. A key characteristic of this optimization strategy is its ability to declutter test suites, improve testing focus, and mitigate the risk of inconsistencies or ambiguities in test results. While reducing redundancy offers benefits in resource optimization and test management, it requires meticulous analysis, collaboration among team members, and proactive maintenance of test suites to prevent the reemergence of redundant test cases.
Prioritizing Unit Tests for Critical Components
Prioritizing unit tests for critical components entails focusing testing efforts on the most vital and high-risk functionalities of the software application. By prioritizing tests based on business impact, complexity, or frequency of use, developers can ensure that critical areas receive thorough and targeted testing coverage. A key characteristic of this prioritization strategy is its role in mitigating potential risks, identifying crucial defects early in the development cycle, and safeguarding the core functionalities of the software. While prioritizing unit tests offers advantages in risk management and quality assurance, it demands strategic planning, collaboration with stakeholders, and continuous reassessment of testing priorities to align with evolving project requirements.
Conclusion
Summary of Key Points
Recap of Java Unit Testing Basics
As we revisit the foundational principles of Java unit testing, a fundamental component that emerges is the emphasis on creating robust test cases to ensure the reliability and functionality of code snippets. This reflective exercise on the basics of unit testing serves as a cornerstone for laying a sturdy framework in Java development processes. By adhering to standardized testing practices and leveraging the power of test-driven development, the recap of Java unit testing basics reinforces the bedrock of software quality assurance methodologies, enabling practitioners to deploy resilient and error-free applications.
Highlighting Advanced Strategies Discussed
Delving into the realm of advanced strategies for Java unit testing offers a profound exploration of innovative techniques and methodologies aimed at amplifying testing efficiency. Through the exploration of parameterized tests, mockito frameworks for mocking, and test suite optimization, the article elucidates how incorporating these sophisticated approaches can drastically enhance the efficacy of unit testing endeavors. By shedding light on these cutting-edge methodologies, the article underscores the evolution of testing paradigms within the Java ecosystem, paving the way for more agile and resilient software development practices.
Emphasizing the Importance of Unit Testing in Java Development
In underscoring the critical role of unit testing in Java development, the article accentuates the indispensable nature of quality assurance measures in ensuring the seamless functionality of software applications. By placing a strong emphasis on the value proposition of unit testing, the narrative elucidates how adherence to meticulous testing protocols not only fortifies the resilience of codebases but also fosters a culture of continuous improvement and innovation within development teams. Through highlighting the symbiotic relationship between unit testing and software quality, the article advocates for a robust testing framework that proactively mitigates risks and enhances the overall efficacy of software products.
Final Thoughts
Encouraging Implementation of Efficient Unit Testing Practices
The narrative on efficient unit testing practices serves as a clarion call for practitioners to cultivate a mindset of precision and rigor when executing testing protocols. By advocating for the adoption of industry best practices and proactively iteratively improving testing processes, the article empowers developers to forge a path towards excellence in software quality assurance. Through embracing efficient unit testing practices, developers can streamline their workflows, accelerate time-to-market, and elevate the overall quality of software deliverables.
Inspiring Continuous Learning and Improvement in Testing Skills
The discourse on continuous learning in the realm of testing skills serves as a driving force for encouraging developers to proactively expand their knowledge horizons and stay abreast of emerging trends in the testing landscape. By fostering a culture of continuous improvement, the article promotes a growth mindset that culminates in sharper testing acumen, enhanced problem-solving capabilities, and a deeper understanding of the nuances within Java unit testing. Through inspiring developers to embark on a journey of lifelong learning, the article catalyzes a paradigm shift towards more resilient and adaptive testing practices that cater to the evolving demands of the digital age.
Inviting Feedback and Suggestions for Future Articles
The inclusive ethos of inviting feedback and suggestions for future articles conveys a commitment to nurturing a vibrant community of learners and thought leaders within the realm of software development and quality assurance. By actively soliciting input from readers and stakeholders, the article signals a willingness to evolve and adapt based on the evolving needs and preferences of its audience. Through fostering a culture of open dialogue and constructive critique, the article cultivates a dynamic feedback loop that fosters continuous improvement in content quality, relevance, and depth, laying the groundwork for a collaborative and enriching educational ecosystem.