Unveiling the Intricacies of Python String Class Operations
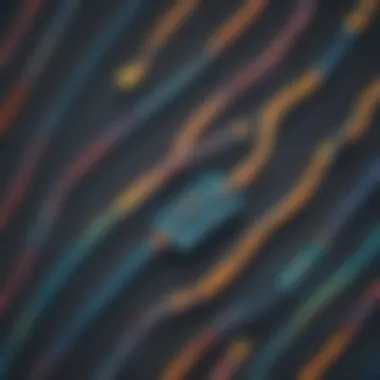
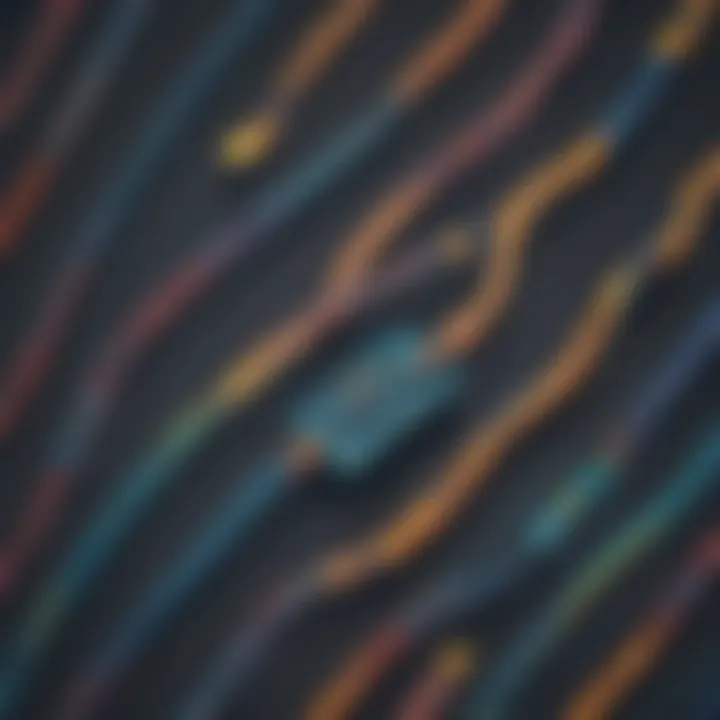
Overview of Topic
- Introduction to Python String Class: The Python String Class is a fundamental component in Python programming, essential for storing and manipulating text data efficiently. Understanding its intricacies is paramount for any programmer aiming for proficiency in Python.
- Significance in Tech Industry: Given that Python is one of the most popular programming languages in the tech industry, mastery of the Python String Class opens up numerous opportunities for developers, from web development to data science.
- Brief History and Evolution: The Python String Class has evolved over the years to become a versatile tool, with constant improvements and updates ensuring its relevance in modern programming practices.
Fundamentals Explained
- Core Principles and Theories: The Python String Class operates on the core principle of treating text data as a sequence of characters, with each character having a specific index within the string. Understanding this hierarchy is crucial for efficient string manipulation.
- Key Terminology and Definitions: Concepts such as immutability (strings cannot be changed once created) and concatenation (joining strings together) form the bedrock of string operations in Python.
- Basic Concepts and Foundational Knowledge: Learning how to declare, access, and modify strings lays the foundation for mastering more advanced string handling techniques and functions.
Practical Applications and Examples
- Real-World Case Studies: From text processing in natural language processing algorithms to data cleaning in machine learning projects, the Python String Class finds extensive application in various real-world scenarios.
- Demonstrations and Hands-On Projects: In this section, we will explore hands-on examples that showcase how to use string methods effectively, reinforcing the practical usability of Python's string manipulation capabilities.
- Code Snippets and Implementation Guidelines: By providing code snippets along with detailed explanations, readers will gain a clear understanding of how to implement different string operations in their own programming projects.
Advanced Topics and Latest Trends
- Cutting-Edge Developments: The latest trends in Python string manipulation revolve around optimizing performance through efficient algorithms and exploring new ways to handle large amounts of text data effectively.
- Advanced Techniques and Methodologies: Dive deep into advanced topics such as regular expressions, formatting, and encoding methods to enhance your string manipulation skills and solve complex problems more elegantly.
- Future Prospects and Upcoming Trends: As technology continues to evolve, staying updated on the latest advancements in string processing will be crucial for developers looking to streamline their code and stay ahead of the curve.
Tips and Resources for Further Learning
- Recommended Books, Courses, and Online Resources: Explore a curated list of resources that offer in-depth coverage of string manipulation in Python, ranging from beginner-friendly tutorials to advanced courses for seasoned professionals.
- Tools and Software for Practical Usage: Discover productivity tools and software applications that can streamline your string processing workflow, making your programming tasks more efficient and error-free.
Introducing the Python String Class
The Introduction section sets the stage for our exploration of the Python String Class, a fundamental component in programming languages. Understanding the nuances of this class is crucial for anyone delving into Python programming. By comprehensively examining the various features and functionalities of the String Class, readers will gain invaluable insights into how it can be effectively utilized in their coding endeavors. This section serves as a gateway to unraveling the intricacies and potential of working with strings in Python.
Overview of Python String Class
Understanding the Fundamental Concepts
The crux of Understanding the fundamental concepts lies in grasping the essential building blocks of strings within Python. By delving into this aspect, individuals can enhance their understanding of how strings operate and interact within the Python environment. This foundational knowledge is paramount for mastering string manipulation and manipulation operations within Python programming. The unique characteristic of Understanding the fundamental concepts is its ability to provide a solid groundwork for more advanced string manipulations, making it a staple choice for those looking to fortify their Python skills.
Importance of Strings in Programming
The significance of strings in programming cannot be understated. They serve as a vital component in handling textual data and performing various operations on it. Understanding the various operations that can be carried out on strings is crucial for effective programming. The importance of strings in programming lies in their versatility and ubiquity across different programming languages. By harnessing the power of strings, programmers can streamline their coding processes and optimize the efficiency of their programs. This section delves into the pivotal role that strings play in programming and the myriad benefits they offer to programmers.
History and Evolution
Origins of Python String Class
Exploring the Origins of Python String Class sheds light on the inception and development of string handling within Python. Tracing back to its roots provides valuable insights into the design choices and considerations that have shaped the String Class over time. The key characteristic of Origins of Python String Class is its ability to showcase the evolution of string manipulation techniques and practices within Python. By understanding the origins of the String Class, programmers can appreciate the thought processes that have influenced its current state and functionality.
Notable Developments Over Different Versions
Notable developments over different versions highlight the evolution and enhancements that the String Class has undergone throughout various iterations of Python. By chronicling these advancements, programmers can track the progression of string handling capabilities within the language. The key characteristic of Notable developments over different versions is its reflection of Python's commitment to enhancing string manipulation functionality and efficiency. This section explores how Python has continually refined its string handling mechanisms to cater to the evolving needs of programmers, thereby solidifying its position as a robust programming language for string operations.
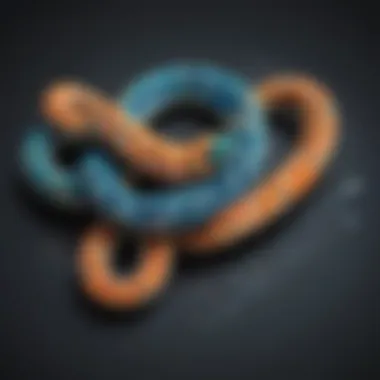
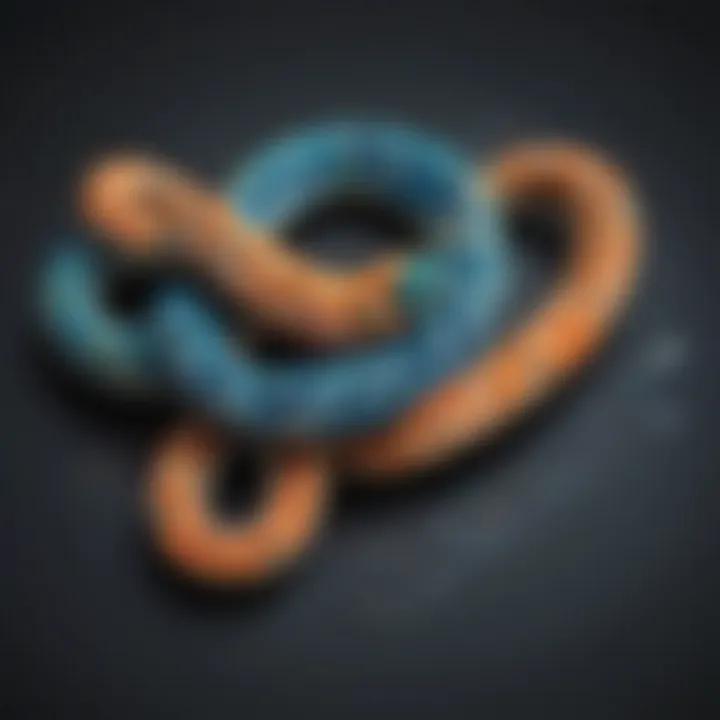
Basic Operations
In the realm of programming with Python, mastering Basic Operations within the Python String Class is paramount. These operations encompass fundamental processes like string creation, access, concatenation, repetition, length determination, and slicing. Although seemingly rudimentary, these operations serve as the building blocks for more complex manipulations. Understanding each aspect of Basic Operations is crucial for efficient programming and data manipulation, making it a cornerstone in Python string manipulation.
String Creation and Access
Creating strings
Creating strings in Python involves the initialization of sequences of characters enclosed within single (' ') or double (" ") quotes. This action is pivotal as strings form the basis of textual data processing in Python, allowing programmers to store and manipulate textual information effectively. The unique feature of creating strings lies in its flexibility, enabling the incorporation of alphabets, numbers, symbols, and special characters. While creating strings is a preferred choice for data representation, it is essential to consider memory allocation and encoding to optimize string handling.
Accessing characters in strings
Accessing characters in strings enables programmers to retrieve individual elements within a string based on their position or index. This operation is significant for tasks requiring character-level manipulation or extraction of specific information from a string. By accessing characters in strings, programmers can perform tasks like screening for particular characters, modifying specific elements, or analyzing textual data in-depth. However, it is crucial to be cautious with out-of-bound indexing to prevent errors and ensure the integrity of the string data.
String Concatenation and Repetition
Combining strings
String concatenation involves merging two or more strings to form a single, unified string. This operation is valuable for tasks that involve combining textual data or creating output messages by joining multiple strings. The primary advantage of string concatenation is its simplicity and versatility, allowing programmers to construct dynamic strings by merging various text entities. While efficient for string manipulation, excessive concatenation can impact program performance, necessitating careful consideration of concatenation frequency and string length.
Repeating strings
String repetition entails duplicating a particular string multiple times to generate a repeated sequence. This operation finds utility in scenarios where duplicating text or generating patterns is required. The key benefit of string repetition is its ability to streamline code by eliminating redundant statements and enhancing code readability. However, excessive string repetition can lead to code verbosity and inefficiency, warranting judicious use of this operation in programming contexts.
String Length and Slicing
Finding string length
Determining the length of a string involves calculating the number of characters present in the given string. This operation is crucial for tasks necessitating an understanding of the string size or validating input data constraints. The primary advantageous characteristic of string length determination is its simplicity and speed, allowing for quick assessments of string sizes. However, incorrect length calculations can lead to errors in data processing, emphasizing the importance of accurate length evaluations.
Slicing strings
Slicing strings enables programmers to extract specific segments or substrings from a larger string based on defined indices or ranges. This operation is beneficial for isolating portions of text, manipulating substrings, or partitioning textual data into manageable segments. The main advantage of slicing strings lies in its precision and efficiency, facilitating targeted data extraction without the need for complex algorithms. Nonetheless, meticulous attention to slicing boundaries is necessary to avoid extracting incorrect substrings and ensure accurate data manipulation.
Advanced Techniques
In this section, we will delve deeper into the advanced techniques associated with the Python String Class. Understanding these advanced techniques is crucial for enhancing the efficiency and effectiveness of string manipulations in programming. By mastering these techniques, programmers can elevate their skills and optimize their code for better performance. We will explore key elements such as upper and lower case conversion, substring extraction, and replacing characters in detail, shedding light on their significance and practical applications.
String Manipulation Methods
Upper and Lower Case Conversion
When it comes to case conversion in strings, the Python String Class offers a powerful feature that allows users to easily switch between uppercase and lowercase representations of text. This functionality plays a vital role in text processing tasks, ensuring consistency and uniformity in the output. The ability to convert cases efficiently is particularly advantageous, especially when dealing with user inputs or formatting requirements. While this feature simplifies case manipulation, it's essential for programmers to consider potential limitations, such as language-specific behaviors or encoding complexities, when implementing case conversion.
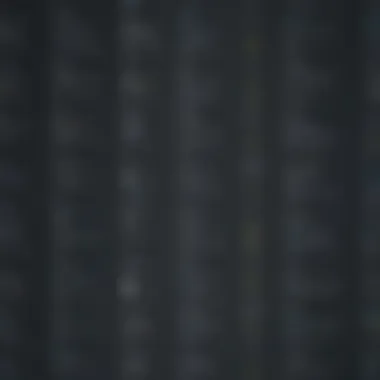
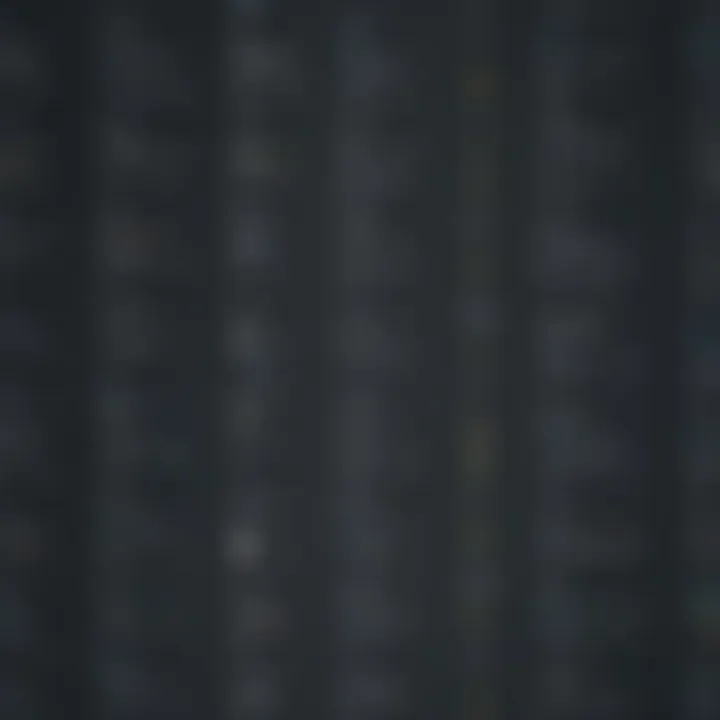
Substring Extraction
The process of extracting substrings from a larger string is a fundamental operation that can be performed using the Python String Class. Substring extraction enables programmers to isolate specific portions of text based on defined criteria, offering flexibility and precision in data manipulation. This functionality is pivotal in tasks where selective information extraction is needed, such as parsing structured data or filtering content. However, it's important to note that substring extraction may pose challenges related to indexing, boundary conditions, or placeholder dependencies, necessitating careful attention during implementation.
Replacing Characters
Replacing characters within a string is a common task that programmers often encounter when working with text data. The Python String Class provides robust capabilities for character replacement, allowing users to substitute one or more characters with different values efficiently. This feature is valuable in scenarios where text corrections, transformations, or pattern-based substitutions are required. Despite its utility, character replacement should be approached cautiously to avoid unintended modifications or disruptions to the string structure. It's essential for programmers to validate the replacement logic thoroughly to maintain data integrity and alignment with the intended outcomes.
Formatting and Interpolation
String Formatting Using Placeholders
The utilization of placeholders in string formatting is a versatile technique supported by the Python String Class, enabling dynamic value insertion and template-based content generation. This approach streamlines the creation of structured text output by replacing placeholders with corresponding variables or values at runtime. The efficiency of this method lies in its adaptability to diverse data types and formatting styles, enhancing the readability and conciseness of generated strings. While string formatting with placeholders offers significant advantages in code manageability and presentation, careful consideration should be given to formatting conventions, argument sequencing, and compatibility with target output formats to ensure seamless integration and coherence.
String Interpolation with Variables
String interpolation with variables enhances the flexibility and expressiveness of string manipulation in Python, facilitating direct embedding of variables and expressions within text literals. This dynamic approach to string construction enables dynamic context inclusion and value rendering, fostering code clarity and simplifying complex string concatenation tasks. The use of string interpolation with variables is particularly beneficial in scenarios requiring real-time data integration or contextual information embedding. However, programmers must exercise caution in managing variable scopes, data types, and interpolation patterns to prevent ambiguity or runtime errors in string composition.
Searching and Modifying
Finding Substrings
The Python String Class offers robust functionality for searching and extracting substrings within textual data. Finding substrings involves pattern matching and location identification within the parent string, allowing users to pinpoint specific content segments efficiently. This capability is pivotal in text parsing, keyword identification, or content analysis applications, offering precise search results and seamless integration with other data processing tasks. While substring search enhances data retrieval and analysis, programmers must account for case sensitivity, substring variations, and search algorithms' efficiency to optimize the search process and ensure accurate results.
Modifying String Contents
Modifying string contents involves altering the text values or structure within a string object using the Python String Class methods. This process enables users to manipulate text data, perform textual transformations, or update specific elements within the string seamlessly. By understanding how to modify string contents effectively, programmers can implement data sanitization, formatting adjustments, or content updates with precision and consistency. However, it's imperative to validate the modification logic, handling of edge cases, and impact on surrounding text to maintain data coherence and semantic integrity throughout the content modification process.
Additional Features
When delving into the exploration of the Python String Class, one cannot overlook the importance of the Additional Features section. This segment serves as a crucial component in understanding the intricacies that govern strings within the Python programming realm. Additional Features encompass a range of advanced functionalities and elements that enhance the versatility and utility of strings in Python. From handling escape sequences to character encoding, the Additional Features section provides programmers with essential tools to manipulate and optimize string operations efficiently.
Escape Sequences
Utilizing escape characters:
A fundamental aspect of the Escape Sequences in Python's String Class is the utilization of escape characters to represent special characters within strings. These escape sequences, such as '\n' for newline or '\t' for tab, play a pivotal role in ensuring precise and controlled string output. They enable programmers to include non-printable characters or characters with special meanings without disrupting the string structure. Despite the added complexity of incorporating escape characters, their presence significantly enhances the readability and functionality of strings within the Python String Class.
Special characters in strings:
The discussion on Special characters in strings sheds light on the unique characters that hold particular significance within the realm of string manipulation in Python. These special characters, ranging from escape sequences to non-ASCII characters, offer programmers a broad spectrum of possibilities in formatting and representing textual data. By understanding the nuances of special characters, developers can navigate encoding challenges and ensure consistent handling of diverse character sets. Although special characters introduce nuanced complexities, their strategic use can greatly enrich the expressive power of Python strings.
Raw Strings and Encoding
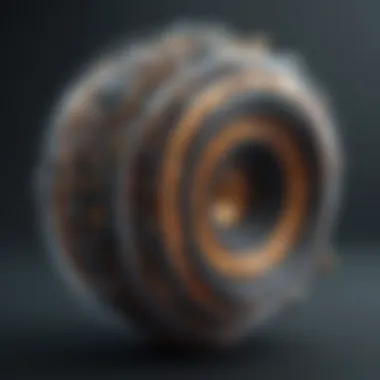
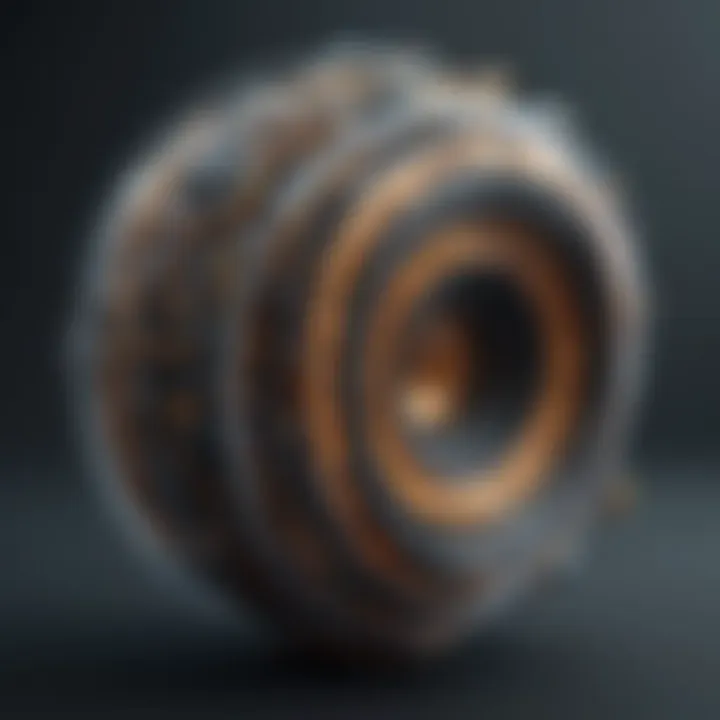
Handling raw strings:
Raw strings play a vital role in preserving the literal values of characters within Python strings without interpreting escape sequences. This aspect proves indispensable when working with pathnames, regular expressions, or any situation where backslashes must retain their literal meaning. By utilizing raw strings, programmers can prevent unintended character conversions and maintain the original representation of textual data, thereby enhancing code reliability and clarity.
Character encoding in strings:
Character encoding in strings explores the critical domain of representing characters from various languages and symbol sets within Python strings. Python supports a myriad of encoding schemes such as UTF-8, ASCII, and Latin-1, enabling seamless integration of multilingual content. Understanding character encoding is essential for handling internationalization and ensuring compatibility across different platforms. While character encoding enriches the versatility of strings, mismanagement or mismatch of encoding schemes can lead to data corruption and processing errors.
Immutable Nature of Strings
Understanding immutability:
The Immutable Nature of Strings in Python underscores the principle that once a string is created, its contents cannot be altered or modified. This inherent immutability confers stability and predictability to string objects, eliminating the risk of unintended changes during program execution. While this immutability may seem restrictive, it simplifies memory management and enhances thread safety in concurrent programming environments.
Implications in string operations:
The Immutability of Strings in Python inherently influences the approach to string operations, emphasizing the creation of new string objects rather than modifying existing ones. By recognizing the implications of immutability, programmers can adopt efficient coding practices and optimize memory usage. While immutability ensures data integrity and consistency, it necessitates a paradigm shift towards functional programming methodologies for string manipulation tasks.
Common Challenges and Solutions
In the realm of programming, particularly in the context of Python String Class exploration, understanding common challenges and their corresponding solutions is paramount. Delving into the nuances of encoding, decoding, performance considerations, and handling special characters can significantly impact the functionality and efficiency of code implementation. By addressing these challenges proactively, programmers can streamline operations, enhance output quality, and minimize potential errors, optimizing the overall programming experience.
Encoding and Decoding
Dealing with different encodings
When it comes to dealing with different encodings, it is crucial to grasp the diverse character encoding schemes that exist, such as UTF-8, UTF-16, ASCII, and more. Understanding how various encodings interpret, store, and communicate characters is essential for seamless data processing and communication within Python String Class operations. By selecting the appropriate encoding method based on project requirements and compatibility standards, programmers can ensure data integrity, cross-platform support, and multilingual content representation, thereby enhancing the robustness and versatility of their applications.
Unicode handling in strings
Within the domain of Python String Class exploration, proficient handling of Unicode characters is indispensable for catering to global audiences and encoding a broad spectrum of symbols, languages, and emojis. Embracing Unicode compatibility empowers programmers to transcend language barriers, support internationalization efforts, and ensure consistent character representation across different platforms and mediums. Implementing Unicode handling practices fortifies the reliability and inclusivity of string operations, promoting effective communication and user engagement in diverse linguistic contexts.
Performance Considerations
Optimizing string operations
Efficiently optimizing string operations within the Python String Class environment involves employing algorithmic strategies, data structures, and built-in functions to expedite text processing, concatenation, and manipulation tasks. By fine-tuning code logic, minimizing redundant computations, and leveraging optimized libraries, developers can boost runtime performance, memory efficiency, and overall computational speed, delivering responsive and resource-efficient software solutions. Prioritizing performance considerations fosters code scalability, responsiveness, and user satisfaction, elevating the programming experience to new levels of efficiency and effectiveness.
Memory management strategies
In the quest for optimal memory utilization and resource allocation, adopting effective memory management strategies is pivotal for mitigating memory leaks, optimizing storage usage, and enhancing code stability within the Python String Class ecosystem. Implementing techniques like garbage collection, memory pooling, and object recycling helps control memory allocation, prevent fragmentation, and facilitate efficient memory deallocation. By implementing sound memory management practices, developers can ensure sustainable performance, robust system stability, and seamless string operation execution, laying the foundation for scalable and resilient software solutions.
Handling Special Characters
Escaping special characters
The meticulous handling of special characters, such as newline characters, tabs, backslashes, and quotes, is essential for preserving data integrity, avoiding parsing errors, and safeguarding code clarity within string representations. Implementing escape sequences or raw string literals enables developers to denote special characters explicitly, resolve ambiguity, and prevent unintended character interpretations during program execution. By employing robust escaping mechanisms, programmers can fortify data validity, support complex string formatting, and enhance code readability, optimizing the interpretability and reliability of string-based operations.
Character encoding issues
Navigating character encoding issues within the Python String Class landscape entails addressing encoding discrepancies, charset inconsistencies, and inputoutput stream mismatches that may arise during data exchange or manipulation. Resolving encoding challenges requires adopting standardized encoding practices, handling encoding errors gracefully, and validating input data to ensure seamless text processing and communication. By rectifying character encoding ailments promptly, developers can avert data corruption, promote interoperability, and safeguard data fidelity, bolstering the resilience and robustness of string-handling mechanisms.
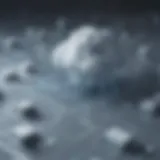
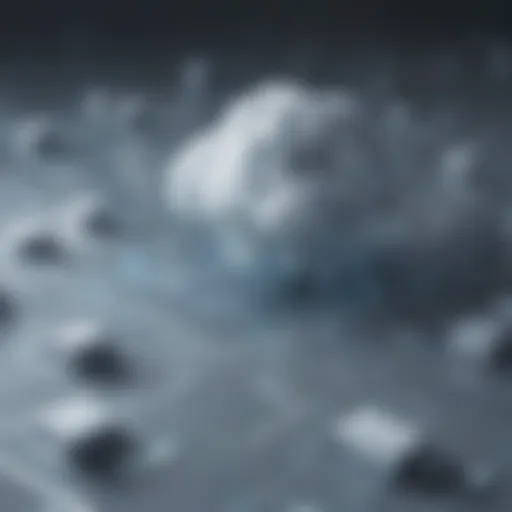
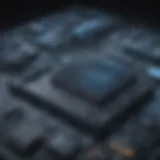
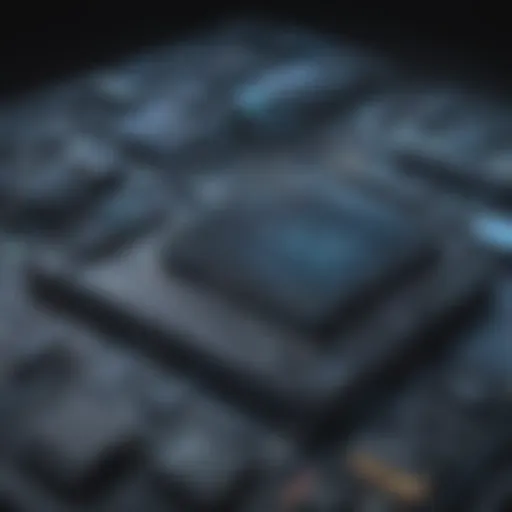