Understanding Android Activity: Comprehensive Guide
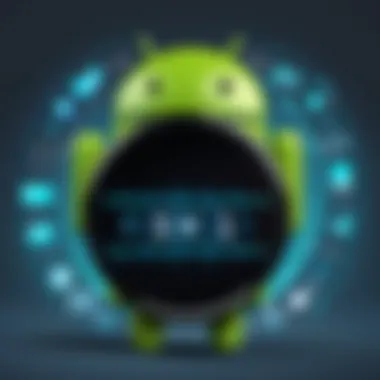
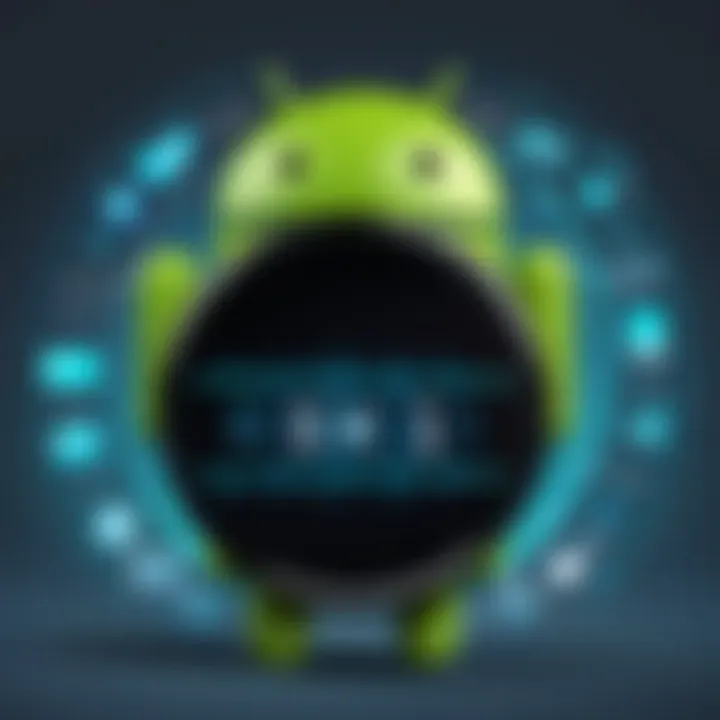
Overview of Topic
Intro to the Main Concept Covered
Android Activity is a fundamental building block in the Android operating system, acting as an interface for user interaction. Whether users engage with a single screen or navigate through multiple views, activities dictate how users experience applications. From managing UI components to processing user input, understanding activities is central to proficient Android development.
Scope and Significance in the Tech Industry
The significance of Android Activity extends beyond basic application development. With Android being one of the most widely used mobile operating systems globally, mastering activity management can elevate a developer's skillset. Efficient handling of activities can lead to better user experiences, directly impacting app success in terms of user retention and engagement.
Brief History and Evolution
Since the introduction of Android in 2008, activities have evolved substantially. Initially, activities were fairly simple, but as applications grew in complexity, so did the architecture surrounding them. The Android framework has added layers such as fragments and intents that facilitate smooth transitions and multi-screen applications, illustrating the dynamic nature of Android development.
Fundamentals Explained
Core Principles and Theories Related to the Topic
At the heart of Android activities are key concepts: lifecycle management and UI manipulation. Understanding how to effectively manage the lifecycle of an activity ensures optimal performance and memory usage, preventing crashes and providing a seamless user experience.
Key Terminology and Definitions
Some essential terms associated with Android Activity include:
- Lifecycle: The stages an activity undergoes, impacting its availability to the user.
- Intent: A message to initiate actions, such as starting activities or communicating between components.
- Fragment: A reusable component of an activity's UI, enabling flexible user interface design.
Basic Concepts and Foundational Knowledge
Grasping fundamental concepts serves as a foundation. Each activity in an Android application can manage its state changes through various lifecycle methods like , , and . Ignoring these intricacies can lead to applications that are sluggish or unresponsive, demonstrating the necessity of comprehending the entire activity lifecycle chain.
Practical Applications and Examples
Real-world Case Studies and Applications
Many successful applications leveraging Android Activity include popular navigation apps and social media platforms. For instance, Ubereats utilizes activities to navigate between order selection, delivery tracking, and user profile management.
Demonstrations and Hands-on Projects
Participants could benefit from practical demonstrations. Developing a basic 'Hello World' app using activities is a standard starting point, allowing learners to visualize how activity interactions are structured.
Code Snippets and Implementation Guidelines
A simple way to create your first activity looks like this:
Advanced Topics and Latest Trends
Cutting-edge Developments in the Field
As Android continues evolving, Jetpack Compose introduces new ways to handle UI without the complications of XML layouts. Understanding how activities work within Compose enhances a developer's toolkit significantly.
Advanced Techniques and Methodologies
Adopting patterns such as the Model-View-ViewModel (MVVM) architecture can help streamline event handling between activities and UI. This separation leads to cleaner and more maintainable codebases.
Future Prospects and Upcoming Trends
Noteworthy advancements include enhancing user experience through artificial intelligence and machine learning integrated into activities, making applications smart and interactive.
Tips and Resources for Further Learning
Recommended Books, Courses, and Online Resources
Finding the right educational material can facilitate better understanding. Notable resources include:
- Android Programming: The Big Nerd Ranch Guide (book)
- Developing Android Apps with Kotlin (course on Udacity)
- Online forums like reddit.com for community-driven insights.
Tools and Software for Practical Usage
Software like Android Studio is essential for developing and managing activities. Its built-in tools simplify UI design and code writing, making development efficient and intuitive.
To master Android activity management requires dedication and practice. Embracing continuous learning can greatly enhance your capabilities as a developer.
Prelims to Android Activity
Definition of Android Activity
In the realm of Android development, an Activity serves as a fundamental building block for creating a user interface. An Activity represents a single screen within an application, allowing users to interact with various features and functionalities. It acts as a tangible interface that shows elements like buttons, text views, and images. By managing the lifecycle and behavior related to user interactions, activities are crucial for providing a consistent experience across different devices and resolutions.
Importance of Activities in Android Development
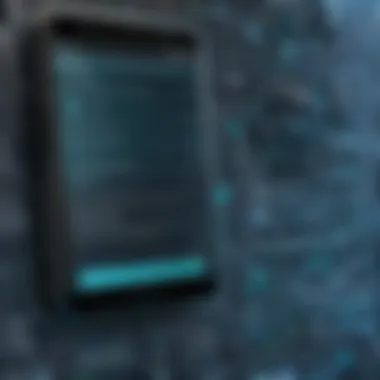
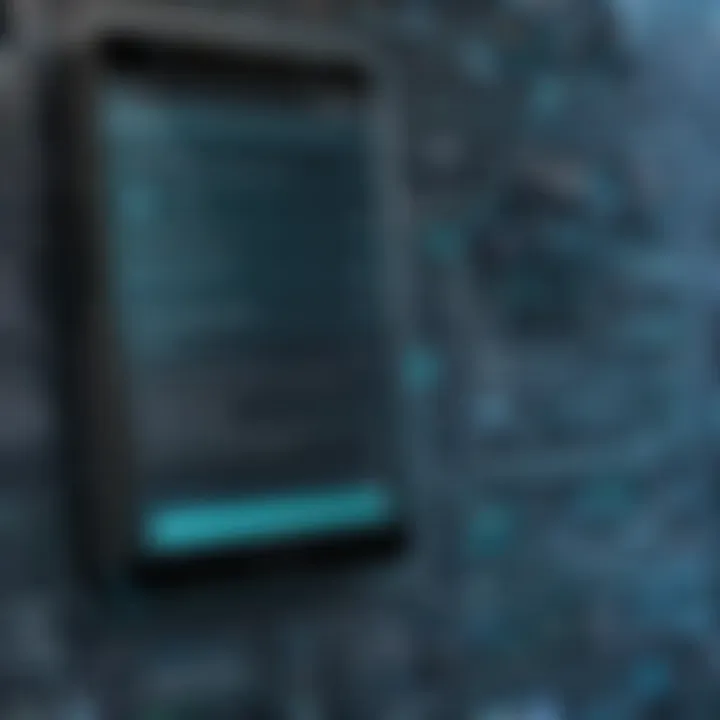
Activities are vital for the overall structure of Android applications. They enable developers to create responsive user interfaces and manage user interactions efficiently. These components play an essential role by:
- Encapsulating UI Logic: Each Activity can showcase distinct UI routines, allowing developers to organize their code better. This separation enhances readability.
- Managing State: Activities help in preserving the state when navigating between different screens by communicating necessary contextual information.
- Supporting User Input: Direct user interactions occur within activities. They can handle touch inputs, swipes, and gestures, making them central to usability.
- Facilitating Intent Navigation: Activities interact seamlessly using intents, which can launch other activities, creating a dynamical user experience.
Activities in Android provide a structured approach for designing workflows that directly engage users. Understanding their role is essential for effective app development.
In summary, activities offer a foundation that underpins applications, influencing their functionality and user experience. Over the following sections, we will dive deeper into the lifecycle of activities and other components interlinked with Android activities.
Lifecycle of an Android Activity
The lifecycle of an Android Activity is crucial for developing robust and user-friendly applications. Understanding how activities transition through various states allows developers to manage resources effectively and maintain a smooth user experience. Activities are the entry points for user interaction in Android, so it is essential to grasp their lifecycle methods. This section will delve into specific elements, benefits, and the nuances of the activity lifecycle to ensure better application performance and user satisfaction.
Understanding Activity Lifecycle Methods
Activity lifecycle methods are a set of callbacks that inform the application about its current state. The major lifecycle methods are:
- : This is the first method called when an activity is created. Initial setup, such as loading the user interface, occurs here.
- : The activity becomes visible to the user but is not yet in the foreground. This method is a good place to start animations or refresh UI components.
- : The activity is now in the foreground and user interaction is possible. Any functionality required for interaction should be prepared in this callback.
- : The activity is partially obscured, typically when a new activity is starting. This is a good time to pause processes that should not run when the activity is not interactive.
- : The activity is no longer visible. Usually, resources that are not needed can be released at this stage.
- : This method is called just before the activity is destroyed. Cleanup tasks and saving application data should be performed here.
Understanding these methods in detail enables developers to manage the resources better, handle potential leaks, and deliver an efficient application that adapts to users' actions.
State Transitions in an Activity
State transitions from one lifecycle state to another can be unpredictable, making it essential for developers to build with this in mind. Several transitions common occur:
- From Created to Started: Invoked when the activity is brought from behind the scenes to the foreground.
- From Started to Resumed: Typically happens when a new activity is opened.
- From Resumed to Paused: Occurs when the user navigates away from the activity temporarily.
- From Paused to Stopped: Happens when the activity is no longer visible.
- From Stopped to Destroyed: The final state as the activity is terminated or the application process is finished.
Understanding these transitions prevents potential issues, such as memory leaks or unnecessary resource consumption. Designers should plan functionality that responds to these states efficiently, ensuring that ongoing tasks are halted or adjusted correctly without impacting performance.
Handling Configuration Changes
Android devices will often change configuration based on various factors, such as screen orientation or hardware keyboards being attached. These configuration changes can disrupt activity lifecycles. Here are some strategies for managing them:
- Override methods: Use to respond to specific configuration changes without restarting the activity.
- Save state: Once in , it is recommended to save the current UI state using , to restore the state when returning to this activity later.
- Manifest declarations: Customize the behavior of your activity in the AndroidManifest.xml file by using the attribute to detect changes and avoid the activity from restarting, depending on the specific requirement.
Handling these configuration changes is vital for a seamless user experience. Proper management ensures users can interact with applications reliably across different scenarios.
Activities and User Interaction
Understanding activities in the context of user interaction is crucial for creating effective Android applications. Activities serve as the primary interface through which users engage with the app's functionality. They shape the user experience, dictating how users interact with content, make selections, and navigate within the app. A well-designed activity can significantly enhance usability and overall satisfaction, while a poorly constructed one can lead to confusion and frustration. Therefore, knowing how to create interfaces that are intuitive and responsive to user inputs is essential for developers.
Creating an Activity Layout
Creating an activity layout involves structuring the visual components of an activity. This layout serves as the canvas for displaying user interface elements such as buttons, text fields, images, and menus. Using the Android framework, developers can specify the arrangement of these elements through dynamic layouts or static XML files. When designing layouts, it is crucial to consider factors such as screen size, orientation, and device capabilities. Here are some of the core components commonly utilized in layout creation:
- LinearLayout: Arranges children in a single column or row.
- RelativeLayout: Allows building composite layouts based on the relative positions of child views.
- ConstraintLayout: Offers flexible positioning of views by defining constraints.
For instance, XML can be utilized to define a simple activity layout like so:
This example presents a vertical layout containing a welcoming text and a button, establishing a fundamental structure for user interaction.
Designing User Interfaces with
User interface design in Android often employs XML, which allows developers to define layouts in a separate file, ensuring a clear separation between visual presentation and logic. By utilizing XML for interface design, developers can create reusable components and maintain consistency across various parts of the application. Additionally, XML facilitates the automatic adaptation of user interfaces for multiple screen sizes and densities, which is informative in today's diverse device ecosystem.
The straightforward declaration of interface elements in XML enables quicker iterations during the design phase. Incorporating styles and themes becomes hassle-free. One can easily apply attributes across many layouts, promoting a cohesive look and feel within the app. Moreover, using the layout editor available in Android Studio can help visualize how these UI components will appear in the app, providing immediate feedback and adjustments.
Responding to User Inputs
User inputs drive interaction within Android applications, and effectively responding to these inputs is fundamental for a positive user experience. Activities must be designed to listen and react to different types of user interactions, such as taps, swipes, or gestures. OnClickListeners are integral to this process, as they allow applications to define actions triggered by user actions on UI elements.
Here are a few common events that developers should handle:
- Button Clicks: Respond to user taps to initiate actions.
- Text Input: Capture user-entered data for processing.
- Gestures: Register complex interactions like swipes or pinches to enable features.
An example implementation for handling button clicks in an activity could look like this:
In this code snippet, clicking the startButton triggers an intent to open the next activity, demonstrating a direct response to user input. Crafting effective interactions not only enrich users' experience but also ensures that the application feels responsive and engaging.
Effective user interaction is paramount. Thoughtful UI design aligned with seamless input response helps retain user focus and engagement.
In summary, understanding how to create activity layouts, design user interfaces using XML, and respond to user inputs is essential for developers. These factors contribute significantly to the overall user experience and ultimately determine the quality of the application.
Intents and Activity Communication
Intents are central to the communication between components in an Android application. They serve as messengers, facilitating interactions and data exchange. Understanding intents is crucial for developers because they enable various functionalities within an app, like starting new activities and broadcasting messages.
Using intents properly can enhance user experience by promoting seamless transitions between activities. With the knowledge of how to manipulate intents, developers can create more responsive apps. This section will delve into the various facets of intents, focusing on what they are, how they are used, and how they facilitate communication between activities.
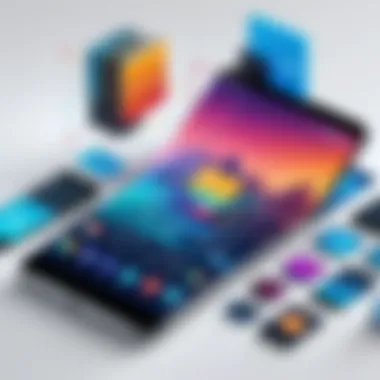
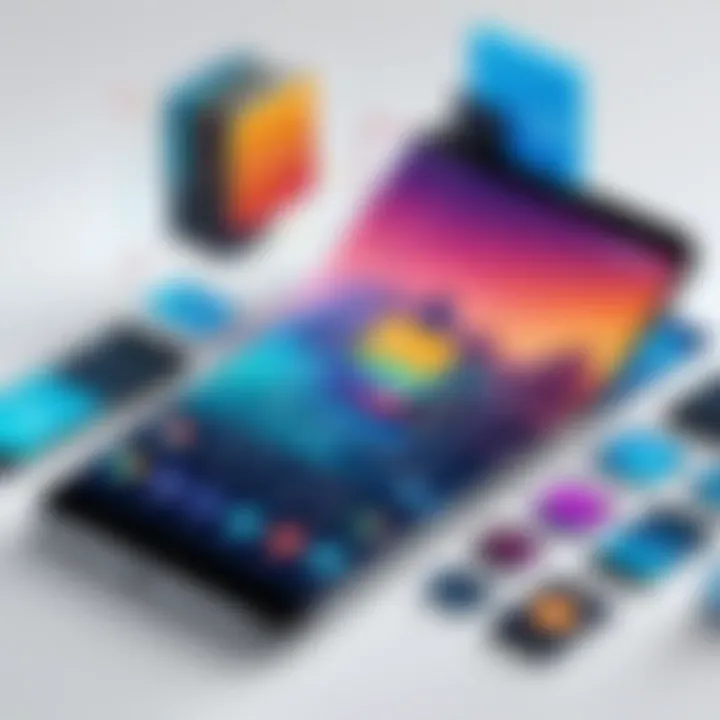
What are Intents?
Intents are messages that allow Android components to request actions from other components. Primarily, there are two types of intents - explicit and implicit. Explicit intents specify the component to start, while implicit intents declare a general action to perform, allowing the system to find the appropriate component to handle it.
Example scenarios for using explicit intents include:
- Launching a specific activity within the same app.
- Directing an intent to a service to perform a task.
On the other hand, implicit intents allow:
- Making the system handler configure functionality like opening a webpage in a browser.
- Invoking shared standards among different applications effectively.
Understanding these types embodies the essence of why intents are a fundamental part of Android development.
Starting Activities with Intents
To start an activity using an Intent, a developer can utilize the method. This approach is straightforward and efficient. First, you create an Intent, pass the activity class that you want to start, and finally, call to trigger it.
Here’s a basic example in code:
This simple strategy highlights the explicit intent. It efficiently switches from one activity to another.
When actions need to be performed that involve multiple components, implicit intents come into play. They need a defined action string to execute the appropriate activity. For example:
In this case, the system determines the best way to fulfill the request. This flexibility makes implicit intents particularly useful for leveraging existing features across applications.
Passing Data between Activities
Data passage between activities can be crucial for maintaining context throughout a user’s journey in the app. Intents allow information to be bundled and sent as part of the activity switch.
Key components for passing data include:
- Extras: Use to attach data to the intent.
- Result: Get results back using and handle responses.
Example of passing a String value:
Retrieving the data in the target activity can be done with:
Data types are varied and can include primitive types, Serializables, or Parcels. In summary, intents are not just conduits for initiating new activities. They embody a mechanism that fosters a nuanced communication system that is essential for cohesive app designs.
It is critical for Android developers to leverage intents not only as command execution entities but as a robust toolset for managing data flows within the platform.
FFragments and Activities
Fragments are an integral part of Android development, providing developers with reusable components that enhance user experience. They allow for flexible UI designs and can manage their own lifecycle, separating concerns and improving code maintainability. This section offers readers insight into the function and structure of fragments within the broader activity lifecycle, elucidating their significance in Android applications.
Understanding Fragments
A fragment represents a modular section of an application’s user interface. Unlike activities, which cater to full-screen UI, fragments focus on a portion of the screen. They are more lightweight, enabling multiple fragments to co-exist within a single activity. This modularity is crucial as it enhances
- Reusability: Fragments can be reused in different activities, thereby reducing code duplication.
- Flexibility: UI can be adjusted dynamically across different screen sizes, particularly important for tablets and phones.
- Lifecycle Management: Each fragment maintains its own lifecycle, which works in tandem with the parent activity.
Android encourages optimal fragment usage along with activities to create cohesive mobile experiences, aligning with user expectations and comfort.
Adding Fragments to Activities
Integrating fragments with activities begins with defining the fragment in an XML layout. Once the layout has been established, the next step is to utilize a to manage fragment transactions. The typical process involves:
- Instantiate the Fragment the application wishes to display.
- Utilize to add, replace, or remove fragments within the activity.
- Commit the transaction to finalize the updates.
Here is an illustrative example:
This code snippet clarifies the practical approach some developers might use to add fragments dynamically. Understanding this process is key to facilitating effective communication between fragments and activities.
Managing Fragment Transactions
The management of fragment transactions is vital to maintain app performance and prevent potential memory leaks. Developers should abide by certain best practices, including:
- Use Fragment Lifecycle Awareness: Ensure that fragments respond appropriately to lifecycle changes, enhancing stability and performance while apps run.
- Essentiality of method: Always execute the commit method after defining your FragmentTransaction to confirm the transaction execution.
- Transaction States: Ensure state changes synchronize correctly to avoid inconsistencies between fragment states and activity states.
Fragment transactions are like building blocks of a large structure, ensuring each piece fits seamlessly within the design of the application.
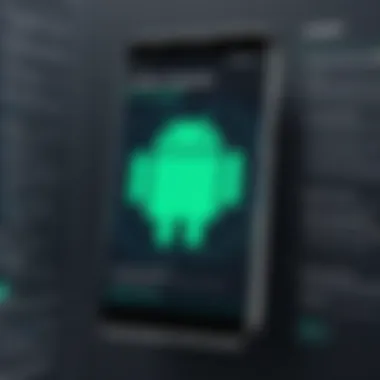
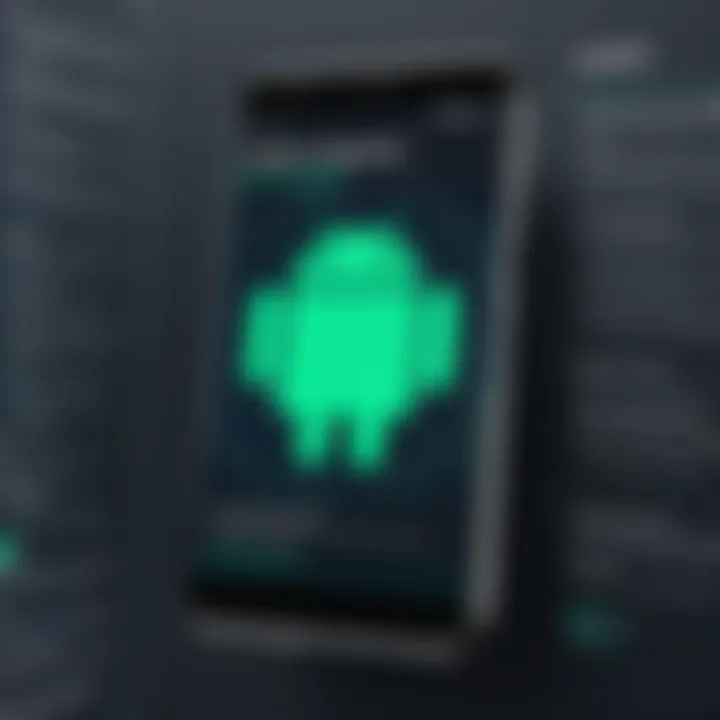
Best Practices for Activity Management
Effective management of Android Activities is critical for developing reliable and efficient applications. Implementing best practices not only improves memory and resource utilization but also enhances the overall user experience. Each practice addresses specific challenges encountered when dealing with Activities. Here are some important elements to consider for managing Activities correctly:
Memory Management in Activities
Memory management plays a vital role in Android development, notably due to its limited resources. Proper management can help prevent memory leaks, which may result in significant performance issues. Here are some key points:
- Activity Destruction: Understand when Activities are destroyed. Override methods like or to release resources that are not needed. Explicitly call for Activities that are no longer required.
- Avoiding Memory Leaks: Be cautious when holding references to activity context in static fields or inner classes. Using the application context instead can help prevent leaks.
- Using Weak References: Consider employing weak references for handling callbacks or listeners. This approach minimizes the contention between Activity lifecycle states and helps with garbage collection.
Implementing these memory management practices ensures that Activities make efficient use of resources and remain responsive to user interactions throughout their lifecycle.
User Experience Considerations
Designing with user experience in mind is crucial for maintaining a positive relationship between users and applications. High UI responsiveness and fluid navigation contribute significantly to user satisfaction. Key considerations include:
- State Preservation: Maintain the state of an Activity during configuration changes, like orientation changes, to ensure a seamless user experience. Utilize methods such as to save UI state.
- Transition Animations: Use appropriate animations during Activity transitions. Proper visual feedback assists users in understanding their navigation flow within the app.
- Error Handling: Sample errors gracefully. Prompt users for necessary actions and guide them through potential solutions, contributing positively to the app's usability and reliability.
By prioritizing user experience considerations, developers are encouraged to deliver applications that are user-friendly and intuitive.
Decoupling Activities and Business Logic
Separating business logic from Activities enhances maintainability and testability of Android applications. This decoupling ensures a clean architecture. Here are practices to follow:
- Model-View-Presenter Pattern: Implementing the Model-View-Presenter design pattern can cleanly separate UI components and business logic, making the codebase easier to manage.
- Use of ViewModels: Using Android's architecture components to manage UI-related data lifecycle wisely separates the data management from the Activity life cycle.
- Repository Pattern: This pattern allows for centralized data management outside of Activities. This keeps Activities focused solely on UI duties.
Decoupling allows developers to create scalable applications. It also simplifies unit testing, given that individual components can be verified in isolation.
By adhering to best practices for Activity management, developers can create robust, scalable applications that perform reliably and deliver enriching user experiences, adapting successfully to Android's dynamic nature.
Testing Android Activities
Testing is a critical aspect of software development that often dictates the performance, reliability, and quality of mobile applications. In the context of Android development, testing activities becomes even more essential because these components play a pivotal role in user interaction and overall application performance. Ensuring that activities function as intended can dramatically improve user satisfaction and reduce bugs in production.
Effective testing allows developers to catch errors early in the development cycle. By identifying issues within activities during testing phase, developers can make necessary adjustments before release. This reduces the likelihood of negative feedback from users and can save significant time and resources in future debugging efforts.
The consideration of testing Android activities also encompasses different content types, which include the testing practices that specify how to achieve a comprehensive evaluation of activities. This dual-pronged approach helps in focusing both on the functionality and user experience, creating an application that meets user expectations and operates optimally on various devices.
Unit Testing Activities
Unit testing is a process where individual components of an application are tested in isolation to validate their functionality. In the case of Android activities, unit tests can confirm that an activity behaves as expected before integrating with other components. This is essential for maintaining the integrity of the codebase.
Unit tests can help with the following aspects:
- Method validation: Ensuring that activity methods execute correctly based on given inputs.
- State management: Checking that activities handle different states properly and consistently.
Mockito, a popular mocking framework for Java, is valuable for unit testing Android activities. It allows developers to test components without depending on their external factors. Using Mockito, one can simulate behavior of classes and ensure that activity methods are invoked in an expected manner.
Here is a simple example using Mockito for unit testing an activity:
This unit test checks whether the starts as expected. If it does not return , then a review is required on the implementation.
UI Testing Practices
UI testing plays a crucial role in verifying the interaction and visual presentation of Android activities. Unlike unit testing, which focuses on the functionality of methods in isolation, UI tests assess how activities respond to user actions. These tests help confirm that an application operates correctly under user interaction.
Common practices for UI testing include:
- Automated Testing: The use of frameworks like Espresso allows for automatic UI testing which measures how the application behaves and interacts with user actions. This method can simulate app environments and provide comprehensive data on performance.
- Manual Testing: While automation is effective, manual testing can provide crucial insights into the user experience by simulating real user interactions. This method often reveals issues that automated frameworks might miss.
It's essential that both previous stages of testing effectively work their magic before reaching this phase. Relying on UI testing alone could lead to overlooking underlying issues that exist at the functional level. Thus, a well-rounded testing strategy should apply unit, integration, and UI tests to gain a robust assessment of Android activities.
“Never stop testing, and your user will never stop enjoying your app.”
Investing time and resources in varied testing practices fosters stronger and more reliable applications. Ultimately, understanding that activity testing is not merely a checkbox in development can lead to significant advantages, setting the foundation for high-quality Android applications that users can trust.
End
Understanding Android Activity is crucial for both new and seasoned developers. This article has traversed the various facets of activities, starting from their definition to their relationship with other components like fragments and intents. A nuanced understanding of activities aids in strengthening app architecture.
Below are essential elements we covered:
Recap of Key Points
- Definition and Role: Activities are ones that provide the user interface for Android applications.
- Lifecycle Management: The lifecycle of an activity affects how an application behaves in different scenarios, including dealing with memory and state changes.
- Interaction Design: Creating effective layouts and responding to user inputs are central to user retention and satisfaction.
- Intents: This crucial component helps activities to interact and pass data seamlessly.
- Testing: Proper testing of activities ensures reliability and performance in user experiences.
Effective activity management not only enhances user engagement, but also promotes maintainability and scalability within applications.
Future Trends in Android Activity Development
As we look forward, certain trends are emerging in the realm of Android activities:
- Increased Usage of Jetpack Compose: Developers are shifting towards composable functions instead of traditional XML that can make designing interfaces more systematic and less error prone.
- Focus on Dynamic UI: Activities might evolve to become more context-aware, enhancing personalization and interaction based on user data and real-time information.
- Fragment Harmonization: The relationship between activities and fragments is expected to become more integrated, improving architecture efficiency.
- Enhanced Testing Tools: Embracing advanced testing frameworks and tools can make the act of testing activities easier and more comprehensive.
To conclude, being informed about these trends can significantly impact the future success of apps within an ever-evolving market.
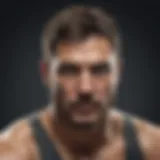
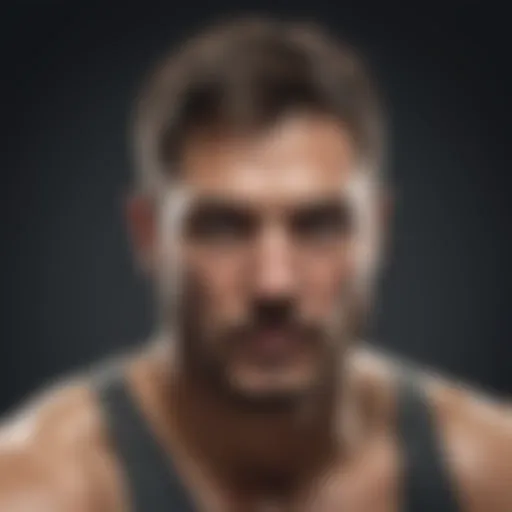