Practical Python Projects for Beginners
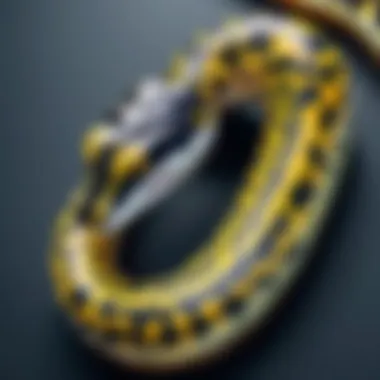
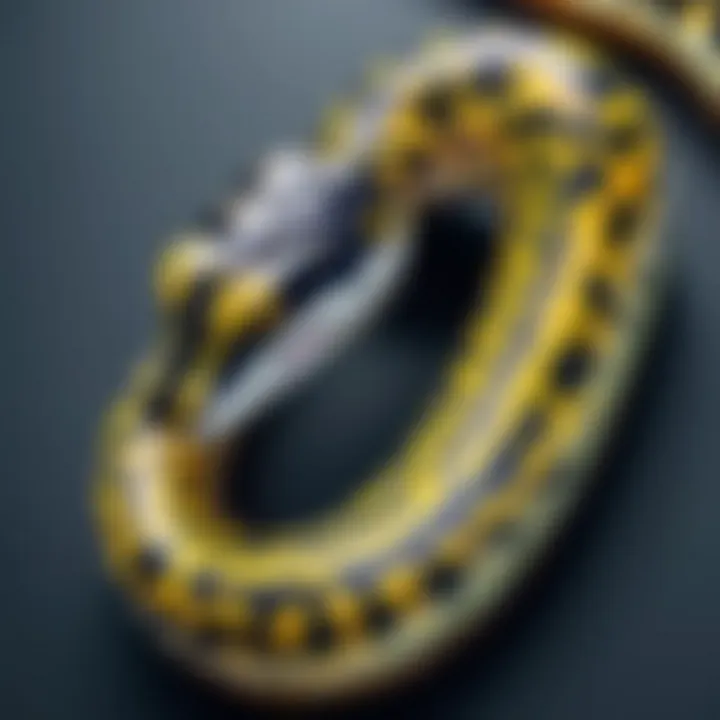
Overview of Topic
Learning Python is a journey many embark upon due to its simplicity and versatility. Python is not just a programming language; it serves as a powerful tool in various fields, from data analysis to web development. Understanding the practical applications of Python can help beginners grasp how this language integrates into real-world scenarios. This exploration will outline key areas where Python can be effectively utilized, showcasing projects and concepts to enhance both learning and technical skills.
The significance of Python in the tech industry continues to grow due to its applicability in emerging technologies, data science, and automation. As more industries adopt data-driven decision-making, the demand for skilled Python developers increases correspondingly. Thus, comprehending these applications is critical for anyone beginning their journey in programming.
A brief history of Python reveals its inception in the late 1980s, with a release in 1991. Since then, Python has evolved significantly, with contributions from a vast global community.
Fundamentals Explained
At its core, Python embodies principles of simplicity and readability. This makes it an ideal language for beginners. Key terminology such as 'syntax', 'variables', and 'data types' is essential for understanding how to code effectively. Knowledge of these foundational concepts provides a framework upon which more complex applications can be built.
Some basic concepts to grasp include:
- Variables: Containers for storing data values.
- Data Types: Different kinds of data, such as integers, strings, and lists.
- Control Structures: Instructions that control the flow of the program, like loops and conditionals.
Practical Applications and Examples
Real-world applications of Python demonstrate its versatility and power. Here are several areas where beginners can engage in practical projects:
Data Manipulation
Using libraries such as Pandas and NumPy, beginners can work with large datasets to clean and manipulate data. A common beginner project can involve analyzing a CSV file and generating useful summaries.
Web Development
Frameworks like Flask and Django allow beginners to create web applications quickly. For instance, building a simple blog can introduce users to HTML, CSS, and the essence of web interactivity.
Automation
Python excels in automating repetitive tasks via scripting. A beginner could create a script that automates the renaming of multiple files in a directory, demonstrating real-world workflow efficiencies.
Here’s a code snippet for renaming files in a folder:
Tips and Resources for Further Learning
For those keen on expanding their Python knowledge, several resources are invaluable. Some recommended materials include:
- Books: "Automate the Boring Stuff with Python" by Al Sweigart is great for practical projects.
- Online Courses: Websites like Coursera and edX offer structured learning paths.
- Communities: Joining forums on Reddit or participating in Facebook groups can provide support and additional resources.
Engagement with the broader community is essential for growth and understanding. The exchange of ideas can lead to enhanced learning and collaboration.
By applying Python in meaningful ways, beginners can not only build foundational skills but also prepare for more complex challenges. The journey ahead is filled with opportunities to innovate and create impactful solutions.
Prelude to Python
Python has emerged as a predominant programming language in various fields including data science, web development, automation, and more. This section aims to present the essential role that Python plays in today’s technology landscape, especially for beginners looking to harness its power and functionality. The ability to use Python for diverse applications makes it an attractive choice for anyone interested in coding.
The Significance of Python in Today's Tech Landscape
The tech industry is constantly evolving, and with that evolution comes a demand for versatile programming languages. Python stands out due to its ease of learning and readability, which are crucial for beginners. According to a survey by Stack Overflow, Python ranked as one of the most loved languages among programmers. This popularity can be attributed to the following factors:
- Wide Adoption: Many leading companies, like Google and Netflix, utilize Python for different projects. This indicates its robustness and reliability.
- Community Support: The large community of Python developers means there is a wealth of resources, tutorials, and forums available for assistance.
- Versatility: Python can be used in web development, automation, and data science, making it a jack-of-all-trades.
"Python is powerful and fast; plays well with others; runs everywhere; is friendly & easy to learn; is Open." – Python's official website
Understanding the significance of Python will help beginners see the potential paths they can take as they dive into this language.
Python's Features and Benefits for Beginners
Diving into Python, beginners encounter a language that not only is approachable but also comes with a significant set of features that enhance their learning experience. Some notable features include:
- Simple Syntax: Python’s syntax resembles natural language, which simplifies the learning process. This allows beginners to focus on concepts rather than struggling with complicated syntax rules.
- Comprehensive Libraries: Python offers extensive libraries such as NumPy, Pandas, and Matplotlib which cater to diverse functionalities, allowing beginners to unlock advanced capabilities without much hassle.
- Interpreted Language: Since Python is interpreted, code can be run directly in the interactive shell, facilitating quick testing and iteration.
- Cross-platform Compatibility: Python is available on various operating systems like Windows, macOS, and Linux, enabling learners to work seamlessly across platforms.
These features create a solid foundation for beginners, making Python not just a language to learn but a gateway to multiple opportunities in tech.
Setting Up Your Python Environment
Setting up your Python environment is a critical first step for anyone beginning their journey with this versatile programming language. Whether you're aiming to tackle data analysis, web development, or automation tasks, it is essential you have the right setup. A well-configured environment streamlines your workflow and enhances your learning by providing the necessary tools at your fingertips.
Installation of Python
To start, you must install the Python programming language. This process can seem daunting for beginners, but it is straightforward. You can download the latest version of Python from the official Python website. Ensure you choose the right version for your operating system, whether it’s Windows, macOS, or Linux.
To install Python on Windows:
- Download the installer from Python's official website.
- Run the installer, and make sure to check the box that says "Add Python to PATH."
- Follow the on-screen instructions to complete the installation.
On macOS and Linux, the installation often involves using the terminal. For macOS users, you can also consider using Homebrew, a package manager that simplifies installations.
After installation, you can verify whether Python is installed correctly by opening your terminal or command prompt and running the command:
This should return the installed version of Python, confirming a successful installation.
Choosing an Integrated Development Environment (IDE)
The next step involves selecting an Integrated Development Environment (IDE), which is crucial for writing and testing your Python code efficiently. An IDE provides a user-friendly interface and various tools like code suggestions, debugging capabilities, and syntax highlighting. Beginners may find IDEs such as PyCharm, Visual Studio Code, or Jupyter Notebook particularly advantageous.
- PyCharm: Highly popular among Python developers, it offers powerful features, though the community version is sufficient for beginners.
- Visual Studio Code: A lightweight and customizable editor that supports many programming languages, including Python, via extensions.
- Jupyter Notebook: Ideal for data science projects and exploratory programming, as it allows for inline code execution and visualization.
Choosing your IDE may depend on your specific focus area. If you are inclined towards data analysis, Jupyter Notebook could be your best bet. Alternatively, if you aim for software development, you might prefer PyCharm or Visual Studio Code.
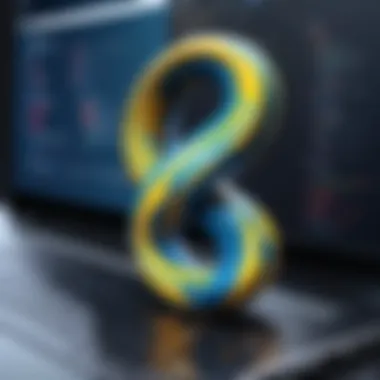
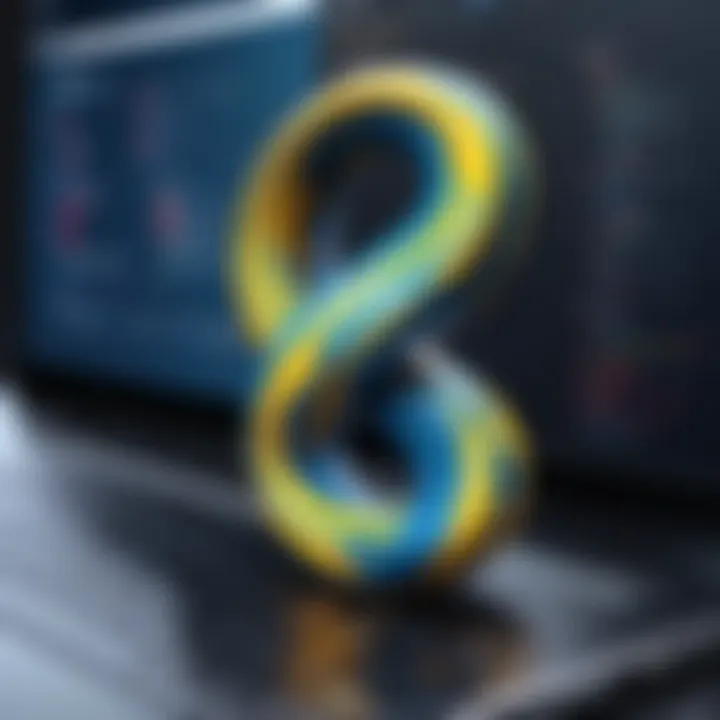
Essential Packages and Libraries to Install
Once Python and an IDE are set up, the next step is to install essential packages and libraries. Python's extensive library infrastructure is one of its core strengths, allowing you to accomplish a wide range of tasks. Some critical libraries to consider for beginners include:
- NumPy: For numerical computing and data manipulation.
- Pandas: This library is pivotal for data analysis and manipulation, offering features for handling structured data.
- Matplotlib: Primarily used for data visualization, allowing you to create a variety of static, animated, and interactive plots.
- Flask: A micro web framework for building web applications quickly and easily.
To install any of these packages, you can use Python's package manager, pip. Run the following command in your terminal:
Replace with the name of the library you wish to install.
Setting up your Python environment might seem like a minor detail, but it lays the groundwork for effective learning and development. Each component plays an integral role in the process, enabling you to explore the wide-ranging applications of Python.
Basic Python Concepts for Beginners
Understanding fundamental Python concepts is essential for beginners. Mastering these basics lays the groundwork for more advanced programming skills. A solid grasp of variable usage, data types, control structures, and functions ensures that students can effectively write and troubleshoot Python code. These foundational elements clarify programming logic, enabling learners to develop practical applications in their projects. They also enhance comprehension when diving into specialized areas like data science or web development.
Understanding Variables and Data Types
Variables are the starting point in programming. They allow us to store data values. In Python, a variable is created by assigning a value to a name. For example:
In this example, and are variables. The data types of variables can vary. Python supports several data types, including integers, floats, strings, and booleans.
- Integers: Whole numbers, positive or negative.
- Floats: Decimal numbers, such as 9.99.
- Strings: Text data, enclosed in quotes.
- Booleans: Represents one of two values, True or False.
Selecting the correct data type is crucial for efficient program use, as operations can differ across types. This selection directly impacts memory usage and performance.
Control Structures: Conditionals and Loops
Control structures govern the flow of a program. Conditionals allow execution of certain statements based on specific conditions. The most common conditional is the statement:
This code evaluates if is 18 or older before printing the message. If the condition is not met, no message appears.
Loops are also essential for repeating actions. The loop iterates over a sequence, while the loop continues as long as a condition holds. For example:
This loop prints numbers from 0 to 4. Control structures facilitate decision-making and repetitive tasks, which are vital in programming.
Functions and Scope
Functions allow for code modularity. A function is a block of code defined to perform a specific task. They enhance code organization and reusability. For instance:
This function takes a parameter and returns a greeting. Functions help in reducing redundancy in code.
The concept of scope dictates the visibility of variables in programming. A variable defined within a function is not accessible outside of it. This principle helps to maintain clean and manageable code. Proper understanding of functions and scope ensures effective program structure and design.
"Mastering basic concepts build the real foudation for advanced skills."
In summary, Python's basic concepts are vital for beginners. Proficiency in variables, control structures, and functions sets students on the right path. Knowing how to manipulate data effectively aids in creating significant projects. Python's design simplicity further amplifies these benefits, allowing developers to focus more on problem-solving than on complex syntax.
Projects for Beginners
Engaging with projects is an essential component of the learning process for those delving into Python. Projects not only solidify theoretical concepts, but they also allow beginners to apply their skills in practical scenarios. This hands-on approach fosters a deeper understanding of programming principles and helps in reinforcing what has been learned. By working on projects, learners can build a portfolio that demonstrates their capabilities.
In the realm of Python, there are numerous projects suitable for beginners. Each project challenges students to think critically and creatively, pushing them to find solutions to real-world problems. Importantly, these projects can be tailored to an individual's interests, enhancing motivation and engagement. Moreover, completing projects contributes not just to skill development but also to confidence building, as learners witness their ideas taking shape.
Building a Simple Calculator
A simple calculator is often one of the first projects undertaken by beginners. It involves the basic operations such as addition, subtraction, multiplication, and division. By embarking on this project, learners can grasp fundamental concepts like user input, data types, and functions. A calculator's design prompts students to think about how to structure their code effectively.
For instance, the flow of the program might look like this:
- Request input from the user for the two numbers and the operation.
- Perform the operation based on user input.
- Display the result.
This end-to-end approach will not only teach new coders about the arithmetic logic but will also introduce them to functions. This basic project thus forms a solid foundation for more complex programming tasks.
Creating a To-Do List Application
A to-do list application serves as a practical project that combines user interaction with data management. In this project, learners create an interface for users to add, delete, and view tasks. This task improves understanding of lists and loops, as students will need to manage multiple items within their code.
Moreover, this project can be expanded with features such as prioritizing tasks or marking them as completed. Enhancing a simple to-do list not only broadens the scope of learning but also encourages personal creativity. This further lays the groundwork for understanding real-world applications and software design.
Investment Tracker Using Python
Developing an investment tracker allows beginners to engage directly with financial concepts and data manipulation. This project typically includes features for tracking income, expenses, and calculating profits or losses. By working on an investment tracker, learners are also exposed to libraries such as Pandas, facilitating hands-on experience with data analysis.
Data visualization might also become an integral part of this project, adding layers of complexity and learning opportunities. Students can understand how to manage larger datasets effectively and illustrate financial trends, honing their ability to work with real-world financial problems.
Weather Application with API Integration
Creating a weather application introduces beginners to the world of APIs, an invaluable skill in modern programming. This project involves fetching data from a weather service and displaying it to the user in a readable format.
Through this task, learners can develop an understanding of JSON data formats, handling requests, and managing application logic. Additionally, the project can be expanded to include features like location-based services or alerts for severe weather. This adaptability showcases the versatility of Python and its practical applications.
Engaging with these beginner projects not only builds foundational skills but also enhances critical thinking and problem-solving abilities.
In summary, working on practical projects is vital for beginners as it bridges the gap between theory and real-world application. Projects such as building a calculator, creating a to-do list application, developing an investment tracker, or a weather application with API integration serve as invaluable learning milestones in the journey of mastering Python.
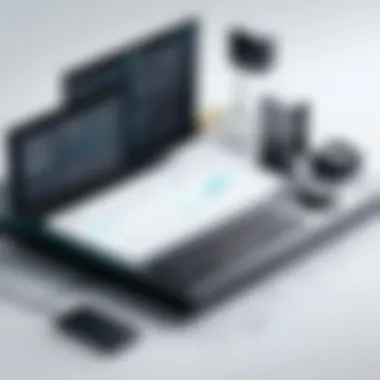
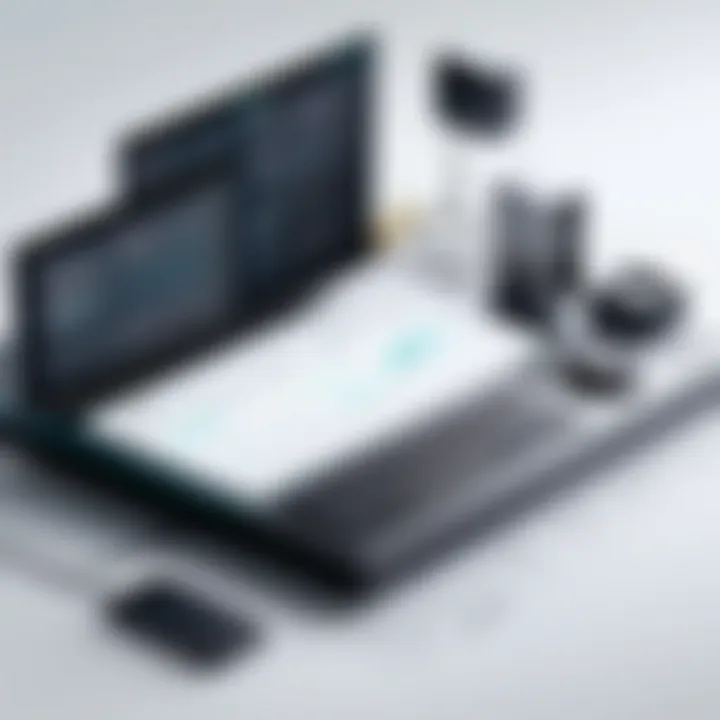
Data Handling with Python
Data handling is a crucial aspect of modern programming, especially in the era of big data. As Python becomes increasingly popular among data scientists and analysts, understanding its capabilities in handling data is essential. This section explores how Python can be utilized for efficient data manipulation, analysis, and visualization. By mastering these skills, beginners can significantly enhance their technical repertoire.
Intro to NumPy for Data Manipulation
NumPy is a powerful library often regarded as the backbone of data manipulation in Python. It provides numerous functions and tools that allow users to work with multi-dimensional arrays and perform mathematical operations seamlessly. The primary advantage of using NumPy is its ability to handle large datasets more efficiently than traditional Python lists.
Key benefits of using NumPy include:
- Performance: Operations on NumPy arrays are significantly faster than operations on native Python lists due to its optimized C backend.
- Functionality: NumPy provides a vast array of mathematical functions, enabling complex calculations on entire datasets without the need for loops.
- Convenience: With its easy-to-use syntax, NumPy simplifies complex data tasks.
Here is a basic code snippet to demonstrate creating a NumPy array:
This simple example illustrates how easy it is to create an array using NumPy, setting the stage for more advanced manipulations.
Working with Pandas for Data Analysis
Pandas is another staple library in the realm of data analysis, particularly known for its data frame functionality. It provides tools that enable users to manipulate and analyze structured data efficiently. Understanding Pandas is essential for anyone looking to perform data analysis with Python.
Benefits of working with Pandas include:
- Data Frame Structure: Similar to a spreadsheet, a data frame allows for labeled axes and the organization of data into columns and rows.
- Data Handling Options: Pandas makes it easy to read and write data from various formats, including CSV, Excel, and SQL databases.
- Data Manipulation: With Pandas, you can easily filter data, perform group operations, and handle missing values, which is often a necessary step in the preprocessing phase.
Here’s a short example of how to create a data frame using Pandas:
This example outlines the simplicity of creating a data frame, showcasing the power of Pandas for structured data analysis.
Data Visualization Using Matplotlib
Matplotlib is a widely used library for data visualization in Python. It enables users to create static, interactive, and animated visualizations in Python, making it easier to showcase data findings clearly. Data visualization plays a fundamental role in analytics, as it can help stakeholders understand trends and insights at a glance.
Utilizing Matplotlib offers several advantages:
- Variety of Plots: Users can create a range of plots such as line charts, bar graphs, and scatter plots, all customizable to suit specific presentation needs.
- Integration: Matplotlib works seamlessly with NumPy and Pandas, allowing for effective visualization of data directly from these libraries.
- Aesthetic Control: Fine-tuning plots is straightforward, enabling users to modify aspects like colors, labels, and titles easily.
For example, here’s how to create a simple line plot using Matplotlib:
This basic code demonstrates how to create a simple yet effective visualization, laying the foundation for more complex graphical representations.
"Understanding data handling in Python is essential for anyone looking to excel in data-related fields. Mastery of NumPy, Pandas, and Matplotlib can significantly impact analysis and insights."
Through learning these libraries, beginners can harness Python’s full potential for data handling, thus paving the way for advanced studies and applications in data science and analytics.
Exploring Web Development with Python
Web development has become a crucial skill in today's digital landscape. Python stands out as a versatile tool for both beginners and seasoned developers. This section will focus on the significance of web development with Python, particularly highlighting the ease of use, robust frameworks, and the community support available.
Prolusion to Flask Framework
Flask is a lightweight web framework that allows for quick development of web applications. It is often preferred for its simplicity and flexibility. One of the key advantages of Flask is its minimalistic approach, which lets developers focus on writing the core functionality without being bogged down by unnecessary complexity.
Flask is built on top of WSGI (Web Server Gateway Interface) and Jinja2 templating system, making it an excellent choice for beginners. It provides the essential tools needed to create web applications efficiently. The modular nature allows developers to integrate additional components as needed, promoting scalability.
Creating a Basic Web Application with Flask
To create a web application using Flask, one begins by setting up a development environment. This involves installing Flask via pip and creating a basic directory structure for your project. The fundamental components of a Flask app include the main application file, templates for rendering HTML, and static files for CSS and JavaScript.
Here’s a simple example of a basic Flask application:
In this code, we import Flask, create an application instance, define a route for the home page, and render a template when the route is accessed. Running this application will serve a simple webpage that you build, providing a hands-on introduction to web development in Python.
Understanding RESTful APIs in Web Development
RESTful APIs are essential in modern web applications. They allow different software systems to communicate over the web. Python's capabilities make it easy to build and consume RESTful APIs. A RESTful API works through standard HTTP methods such as GET, POST, PUT, and DELETE, enabling seamless interactions between clients and servers.
In Flask, creating a RESTful API is straightforward. By defining routes that correspond to different HTTP methods, you can handle requests and send responses effectively. For example, an API endpoint can be created to manage a collection of resources like users or products.
Key Points:
- Flask is great for beginners in web development.
- RESTful APIs enable effective communication between systems.
- Hands-on projects help solidify learning and understanding.
Getting Started with Game Development
Getting started with game development is crucial for beginners looking to apply their Python skills practically. This area offers an exciting blend of creativity and technicality. It allows learners to not only enhance their programming abilities but also to engage in problem-solving and critical thinking. Playing games is often intuitive, but creating them requires understanding various concepts and frameworks.
In this section, we will dive into the significance of game development with Python, especially through the use of the Pygame library, which simplifies many aspects of development. Python's simplicity and readability make it a suitable choice for newcomers in programming and game creation. Furthermore, developing games provides tangible projects that show progress, which can be motivating for learners. Here, the focus will be on practical applications that reinforce theoretical concepts.
Preamble to Pygame for Game Development
Pygame is a widely-used library designed for game development in Python. It provides easy access to graphics, sound, and more. By utilizing Pygame, beginners can concentrate on game design and logic without getting bogged down by complex code. The package supports 2D graphics, allowing developers to create diverse and engaging games.
The importance of Pygame lies in its user-friendly approach. With Pygame, newcomers can learn about game loops, handling events, and managing graphics and sound with less effort. Pygame also has a strong community and plenty of tutorials available online to help learners get started.
With Pygame, novices can develop games step by step, enhancing not only their coding skills but also their creativity. Considering the vast demand for mobile and computer games, learning to use Pygame equips beginners with marketable skills and provides a platform to showcase their creations.
Creating a Simple Game: Step-by-Step Guide
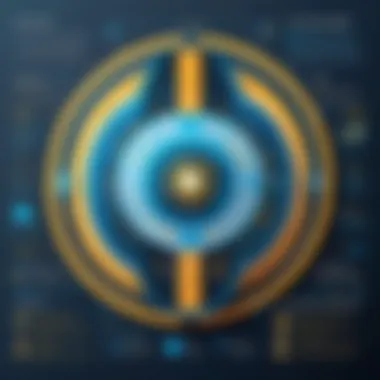
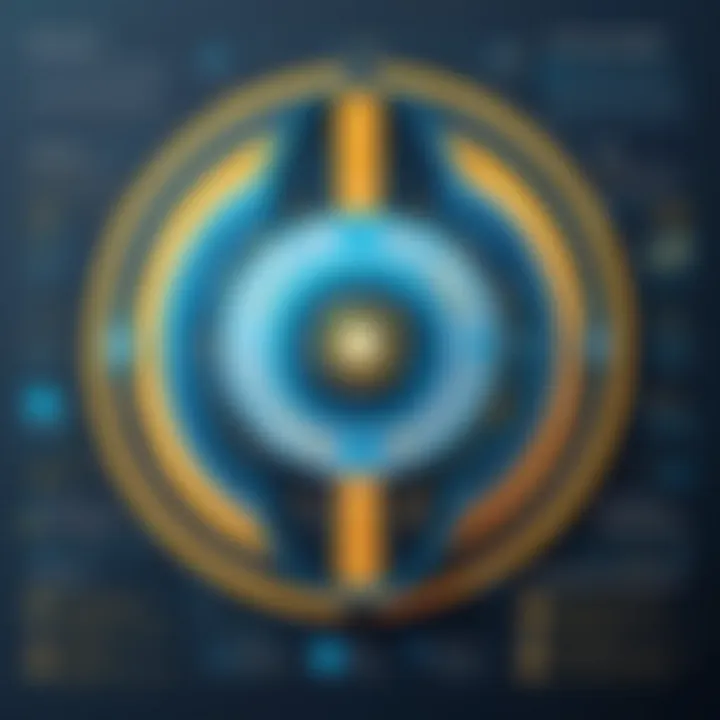
Creating a simple game is an excellent way to practice programming in Python. This process will walk you through some essential components while providing hands-on experience with Pygame.
- Setting Up Pygame: First, install Pygame if it is not already on your system. This can be done via pip using the command:
- Initialize Pygame: At the start of your script, initialize Pygame with the following code:
- Create a Game Window: Define dimensions for your game window and create it:
- Game Loop: Implement the main game loop to keep the game running. This loop handles events and updates the display:
- Game Logic: Within the game loop, you can start to add simple graphics, text, and game characters. Use images for sprites or design basic shapes.
- Ending the Game: To exit the game properly, include the cleanup code after the loop:
By following these steps, beginners not only learn how to code but also understand how game design works, focusing on player interaction and experience. Each game developed adds to the learner's portfolio and demonstrates their growing expertise in Python.
Automation Scripts with Python
Automation is an essential aspect of programming, especially for beginners who want to enhance their skills and efficiency. In this section, we explore how Python can be utilized to create automation scripts. Automating repetitive tasks can save time and reduce human error, which is valuable in both personal and professional settings.
Python's simplicity and robust libraries make it a preferred choice for automation. From file management to web scraping, the capabilities of Python span across various tasks, providing a practical foundation for learners. Understanding automation will not only strengthen programming skills but also introduce concepts relevant to real-world applications.
Using Python for File Management Automation
File management can become tedious if done manually. Python’s built-in libraries, such as and , enable users to automate these tasks efficiently.
Here are some common tasks that can be automated:
- File Organization: Automatically moving files into designated folders based on file types.
- Backup Creation: Scheduling automated backups of important files to prevent data loss.
- Deleting Unneeded Files: Setting scripts to remove files that are no longer necessary.
To illustrate, here is a basic example of a Python script that organizes files in a directory based on their extension:
This script categorizes and files into separate folders. It utilizes to list files and for moving them. It's simple, yet demonstrates the power of automation.
Automating Web Scraping Tasks
Web scraping is another domain where Python shines. It allows users to extract data from websites, enabling the automation of data collection tasks. Libraries like and make web scraping accessible for beginners.
Some typical applications include:
- Data Collection for Analysis: Collecting data for research or analysis.
- Price Tracking: Monitoring prices of products over time to recognize trends.
A fundamental example of a web scraping script is as follows:
This script fetches the HTML content of a webpage and extracts all `` headlines. Understanding the basic structure of web pages is essential when scraping data, as it helps in targeting specific segments of the content effectively.
Automation scripts using Python not only enhance efficiency but also allow beginners to engage with practical coding tasks, reinforcing essential programming skills.
Exploring Data Science with Python
Data science has become an essential field in today's digital economy. As organizations increasingly rely on data-driven decision-making, understanding data science concepts is crucial. Python stands out as a powerful tool for this purpose. It offers a robust ecosystem of libraries and frameworks that facilitate data manipulation, analysis, and visualization. For beginners, the relevance of Python in data science cannot be underestimated. It provides an accessible entry point into complex topics and empowers individuals to tackle real-world challenges.
Python's versatility enables beginners to grasp fundamental concepts without getting overwhelmed by syntax complexities. As one engages in data science projects, crucial skills such as statistical analysis, data cleaning, and visualization are developed. Additionally, grasping data science concepts lays a foundation for advanced studies in fields such as machine learning and artificial intelligence.
"The future of data science lies in its accessibility and the ability to harness effective tools to interpret complex data."
Thus, this section will cover the fundamental concepts of data science and simple machine learning models, equipping readers with necessary skills.
Preface to Data Science Concepts
Data science combines several disciplines, including statistics, computer science, and domain expertise. It aims to extract insights from structured and unstructured data. To begin with, understanding key terminologies is vital. Here are some essential concepts:
- Data Exploration: This involves analyzing data sets to summarize their main characteristics, often with visual methods.
- Data Cleaning: This crucial step involves removing inaccuracies, handling missing values, and correcting errors in the data.
- Model Development: This entails creating algorithms that can predict outcomes or classify data based on historical data.
- Data Visualization: Clear visual representation of data helps in understanding trends, patterns, and outliers.
Being familiar with these concepts enables beginners to navigate the data science landscape effectively. It also prepares them for working with Python libraries like Pandas and Matplotlib, which are widely used in the field.
Implementing Simple Machine Learning Models
Machine learning is a significant aspect of data science. It allows systems to learn from data patterns without being explicitly programmed. Implementing simple machine learning models in Python can be highly beneficial for beginners. Here’s how to get started:
- Define the Problem: Clearly understanding the problem is the first step. It could be classification, regression, or clustering.
- Collect Data: Gather relevant data that relates to your problem. This data can come from various sources, such as APIs or databases.
- Preprocess the Data: Employ necessary techniques to clean and transform the data. This often involves normalization or feature selection.
- Choose a Model: Select a simple model such as Linear Regression for easy initial implementations. Libraries like Scikit-learn make this selection straightforward.
- Train the Model: Use a portion of your data to train the model.
- Evaluate the Model: Test the model against the remaining data to gauge its performance.
- Refine the Approach: Based on evaluation, refine the model parameters or try different algorithms as needed.
Implementing machine learning in Python can seem daunting initially, but with practice, it becomes manageable. Practical experience with simple models will provide a firm grasp of more advanced machine learning techniques in future endeavors.
End and Further Resources
As we conclude this journey through practical applications of Python for beginners, it is essential to reflect on the core principles and benefits discussed throughout the article. This section serves to consolidate the learnings and provide pathways for further exploration. The conclusion is more than just a summary; it encapsulates the importance of continuous learning in Python. For beginners, understanding how to transition from foundational knowledge to real-world applications is critical.
Throughout this article, we covered various essential topics, including data handling, web development, game development, and automation scripts. Each section aimed to provide you with the practical skills required to tackle projects that are meaningful and engaging. Whether it was developing a weather application or automating web scraping tasks, the underlying emphasis was on hands-on experiences.
The benefits of mastering these applications cannot be overstated. Not only do they build technical skills, but they also foster critical thinking and problem-solving abilities, which are highly valued in today’s tech landscape. Moreover, tackling real-world projects enhances your portfolio, making you more attractive to potential employers.
There are several considerations to keep in mind as you continue your Python journey. Staying updated with the latest trends in Python development is important. Engaging with online communities can provide support and new insights.
"Learning Python is not just about understanding the syntax. It’s about applying that knowledge in innovative ways."
Summarizing Key Learnings
In summary, the key points discussed in this article include:
- The significance of Python and its extensive applications in various fields.
- Hands-on projects like simple calculators, web applications, and data analysis, aimed at cultivating a practical understanding.
- The importance of continuous practice and engagement with Python through real-life scenarios.
- Building a strong foundation in Python, while exploring advanced concepts like machine learning and data science.
- The value of connecting with other learners and fostering a community.
Recommended Resources for Continued Learning
To extend your learning beyond this article, consider the following resources:
- Python Official Documentation: A precise source for learning about Python syntax and functionalities. You can find it at python.org.
- Codecademy: An interactive platform to learn Python and other programming languages. Great for hands-on learners.
- Coursera: Offers courses on Python for beginners and advanced applications, developed by reputable institutions.
- edX: Another resource for a structured learning progression in Python.
- Reddit: Engaging with communities like r/learnpython can provide support and answers to specific questions you might have as you progress.
- Books: Consider titles like "Automate the Boring Stuff with Python" by Al Sweigart for practical applications and further projects.
Leveraging these resources will aid in maintaining momentum in your learning journey and building practical skills that are highly applicable in real-world scenarios.