Understanding AngularJS Components: A Comprehensive Guide
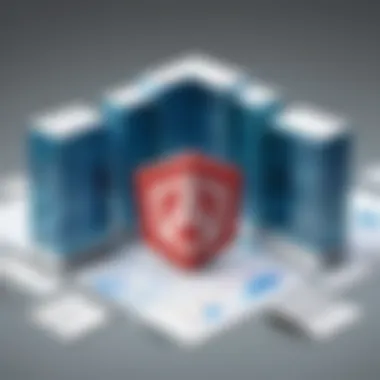
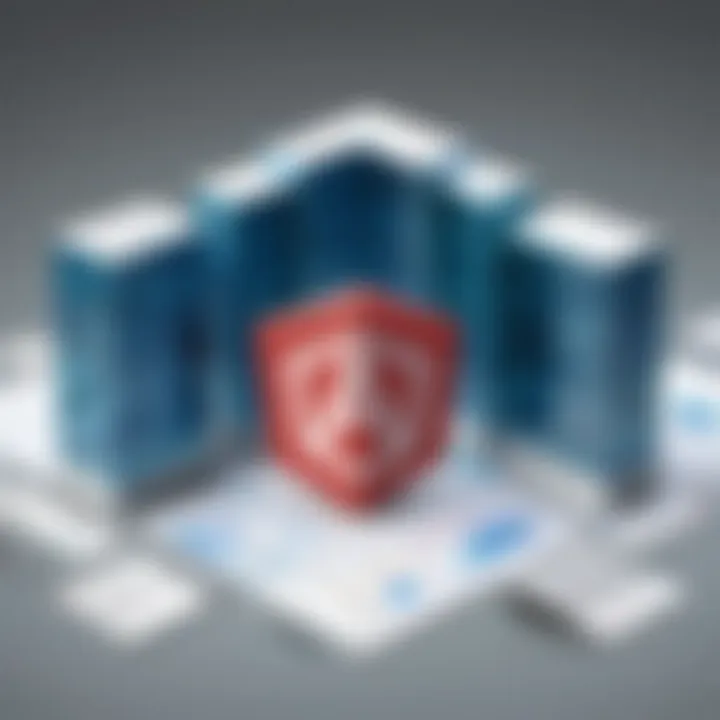
Overview of Topic
Intro to the Main Concept Covered
AngularJS components are a pivotal part of the framework that allow developers to create reusable, maintainable web applications. A component encourages a modular approach to building user interfaces. It encapsulates HTML, CSS, and JavaScript into a self-contained unit, promoting clarity and organization.
Scope and Significance in the Tech Industry
AngularJS is essential for web development today. Its component-based architecture reduces redundancy and allows for easier testing and debugging. This is especially important in large-scale applications that demand scalability and maintainability. Companies seeking efficient development workflows increasingly adopt AngularJS components.
Brief History and Evolution
Since its inception in 2010, AngularJS has undergone significant changes. The introduction of components in later versions marked a major shift in how applications are built within the Angular ecosystem. This evolution reflects the industry's move towards more structured and efficient development practices.
Fundamentals Explained
Core Principles and Theories Related to the Topic
- Encapsulation: Each component encapsulates its own data and behavior. This reduces the chances of interference from other parts of the application.
- Reusability: Components can be reused in different parts of an application or in different projects entirely.
- Separation of Concerns: This promotes better organization of code, where different functions are handled by distinct components.
Key Terminology and Definitions
- Component: A self-contained unit with its own view (HTML), behavior (JavaScript), and data (CSS).
- Template: The HTML part of a component that defines what the user sees.
- Controller: Manages the data of a component and responds to user actions.
Basic Concepts and Foundational Knowledge
Understanding AngularJS components is foundational for anyone learning web development using this framework. Familiarity with the Model-View-Controller (MVC) pattern is also beneficial as components often utilize this pattern for structuring code effectively.
Practical Applications and Examples
Real-world Case Studies and Applications
Many companies, including Google and Microsoft, utilize AngularJS. They implement components to develop complex applications with great efficiency. A good example is Gmail, which uses AngularJS components to manage different functionalities.
Demonstrations and Hands-on Projects
Building a simple AngularJS component can start with defining the component in script, followed by HTML templates. You could create a user profile card as a component, containing details and an avatar.
Code Snippets and Implementation Guidelines
Here is an example of a simple AngularJS component:
Advanced Topics and Latest Trends
Cutting-edge Developments in the Field
Frameworks evolve rapidly. AngularJS is not an exception. The continual updates and improvements emphasize the move towards components and better performance.
Advanced Techniques and Methodologies
Components can be optimized using lazy loading and AOT (Ahead of Time) compilation to enhance performance. These methods are key to managing large applications efficiently.
Future Prospects and Upcoming Trends
With the shift towards microservices and serverless architectures, components will play an even more critical role. Developers must stay informed about new updates and best practices in AngularJS.
Tips and Resources for Further Learning
Recommended Books, Courses, and Online Resources
- AngularJS: Up and Running by Shyam Seshadri
- Online course on platforms like Coursera or Udemy on AngularJS basics
Tools and Software for Practical Usage
Using tools like Visual Studio Code or Angular CLI can streamline component development. Familiarization with debugging tools is also recommended for effective troubleshooting.
Explore the official AngularJS documentation for comprehensive guides and additional resources: angularjs.org
Prelims to AngularJS Components
In the realm of modern web development, AngularJS has established itself as a powerful framework for building dynamic applications. This section delves into the significance of AngularJS components, which serve as the building blocks of any robust AngularJS application. A well-structured application enhances maintainability, encourages reusability, and allows for more effective collaboration among developers.
Components encapsulate functionality, including HTML templates and CSS styles, enabling developers to create self-contained units that can be easily managed. Understanding their role is crucial for those venturing into AngularJS or looking to refine their skills in building scalable applications. Here, we will explore their definitions, evolution, and the distinctions between components and directives, providing a solid foundation for further exploration of the topic.
Definition of Components
Components are distinct entities in AngularJS that encapsulate both the view (HTML) and the logic (JavaScript) of a specific section of the user interface. They allow developers to break down applications into smaller, manageable parts. Each component can be developed, tested, and updated independently, making it easier to work on larger projects. The key features of a component include:
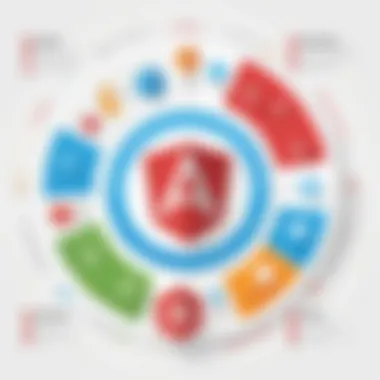
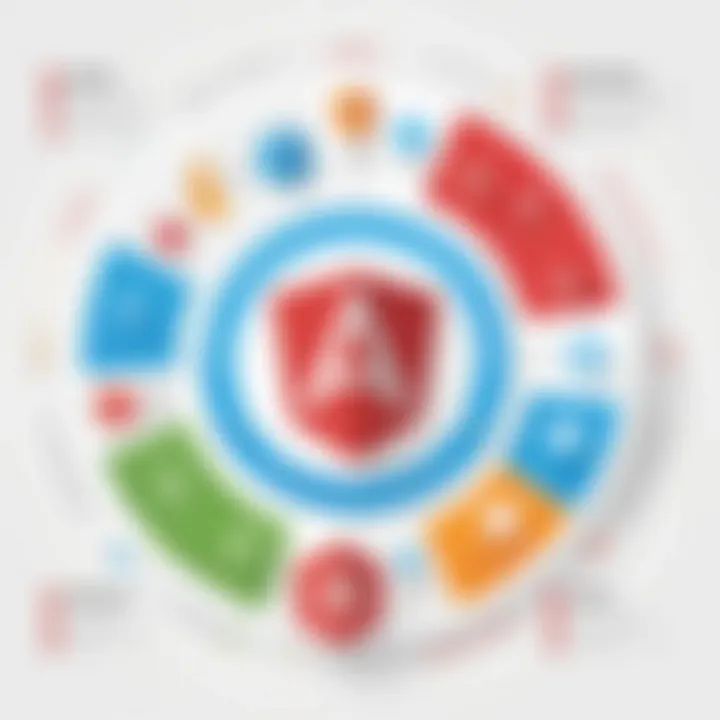
- Template: Defines how the view will appear to users.
- Controller: Contains the business logic for the component, controlling how the view behaves.
- Metadata: Provides additional information about the component, such as its selectors and dependencies.
The ability to create individual components fosters a clean architecture, streamlining both development and maintenance processes.
Evolution of AngularJS
AngularJS has undergone significant changes since its inception, transitioning from simpler architectures to more sophisticated models. Initially launched in 2010, it introduced a new paradigm for handling web applications. As the landscape of web development evolved, so did AngularJS, incorporating essential features such as dependency injection and two-way data binding. These enhancements led to the introduction of components in Angular 1.5, which aimed to improve the way developers create reusable UI elements. The shift to a component-based architecture mirrors wider industry trends towards modular and maintainable code, reflecting an understanding of the complexities involved in large-scale applications.
Difference Between Components and Directives
While both components and directives are essential in AngularJS, they serve different purposes. Components are primarily designed for creating UI elements with encapsulated functionality, while directives are more about extending HTML capabilities through behavior rather than structure. Here are some distinctions:
- Scope: Components have an isolated scope, allowing for better encapsulation. Directives typically use shared scopes.
- Intent: Components are suited for instances where a view and behavior need to be co-located. Directives are often used to add behavior to existing elements without necessarily creating a new view.
- Usage: Components are easier to use in templates as they act like custom tags. Directives require more complex syntax, often leading to confusion among developers.
Component Architecture
Component Architecture in AngularJS is crucial for developing scalable and maintainable web applications. This architecture allows developers to break down complex user interfaces into smaller, manageable pieces called components. Each component encapsulates its own functionality and can be reused throughout the application, promoting both code cleanliness and efficiency.
When thinking about Component Architecture, consider the following elements:
- Modularity: Components are self-contained units. This modular approach simplifies development and enhances collaboration among team members. If one group is working on a specific component, their changes do not affect others.
- Reusability: By designing components to be reusable, developers can save significant time. A single component could serve multiple parts of an application without the need for redundant code.
- Separation of Concerns: Components separate logic, template, and styles from one another, making it easier to manage and test.
In summary, an effective Component Architecture leads to higher productivity, better organization, and ultimately a more robust application.
Structure of a Component
A component in AngularJS typically consists of three main parts: the template, the class, and the metadata. These elements work together to define what the component does, how it is presented, and how it behaves within the application.
- Template: This defines the HTML view of the component. It specifies how the data will be displayed to the user. Using Angular's syntax, templates can include expressions, directives, and even other components.
- Class: The component class contains the business logic. It defines the properties and methods needed for the component to function. Here, you can implement data manipulation or response to user events.
- Metadata: Metadata is added using a decorator. The @Component decorator provides Angular with the necessary information about the component, including its selector, template, and styles.
Including these three structural elements ensures that the component is functional and adheres to AngularJS principles.
Template and Styles
Templates act as the interface of the component. They bind to the component's class to present data and receive user input.
- Variables in the Template: You can use expressions to render properties from the component class directly into HTML, allowing dynamic updates when the data changes.
- Directives: AngularJS directives enhance templates by adding functionality, such as conditional rendering with *ngIf or looping through lists with *ngFor.
- Styles: Styling can be applied directly within the component using encapsulated styles in a style block. This prevents global CSS from leaking into your component's presentation, maintaining its distinct look.
Class and Metadata
The component class is where the logic resides. It should be designed to be as lightweight as possible to optimize performance. Here are key aspects:
- Properties: These define the data model for the component. You can establish default values, which can later be modified through user interactions.
- Methods: Utilize methods within the class to handle events and business logic, ensuring a clear separation of the view and logic.
Furthermore, metadata, achieved through the @Component decorator, provides Angular with identifiers critical for component operation. This includes:
- : Determines how and where the component appears in your HTML.
- or : Points to the component’s HTML structure.
- or : This field applies styles specific to the component.
Lifecycle of AngularJS Components
The lifecycle of AngularJS components is a crucial aspect of their functionality. Understanding this lifecycle allows developers to manage their applications effectively. Each phase in the lifecycle has specific hooks and events that developers can respond to, which helps in optimizing performance and enhancing user experience. By comprehending the lifecycle, one can make informed decisions about how to design and implement components in a scalable manner.
Creation and Initialization
When an AngularJS component is created, it goes through the initialization phase. This phase sets up the component and prepares it for interaction. It begins with the constructor method, where initial values are set and dependencies are injected.
During creation, a component's inputs can also be evaluated. These inputs are properties that can receive data from parent components. For instance, when a component receives a new input value, this can be handled using the ngOnChanges method. This prepares the component to represent the provided data accurately.
In addition, it's important to perform setup operations in this phase. For instance, when you need to activate event listeners or set up subscriptions to data sources, these tasks should take place during initialization. The creation process is critical; if mismanaged, it can lead to performance issues down the line.
Change Detection
Change detection is another vital stage in the lifecycle of AngularJS components. It allows AngularJS to track changes to component data and update the view accordingly. The framework uses a mechanism known as the digest cycle to check for changes.
During this cycle, AngularJS compares the previous and current state to determine if any updates are necessary. This comparison is done automatically, but in more complex applications, developers can optimize change detection using strategies such as OnPush, which checks a component's state only when its inputs change.
Change detection is essential for responsive and interactive applications. If a change occurs, AngularJS re-renders the affected part of the UI, ensuring that the user gets the most up-to-date information. Failure to manage change detection appropriately can result in stale data being presented. Implementing change detection strategies helps in reducing unnecessary updates and improves performance.
Destruction of Components
The final phase in a component's lifecycle is destruction. This phase is critical for cleaning up and releasing resources that the component may have used. When a component is destroyed, AngularJS calls the ngOnDestroy method.
In this method, developers should remove any event listeners, clear intervals, or unsubscribe from observables to prevent memory leaks. Failure to do so can lead to performance degradation and can cause issues in long-running applications.
As a best practice, always ensure that the necessary cleanup is performed. It not only leads to better performance but also contributes to an overall robust application architecture.
"A clean lifecycle management is essential for maintaining performance in AngularJS applications."
Data Binding in Components
Data binding is a key concept in AngularJS components, significantly enhancing the interaction between the user interface and the underlying data model. Understanding how data binding works is essential for developing robust AngularJS applications. It allows for efficient data management and seamless updates in the user interface, making it a fundamental element of interactive web applications.
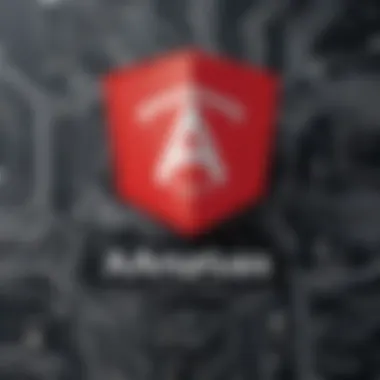
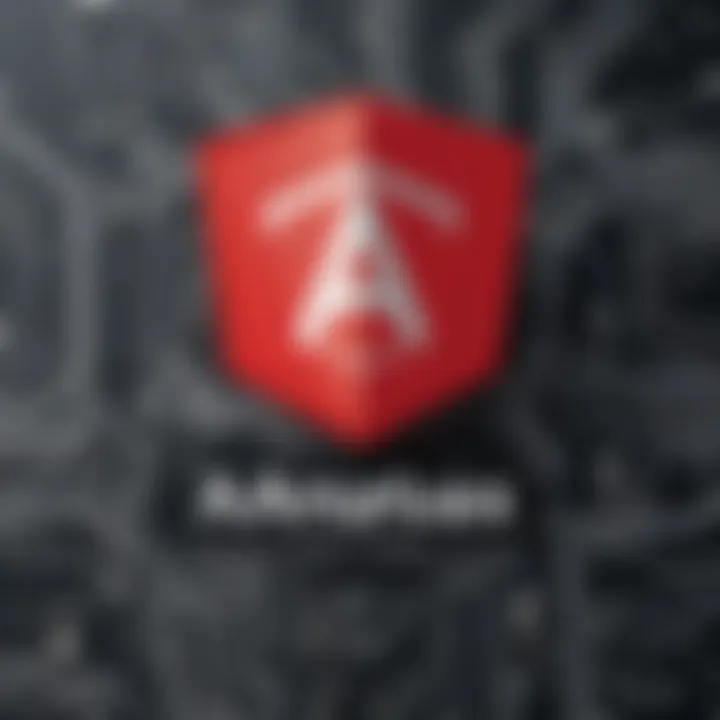
One-Way Data Binding
One-way data binding refers to the flow of data in a single direction. When developers utilize one-way data binding in AngularJS, the data only moves from the model to the view. This means that changes in the model are reflected in the view, but not vice versa. This is particularly beneficial for scenarios where the user interface needs to display data without requiring user input to alter the underlying model.
Key characteristics of one-way data binding include:
- Simplicity: It reduces complexity since the view does not affect the model. This makes debugging easier as developers can focus on tracking one data flow.
- Performance: One-way data binding can lead to performance improvements because it minimizes the number of watchers AngularJS needs to maintain. This is particularly advantageous in large applications with extensive data lists or complex UI.
- Readability: Using one-way data binding enhances code readability by clearly defining how data flows within the component.
Here is an example of one-way data binding in AngularJS:
In this snippet, the variable is set, and any bound UI elements would reflect this value without allowing direct changes to it from the interface.
Two-Way Data Binding
Two-way data binding, on the other hand, allows bidirectional data flow between the view and the model. In this model, any changes made by the user in the view are instantly reflected in the model, and any updates to the model are likewise shown in the view. This feature is particularly useful for forms and interactive components where user input is a crucial part of the application.
Some important aspects of two-way data binding include:
- Interactivity: It enhances the responsiveness of the application by allowing user inputs to directly affect the application state. This is especially useful in scenarios where frequent updates are expected.
- Model Synchronization: Developers can ensure that the UI accurately represents the current state of the model, which helps in maintaining consistency across the application.
- Real-time Validation: With two-way data binding, immediate feedback can be provided to users, such as form validation messages that appear as soon as an error is detected.
An example of two-way data binding is shown below:
In this example, the directive creates a two-way binding between the input element and the variable in the scope. As the user types into the input field, is updated in real-time.
Important Note: Understanding the implications of both one-way and two-way data binding can significantly streamline the design and development processes. It helps developers make informed choices about how to structure their components and data flows.
In summary, mastering data binding in AngularJS components is crucial for building dynamic web applications. The choice between one-way and two-way binding should be informed by the specific requirements of the application, balancing performance with interactivity.
Using Services in Components
AngularJS services are essential parts of building effective and efficient applications. They facilitate code reuse and help maintain cleaner architecture. Services allow for encapsulation of common functionalities and can significantly simplify component logic. By separating concerns, developers can manage complexity better.
Injecting Services
Injecting services into AngularJS components is a critical aspect that allows components to access shared functionalities. The process begins by defining a service using the method. Within this service, developers can encapsulate specific behavior, such as data fetching, business logic, or utility functions, without directly coupling the service to the component.
Here’s a simple example of a service definition:
Once the service is defined, it can be injected into a component. This is done through the constructor function of the component, allowing the component to leverage the service's capabilities. For instance:
This component now has access to , enabling it to retrieve data effectively and maintain a clean separation between the component's responsibilities and data management logic.
Sharing Data Between Components
Sharing data between AngularJS components is crucial for developing responsive applications. Services facilitate data sharing, enabling different components to access and modify the same data source. This approach ensures that changes in one component can be reflected in others, maintaining consistency throughout the application.
To illustrate, let’s consider a scenario where two components need to share user information. By using a shared service, both components can subscribe to the data. The service functions as a central data repository, where a state management pattern can be applied.
Here is a basic example of a service designed to hold user data:
Each component can interact with to get the current user information. For example:
In this manner, updating the user in the component reflects the changes in the component immediately. This demonstrates the power of services in maintaining shared state while promoting component isolation. It encourages a modular approach that enhances both maintainability and testability in modern web applications.
In summary, using services in AngularJS components not only simplifies code but also provides a robust architecture for data management.
Routing with Components
Routing in AngularJS plays a crucial role in structuring web applications. It allows developers to define how different components can be navigated within a single page application. With proper routing, users can experience seamless transitions between different views. This section will detail the key aspects of routing with components, outlining its importance and emphasizing best practices.
Configuring Routes
When configuring routes in AngularJS, developers must utilize the service. This service allows the application to map URLs to specific components. Here’s a basic example:
In this configuration, different routes are associated with specific templates and controllers. This separation follows a modular approach. Modularity is essential as it enhances maintainability, making it easier to scale applications over time. Each route corresponds to a view, which is loaded dynamically as the user navigates through the application. It is crucial to ensure that the templates are responsive and performant, providing a smooth user experience.
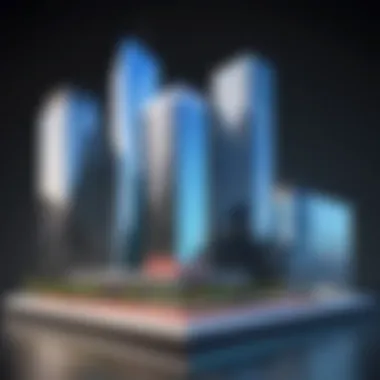
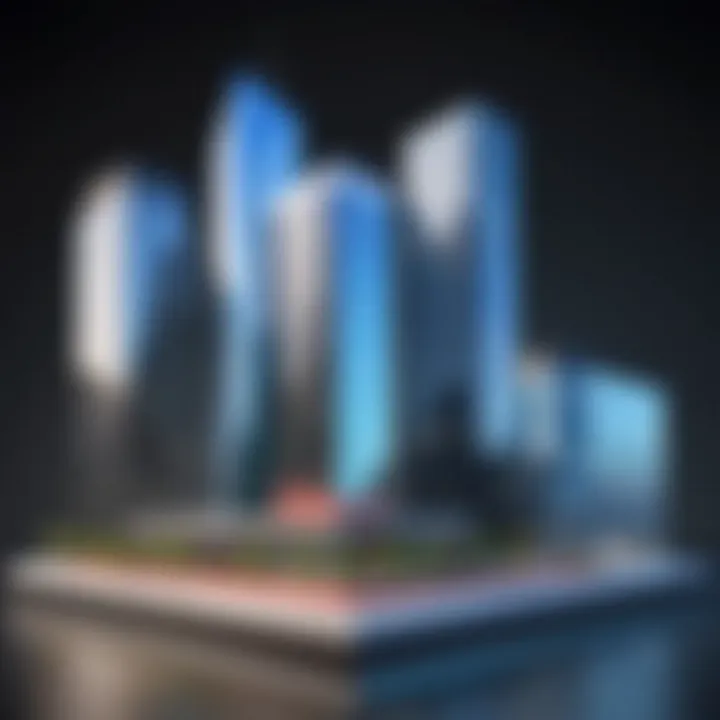
Nested Components
Nested components allow developers to create a hierarchical structure within an application. This structure can enhance organization and reuse of components. For instance, an application may have a main component with nested sub-components. Each sub-component can have its routing configuration, which can either be standalone or combined with the parent component’s routes.
To implement nested routing, follow a pattern similar to the following:
With nested routes, the application can display different components based on the path. This approach is advantageous for building complex interfaces where user contexts vary. Organizing components this way can lead to improved readability and easier changes.
Component Communication
Component communication is vital in AngularJS as it enhances the interaction between different components. It allows seamless data exchange and event handling, which are crucial for building complex applications. Understanding how components communicate helps developers create more organized, maintainable, and scalable code. In this section, we will explore three primary methods of component communication: input properties, output events, and service-based communication. Each method has its specific use cases and benefits, which we will detail below.
Input Properties
Input properties are a fundamental mechanism for passing data from a parent component to a child component. By defining parameters in the child component, the parent can bind data directly, providing dynamic behavior and flexibility.
Using input properties promotes a clean data flow. They ensure that child components depend solely on the data provided by their parents, thereby maintaining a clear hierarchy. This sort of one-way data binding makes it easier to track changes and debug issues. Here’s a simple code snippet to illustrate the usage of input properties:
In this example, is an input property in . Parent components can pass the required data to , thus facilitating effective communication.
Output Events
Output events facilitate communication in the opposite direction, from child components to parent components. When a child component needs to notify its parent about an action or change, it uses output events. By configuring a one-way data flow, AngularJS enforces a rule that interactions should be predictable and easy to follow.
In practice, developers emit events using AngularJS’s built-in or methods. This allows a child component to trigger an event that parents can catch and respond to accordingly. Here’s an example:
In this snippet, the child component emits an event called . The parent component should listen for this event and handle it as necessary. Thus, output events provide a powerful way to manage events across the component hierarchy.
Service-Based Communication
Service-based communication is an alternative approach that involves using shared services to facilitate data exchange between components. By leveraging dependency injection, components can access the same service instance, enabling them to share state and interact indirectly. This method is particularly useful in more complex applications that require synchronization across multiple components.
Services are singleton objects that persist for the lifetime of the application. To use a service, developers define it as a module and then inject it into the components that require access. Here’s a simplified example:
In this example, enables and to access and update the same piece of data. This method simplifies data management and helps maintain consistency.
Best Practices for AngularJS Components
AngularJS components are vital for building robust, maintainable web applications. Understanding best practices is key to achieving high performance and ensuring scalability of your projects. This section elucidates the importance of adhering to specific guidelines in component development. These best practices foster a clear architecture, enhance reusability, and streamline the process of debugging and testing. By implementing such practices, developers can create components that are easy to manage and extend over time.
Reusability and Modularity
Reusability is an essential principle in software development, particularly in AngularJS components. When components are designed to be modular, they can be used in different parts of the application without modification. This not only saves development time but also reduces the chances of bugs.
Some key considerations for enhancing reusability include:
- Define Clear Inputs and Outputs: Components should clearly specify their input properties and output events. This creates a predictable interface for other components to interact with.
- Avoid Application-Specific Logic: Keep components generic. Logic that pertains to a specific application use case should be handled in services instead of being embedded directly in components.
- Utilize AngularJS Libraries: Leverage existing libraries and modules to enhance functionality without reinventing the wheel. The community provides numerous resources, and utilizing them can speed up development.
Performance Optimization
Optimizing performance should be a priority when developing AngularJS components. Inefficient components can slow down applications, particularly those handling a large number of elements or frequent updates. Here are some strategies for optimizing performance:
- Limit Watchers: Each two-way data binding creates a watcher. Reducing the number of watchers can lead to better performance. Use one-way data binding when possible.
- Use Track By in ng-repeat: When displaying lists, using can minimize the amount of DOM manipulation necessary and thus enhance rendering speed.
- Lazy Loading: Split larger components into smaller parts. Load these smaller components when needed rather than all at once, as this can greatly decrease initial load time.
Testing Components
Testing is crucial in ensuring that components behave as expected. A thorough testing strategy also contributes to the maintainability of the codebase. AngularJS provides built-in tools that facilitate effective testing. Here are the best practices to consider:
- Write Unit Tests: Each component should have corresponding unit tests. Use frameworks like Jasmine or Mocha for writing these tests. Unit tests help to verify the functionality of individual components in isolation.
- Utilize End-to-End Testing: Use tools like Protractor to execute end-to-end testing. This ensures that components interact correctly within the broader application framework, providing a complete view of application behavior.
- Automate Testing: Implement continuous integration systems that automatically run tests whenever changes are made. This helps identify and address issues early in the development process.
Following best practices for AngularJS components will lead to an efficient development process and a high-quality application that can adapt to future requirements.
By following these best practices, developers not only improve the overall quality of their AngularJS components but also enhance their own productivity. With a focus on reusability, optimization, and testing, developers can create components that are both powerful and easy to maintain.
Finale
In this article, we explored AngularJS components, which serve as fundamental building blocks in web application development. The discussion highlighted the architecture of components, their lifecycle, and their roles in improving application scalability and maintainability. Understanding these concepts is crucial for leveraging the full potential of AngularJS in creating robust applications.
The Future of AngularJS Components
The future of AngularJS components appears both promising and challenging. While AngularJS has paved the way for a more component-oriented approach in web development, the landscape of front-end frameworks is rapidly evolving. Technologies like Angular, React, and Vue.js continue to emerge and shape best practices.
Developers will need to adapt and stay informed about advancements in the industry. Embracing new features or even transitioning to newer frameworks may become necessary. However, the principles learned from AngularJS components, such as modularity and reusability, will remain relevant across different technologies. The mindset of creating cohesive components will provide a solid foundation for future projects.
Final Thoughts
As we conclude the discussion on AngularJS components, it is evident that mastering this topic is beneficial for developers at all levels. The ability to build reusable and maintainable components can lead to cleaner and more organized code. Furthermore, understanding the intricacies of component communication, lifecycle methods, and data binding equips developers with the tools needed to craft efficient web applications.
In summary, AngularJS components are not just a collection of code. They embody design principles that can enhance the quality of web applications. Whether you are just starting your journey in programming or are an experienced developer, delving deeply into this topic will pay dividends in your future programming endeavors. Keep exploring, practicing, and refining your skills as you navigate the world of AngularJS and beyond.