In-Depth Exploration of Python Packages and Their Uses
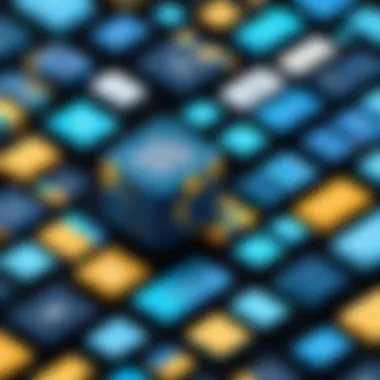
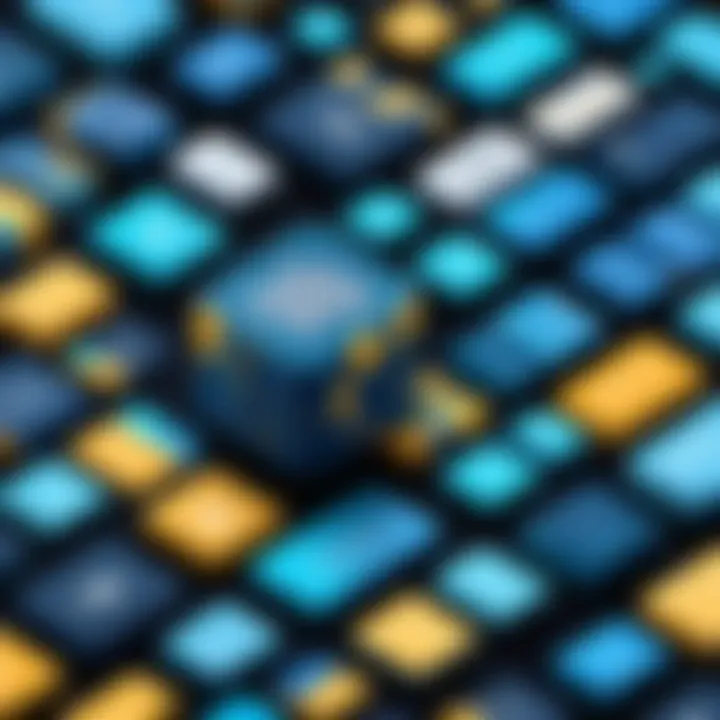
Overview of Topic
Preamble to the main concept covered
Python is a highly versatile programming language known for its simplicity and readability. One of its most powerful features is the extensive range of packages available. These packages allow developers to extend the language's functionalities and cater to a multitude of applications, ranging from basic data manipulation to complex web applications. This section will provide a foundation to understand what Python packages are and their implications in the tech industry.
Scope and significance in the tech industry
The tech industry has seen an exponential growth in the use of Python over the last decade. Organizations use Python for various purposes, including data analysis, machine learning, artificial intelligence, web development, and automation. The availability of packages not only enhances productivity but also allows developers to leverage existing solutions rather than reinventing the wheel.
Brief history and evolution
Python packages date back to the language's inception. Initially, the Python Standard Library provided a selection of built-in modules and tools. As Python gained popularity, many third-party packages emerged. Developers worldwide contribute to the Python Package Index (PyPI), which currently hosts thousands of packages. The evolution reflects an ongoing commitment to improving and expanding Python's capabilities.
Fundamentals Explained
Core principles and theories related to the topic
At their core, Python packages are directories containing Python scripts and other resources. These packages follow a systematic organization with clear naming conventions, allowing developers to easily understand their structure and functionality.
Key terminology and definitions
- Package: A way of organizing related modules. A package can contain sub-packages and modules.
- Module: A single file containing Python code which can define functions, classes, and variables.
- Library: A collection of packages and modules that provide specific functionalities.
Basic concepts and foundational knowledge
Understanding packages begins with knowing the installation and usage process. Python uses as a package manager, which simplifies the installation and management of packages.
Practical Applications and Examples
Real-world case studies and applications
Python packages find applications in various fields. For instance, data scientists frequently use Pandas for data manipulation and Matplotlib for visualization. In web development, Flask and Django serve as powerful frameworks for building robust applications.
Demonstrations and hands-on projects
For those new to Python packages, it is helpful to work on small projects to grasp their utility. A project could involve scraping data using Beautiful Soup, analyzing it with Pandas, and visualizing results with Matplotlib.
Code snippets and implementation guidelines
Here is a simple example demonstrating the installation and use of the Pandas package:
Advanced Topics and Latest Trends
Cutting-edge developments in the field
Recent advancements in Python packages include integration with machine learning frameworks like TensorFlow and PyTorch. These packages allow for sophisticated data modeling and analysis, making Python a staple for machine learning tasks.
Advanced techniques and methodologies
Developers are increasingly adopting microservices architecture, where different functionalities are encapsulated into separate packages. This approach enhances code reusability and simplifies maintenance.
Future prospects and upcoming trends
As Python continues to evolve, the landscape of packages will also change. Enhanced AI capabilities and growing interest in data analysis will likely lead to the development of more specialized packages tailored to niche applications.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
- "Python Crash Course" by Eric Matthes
- Online courses on platforms like Coursera and Udemy
- Python’s official documentation at python.org
Tools and software for practical usage
Use integrated development environments (IDEs) like PyCharm or VS Code to manage and develop Python packages effectively. They offer features like code completion and debugging tools that streamline the coding process.
Python’s rich ecosystem of packages makes it a preferred language across various industries. Understanding and leveraging these packages can significantly enhance a developer's productivity and capability.
Prelims to Python Packages
Python packages play a vital role in the language's ecosystem. They allow developers to extend the default functionality of Python easily. Understanding packages is crucial for anyone looking to leverage Python for various applications, including data analysis and web development. The systematic organization of code into packages promotes reusability, which can significantly enhance productivity. This article covers essential aspects of Python packages, providing a foundation for students and professionals alike.
Definition of a Package
In programming, a package is a collection of modules that can include various functionalities and tools. Python packages are designed to group related code together in a systematic way. Typically, a package contains an file that allows Python to recognize it as a package. The file can be empty, or it may execute initialization code for the package. This organization helps users manage dependencies more effectively and avoid conflicts among different modules.
Importance in Python Programming
The significance of packages in Python programming cannot be overstated. They allow for better code organization and simplified maintenance. By using established packages, developers can spend less time on repetitive tasks and focus on solving specific problems. Furthermore, the Python community has created thousands of third-party packages, each serving unique purposes.
Here are a few reasons why packages are essential in Python programming:
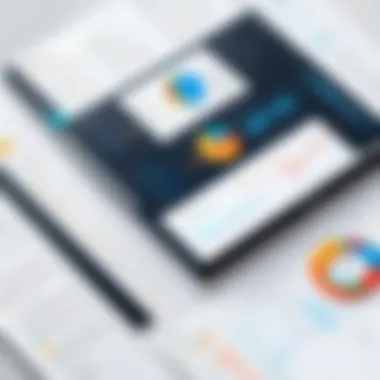
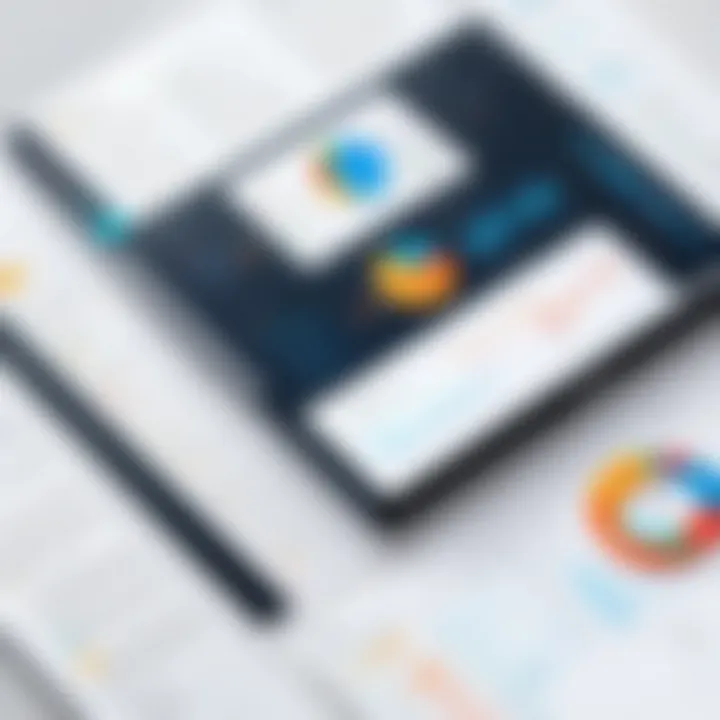
- Modularity: Packages break down larger applications into smaller, manageable components. This modularity simplifies debugging and testing.
- Reusability: Developers can use packages across different projects without rewriting the same code. This feature not only saves time but also enhances consistency.
- Collaboration: Teams can work together more effectively by using shared packages that define common functionalities. By mastering package usage, team members can integrate their work with that of others seamlessly.
"Well-organized code leads to clarity and efficiency in programming."
With a solid understanding of Python packages, individuals can harness their full potential. This foundational knowledge allows developers to explore more advanced topics in Python programming. Whether designing simple scripts or complex applications, mastering packages enhances efficiency.
Standard Library Packages
Standard library packages are an essential aspect of Python, providing built-in functionalities that simplify many programming tasks. Unlike third-party packages, those in the standard library come pre-installed with Python, which means no additional installation is required. This accessibility makes them a go-to resource for developers, allowing them to focus more on solving problems rather than on setting up tools.
There are numerous benefits to using standard library packages. They are generally well-documented, stable, and optimized for performance within the Python environment. Additionally, they are maintained by the core Python development community, ensuring compatibility across various Python versions.
From file I/O operations to data manipulation, the standard library encompasses a wide range of functionalities. This coverage helps in reducing reliance on external packages, which can sometimes introduce compatibility issues or added complexity.
Overview of the Standard Library
The standard library in Python is a collection of modules and packages that are included with Python installations. These modules cover a broad spectrum of needs, including operating system interaction, mathematical operations, and data serialization.
Python's emphasis on simplicity and readability is evident in how its standard library is organized. Each module serves specific functions, facilitating the development process by providing commonly required tools and functionalities.
Examples of Common Standard Packages
os
The module provides a way of using operating system functionalities. It allows for interactions like file and directory management, making it a critical package for developers dealing with file paths and system variables. One of its key characteristics is cross-platform compatibility, ensuring that paths can function in a consistent manner on different operating systems.
A unique feature of is the ability to interact with environment variables. This functionality is beneficial for managing deployment configurations without hardcoding values into your application. However, on some systems, certain operations may require elevated permissions, which could be seen as a disadvantage for less-privileged users.
sys
The module is integral for system-specific parameters and functions. By providing access to command-line arguments and the Python interpreter’s information, it assists developers in creating robust command-line interfaces.
Its key characteristic includes the ability to manipulate the Python runtime environment, making it a powerful tool when building scripts and applications. The unique feature of is the variable, which allows developers to alter where Python searches for modules. However, tweaking this path requires caution, as incorrect configurations can lead to import errors.
math
The module provides mathematical functions, constants, and operations. It enhances Python's capabilities by offering a wide range of mathematical tools which are not available in standard operators.
Including this package in projects is hugely beneficial because it includes functions for trigonometry, geometry, and more. The unique feature of is the inclusion of constants like and , providing exact values for calculations. However, it might be worth noting that operates exclusively with floating-point numbers, which could lead to precision issues when handling very large or very small numbers.
datetime
The module supplies classes for manipulating dates and times. With precise tools for time arithmetic, it plays a vital role in applications that need date and time management.
Its key characteristic is versatility, as it allows operations like comparing, subtracting, and formatting dates. A unique feature is its support for timezone-aware datetimes, which is crucial for applications that operate across different time zones. However, working with time zones can complicate implementations, particularly for developers less experienced in time zone management.
Usage Scenarios
Standard library packages are used in myriad scenarios ranging from simple scripts to complex applications. Examples include:
- File Management: Using for navigating file systems or reading and writing files.
- Mathematical Computations: The module's functionality is often utilized in scientific computing and engineering applications.
- Date Management: The module is essential in logging events in software applications or managing schedules.
- System Interface: Developers leverage the module when creating command-line tools, making use of command-line arguments and environment manipulation.
Popular Third-Party Packages
Popular third-party packages play a crucial role in extending Python’s capabilities. They enhance the language's core functionality, providing specialized tools for various tasks that standard library packages may not cover. The wide range of available third-party packages addresses numerous sectors, from data analysis and machine learning to web development. This section explores what defines a third-party package and highlights some of the most popular options, showcasing their importance and practicality in real-world applications.
What Defines a Third-Party Package
A third-party package in Python refers to any collection of modules that is not included in the standard library. These packages are developed by individuals or organizations outside of the Python Software Foundation. They are made available through package repositories such as the Python Package Index (PyPI). The flexibility of third-party packages allows developers to cater their software solutions to specific needs without reinventing the wheel. By leveraging these packages, developers can save time and effort while benefitting from the community’s expertise and innovations.
Data Manipulation Packages
Data manipulation is a fundamental aspect of data analysis. Among the most sought-after tools for this purpose are Pandas and NumPy.
Pandas
Pandas is designed specifically for data manipulation and analysis. Its primary feature is the DataFrame object, which allows for the easy handling of structured data. This is especially useful in data cleaning and transformation tasks.
Pandas stands out due to its ability to manipulate large datasets seamlessly. It offers robust functionality for filtering, aggregating, and merging data sources. Users find it beneficial because it simplifies complex data operations. However, users should be cautious about memory usage when dealing with very large datasets, as it may lead to performance issues.
NumPy
NumPy is essential for numerical computing in Python. It introduces support for large, multi-dimensional arrays and matrices, along with a comprehensive library of mathematical functions. This package is often used in tandem with Pandas.
The key characteristic of NumPy is its speed. Computation with NumPy arrays is significantly faster than using standard Python lists. This efficiency makes it a popular choice for data scientists. However, learning to use NumPy effectively requires understanding its array-oriented structure, which may pose a challenge to beginners.
Data Visualization Packages
Effective data visualization is vital for interpreting data insights. Matplotlib and Seaborn are two of the most prominent packages in this field.
Matplotlib
Matplotlib is a versatile plotting library that provides a wide range of visualizations, from simple line plots to complex 3D representations. Its customization options are extensive, allowing users to create tailored visualizations.
One of the advantages of using Matplotlib is its flexibility in generating static, animated, and interactive plots in Python. However, the learning curve can be steep for new users, especially when aiming for high-quality visuals. Proper configurations can lead to more intuitive plots but may require time to master.
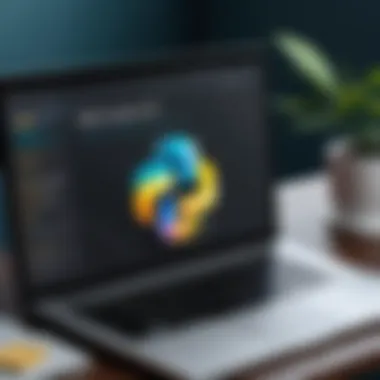
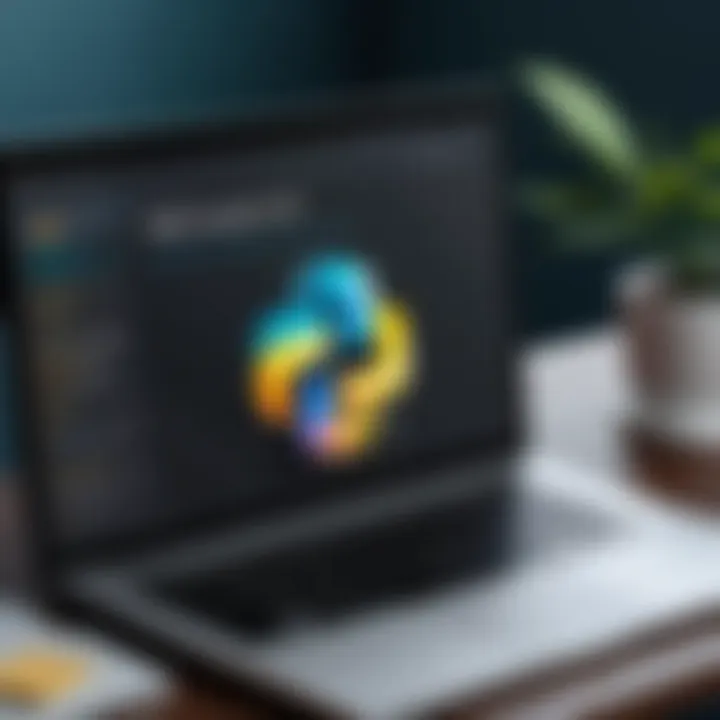
Seaborn
Seaborn builds on Matplotlib and introduces additional enhancements for statistical data visualization. It features a more attractive default style and offers built-in themes, making it easier to create appealing graphics.
The unique feature of Seaborn is its ability to simplify the process of creating complex visualizations. It integrates well with Pandas, allowing for seamless usage with DataFrames. On the downside, it may be less flexible than Matplotlib for certain specialized plots, which may limit some advanced visualization tasks.
Machine Learning Frameworks
Machine learning is a prominent field benefitting from various Python packages. Among the top frameworks are scikit-learn, TensorFlow, and PyTorch.
scikit-learn
Scikit-learn focuses on providing a simple and efficient tool for data mining and machine learning tasks. It supports various supervised and unsupervised learning algorithms. Its simplicity and ease of use makes it popular among beginners in machine learning.
A key characteristic of scikit-learn is its consistent interface for different types of models. This uniformity simplifies switching between algorithms during experimentation. However, it may not be the best choice for deep learning tasks, as its core functionality is designed primarily for traditional machine learning methods.
TensorFlow
TensorFlow is a powerful library for deep learning applications. It allows users to build complex neural networks with minimal effort. The package provides extensive tools for model deployment, making it suitable for both research and production environments.
One of TensorFlow's significant advantages is its scalability. It can handle a massive amount of data across distributed systems. However, its complexity can be a barrier for beginners trying to grasp its full capabilities.
PyTorch
PyTorch has gained recognition for its dynamic computation graph, which makes it easier to work with. This feature allows developers to change the network behavior on-the-fly, which provides considerable flexibility during model building.
The community around PyTorch is vibrant, and it is frequently used in academic research. However, its performance in production compared to TensorFlow can be less optimal in some cases, leading developers to carefully choose which framework suits their needs better.
Web Development Packages
Python’s strong presence in web development is supported by frameworks like Flask and Django.
Flask
Flask is a micro-framework that is lightweight and easy to set up, ideal for smaller applications. It allows developers to start with essential components and expand as necessary. This flexibility is one of its most attractive features, allowing for rapid development.
Although it provides simplicity, Flask does not include many features out-of-the-box, which could result in more time spent coding. However, its modular nature makes it suitable for building custom applications.
Django
Django is a full-fledged web framework that follows the “batteries included” philosophy. This means it comes with many built-in features, such as authentication, database ORM, and admin dashboards.
Django’s strength lies in its ability to promote rapid development and clean design. This framework is particularly beneficial for larger applications. However, its complexity can be overwhelming for beginners, and it may impose more structure than desired in certain projects.
Overall, popular third-party packages are an essential aspect of Python, significantly enriching its ecosystem. Each package contributes specific strengths, catering to a variety of user needs. Understanding these tools allows developers to harness Python's potential more effectively.
Package Management Tools
Understanding package management is crucial for any Python programmer. These tools simplify the process of installing, upgrading, and managing packages, which are essential components of Python programming. Using the right package management tool can significantly enhance productivity and allow for better project organization, which in turn culminates in more efficient coding practices.
Prolusion to Pip
Pip is the most widely used package management system for Python. It allows users to install and manage software packages written in Python. Pip is included with Python installations from version 3.4 onward, making it readily accessible to most users.
Benefits of Pip include:
- Simplicity: The command-line interface is designed to be intuitive. Installing a package can often be done with a simple command like .
- Dependencies Handling: Pip automatically manages package dependencies, ensuring that all necessary packages are installed to run your desired software.
- Wide Adoption: Being the default package manager, it has a large community and a vast repository of packages available on the Python Package Index (PyPI) that can be easily accessed and installed.
Using Virtual Environments
Virtual environments are an important concept when working with Python packages. They provide a way to create isolated environments for different projects, which can help avoid conflicts between package versions.
In simple terms, using a virtual environment allows you to manage project dependencies on a per-project basis. This means you can run multiple projects on the same machine without the risk of package version conflicts.
Key points to remember about virtual environments:
- Separation of Projects: Each virtual environment is independent, so you can use different versions of packages for different projects.
- Easy Management: Tools like or can help in creating these environments effortlessly.
- Environment Activation: Users need to activate the virtual environment before they can work in it. Commands like put you into the specific environment.
Conda Package Management
Conda is another powerful package manager that is often used in data science. Unlike Pip, which is focused predominantly on Python packages, Conda can manage packages from different languages and even dependencies across programming languages. This makes it particularly useful for data science projects that may involve packages from R, Ruby, and others.
Why to consider using Conda:
- Cross-Language Management: It handles packages and dependencies not just from Python, but across various programming languages.
- Environment Management: Conda allows easy creation and management of environments. With its integrated tools, isolating projects becomes seamless.
- Comprehensive Package Repository: The Anaconda repository includes numerous packages that are often used in scientific computing, data analysis, and machine learning, providing users with a rich resource.
In summary, a strong understanding of package management tools like Pip and Conda is vital for efficient Python development. By managing packages properly, programmers can enhance project organization and simplify the coding process, allowing for more focus on solving problems rather than dealing with dependencies.
Developing Custom Packages
In the context of Python programming, developing custom packages allows for the creation of reusable components tailored to specific needs. This process is crucial for efficient coding and streamlined project management. When you create a custom package, you encapsulate functionality that can be shared across different scripts or projects. This promotes not just code reusability but also easier debugging and testing of components in isolation.
Custom packages can address unique application requirements that might not be sufficiently met by existing packages. They let programmers structure their code logically and organize it in a way that reflects its functionality. As you grow your coding skills, understanding how to develop packages becomes essential. Python's package development framework encourages clean coding practices, which in turn accelerates both development speed and collaboration with others.
Creating packages also aids in maintaining consistency across projects. When a package is well-structured and well-documented, it serves as a reliable foundation for future projects. Moreover, effective package development can significantly improve project delivery times, especially in larger teams where multiple developers work in parallel.
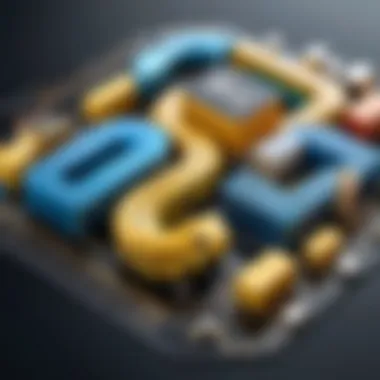
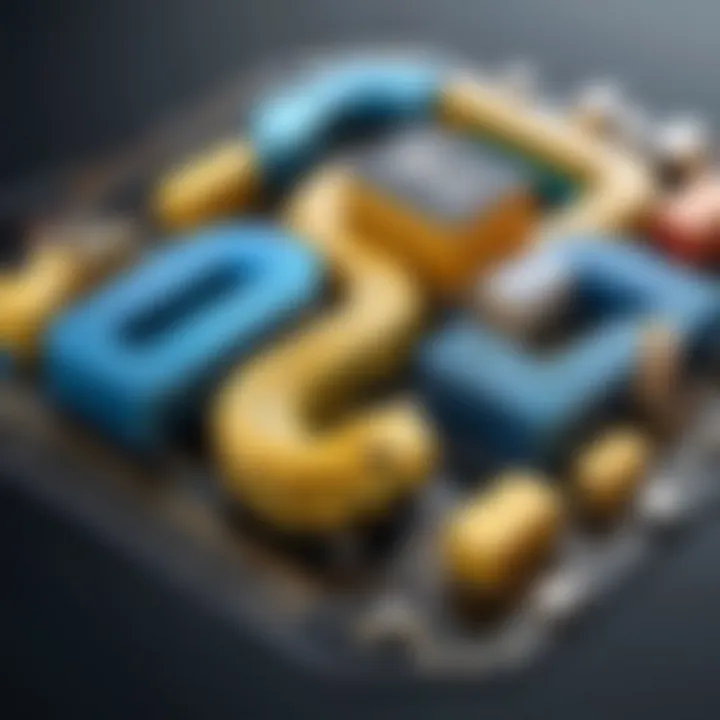
Creating a Basic Package
Creating a basic Python package is straightforward. The following steps guide you through the process:
- Create a Directory: Start by creating a directory that matches your package name. This acts as the root for your package.
- Initialize the Package: Inside this directory, add an file. This file indicates that this directory should be treated as a package. You can leave it empty, or use it to initialize the package by including relevant code.
- Write Your Code: Create additional Python files (.py) inside your package directory. Each file can contain functions or classes that form the core functionality of your package.
- Package Metadata: For better organization, include a file in your package's root. This file contains metadata like the package’s name, version, and any dependencies required to run it.
- Installation: To install your package locally, run the following command in the terminal from your package directory:This command tells Python to install the package from the current directory.
By following these steps, you will have a basic package set up that can be used in other Python projects or distributed to others.
Best Practices for Package Development
Developing a custom package is not simply about coding; it involves adhering to certain best practices that can enhance code quality and user experience. Here are key practices to keep in mind:
- Modular Design: Keep functionality separate and organized. Each module should handle a distinct aspect of functionality to prevent complex interdependencies.
- Documentation: Provide comprehensive documentation. Use docstrings to explain what each module, function, or class does. This is essential for both users and future maintainers.
- Version Control: Employ version control systems like Git. Maintain separate branches for development, testing, and production code to manage changes efficiently.
- Testing: Implement unit tests to validate that each component of your package works correctly. Consider using frameworks like or for this purpose.
- Compliance: Follow Python community conventions for code style. Adhering to the PEP 8 guidelines increases the readability of your code.
- Distribution: Plan for easy distribution and installation. Utilize tools like to package your project into a format compliant with the Python Package Index (PyPI).
Developng custom packages not only enhances your coding skillset but also opens the door to contributing to the Python community, allowing others to benefit from your work.
Implementing these best practices will make your packages robust, maintainable, and easier for others to utilize. A well-developed package will stand the test of time and adaptability as your programming journey evolves.
Exploring Package Documentation
Documentation serves a critical role in the overall experience when working with Python packages. It is not just a supplementary aspect but rather a cornerstone that shapes how effectively a user can utilize a package. A well-structured documentation provides clarity on the functionalities, usage examples, and best practices associated with a package. For individuals, especially those who are new to programming, effective documentation can lead to better understanding and ease of use. It reduces the learning curve and minimizes errors that may arise from misunderstanding how a function or class works.
Furthermore, documentation enhances the maintainability of code by providing insights into how packages interact with one another. In an ever-evolving software landscape, knowing how to reference documentation efficiently can be a game-changer for developers and data scientists alike. Therefore, exploring package documentation is essential for anyone looking to maximize the utility of the Python programming ecosystem.
The Role of Documentation
Documentation facilitates interaction between the user and the package itself. It outlines functionalities and provides clear examples that aid programmers in implementing them. Without this essential guidance, users may struggle to remember the syntax or find ways to apply various functions. In complex scenarios, well-written documentation can also highlight edge cases or common pitfalls, thus helping to prevent bugs or unexpected behavior in applications.
In essence, documentation serves multiple roles: it is a reference guide, an educational tool, and a support system for users as they navigate the intricacies of Python packages. Every example or usage note can mean the difference between success and frustration when one is working with a package.
Finding Package Documentation Online
ReadTheDocs
ReadTheDocs is a platform designed to host documentation for various projects, particularly open-source ones. It is widely used because it simplifies the process of accessing package documentation. The key characteristic of ReadTheDocs is its automatic generation of comprehensive documentation from the code repositories. This means that any changes in the code can be seamlessly reflected in the documentation.
One unique feature of ReadTheDocs is its ability to allow users to read documentation for different versions of a package. This facility is beneficial because it lets users understand changes across versions, which is crucial for maintaining compatibility in ongoing projects. However, it may occasionally suffer from inconsistencies if the documentation is not carefully maintained alongside the code changes.
Official Package Repositories
Official package repositories, such as the Python Package Index (PyPI), play a significant role in distributing Python packages. They typically include links to documentation on the package's own website, fostering a direct connection between the package and its instructional materials. A key characteristic of these repositories is the ease of access they provide. Users can quickly search for packages and find the relevant documentation in one location.
A unique feature of official repositories is the inclusion of user feedback and download statistics, which adds a layer of trustworthiness to the packages listed. This can help users assess the popularity and reliability of a package based on community engagement. However, because the documentation varies by package, the quality can be inconsistent and some developers may not provide adequate instructions.
Choosing the Right Package
Choosing the right package is essential for effective Python programming. The multitude of available packages can often lead to confusion. A well-chosen package can significantly enhance development speed and application efficiency. Conversely, selecting an incompatible or poorly-maintained package may result in wasted time and resources. Understanding the nuances of package selection is critical for any programmer, regardless of skill level.
Assessing Application Needs
The first step in choosing a package is to carefully assess the specific needs of your application. Consider what functionalities are essential for your project. Does it need data manipulation, or will it require visualization features? For instance, if you are dealing with complex numerical data, using NumPy might be the best choice. If your focus is on creating charts and graphs, Matplotlib would be more pertinent.
Additionally, think about how the package integrates with the existing system. It is important to ensure compatibility with your current tools and languages. Make a list of necessary features and compare them with the capabilities offered by various packages. Ultimately, aligning your package choice with your application requirements sets a solid foundation for project success.
Evaluating Package Performance
Once you narrow down your choices, the next step is to evaluate package performance. This can include several factors such as speed, memory usage, and scalability. You may want to look for benchmarks or performance tests that compare similar packages. For example, if you are choosing between TensorFlow and PyTorch for a machine learning project, investigate their performance metrics on similar tasks to guide your decision. Additionally, consider how well the package handles large datasets and its efficiency in resource management.
Performance also ties into responsiveness. If a package has a history of being slow or inefficient, it may hinder development. Therefore, gathering empirical evidence about a package's performance from multiple sources can offer reassurance as you finalize your choice.
Community Support and Maintenance
Finally, community support and ongoing maintenance play a significant role in the longevity and reliability of a package. A well-supported package usually has a strong community around it. This ensures that regular updates are issued and that bugs are addressed promptly. Thus, it is wise to check the package's repository on GitHub, for example, to see its update frequency and how actively issues are resolved.
Packages with abundant community support often provide extensive documentation, tutorials, and forums. These resources can help you troubleshoot issues during development and improve your understanding of the package.
On the other hand, if a package is rarely updated or has low community engagement, it may become obsolete quickly. Consequently, always assess the health of the community surrounding a package before making a final decision.
"A good package is like a sound investment; it pays off over time if chosen wisely."
In summary, thorough consideration of your application's needs, capable performance evaluation, and the stability of community support are pivotal components in selecting the right Python package. These steps can guide you towards making informed decisions, ultimately leading to successful project development.
Finale
In this article, we explored the intricate world of Python packages. It is important to recognize how these packages can elevate programming practices. They allow for integration of complex functionality without reinventing the wheel. This not only saves time but also enhances productivity.
Recap of Key Points
Throughout the discussion, we have highlighted several critical aspects, such as:
- Definition and Importance: Packages are a fundamental part of Python, enabling code reusability and modular program design.
- Standard Library vs. Third-Party Packages: Each has its own role in the ecosystem. The standard library provides essential tools, while third-party packages expand capabilities further.
- Package Management Tools: Tools like Pip and Conda are key in handling installations and dependencies efficiently.
- Developing Custom Packages: Creating tailored packages fulfils specific project needs, which fosters innovation and customization.
- Documentation and Support: Good documentation is crucial for effective use of any package, and community support plays a significant role in its sustainability.
- Choosing the Right Package: Depending on application needs, evaluating performance, and ensuring proper support are vital steps in package selection.
Future Trends in Python Packages
The landscape of Python packages is continually evolving. Several trends are worthwhile to note:
- Increased Automation: As machine learning advances, automation in packaging and distribution of Python applications will likely become more common.
- Focus on Data Science: More packages are emerging to cater to data analytics, driven by the growing demand for data scientists.
- Integration with Cloud Services: Packages that facilitate easy integration with platforms such as AWS or Azure are becoming more prevalent.
- Improved Community Platforms: The rise of collaborative platforms like GitHub allows easier access to packages and fosters more robust community support.
In summary, understanding Python packages can create a significant impact on programming efficiency and capability. As new technologies emerge, keeping updated with the latest packages and their trends will be crucial.