Mastering Tree Maps in Java: Features and Best Practices
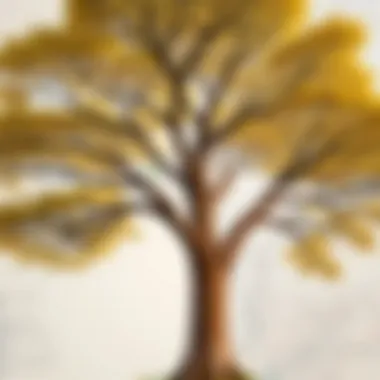
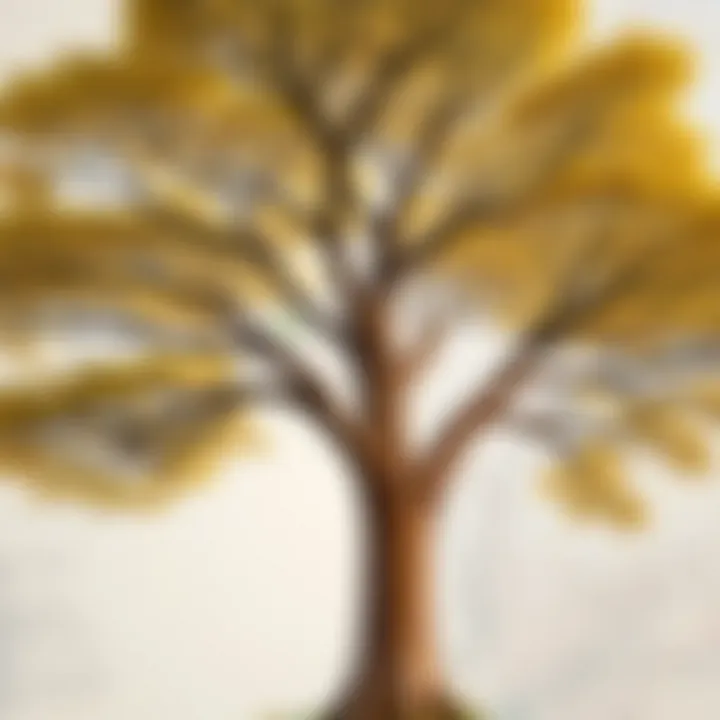
Overview of Topic
Tree Maps in Java are a unique form of data structures that hold a significant place in programming. At their core, they integrate the features of both a map and a sorted tree, which can be quite beneficial in various scenarios. Unlike traditional hash maps, Tree Maps maintain entries in a sorted order, making it easier to retrieve and manipulate data efficiently based on keys.
Prelims to the main concept covered
The concept of Tree Maps revolves around storing key-value pairs where each key is unique and kept in a natural order. When one thinks of keeping data for quick lookups but also needing that data to be sorted, Tree Maps provide an elegant solution. Their implementation allows developers to perform operations with logarithmic time complexity, thanks to the underlying red-black tree mechanism employed for storage.
Scope and significance in the tech industry
With the growing importance of data processing, especially in fields like data analytics and application development, Tree Maps have become indispensable. They find utility in applications requiring sorted data, as well as in scenarios needing high performance on search operations. Developers are compelled to understand Tree Maps for efficient coding practices, contributing to cleaner solutions and robust applications.
Brief history and evolution
Java introduced the Tree Map class in Java 1.2 as part of the Java Collections Framework. Initially, developers relied on various sorting methods, which often led to convoluted code and performance bottlenecks. The introduction of the Tree Map class solved this issue by providing a standard implementation that leverages sorting automatically while integrating seamlessly with other collections.
Fundamentals Explained
To grasp Tree Maps, one must first understand their core principles and associated terminology.
Core principles and theories related to the topic
Tree Maps maintain a strict order of keys. This property distinguishes them from hash maps, which do not guarantee any order. The ordered structure permits operations that can locate, insert, and delete entries at an efficient pace.
Key terminology and definitions
- Key: A unique identifier for the value stored in the Tree Map.
- Value: The data associated with a key.
- Entry: A key-value pair in the map.
- Natural order: The order defined by the Comparable interface or a Comparator specified at Tree Map creation.
Basic concepts and foundational knowledge
Before jumping into implementation, it's essential to understand how Tree Maps leverage node structures. Each node embodies an entry and is linked to its parent and child nodes, allowing for efficient navigation throughout the tree.
Practical Applications and Examples
The practical use of Tree Maps extends across many domains.
Real-world case studies and applications
Consider a financial application where transaction records need to be stored by date. A Tree Map can effortlessly manage these entries, allowing users to retrieve records efficiently based on chronological order.
Demonstrations and hands-on projects
Imagine building a simple application that manages a contact list. By using a Tree Map, contacts can be sorted alphabetically, which simplifies finding a specific entry:
Code snippets and implementation guidelines
In the above code example, the contacts are maintained in alphabetical order. Developers can enhance this basic implementation by adding methods for updating or deleting entries, showcasing the flexibility of Tree Maps.
Advanced Topics and Latest Trends
Despite being introduced a while ago, Tree Maps are not static in their use. Developers consistently find new methods and techniques to exploit their efficiency.
Cutting-edge developments in the field
As programming moves towards functional paradigms, integration of Tree Maps with streams is gaining popularity. This amalgamation enhances data processing capabilities, making it easier to perform complex transformations.
Advanced techniques and methodologies
One advanced technique involves combining Tree Maps with multi-threading. This can present challenges in terms of synchronization but pays off in enhancing efficiency, especially in applications requiring concurrent data access.
Future prospects and upcoming trends
As data structures evolve, Tree Maps will likely see integrations with machine learning algorithms, which rely heavily on sorted data for model training and predictions. The ability to manage large datasets efficiently is an area ripe for exploration and development.
Tips and Resources for Further Learning
Understanding Tree Maps is crucial for improving programming skills. Here are some recommendations:
- Books: "Effective Java" by Joshua Bloch - provides deep insights into Java collections.
- Courses: Online platforms like Coursera and Udacity often have dedicated Java programming courses.
- Tools: Integrated Development Environments like IntelliJ IDEA or Eclipse optimize coding and debugging.
For additional research or discussions, sites like Reddit and Wikipedia can be beneficial.
Tree Maps are not just a niche tool; they are an essential part of any Java developer's toolkit.
Prolusion to Tree Maps
In the nimble world of programming, developers often encounter a medley of data structures that are intricately woven into the fabric of efficient coding. One such gem is the Tree Map, a sophisticated instrument that combines the essences of ordering and association. Understanding Tree Maps is crucial not just because they offer a hierarchical storage model, but also for their pivotal role within the Java Collections Framework—an integral component of the language's robust ecosystem.
Definition of Tree Map
A Tree Map is a navigable map that implements the SortedMap interface. In simpler terms, it uses a red-black tree structure to organize data in key-value pairs while maintaining a sorted order. This ordered approach allows for efficient querying, as elements can be retrieved in a sorted manner. The keys in a Tree Map are unique and should be comparable, either by natural ordering or through the use of specified comparators. The balancing mechanism (in this case, the structure of the red-black tree) ensures that the operations remain efficient, generally achieving a time complexity of O(log n) for operations like insertion, deletion, and retrieval. The distinct feature of the Tree Map lies in its ability to maintain this order dynamically as new entries are added or existing ones modified.
Importance in Java Collections Framework
Tree Maps are not just another tool in your coding toolkit; they hold significant importance in the Java Collections Framework. Here are a few reasons why:
- Sorting Convenience: Unlike a HashMap, which does not guarantee any specific order, a Tree Map keeps keys sorted. This feature is invaluable when you require a predictable iteration order.
- Navigable Features: Tree Maps come with the benefit of the NavigableMap interface, which allows you to find the closest matches, ceiling or floor entries for given keys. This can be a game-changer in applications where finding related data points is crucial, like suggestion features in a search engine.
- Memory Efficiency: Given that Tree Maps implement a more space-optimized structure compared to alternative implementations while still offering sorted order, they can be an attractive choice for memory-sensitive applications.
Taking into consideration all these advantages, if you're working on data-heavy applications that require structured storage with efficient access, you'll find Tree Maps to be tremendously beneficial. They've become a staple for many real-world applications, showing just how relevant and helpful they are in day-to-day coding endeavors.
Remember, selecting the right data structure can often make the difference between a smooth-running application and one that stumbles over performance issues.
Understanding the Data Structure
Understanding the data structure that underpins Tree Maps is essential—especially if you're aiming to harness the full potential of Java's Collections Framework. Tree Maps provide not just a way to store key-value pairs, but also an efficient manner to keep them sorted, allowing for fast retrievals and modifications. The beauty of using Tree Maps lies in how they organize and maintain order, unlike some other map types that can seem chaotic. It all boils down to the structure of the nodes and how they interact with one another, ensuring optimal performance as your dataset grows.
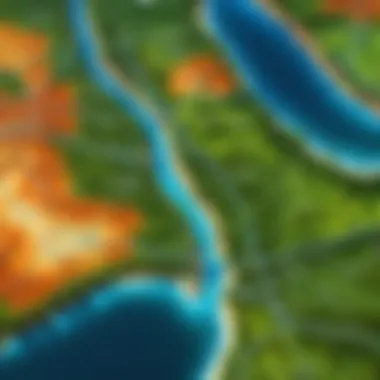
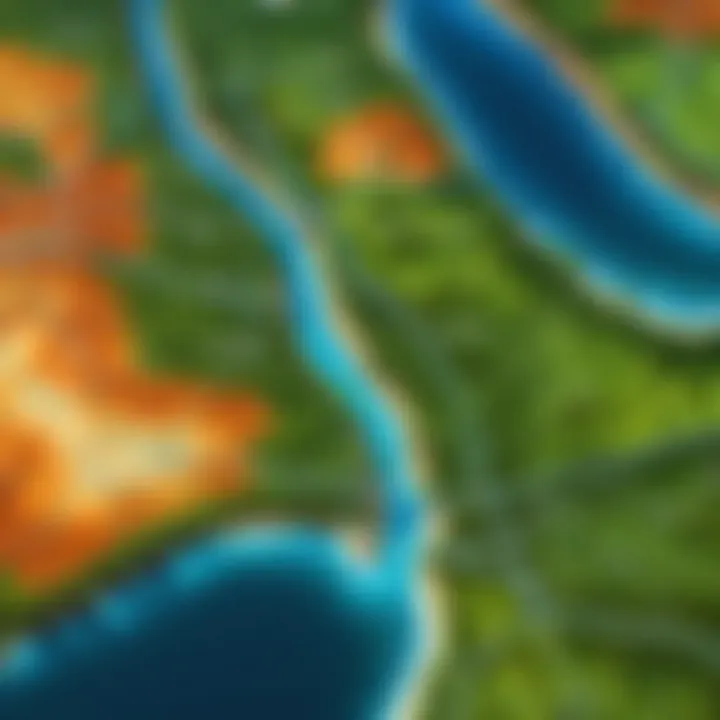
This section will delve into the mechanics of Tree Maps, providing clarity on their inner workings, and underscoring why understanding the data structure is vital for programming with Java.
How Tree Maps Work
Tree Maps operate on a binary tree structure, with nodes that store key-value pairs. At its heart, each key defines a unique path down the tree, with lesser keys positioned to the left, and greater ones to the right. This unique positioning allows for efficient searching, insertion, and deletion operations. The magic happens when you consider the balanced nature of the tree which, when kept in check, ensures that operations can be performed in logarithmic time.
Here’s an illustrative breakdown of how a basic insertion might work:
- Compare the new key with current nodes.
- Move left or right depending on whether it's smaller or larger.
- If the key is found, simply update the value. If it isn’t found, create a new node on the proper side.
This method of organization not only facilitates quicker access but also retains order, which can be crucial for many applications where data trends or relationships must be clearly observed.
Binary Search Tree Overview
Diving deeper, Tree Maps are often implemented using a specific type of binary search tree. A binary search tree (BST) has a straightforward governing principle: every node must be greater than all nodes on its left and less than those on its right. This makes searching for keys a swift endeavor. However, using a simple BST can lead to imbalances; imagine a scenario where you insert keys in increasing order. You could easily end up with a structure resembling a linked list, thus degrading performance drastically.
Balancing Mechanisms
To avoid the pitfalls of simple binary search trees, balancing mechanisms are employed to keep the tree optimally structured.
Red-Black Trees
Red-Black Trees are a specific kind of binary search tree that maintains balance through color coding nodes as either red or black. This characteristic plays a crucial role in ensuring that the tree remains roughly balanced, effectively preventing degenerate behaviors. A key point of Red-Black Trees is that they enforce specific properties during insertions and deletions, enabling fast rebalancing.
The main advantages of Red-Black Trees include:
- Efficiency: Operations like insertion and deletion remain on average O(log n).
- Balance Maintenance: Their strict coloring rules ensure a balanced tree, preventing poor performance even with several insertions and deletions.
On the flip side, their complexity in implementation can be a drawback, especially for beginners.
AVL Trees
AVL Trees represent another option when it comes to balancing. Named after their creators, Adelson-Velsky and Landis, they use a straightforward but effective balance factor to ensure that the tree stays balanced after every operation. Each node keeps track of heights of its left and right subtrees—if the difference exceeds 1, rotations are performed to restore balance.
The noteworthy aspects of AVL Trees include:
- Higher Balance Efficiency: They often maintain a stricter balance compared to Red-Black Trees, meaning faster lookups.
- Simplicity: The rebalancing operation is relatively straightforward, making it easier to understand.
However, this comes at the cost of slower insertion and deletion times as rotations may occur more frequently.
“Choosing the right balancing mechanism can be as crucial as selecting the data structure itself.”
Through a thorough understanding of these data structures and their balancing mechanisms, developers can make informed choices about integrating Tree Maps into their Java applications effectively.
Comparison with Other Map Implementations
When working with data structures, it’s essential to choose the right tool for the job. Tree Maps and Hash Maps are two prevalent implementations in Java, each having its unique characteristics and use cases. Understanding the differences is foundational, especially for those diving into the realm of programming and data management.
Tree Map vs Hash Map
Tree Maps are designed based on a red-black tree data structure, which inherently maintains a sorted order of keys. This structure makes it possible to perform operations such as range queries and ordered retrieval. Conversely, Hash Maps operate through a hash table mechanism, which enables quicker access to elements but does not preserve any order among entries. In practical terms:
- Ordered vs. Unordered: Tree Maps keep entries sorted according to their keys, providing an ordered view, while Hash Maps do not guarantee any order.
- Access Speed: In terms of performance, Hash Maps generally allow for constant time complexity for and operations, while Tree Maps, with their logarithmic time complexity, can be slower for these operations. This means that if speed is of the essence, particularly with large datasets, Hash Maps may have the edge.
- Memory Usage: Tree Maps are typically more memory-intensive due to the additional pointers required to maintain the tree structure, while Hash Maps can be more memory-efficient, depending on the size and distribution of hashed entries.
- Use Cases: When one needs to maintain a sorted order or perform range queries, Tree Maps come into play. In contrast, if the goal is quick access and insertion without regard to order, Hash Maps might be the preferred choice.
Advantages and Disadvantages
When pitting Tree Maps against Hash Maps, it is crucial to weigh their respective merits and drawbacks, as outlined below:
Advantages of Tree Maps:
- Sorted Order: Elements are automatically sorted, allowing for operations like , , and range retrieval with ease.
- Navigable Map Interface: Tree Maps implement the NavigableMap interface, providing methods to navigate through the map efficiently.
- Consistent Performance: The performance is more predictable. In scenarios with a high volume of data modifications, Tree Maps can provide more stable performance than Hash Maps, as operations do not degrade as dramatically.
Disadvantages of Tree Maps:
- Slower Performance: Operations like insertion and retrieval can be slower than with Hash Maps due to the logarithmic overhead.
- Increased Memory Use: The structure requires more overhead for maintaining the tree, leading to increased memory consumption.
In summary, both Tree Maps and Hash Maps have their place in a well-rounded programmer's toolkit. The choice depends on specific needs around ordering, performance, and memory considerations.
"The right data structure can make all the difference."
Understanding these distinctions allows new programmers and seasoned IT professionals alike to make informed decisions tailored to their coding challenges.
Core Features of Tree Maps
Tree Maps are more than just another data structure in Java; they offer specialized functionalities that can be crucial for specific coding scenarios. Understanding the core features of Tree Maps enriches one’s programming toolkit, particularly when striving for efficiency and organization in data handling. From their method of storing data to the way they inherently maintain order, these characteristics set Tree Maps apart from other mapping techniques like Hash Maps.
Key-Value Pair Storage
At the heart of any map data structure lies the concept of key-value pair storage. In Tree Maps, this concept is implemented with robust integrity. Each entry contains a unique key associated with a specific value. Unlike some other data structures, Tree Maps ensure a specific arrangement of those keys based on their natural ordering or a custom comparator defined by the user. This order is paramount when one considers retrieval speeds. When data is maintained in a sorted state, it not only quickens the search process but also simplifies certain algorithms that depend on key order.
For instance, if one is managing a phone book application, the keys (contact names) can be sorted in alphabetical order. Retrieving a name starts off as simple as skimming through the first few entries rather than searching through an unsorted list.
To illustrate this, consider a small section of code:
In this example, retrieving Charlie's number would be quite efficient in a Tree Map versus a Hash Map, where no inherent order is preserved.
Ordered Nature and Its Implications
The ordered nature of Tree Maps carries significant implications, especially when it comes to operations that require sequence. In coding, the necessity to access elements in a specific order frequently arises. For example, in a scoring application, you might need to display the scores of players in descending order. Because Tree Maps maintain their entries in a sorted state, developers can seamlessly achieve these requirements without additional sorting logic.
The implications of this order are twofold:
- Efficiency: Retrieval methods like and become straightforward because the keys are always arranged in a manner that allows swift access.
- Flexibility: Developers can implement various subsets of functions to return views of the map, thanks to methods such as , which allows them to focus on a certain range of keys.
In practical terms, knowing that you can efficiently extract information from a Tree Map without the fear of an unordered structure hanging like a cloud overhead lets developers write cleaner, more effective code.
"In a world increasingly driven by data, the ordered nature of Tree Maps can feel like a reassuring guide through the chaos of information."
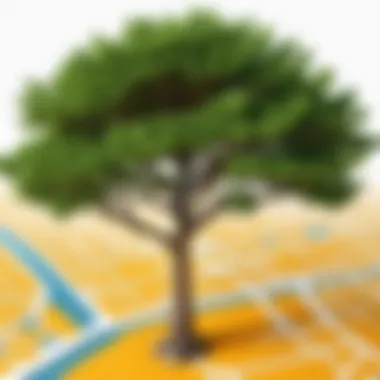
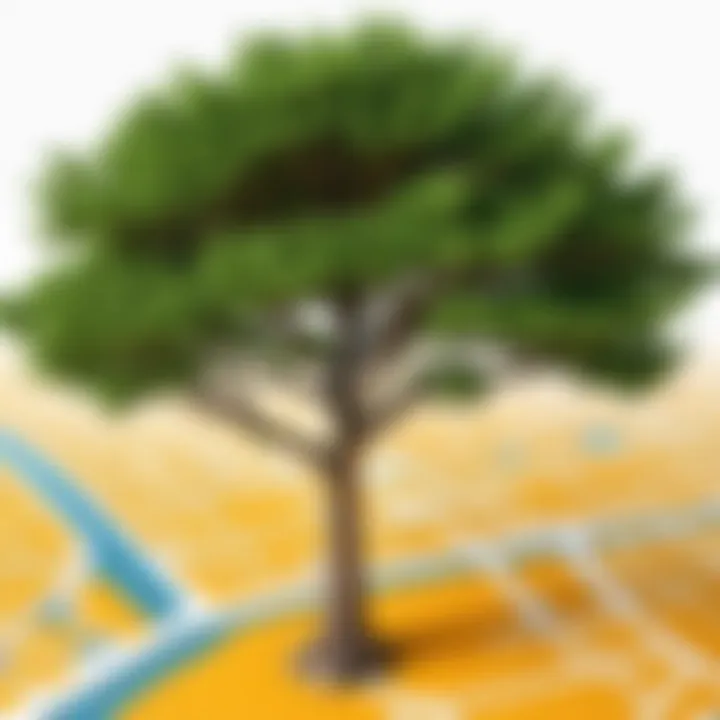
Tree Maps are not just a tool but an enablement mechanism that aligns with the structured thinking required for effective programming. Whether you're a student grappling with Java basics or an IT professional tackling complex programming challenges, recognizing these core features puts you a step ahead in harnessing the true potential of Tree Maps.
Common Use Cases for Tree Maps
Understanding where Tree Maps fit within programming can strengthen your application design, as they shine in various scenarios that require maintaining a clear structure and quick access to sorted data. This section delves into the specific use cases where Tree Maps prove their worth, exploring the nuances of their usability and the reasons behind their choice in practical applications.
Data Sorting Applications
One of the primary use cases for Tree Maps lies in data sorting. In situations where the order of elements is crucial, Tree Maps provide an efficient method for maintaining sorted key-value pairs. Their ability to automatically keep entries in order means that retrievals can be done with ease. For example, imagine you’re working on a grading system where students’ scores need to be listed from highest to lowest. Here’s how a Tree Map shines:
- Automatic Ordering: When inserting student scores with IDs as keys, the Tree Map manages their order without requiring extra sorting logic.
- Quick Access: When a user requests the top five scores, the Tree Map allows for efficient navigation through its inherent ordering.
This capability doesn't just stop at scores; you could apply it to any numerical data that needs prioritization or ordering by dates, making Tree Maps invaluable in numerous applications, from retail to finance.
Implementing Caches
Caches are critical in many systems, allowing for quicker access to frequently used data. Tree Maps can be used as an effective caching mechanism because of their efficient search capabilities and ordered structure. For instance, consider a situation involving an API that retrieves user information. Instead of querying a database each time a request is made, using a Tree Map can help implement a caching layer, like this:
- Keyed by Unique Identifiers: User IDs can serve as keys, while user info can be the values. New requests can be quickly checked against the Tree Map to see if the info is cached, reducing the need for database hits.
- Order Sensitivity: By keeping the cache entries sorted, you can implement strategies like least recently used (LRU) eviction based on timestamps stored alongside data, ensuring your cache remains efficient.
In this way, Tree Maps not only optimize the speed of data retrieval but also enhance memory management in resource-sensitive applications.
Ranking and Scoring Systems
Many applications necessitate a method for ranking items, such as leaderboards in gaming or product ratings in e-commerce. Tree Maps fit seamlessly into these scenarios due to their ability to sort and manage complex data. When creating a leaderboard for gamers, for instance, you can utilize a Tree Map as follows:
- Dynamic Updates: As scores change, updating a Tree Map keeps everything in order while granting quick access to top ranks.
- Multi-level Sorting: You could incorporate secondary keys, such as player names or levels, to ensure that if two players have the same score, one can still discern who ranks higher based on additional criteria.
This adaptability, combined with quick look-up times, makes Tree Maps a robust solution for any system requiring systematic rankings.
Utilizing Tree Maps in practical scenarios amplifies both efficiency and clarity, ensuring programmers can focus more on core functionality rather than sorting issues.
Working with Tree Maps in Java
Understanding how to work with Tree Maps in Java is crucial for developers looking to harness the capabilities of this powerful data structure. Tree Maps offer an ordered collection of key-value pairs, ensuring that the keys are sorted according to their natural ordering, or according to a specified comparator. This makes them favorable in situations where retrieval and range queries are needed, such as search engines or databases.
In essence, Tree Maps blend efficiency with usability, allowing programmers to manage large datasets while maintaining quick access and modification capabilities. Key operations like insertion, deletion, and retrieval are the pillars of working with Tree Maps, and they lead to improved performance in various applications. Let's break down the basic operations that lay the foundation for utilizing Tree Maps effectively.
Basic Operations
Insertion
Insertion in Tree Maps is the process by which a new key-value pair is added. An interesting aspect of insertion is that, unlike in Hash Maps, the keys are always sorted. This sorting facilitates efficient searching, which plays a pivotal role in the performance of applications needing ordered data access. The primary feature of insertion here is its logarithmic time complexity due to the underlying red-black tree structure.
This aspect of insertion is beneficial, especially when compared to simpler structures, where the lack of ordering can lead to longer access times. However, one unique characteristic is that if an attempt is made to insert a key that already exists, the value gets updated rather than creating a duplicate entry. This not only prevents clutter but also helps maintain data integrity.
In practice, a programmer might implement insertion as follows:
This example shows that the value for "apple" has been updated from 10 to 30.
Deletion
Deletion in Tree Maps involves removing existing key-value pairs and is fundamental to managing dynamic datasets. A characteristic feature of deletion is that it maintains the integrity of the tree structure; after deletion, the tree reorganizes itself to ensure proper balancing and order. This capacity to maintain structure aids applications requiring frequent updates.
What makes deletion favorable is its ability to run in logarithmic time as well, like insertion. However, has its intricacies. If a key-value pair is deleted, the balance of the Tree Map can be affected, necessitating the tree balancing. One downside might be that with frequent deletion operations, there's potential for overhead and increased memory consumption as the tree reorganizes.
An example of deletion:
Retrieval
Retrieval is the act of accessing the value associated with a specific key. Tree Maps shine in this aspect due to their sorted nature. The key characteristic of retrieval here is its efficiency; accessing a value by key remains at logarithmic time complexity.
This efficient retrieval process is invaluable, especially in use cases where fast lookups are critical. Whether querying for user data in a web application or fetching configurations in a system, Tree Maps enable speedy access to data without extensive searching.
Also, it is worth noting that since Tree Maps maintain order, one can easily retrieve ranges of keys, making this feature particularly useful.
For instance:
Advanced Operations
Now that we have covered the basic operations, let's delve into some advanced functionalities offered by Tree Maps.
Sub-maps
The concept of sub-maps refers to the ability to create a view of a portion of the Tree Map's data. This functionality is significant for applications needing to operate on a specific range of keys. The primary feature of sub-maps is that they provide an efficient means to work with segments of data without the overhead of copying data structures.
For example, suppose a user wants to fetch data in a specific range, say between "apple" and "mango". Sub-maps would allow this operation to happen in a streamlined manner:
This advanced operation can enhance efficiency in scenarios involving filtering and limiting access to data, making it a beneficial addition to Tree Map usage.
Navigable Map Interface
The Navigable Map interface extends the functionalities of the standard Map interface to offer navigation capabilities. It allows for operations that provide views based on a certain key order, enhancing the overall usability of Tree Maps. The distinct feature here is its support for methods like , , , and that allow developers to navigate quickly through the keys.
For instance, a developer can find the highest key less than or equal to a given key, which can be particularly useful in quantitative analyses or determining thresholds in datasets.
This gives programmers a robust mechanism to implement more complex data handling capabilities within their applications.
In terms of examples, consider:
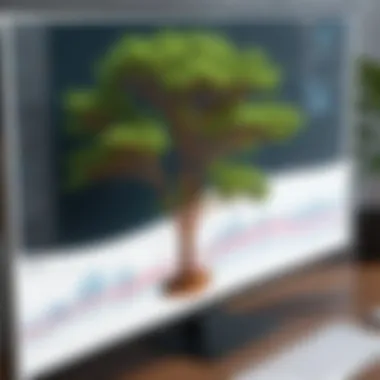
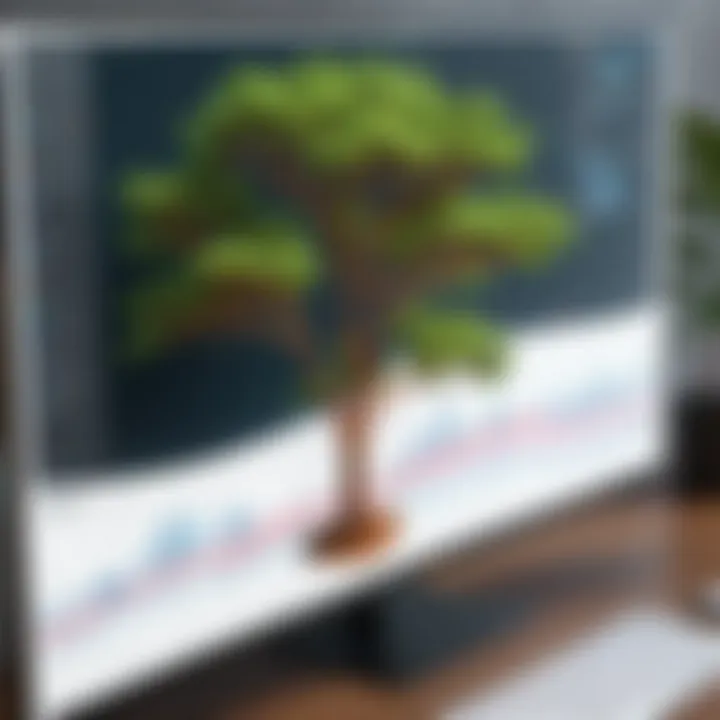
In summary, understanding basic and advanced operations of Tree Maps not only contributes to efficient programming but also enhances the flexibility of data management in Java. These insights facilitate effective implementation, supporting developers in building high-performing applications.
Performance Considerations
When working with Tree Maps in Java, understanding performance considerations is paramount. This plays a significant role in application efficiency and the overall user experience. Tree Maps, owing to their unique properties, offer performance trade-offs that make them suitable for specific scenarios. One critical aspect is the need for developers to be aware of the implications of time and space complexity. Knowing how these factors influence performance can lead to better decisions and optimizations in coding.
Time Complexity Analysis
Tree Maps provide a structure that inherently comes with trade-offs regarding time complexity. One of the most notable attributes of Tree Maps is their reliance on binary search trees, typically red-black trees. Here’s how the operations break down:
- Insertion: The average time complexity of inserting a key-value pair into a Tree Map is O(log n), where n is the number of elements in the map. This logarithmic time complexity stems from the tree's balanced structure, making it efficient to find the appropriate location for each new entry.
- Deletion: Similar to insertion, deletion also operates with a time complexity of O(log n). This matches well with the expectation of balanced structures, ensuring that even after an element is removed, the tree remains balanced and efficient.
- Lookup/Retrieval: When retrieving an element based on its key, the average time complexity remains at O(log n), providing a consistent and reliable access time regardless of the map's size.
The performance consistency of these operations assures developers that as data scales, efficiency is maintained without the performance pitfalls often seen in other implementations. However, care must be taken. For large datasets, the balance of the tree can impact time complexity, so it is crucial to monitor and maintain the tree structure.
Space Complexity Implications
Space complexity in Tree Maps is a consideration that can often get overlooked. The memory usage can impact the performance of an application, especially when working with large data sets. The space complexity of Tree Maps primarily involves:
- Storage of Nodes: Each element in the Tree Map is stored as a node, which carries not only the key and value but also pointers to the child nodes. Consequently, this overhead means that the space complexity is O(n), as each node contributes to the total memory usage corresponding to the number of entries in the map.
- Balancing Structures: The balancing mechanisms, like red-black trees, introduce additional overhead, slightly increasing the space required. This is not excessive but is something to keep in mind when designing memory-limited applications.
In general, while Tree Maps facilitate a stored order and quick access, their space efficiency matches the scale of performance. Therefore, understanding the interplay of time and space complexities is vital in implementing Tree Maps effectively. This ensures not just functional efficacy but operational efficiency in real-world applications.
Key Insight: Always balance between time efficiency and space usage when utilizing Tree Maps to get the most out of their structure without overloading your application.
Concurrency and Tree Maps
Concurrency in programming refers to the ability of multiple tasks to make progress within the same period of time. In the context of Java's Tree Maps, understanding concurrency is essential especially when dealing with multi-threaded environments. When several threads access a Tree Map simultaneously, it can lead to various issues such as data inconsistency and unexpected behavior. Hence, recognizing the intricacies of using Tree Maps in concurrent scenarios provides developers with the necessary tools to implement effective solutions.
Thread Safety Mechanisms
To ensure that a Tree Map is thread-safe, developers have several mechanisms at their disposal. These mechanisms aim to prevent multiple threads from altering the map simultaneously, leading to potential data corruption. One common approach is to use the method. Here’s a quick rundown of how this works:
- Wrap the Tree Map: By passing the Tree Map to the method, you create a synchronized view of the original map. This ensures that all operations on the map are thread-safe, as they will be executed one at a time, preventing any overlap that might cause issues.
- Synchronized Blocks: When performing multiple operations on the map that require checks or more than one update, using synchronized blocks might be more efficient. This way, a specific portion of code can be locked for a single thread at a time without blocking access to the entire map.
Example of Creating a Thread-Safe Tree Map
This example shows how to create a synchronized version of a Tree Map, which can be crucial for maintaining data integrity when multiple threads are at play.
Synchronization Strategies
While thread safety is critical, having synchronization strategies that strike a balance between safety and performance is equally important. Not every situation requires complete locking. Here are some strategies worth considering:
- Read-Write Locks: Using , allows multiple threads to read from the Tree Map concurrently while restricting updates to one thread at a time. This is particularly beneficial when read operations vastly outnumber write operations.
- Lock Striping: This strategy involves using multiple locks for different segments of the Tree Map. It reduces contention for the same resource, as different threads may work on separate portions of the map.
- Optimistic Locking: Instead of locking a resource for the duration of an operation, this approach checks for conflicts before committing changes. If a conflict is detected, the changes will be rolled back or retried.
Implementing the right synchronization strategy can greatly affect performance in a multi-threaded application. The right choice depends on your application's specific needs, including the ratio of read to write operations, expected contention among threads, and overall performance goals.
In a nutshell, effective concurrency management with Tree Maps goes beyond just thread safety. It demands tailored strategies that align with the requirements of the application, ensuring both data integrity and system responsiveness.
Best Practices for Using Tree Maps
When it comes to working with Tree Maps in Java, understanding the best practices cannot be stressed enough. Its design, which integrates a sorted navigable map interface, makes it an invaluable asset for various programming scenarios. Knowing how to harness the strengths of Tree Maps significantly influences both the efficiency of your code and the runtime performance of your applications.
To effectively utilize Tree Maps, one must consider several key elements. First and foremost is choosing optimal data types for keys and values, as this directly impacts your application's behavior when managing the collection. Then, employing custom comparators can lead to enhanced efficiency in searching and data retrieval operations.
"The essence of great programming lies in both the art of selection and the craft of arrangement."
Optimal Data Types for Keys and Values
The choice of data types for keys and values in a Tree Map is central to its implementation. Using the right types not only ensures clear logic but also impacts performance. For instance, if you're planning to sort a collection of user objects by their names, using a type for keys would be more intuitive than an value, which might lead to confusion and complications when natural ordering is essential.
Consider the following points when selecting data types:
- Simplicity: Opt for basic types or well-defined classes to avoid complications later.
- Immutability: Prefer immutable types for keys. Changing a key after it's been placed in a Tree Map can lead to inconsistencies and unpredictable behavior. For instance, using a class like is recommended as it cannot be altered once created.
- Natural Ordering: Understand that the default ordering relies on keys implementing the interface. Thus, ensure that the classes you use for keys are properly configured for comparison.
This thoughtful selection process lays the groundwork for a robust implementation and often results in fewer bugs during the application phase.
Custom Comparators for Enhanced Efficiency
While Tree Maps come equipped with natural ordering, there are cases where a more sophisticated approach is warranted. This is where custom comparators shine. By implementing the interface, you can dictate exactly how the keys are ordered within the Tree Map. This is crucial for applications dealing with diverse data types or specific sorting requirements.
Advantages of using custom comparators include:
- Flexible Sorting Orders: Sometimes, you might want to sort in descending order or based on specific attributes of an object. Custom comparators allow you to achieve this easily.
- Complex Object Comparison: If your keys are complex objects, a comparator can define how to compare different attributes instead of relying on the cumbersome and often confusing method of natural sorting.
- Performance Boost: In cases with large datasets, optimized comparators can streamline search operations and overall performance, potentially reducing time complexity in certain scenarios.
Here’s a small example of implementing a custom comparator:
In this example, we define a custom comparator that sorts the keys in reverse order, which can enhance the usability of the data structure for certain applications.
Integrating these best practices into your use of Tree Maps will help sculpt your data handling into something structured and efficient, enabling you to maintain performance while managing increasingly complex sets of information.
The End and Future Insights
As we draw our exploration of Tree Maps in Java to a close, this section emphasizes the significance of what we’ve covered and ponders on its future. Tree Maps offer a structured way to handle key-value pairs while maintaining order—an attribute that enhances data management in various applications. By mastering Tree Maps, developers can optimize their programs, leading to more efficient data processing and retrieval.
Recap of Key Points
In our comprehensive guide, we’ve touched on various essential aspects of Tree Maps. A quick review of the major points includes:
- Definition and Importance: Tree Maps are part of Java’s Collections Framework, providing an ordered representation of key-value pairs.
- Data Structure Understanding: We’ve detailed their workings, including the binary search tree concept and how balancing mechanisms, such as Red-Black Trees, play a vital role in their operations.
- Comparative Analysis: We’ve examined how Tree Maps stand against other implementations like Hash Maps, their advantages, and identified potential downsides.
- Practical Applications: Real-world use cases were highlighted, showcasing scenarios where Tree Maps prove to be especially beneficial.
- Concurrency Considerations: The presence of threading safety measures is crucial for modern applications, a point we thoroughly discussed.
- Best Practices: We also dove into optimal data types and the value of custom comparators in leveraging Tree Maps effectively.
Potential Developments in Tree Map Technology
The realm of programming is continuously evolving, and so are the data structures we utilize. Looking ahead, we can expect several exciting advancements in Tree Map technology:
- Integration with Machine Learning: As the demand for data-driven applications grows, merging Tree Maps with machine learning algorithms might offer hotly sought-after performance enhancements for real-time analytics.
- Improved Concurrency Models: Future versions may introduce even more efficient synchronization methods or advanced concurrency controls, making Tree Maps more appealing for multi-threaded applications.
- Enhanced User Customization: From their algorithms to the handling of complex data structures, we could see a rise in user-defined functions and flexibility in how Tree Maps operate, accommodating diverse needs across project specificaitons.
- Cross-Language Implementations: With such structure being highly applicable in different programming environments, the potential for similar data structures in other languages may influence how Tree Maps are designed and utilized.
"The future is not something we enter. The future is something we create."
- Leonard I. Sweet
In summary, Tree Maps in Java are not just a fundamental part of data structures but a pivotal subject worth constant exploration as new technologies surface and coding paradigms shift. As you delve deeper into the world of programming, understanding Tree Maps will undoubtedly serve as a valuable asset in your toolkit.