Sending Email via JavaScript: A Comprehensive Guide
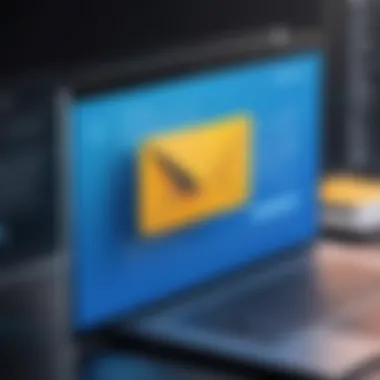
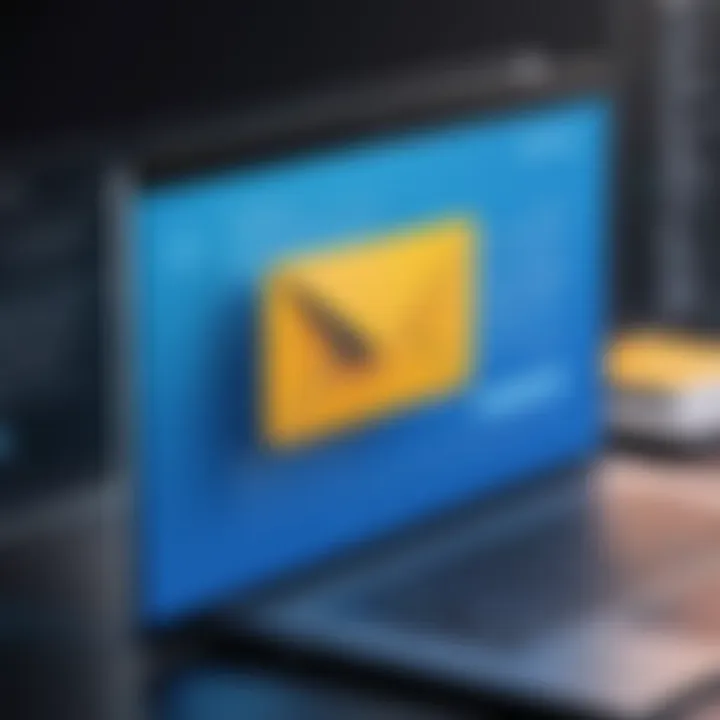
Overview of Topic
Prolusion to the main concept covered
In modern web development, JavaScript holds a crucial position. One of its practical applications is sending emails directly from applications. While JavaScript primarily operates on the client side, integrating email features can enhance user engagement and facilitate seamless communication. This guide delves into the various methods used to implement email functionalities with JavaScript, aiming to provide in-depth knowledge.
Scope and significance in the tech industry
The ability to send communications through emails embedded in web applications has transformed how businesses and developers engage users. By understanding how to effectively harness JavaScript for this purpose, developers create experiences that not only aid in communication but also foster user connectivity. This helps to personalize user experiences rapidly and effectively, resulting in improved interactions.
Brief history and evolution
Initially, sending emails required extensive knowledge of back-end technologies. The evolution of JavaScript and various libraries has transformed this process, offering simplified client-side operations. Over the past decade, features and frameworks like Node.js and various APIs have made it more manageable to integrate direct email sending functionalities in web applications. Understanding this evolution is significant to grasp current and future potentials of JavaScript in this area.
Fundamentals Explained
Core principles and theories related to the topic
Traffic to applications often requires utilizing communication channels. Email stands as a reliable method. JavaScript employs various libraries and protocols to enable this communication. Understanding how JavaScript interacts with back-end services, like SMTP servers, is fundamental. To achieve this, certain operations are essential: user authentication, email composition, and sending protocols.
Key terminology and definitions
- JavaScript: A scripting language primarily used for creating interactive features on websites.
- SMTP: Simple Mail Transfer Protocol, a standard protocol for sending emails.
- Node.js: An open-source softwrare platform for executing JavaScript code outside of a browser.
Basic concepts and foundational knowledge
Understanding Promises in JavaScript is paramount, especially when working with asynchronous commands related to sending emails. Familiarity with APIs and how they interact with JavaScript is also significant. This foundation aids developers in navigating complexities and prevents common pitfalls while executing email functionalities.
Practical Applications and Examples
Real-world case studies and applications
Companies often implement email via JavaScript in various forms. A common instance is user registration, where confirmation emails are automatically sent to newly registered users. For instance, platforms like GitHub use these techniques within their workflow.
Demonstrations and hands-on projects
To practice sending emails with JavaScript, consider a simple project: a contact form that sends the userβs input to an email address. This enhances skills in JavaScript while offering functional output in everyday applications.
Code snippets and implementation guidelines
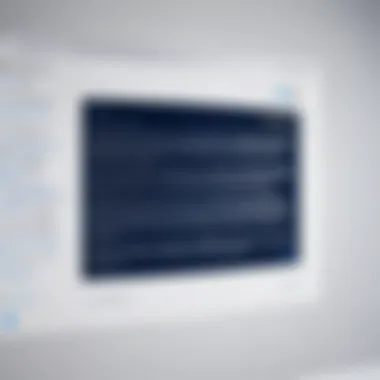
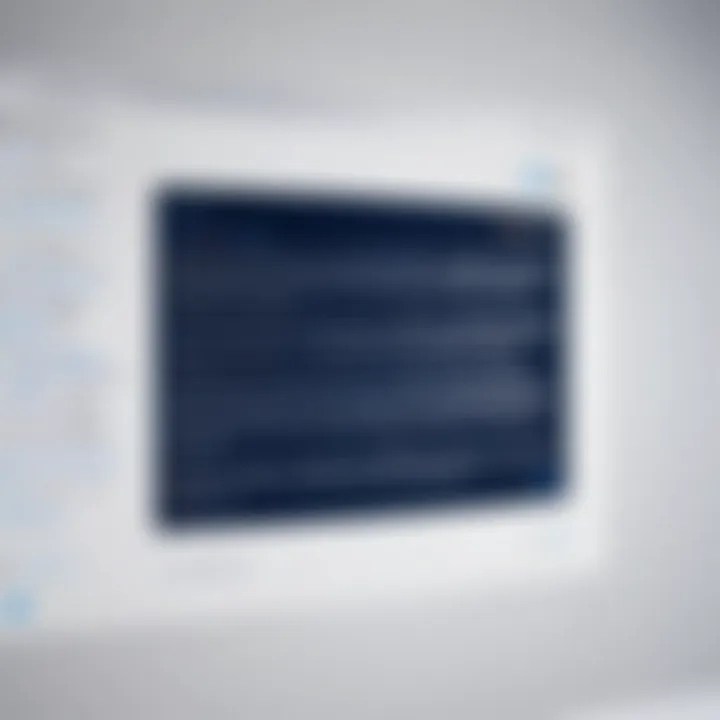
Here's a basic example using Node.js:
This snippet can be modified as needed, showcasing how JavaScript can effectively facilitate email sending.
Advanced Topics and Latest Trends
Cutting-edge developments in the field
With advances in technology, newer libraries and tools ensure streamlined functions. Using APIs allows easy send processes without delving into server configurations directly. This is reshaping how businesses think about engagement through email.
Advanced techniques and methodologies
As you advance in email sending via JavaScript, explore techniques like integrating templates for personalized emails. APT technologies also support Enhanced email activities enabling rich user interaction.
Future prospects and upcoming trends
The growth of serverless architecture allows JavaScript to minimize back-end complexity, promising an increase in email features and integrations in future web applications. Doing so could prompt enhanced user experiences and efficiencies.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
- Eloquent JavaScript by Marijn Haverbeke β provides insights into JavaScript.
- Node.js Web Development by David Herron β covers server-side of JavaScript operations deeply.
Tools and software for practical usage
Leveraging libraries like Nodemailer and APIs such as SendGrid make sending emails efficient while enabling developers to focus on enhancing applications instead of getting bogged down by underlying complexities:
- SendGrid: Provides API services to send emails easily.
- Mailgun: An SMTP service with advanced sending options.
Understanding these resources aids in grasping email integration in the world of JavaScript. For ongoing discussions, you may explore platforms such as Reddit where many programmers share their stories and ask questions. Further knowledge can be found by diving into Wikipedia and fellow tech sites like Britannica.
By exploring the intricacies of sending emails via JavaScript, developers gain not only functionality but a reliable and engaging means of communication for their applications.
Intro to Sending Emails from JavaScript
Sending emails is an essential functionality in modern web applications, and including it within JavaScript provides an added layer of interactivity for users. Whether for notifications, user registrations, or confirmations, integrating email capabilities enables developers to communicate directly with users. Yet, achieving this can pose several challenges. By exploring how to send emails via JavaScript, this guide aims to clarify the methods employed in such implementations.
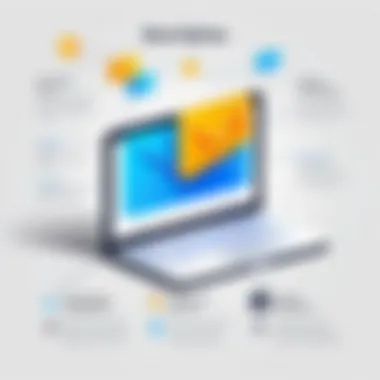
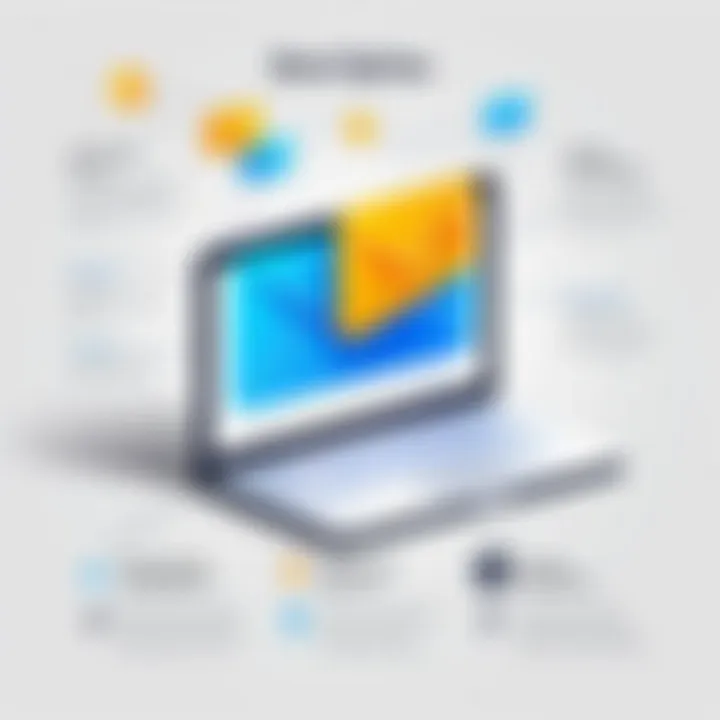
Overview of Email Functionality
Email functionality in web applications provides a direct line between the application and its users. It streamlines communication processes, allowing applications to send alerts, updates, or important information with just a few lines of code. Sending emails goes beyond basic functionality; it involves understanding the nuances of both client-side and server-side implementations. Each approach presents different considerations, such as security, user authentication, and data handling. Leveraging frameworks and libraries like Nodemailer or EmailJS helps encapsulate complex operations into manageable functions. This provides developers with powerful tools to address email services efficiently.
Common Use Cases for Sending Emails
There exist various scenarios where sending emails can enhance user experience and application utility. Here are a few key use cases:
- User Registration: Informing users of successful sign-ups or providing activation links.
- Password Reset: Assisting users by sending password reset links directly to their registered emails.
- Order Confirmations: Notifying users about successfully placed orders in e-commerce platforms.
- Alerts and Notifications: Sharing important updates, reminders, or marketing promotions with users.
- Feedback Requests: Engaging users post-interaction to gather feedback and improve services.
Effectively integrating email functionalities into web applications not only enriches user interaction but also builds trust and transparency. Each use case emphasizes the need for reliable and secure methods for sending emails, ensuring that communications are personalized and timely.
Effective integration of emails fosters interaction and builds trust with end users.
JavaScript Environment: Client-Side vs Server-Side
JavaScript plays a crucial role in web development today. Understanding the differences between client-side and server-side environments is essential for tasks like sending emails through JavaScript. Each environment has its strengths and limitations, which heavily influence method and functionality.
Understanding Client-Side Limitations
Client-side JavaScript runs in the user's browser. This means that the code is executed on the user's machine, not on a web server. While this type of operation provides reactivity and direct user interaction, it introduces several limitations, especially when sending emails:
- Security Issues: Sending emails directly from client-side code exposes sensitive credentials, such as SMTP usernames and passwords, to the public. This raises clear security risks.
- Browser Compatibility: Each browser may interpret JavaScript in slightly different ways. Thus, some functionality may not work across all browsers, leading to inconsistencies in user experience.
- Limited Protocol Access: Client-side scripts cannot directly connect to an SMTP server. Email sending functionality typically requires server access, which client-side scripts cannot achieve on their own.
- User Dependency: Actions like sending emails require user interactivity, such as forms completion, affecting reliability.
Because of these reasons, developers often avoid integrating email functionalities purely on the client side without having server support.
The Role of Server-Side Technologies
Server-side JavaScript, particularly using environments like Node.js, handles email sending processes more effectively. Here are the advantages of utilizing server-side technologies:
- Security: Sensitive information and logic remain on the server, inaccessible to users. Only necessary data is sent to client systems, minimizing exposure to attacks.
- Higher Control and Customization: Developing with a server-side framework allows for finer control of email formatting, queuing, and delivery processes. This is crucial for applications that handle extensive email functionalities.
- Better Performance and Reliability: Server-side environments can manage heavy Lambda architectures and API calls without affecting the end-user experience directly. An efficient server can send multiple emails simultaneously.
- Integration with Other Back-End Services: Easier integrations with databases and other server-side APIs enhance functionality. For instance, you might pull user data dynamically to personalize emails.
In summary, the trade-offs between client-side and server-side methods are significant. While client-side can facilitate user interactions, server-side technologies often provide necessary controls and security for tasks like sending emails.
This understanding informs the design of applications and helps developers choose the most appropriate strategies for implementing email functionality.
Using SMTP for Email Sending
The Simple Mail Transfer Protocol (SMTP) is central to the email sending process, and understanding its functionality is crucial when sending emails via JavaScript. SMTP is the standard protocol that governs the transmission of emails between servers and provides a robust framework for effective communication. Utilizing SMTP not only ensures that your emails are sent efficiently, but it also facilitates aspects like error handling and delivery status verification. A comprehensive grasp of SMTP can significantly impact your ability to integrate email functionality into JavaScript applications.
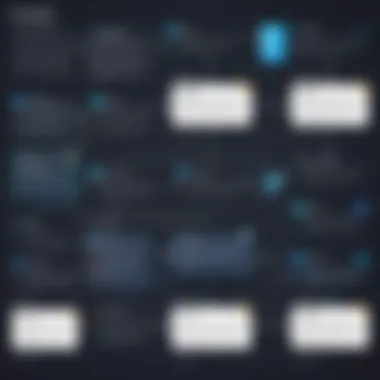
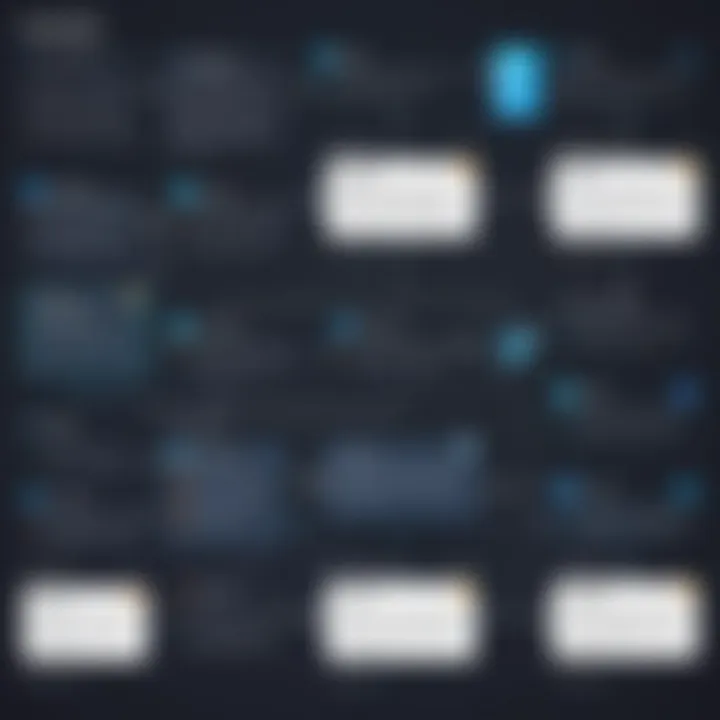
Overview of SMTP Protocol
SMTP is a protocol used to send emails between servers. It operates on the client-server model, where commands from the client are delivered to an SMTP server that then forwards the email to the recipient's email server. Like any network protocol, understanding its commands and data flow can enhance how you utilize it.
Key Elements of SMTP:
- Communication: The protocol works over Transmission Control Protocol (TCP). SMTP generally operates on port 25, though it can also use ports 587 and 465 for secure communication.
- Text-Based Protocol: Everything in SMTP is sent as text, making it easy for developers to understand the commands that are sent to the server.
- Statelessness: Each SMTP session is independent. Once a message is analogously passed, no memory of the previous sessions is kept.
- Error Codes: Responses include numeric status codes, aiding detection and troubleshooting of issues.
SMTP serves as the backbone for the email sending process and understanding its structure is critical for any developer aiming to implement effective functionality in their applications. The details outlined become vital as we discuss the practical application through JavaScript.
Establishing SMTP Connections
Setting up an SMTP connection typically involves three core steps: resolving the SMTP server, authenticating the user, and collaborating with the server for message transfer.
- Networking Requirements: Ensure that your server's firewall permits connections on the SMTP ports being utilized. Connection failures can often trace back to network restrictions.
- Authentication Procedures: Many SMTP servers require login credentials to authenticate users before sending emails. Knowing how to handle Authentication mechanisms, such as Basic, OAut, or CRAM-MD5, can secure your communications. Implementing the right credentials ensures your messages are not flagged as spam.
- Email Formatting: Craft your emails in compliance with the SMTP standards, including defining subjects, text, and HTML content. Invalid formatting can potentially lead to delivery failures. Make use of code samples for setting up and expressing connections in your application. For instance:
By grasping these essential components of connecting to SMTP servers, you position yourself to effectively handle sending and receiving emails within mere layers of JavaScript logic. Furthermore, it empowers you to diagnose potential late-stage errors and provide adjustments swiftly. By practicing SMTP fundamentals properly, you'll also improve email sending success overall.
Understanding SMTP is essential in programmer arenas, as it lays the path for secure and organized email transmission. Streamline your connections, and enhance your capabilities subsequently.
Email Sending Libraries in JavaScript
Email sending libraries play a crucial role in the JavaScript ecosystem, especially for developers seeking to implement email features in their applications. These libraries provide structured approaches that simplify the process of sending emails compared to building functionalities from scratch. Using a robust library can save time and reduce potential errors in the implementation. Each library serves specific needs, depending on whether the application runs on a client or server. This section will delve into two popular libraries, highlighting their benefits and considerations.
Nodemailer for Node.
js
Nodemailer is a popular library built for Node.js, primarily aimed at server-side applications. Its main advantage lies in the simplicity with which developers can send emails using SMTP. Nodemailer supports various transport methods such as SMTP, Sendmail, and native stmp, making it versatile for multiple use cases.
Using Nodemailer is convenient for beginners as well as experienced programmers. The library is well-documented. With a few lines of code, developers can create and send emails easily. It supports attachments, HTML content, and custom headers, offering flexibility for more user-oriented email messages. Below is a basic example of how to use Nodemailer:
const nodemailer = require('nodemailer');
const transporter = nodemailer.createTransport( host: 'smtp.example.com', port: 587, auth: user: 'your-email@example.com', pass: 'yourpassword'
const sendEmail = (to, subject, text) => const mailOptions = from: 'your-email@example.com', to: to, subject: subject, text: text
transporter.sendMail(mailOptions, (error, info) => if (error) return console.log('Error occurred: ' + error.message); console.log('Message sent: %s', info.messageId);