The Ultimate Guide to JavaScript Programming: Mastering Concepts, Functions, and Applications
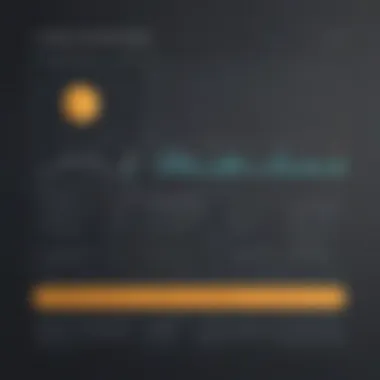
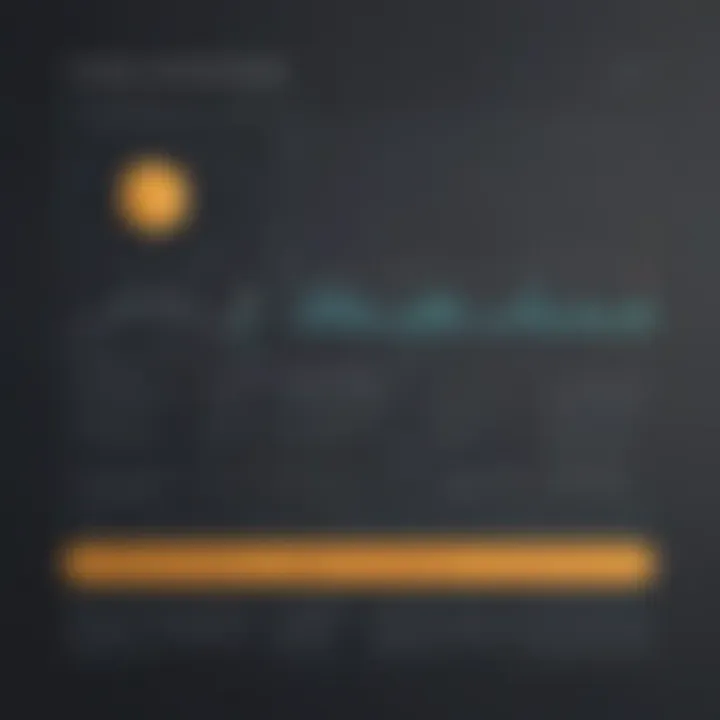
Fundamentals Uncovered
JavaScript is a powerful and versatile programming language that forms the backbone of many dynamic websites and web applications. Understanding the fundamental aspects of JavaScript is crucial for any aspiring developer or IT professional. By delving into the core principles and theories of JavaScript, individuals can grasp the essence of how this language operates within the digital realm. Key terminologies such as variables, functions, and loops play a pivotal role in comprehending JavaScript's foundational concepts and logic. Building a solid foundation in JavaScript entails mastering these basic concepts to navigate through more complex programming tasks with ease and precision.
Practical Implementations and Illustrative Scenarios
Immersing oneself in real-world case studies and practical examples is essential for honing JavaScript skills effectively. By exploring hands-on projects and dissecting code snippets, individuals can bridge the gap between theoretical knowledge and practical application. Demonstrations allow programmers to witness JavaScript's capabilities firsthand, enabling them to develop the intuition and proficiency needed for efficient coding practices. The implementation guidelines offered in this section provide clear, step-by-step instructions to assist readers in applying JavaScript concepts to their own projects, fostering a deeper understanding of the language's versatility and functionality.
Advanced Exploration and Emerging Trends
As technology evolves rapidly, staying abreast of advanced topics and cutting-edge trends in the JavaScript landscape is paramount for professional growth. This section delves into the latest developments in JavaScript, offering insights into advanced techniques and methodologies utilized by industry experts. Discovering future prospects and upcoming trends not only enriches one's knowledge base but also encourages experimentation and innovation within the programming sphere. By immersing oneself in the forefront of JavaScript evolution, individuals can broaden their skill set and adapt to the ever-changing demands of the tech industry.
Tips and Valuable Resources for Continued Learning
Seeking avenues for continuous education and improvement is key to mastering JavaScript. This section provides a curated list of recommended books, courses, and online resources designed to enhance readers' proficiency in JavaScript programming. Exploring supplementary tools and software applications can further aid individuals in their coding endeavors, providing practical solutions to common programming challenges. By leveraging these resources effectively, aspiring developers can embark on a journey of lifelong learning and skill enhancement, propelling their programming career to new heights.
Introduction to JavaScript
Understanding the Basics
History of JavaScript
JavaScript's history is a rich tapestry woven with innovation and creativity. Originally developed by Brendan Eich in 1995, JavaScript has evolved into a powerhouse language that drives the modern web. Its legacy of being the first language to enable dynamic content on websites remains unmatched. The history of JavaScript is intertwined with the evolution of internet technology, making it a trailblazer in the realm of programming languages. Its adaptability and compatibility across different platforms make it a popular choice for this article, where we delve deeper into its roots and significance.
Key Features of JavaScript
The key features of JavaScript are like building blocks that form the backbone of its functionality. From its lightweight and interpreted nature to its support for object-oriented programming, JavaScript offers a host of benefits that set it apart from other languages. Its dynamic typing and versatility in handling different data types make it a preferred choice for developing interactive web applications seamlessly. Exploring the key features of JavaScript in this article will shed light on why it is a powerhouse language in the world of web development.
Setting Up Development Environment
Setting up a robust development environment is crucial for harnessing the full potential of JavaScript. Whether choosing an Integrated Development Environment (IDE) or opting for a text editor with plugins, the development environment plays a vital role in enhancing productivity and efficiency. Configuring tools like Git for version control and Node.js for backend development sets the stage for seamless coding experiences. Although it may have its learning curve, the setup of a development environment proves to be a beneficial choice for this article, empowering readers to kickstart their JavaScript journey on the right foot.
Advanced JavaScript Techniques
Functions and Scope
Closures and Callbacks
Exploring closures and callbacks within JavaScript sheds light on the inner workings of the language. Closures, a fundamental concept, provide a powerful way to encapsulate variables and preserve their values within functions. This feature contributes significantly to maintaining data integrity and enhancing code security. Additionally, callbacks play a vital role in asynchronous operations, allowing functions to execute once certain tasks are completed. Their asynchronous nature enables seamless handling of time-intensive processes, enhancing overall program efficiency.
Arrow Functions
The introduction of arrow functions has revolutionized JavaScript syntax, offering a concise and elegant approach to function declaration. These functions provide a more streamlined way to write function expressions, reducing unnecessary verbosity and enhancing code readability. Their lexically scoped 'this' keyword simplifies context binding, making them a preferred choice for developers aiming for cleaner and more maintainable code bases.
Higher-Order Functions
Embracing higher-order functions amplifies the functional programming paradigm within JavaScript. These functions either accept functions as arguments or return functions, enabling developers to leverage the power of first-class functions. By facilitating code reusability and promoting modular design, higher-order functions enhance the flexibility and scalability of JavaScript applications. Their ability to abstract common patterns simplifies complex algorithms and promotes code efficiency across projects.
Object-Oriented Programming
Classes and Prototypes
Classes and prototypes constitute the building blocks of JavaScript's OOP model, facilitating the creation of blueprints for object instances. Classes offer a blueprint for creating objects with predefined properties and methods, promoting code reusability and organization. On the other hand, prototypes allow objects to inherit properties and behaviors from other objects, establishing a hierarchical structure for efficient code management.
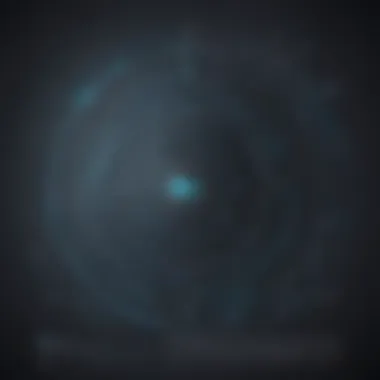
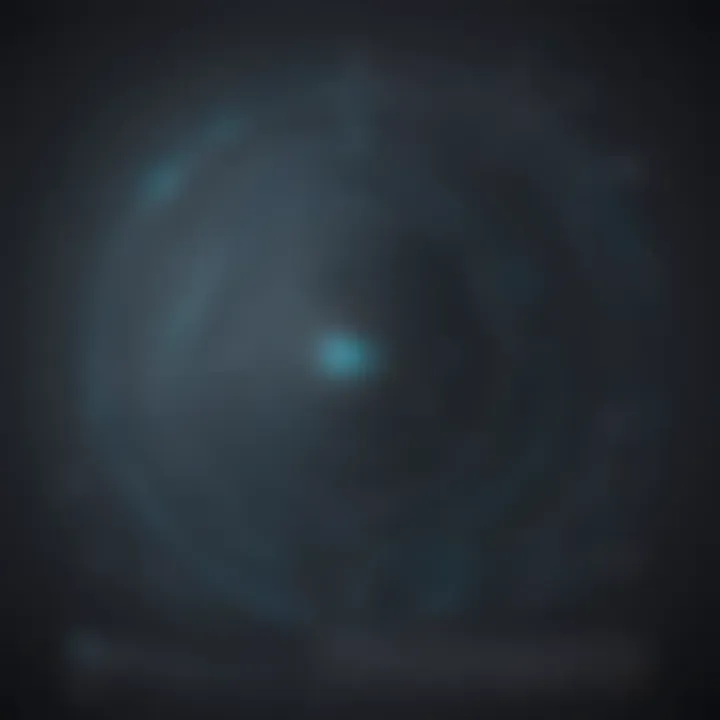
Inheritance and Polymorphism
Inheritance and polymorphism are key concepts within OOP that foster code reuse and flexibility. Inheritance enables objects to acquire properties and methods from parent objects, promoting a hierarchical structure and reducing code duplication. Polymorphism, on the other hand, allows objects to exhibit different behaviors based on their specific implementations, enhancing code adaptability and promoting modular design.
Encapsulation
Encapsulation encapsulates data and methods within a defined boundary, shielding internal complexities from external manipulation. This concept fosters data integrity, promoting information hiding and enhancing code security. By encapsulating related functionalities within objects, developers can achieve greater control over data access and manipulation, leading to more robust and maintainable code bases.
DOM Manipulation and Event Handling
Interacting with the DOM
Selecting Elements
Selecting Elements is a fundamental aspect of DOM manipulation. It involves targeting specific elements within the DOM hierarchy to retrieve, modify, or manipulate their properties. The process of selecting elements allows developers to access and interact with various parts of the webpage, such as buttons, forms, text, and more. By understanding how to select elements effectively, developers can streamline their coding process, improve performance, and create engaging user interfaces.
Modifying Element Attributes
Modifying Element Attributes plays a key role in altering the visual and functional characteristics of DOM elements. By changing attributes like color, size, visibility, or behavior, developers can dynamically update the appearance and behavior of elements on the webpage. This ability to customize element attributes dynamically enhances the interactivity and aesthetics of web applications, leading to a more engaging user experience.
Creating and Removing Elements
Creating and Removing Elements involves dynamically adding or deleting elements within the DOM structure. This process is essential for generating content on the fly, such as inserting new paragraphs, images, or buttons based on user interactions. By dynamically creating and removing elements, developers can update the webpage content in real-time, providing a seamless and interactive browsing experience for users.
Event Handling
Event Handling is a critical aspect of JavaScript programming that enables developers to respond to user interactions and trigger appropriate actions. By attaching event listeners to DOM elements, developers can detect user actions like clicks, keypresses, or mouse movements and execute corresponding functions. Event Handling facilitates the creation of interactive web applications by enabling developers to capture and process user input effectively.
Event Listeners
Event Listeners are mechanisms in JavaScript that listen for specific events on DOM elements and trigger callback functions when those events occur. By utilizing event listeners, developers can build interactive functionalities like buttons that respond to clicks, forms that validate user input, or sliders that adjust content based on user actions. Event Listeners enhance user engagement and interactivity by enabling responsive behaviors in web applications.
Event Propagation
Event Propagation refers to the mechanism by which browser events are handled and propagated through the DOM hierarchy. Understanding event propagation is crucial for managing event flow and ensuring that event handlers at different levels of the DOM structure function as intended. By comprehending event propagation, developers can control how events are handled, prevent conflicts, and create more robust event-driven applications.
Event Delegation
Event Delegation is a design pattern in JavaScript that allows developers to handle events efficiently on multiple elements with a single event listener. Instead of attaching event listeners to individual elements, developers can delegate event handling to a common ancestor element. Event delegation simplifies event management, improves performance, and reduces code complexity in scenarios where multiple elements share similar event behaviors.
Asynchronous JavaScript
In the fast-paced world of web development, Asynchronous JavaScript plays a pivotal role. Understanding the importance of Asynchronous JavaScript is crucial for any programmer striving to build efficient and responsive web applications. By allowing tasks to run independently without waiting for others to finish, Asynchronous JavaScript enhances the overall user experience. It enables handling multiple operations simultaneously, ensuring that the application remains dynamic and interactive. Embracing Asynchronous JavaScript in this article empowers readers to explore the realm of non-blocking code execution and maximize the performance of their JavaScript applications.
Promises and AsyncAwait
Working with Promises
Working with Promises is a fundamental aspect of modern JavaScript development. Promises simplify asynchronous operations by providing a cleaner and more structured way to handle asynchronous code. The key characteristic of Promises is their ability to represent a value that may produce a result in the future, whether resolved or rejected. This feature makes Promises a popular choice in this article because they offer a clear and concise method for managing asynchronous tasks. While Promises streamline error handling and offer improved readability, developers must be cautious of potential nesting complexities that could occur when chaining multiple Promises.
Asynchronous Functions
Asynchronous Functions are essential for executing tasks concurrently in JavaScript applications. Their key characteristic lies in their ability to initiate multiple tasks without blocking the main thread. This asynchronous approach enhances efficiency by preventing processes from halting while waiting for others to complete. By leveraging Asynchronous Functions in this article, readers can grasp the power of parallel execution and write code that responds swiftly to user interactions. However, developers should exercise caution to avoid callback hells and ensure proper error handling to maintain code integrity.
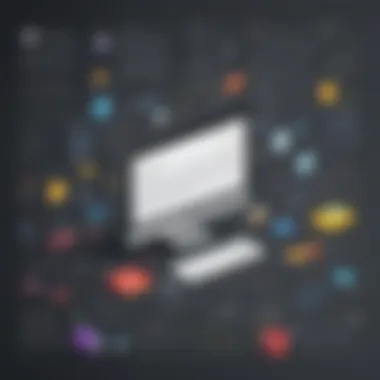
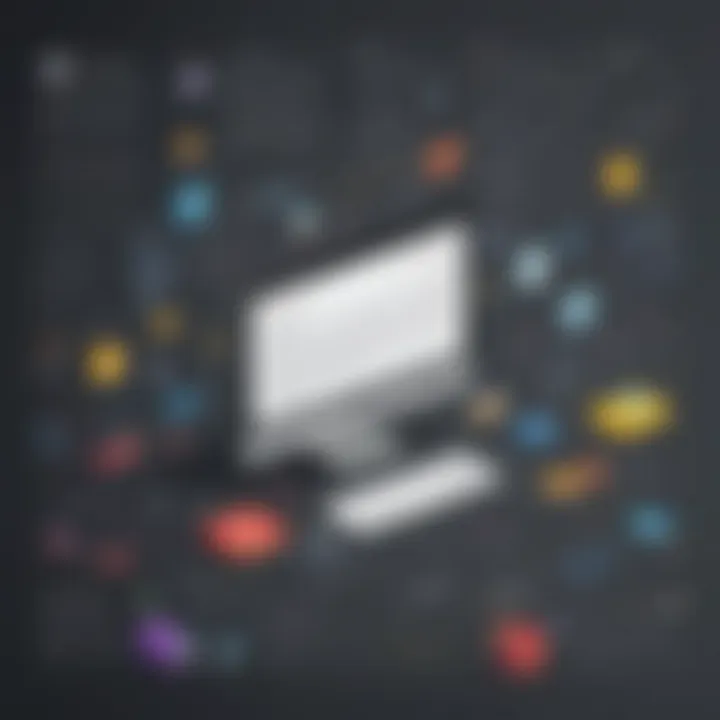
Error Handling
Error Handling is a critical component of asynchronous JavaScript programming. Effectively managing errors can prevent application crashes and enhance the overall user experience. The key characteristic of robust error handling lies in promptly identifying and resolving issues that arise during asynchronous tasks. By focusing on meticulous error handling in this article, readers gain insights into ensuring code reliability and resilience. While proficient error handling is beneficial for debugging and maintaining code quality, overcomplicating error management could lead to code bloat and impact performance negatively.
AJAX and Fetch API
Making API Requests
Making API Requests is a common task in modern web development that Fetch API simplifies significantly. The key characteristic of making API requests lies in the ability to retrieve data from servers asynchronously without reloading the page. This feature promotes dynamic content updates and enhances the responsiveness of web applications. By incorporating Making API Requests in this article, readers can master the art of fetching data seamlessly and integrate external services with confidence. Nevertheless, developers should consider security measures such as handling tokens and implementing proper API request configurations to mitigate potential vulnerabilities.
Handling Responses
Handling Responses efficiently is vital for processing data retrieved from API requests. The key characteristic of response handling involves parsing and extracting relevant information from the received response. This ensures that data is utilized effectively within the application, enhancing user interactions. By emphasizing proper response handling in this article, readers can smoothly manage API data and present it in a structured format. However, developers must be attentive to error responses and edge cases to maintain application robustness and data consistency.
Data Serialization
Data Serialization is instrumental in converting complex data structures into a format that can be transmitted and stored easily. The key characteristic of data serialization lies in its role in maintaining data integrity during communication between client and server. By delving into Data Serialization in this article, readers can harness the power of structured data interchange and build scalable applications. Despite its advantages in facilitating data communication, improper data serialization could lead to security vulnerabilities such as injection attacks and data exposure. Thus, developers must exercise caution and implement secure serialization techniques for enhanced application security and resilience.
JavaScript Libraries and Frameworks
JavaScript libraries and frameworks play a pivotal role in modern web development, enhancing efficiency, scalability, and overall code quality. By leveraging these tools, developers can streamline the development process, reduce redundant tasks, and benefit from pre-built functionalities. When integrated thoughtfully, libraries and frameworks can significantly boost productivity and code maintainability.
Popular Libraries
jQuery
jQuery revolutionized front-end development by simplifying DOM manipulation and event handling. Its concise syntax and cross-browser compatibility make it a preferred choice for many developers. With extensive documentation and a large community, jQuery offers a robust ecosystem for creating interactive web applications. However, its dependency on older JavaScript practices and potential performance overhead are factors to consider when choosing it for a project.
lodash
lodash is a utility library that provides a wide range of functions for manipulating arrays, objects, and strings efficiently. Its modular design allows developers to include only necessary functions, reducing the bundle size. lodash's functional programming paradigm promotes code readability and maintainability. Despite its benefits, developers need to be cautious of introducing unnecessary abstraction layers that could impact performance.
React
As a popular JavaScript library for building user interfaces, React offers a component-based architecture that promotes code reusability and maintainability. Its virtual DOM implementation improves rendering efficiency, leading to better performance in complex applications. React's declarative approach simplifies state management and allows for easy integration with other libraries. Despite its steep learning curve for beginners, React's benefits in creating dynamic and interactive UIs are widely recognized.
Frameworks
Angular
Angular, developed by Google, is a comprehensive front-end framework that excels in building single-page applications. Its two-way data binding and dependency injection system simplify the development process by reducing boilerplate code. Angular's powerful CLI automates project setup and enhances productivity. However, the complexity of Angular's architecture and terminology can pose challenges for newcomers, requiring a significant learning curve.
Vue.js
Vue.js is a progressive JavaScript framework known for its simplicity and flexibility. With detailed documentation and a gentle learning curve, Vue.js appeals to developers of all skill levels. Its reactivity system ensures efficient updates to the DOM, enhancing performance in real-time applications. Vue's ecosystem of plugins and tools offers additional functionality and customization options. However, Vue's smaller community compared to React and Angular may affect the availability of resources and support.
Node.js
Node.js enables server-side JavaScript execution, allowing developers to build scalable and efficient back-end systems. Its event-driven architecture and non-blocking IO operations enhance performance for handling concurrent requests. Node.js's extensive package ecosystem through npm provides a wealth of libraries for diverse functionalities. However, managing asynchronous operations and ensuring application stability in a single-threaded environment require careful consideration when using Node.js for server-side development.
JavaScript Best Practices
JavaScript Best Practices are essential for any programmer looking to optimize their code. In this article, we delve into the importance of adhering to best practices when writing JavaScript code. By following these practices, programmers can enhance code readability, maintainability, and performance. Not only do best practices streamline the development process, but they also ensure consistency across projects, making collaboration easier and code more robust. Whether it's code optimization, error handling, or debugging, incorporating best practices is paramount for crafting efficient and reliable JavaScript applications.
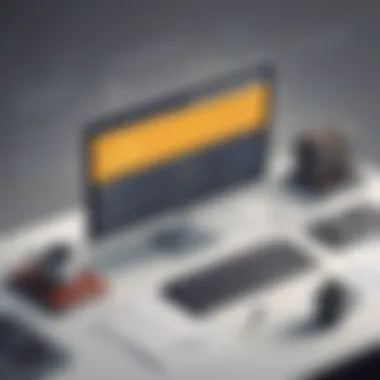
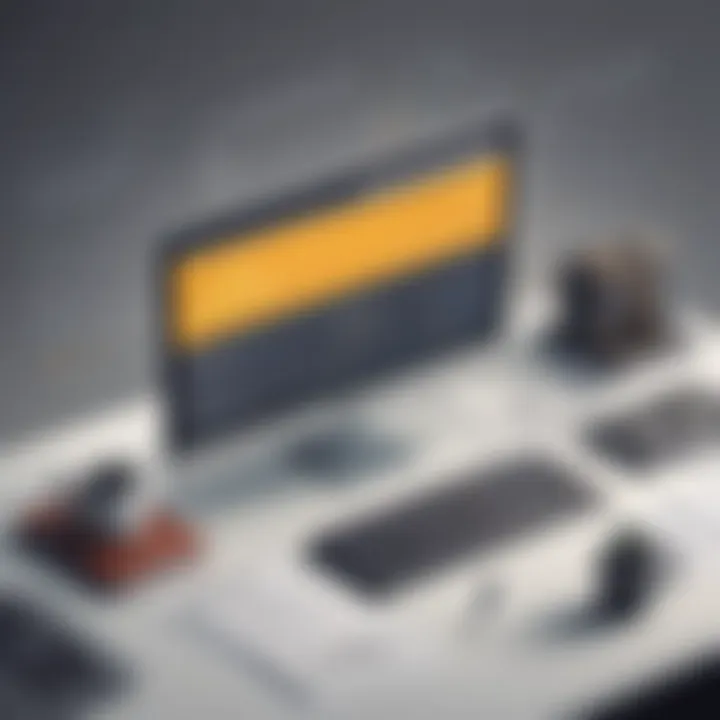
Code Optimization
Minification
Minification is a crucial aspect of code optimization in JavaScript. This process involves reducing the size of code files by removing unnecessary characters like white spaces, comments, and renaming variables to shorter names. By minimizing code size, minification improves website loading times and overall performance. It is a popular choice in this article due to its ability to enhance user experience by accelerating page load times. Despite its benefits, minification may pose challenges during debugging, as the code becomes less human-readable. However, leveraging tools like minifiers can mitigate this issue, making minification an advantageous practice in JavaScript development.
Bundling and Transpilation
Bundling and transpilation play a pivotal role in code optimization for JavaScript projects. Bundling consolidates multiple files into one bundle, reducing the number of server requests and improving load times. Transpilation, on the other hand, converts modern ECMAScript code into a backward-compatible version to ensure cross-browser compatibility. This practice is vital in our article as it streamlines the deployment process and enhances code efficiency. While bundling and transpilation offer various benefits, they can sometimes introduce complexities in managing dependencies and configurations. Nonetheless, with proper tooling and configuration, bundling and transpilation significantly contribute to optimizing JavaScript code.
Code Splitting
Code splitting is another crucial aspect of code optimization that focuses on dividing code into smaller chunks or modules. This practice enhances page load times by loading only necessary code components, resulting in a faster initial page rendering. In this article, code splitting is highlighted for its ability to improve application performance and user experience by loading specific code segments when required. However, code splitting may lead to increased network requests, which could affect loading times if not managed effectively. By strategically implementing code splitting and utilizing techniques like lazy loading, developers can strike a balance between performance optimization and code organization in JavaScript projects.
Error Handling and Debugging
Try-Catch Statements
Try-catch statements are indispensable tools in JavaScript for handling errors gracefully and preventing abrupt program termination. By encapsulating potentially error-prone code within a try block and defining error-handling logic in the catch block, programmers can control how errors are managed within their applications. In this article, try-catch statements are emphasized for their role in maintaining application stability and guiding error flow. While try-catch blocks mitigate the risk of runtime errors, overusing them can lead to performance overhead. Therefore, it is essential to judiciously apply try-catch statements where necessary to strike a balance between error-handling and code efficiency.
Console Logging
Console logging is a fundamental debugging technique in JavaScript that allows developers to print information to the browser console for analysis. By strategically placing log statements in code, programmers can track the flow of execution, inspect variable values, and identify potential bugs or issues. This article underscores the significance of console logging in facilitating the debugging process and gaining insights into code behavior. Although console logging offers invaluable assistance in debugging, excessive logging can clutter the console output, making it challenging to isolate relevant information. Therefore, developers should employ console logging judiciously to extract meaningful debugging details while maintaining code clarity.
Debugging Tools
Debugging tools are software applications or features that assist developers in identifying and resolving errors in their code efficiently. In JavaScript development, tools like browser Developer Tools, IDE debuggers, and linters play a vital role in diagnosing issues, profiling performance, and optimizing code. This article emphasizes the importance of utilizing debugging tools to streamline the development process and enhance code quality. While debugging tools offer comprehensive capabilities for error identification, improper usage or reliance solely on tools can hinder developers from honing their debugging skills and understanding code intricacies. Therefore, integrating debugging tools judiciously alongside manual debugging practices is crucial for comprehensive error handling and code optimization in JavaScript projects.
Conclusion
In the realm of JavaScript learning, the concluding segment is of paramount significance as it encapsulates the essential takeaways and highlights the practical implications of the preceding sections. The Conclusion acts as a gateway for readers to synthesize their newfound knowledge and apply it effectively. By emphasizing the pivotal areas covered throughout the article, such as basic syntax, advanced functions, and practical implementations, learners can solidify their understanding of JavaScript and harness its capabilities.
Key Takeaways
Mastering JavaScript Essentials
When delving into the realm of Mastering JavaScript Essentials, learners are poised to grasp the foundational pillars of this programming language. This section molds the readers' comprehension of essential JavaScript concepts, equipping them with the tools necessary to navigate through complex coding tasks effortlessly. The seamless integration of variables, functions, and control structures not only furthers one's programming prowess but also instills a sense of confidence in tackling intricate coding challenges. Mastering JavaScript Essentials serves as a cornerstone for any aspiring programmer looking to fortify their coding acumen and delve into the realm of sophisticated software development.
Exploring Advanced Concepts
Exploring Advanced Concepts sheds light on the intricate facets of JavaScript that propel learners towards innovation and mastery. This section delves into the nuances of closures, prototypes, and object-oriented programming paradigms, allowing readers to transcend conventional coding boundaries. By unraveling the intricacies of advanced JavaScript functionalities, individuals can broaden their programming repertoire and craft solutions that harmonize efficiency and elegance. Exploring Advanced Concepts cultivates a mindset of continuous evolution, urging programmers to push the boundaries of conventional programming and explore uncharted territories within the JavaScript landscape.
Practical Application in Projects
The Practical Application in Projects section serves as a crucible where theoretical knowledge converges with real-world implementation. Here, readers are encouraged to translate their theoretical understanding of JavaScript into practical solutions that cater to contemporary software development needs. By engaging in hands-on projects, individuals can hone their problem-solving skills, refine their coding style, and elevate their expertise to new heights. The practical application not only reinforces conceptual learning but also instills a sense of accomplishment as individuals witness their coding prowess manifest in tangible outputs.
Continued Learning
Further Resources
The section on Further Resources acts as a treasure trove of additional learning materials, guiding readers towards supplementary sources that enrich their JavaScript journey. By tapping into curated resources, individuals can expand their knowledge base, stay abreast of industry trends, and enhance their programming proficiency. From online tutorials to specialized JavaScript forums, Further Resources paves the way for continuous learning and professional growth within the dynamic realm of JavaScript.
Project Ideas
Embracing the Project Ideas section propels readers towards a realm of creativity and innovation, offering a plethora of potential projects to sharpen one's programming skills. By embarking on diverse coding projects, individuals can experiment with new concepts, troubleshoot challenging scenarios, and cultivate a portfolio that showcases their coding versatility. Project Ideas instigate a spirit of exploration and creativity, urging programmers to embark on coding escapades that not only hone their skills but also spark ingenuity in problem-solving and software development.
Community Involvement
Community Involvement serves as a pillar of support and collaboration within the expansive realm of JavaScript programming. By engaging with like-minded individuals, learners can partake in knowledge-sharing sessions, seek mentorship from seasoned developers, and contribute to the collective growth of the JavaScript community. The collaborative ethos embedded within Community Involvement fosters a culture of camaraderie and exchange, where programmers can glean insights, share experiences, and forge lasting connections that nurture their professional development in the ever-evolving landscape of JavaScript.