Testing Your Java Code Online: A Complete Guide
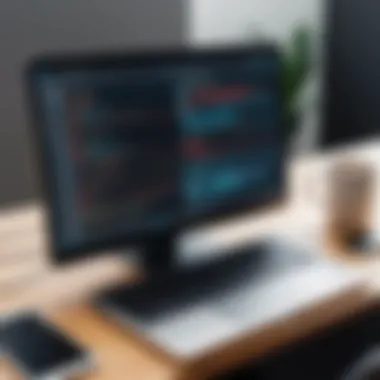
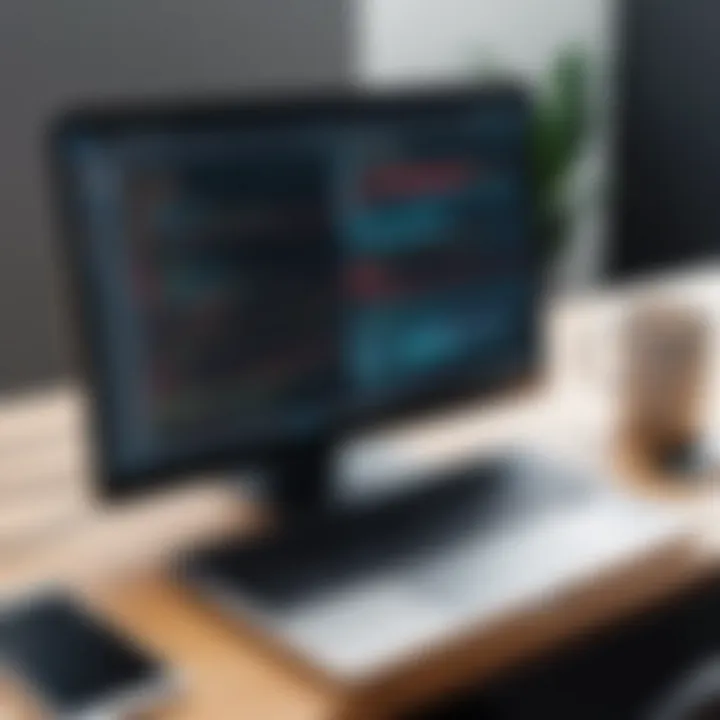
Overview of Topic
Intro to the Main Concept Covered
Testing Java code online has transformed how programmers refine their skills and tackle challenges. By utilizing various online platforms, developers can experiment with their code without the overhead of local installations or configurations. This method not only streamlines the coding process but also fosters collaboration and sharing of coding practices across the globe.
Scope and Significance in the Tech Industry
In recent years, the demand for skilled Java developers has surged, placing significant emphasis on effective testing methodologies. As a language renowned for its versatility, Java powers everything from small apps to large enterprise systems. Being proficient in online testing aids developers in understanding how their code performs in real-world environments, thus enhancing both personal growth and overall project outcomes.
Brief History and Evolution
When Java first emerged in the mid-'90s, testing was often a manual and cumbersome process. Programmers relied heavily on local environments that were often clunky. With the rise of cloud technology, tools like JDoodle and Replit have made it easier to test and debug code without the hassle of installation. The evolution has led to a rich array of platforms that cater to both novice and experienced coders.
Fundamentals Explained
Core Principles and Theories Related to the Topic
At the heart of testing Java code online is the principle of rapid feedback. The goal is to identify issues as soon as possible in the development cycle. This proactive approach reduces the time and resources spent on resolving bugs later on. Additionally, the concept of continuous integration has gained traction, emphasizing the need for consistent testing as codes evolve.
Key Terminology and Definitions
Understanding the lingo can be crucial:
- Compilation: The act of converting Java code into machine language.
- Debugging: The examination of code to identify and correct errors.
- IDE: Integrated Development Environment, a software application providing facilities to programmers for software development.
Basic Concepts and Foundational Knowledge
Before diving into testing, it's essential to grasp foundational concepts like object-oriented programming, as this is core to Java's architecture. Familiarity with data structures and algorithms can also elevate the level of testing effectiveness.
Practical Applications and Examples
Real-World Case Studies and Applications
Consider a software development team working on an e-commerce application. By utilizing platforms such as JUnit and Mockito for online testing, they can simulate user interactions and ensure that the checkout process operates as intended. This real-world application exemplifies the significance of thorough testing in delivering a reliable product.
Demonstrations and Hands-On Projects
An effective way to grasp these platforms is through hands-on projects. For example, building a simple user login system can serve as a practice case for testing the boundaries of your code. Developers can input intentional errors to see how the system handles various situations, which illustrates the effectiveness of online testing environments.
Code Snippets and Implementation Guidelines
The following is a simple code snippet using JUnit that illustrates how to test a method:
This snippet highlights the clarity and precision in online testing platforms that support JUnit.
Advanced Topics and Latest Trends
Cutting-Edge Developments in the Field
As technology continues to evolve, AI-driven testing solutions have emerged, which automate the testing process. Platforms like Test.ai offer capabilities that adapt as manual tests evolve, saving valuable time.
Advanced Techniques and Methodologies
Exploring methodologies such as Test-Driven Development (TDD) can further improve testing practices. TDD encourages writing tests before the actual code, leading to better-designed, bug-free programs.
Future Prospects and Upcoming Trends
As cloud computing advances, online testing tools will likely become more integrated, offering real-time collaboration features that allow teams to test code concurrently from different locations. This shift is expected to bolster remote work efficiency among developers.
Tips and Resources for Further Learning
Recommended Books, Courses, and Online Resources
- Effective Java by Joshua Bloch offers best practices for using Java.
- Online courses from platforms like Coursera and Udacity provide structured learning paths for developers.
Tools and Software for Practical Usage
Some noteworthy tools to explore include
- Replit: An interactive online IDE that supports real-time coding.
- JDoodle: Offers a quick way to run Java code snippets with a variety of features.
Online code testing empowers developers to refine their skills and improve the quality of their work, ultimately impacting the software they produce.
This guide serves as a clear roadmap for anyone interested in navigating the exciting world of online Java code testing. The landscape is rich with resources and opportunities for growth, all at the fingertips of eager learners.
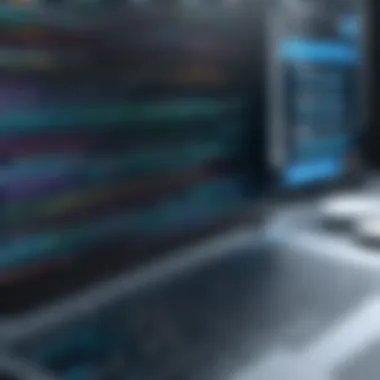
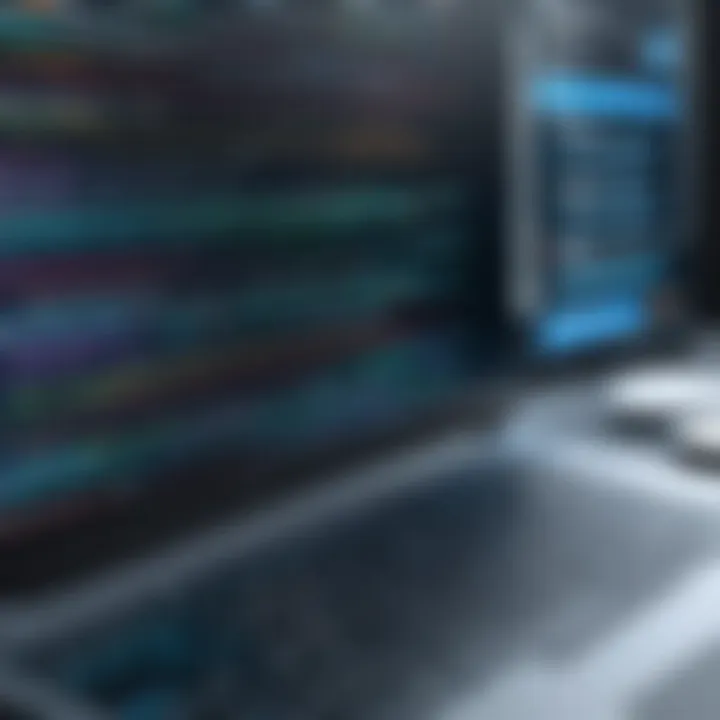
Preface to Java Code Testing
In the ever-evolving landscape of software development, the significance of testing Java code cannot be overstated. It plays a critical role in ensuring that programs function as intended while maintaining quality and performance. Developers, whether novices or seasoned professionals, often face the crucial task of testing their code effectively. The challenge is not only to produce code that works but also to create robust, efficient, and maintainable applications. Flaws can creep into code at any stage, and catching these errors early can save time, money, and headaches down the road.
Importance of Code Testing
Testing code is akin to laying a solid foundation before erecting a skyscraper. Without thorough testing, software applications can become prone to bugs, leading to unexpected failures during runtime. Here are several compelling reasons to prioritize code testing:
- Quality Assurance: Regular testing helps maintain high standards of code quality. Bugs found during earlier stages are easier and cheaper to fix than those identified post-deployment.
- Early Detection: Bugs absorbed into the codebase can snowball, causing significant issues later. By proactively testing as code is developed, issues can be nipped in the bud.
- User Experience: Software riddled with errors can lead to a frustrating user experience. Ensuring that your application is tested can contribute to a more user-friendly product, increasing satisfaction and trust in the software.
Effective testing is a small step that translates to a giant leap in software quality.
- Confidence Building: Developers gain confidence when they know their code has been rigorously tested. This sense of reliability improves productivity and promotes a culture of accountability.
This section forms the bedrock for any developer's journey in honing their coding skills. Every piece of code written should go through testing to solidify its integrity and functionality.
Overview of Online Testing Platforms
In today's fast-paced digital world, online platforms emerge as invaluable tools for testing Java code. They offer convenience and flexibility, making them accessible to individuals regardless of location. Developers no longer need to worry about setting up local testing environments, as these platforms provide everything needed at their fingertips.
These online resources allow users to:
- Run Code Instantly: With just a few clicks, developers can execute their code on various platforms without additional setup.
- Access Diverse Environments: Most platforms support multiple Java versions and configurations, enabling developers to see how their code behaves in different scenarios.
- Collaborate Easily: Many online tools provide sharing capabilities, allowing for teamwork and peer reviews of code, enhancing the learning process.
- Utilize Rich Resource Libraries: Some platforms even include repositories of sample code, educational material, and forums for discussions, making it easier to solve problems and expand knowledge.
The transition to leveraging these online testing tools can dramatically improve the efficiency and effectiveness of Java code testing. By incorporating these platforms into the coding workflow, developers can focus on crafting better solutions while minimizing the risk of errors in their applications.
Understanding Java Programming Language
Understanding the Java programming language is foundational when discussing online testing of Java code. Java, being one of the most widely used programming languages, offers several advantages such as portability, strong typing, and an extensive standard library. Deals with everything from web development to mobile applications, making it crucial for learners and professionals alike to have a solid grasp of its core elements.
Core Concepts of Java
At the heart of Java lie several core concepts that seamlessly blend together to create a powerful programming environment. Let's break these down:
- Object-Oriented Programming: Java is an object-oriented language, meaning it uses objects to represent data and methods. This approach allows for better organization of code and promotes reusability. Understanding concepts like classes, objects, inheritance, polymorphism, and encapsulation is vital.
- Platform Independence: The mantra "write once, run anywhere" embodies the essence of Java. It compiles code into bytecode that runs on the Java Virtual Machine, enabling it to operate on various platforms without modification. This portability is one of Java's significant strengths.
- Strongly Typed Language: Java is a statically typed language, which means that variable types are declared explicitly. This aids in catching errors during compile time rather than at runtime, providing a safety net that is especially vital in larger applications.
- Automatic Memory Management: Java manages memory automatically through garbage collection. This eases the programmer’s burden of memory management, greatly reducing memory leaks and optimizing resource usage.
These concepts lay the groundwork for effective Java coding and are critical when utilizing online tools for testing.
Common Errors in Java Code
Even experienced Java developers can stumble into some common pitfalls. Knowing these errors can save a lot of time and frustration.
- Null Pointer Exceptions: One of the most frequent errors, this occurs when the code attempts to use a null reference. It's essential to check for null values before dereferencing an object.
- ArrayIndexOutOfBoundsException: This happens when trying to access an index that’s outside of the declared range of an array. Always ensure that your loop bounds align with your array size.
- ClassCastException: This error surfaces when attempting to cast an object to a subclass it does not belong to. Make sure to use instanceof checks before performing a cast.
- Compilation Errors: Often a product of typos or syntax mistakes. Java's strong typing makes many compilation errors easily identifiable, aiding in learning to recognize common coding patterns.
- Infinite Loops: A logic error that can lead to resource exhaustion. Guard your loops with proper exit conditions to prevent this issue.
"Learning to identify these common errors is akin to learning to ride a bike; you may fall off a few times, but once you get it, the ride becomes smooth and enjoyable."
In summary, grasping the fundamental aspects of the Java language lays the groundwork for effective coding practices. Understanding these core elements and their associated common pitfalls enhances a programmer's ability to write robust code, especially when leveraging online platforms for testing.
Benefits of Testing Java Code Online
Testing Java code online holds significant value in today’s fast-paced development environment. This approach not only streamlines the testing process but also opens a world of possibilities for programmers ranging from novices to seasoned professionals. Understanding these benefits can greatly enhance one’s coding journey and ability to deliver robust applications.
Accessibility and Convenience
One of the crowning advantages of online code testing is its sheer accessibility. Imagine being able to test your code from any device, anywhere around the globe. Whether you are sipping coffee at a café or lounging at home, the power of the internet puts a comprehensive testing suite within your reach. No more juggling on different devices or environments. You can log on and start coding — a true boon for those who are on the move.
What also adds to the charm is the user-friendly design of many online platforms. Most tools offer a clear interface, making navigation a piece of cake. Especially for students and beginners, this is crucial; they can focus on learning without getting bogged down in complex setups.
You may want to explore platforms like JDoodle or Replit for their easy accessibility, as they let you jump right into coding without any cumbersome installations. It’s as simple as clicking a button.
Immediate Feedback and Diagnosis
Another compelling aspect of online testing tools is the immediate feedback they provide. This function is invaluable for developers looking to fine-tune their code. As you write your Java code and hit 'Run', feedback is generated almost instantaneously.
Here’s how this plays out in practice: a programmer writing a small piece of code might inadvertently miss a semicolon or a variable name. In a traditional IDE, you may only discover this later in the debugging phase, which can waste time. However, with online testing platforms, errors are flagged as you code. This allows for a real-time learning experience, where you can address mistakes on the spot, thereby enhancing your skills faster.
"Immediate feedback transforms the learning landscape. It turns coding into a dynamic interaction rather than a stagnant session."
Furthermore, many of these platforms come equipped with built-in debugging tools that help diagnose issues in your code. This feature aids in understanding why certain snippets don’t run as expected.
In summary, the benefits of testing Java code online range from unmatched accessibility to prompt feedback. Both elements converge to create a potent coding ecosystem, supporting programmers at all levels to improve their skills and increase their productivity.
Popular Online Tools for Testing Java Code
The realm of online code testing keeps expanding, ushering in a wave of practicality and flexibility. In this section, we delve into the tools that stand out in the crowd, aiding both novices and seasoned developers in verifying their Java creations. Knowing which platforms to lean on can significantly improve coding efficiency and enhance learning efficacy. It's like having a Swiss army knife at your fingertips—a well-rounded toolkit that meets various coding needs.
Overview of Available Platforms
When it comes to testing Java code online, several key players have emerged, each with its distinctive features and purposes. Selecting the right one can be akin to picking the right tool from a vast toolbox. Here are a few that have carved a niche:

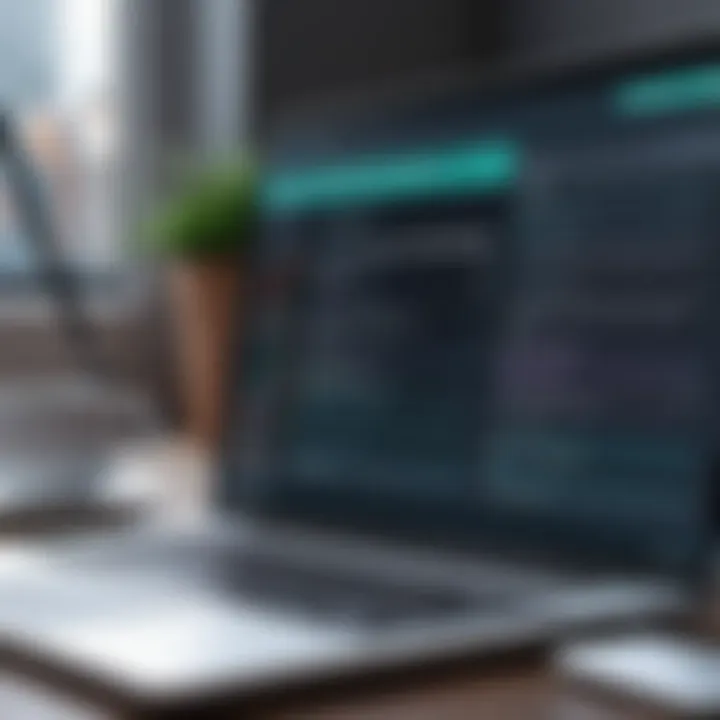
- JDoodle: This tool brings simplicity to the forefront, allowing users to write and run Java code with ease. Its user-friendly interface makes it a prime choice for beginners.
- Replit: Replit has captured the attention of learners and educators alike with its collaborative features. It fosters an environment where multiple users can work on code together, breaking down the barriers of geographical location.
- OnlineGDB: Renowned for its powerful debugging capabilities, OnlineGDB offers an integrated development environment that many professionals gravitate toward.
- Codiva: This tool excels in real-time compilation and online assessments, making it popular for academic settings and coding competitions.
- IDEone: A veteran in the online coding landscape, IDEone allows for testing snippets of code quickly and efficiently. Its support for multiple programming languages broadens its appeal.
Comparative Analysis of Tools
JDoodle
JDoodle stands out for its straightforward functionality and fast execution time. There’s a charm in its simplicity—users can paste their code and run it without diving deep into complex configurations. A key characteristic of JDoodle is its extensive language support, extending beyond Java to include languages like Python and Ruby. This makes it an appealing choice for those dabbling in multiple languages. One unique feature is its support for creating a shareable link to your executed code, which is handy for collaboration or requesting feedback from peers. The downside? It may not provide advanced debugging tools, limiting its efficacy for more intricate projects.
Replit
Replit thrives on collaboration. Its integrated platform allows users to work together in real-time, much like having a coding buddy over your shoulder. What makes Replit particularly engaging is its community aspects, where users can share projects and get real-time feedback from others. The ability to host and deploy applications directly from the platform is another feather in its cap. However, the online nature means that heavy processes might lag, which can be a hiccup for performance-heavy applications.
OnlineGDB
OnlineGDB could be your best ally when debugging complex Java code. This tool facilitates deep dives into issues with its built-in debugger, making it a preferred choice for developers needing to troubleshoot effectively. One of its key features is the debugger that supports step-through execution. This allows users to pinpoint where things go awry in their code. The platform also presents a clean interface—that’s always a quiet win. On the flip side, first-time users might find the range of features slightly overwhelming before getting accustomed to the layout.
Codiva
Codiva earns high marks for real-time compilation. As you type, the code is compiled, providing immediate feedback. This feature is especially useful for learners trying to grasp Java syntax and logic. Its assessment capabilities lend it well to educational environments where instructors can gauge coding ability through assignments. However, Codiva may not be as robust when it comes to extensive project support, constraining seasoned developers seeking comprehensive tools for larger applications.
IDEone
IDEone has been around for a while and has earned its reputation as a reliable go-to for code testing. It's particularly valued for its support for multiple programming languages, which aids developers looking for a multi-purpose platform. The ability to test code segments quickly makes IDEone user-friendly for those with tight deadlines. However, the lack of a collaborative feature could make it less appealing for teams who need to engage collectively on projects.
In summary, each tool has its unique charm and cater to different needs. Ultimately, the choice of a platform may boil down to what fits your particular workflow and objectives. Experimenting with a few might just lead you to the one that resonates best with your coding style.
Frameworks and Libraries for Testing
When it comes to testing your Java code, frameworks and libraries play a pivotal role in streamlining the testing process. They not only provide a structured way to run tests but also help maintain the integrity and reliability of the code. Utilizing these resources effectively can lead to more efficient code development and reduced error rates in the long run. Without a solid testing framework, the process can become cumbersome, with developers spending hours tracking down bugs that could’ve been caught earlier.
JUnit: The Standard Testing Framework
JUnit is arguably the backbone of unit testing in Java. This framework allows developers to write repeatable tests, which can be run automatically, providing a simple way to check if the application behaves as expected. One of its most important features is its integration with various IDEs, making it seamless to run tests without leaving the development environment.
Using JUnit, developers can create test cases as separate classes or methods. This modular approach not only improves code readability but also simplifies the debugging process, as issues can be traced to individual test cases. Also, JUnit supports annotations that give context to tests, such as when they should run or whether they should be ignored.
Mocking Frameworks: Enhance Testing Reliability
Mocking frameworks are essential tools that allow developers to simulate parts of the application, which is particularly useful for isolating specific components during testing. They enable tests to focus on the unit under test, disregarding external dependencies, which can lead to more accurate results. Let’s delve into two prominent mocking frameworks: Mockito and EasyMock.
Mockito
Mockito stands out as a favorite among Java developers due to its straightforward API and versatility. It allows you to create mock objects, define their behavior, and verify interactions. The key characteristic that sets Mockito apart is its use of an expressive domain-specific language (DSL). This makes it easy to read and write tests that clearly communicate intent.
A unique feature of Mockito is its ability to verify that certain actions have taken place in the code, which can be very useful for ensuring that your unit tests cover the desired functionality. However, while it's powerful, developers should be careful not to overuse mocking, as it can sometimes lead to tests that do not reflect real-world scenarios.
EasyMock
EasyMock is another well-regarded mocking framework that provides an alternative for those who prefer a more declarative approach to mocks. The unique feature of EasyMock is that it allows you to create strict mocks or nice mocks. Strict mocks check that all expected calls were made, providing a more rigorous testing framework, while nice mocks allow for flexibility, giving developers some breathing room in what they need to validate.
EasyMock's main advantage lies in its ability to simplify the creation of expectation-based tests, which can reduce the burden on developers who might be dealing with complex dependencies. However, like Mockito, EasyMock also requires a careful balance, as over-mocking can lead to tests that become overly complicated or disconnected from actual application logic.
Mocking frameworks help isolate unit tests, ensuring that they are focused and reliable, reducing false positives caused by dependency complexities.
Best Practices for Online Code Testing
Testing your Java code online can be challenging at times, but following certain best practices can indeed make a remarkable difference. These practices help minimize errors, improve code quality, and enhance the overall development experience. In online environments, where various tools and platforms can be employed, it's crucial to employ strategies that streamline the process and ensure predictable outcomes.
- Understanding the Code Readability – Writing readable code is not merely a recommendation; it's a necessity. Code that is neatly structured, properly indented, and well-commented allows not just you, but also others who may interact with your code, to understand its functionality without too much trouble. By prioritizing readability, you facilitate easier debugging and collaboration, whether you’re flying solo or working within a team.
- Debugging Tools Utilization – Debugging tools are your lifelines for identifying those pesky bugs and inefficiencies in your code. Relying on these can lead to a quicker testing cycle and a more straightforward troubleshooting process. Regardless of the testing environment you're using, being familiar with various debugging options can be a game changer. It enables swift navigation through issues and saves valuable time.
Writing Readable Code
Writing clean, readable code is like drafting a well-structured essay—everyone appreciates clarity. By incorporating consistent naming conventions, appropriate whitespace, and meaningful comments, you not only enhance your own understanding but also create a codebase that’s easier for others to pick up and work with.
This section will outline key practices for writing readable code:
- Consistent Naming: Stick to naming styles, be it camelCase or snake_case. Clear names reflect their purpose.
- Modular Code: Break down large methods into smaller, manageable functions. This not only makes debugging easier, but also improves readability.
- Code Comments: Use comments generously but wisely. Clear comments explaining the "why" behind complicated logic help future-you (or your teammates) grasp the purpose of your code quickly.
Making your code readable pays off, especially during online testing. Platforms often allow other developers to see your code, and they’ll appreciate well-structured snippets that communicate clear logic. In an online test environment, clarity means fewer misunderstandings and faster troubleshooting.
Utilizing Debugging Tools
Debugging in IDEs
Integrated Development Environments, or IDEs, like IntelliJ IDEA, Eclipse, or NetBeans come packed with powerful debugging tools. These IDEs enable a hands-on approach to track down bugs directly within your code as you run it.
A vital characteristic of debugging in IDEs is breakpoint functionality. Breakpoints allow you to halt execution at a particular line of code, providing the chance to inspect variable states and the flow of execution. This feature is invaluable when pinpointing the cause of unexpected behavior in your code.
The essence of using IDEs lies in their rich user interfaces, allowing for an interactive debugging experience. However, it also has its downsides; less experienced developers might find the complexity overwhelming. Still, the customizable features and visual support make IDEs a popular and beneficial choice for effective debugging.
Interactive Debugging in Online Tools
Online coding platforms increasingly include interactive debugging tools that offer an alternative to traditional IDEs. Platforms like JDoodle and Replit support real-time coding, enabling immediate testing and debugging within a shared code environment.
The key characteristic of this method is the immediacy it provides. You can test parts of your code as you write, catching errors before they escalate into bigger issues.
These interactive tools often integrate user-friendly interfaces that facilitate coding and debugging without heavy setup. However, while they’re great for quick tests, they may not support all language features or debugging functions that a complete IDE would.
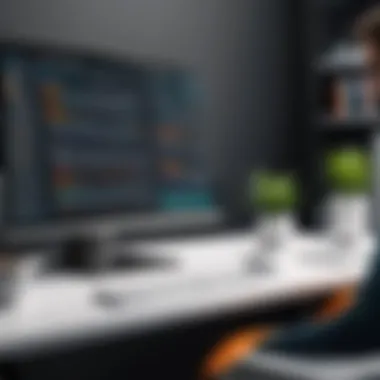
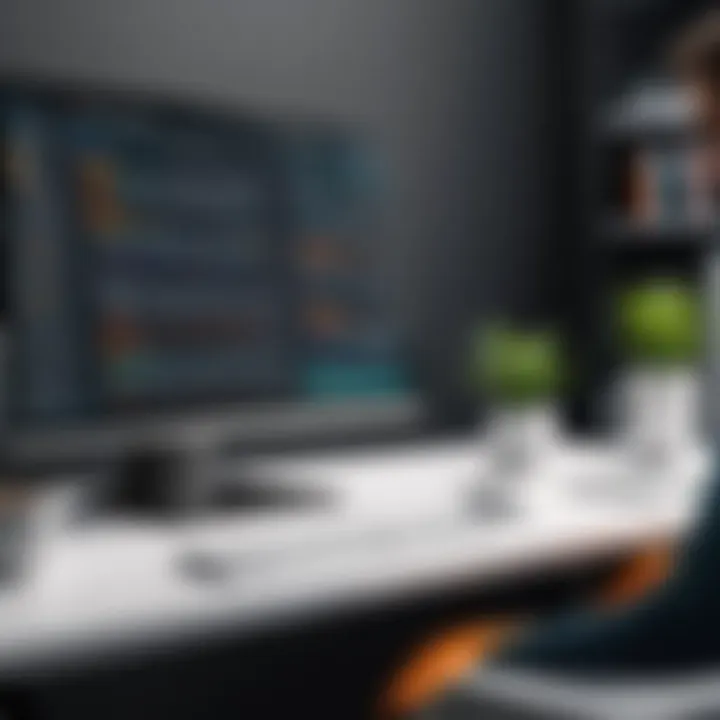
Being aware of the strengths and weaknesses of both IDE debugging and online tools creates a well-rounded approach to debugging. It turns the online testing process into a more efficient and effective experience for learning and improvement.
"The beauty of coding lies not just in creating a solution but also in the clarity with which each step is represented."
Security Considerations When Testing Code Online
When it comes to coding, especially for beginners and experienced programmers alike, utilizing online platforms can seem like a walk in the park. However, lurking beneath the surface are profound security considerations that can’t be ignored. These concerns not only protect your code but also your sensitive data. In this section, we will outline the essential elements of security in online Java code testing, shedding light on the importance of safeguarding both your work and personal information.
Understanding Data Privacy
Data privacy is an increasingly hot topic in today’s digital landscape. When you test your Java code online, you might unwittingly put your data at risk. It’s crucial to understand what kind of information you are sharing with the platform and, consequently, with the potential audience. Quite often, platforms require you to create an account, leading to the necessity of providing personal information.
- Service Terms: Always read the terms of service. Some sites might retain rights to your code even after it has been deleted.
- Data Encryption: Look for platforms that use encryption to keep your data safe. This means, essentially, that your information is scrambled and secure from prying eyes.
- Anonymous Usage: If possible, consider using online tools that allow for anonymous testing. This way, you can mitigate the risk of your identity being linked to specific projects.
Understanding data privacy means you can better secure your own coding environment. The impact of a data breach is tremendous, often leading to identity theft or unauthorized access to sensitive projects.
Risks of Code Exposure
Code exposure is another significant risk associated with online testing. While collaboration and idea exchange are encouraged in the software community, there is a flip side. When you share your code through online platforms, you run the risk of exposing your intellectual property to others who may not have your best interests at heart.
"Just because your code is out there doesn’t mean it’s safe. Make sure to keep a tight lid on sensitive portions!"
Here are some risks to consider:
- Unauthorized Copying: Someone could easily replicate your code and pass it off as theirs. This kind of plagiarism isn’t just ethical—it can have legal implications depending on the situation.
- Vulnerability Exploitation: If your code contains sensitive logic or algorithms, exposing them might allow malicious actors to design attacks specifically tailored to exploit weaknesses in your approach.
- Platform Reliability: Some lesser-known platforms may not have robust security measures in place. This calls for careful consideration before using any unfamiliar tools.
To summarize, testing your Java code online can save time and streamline the development process. However, it is vital to be acutely aware of the security implications associated with using these platforms. By understanding data privacy and potential risks, you’ll be well-equipped to navigate the necessary precautions and protect not just your code, but your overall integrity as a developer.
Integrating Online Testing into Your Workflow
In the world of software development, code testing is not just an optional task; it's essential. With the rapid pace of development, integrating online testing into your workflow becomes crucial. This section delves into the significance of adopting online testing solutions and illustrates how they streamline the typical coding process. By acknowledging the pivotal role these tools play, developers can not only enhance their productivity but also significantly improve the quality of their code.
Creating a Workflow Plan
A well-structured workflow plan lays the foundation for effective online testing. Simply put, a workflow plan outlines the steps you intend to take when coding and testing your application. It serves as a roadmap and helps maintain clarity. To create a robust plan, consider the following elements:
- Define Your Goals: Start by identifying the objectives of your coding project. What do you want to achieve? Clear objectives help steer your testing efforts.
- Choose Your Tools: Pick the right online testing tools that align with your needs. Each tool comes with its unique features, so it's worth doing some research.
- Establish Timeframes: Set aside specific times in your workflow for testing. By incorporating testing sessions, you avoid last-minute scrambles before a deadline.
- Document Everything: Keep record of your findings, test results, and any adjustments made along the way. Documentation aids in tracking progress and makes troubleshooting easier.
Once your workflow plan is in place, you're on a path to a more organized testing process. This can prevent potential issues later on, ensuring smoother development cycles.
Continuous Testing Practices
Incorporating continuous testing practices into your workflow is highly beneficial. In essence, it's about making testing a constant companion throughout your development journey rather than a one-time chore. This proactive approach leads to quicker identification of issues and provides invaluable feedback. Here’s how you can embrace continuous testing:
- Integrate Tests into Your CI/CD Pipeline: Continuous Integration/Continuous Deployment (CI/CD) automates the process of integrating code changes. By including automated tests within your pipeline, you can catch bugs sooner and with less effort.
- Frequent Check-ins: Instead of waiting till the end of a project to test, do so regularly. Frequent testing keeps the codebase healthy and allows for immediate rectification of bugs.
- Set Up Automated Tests: Automated tests save time and offer consistency. These tests can run every time a code change is made, ensuring that previously working functionality hasn’t been compromised.
- Encourage Peer Reviews: Engaging your peers in the review process can uncover issues that might slip past you. Fresh eyes often notice things you may miss.
- Feedback Loop: Create an efficient feedback loop. After testing, make sure to communicate the findings quickly so that corrective measures can be taken without delay.
Future Trends in Online Java Code Testing
As the landscape of software development continues to evolve, so does the way we approach testing Java code. The future trends in online Java code testing are not just buzzwords; they represent significant shifts that can enhance the effectiveness and efficiency of coding practices. By tapping into these emerging technologies and trends, developers can anticipate the needs of tomorrow’s coding environment while also improving their current workflow. The merits of staying ahead in this field cannot be overstated, as they directly influence code quality, collaboration, and the ability to swiftly adapt to changing requirements.
Emerging Technologies in Code Testing
AI-Driven Testing
AI-driven testing has become a hot topic in the realm of software development. This method utilizes artificial intelligence to automate the testing process, leading to often faster and more reliable results. One of the standout features of AI-driven testing is its ability to learn from past test outcomes. This characteristic not only streamlines the process but also helps in predicting potential issues before they manifest into bigger problems. For developers, this means a profound reduction in testing times and a more focused approach to debugging. While AI-driven testing is widely seen as a means to optimize workflows, developers should also consider the dependency it may create on technology and thus proper training is required to utilize it effectively.
Cloud-Based Testing Solutions
Cloud-based testing solutions are changing the way developers conduct their testing. With cloud integration, developers can access their testing environments from anywhere, provided they have internet connectivity. This accessibility is critical, especially for teams that operate in different geographies. A significant aspect of cloud-based testing is its scalability – teams can easily expand their testing resources up or down based on project needs without the hassles of managing physical servers. The downside here could be concerns about data security and privacy, which require stringent measures to mitigate risks effectively. Nevertheless, cloud solutions are indeed a modern-day necessity in the fast-paced world of Java development.
The Evolving Role of Online Platforms
As developers increasingly turn to online platforms for code testing, these tools are continuously evolving to meet their growing needs. What once were merely environments for testing are now becoming more integrated with development processes. The growing trend towards collaboration tools alongside code testing might be seen as a logical step in integrating coding and testing workflows. By simplifying the user experience and offering features like real-time feedback, platforms are making it easier and faster for teams to catch errors and improve overall code quality. This transformation underscores the necessity for developers, particularly those still learning or gettng familiar with Java, to stay updated on these developments, as they can heavily influence their productivity and the final quality of their code.
Ending
In a world where software development is evolving at breakneck speed, the importance of online code testing cannot be overstated. For students, budding programmers, and seasoned IT professionals alike, understanding the various facets of testing Java code online is crucial. This article highlighted the essence of effective testing methods, the myriad of available tools, and best practices to enhance overall coding proficiency.
Recap of Key Insights
As we wrap up, let’s take a moment to revisit the key insights discussed:
- Significance of Testing: Testing is far more than just a precautionary measure; it serves as a foundation for reliable software. By identifying bugs early, developers can save time and resources, ultimately leading to a smoother development cycle.
- Online Platforms: Tools like JDoodle, Replit, and IDEone offer robust environments for testing Java code. These platforms not only simplify the testing process but also provide immediate feedback, allowing for rapid iterations on code.
- Frameworks and Libraries: Leveraging frameworks such as JUnit or Mockito can enhance the reliability of tests. Employing these tools allows coders to create structured tests that can consistently catch errors before deployment.
- Best Practices: Writing readable, maintainable code and utilizing debugging tools effectively can dramatically improve the testing experience. This ensures that the code is not only functional but also understandable for future developers.
In sum, integrating testing into your coding regimen helps build stronger, more dependable software.
Encouragement for Ongoing Learning
The realm of coding and software development is continually changing, and so should your learning approach. Embrace a mindset of lifelong learning. Explore new tools, methodologies, and languages outside of Java. Attend workshops, take online courses, or engage in communities like reddit.com to share your experiences and gain insights from others.
Here are a few actions to consider:
- Experiment with New Tools: Don't hesitate to venture beyond the conventional. Each new platform or framework you dance with teaches you something unique.
- Stay Updated: Follow relevant blogs, forums, and podcasts to keep a finger on the pulse of new testing paradigms.
- Network with Peers: Engaging in discussions with fellow learners or experienced developers can open doors to new ideas and problem-solving approaches.
By adopting these strategies, you not only improve your own coding skills but contribute to a collaborative community of developers. Remember, the journey of learning is ongoing; seize every opportunity to grow.