Comprehensive Python List Interview Questions

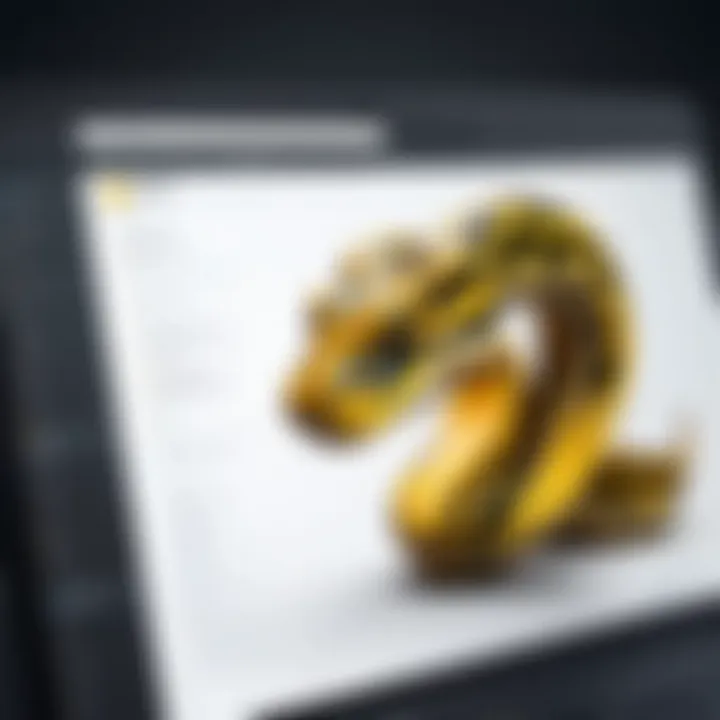
Overview of Topic
Python lists are fundamental to the language, enabling the storage and ordering of data in a flexible manner. They are versatile structures that can hold a mix of data types, making them ideal for a wide range of applications. Understanding how to utilize lists effectively can greatly enhance programming efficiency and capabilities.
The significance of lists in the tech industry cannot be overstated. Lists are prevalent in various applications, from data analysis to web development. As Python continues to grow in popularity, particularly in fields like data science and machine learning, having a strong grasp of how to leverage lists becomes crucial.
Python's history with lists dates back to its inception. Originally modeled after the core principles of the ABC language, Python lists have evolved into a rich, robust feature. Over the years, enhancements and refinements have made Python lists more user-friendly and powerful, accommodating more complex manipulations and data representations.
Fundamentals Explained
To understand Python lists, it is crucial to grasp a few core principles. Lists in Python are ordered collections that are changeable and allow duplicate entries. This means that the elements of a list have a specific order and can be accessed using index values.
Some key terminology includes:
- Element: Each individual item in a list.
- Index: The position of an element in a list, starting from zero.
- Slicing: A way to access parts of the list by defining a range of indices.
Basic concepts include how to create lists, access elements, and modify list contents. For example, a list can be defined as follows:
This line showcases the capability of lists to hold integers, strings, and booleans within a single structure.
Practical Applications and Examples
Python lists have many practical applications. They are commonly used in data storage, manipulation, and transfer. For instance, a list can conveniently store user inputs from web forms or provide dynamic data for algorithm processing.
A demonstration can be seen in this simple example:
This code snippet illustrates how to create a list, add elements, and print the updated list.
In addition, real-world applications include:
- Data Processing: Lists can handle large datasets efficiently.
- Game Development: Utilizing lists to manage inventories and scores.
Advanced Topics and Latest Trends
As Python evolves, so too do the techniques associated with lists. Advanced topics include list comprehensions, which provide a concise way to create lists. Instead of using traditional loops, one can create lists in a single line:
This code generates a list of squared numbers up to 9.
Emerging trends also focus on the integration of lists with libraries such as NumPy and pandas, which enhance the efficiency and capabilities of data manipulation.
Tips and Resources for Further Learning
To deepen your understanding of Python lists, consider the following resources:
- Books: "Automate the Boring Stuff with Python" by Al Sweigart.
- Online Courses: Coursera and Udacity offer various Python programming courses that cover lists comprehensively.
- Documentation: The official Python documentation provides in-depth information about list functionalities.
Tools like Jupyter Notebook or PyCharm can enhance practical usage, allowing for easy experimentation with lists.
By exploring these resources, students and IT professionals can better understand Python lists and implement them skillfully in their programming endeavors.
Understanding Python Lists
Understanding Python lists is crucial for anyone looking to deepen their knowledge of Python as a programming language. Lists are foundational structures used to hold an ordered collection of items. They enable developers to manage groups of related data effectively, making them indispensable in various programming applications.
Benefits of Python Lists:
Python lists offer dynamic sizing, easy manipulation with built-in methods, and versatility in holding different data types. They are used extensively in fields like data science, web development, and automation, where handling data efficiently is necessary.
There are some key considerations when using lists in Python. Understanding how to create, access, and modify lists can significantly enhance code performance and readability. A strong grasp of these operations helps debug potential issues far more effectively.
Definition and Characteristics
A list in Python is a flexible data container that can hold multiple items in a single variable. Unlike arrays in some other programming languages, Python lists can hold mixed data types, including integers, strings, and even other lists.
Characteristics of Python Lists:
- Ordered: Items in a list maintain the order of insertion.
- Mutable: Lists can be changed after they are created. You can add, remove, or modify items.
- Dynamic: Lists can grow and shrink in size as needed.
- Indexed: Each item in the list has an index, starting from 0, allowing precise access to any element.
Overall, these characteristics make lists a highly useful tool for developers.
List Data Types and Structures
Python lists are classified under the collection data types and can hold a variety of elements. Items can be of any type, and they can even be nested within each other, forming a multi-dimensional structure.
Types of Data Allowed in Lists:
- Homogeneous: All items are of the same type, like .
- Heterogeneous: Items are of different types, such as .
- Nested: Lists within lists, e.g., .
This flexibility makes Python lists suitable for various applications.
Comparison with Other Data Structures
When discussing Python lists, it is important to compare them with other data structures like tuples, sets, and dictionaries.
- Lists vs. Tuples:
- Lists vs. Sets:
- Lists vs. Dictionaries:
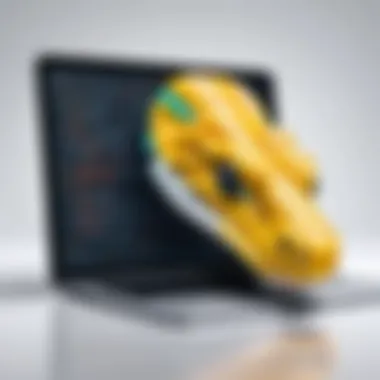
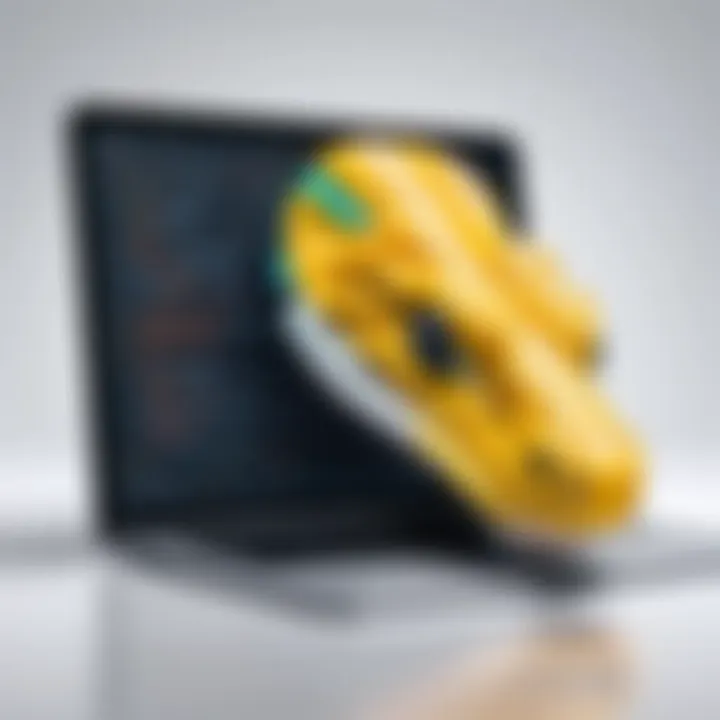
- Lists are mutable, whereas tuples are immutable. Once a tuple is created, it cannot be modified.
- Tuples can be used as keys in dictionaries due to their immutability, while lists cannot.
- Lists are ordered and allow duplicates, but sets are unordered and contain unique elements.
- Sets are used when the uniqueness of items is essential, whereas lists are ideal for ordered collections.
- Dictionaries store key-value pairs and are unordered; lists store indexed items and are ordered.
- Lists are best suited for sequences, while dictionaries excel in mapping relationships between items.
By understanding these differences, developers can choose the appropriate data structure that fits their needs, optimizing performance and clarity in code.
Common Python List Operations
Common Python list operations form the backbone of how developers interact with lists, one of the most versatile data structures in Python. Understanding these operations is crucial for anyone seeking to master Python programming. Lists can be used to store ordered collections of items, making them essential in many programming tasks. The efficiency of operations like creation, access, and modification directly impacts performance and user experience.
In this section, we will discuss key aspects of common Python list operations. We will explore how to create lists, access their elements, and modify existing lists. Knowing how to efficiently manipulate lists is not only beneficial for writing clean and maintainable code but also essential for optimizing performance in data-heavy applications.
Creating a List
Creating a list in Python is a straightforward process, allowing developers to instantiate an empty list or populate it with elements immediately. According to the Python documentation, you can create a list using square brackets, like so:
Alternatively, you can initialize it with elements:
Lists can contain various data types. Here’s an example:
This flexibility makes lists suitable for a wide range of applications, from simple collections to complex data structures.
Accessing List Elements
Accessing elements in a Python list is done through zero-based indexing. Understanding this concept is crucial for efficiently retrieving and manipulating list elements. For example, if you have a list:
You can access elements as follows:
- First element:
- Second element:
- Last element:
List slicing also allows you to access subsets of the list. For instance:
This gives you a new list containing all elements from the second to the last.
Modifying List Elements
Modifying lists in Python involves changing existing items or adding new ones. You can assign new values to existing indices:
Now, will appear as follows:
You can also append new items to the end of a list:
Now would be:
Additionally, you can remove elements using methods like or .
Important:
It's essential to choose modification methods wisely, as some methods alter the list in place, affecting the original data structure.
In summary, mastering common Python list operations enhances your programming skillset, allowing for more efficient data management and manipulation. These fundamental operations lay the groundwork for more complex data handling techniques used in advanced programming and data science.
Advanced List Techniques
In this section, we explore advanced techniques that enhance the utility and performance of Python lists. Mastering these skills is essential for anyone looking to elevate their proficiency with data structures. Advanced list techniques allow developers to write cleaner, more efficient code that handles complex data manipulation tasks more effectively. Embracing these techniques leads to better performance and clarity, making code easier to maintain and understand. Let’s delve into three core advanced techniques: list comprehensions, built-in functions, and nested lists.
List Comprehensions
List comprehensions provide a concise way to create lists. They are often more readable and faster than traditional loops for generating lists. A list comprehension consists of brackets containing an expression followed by a clause, and can also include optional conditions.
For example, the following code illustrates the creation of a list containing the squares of even numbers from 0 to 9:
This code is both compact and expressive. The comprehension iterates through all numbers up to 9, applying the square operation only to even numbers. The resulting list is simple to generate and maintain. This is particularly helpful in scenarios where performance optimization is critical, especially with larger datasets. The use of list comprehensions often results in cleaner and more Pythonic code, contributing to better code quality overall.
Using Built-in Functions
Python provides numerous built-in functions that can be applied to lists, enhancing their functionality. Functions like , , and are especially useful for transforming and filtering lists. These functions allow for functional programming paradigms, making tasks like data transformation more manageable.
For instance, the function can apply a specific operation to every item in a list. Consider the example below:
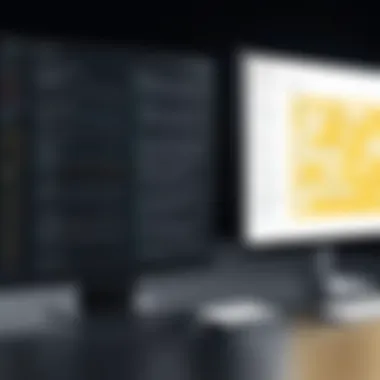
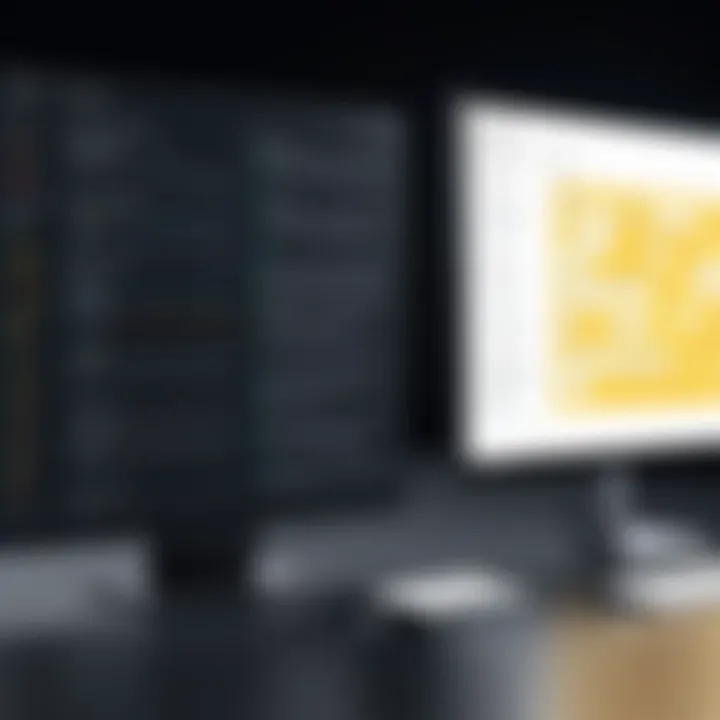
This code squares each element in the list . The use of is beneficial for performance optimizations, as it can handle larger datasets efficiently without the overhead of explicit loops. Understanding these built-in functions is crucial for effectively manipulating lists in Python, thus empowering developers to write efficient and concise code.
Nested Lists in Python
Nested lists, or lists within lists, are a fundamental aspect of Python’s list structure. They enable the storage of complex data types, such as matrices or multi-dimensional arrays, which is essential in various computational tasks.
To create a nested list, you simply define a list that contains other lists as its elements. An example of a 2D matrix representation is as follows:
Manipulating nested lists involves iterating through each element using nested loops. For example, to print each element in the matrix, one might use:
Nested lists offer flexibility but require careful indexing, which can make them harder to work with compared to flat lists. Understanding how to effectively navigate and manipulate nested lists is vital for tasks involving complex structures and can significantly enhance the functionality of applications dealing with multi-dimensional data.
Mastering advanced list techniques is a key step in optimizing your Python code and enhancing your software development skills.
Employing these techniques prepares students and professionals alike for the challenges faced in programming assignments, technical interviews, and real-world applications. Whether it’s simplifying code with comprehensions or harnessing the power of functions, understanding advanced list techniques can greatly accelerate your learning and skill set.
Error Handling and Debugging
Understanding error handling and debugging is critical when working with Python lists. Errors can arise from many sources such as improper data types, incorrect indexes, or unexpected input. Recognizing how to identify and address these issues efficiently can significantly enhance the coding experience and improve software quality. This section discusses common list errors and debugging techniques that can help streamline the development process.
Common List Errors
When working with Python lists, developers may encounter several common errors. Being aware of these issues and knowing how to resolve them can save considerable time and effort. Here are some frequent error types:
- IndexError: This error occurs when you try to access an index that is out of range for the list. For example, if a list has three elements, accessing the fourth element will produce an error.
- TypeError: This can happen when you attempt to perform an operation on incompatible data types. For instance, appending a non-list type to a list can lead to this error.
- ValueError: This error arises when the operation receives an argument of the right type but an inappropriate value. For example, using the method to remove a non-existing element from the list will trigger a ValueError.
- AttributeError: This occurs if you try to call a method on a list that does not exist or is misspelled.
Addressing these errors requires a good understanding of how lists operate in Python. Writing comprehensive tests and keeping an eye out for situations that lead to these common pitfalls can significantly reduce bugs in your code.
Debugging Techniques for Lists
Effective debugging techniques are essential when troubleshooting errors in lists. Employing systematic methods can assist developers in identifying and fixing issues more effectively. Below are some useful debugging strategies:
- Print Statements: Inserting statements throughout your code helps track the flow of execution and show the current state of lists at various points. This can be quite useful for understanding how lists change over time.
- Using the Module: The Python Debugger () allows you to set breakpoints, step through your code, and inspect list contents interactively. This method can reveal unexpected behavior in your list manipulations.
- List Comprehensions for Quick Checks: Using list comprehensions to filter or map lists can also help identify issues. If the result is not as expected, it prompts a reevaluation of the logic used in comprehension.
- Testing with Assertions: Writing assertions can catch errors early. Whenever a list should meet specific conditions, assertions can provide valuable feedback if your assumptions about the data are incorrect.
"A good debugging process not only identifies the error but also helps in understanding the underlying issues more deeply."
In summary, understanding common list errors and employing effective debugging techniques are crucial skills for anyone working with Python lists. Mastering these concepts not only helps avoid common pitfalls but also improves overall code reliability.
Performance Considerations
When working with Python lists, understanding performance considerations is crucial. Performance impacts not only the execution time of your code but also its resource usage. Lists are dynamic arrays in Python, which means they can grow and shrink in size based on your programming needs. However, their operational efficiency varies based on different factors. Hence, grasping the performance nuances can guide developers in writing more efficient and effective code.
Time Complexity of List Operations
To interpret how list operations influence performance, one must recognize the concept of time complexity. Time complexity provides a theoretical framework for analyzing how the time required for an operation grows as the size of the list increases. Python lists support various operations, each with its own time complexity.
- Accessing an element: This operation is done in constant time, O(1). You can directly access an element if you know its index, which is one of the strengths of using lists.
- Appending elements: Adding elements to the end typically operates in amortized constant time, O(1). As long as enough capacity is available, Python allocates space efficiently.
- Inserting elements: This can be time-consuming, as it requires shifting elements. The complexity here is O(n) because in the worst-case scenario, you might move all elements to accommodate the new entry.
- Removing elements: Like insertion, removal can also be O(n) as it may necessitate shifting elements to fill the gap created by the removed item.
Understanding these complexities aids in better decision-making. For instance, if many insertions need to occur, other data structures like might be more suitable due to their lower insertion complexities.
Memory Management in Python Lists
Memory management is another pivotal aspect of performance with Python lists. A list in Python is built over an array that can grow dynamically. When a list exceeds its allocated memory, Python creates a new, larger array and copies the contents of the old array to the new one. This is useful but has serious implications:
- Memory overhead: Lists in Python maintain some extra space for future growth, which can lead to memory wastage if lists do not utilize that space.
- Garbage collection: Python uses automatic memory management, primarily through garbage collection. When objects are no longer in use, memory is reclaimed. However, if lists contain large data or objects, the garbage collection process can introduce latency issues.
- Fragmentation: As lists grow or shrink, fragmentation can occur. Memory might become fragmented over time, which can incrementally slow down performance, especially in large applications.
By being aware of these memory management principles, programmers can optimize the use of lists, ensuring that their scripts run smoothly and effectively. Avoid initializing lists with excessive sizes when they are not required.
Tip: Regular profiling and monitoring of memory usage in applications can highlight potential performance issues related to lists.
In summary, understanding the performance considerations surrounding Python lists requires attention to time complexity and memory dynamics. By integrating this knowledge into programming practices, one can enhance overall application performance.
Practical Applications of Python Lists
Understanding the practical applications of Python lists is vital for programmers at all levels. Using this collection type effectively can lead to efficient and clean code in various projects. Python lists are versatile; they allow for the management of collections of data, facilitating complex data manipulation and analysis tasks. Thus, being proficient with Python lists enhances one’s ability to tackle a range of programming problems across sectors.
Using Lists in Data Analysis
In data analysis, Python lists are commonly used due to their simplicity and ease of use. They provide a straightforward way to store and manipulate data sets. When working with libraries such as pandas, NumPy, or Matplotlib, lists can serve as a foundational building block. For instance, lists can be converted into arrays or data frames, allowing for powerful data operations and visualizations. Many data scientists favor these lists for quick data entry, transformation, and extraction tasks.
Key benefits of using lists in data analysis include:
- Dynamic Sizing: Lists can easily grow or shrink, accommodating varying amounts of data without complicated memory management.
- Ease of Use: Syntax is straightforward, making it simple for beginners to get started without steep learning curves.
- Integration: Lists integrate seamlessly with other libraries, offering extensive functionality for data manipulation.
Moreover, lists allow for easy iteration using loops, enabling data scientists to analyze each entry effectively. Here is an example of how lists can be utilized for calculating averages in data analysis:
Lists in Web Development Projects
In web development, Python lists play a significant role in managing data dynamically. They are frequently used to handle user input, manage data fetched from APIs, or store configuration settings for applications. Lists provide a flexible way to encapsulate collections of items—like user profiles or product information—without the need for more in-depth structures, especially during rapid prototyping stages.
Some considerations for utilizing lists in web development include:
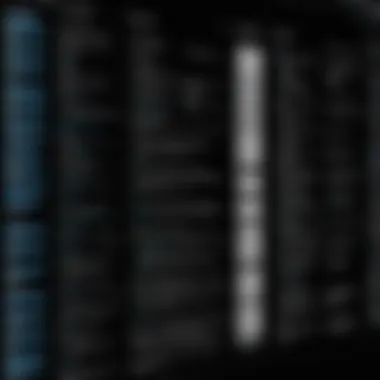
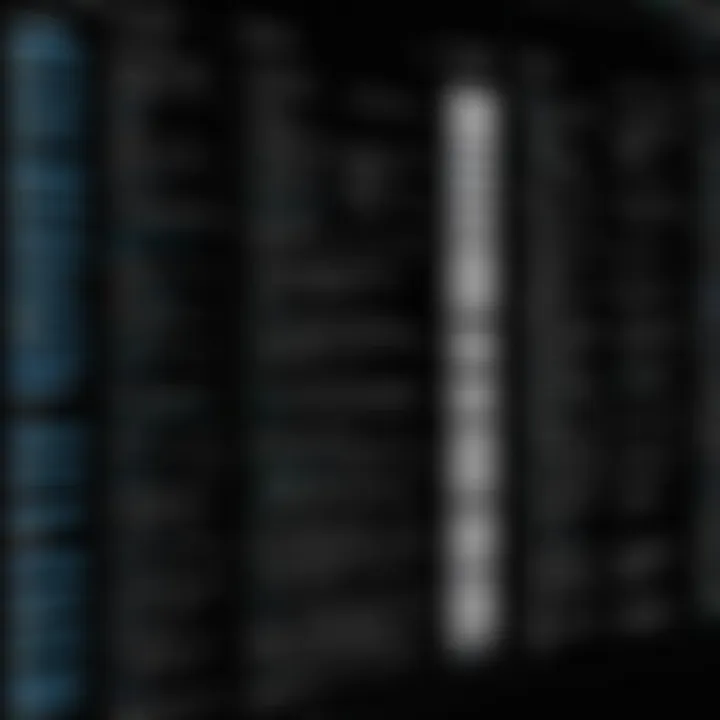
- Performance: For large datasets, lists may not provide optimal performance. For that reason, developers might consider alternative data structures such as dictionaries or sets when appropriate.
- Data Handling: Lists can simplify the handling of collections, such as lists of comments or transactions on a website. Their inherent ordering is beneficial when sequence matters.
- Framework Compatibility: Most Python web frameworks, like Django or Flask, support lists as a core element for organization and data retrieval.
By leveraging lists efficiently, developers can streamline workflows and enhance the interactivity of web applications. Overall, understanding how to implement and utilize Python lists in data analysis and web development significantly enhances a programmer's toolkit.
Interview Techniques and Best Practices
In the context of preparing for Python list interviews, understanding effective interview techniques is essential. The interview often determines whether a candidate possesses both the necessary technical skills and a suitable approach to problem-solving. Navigating the interview process successfully requires a combination of preparation, strategy, and communication.
Being well-versed in Python lists and their applications is crucial, but so is the ability to present that knowledge clearly and confidently. Effective interview techniques can significantly enhance a candidate's chance of success by allowing them to engage fluidly in discussions and demonstrate an in-depth understanding of concepts.
Here are some essential components of interview techniques that candidates should focus on:
- Research the Company and Role: Understanding the company’s culture and the specific requirements of the role can provide insights into what skills may be prioritized.
- Practice Common Questions: Familiarizing oneself with common Python list-related questions can ease the pressure during interviews.
- Mock Interviews: Conducting practice interviews with peers or mentors can build confidence and improve articulation of answers.
"Preparation is the key to success in interviews. A solid grasp of Python lists complements well-informed answers to behavioral questions."
Preparing for Python List Interviews
Preparation is the cornerstone of a successful interview. Candidates should first solidify their foundation in Python lists. Reviewing key concepts, such as list methods, comprehensions, and performance considerations, is vital. Here are actionable steps to prepare effectively:
- Study Basic Concepts: Ensure understanding of how to create lists, access elements, manipulate data, and use built-in functionalities.
- Solve Coding Challenges: Websites like LeetCode or HackerRank can provide practical problems to refine skills.
- Review Advanced Techniques: Look into topics such as nested lists and list comprehensions, as they often appear in more advanced questions.
Keeping a notebook of commonly asked questions and their solutions can serve as a quick reference during final preparations.
Demonstrating Problem-solving Skills
During an interview, it is not only important to provide the correct answer but also to exhibit the process taken to reach that answer. When discussing Python lists, candidates should aim to articulate their approach clearly. Here are some strategies to enhance problem-solving demonstration:
- Think Aloud: Narrate thought processes while tackling a problem. This provides insight into problem-solving skills.
- Break Down the Problem: Divide a complex problem into smaller, manageable parts. This approach not only simplifies the task but also showcases logical reasoning.
- Show Flexibility: Be ready to adapt your solution if given feedback. This displays openness to collaboration and critical thinking.
Being adept at problem-solving is often just as important as knowing the specifics of Python lists.
Communicating Effectively during Interviews
Effective communication plays a critical role in interviews. The ability to convey ideas clearly can significantly impact the interviewer’s perception of a candidate's capabilities. Here are ways to ensure effective communication:
- Use Clear Language: Avoid jargon unless it is necessary. Simple and clear language enhances understanding.
- Be Concise: Focus on delivering complete thoughts without digressing. This keeps the interview on track and allows for more questions.
- Ask Questions: Engage the interviewer by asking clarifying questions. This interaction can demonstrate eagerness to understand and clarify
Fostering strong communication skills can ease tension in interviews while creating a more interactive and engaging environment.
Sample Interview Questions
The section on Sample Interview Questions serves a crucial purpose in the context of preparing for Python programming interviews. Interview questions focused on Python lists provide insights into a candidate's understanding of data structures and their ability to leverage Python's capabilities. Candidates can expect questions that span from fundamental principles to complex problems.
Grasping the nature of these questions can yield substantial benefits. Job seekers gain the opportunity to showcase their knowledge and skills effectively. Additionally, understanding typical queries enables candidates to prepare targeted answers, honing their ability to solve problems efficiently during the interview.
In this section, we categorize the questions into basic, intermediate, and advanced levels. This layered approach allows individuals to assess their readiness and focus their study efforts where needed. Each category addresses different aspects of Python lists, ensuring comprehensive coverage of the subject.
"Preparation is a key factor in achieving success during interviews, especially when it comes to demonstrating your knowledge of Python lists."
Basic Level Questions
Basic level questions focus on foundational understanding of Python lists. Examples include:
- What is a list in Python? This question evaluates the candidate's grasp of the fundamental definition and Nature of lists in Python.
- How do you create a list? This query checks whether the candidate knows the syntax and techniques used to instantiate a list in Python.
- Can you explain indexing in lists? Candidates should understand how lists are indexed, what zero-based indexing is, and how to access elements.
These basic questions lay the groundwork for comprehending more intricate topics later.
Intermediate Level Questions
Intermediate questions delve deeper into Python lists, requiring more than just surface knowledge. For instance:
- How do you add elements to a list? Here, candidates must demonstrate knowledge of methods such as , , and .
- Discuss list slicing and its applications. Understanding list slicing is essential; candidates should know the syntax and use cases where slicing is advantageous.
- What are list comprehensions, and how can they be used? This assesses the candidate's ability to write concise and efficient code using list comprehensions.
Intermediate questions test how well candidates can apply their knowledge to real-world scenarios.
Advanced Level Questions
Advanced questions challenge candidates to think critically and apply their knowledge in complex situations. They might include:
- What are the performance implications of using lists vs. other data structures? This question explores the candidate's grasp of efficiency, including time and space complexity.
- Can you demonstrate how to merge two lists without using built-in functions? Candidates should be prepared to discuss algorithmic approaches and efficiency.
- How do nested lists function in Python, and what are their uses? This explores the understanding of lists within lists and their applications.
Advanced questions are pivotal for evaluating a candidate's depth of knowledge and problem-solving abilities.
Post-Interview Reflection
Reflecting on an interview is a crucial step that can significantly impact one's professional development. This process not only helps candidates assess their performance but also provides insight into their strengths and weaknesses regarding Python list concepts. Engaging in post-interview reflection is a step toward mastery, benefiting both the immediate and the long-term aspects of personal growth.
An effective post-interview reflection involves multiple elements. First, candidates should consider their technical responses. Did they correctly answer questions about list operations or advanced techniques like list comprehensions? This evaluation helps identify gaps in knowledge that may require further study. Additionally, reflection on communication skills is paramount. How well did they articulate their thought process? A clear expressiveness in explaining Python concepts can set one apart from others.
Taking time to contemplate these factors can lead to improved performance in future interviews. Candidates should also assess their comfort with practical applications of lists, which are often integral during technical interviews. As a result, one may find themselves gathering new resources and insights to enhance their understanding of Python lists further.
"The post-interview reflection takes the learning experience to new heights, shaping candidates into better professionals."
Analyzing Interview Performance
Analyzing performance after an interview is fundamental in fostering a growth mindset. Candidates should begin by recalling the questions asked, particularly those that caused hesitation. Were there specific list methods they struggled to explain? By documenting these instances, candidates can prioritize areas for improvement.
Another effective strategy is to seek feedback from peers or mentors familiar with Python. Discussions about what went well, what did not, and possible solutions can provide clarity and direction. It might also help to practice mock interviews centered around Python lists to enhance confidence and ability in future engagements. Using self-assessment tools, such as marking down the accuracy of answers and evaluating clarity of concept explanations, adds another layer to this analysis.
Continuous Learning from Feedback
The journey of learning never truly ends, especially in the fast-evolving world of programming. Continuous learning is vital for those aiming to refine their understanding of Python lists. Feedback from interviews should serve as a beacon guiding further education. Candidates should identify patterns in the feedback received. Is there a recurring theme, such as difficulties with nested lists? This insight drives targeted learning.
Cultivating a routine of reviewing Python documentation, participating in online coding challenges, or engaging with community forums like Reddit can bolster knowledge. Furthermore, embracing mistakes made during interviews can transform into valuable learning experiences. By acknowledging errors, whether in syntax or logic, candidates become adept at handling similar situations in the future.