A Complete Guide to Starting Your Python Script
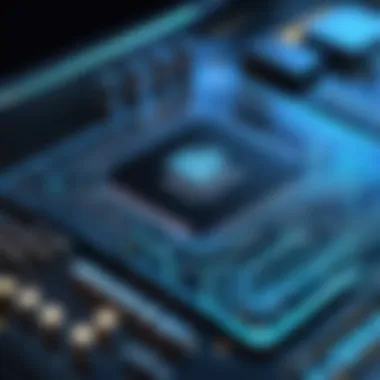
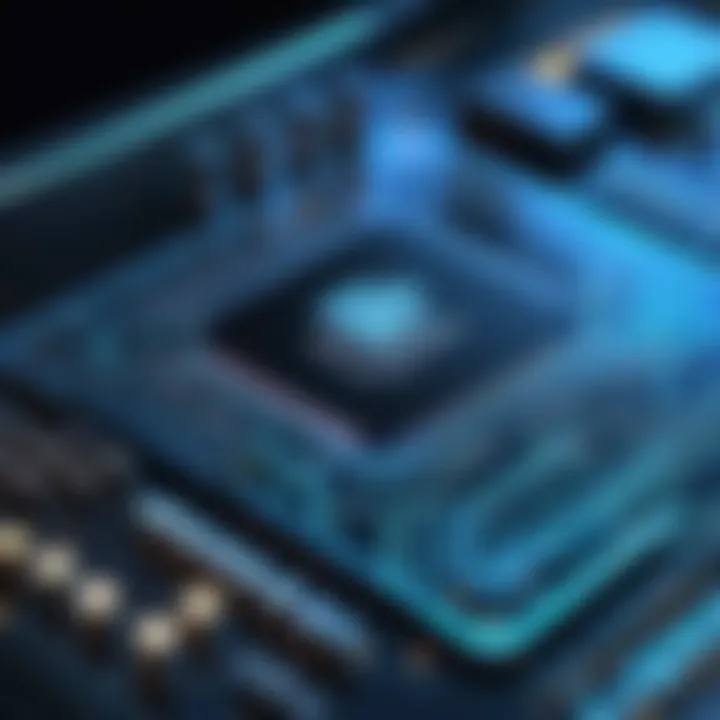
Overview of Topic
Starting a Python script might seem straightforward, but it opens the door to a world brimming with possibilities. This guide dives into the nuts and bolts of kicking off a Python project, whether you're dipping your toes into programming or looking to refine your craft.
Preamble to the main concept covered
Writing a Python script is essentially about translating logical steps into code. Each script is an extension of your thoughts, reflecting how you want to interact with data or automate tasks. At core, it involves understanding Python's syntax and its relationship with the underlying operating system.
Scope and significance in the tech industry
In todayās fast-paced tech landscape, Python is more than just a programming language; itās a key player in data science, machine learning, web development, and automation. Organizations lean on Python for its simplicity and power, making it a staple in the toolkit of any IT professional. Starting a script effectively lays a strong foundation for problem-solving and creative software development.
Brief history and evolution
Python came into the scene in the late 1980s, crafted by Guido van Rossum. Since its inception, it has evolved significantly, with rich libraries and frameworks sprouting around it. Initially perceived as a hobby project, Python has now turned into a go-to language for both newcomers and seasoned pros alike.
Fundamentals Explained
Grasping the fundamentals is crucial for successfully beginning any Python script. These principles form the bedrock on which all Python coding stands.
Core principles and theories related to the topic
Every programming language has guiding principles. Python emphasizes readability and simplicity. This philosophy promotes writing code that others can easily understand, which is especially important in collaborative environments.
Key terminology and definitions
- Script: A collection of Python statements that a Python interpreter executes.
- Compiler vs. Interpreter: Compilers convert code into machine language before execution, while interpreters execute code line by line.
- Environment: The configuration under which Python scripts run, such as IDEs or command-line interfaces.
Basic concepts and foundational knowledge
Before starting a script, understand the building blocks:
- Variables: Named storage for data.
- Data Types: Such as integers, floats, strings, and lists.
- Control Structures: These dictate the flow of the script, including loops and conditionals.
Practical Applications and Examples
To put theory into practice, looking at real-world applications can be enlightening.
Real-world case studies and applications
Consider automating the daily weather report for your business. A Python script utilizing an API can fetch this data and format it into an email or display it on a dashboard. This cross-application of skills boosts efficiency across various sectors.
Demonstrations and hands-on projects
A simple scripting exercise involves calculating the sum of user-inputted numbers. This project reinforces input handling and logic flow. Just imagine the satisfaction of seeing your script work seamlessly!
Code snippets and implementation guidelines
Hereās a basic example of a Python script handling user input:
This snippet illustrates user interaction and basic arithmetic, fundamentals for any budding programmer.
Advanced Topics and Latest Trends
Once the basics are in your back pocket, exploring advanced topics becomes vital.
Cutting-edge developments in the field
The rise of artificial intelligence and machine learning has turbocharged Pythonās relevance. Libraries such as TensorFlow and PyTorch are at the forefront of this revolution, offering progressive techniques for data analysis and algorithm development.
Advanced techniques and methodologies
Understanding concepts like object-oriented programming, functional programming, and decorators can dramatically enhance your scripts. These methodologies promote code reusability and efficiency, enabling complex projects to run smoothly.
Future prospects and upcoming trends
As Python continues to evolve, staying updated with trends like asynchronous programming and improved concurrency models will be beneficial. The landscape is ever-changing, and adapting to these shifts ensures that programmers remain at the cutting edge.
Tips and Resources for Further Learning
To truly master Python scripting, continuous learning is key.
Recommended books, courses, and online resources
- "Automate the Boring Stuff with Python" by Al Sweigart
- Courseraās Python for Everybody course
- The official Python documentation at python.org
Tools and software for practical usage
Leverage tools such as Visual Studio Code or PyCharm for coding. Both provide helpful features like code completion and debugging capabilities that simplify the coding process.
Learning Python opens up a world of opportunities, driving innovation and increasing productivity. Start scripting with confidence and watch your ideas transform into tangible solutions.
Understanding Python Scripts
Understanding Python scripts is a cornerstone for anyone interested in learning programming or delving deep into software development. It establishes a foundation that connects theory to practice. Essentially, a Python script functions as a set of instructions that the Python interpreter understands and executes. Learning to create these scripts not only empowers individuals to automate tasks but also cultivates problem-solving skills that are vital in tech.
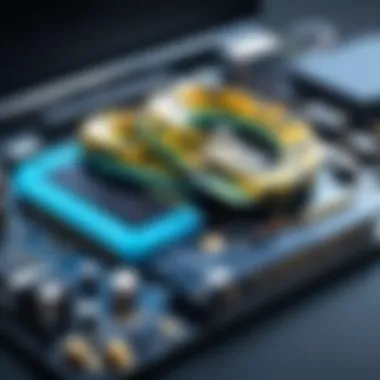
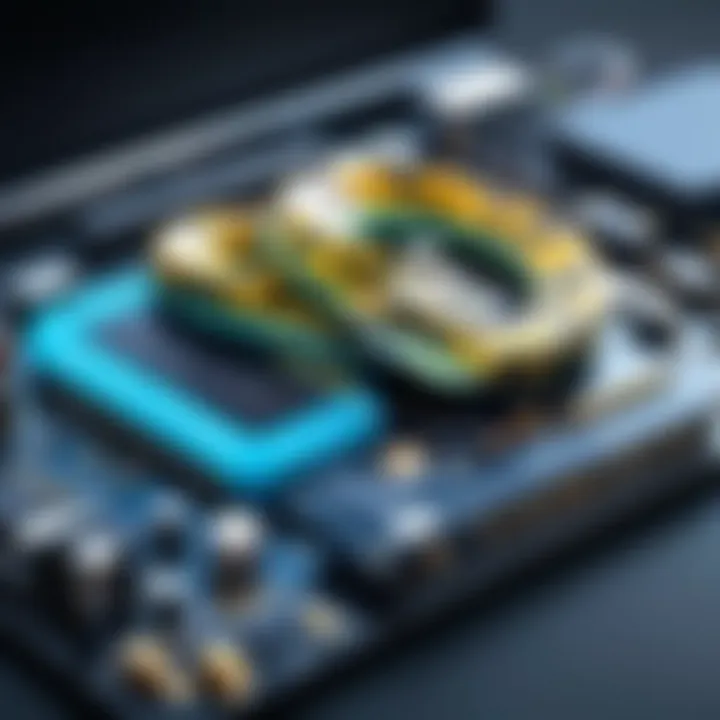
Definition of a Python Script
A Python script can be simply defined as a file containing a sequence of commands that can be executed by the Python interpreter. Typically, these scripts have a file extension, which signifies that the contents adhere to Python's syntax and semantics. The beauty of Python scripts lies in their versatility. They can range from basic fruit loopsājust a few lines of codeāto complex algorithms for data analysis and web applications.
In essence, defining a Python script involves more than just understanding the syntax; itās about grasping the logic behind it. You might say, a script is like a recipe in a cookbook. It lists instructions in a particular order to produce a final dish or outcome. Similarly, a Python script directs how data should be processed, manipulated, and outputted.
Significance of Scripts in Programming
Scripts play a pivotal role in modern programming. They serve numerous essential functions that enhance productivity, efficiency, and creativity in coding. Here are a few critical aspects that underscore the importance of scripts:
- Automation: Scripts allow you to automate repetitive tasks, saving valuable time and reducing the margin for human error. Whatās a better way to spend your day than letting a program do your work while you kick back?
- Prototyping: They offer an easy way to prototype ideas. Need to test an algorithm? Write a quick script, run it, and see if it works the way you intended.
- Integration: Scripts can integrate with existing software systems, thus enabling seamless data exchange and functionality enhancement. This is particularly valuable in environments with varied technologies at play.
- Community and Collaboration: The nature of scripting encourages sharing and collaboration among developers. The open-source community thrives on collaboration, fostering environments where scripts can evolve and improve collectively.
"A script doesn't just run a program; it tells the computer how to think and act, shaping the very fabric of automation."
Understanding Python scripts is the gateway to more advanced programming concepts and techniques. As you arm yourself with this knowledge, you will find yourself better equipped to tackle more complex coding challenges.
Setting Up the Environment
Setting up the environment is a crucial step for anyone looking to start scripting in Python. It lays the groundwork for writing and executing code efficiently. The right environment ensures that developers can easily manage packages, run scripts, and troubleshoot issues without unnecessary headaches. Other factors like system compatibility, resource requirements, and personal preferences can significantly influence how one approaches this setup.
Moreover, a well-configured environment can enhance learning by providing tools that help visualize or debug the code, making it easier for beginners to grasp core concepts. To that end, letās delve into two main aspects: choosing an Integrated Development Environment (IDE) and installing Python itself.
Choosing an Integrated Development Environment (IDE)
Selecting the right Integrated Development Environment is like picking the right tool for a craftsperson. IDEs simplify the coding experience, combining several essential features within a single interface. They provide text editing, syntax highlighting, debugging options, and more.
Here are some popular options to consider:
- PyCharm: A powerful IDE that offers complete code management and great debugging capabilities. The Community edition is free, catering particularly well to beginners.
- Visual Studio Code (VS Code): Lightweight and highly customizable, it's widely favored because of its wide-ranging extensions. It's excellent for learning as it supports multiple languages alongside Python.
- Jupyter Notebook: Particularly suitable for data science, it allows users to create and share documents containing live code, equations, and visualizations.
The choice of IDE can significantly affect your productivity and comfort while coding, thus wisely investing time in selecting one that aligns with your aims is recommended. In the long run, it can save you a lot of frustration.
Installation of Python
Installing Python is the first tangible step into the world of programming with this language. It involves a couple of key procedures:
Downloading Python
Downloading Python can vary slightly depending on your operating system, but it fundamentally consists of visiting the official Python website. The key characteristic of downloading Python here is getting the right version suited to your system. The latest version usually comes with significant improvements and bug fixes, making it the most recommended option for those who want to take advantage of new features.
Also, in general, Python provides two major versions: Python 2 and Python 3, and while Python 2 has been deprecated, many users still reference it for legacy systems. Hence, unless there is a specific reason to stick to an older version, opting for Python 3 is the wise choice for every new project. The ease of getting these installers online has certainly made it a popular option among beginners.
Paying attention to the choice of 32-bit or 64-bit versions is essential as well since installing the incorrect one can lead to compatibility issues down the line, potentially complicating future coding endeavors.
Installation Procedures
Once downloaded, installation procedures involve running the installer. This step should not be taken lightly, as comprehensive configuration options are presented during the installation process. Users should check the box that adds Python to their system path because this makes it much easier to run scripts from the command line.
Upon installation, the Python launcher will typically be available, streamlining the process of managing multiple Python versions on a single system. This flexibility is hugely beneficial as projects evolve and requirements shift. At the same time, being mindful of default settings during installation is prudent; itās essential to know whatās being installed on your machine to avoid bloatware.
Verifying Installation
Verifying the installation of Python is a simple yet critical step. After installation, open your command line interface and type , or use if you installed it with that tag. The system should respond with the installed version number. If this happens, congratulations! Your installation is successful and ready to use.
Not only does this check confirm that Python is operational, but it also instills a sense of confidence in users, particularly beginners. Knowing that everything works smoothly is a great motivator to start coding. Additionally, verifying ensures that configuration issues are caught early, sidestepping potential headaches later on.
Creating Your First Script
Creating your first Python script is an essential milestone for any aspiring programmer. It not only builds confidence but also sets the foundation for understanding the programming language's functionality and syntax. This section will dive into various components that form the backbone of a basic script, emphasizing key elements that every programmer must grasp in order to write meaningful code.
Basic Structure of a Python Script
Understanding the basic structure is crucial. A well-defined structure can make your code easy to read and follow, catering both to the author's future self and to other programmers who might look at your work later. A script typically consists of three primary elements: comments and documentation, importing libraries, and defining functions.
Comments and Documentation
Comments play a vital role in coding. They serve as notes for anyone who reads the code laterābe it you or someone else. A key characteristic of comments is that they won't affect the program's execution. This makes them a beneficial choice for conveying ideas or explanations without changing how the code runs.
- Unique Feature: In Python, comments begin with a . This means that anything written after the on the same line is disregarded by the interpreter.
- Advantages: Comments improve code readability and maintenance. They can clarify complex sections of code that might confuse a future reader.
- Disadvantages: Too much commenting can clutter code, making it hard to follow. Finding the right balance is essential.
Importing Libraries
Importing libraries is where Python's power truly shines. By leveraging existing libraries, you can save time and take advantage of pre-written functions. This is crucial as many developers don't want to reinvent the wheel for common tasks.
- Key Characteristic: Python offers a host of librariesāstandard ones like , as well as third-party options from an extensive ecosystem. This makes importing libraries a popular practice.
- Unique Feature: You can import whole libraries or just specific functions, depending on your needs.
- Advantages: This practice encourages code reuse, making scripts shorter and more efficient.
- Disadvantages: Over-relying on external libraries may lead to performance issues if the libraries are not optimizing their code properly.
Defining Functions
Defining functions is a core aspect of Python programming. Functions allow you to encapsulate code for reuse and simplify complex tasks into manageable blocks. A key characteristic of functions is that they can be called multiple times within the same script, which promotes modularity and reduces redundancy.
- Unique Feature: In Python, a function is defined using the keyword, followed by the function name and parentheses.
- Advantages: Functions provide clarity. By reducing repetitive code, they make it easier to troubleshoot specific segments while enhancing code organization.
- Disadvantages: Creating a multitude of small functions may lead to fragmentation. It's vital to find a balance between functionality and simplicity.
Writing Your Script
When it comes to writing your script, careful attention to elements like code organization, logical flow, and syntax best practices can tremendously impact your programming experience.
Code Organization
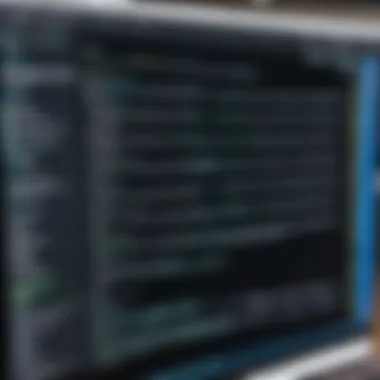
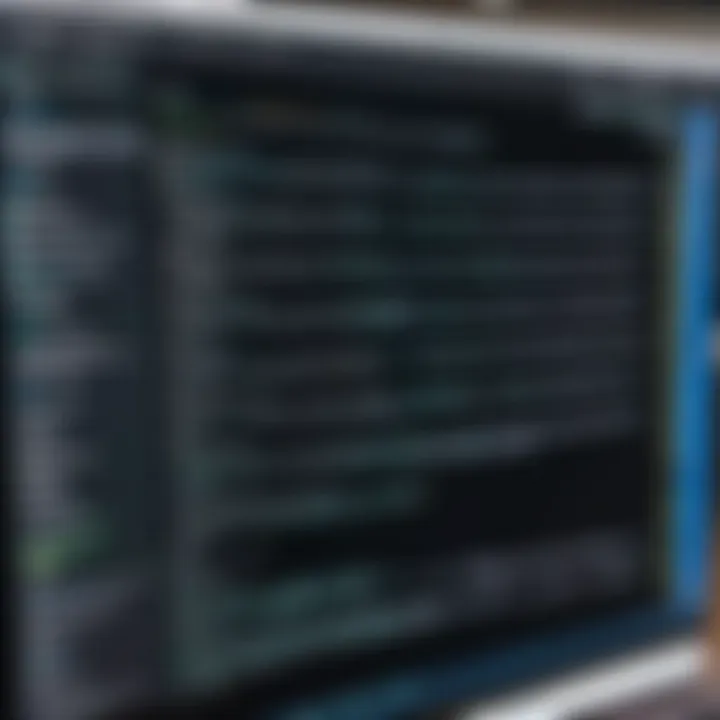
Organizing code is more than just a neat arrangement of text. It encompasses how you format your code, arrange functions, and maintain clear logical distinctions among scripts. Proper code organization prevents confusion and allows for more efficient debugging.
- Key Characteristic: Most scripts are segmented into logical parts corresponding to specific tasks they accomplish.
- Unique Feature: Create separate files for large projects to make it more manageable, promoting modularity and separation of concerns.
- Advantages: A well-organized codebase is straightforward to understand and maintain. Comments, organized sections, and consistent naming conventions support this process.
- Disadvantages: Neglecting organization can lead to complicated code that is frustrating to work with and debug.
Logical Flow
The logical flow is the sequencing of operations in your code. An intuitive flow means that anyone reading your code will easily follow the logic behind it. Itās essential to ensure that the code runs smoothly from one section to another without abrupt jumps or confusion.
- Key Characteristic: Using clear and appropriate controls, such as loops and conditionals, supports logical flow.
- Unique Feature: Tools like flowcharts can help visualize the logical sequence, especially in complex scripts.
- Advantages: A coherent logical flow makes the script easier to debug and understand, enhancing readability.
- Disadvantages: Disorganized flow can lead to bugs, often requiring extensive debugging efforts that can sap your time and energy.
Syntax Best Practices
Proper syntax is foundational. Adhering to syntax best practices reduces errorsāboth during writing and executionāensuring that the code runs as intended.
- Key Characteristic: Python is known for its readability, and following syntax rules enhances this characteristic.
- Unique Feature: Indentation in Python is not just for aesthetics; it's part of the syntax that determines how code blocks are structured.
- Advantages: Consistent syntax helps new programmers read and understand your code. It minimizes confusion.
- Disadvantages: Deviating from standard syntax can cause significant misunderstandings, leading to incorrect program logic or execution errors.
Remember, writing your first script is just the beginning. With time and practice, youāll find your rhythm and develop your own style of scripting.
Running Python Scripts
Running Python scripts is a crucial step in the programming process. Itās where your code transforms from mere lines of text into actionable tasks that can solve problems, automate tasks, or handle data. In this section, youāll learn the various methods to run your Python scripts, understand the advantages of each, and find tips to troubleshoot common pitfalls along the way.
Using the Command Line Interface
The command line interface (CLI) is a powerhouse when it comes to running Python scripts. It offers a basic but powerful way to interact with your operating system through text commands. Working through the CLI might seem old school to some, yet it provides a level of control that graphic-based interfaces often can't match.
Open Command Prompt
Opening the command prompt is the very first step in executing a Python script using the CLI. This action lays the groundwork for all subsequent commands. The command prompt, or terminal for the UNIX users out there, allows direct interaction with the file system and program execution. One of the key characteristics of the command prompt is its simplicity; it lacks the frills of graphical user interfaces. This fuss-free nature can actually contribute to an enhanced focus on coding and script execution.
To open the command prompt on Windows, just search for "cmd" in the start menu. On macOS, you can find it in the utilities section, while Linux folks can grab it from the applications menu.
Advantages of using the command prompt include:
- Speed: Once you become comfortable with commands, navigating and executing scripts becomes much faster than clicking through windows.
- Control: You can carry out powerful commands, piping data or chaining commands together.
- Scripting: You can create batch scripts, condensing multiple commands into one file, making repetitive tasks easier.
Disadvantages may also include a steeper learning curve for new programmers, as not everyone is familiar with command languages.
Execute Your Script
Executing your script at the command line is done using a simple command that takes the name of your script as an argument. For instance, typing (assuming your script is named ) will run that script. This method is both straightforward and efficient, offering the significant benefit of immediate feedback in a console window after executing the command.
The specificity of this execution method is one of its strong points. Youāre able to see errors and status messages directly in the terminal. This is an important characteristic for debugging your script as it runs.
Yet, itās important to be aware of the file path where your script is placed. If you're not in the right directory, the command won't work. Here are few advantages:
- Immediate Output: You can see your scriptās output right after it runs, making it easier to troubleshoot.
- Error Messages: Direct access to error messages can quickly guide you to what might be wrong in your code.
On the flip side, remember that if you have to run multiple scripts or gather insights from longer outputs, navigating through the command line might become cumbersome.
Understanding Output
Understanding the output generated by your scripts is equally important as writing the code itself. The output can help you gauge if your script is functioning correctly. When you run a Python script via the command line, the results of your script will typically print to the console unless youāve directed them otherwise.
Highlighting a key trait about output is that it helps you verify not only if your code is correct but also how well it performs and what kind of data it processes. A successful output might yield the expected result; however, itās worth noting that unexpected output is equally important, as it often indicates bugs or logical issues in the script.
Some benefits of understanding the output include:
- Debugging: The output can help you trace errors back to specific lines of code.
- Performance Metrics: By analyzing the output, you can measure how well your script runs, which can lead to optimizations.
Itās also crucial to remember that sometimes outputs can get quite verbose or complex, depending on what you're trying to achieve. Being prepared to sift through outputs efficiently will save you time in the long run.
Executing Scripts from within IDE
For those using integrated development environments, executing Python scripts can become even smoother. IDEs like PyCharm, Visual Studio Code, or even Jupyter Notebook provide built-in functionalities that allow you to run your scripts either on a click or a specified keyboard shortcut.
Using an IDE can greatly enhance productivity for many, as such environments typically combine editing and running your code in one space. However, it's also important to understand how to utilize the IDEās specific features to enhance your script-running experience effectively.
Error Handling in Python Scripts
Handling errors effectively is a crucial element in programming with Python. In fact, it can make the difference between a well-functioning script and a program that fails unexpectedly, leaving users in a lurch. Awareness of the potential pitfalls and crafting strategies to manage them elevates a coderās proficiency. Moreover, learning to handle errors is about embracing the unpredictability inherent in coding. This section aims to illuminate various aspects of error management in Python scripts, the common errors encountered, and the tools available to address them.
Common Errors and Their Solutions
In the journey of coding, itās practically inevitable to encounter errors. These can range from syntax mishaps to runtime issues. Understanding these common errors can make a world of difference.
- Syntax Errors: This is like having a typo on your exam paper - it stops everything in its tracks. You usually see these when the code violates Python's grammar rules. The solution? Read the error message closely and fix the syntax.
- Runtime Errors: These occur while the code is being executed. Imagine turning the key in your car and nothing happens ā same feeling. For instance, attempting to divide by zero leads to such an error. The solution involves checking data values before operations.
- Logical Errors: These are tricky because the code runs without complaints, but the output isnāt what you expect. Itās like following a recipe but ending up with a dish that tastes wrong. The fix here is thorough testing and adding print statements or using interactive debuggers to trace your logic.
Utilizing Exception Handling
When errors do occur, exception handling is like a safety net that can prevent your program from crashing. It provides the structure needed to respond gracefully to unexpected situations.
Try and Except Blocks
The try and except blocks are fundamental in Python's error handling. Hereās how it works: you place the code that might throw an error in the block. Subsequently, if an error is encountered, control passes to the block. This setup ensures your program continues running without an abrupt halt.
- A key characteristic of try and except blocks is simplicity. It's relatively easy to implement, making it a popular choice for error handling.
- One unique feature is that you can catch specific errors. This is beneficial because it allows precise control over different error types, avoiding overly broad catch-alls that can mask issues.
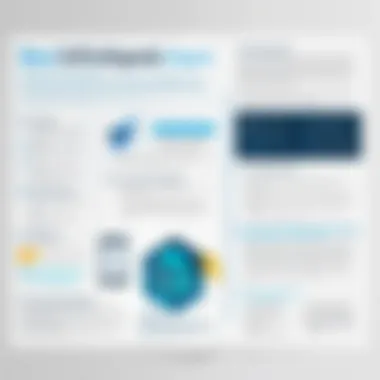
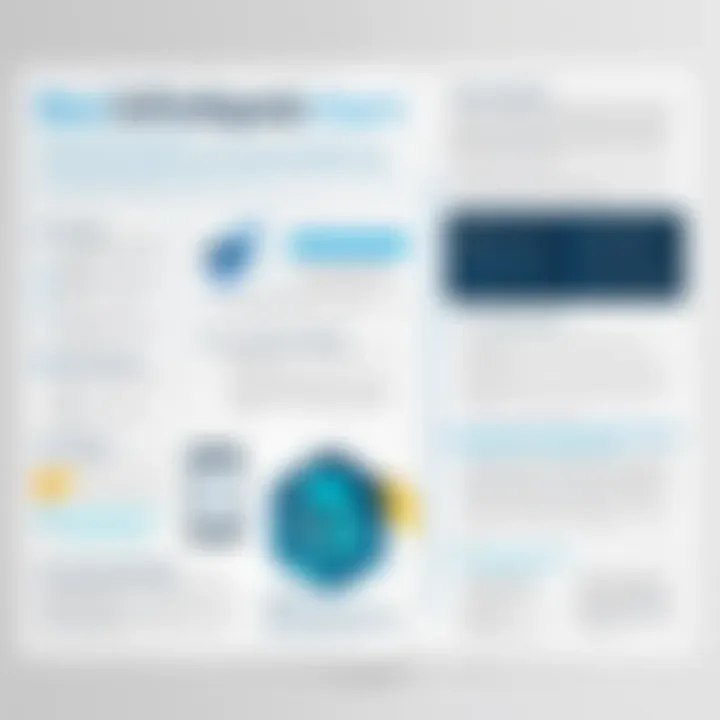
Yet, reliance solely on try and except can be a double-edged sword. It might lead to ignoring underlying problems if errors are not appropriately logged and handled.
Finally Statement
The finally statement is another tool in the exception handling toolbox. Regardless of whether an error was raised or caught, the code in the block will always execute.
- This characteristic of the finally statement is its reliability. It's beneficial for executing cleanup actions, such as closing files or releasing resources. This is especially useful in larger applications where resource management is essential.
- The unique aspect of the finally statement lies in its assurance that critical code runs after try and except blocks, regardless of the outcome. There's a sense of security in knowing that even if an error occurs, the cleanup will happen.
However, it's important to use the finally block judiciously. Overusing it for tasks that could be handled in other ways can lead to a cluttered codebase.
In summary, understanding and implementing error handling through try and except blocks and finally statements is integral for developing robust Python scripts. By acknowledging potential errors and planning for them, programmers can create smoother and more reliable user experiences.
Best Practices for Python Scripting
When embarking on Python scripting, understanding best practices can be a game changer. Just like knowing how to navigate a maze makes all the difference in reachin' the end, following these practices can enhance your programming journey significantly. The importance of code structure, readability, and performance isn't merely about adherence to norms; it's about evolving as a proficient developer.
Code Readability and Style
Code readability is akin to good handwriting in an exam paper; if it's not legible, the content may not get a fair evaluation. In Python, readability isn't just about aesthetics ā it's a fundamental principle. It allows other developers (or even your future self) to comprehend your logic without scratching their heads like confused chickens.
Here are a couple of key points to consider for enhancing the readability of your scripts:
- Use Meaningful Variable Names: Instead of variable names like , , or , opt for descriptive names such as , , or . This clarity helps convey intent right away.
- Consistent Indentation and Formatting: Sticking to consistent indentation helps prevent errors and confusion. Python relies on indentation levels, so mixing tabs and spaces could lead to headaches. The popular convention is aligning your code with either four spaces or a tab.
Additionally, consider using docstrings to document your functions. Here's an example:
By including this documentation, anyone reading your code will instantly know what the function does, reducing the time taken to decipher what something is meant to do.
Optimizing Performance
Optimizing your scriptās performance is like tuning an engine for speed; it ensures your code runs smoother and quicker, ultimately leading to a better experience for the user. Python isn't the quickest language out there, but with careful considerations, you can squeeze out a bit more speed. Hereās how:
- Profile Your Code: Before optimizing, understand where the bottlenecks are. Python provides tools like that help identify slow sections of your code.
- Avoid Unnecessary Loops: Instead of looping through elements multiple times, try using list comprehensions or built-in functions. They are typically faster and cleaner.
For example, instead of this:
You could write:
- Use Libraries Wisely: Donāt reinvent the wheel. Libraries like NumPy for numerical data or Pandas for data manipulation can leverage lower-level implementations that execute faster than standard Python loops.
Always remember, a fast script is good, but a readable, maintainable script is better.
By integrating readability and performance best practices, you lay a solid foundation for not just working on current projects, but as you advance, these guidelines will serve as cornerstones in how you approach programming challenges in the future.
Advanced Topics in Python Scripting
When delving into the realm of Python programming, one cannot overlook the advanced topics in scripting. These concepts serve as building blocks that elevate basic scripts into robust and efficient programs. Understanding how to utilize modules, manage packages, and integrate with databases will not only enhance oneās coding abilities but will also expand the potential applications of Python in various projects.
Working with Modules and Packages
Working with modules and packages is integral to streamlining workflow and reducing redundancy in code. Modules are files containing Python code, while packages are collections of modules. This structural hierarchy allows programmers to efficiently organize their code, leading to improved maintainability and collaboration.
Creating Custom Modules
Creating custom modules is an excellent way to encapsulate code that performs specific functions. Imagine developing a module that handles user authentication in a web application. By defining this functionality in a separate module, you gain several benefits. One key characteristic of creating custom modules is reusability. Once you write a module, you can easily import it across different scripts without needing to rewrite the code. This promotes a clean coding environment and ensures consistency.
The unique feature of custom modules lies in their ability to abstract complex functionalities into a simple interface. For instance, you could expose only necessary functions while keeping the internal workings hidden. While this has many advantages, such as better organization and ease of debugging, itās vital to maintain thorough documentation. Otherwise, a well-organized module can become a black box to other developers working on the same project.
Using Third-Party Packages
On the flip side, using third-party packages can significantly speed up development and introduce powerful tools into your script. Libraries like NumPy, pandas, or requests provide pre-built functions that handle data manipulation, analysis, and web requests, respectively. The major characteristic here is convenience. Why write your own code to handle complex mathematical computations when reliable libraries already exist?
One of the unique features of third-party packages is the active community that often surrounds them. This means that any issues you encounter can usually be troubleshot by consulting online forums or documentation. However, before incorporating a package, itās crucial to consider its longevity and maintenance; relying on obsolete or poorly maintained packages can lead to significant hurdles down the road.
Integrating with Databases
Integrating Python scripts with databases opens doors to dynamic data manipulation and storage capabilities. Whether it's retrieving information for a web application or logging data from an IoT device, understanding how to connect to and interact with databases is essential.
When you integrate with databases, you often utilize SQL or Object-Relational Mapping (ORM) techniques. Both allow you to perform complex queries and ensure data integrity, but they require different skill sets. Knowing how to manage database connections, execute commands, and handle results defines a competent programmer's edge. Keep in mind that each database solution has its own quirks, and taking the time to understand these nuances will pay off in responsiveness and efficiency.
Closure
The conclusion of an article on starting a Python script is crucial, as it reinforces the foundational knowledge imparted throughout the text and paves the way for future exploration in the world of programming. Summarizing the key insights helps to reinforce learning, ensuring that readers walk away with a clear understanding of what they have grasped. It's more than just a wrap-up; it's an encapsulation of the journey undertaken in previous sections.
Recap of Key Points
In this guide, several pivotal points were outlined to equip you for starting a Python script:
- Understanding Python Scripts: Established what defines a Python script and its significance in the broader programming landscape.
- Setting Up the Environment: Discussed the necessity of having the right tools, including choosing an appropriate Integrated Development Environment (IDE) and the steps to install Python.
- Creating Your First Script: Introduced the structure and elements of a Python script, emphasizing comments, functions, and proper syntax to aid readability.
- Running Python Scripts: Covered various methods of executing scripts, from using the command line to running scripts within an IDE, making it clear how scripts function in practice.
- Error Handling: Provided insights on common pitfalls and the usage of exception handlingāan essential skill to manage program errors effectively.
- Best Practices: Highlighted the importance of code readability and performance, essential attributes for successful programming.
- Advanced Topics: Briefly touched on expanding skills by using modules, packages, and integrating databases, which users will find beneficial as they evolve in their coding journey.
This summarization sends the message that a solid foundation has been laid, readying readers for deeper pursuits in programming.
Future Learning Paths
With the knowledge of starting a Python script firmly in place, you are positioned to branch out into more advanced programming concepts and practical applications. Consider the following paths:
- Deep-Dive into Python Libraries: Learn about libraries such as NumPy and Pandas that are used for data manipulation and analysis.
- Web Development: Explore frameworks like Flask or Django, which facilitate the building of web applications with Python.
- Data Science and Machine Learning: Investigate how to harness Python's capabilities in data science, machine learning, and artificial intelligence, with tools like TensorFlow and Scikit-learn.
- Software Development Practices: Delve into software engineering principles, version control with Git, and testing methodologies to ensure high-quality code.
Being curious and open to continuously learning will maximize your potential as a programmer. Take advantage of resources like Wikipedia, Britannica, and communities on Reddit and Facebook to enhance your knowledge base. This is just the tip of the icebergākeep your eagerness alive, and you will continually evolve as a proficient coder.
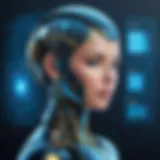
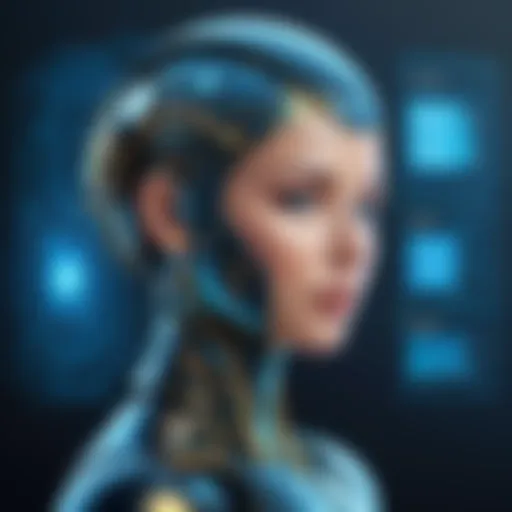