Starting a Node.js Project: A Comprehensive Guide
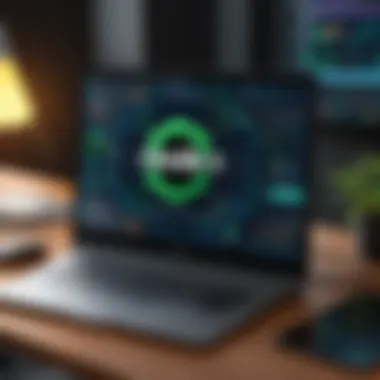
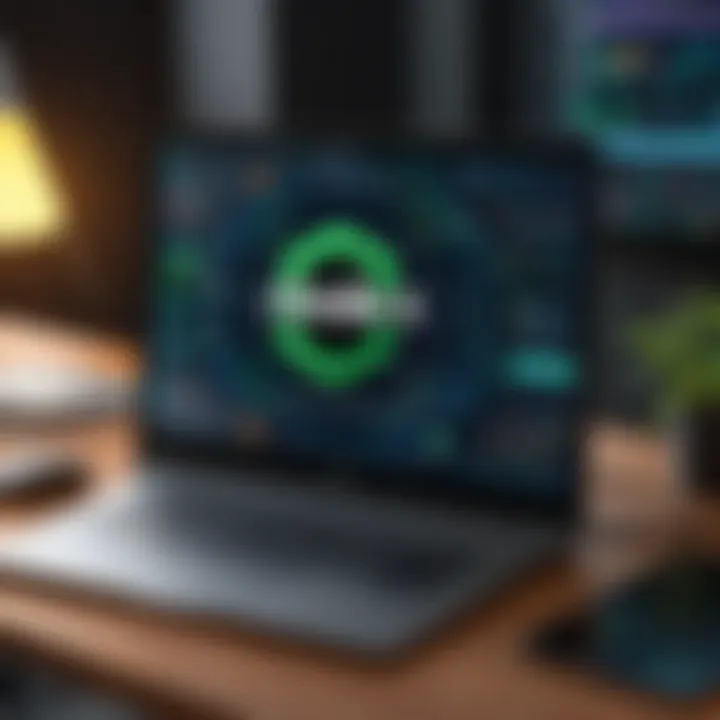
Overview of Topic
Starting a Node.js project can seem daunting, especially for those who are just tiptoeing into the realm of JavaScript and server-side development. This guide unpacks the journey of initiating a Node.js project step by step. By the end, readers will have a solid grasp of the fundamentals required to set their own projects in motion.
The significance of Node.js in the tech industry cannot be understated. Originally released in 2009, this JavaScript runtime has changed the way we build applications by allowing developers to use JavaScript both on the client and server side. It spurred the rise of event-driven programming models and non-blocking I/O, which are now foundational in the development of modern web applications.
Fundamentals Explained
To lay the groundwork, it’s crucial to understand a few core principles and terminologies surrounding Node.js.
- JavaScript Runtime: Node.js is built on Chrome's V8 JavaScript engine, enabling execution of JavaScript code outside of a web browser.
- Event-Driven Architecture: A programming paradigm where the flow of the program is determined by events such as user actions or sensor outputs.
- Non-blocking I/O: Unlike traditional I/O operations, non-blocking I/O allows Node.js to handle multiple operations at the same time, which can significantly boost application performance.
Being familiar with these concepts not only aids in grasping how Node.js works but also serves as a solid foundation as we move forward with this guide.
Practical Applications and Examples
Node.js has been adopted by numerous companies across various sectors. Notable examples include Etsy, LinkedIn, and Netflix, all of which benefit from its ability to handle large-scale applications with ease and speed. Here’s an example of a simple HTTP server using Node.js, just to give you a taste of what you can achieve:
This basic server listens on port 3000 and responds with "Hello World" to any incoming request. Simple yet effective!
Advanced Topics and Latest Trends
As the ecosystem evolves, several advanced techniques have popped up, like microservices architecture, which allows large applications to be built as a suite of smaller, independently deployable services. This approach has gained traction because it enhances scalability and maintainability.
Furthermore, tools like Docker and Kubernetes are increasingly integrated into Node.js development, streamlining workflows and simplifying deployment processes.
Tips and Resources for Further Learning
For those eager to dive even deeper, here are some recommended resources:
- Books: "Node.js Design Patterns" by Mario Casciaro, a gem for understanding structure and scalability.
- Online Courses: Platforms like Udemy and Coursera feature courses that cater to varying levels of experience, focusing on practical, hands-on learning.
- Tools: VSCode is an excellent choice for a development environment. Additionally, use npm for managing packages effortlessly.
Settling into Node.js is a remarkable journey where every project leads to new insights. Happy coding!
Understanding Node.js
In the world of modern web development, Node.js stands out as a transformative technology that empowers developers to create server-side applications using JavaScript. Understanding this platform is crucial for anyone venturing into building web applications with robust performance and scalability. One of the primary reasons Node.js has gained traction is its event-driven, non-blocking I/O model, which makes it exceptionally efficient for data-intensive real-time applications. This model allows developers to handle multiple connections simultaneously with minimal overhead, thereby enhancing user experience.
Node.js isn't just a fad; its practical applications and benefits are significant. With a single-threaded architecture that employs event looping, it reduces the complexity traditionally associated with multi-threaded environments. Furthermore, JavaScript's ubiquity in front-end development creates a seamless transition for developers to extend their skills to the back-end.
What is Node.js?
Node.js is built on Chrome’s V8 JavaScript engine, enabling developers to execute JavaScript code server-side. It breaks the barrier of the traditional web server model by allowing JavaScript to be used beyond the browser, providing a consistent language across both the front and back ends. Developed in 2009 by Ryan Dahl, Node.js was conceived with the intent to optimize throughput and scalability in web applications.
This non-blocking I/O model is what gives Node.js its signature performance. To put it plainly, when a Node.js application needs to read a file from the filesystem, it can send out the request and carry on with other tasks, rather than waiting idly for the file to be read. In effect, Node.js can juggle numerous requests at once, making it ideal for serving high-traffic applications.
Core Features of Node.js
Node.js brings several core features to the table that define its performance and functionality:
- Asynchronous and Event-Driven: Thanks to its event-driven non-blocking architecture, Node.js can handle multiple connections concurrently without incurring the overhead typically associated with threading. This is particularly beneficial for applications that require high throughput.
- Single Programming Language: Leveraging JavaScript for both client-side and server-side code leads to a smoother development process, allowing developers to remain focused and coherent in their coding practices.
- Rich Ecosystem: The Node Package Manager (NPM) hosts a staggering number of open-source libraries and modules, simplifying the process of adding functionalities without reinventing the wheel.
- Fast Execution: Node.js’s use of the V8 engine translates into quicker execution of JavaScript code, enhancing application performance significantly.
Common Use Cases
The versatility of Node.js has led to its adoption for various types of applications, including but not limited to:
- Real-Time Applications: Tools like chat applications or collaborative platforms benefit extensively from Node.js's ability to manage real-time data flow effortlessly.
- API Servers: It’s increasingly common to use Node.js for building RESTful APIs that require quick, efficient data exchange between servers and clients.
- Streaming Applications: The non-blocking nature of Node.js allows for smoother data streaming, as seen with video or audio streaming services.
- Single-Page Applications (SPAs): Node.js provides the backend with the responsiveness needed for SPAs, enhancing the overall user experience.
In a nutshell, grasping the concept of Node.js can be the cornerstone for aspiring developers looking to excel in the tech landscape today. This foundation will be pivotal as we explore further aspects of initiating a Node.js project.
Prerequisites for Starting a Node.js Project
Before you dive into the deep end of Node.js development, it's crucial to lay down a solid foundation. Understanding the prerequisites sets the stage for a smoother journey ahead. It’s not just about having the right tools; in fact, a grasp of the essential elements can significantly enhance your development experience and help avoid potential pitfalls.
System Requirements
To run Node.js effectively, your system must meet certain specifications. Here’s what you generally need:
- Operating System: Node.js is compatible with various operating systems such as Windows, macOS, and Linux. Make sure you are running a recent version to avoid compatibility hiccups.
- Processor: Any modern processor will typically suffice. However, a multi-core processor is ideal for smoother multitasking while developing.
- Memory (RAM): At least 8 GB of RAM is recommended to handle bigger applications and smooth workflow.
- Storage: You’ll need sufficient disk space for Node.js itself and for any packages you might install. SSDs are preferable for faster data access.
By ensuring these requirements, you’ll have a reliable environment that can handle Node.js applications effectively.
Required Software Installation
Node.js installation
When it comes to installing Node.js, it’s known for its ease of installation and accessibility. You can use the official Node.js website to download the installer for your operating system. Here’s what makes Node.js installation a stellar choice:
- Simplicity: The process is straightforward. Just a few clicks, and you’re on your way. The installers often come bundled with essential components, making setup less tedious.
- Version Control: Node.js provides a method to keep multiple versions on your machine using tools like . This is extremely useful when working on different projects requiring distinct Node.js versions.
However, one potential drawback is the complexity of troubleshooting when conflicts arise with existing installations or global packages. But with patience and persistence, you'll soon conquer any issues.
NPM basics
Once Node.js is installed, you can access npm, its package manager, which is a pivotal part of the ecosystem. Simply put, npm allows you to install, manage, and publish packages your application may need. Here’s why npm is often hailed as a game-changer:
- Extensive Library: With millions of packages available, developers have the luxury of leveraging a vast library. Need something? There’s a decent chance someone’s already built it.
- Version Management: npm facilitates the ability to specify exact versions of packages, ensuring that your project doesn’t break due to unexpected changes. It’s like having a safety net when balancing dependencies.
However, npm can sometimes lead to dependency hell, where multiple versions of a package conflict. Consequently, it demands careful management and attention. By mastering npm basics, you're already ahead of the game in handling your Node.js project.
The comprehensive understanding of prerequisites before starting a project can save you time and frustration down the road.
Arming yourself with these solid prerequisites sets the groundwork for a successful journey into the Node.js ecosystem. Starting off with the right tools and knowledge, you’ll find the development path less rocky and filled with possibilities.
Setting Up the Project Structure
Setting up the project structure is a pivotal step when embarking on a Node.js project. A well-organized structure not only enhances readability but also streamlines collaboration among team members. It’s like laying the groundwork for a sturdy building – without it, you might find yourself grappling with chaotic files and unwieldy code. A clear structure also speeds up development, as you’ll know where to find crucial resources without combing through endless directories.
Pro tip: Think of setting up the structure as an investment in future productivity. When the structure is solid from the beginning, it reduces headaches later on.
Creating the Project Directory
Creating the project directory is among the first actions you’ll take. It acts as the foundation where everything else will reside. The directory should have a meaningful name, correlating closely with the project’s objective. For instance, if you're developing a weather app, naming your directory makes it clear right off the bat what to expect within.
You can easily create this directory via the terminal using a command like:
Once the directory is ready, navigate into it using:
By doing this, you create a dedicated space for organizing all related files, libraries, and resources, preventing any clutter that could hinder project growth.
Defining a Package.json File
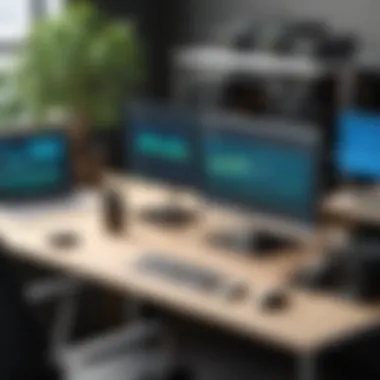
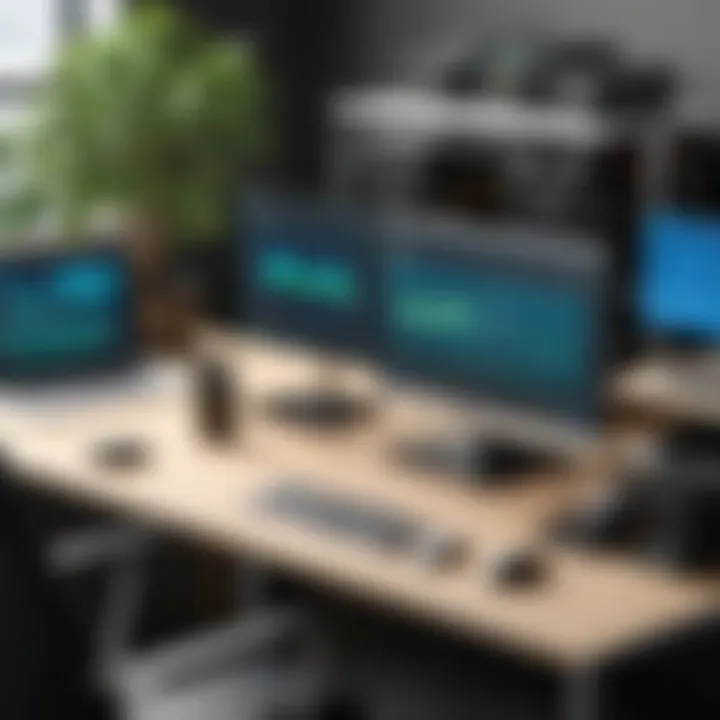
Using npm init
When you initiate your Node.js project, the command is your first stop. This helpful command generates a file, which acts as the project's backbone. Think of it as your project’s blueprint, outlining dependencies, scripts, and metadata required for your application.
After executing:
you'll be guided through various prompts to define aspects like the project name, version, and description. The simplicity of it, combined with the ability to customize your inputs, is what makes a staple. It's both user-friendly and efficient, helping you create a clean, informative in no time.
A unique feature to note is its capacity for customization. You might not need to fill every field initially, but over time as your project evolves, you can update this file to meet your changing needs.
"The file is like a license plate for your app; it identifies and provides necessary info to get it running."
Understanding package.json fields
A thorough grasp of the fields is critical for anyone aspiring to be proficient in Node.js development. This file encompasses various key attributes such as , , , and . Let's break down some specific ones:
- name: Specifies your project’s name. It must be unique if you plan to publish it.
- version: Follows semantic versioning, which is crucial for tracking changes.
- dependencies: Lists the libraries your project needs to run, allowing for easy management of packages.
Understanding each of these fields allows for better control of your project’s setup and lifecycle. An added benefit is being able to automate tasks through scripts outlined in the , which can save time and help maintain consistency.
Folder Organization Best Practices
When organizing your folder structure, adopting best practices can make an enormous difference. A common setup includes directories like:
- src: Where your source code resides.
- tests: For your test files, keeping your testing strategy clear.
- public: This is where static assets live, like images or stylesheets.
The goal is to keep your directories intuitive and maintainable. Don’t hesitate to adjust this structure as your project grows; flexibility can be key to managing complexity. Furthermore, documentation for your folder arrangement can help onboard new developers more efficiently, making it clear where to find what they need.
In short, a thoughtful project structure is a crucial investment in building scalable and manageable applications using Node.js.
Installing Dependencies
When embarking on a Node.js project, understanding how to manage dependencies effectively is vital. Dependencies are essential elements that your application relies on to function optimally. By installing the right packages, you not only boost the performance of your application but also streamline development processes. This section will delve into what dependencies are, how you can install them using npm, and the importance of keeping them updated.
What are Dependencies?
Dependencies in the context of software development refer to libraries or packages that your application needs to perform certain functions. They can be thought of as external tools; without them, the application might limp along or, worse yet, not function at all. For instance, if you’re building a web application that handles HTTP requests, you might rely on the Express framework as a dependency.
In practical terms, this means that whenever you encounter functionality that isn’t built into your standard Node.js runtime, you’re likely dealing with a dependency. And that’s where package managers like npm come into play. npm manages installation, updates, and dependency resolution, saving you a great deal of manual effort.
Using npm to Install Packages
npm, short for Node Package Manager, is your go-to tool in the Node.js ecosystem for managing libraries. It simplifies adding new packages and ensures that everything is in line with your project needs.
Installing specific packages
When it comes to installing specific packages, that’s where npm shines. By running a simple command, you can pull in any number of packages from the npm repository. For example, to install the popular Express library, you’d run:
What makes this process compelling is the ability to include essential functionalities without reinventing the wheel. By leveraging existing, trusted packages, you save time and reduce the likelihood of introducing bugs. Moreover, packages often come with community support, so if you hit a snag, there’s a high chance someone else has faced a similar issue.
However, one should be cautious. Carefully consider which packages you choose to include, as adding too many can bloat your application, slowing down performance.
Understanding versions and updates
Software is inherently mutable, and npm’s version control helps you manage this change. Each package in the npm registry comes with versions, which ensures that your application runs smoothly without unexpected breaking changes.
You can specify which version of a package to install by appending it to the package name. For instance:
Keeping your dependencies updated is not just about staying current; it’s also about security. Outdated packages can harbor vulnerabilities that might expose your application to risks. On occasion, updates might also include new features that can further enhance your project.
Tip: Regularly review your package.json file and consider using to see which dependencies have new versions available.
Staying on top of these updates requires a strategy; you could establish a routine for checking and updating, or leverage tools like Dependabot, which can automate much of this task.
In summary, mastering dependency management through npm is a cornerstone of successful Node.js development. By understanding both what dependencies are and how to handle them, you set a firm foundation for your application that can scale and adapt as needed.
Building a Basic Server
Building a basic server is a fundamental step when starting a Node.js project. It acts as the heart of your application, handling incoming traffic and serving responses. Understanding how to create a server is crucial as it lays the groundwork for more complex functionalities.
Once you have your server in place, you open the door to endless possibilities. Users can communicate with your application, send data, and receive responses through established routes. The process also familiarizes you with asynchronous programming, which is a key characteristic of Node.js. With the ability to handle many connections at once, a properly set up server becomes an efficient tool in a developer's arsenal.
Some benefits of building a basic server include:
- Real-time communication: Node.js excels at asynchronous operations, making it great for real-time applications like chats or notifications.
- Scalability: This approach allows for easy scaling as demand increases.
- Seamless integration: Setting a server paves the way for interactions with databases and APIs, transforming simple requests into powerful functionalities.
Every journey begins with a single step. In this case, creating your first server file is that step.
Creating Your First Server File
To kick things off, you need to create your first server file. This file will act as the entry point to your application. A basic server setup is quite straightforward. Start by creating a file named in your project directory. Then, include the following code:
This code snippet creates an HTTP server that listens on port 3000. It defines a function that serves a simple 'Hello, World!' message to any incoming request.
Handling HTTP Requests
Now that you have a basic server running, the next step is to handle HTTP requests. Proper management of requests is vital to ensure that your server operates correctly and efficiently. This section will concentrate on two main components: setting up routes and sending responses, which are foundational aspects of any web server.
Setting Up Routes
Setting up routes is about determining what to do when your server receives requests on different endpoints. Routes point to specific functions in your code that handle requests coming in for those endpoints. This structure is essential as it segregates handling logic, keeping the application organized.
A key characteristic of using routes is modularity. It allows you to expand and maintain your application easily. For instance, as your project scales, you can add new routes without overwhelming your original code structure. Each route effectively acts like a fork in the road, leading to different functionalities based on user input.
The advantages of setting up routes include:
- Clarity: More readable and manageable code by separating functionalities based on URL paths.
- Expandability: Facilitates the addition of future features or endpoints without major overhauls to the existing code.
- Testing: Isolated routes make it easier to test each part of your application independently.
Nevertheless, the downside to this modular approach might include initial complexity, especially if you're not accustomed to managing numerous files and routes.
Sending Responses
After defining your routes, the next critical step is sending responses back to the client. Every time a request hits a certain route, it is imperative to respond appropriately. This section ensures that the client knows the result of their request—whether it was successful, if there was an error, or if they simply need some information.
The process of sending responses can significantly enhance user experience. A distinguishing feature of effective response management is flexibility. Depending on the desired interaction, responses can range from simple messages to complex data structures in JSON format.
Here’s why sending responses is crucial:
- User Feedback: Ensures clients receive important updates or data after actions.
- Data Communication: It can send structured data, which is essential for applications working with databases.
- Error Handling: Proper responses help in diagnosing issues effectively.
On the flip side, if responses are not well structured, it could lead to miscommunications, leaving users confused about what happened with their requests. Always aim for clarity and accuracy in your messages.
In summary, building a basic server is more than just getting a program to run. It's about establishing a solid foundation for your upcoming developments. By creating your first server file, handling HTTP requests, setting up routes, and sending responses, you are not just following steps but preparing your application for real-world use.
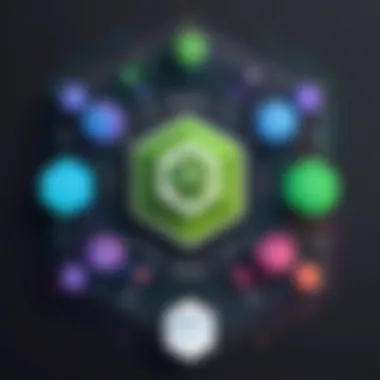
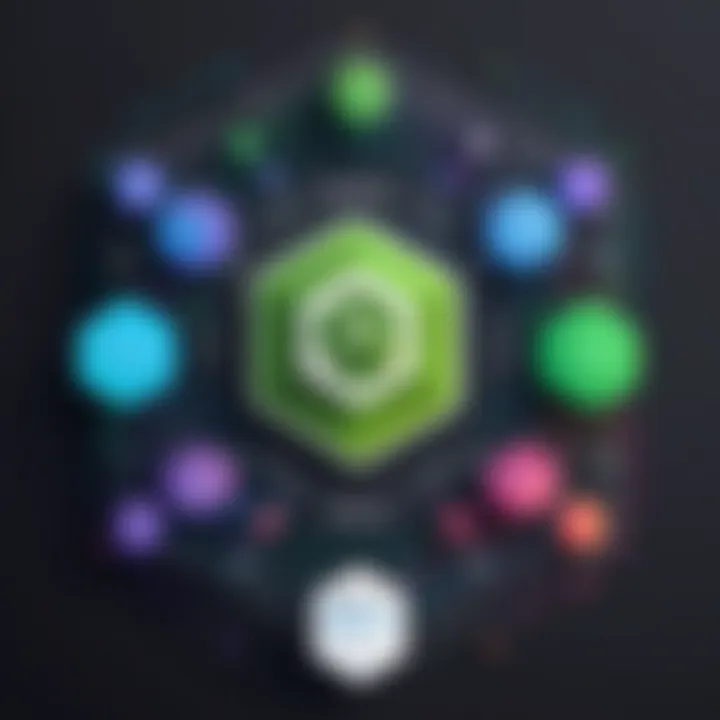
Environment Configuration
Environment configuration is a cornerstone of building a robust Node.js application. Properly managing the various environments—like development, testing, and production—can make all the difference in how smoothly an application runs. Misconfigurations can lead to a domino effect of issues that could hinder performance or even cause potential failures in production.
More than just a setting, environment configuration helps in tailoring your application to the unique needs of each stage of the deployment lifecycle. This section delves into how to manage different environments and use environment variables effectively for your projects, enhancing flexibility and security.
Managing Different Environments
When working on a Node.js project, it’s vital to differentiate between various environments. Each environment typically serves a different purpose and has its own set of configurations. For example, the development environment is designed for building and testing code, often featuring debugging tools and logging options. Conversely, a production environment is tailored for performance with optimizations, strict error handling, and security features in place.
In order to streamline your workflow, you should create profiles that can be easily switched. You can achieve this by using environment variables, scripts, or configuration files. It allows you to keep sensitive information localized and avoid using hard-coded values directly in your code. The best practice is to maintain separate files for each environment that your application will run in, be it staging, production, or testing.
Using Environment Variables
Environment variables are a powerful tool for configuration. They allow you to handle configuration settings outside of your codebase, which is crucial for keeping sensitive information—like API keys or database passwords—out of version control.
Setting up .env files
Creating a file is one of the simplest ways to manage environment variables. This file is structured as key-value pairs that your application can read to configure its environment settings. Here’s how a typical file looks:
A key benefit of utilizing a file is portability. Whether you are on your local machine or deploying to a server, the setup remains consistent. Additionally, many libraries, like dotenv, can automatically parse this file and load the variables into . While setting up a file is straightforward, you should not forget to add it to your file to prevent accidental uploads to repositories.
Accessing variables in code
Once you've set your environment variables up, accessing them in your Node.js application is as easy as calling . This is where the real magic happens, as it allows your application to adapt on the fly based on the environment it is operating in.
Here’s a simple example:
The unique feature here is that your application becomes much more adaptable. It reads the variable values at runtime instead of relying on the hardcoded ones. Consequently, this minimizes the risk of exposing sensitive data or making unintentional changes between environments.
However, one should also be cautious as improper management of environment variables can lead to confusion and potential security issues. A solid understanding of what each variable does and where it is used is key to maintaining a well-functioning application.
In summary, effective environment configuration not only protects sensitive information but also lays a stable foundation for deploying your Node.js applications in various contexts, thereby setting your project on a path for success.
Debugging Node.js Applications
Debugging is a crucial step in the software development process, especially when working with Node.js applications. The asynchronous nature of Node.js can lead to situations that are tricky to unravel. When things go awry, effective debugging can save both time and frustration. Understanding how to properly debug allows developers to pinpoint issues, improve code quality, and enhance performance. Given that Node.js applications often run in a server environment, ensuring they are bug-free is essential for providing a reliable user experience.
Common Debugging Techniques
Debugging techniques vary widely, but there are some common methods that can be applied beneficially in Node.js projects:
- Console Logging: One of the simplest yet effective methods is to use to output variable states or flow execution. This can help understand what's happening inside a function or at a certain point in your code.
- Error Handling: Always manage errors in your asynchronous functions. Use blocks or promise rejection handling to identify where things might be going south.
- Stack Traces: Take advantage of error stack traces. When an error occurs, inspecting the stack trace gives insights into the call history and where the problem originated.
- Node Inspector: This builds on the previous techniques by enabling a more sophisticated debugging experience, integrating with the Chrome Developer Tools, allowing you to set breakpoints right within your code.
"Debugging is like being the detective in a crime movie where you are also the murderer." – Unknown
Using Node.js Debugger
Node.js comes with its built-in debugger, which is powerful and might be underutilized by many developers. Here's how you can leverage it effectively:
- Starting the Debugger: Launch your application with the flag from the command line. For instance:This command tells Node.js to start in debugging mode, making it possible for you to connect via Chrome Developer Tools.
- Adding Breakpoints: You can insert statements directly into your code. When the execution reaches this line, it will pause, allowing you to inspect the current context.
- Inspecting Variables: Once the debugger hits a breakpoint, you can view and modify variables in real-time. This can be done directly from the console in Chrome Developer Tools.
- Step Through the Code: Use the stepping options to run lines of code one at a time, allowing for close examination of how the application behaves.
By employing these techniques, the debugging process in Node.js can be streamlined and made much more efficient, helping to quickly identify and fix bugs that might otherwise complicate the development lifecycle.
Testing Your Node.js Project
Testing isn’t just a box to check off before you hit the deploy button; it’s the backbone of a reliable Node.js application. Whether you're fresh to programming or a seasoned coder, you understand that bugs can creep up like unwanted weeds in the garden. Effective testing can identify these weeds early, saving you from surprises down the road. The importance of testing in Node.js lies not only in ensuring your code runs as expected but also in building confidence for further development.
Overview of Testing Frameworks
Diving into the world of testing, frameworks abound. You’ve got your widely-used ones like Mocha and Jasmine, along with the more modern Jest, not to mention others like Ava and Qunit. Each framework comes with its own philosophy and ways of structuring tests. Consider what you need when choosing one:
- Mocha: This flexible framework is known for its simple setup and broad range of assertions from Chai. Perfect for those wanting customization.
- Jest: Created by Facebook, Jest is a complete package for unit testing and integration testing, offering fast execution and a slick API.
- Jasmine: This behavior-driven testing framework is straightforward, making it easy to write, with an organized syntax. Ideal for newcomers.
- Ava: It's known for its minimalistic approach and runs tests concurrently, which is great for performance.
Ultimately, the choice depends on your project requirements and personal preferences. Each can elevate your testing game and add a robust layer to your development process.
Writing Unit Tests
Now, let's roll up our sleeves and put theory into practice with unit tests. Unit testing focuses on individual components, ensuring they behave the way they should in isolation. This is like checking your car parts before taking a road trip: you want to be sure you'll get to your destination without a hitch.
Consider the following steps to write unit tests in your Node.js application:
- Set Up Your Testing Environment: Install your chosen framework. For Jest, this can be done simply by running:
- Create a Test Folder: It’s a good practice to keep your tests organized. A common structure is to have a folder named at your project root.
- Write Your First Test: Start small. Here’s an example test using Jest on a simple function:
- Run Your Tests: Using the command line, run your tests with:
- Refactor and Repeat: You may not get it right from the get-go, and that’s perfectly fine. Refactor your code and tests as necessary, ensuring all are passing as you enhance functionality.
Remember: Regularly running tests helps maintain code quality and can alert you to issues early in the development lifecycle.
Automated testing is an invaluable asset for any Node.js developer. It not only reduces manual testing burdens but also helps create a safer environment for users and developers alike by ensuring consistent code functionality. In summary, whether you're crafting a simple app or building the next big web service, ensure that a solid testing strategy sits at the core of your development approach.
Performance Optimization
Performance optimization plays a pivotal role in the development of any Node.js application. As projects expand in complexity and scale, the efficiency of an application can significantly influence its success. An optimized application tends to work faster, utilize resources more effectively, and provide a smoother user experience. This results in not only higher user satisfaction but also lower operational costs over time. Therefore, understanding how to spot and resolve performance issues is not just a luxury—it's a necessity for every developer.
Identifying Bottlenecks
To kick things off, identifying bottlenecks is a critical first step in performance optimization. Much like a clogged drain that slows the flow of water, bottlenecks in a Node.js application can arise from various areas such as database queries, network issues, or even inefficient code execution.
- Common Bottlenecks:
- Inefficient Database Queries: Taking longer than expected to process requests.
- Blocking Operations: Code that halts the entire thread can lead to poor performance.
- Excessive Middleware: Using too many middleware functions can add unnecessary processing time.
To spot these performance drains, developers can use profiling tools like Node.js built-in profiler or external options such as Clinic.js. These tools provide insights on where the application spends most of its time, allowing developers to target specific areas for improvement.
"Identifying bottlenecks is like finding a needle in a haystack, but it's crucial for smooth sailing in your project!"
Techniques for Improvement
Once you’ve pinpointed the bottlenecks, it’s time to roll up your sleeves and get your hands dirty with techniques that can lead to improvement. Here are some strategies to consider:
- Asynchronous Programming:
Leveraging Node.js's non-blocking architecture allows multiple operations to run concurrently. Use or Promises to handle tasks without holding up the main thread. - Caching Mechanisms:
Integrating caching can significantly speed up response times. Libraries like or can store frequently requested data, reducing the load on your database and speeding up access to critical information. - Load Balancing:
Distributing network or application traffic across multiple servers ensures that no single server bears the entire load, which can prevent performance degradation during peak usage times. - Optimizing Code:
Assessing your code for logic that can be simplified can yield quick wins. For instance, reducing nested loops or avoiding unnecessary computations will make your algorithms run faster. - Choosing the Right Packages:
Not all packages are made equal. Some may introduce latency. Thoroughly vet dependencies and stay updated with more performant alternatives when available.
Deployment Strategies
Deploying your Node.js application isn't just an afterthought; it's a crucial step that often dictates the application's overall performance and scalability. Good deployment strategies can help ensure your app runs smoothly in production, withstands traffic spikes, and remains secure from vulnerabilities. Understanding how to deploy efficiently sets a robust foundation as your project matures.
Choosing a Hosting Service
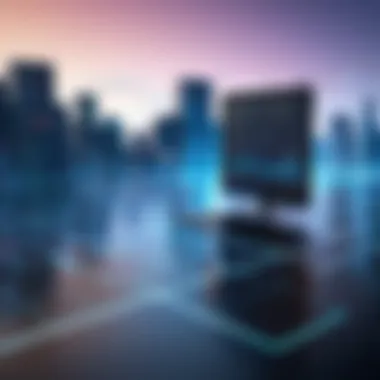
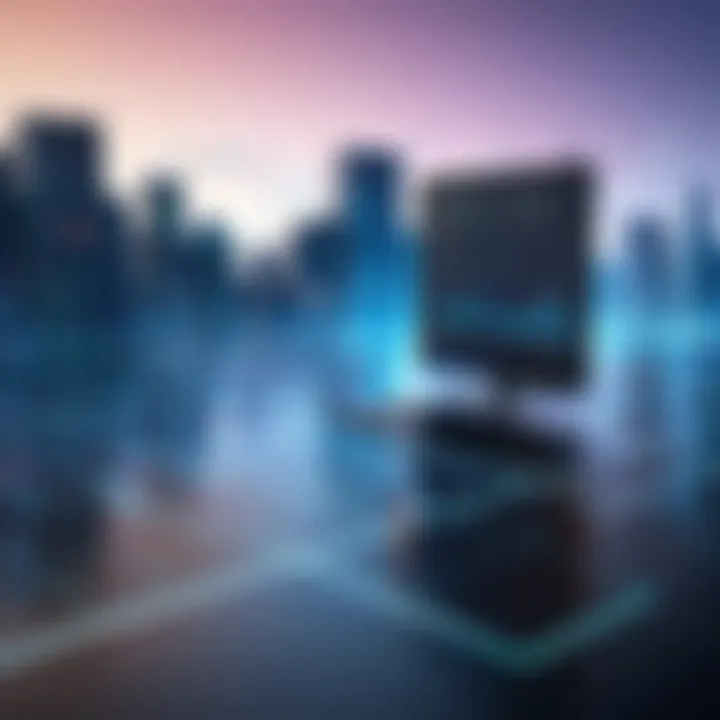
The first logical step post-development is to select a hosting service. A myriad of options exists, each with benefits and drawbacks, depending on what your app needs. When evaluating a hosting service, consider the following factors:
- Scalability: As your application grows, your hosting should be able to scale alongside. Look for services offering seamless scalability options, such as Automatic Scaling, so you aren’t caught off guard during peak usage.
- Cost: Weigh the pricing structures. Some providers charge based on usage, while others have fixed rates. Calculate projected expenses based on your needs.
- Support: Efficient customer support can make a world of difference when faced with technical issues. Choose a provider known for timely and helpful assistance.
- Performance: Consider the server specs, geographical data centers, and uptime guarantees. Latency can adversely affect user experience.
Some popular options for hosting Node.js applications include:
- Heroku: Known for its ease of use, ideal for beginners or small projects.
- DigitalOcean: Offers more control and flexibility for experienced developers.
- AWS (Amazon Web Services): The heavyweight in cloud services, great for larger, scalable applications, but it does come with higher complexity.
Selecting the right hosting service isn't a one-size-fits-all approach. Take time to analyze how each aligns with your project's goals and budget.
Setting Up Your Application for Production
Getting your application ready for production goes beyond just hosting it online. A successful deployment also involves configuring various elements to ensure reliability, security, and performance. Here are some considerations:
- Use Environment Variables: Keep sensitive information like API keys and database passwords out of your codebase. Implement environment variables for better security.
- Optimize for Performance: This is where you need to ensure that your app is fast and efficient in its operations. Set up caching strategies and consider using tools like Load Balancers to distribute traffic effectively.
- Error Handling: Implement comprehensive logging systems that help you track issues in real-time. Services such as Sentry or Loggly can be integrated to help with monitoring.
- Security Best Practices: Protect your app from common vulnerabilities like SQL injection and XSS by implementing measures such as proper input validations and regular dependency checks.
"An ounce of prevention is worth a pound of cure."
Lastly, it’s crucial to automate the deployment process using CI/CD tools like Jenkins or GitHub Actions. Automation minimizes human error and streamlines future deployments, making updates smoother and more efficient.
By taking these considerations into account, you position your application for resounding success in a live environment.
Continuous Integration and Continuous Deployment (/)
In the realm of software development, Continuous Integration and Continuous Deployment (CI/CD) serve as pivotal methodologies that not only boost efficiency but also enhance overall project quality. The adoption of CI/CD practices allows teams to automate the integration of code changes from multiple contributors into a shared repository. This process detects problems early, empowering developers to address issues before they snowball into larger complications. Furthermore, deploying updates frequently and reliably builds user trust and satisfaction, which is a crucial aspect of maintaining successful applications.
The implementation of CI/CD practices can yield numerous benefits, including improved collaboration among team members, faster time-to-market for new features, and a significant reduction in the "it works on my machine" dilemma. By automating repetitive tasks, developers can focus more on writing code and refining features rather than getting bogged down by manual processes.
"CI/CD is not just about tools or processes; it's about fostering a culture of collaboration and iteration within teams."
Understanding / Foundations
To grasp CI/CD fully, it's important to outline its core foundations. Continuous Integration refers to the practice in software development where team members integrate their work, ideally multiple times a day. Each integration triggers an automated build and testing sequence, ensuring the newly integrated code adheres to the predefined project standards. This process is instrumental in spotting integration bugs swiftly, thus minimizing the issues that could potentially arise later on.
On the other side of the coin, Continuous Deployment builds on CI by ensuring that every change passes through the automated tests and is automatically deployed to the production environment without manual intervention. This workflow accelerates the release of new functionalities, enabling products to adapt quickly in a fast-paced digital landscape. Continuous Deployment should not be confused with Continuous Delivery, where the latter ensures that every change is ready to be deployed, but may require a manual trigger to actually release the build into the production.
Implementing / Pipelines
Setting up a CI/CD pipeline could sound daunting, but once you understand the steps and tools involved, it becomes a straightforward process. Here’s a breakdown to help you navigate through the implementation:
- Choose a CI/CD Tool: Select a tool that aligns with your project needs. Popular options include Jenkins, GitHub Actions, GitLab CI, and CircleCI.
- Set Up Version Control: Ensuring your code is version-controlled (commonly using Git) is essential. Your CI/CD tool will build from a repository, so having your source code neatly organized and versioned is non-negotiable.
- Define Pipeline Stages: Outline the stages of your pipeline. Typical stages might include:
- Implement Automated Tests: Craft a suite of tests that automatically verify the application’s functionality at various levels, such as unit tests, integration tests, and end-to-end tests.
- Configure Deployment Settings: Design your deployment settings to include rollbacks, so if a deployment fails, you can return to the last stable version smoothly.
- Monitor and Iterate: Once your pipeline is in place, continuously monitor its performance and gather feedback. Regularly revisit the pipeline to make adjustments and optimize the process.
- Build: Compiles the code and runs unit tests.
- Test: Executes automated tests to validate functionality.
- Deploy: Automatically deploys to a testing or production environment.
- Monitor: Observes application performance post-deployment.
By embedding CI/CD into your Node.js project’s workflow, you're not just enhancing the technical aspect of development; you're also promoting a culture of continuous improvement and adaptability, which is vital in today’s rapidly evolving tech environment.
Securing Your Node.js Application
When venturing into the realm of Node.js, prioritizing security is akin to locking your front door before leaving the house. It's essential not just for keeping your data safe, but also for maintaining trust with your users. In this section, we'll break down the significance of securing your Node.js application, highlighting the various security threats and the best practices to mitigate them.
Common Security Threats
As the saying goes, "forewarned is forearmed." Understanding the types of security threats that lurk around every corner can significantly enhance your ability to defend against them. Below are several common threats faced by Node.js applications:
- Injection Attacks: This includes SQL injection and NoSQL injection attacks that occur when an attacker tries to manipulate queries to gain unauthorized access to data.
- Cross-Site Scripting (XSS): An attacker injects malicious scripts into content that's served to users, which can lead to data theft or misinformation.
- Denial of Service (DoS): This threat aims to make your application unavailable by overloading it with too many requests or exploiting vulnerabilities.
- Broken Authentication: Poorly implemented authentication mechanisms can lead to unauthorized access, where attackers can impersonate legitimate users.
Each threat not only exposes vulnerabilities but also can severely damage your application's reputation and user trust. So, vigilance is key.
Implementing Security Best Practices
Securing your Node.js application is not a one-and-done job; it requires ongoing diligence and adherence to best practices. Below are essential security strategies to embed into your development workflow:
- Use HTTPS: Always serve your application over HTTPS. This encrypts data in transit and makes it significantly harder for attackers to intercept sensitive information.
- Sanitize Inputs: Always validate and sanitize user inputs to protect against injection attacks. Libraries like can help you limit what kind of data is accepted.
- Set Security Headers: Implement security headers like Content Security Policy (CSP), X-Content-Type-Options, and X-Frame-Options to mitigate XSS risks.
- Implement Rate Limiting: To defend against DoS attacks, use rate limiting techniques in your application by employing middleware like .
- Update Dependencies: Regularly review and update your dependencies. Using tools like can help identify vulnerabilities in your packages and alert you whenever a security patch is available.
"Security is not a product, but a process."
These strategies form the backbone of an effective security protocol for your Node.js application. It's through vigilant attention to detail and a proactive approach that you can safeguard both your application and your users' data.
Maintenance and Updates
Keeping your Node.js application in top-notch shape is not just a one-and-done task; it’s an ongoing aspect that significantly contributes to the longevity and efficiency of your project. The world of technology evolves rapidly, and if you don’t keep your application updated, you risk facing security vulnerabilities and inefficient performance. In this section, we will explore why regular maintenance is indispensable and how it plays a crucial role in the sustainability of your Node.js project.
Regular maintenance allows developers to identify and rectify issues proactively. It encompasses going over log files, monitoring performance metrics, and adjusting configurations as necessary to ensure your application runs smoothly. Moreover, maintaining a schedule fosters a habit of diligence among developers, preventing bugs from piling up and causing major headaches down the line.
"An ounce of prevention is worth a pound of cure." - Benjamin Franklin
In terms of updates, while it might seem tedious to ensure that all dependencies are current, it's a fundamental task that can save you from potential chaos in the future. Old versions of packages can become a breeding ground for security exploits, so keeping up with updates helps to safeguard your application.
Establishing a Regular Maintenance Schedule
When we speak about establishing a maintenance schedule, picture it like routine health check-ups for your application. Just as you wouldn’t want to avoid a doctor’s appointment for years, your Node.js project deserves consistent attention.
Create a timeline that fits your project needs. Weekly or bi-weekly checks make sense for smaller projects, while larger applications may benefit from even more frequent reviews. Here are a few essential tasks to include in your maintenance schedule:
- Review Error Logs: Regularly examine logs to catch any errors before they escalate.
- Monitor Performance Metrics: Use tools like New Relic or PM2 to keep tabs on your application’s responsiveness and resource utilization.
- Updating Documentation: As your application evolves, so should your documentation. Ensure everything from setup instructions to troubleshooting guides are updated.
The objective of a regular maintenance schedule is to develop a rhythm that allows issues to be detected promptly, resulting in quicker remediation.
Keeping Dependencies Up to Date
Dependency management can often feel like a game of three-dimensional chess. It’s not just about knowing what pieces you have on the board; it’s also vital to understand how updating one affects the others. Keeping your dependencies updated reinforces the security of your application while enabling access to the latest features and bug fixes.
Utilize tools like npm-check-updates or npm outdated to easily spot dependencies that need updating. When updating, consider the following:
- Semantic Versioning: Familiarize yourself with the versioning system that many packages employ. Understanding major, minor, and patch updates can help you decide how to proceed.
- Backup Your Work: Before making wholesale updates, back up your original code to prevent mishaps.
- Test Thoroughly: Run comprehensive tests after updating to catch any breaking changes early.
A regular update routine is as important as it sounds; it could spell the difference between a smooth sailing application and one prone to disruptions. While some may view updates as mere inconveniences, consider them as opportunities for refinement and growth.
Keeping a close eye on your application’s health not only streamlines development but also enhances user trust, ultimately benefiting your project's success.
Best Practices for Node.js Development
When it comes to developing a Node.js application, adhering to best practices is not just a fancy suggestion. It's a necessity for ensuring longevity, scalability, and maintainability of the project. Following best practices helps prevent headaches in the future and allows you to craft a robust application right from the start. Let’s explore key elements that define what these best practices are, their benefits, and important considerations to keep in mind.
Code Quality and Maintenance
Code quality should never take a backseat in Node.js development. Writing clean and maintainable code is much like laying the foundation of a building. If the base isn’t solid, the structure becomes weak and prone to collapse. Here’s why code quality is critical:
- Readability: Code should be easy for others (or you, months down the line) to read. Using descriptive variable names and consistent indentation style makes your code more understandable.
- Modularity: Break your code into smaller, reusable components. This not only improves readability but makes debugging easier and allows for better collaboration among developers. Each component can be developed independently.
- Version Control: Use tools like Git to maintain your code. Version control is essential for tracking changes, collaborating with others, and reverting to stable versions when necessary.
To keep your code base healthy, consider tools like ESLint or Prettier. They help in identifying problematic patterns in your JavaScript and enforcing coding conventions. Just a small extra step can save you tons of time sorting out bugs later.
“An ounce of prevention is worth a pound of cure.” – Benjamin Franklin
Documentation Strategies
In the world of software development, documentation is often overlooked, but it’s the glue that holds everything together. Good documentation acts as a roadmap for your project and can guide developers at all levels. Here’s why investing time in documentation is worthwhile:
- Onboarding New Developers: When new team members come on board, good documentation helps them understand how the code works and the project's structure without the need for extensive hand-holding.
- Knowledge Sharing: Documentation serves as a repository of knowledge. It allows your team to share insights and resources, preventing information silos from forming.
- Future Maintenance: When developers must revisit the code after months or years, solid documentation eases the burden. It clarifies the reasoning behind complex decisions and explains how to navigate the project.
To create effective documentation, consider these strategies:
- Keep it Updated: Documentation should evolve alongside your codebase. Regularly revisiting your documentation to align it with the latest updates prevents it from becoming obsolete.
- Incorporate Examples: Real-life code snippets help in illustrating complex concepts, making it easier for others to grasp them.