Starting a Node Server: A Comprehensive Guide
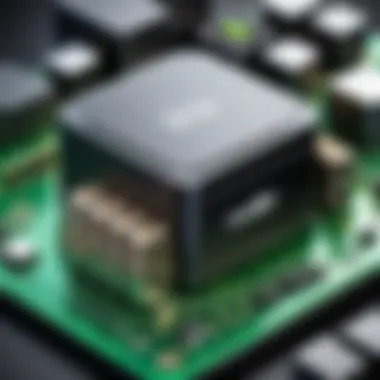
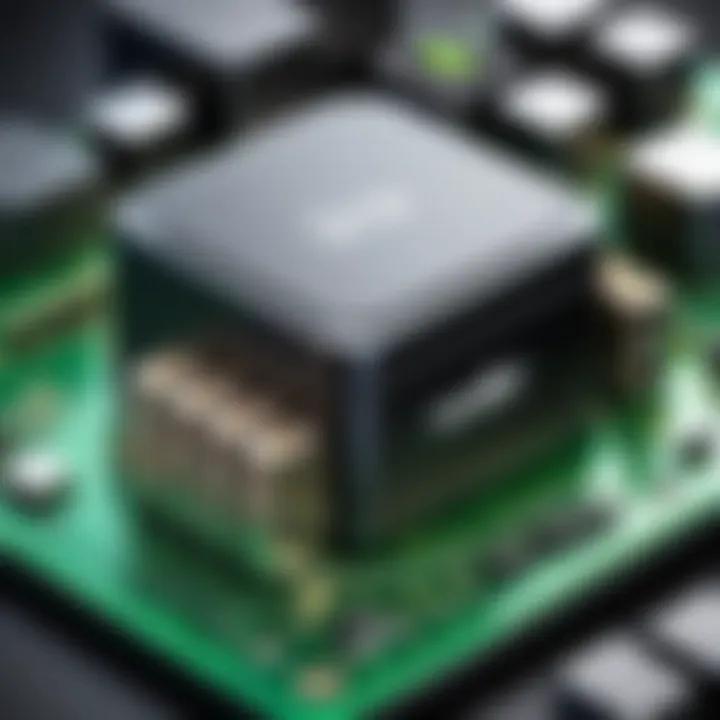
Overview of Topic
Starting a Node.js server can seem daunting for those new to programming, yet dissecting this process reveals its intrinsic value in today's tech world. Node.js embodies a powerful runtime environment built on Chrome's V8 engine. But understanding this tech marvel isnât just for seasoned pundits; it directly affects anyone getting into backend development.
The need for scalable and high-performance applications has rapidly evolved. Before the advent of Node.js in 2009, developers often relied on more traditional languages and architectures that couldn't easily handle concurrent processing. Node.js emerged as a game-changer with its event-driven, non-blocking I/O model. This architecture allows developers to manage numerous connections simultaneously, making Node.js ideal for real-time applications.
In this article, we will demystify starting a Node.js server, focus on the necessary components, and explore potential challenges. We'll break it down into different sections, ensuring a robust understanding of the key aspects surrounding server initialization and maintenance.
Fundamentals Explained
To lay a solid groundwork, itâs essential to grasp some core principles behind Node.js. The asynchronous nature of Node.js allows executing multiple operations without waiting on each to finish sequentially. This is where our main terminology comes into play:
- Event Loop: This is the heart of Node.js, allowing it to perform non-blocking I/O operations.
- Callback: A function passed to another function, which will execute after the completion of the first function.
- Modules: Individual units of functionality that can be loaded into a Node.js application, promoting reusability.
Additionally, understanding the difference between synchronous and asynchronous processes is crucial. Synchronous code runs linearly, while asynchronous code allows operations to occur independently, optimizing your server's performance considerably.
Practical Applications and Examples
Imagine you are developing a chat application. With Node.js, you can create a real-time communication platform where multiple users can engage without delay. Here's a simple demonstration with , one of the most popular frameworks built on top of Node.js:
This snippet sets up a basic server that listens on port 3000. Explore modifications to add features or manage data more effectively.
Advanced Topics and Latest Trends
As Node.js continues to mature, multiple trending methodologies emerge. For example, Microservices Architecture is gaining traction. It entails breaking an application down into smaller services that can be developed and deployed individually. This not only improves scalability but also eases the management of complex applications. Furthermore, the integration of TypeScript with Node.js has become increasingly popular, allowing developers to leverage static typing, ultimately enhancing code quality and maintainability.
Tips and Resources for Further Learning
To dive deeper and refine your Node.js skills, consider these valuable resources:
- Books: "Node.js Design Patterns" by Mario Casciaro is an excellent starting point.
- Online Courses: Platforms like Udacity or Coursera offer specialized courses on Node.js development.
- Communities: Sites like Reddit have a thriving Node.js community, where you can ask questions and share insights.
In summary, the journey towards mastering Node.js server initiation is laden with exciting challenges and ample opportunities for robust application development. Harnessing the elements explored here provides a solid foundation, propelling you into the vast ecosystem of server-side programming.
Foreword to Node.js
Node.js has made quite a splash in the world of web development and server-side programming, and thatâs no accident. Understanding Node.js is paramount for anyone looking to venture into backend programming, particularly when developing scalable applications. By utilizing its unique architecture, developers can build applications that handle numerous connections simultaneously without breaking a sweat.
This section delves into the essence of Node.js, highlighting its fundamental principles and the advantages it brings to the table. Itâs not just a trend; it's a major shift toward more efficient and adaptable server solutions. You may be wondering, "What sets Node.js apart from traditional server technologies?" Well, grabbing hold of its core ideas can truly enhance your programming repertoire.
What is Node.js?
Node.js is essentially a runtime built on the V8 JavaScript engine, which was developed by Google. It allows you to execute JavaScript code on the server-side, a concept historically reserved for the clientâs browser.
With that, Node.js enables developers to write both server-side and client-side code in JavaScript.
However, its appeal goes beyond mere language uniformity. Node.js operates on an event-driven, non-blocking I/O model, which ensures that operations can scale efficiently. This capacity for scalability has gained the nod from budding developers and seasoned pros alike, making it an attractive option for a range of applications from personal projects to large-scale enterprise solutions.
Key Features of Node.js
- Single-threaded Model
One significant characteristic of Node.js is its single-threaded architecture. At first glance, that might seem limiting. You're used to thinking that more threads mean better performance, right? However, Node.js flips that notion on its head. It utilizes a single-threaded event loop to handle multiple connections concurrently, which minimizes the overhead that typically comes with multi-threading. This unique handling allows developers to build more efficient applications that can serve many users at once without crashing or slowing down. - Asynchronous Processing
Asynchronous processing is where Node.js really shines. This feature allows tasks to be processed independently from the main thread. Instead of waiting for one task to finish, Node.js will kick off the next one, keeping things moving. This leads to a more responsive server. When leveraging this feature, you're not just waiting around for something to happen, but rather maximizing system resources effectively. - Non-blocking I/O
When it comes to I/O operations, traditional models can become bottlenecks. Enter non-blocking I/Oâa cornerstone of Node.js. With non-blocking I/O, operations like reading files or fetching data from a database won't halt the execution of other tasks. In other words, while your program waits for these I/O tasks to finish, it can carry on with others. This feature effectively enhances performance and reduces latency issues, making it an optimal choice for real-time applications.
To summarize, Node.js offers an efficient approach to building applications that require high scalability and performance, leveraging its single-threaded model, asynchronous processing, and non-blocking I/O capabilities.
Prerequisites for Starting a Node Server
Before diving headfirst into setting up a Node server, it's crucial to understand the essential building blocks that lay the foundation for your development journey. Let's face it, starting with a solid ground makes all the difference.
At its core, the prerequisites for starting a Node server are all about ensuring you have the right tools and knowledge in place. It's like trying to bake a cake without knowing what ingredients you need or which oven works best. Having Node.js and npm (Node Package Manager) installed is just scratching the surface. You also need to establish a conducive working environment and familiarize yourself with the command line, which can feel daunting if you're not accustomed to it. This groundwork will significantly ease your path towards creating robust applications, and ultimately, boost your confidence as you navigate through coding.
Install Node.js and npm
Choosing the Right Version
When it comes to selecting the version of Node.js for installation, the choice often depends on the compatibility with your project. The latest stable version is typically the best route to take, offering up-to-date features and security patches. However, if you're working on an established project, you may be inclined to stick with an earlier version to avoid breaking changes.
One notable aspect of choosing the right version is the Long-Term Support (LTS) release. This version is especially designed for production environments, providing a stable platform without the frills and potential kinks that come with the latest features in current versions. Opting for LTS ensures you're making a beneficial choice for your server stability and security, which goes hand-in-hand with the essentials of maintaining a reliable application.
Installation Steps
Installing Node.js and npm isnât as tricky as climbing Mount Everest, though it does require some attention to detail. The typical approach involves downloading an installer from Node.jsâs official website, running it, and following prompts. Windows, macOS, and Linux systems have their own quirks during installation, so being aware of these specifics is beneficial.
Once the basic installation is complete, checking that everything's running smoothly can be done through the command line using commands like and . This quick verification is critical because it reassures you that your setup is functional and ready for the next steps.
Setting Up Your Environment
IDE Selection
Choosing the right Integrated Development Environment (IDE) can be the beacon that lights your coding path. Popularly, tools like Visual Studio Code, WebStorm, and Atom take center stage due to their rich ecosystems and extensibility.
Visual Studio Code, in particular, stands out with its straightforward interface, built-in terminal, and support for a wide array of extensions. That makes it a solid companion for beginners and seasoned coders alike. Opting for a user-friendly IDE prevents the intimidation of overwhelming settings and allows you to focus on what truly matters â writing code.
Basic Command Line Knowledge
Having basic command line knowledge is like possessing a secret weapon in the tech world. Understanding commands such as , , and are fundamental when working with Node.js. This knowledge not only boosts your efficiency but also empowers you to navigate your project's directories with ease.
Moreover, command line skills come with the added benefit of making you more versatile as a developer. They can help you interact with version control systems or even deploy your applications. While it might feel a bit tedious to learn at the start, mastering this skill can open a treasure trove of possibilities in your programming journey.
"Programming isn't about what you know; it's about what you can figure out."
â Chris Pine
As we wrap up this section, keep in mind that these prerequisites are not just hurdles to jump over but solid scaffolding on which you can build impressive Node applications. Proper setup saves you time and potential headaches later on.
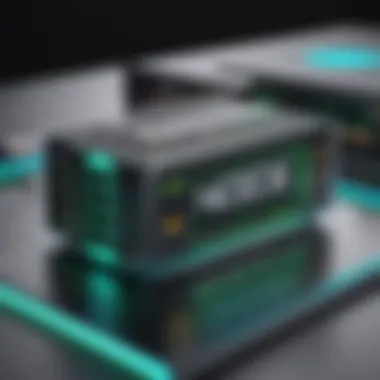
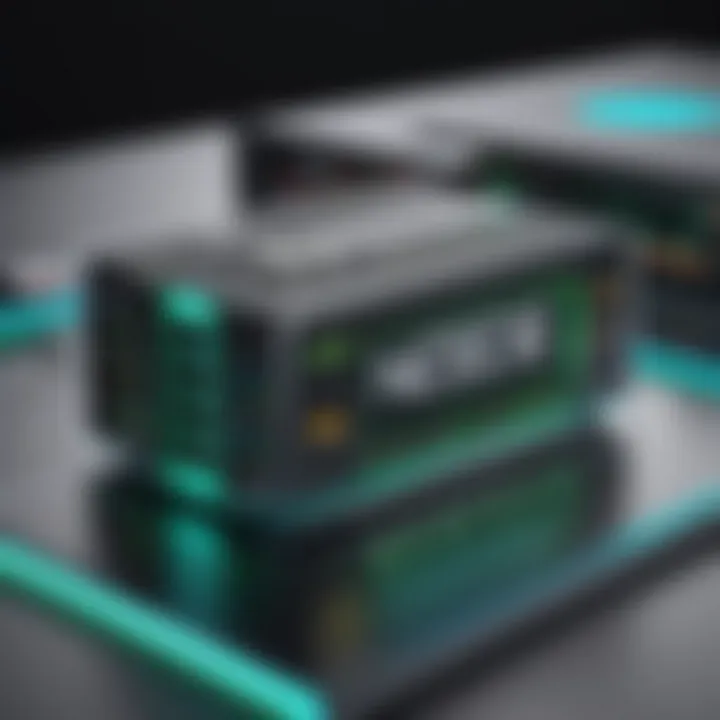
Creating Your First Node Server
Setting up your first Node server is a crucial milestone for anyone venturing into web development. This process acts like a rite of passage, marking the transition from theoretical knowledge to practical application. In the digital world, a Node server serves as the backbone for scalable applications, allowing developers to handle numerous connections concurrently. This section will lay the groundwork for creating a simple yet effective Node server, focusing on the crucial elements required to get it off the ground.
Basic Server Structure
Creating a solid foundation for your server is essential. Let's break it down into two key components: importing required modules and defining server logic.
Importing Required Modules
When it comes to setting up a Node server, one of the first steps involves importing the necessary modules. The module is a linchpin for server functionality in Node.js. By requiring this module, you gain access to a suite of tools that allow you to create HTTP servers and handle requests. In essence, importing required modules clears the path towards harnessing the capabilities of Node.
A key characteristic of importing these modules is the simplicity it offers. Unlike many languages that might require extensive configurations, Node.js allows for a straightforward approach. To import the module, you can use the following line of code:
This a succinct yet powerful way to initiate your server's journey. The unique feature here is the asynchronous nature that Node exhibits, making it a highly favorable choice for real-time applications. The drawback, however, is that if you don't correctly structure the modules, debugging can quickly become a labyrinthine endeavor. Still, the advantages far outweigh the consâsimplicity and power rolled into one.
Defining Server Logic
After you've imported your required modules, defining your server logic becomes the next significant stage. This involves setting up the responses your server will handle and the actions it will perform when a request comes in. At its core, server logic functions as the brain of your server, interpreting incoming requests and producing corresponding responses.
A beneficial aspect of defining server logic is the flexibility it provides. You can craft logic that serves various endpoints, meaning your server can handle multiple routes seamlessly. For instance, consider the following snippet:
This code creates a basic server responding with "Hello World" to any request. The unique feature of this approach is the capability to scale; as your application grows, so too can your logic, allowing for more complex interactions. However, a disadvantage might be the risk of overcrowding your server with too much logic at once, which can lead to maintenance challenges.
Starting the Server
Once the structure is in place, the next step is getting your server started. This involves two primary components: listening on a port and handling requests.
Listening on Port
Listening on a port is what keeps your server alive and ready to accept incoming connections. It's a straightforward yet fundamental aspect, and it underscores the concept of communication between your server and users.
A distinctive feature of ports is that they allow multiple services to operate on the same machine, each distinguished by a unique port number. For instance, here's how you can make your server listen on port 3000:
This line initiates the server and makes it accessible through the specified URL. While this might seem like a minor detail, it's a critical step in connecting your application to the wider internet. But remember, if the port is already in use, your server won't start, adding an extra layer of consideration when deploying.
Handling Requests
Once the server is listening, you must prepare to manage incoming requests. Handling requests means dealing with what clients send and responding appropriately. This might include serving static files or JSON data, among other responsibilities.
A key characteristic of request handling is adaptability. Whether your server needs to deliver a simple HTML page or complex data, the logic crafted earlier can be adjusted accordingly. One able approach to manually manage requests would look like this:
This snippet allows you to log requests as they come in. The unique advantage here is logging can help diagnose issues and understand user interactions better. On the flip side, if mismanaged, excessive logging can slow performance.
"The moment you visit Node.js, you begin a journey towards mastering efficient server-side programming."
Configuring Your Node Server
Configuring your Node server is not just a checkbox on a development checklist; it's the backbone that holds everything together. This phase sets the stage for your application, enabling it to operate smoothly right from the get-go. Basically, how you configure your server can affect performance, security, and even future scalability.
When it comes to the details, using environment variables and setting up middleware are key themes that affect how your server interacts with various environments and handles requests.
Using Environment Variables
Understanding .env Files
So, whatâs the deal with .env files? Theyâre crucial, really. A .env file is where you neatly tuck away environment-specific settings like API keys, database URIs, or any confidential information. Picture this file as a secure storage box for your sensitive credentials, making them less vulnerable. The practice of using .env files has become quite popular among developers because it separates configuration from code, promoting better security and maintainability.
One of the neat characteristics of .env files is their ease of use. You just define your variables in a simple key-value pair format, for example:
A drawback, though, could be that if not handled properly, you might accidentally expose this file through version control systems like Git. That's why understanding the context around them is important. Plenty of developers use dotenv, a popular package, to load these variables when the application starts.
Accessing Environment Variables in Node
Now, accessing these environment variables in Node is a breeze. You can simply use , and voilĂ , youâve got access to your secure strings. This makes your code cleaner, because youâre not hardcoding secrets everywhere.
The ability to dynamically access these variables provides great flexibility. You can change configurations without altering your code base, which is invaluable during production. However, the limitation comes when a typo slips through; if you request a variable that doesnât exist, youâll end up with undefined values, which could lead to unexpected behavior.
Setting Up Middleware
Prelude to Middleware
Middleware is often seen as the unsung hero of Node applications. It's essentially functions that get executed during the lifecycle of a request to your server. What makes middleware so special is its ability to enhance the way your application processes data. From logging requests to validating incoming data, middleware assumes many important roles.
This is a beneficial approach because it allows you to keep your code modular and easier to maintain. Instead of writing the same logic repeatedly across different routes, middleware serves as a reusable solution to common tasks.
Commonly Used Middleware Packages
When you look into middleware, various packages stand out. Express.js is one of the most widely used frameworks, and it comes with built-in middleware that simplifies common tasks. For instance, is a middleware for parsing incoming request bodies, while enables Cross-Origin Resource Sharing, which is essential for APIs.
These packages offer their unique features; however, they also involve some trade-offs. Implementing too many pieces of middleware can potentially slow down your server, so it's crucial to choose wisely. By understanding the strengths and weaknesses of each, you can streamline your application for optimal performance.
Middleware is your friend; it fills in the blanks that your server canât cover by itself, enhancing both the security and functionality of your app.
Error Handling in Node Server
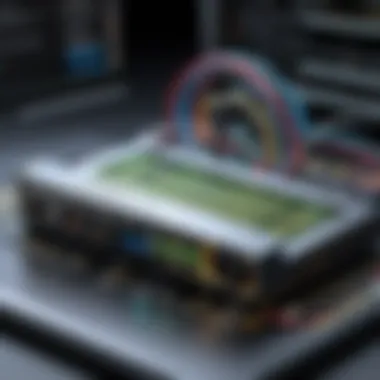
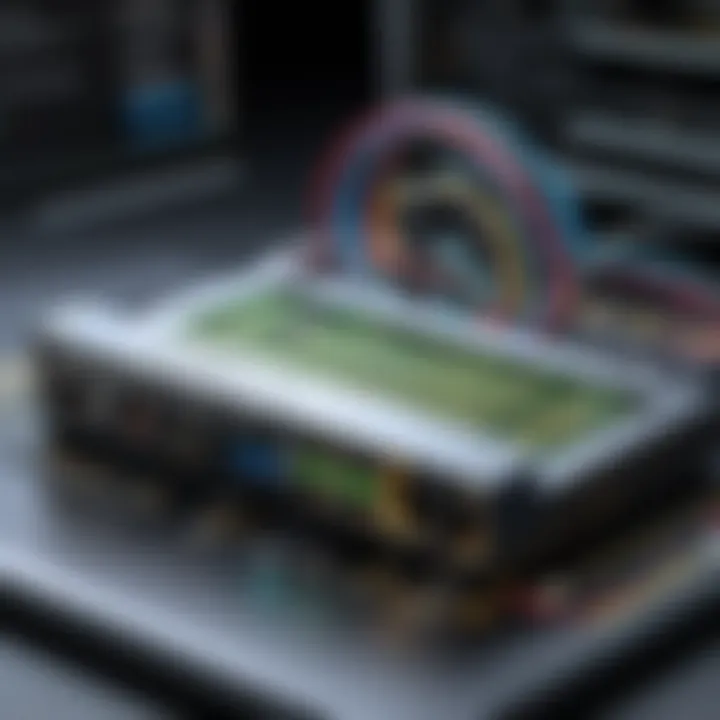
Handling errors effectively is vital when creating a Node.js server. Errors can be like a pebble in your shoe; they may seem small but can significantly hinder performance and user experience. By having a robust error handling strategy, developers can catch issues before they escalate and ensure that the application runs smoothly. In this section, we will explore common error scenarios, their implications, and the best mechanisms to manage them.
Common Error Scenarios
Request Errors
Request errors are a common hurdle in any server application. These occur when the server encounters a problem while processing incoming requests, which can lead to 404 errors or timeouts. The important characteristic of request errors is how they can arise from invalid input or unreachable endpoints. For many developers, understanding these errors provides a clearer picture of client-server interactions.
Unique Feature of Request Errors: Their unpredictability. Request errors can happen due to user mistakes or network issues. This variability is what makes identifying and addressing them crucial. For example, a user might send a bad request that the server can't interpret. The advantages of dealing with such errors include improved user experience and resilience in the application. However, they can be time-consuming to debug, especially in a production environment.
Server Crashes
Server crashes represent a more severe issue, where the server becomes unresponsive or goes offline unexpectedly. This type of error can be detrimental, causing downtime and frustrating user experience. A key characteristic of server crashes is their often unexpected nature. Sudden spikes in traffic or unhandled exceptions can trigger a crash, making monitoring essential for preventing such occurrences.
Unique Feature of Server Crashes: The often catastrophic impact on the applicationâs availability. When a crash occurs, users may receive no response or error messages. The advantages of proactively addressing potential crash points include enhanced reliability and user trust. However, it can require a substantial amount of time and effort to diagnose the root causes of crashes, complicating the maintenance process.
Implementing Error Handling Mechanisms
To manage and mitigate the effects of errors effectively, developers should implement solid error handling mechanisms.
Using try-catch
Using try-catch blocks is an essential practice for controlling errors in Node.js applications. The fundamental aspect here is that try-catch allows developers to "try" a piece of code and gracefully handle any exceptions that arise, preventing the entire application from crashing. It's popular among developers mainly because it promotes a fail-safe environment.
Unique Feature of try-catch: It isolates and manages errors locally. By catching errors right where they occur, the consequences can be managed without affecting the entire server. The robust nature of this method lies in its simplicity and ease of implementation. However, overusing try-catch without proper structure can lead to scattered error management, making it difficult to maintain.
Error Handling Middleware
Error handling middleware is another critical component for managing errors in Node.js applications. This middleware acts as a centralized point for all errors. One significant aspect of error handling middleware is its ability to streamline how errors are processed across different routes of your application. This feature makes it an attractive choice for maintaining organized code.
Unique Feature of Error Handling Middleware: The capability to catch errors across multiple routes. By funneling errors to one middleware, developers can manage them cohesively. This results in better readability and maintainability of the code. However, it does require an understanding of how middleware interacts with the request-response cycle in an application, which can pose initial learning challenges for newcomers.
"An ounce of prevention is worth a pound of cure." By proactively addressing errors, developers can save loads of time and deliver a smoother experience for their users.
Deployment Considerations
When it comes to launching your Node server, the deployment phase is critical. This stage determines how your application will run in a real-world setting, affecting everything from performance to reliability. A well-planned deployment can make your app robust, responsive, and safe from potential threats. The focus here is on choosing the right hosting platform and adhering to best practices that will ensure your application is prepared for prime time.
Choosing a Hosting Platform
Choosing the right hosting platform can be a make-or-break decision for your Node server. It is essential to evaluate each option based on aspects such as ease of use, cost, scalability, and support for Node.js.
Heroku
Heroku is often regarded as a gateway for many developers starting with cloud deployment. One of the standout characteristics of Heroku is its simplicity. With a smooth learning curve, deploying and scaling applications can be done with just a few commands. This makes it a beneficial choice for beginners who are nervous executing server deployments while still allowing more experienced developers to deploy quickly without too much hassle.
A unique feature of Heroku is its Add-ons Marketplace, which offers a plethora of third-party tools that can easily integrate with your application. For instance, you can add databases or monitoring tools with minimal setup. However, Heroku may become costly as your app scales due to its pricing model that charges per resource utilized. Therefore, while itâs excellent for quick launches, itâs important to keep an eye on expenses as traffic grows.
DigitalOcean
DigitalOcean shines with its flexibility and is often seen as a solid choice for developers looking for more control over their infrastructure. With the ability to create Droplets, DigitalOcean allows you to choose from a variety of configurations tailored to your needs, which means you can optimize costs by only paying for what you use. This makes it particularly appealing to those who understand the ins and outs of server management.
Another notable feature is the Community Tutorials that DigitalOcean offers. These resources are invaluable for troubleshooting and mastering server management skills, which can enhance your application's performance. On the flip side, the downside is that it may require more technical know-how compared to more packaged solutions like Heroku.
Best Practices for Production Environment
A production environment is where your application will be subjected to actual load, so best practices here are not just beneficial; theyâre essential.
Security Configurations
Security configurations are paramount in safeguarding your application from myriad threats. Some key characteristics include implementing SSL, using secure HTTP headers, and ensuring proper user authentication. These are foundational elements in protecting your data and users, pointing to why due diligence in security is so critical.
A unique aspect of security configurations is the use of tools like Helmet, a middleware that helps secure your Express apps by setting various HTTP headers. However, neglecting these configurations might lead to disastrous data breaches or other vulnerabilities that can tarnish your reputation and lead to significant financial loss.
Performance Optimization
Performance optimization cannot be overlooked either. Optimizing performance involves various tactics such as load balancing, using caching strategies, and minimizing resource load times. A key characteristic of performance optimization is its ability to drastically improve user experience. A fast and responsive app is likely to enhance user retention and satisfaction, a goal for any application developer.
One unique feature of performance optimization is the use of Cluster Mode in Node.js, which can leverage multi-core systems to improve performance. However, if not implemented correctly, these optimizations can complicate your codebase and debugging processes, leading to a increased complexity.
In summary, deploying a Node.js server is not just a technical task. It involves careful consideration of hosting platforms and implementing best practices for securing and optimizing your application. Keeping these factors in mind ensures a successful deployment that can handle the rigors of real-world use.
"Failing to plan is planning to fail." - A reminder that deployment decisions shouldnât be taken lightly.
Testing Your Node Server
Testing your Node server is paramount. It ensures your application runs as intended, floods you with confidence before you hit the world with your project, and helps catch unforeseen issues that could arise in different environments. You wouldnât want to launch a beautiful ship only to have it sink on its maiden voyage because somebody forgot to check the bolts, right? This section delves into two primary types of testing: unit testing and integration testing, each with their own unique roles in maintaining a healthy Node server.
Unit Testing Basics
Unit testing is the process of verifying that individual pieces of your code behave as expected. It resembles taking a magnifying glass to the nuts and bolts of your project, ensuring every single one is polished and performing correctly. This type of test provides immediate feedback during development, which is invaluable when sifting through large codebases.
Choosing a Testing Framework
When it comes to unit testing in Node.js, selecting the right testing framework is crucial. A popular choice is Mocha. Its flexibility and simplicity make it a fan favorite. Mocha allows you to structure your tests as you see fit, whether you prefer behavior-driven or test-driven development. How you can write clear and expressive tests is a leading edge, plus it facilitates both synchronous and asynchronous testing.
Despite its advantages, Mocha doesn't come preload with assertion libraries, so you may find yourself batteling with what to choose next. Chai, for example, is often paired with Mocha to enhance expressiveness in assertions. The necessity to bring additional tools into the mix may complicate things slightly for beginners, but ultimately it amounts to a custom-fit solution that lets you tailor your testing environment.
Writing Basic Tests
Writing basic tests is like crafting a sturdy foundation before erecting the walls. In Node.js, you could start by setting up a simple test case that checks the functionality of a function. The goal is clarity and safety; thus, ensuring that each function performs its duty accurately is key.
A unique feature of writing these tests in Node is its native support for async testing, making it easier to handle promises. Once youâve set up your environment using Mocha with Chai, youâll find that writing readable and maintainable tests gets more natural over time. However, one drawback can be the initial learning curve, particularly if you're not familiar with how testing frameworks operate.
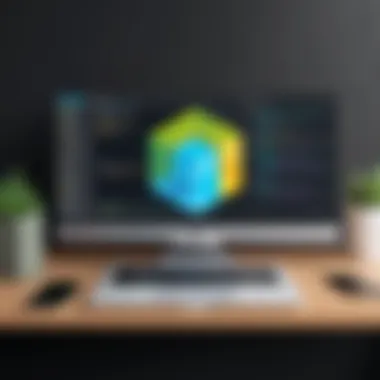
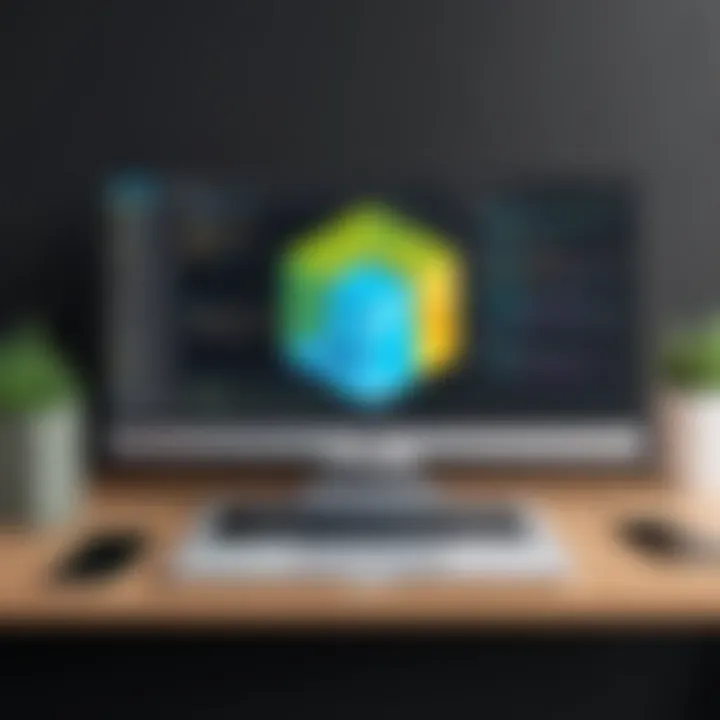
Integration Testing
Integration testing dives deeper than unit testing by checking how well different modules of your application cooperate with each other. Itâs essential for ensuring that as parts of the ship are locked together, they truly work in harmony.
Setting Up Test Cases
Setting up test cases for integration tests requires a shift in focus from the individual parts of your application to their interplay. A common framework that aids this process is Jest, which offers an all-in-one testing solution and provides built-in mocking capabilities. The seamless handling of asynchronous operations makes it a solid choice, especially when verifying interactions between server APIs.
The downside could stem from its abstraction; for those who wish to have more control over specific aspects, the magic that Jest works behind the scenes might feel like a double-edged sword. Simplifying the process is beneficial but can obscure the underlying mechanics that some developers wish to understand more intimately.
Testing API Endpoints
Finally, testing API endpoints is part and parcel of verifying that your application can handle requests correctly. Utilizing tools like Postman for manual tests can be handy, yet pairing it with automated tests enhances your reliability and enables continuous integration workflows.
When testing API endpoints, you examine HTTP requests and responsesâconfirming that data flows smoothly between client and server. A major benefit of automated API testing is efficiency: once youâve scripted what you need, you can run those tests repeatedly without extra work.
However, maintaining these tests can sometimes fall into a gray area; if your endpoints shift frequently, your tests need to keep pace. Thus, a balance must be struck between thorough coverage and manageability.
Remember: Testing is not just a luxury for developersâit's a lifeline that can save you a nasty headache down the road. Whether it's unit tests for individual components or integration tests to ensure your modules play nicely together, getting a handle on testing now means you'll have a more robust application later.
Performance Monitoring
Monitoring the performance of a Node.js server is not just some technical nicety; itâs a necessity. As applications grow and user demand increases, keeping tabs on how well your server is doing becomes crucial. Performance monitoring equips developers with insights into how their applications behave in real-world situations. The benefits of having a robust monitoring system in place are manifold: it allows for early detection of anomalies, optimization of resource usage, and ultimately, ensures a better user experience.
Consider this: if you donât know your serverâs weak points, how can you possibly fix them? Regular performance checks help identify bottlenecks, be it due to memory leaks, slow queries, or inadequate resources. Asking questions like "Why is this response lagging?" or "How can I better handle concurrent requests?" can steer developers toward solutions that boost efficiency.
Furthermore, monitoring provides a data-driven approach to decision-making regarding server scaling and upgrades. Knowing when to ramp up your resources can save money and ensure that your application meets user demands without a hitch.
Tools for Monitoring
Different tools cater to various monitoring needs, from simple marking systems to comprehensive performance analysis setups. Among the notable solutions are Prometheus and New Relic.
Prometheus
Prometheus stands out in the realm of monitoring tools. It is an open-source monitoring solution that emphasizes reliability and ease of use. One of its key characteristics is its ability to scrape data from configured endpoints at specified time intervals. This feature is beneficial for continuously tracking metrics and performance data, which is essential for timely insights.
Unique Feature: One notable capability of Prometheus is its robust query language, PromQL, which allows users to extract and manipulate data with a considerable degree of flexibility. This makes it easy to examine past performance or project future metrics.
In terms of advantages, Prometheus provides excellent scalability and can handle service discovery seamlessly, which is a boon for dynamically changing environments. However, it does come with challenges; setting up and configuring Prometheus to cater to specific needs might require a steep learning curve for newcomers.
New Relic
New Relic is another prominent player in this field, known for its cloud-based performance monitoring. The main draw for many is its comprehensive dashboard that gives an overview of application performance metrics at a glance. This simplifies the task of monitoring complex systems that involve multiple services.
Unique Feature: One of New Relic's standout features is its APM (Application Performance Monitoring) capabilities, which allows developers to trace transactions across distributed systems. This is particularly useful for pinpointing performance issues that might arise in a microservices architecture.
While New Relic offers a host of powerful features, downsides such as costs can be a significant consideration for smaller projects or startups. Still, with its rich feature set and robust support, developers often find that the benefits outweigh potential drawbacks.
Key Performance Metrics
When monitoring server performance, a few metrics become the golden nuggets that everyone tends to look at: response time and throughput. Understanding these metrics can lead to better optimization and troubleshooting.
Response Time
Response time, or the duration it takes for the server to respond to a client's request, is one of the most critical metrics. A low response time indicates a swift server, which ultimately means better user experience. This key characteristic makes it immensely valuable for any node server operation.
Unique Feature: A fascinating aspect of response time measurement is that it can often identify specific issues within the server's processing logic. Tools like Prometheus can visualize this data in real-time, enabling quick modifications when necessary.
While there are advantages to focusing on response time, one must also be mindful of the variability in load and response. Whatâs fast under low load might become slow when traffic surges, which should prompt developers to stay vigilant regarding scalability resource allocation.
Throughput
Throughput measures how many requests your server can handle in a given timeframe. A higher throughput is generally indicative of a more efficient server. Like response time, throughput provides critical insights into how well a server performs under varying loads.
Unique Feature: The beauty of throughput monitoring lies in its capability to guide architecture decisions. If latency becomes an issue, analyzing throughput might reveal whether the issue lies in the infrastructure or the application's design.
Just like response time, throughput has its quirks. An application can exhibit high throughput but might suffer from poor response times under high concurrency. Hence, striking the right balance between the two metrics is vital for optimal server performance.
Epilogue and Further Reading
Wrapping up this comprehensive guide, itâs vital to understand the significance of weaving together the various aspects of starting and managing a Node.js server. This section serves to consolidate the knowledge gained and point you toward resources for further growth in your understanding of Node.js.
Recap of Key Points
Throughout the guide, we explored the fundamental elements required to start a Node.js server. One of the core focuses was the various configurations and features that define Node.js, such as asynchronous processing and non-blocking I/O.
We discussed the importance of properly setting up your environment, highlighted how to create and configure a server, and delved into error handling and performance monitoring. Each topic builds upon the previous one, creating a clear pathway from initial setup to deployment and beyond.
To sum it up:
- Understanding Node.js: Key features and principles are crucial.
- Environment Setup: Ensures a smooth development process.
- Server Creation and Configuration: The backbone of your application.
- Error Handling Mechanisms: Essential for application stability.
- Performance Monitoring: Helps you keep track of how your server performs in real-time.
Resources for Continued Learning
To deepen your knowledge and skills, engaging with resources that further explain and illustrate the concepts of Node.js is key. Here are two prominent sources you might want to consider:
Online Tutorials
Online tutorials are an excellent way to gain hands-on experience and visual understanding of the topics you've learned. Often, these tutorials provide step-by-step guides that are interactive, making them a practical choice for grasping complex concepts in a digestible format. A key characteristic of these tutorials is that they often cater to various skill levels, allowing you to start as a beginner and gradually tackle more advanced subjects.
Unique features include the opportunity for practice through exercises and projects, which directly relates to your learning experience. However, one must be wary of the quality of different tutorials, as not all may be created equal. Itâs always wise to check the credibility of the source offering the tutorial.
Node.js Documentation
The official Node.js documentation is perhaps the most exhaustive resource available for anyone working with Node.js. Itâs frequently updated and provides comprehensive insights into its API and various modules. The key characteristic here is the level of detail it offers, which is critical for developers looking to understand the inner workings of Node.js.
This resource is beneficial as it serves as an authoritative reference point when questions arise during development. The unique feature of this documentation is its structured layout, guiding you seamlessly through the setup, module documentation, and usage examples. A potential downside could be the complexity of the wording; at times, it may feel overwhelming to newcomers. Nonetheless, with patience, it becomes an invaluable asset.
"The only source of knowledge is experience." â Albert Einstein
With these resources at your fingertips, you have the foundation necessary to further your journey within the realm of Node.js. Embrace the learning process; every line of code written contributes to your skills and confidence as a developer.
Remember, programming is a continuous journey, not a destination.