Mastering Class-Based Object-Oriented Programming
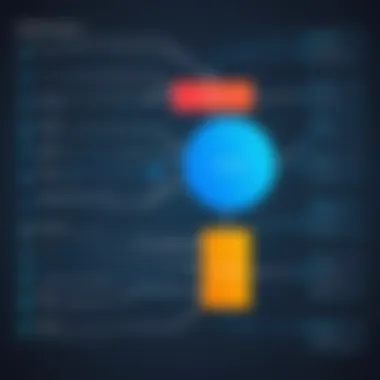
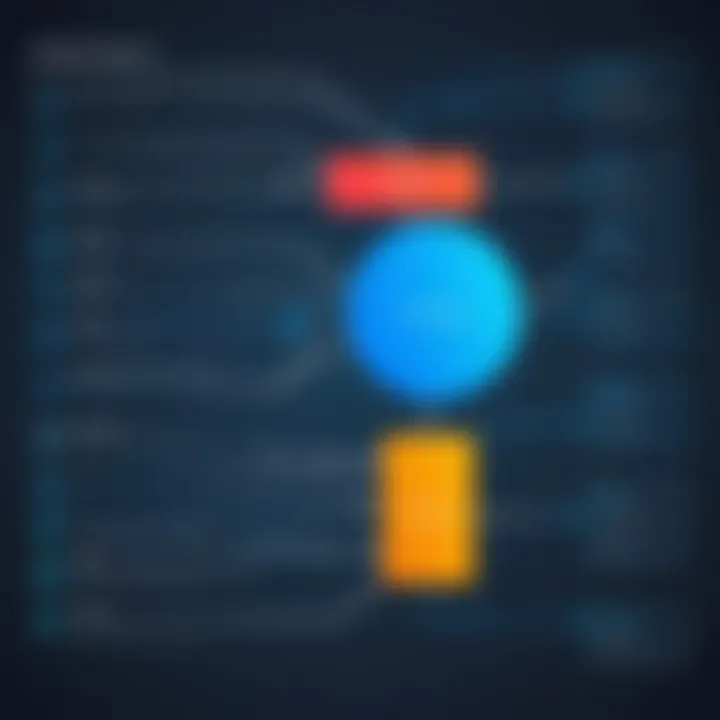
Overview of Topic
Preface to the Main Concept Covered
Class-based object-oriented programming (OOP) serves as a cornerstone of modern software engineering. This paradigm allows programmers to construct robust systems by emphasizing key design principles such as modularity and code reuse. By harnessing the concept of classes and objects, developers create blueprints for real-world entities, enabling greater abstraction and flexibility. This approach facilitates a more organized and efficient coding style, making it a preferred choice for many programmers.
Scope and Significance in the Tech Industry
The relevance of class-based OOP extends beyond academia; it plays a crucial role in various applications across industries. From web development to enterprise software, understanding OOP principles empowers developers to tackle complex systems and manage large codebases. Moreover, OOP languages like Java, C++, and Python are widely used, underscoring the importance of mastering this paradigm within the tech ecosystem.
Brief History and Evolution
The evolution of object-oriented programming can be traced back to the 1960s, with languages like Simula pioneering the concept. Over the decades, several other programming languages have adopted and refined OOP principles. Notably, in the 1980s, C++ merged procedural and object-oriented concepts, enhancing applicability across programming scenarios. Nowadays, programming languages continue to evolve, integrating OOP principles with new paradigms to adapt to changing computational needs.
Fundamentals Explained
Core Principles and Theories Related to the Topic
In class-based OOP, several core principles define its structure and functionality:
- Encapsulation: This principle emphasizes bundling data and methods within a single unit (class) while restricting direct access to some components.
- Inheritance: Inheritance allows a class to inherit properties and methods from another class, promoting code reuse and logical hierarchy.
- Polymorphism: This principle enables methods to do different things based on the object it is acting upon, providing flexibility in code.
Key Terminology and Definitions
To understand OOP fully, some fundamental terms must be clarified:
- Class: A blueprint from which objects are created.
- Object: An instance of a class containing data and methods.
- Method: A function defined within a class.
- Attribute: A variable that holds data within a class.
Basic Concepts and Foundational Knowledge
Grasping these principles and terminologies is vital for anyone seeking knowledge in OOP. The interaction between classes and objects forms the backbone of this programming paradigm. Each object can be viewed as a self-contained module featuring its data and operations. This encapsulation reinforces security and integrity, ensuring that objects do not interfere with each other's states.
Practical Applications and Examples
Real-World Case Studies and Applications
OOP is prevalent in software design, including web applications, gaming, and mobile software. For example, in the development of a banking application, classes can represent customers, accounts, and transactions. These classes can inherit properties and methods from generalized classes, streamlining the creation of complex functionalities.
Demonstrations and Hands-on Projects
Consider a basic implementation of a class in Python:
This code snippet exhibits how to create a simple class, instantiate it, and invoke a method. Such examples reinforce theoretical knowledge through practical application.
Code Snippets and Implementation Guidelines
When implementing OOP in any project, consider the following best practices:
- Always define clear responsibilities for each class.
- Use meaningful names for attributes and methods.
- Prioritize encapsulation to safeguard data.
Advanced Topics and Latest Trends
Cutting-edge Developments in the Field
As technology advances, the need for more efficient and scalable programming solutions drives innovations in OOP. Some emerging trends include the integration of OOP with functional programming to enhance versatility and performance.
Advanced Techniques and Methodologies
Techniques such as design patterns come into play when building complex systems. Patterns like the Singleton and Factory help manage object creation and lifecycle more effectively.
Future Prospects and Upcoming Trends
The future of OOP looks promising as it adapts to new challenges in software development. Concepts such as aspect-oriented programming are gaining traction, allowing for cleaner separation of cross-cutting concerns within applications. As AI and machine learning become increasingly prevalent, effective use of OOP will likely be crucial in efficiently managing data and operations in these domains.
Tips and Resources for Further Learning
Recommended Books, Courses, and Online Resources
To deepen your understanding of OOP, consider the following resources:
- "Head First Object-Oriented Analysis and Design" by Brett McLaughlin.
- Online platforms like Coursera and Udemy offer courses tailored for programming languages with OOP features.
Tools and Software for Practical Usage
Familiarize yourself with Integrated Development Environments (IDEs) such as IntelliJ IDEA for Java, or PyCharm for Python, which can enhance productivity and facilitate learning.
OOP remains a fundamental aspect of software development, enabling developers to create efficient and maintainable systems. Understanding its principles not only improves coding skills but also prepares one for advanced software engineering roles.
Foreword to Object-Oriented Programming
Object-Oriented Programming (OOP) represents a significant paradigm in the field of software development. Understanding the foundational concepts of OOP aids developers in structuring their code more effectively, promoting better organization and code management. In this section, we will delve into what defines OOP and its evolution over time.
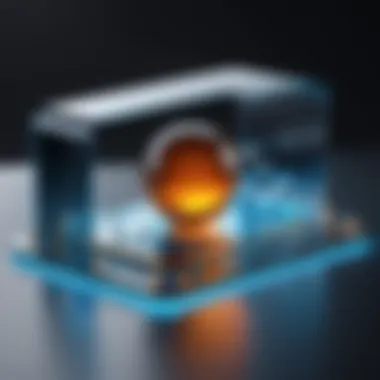
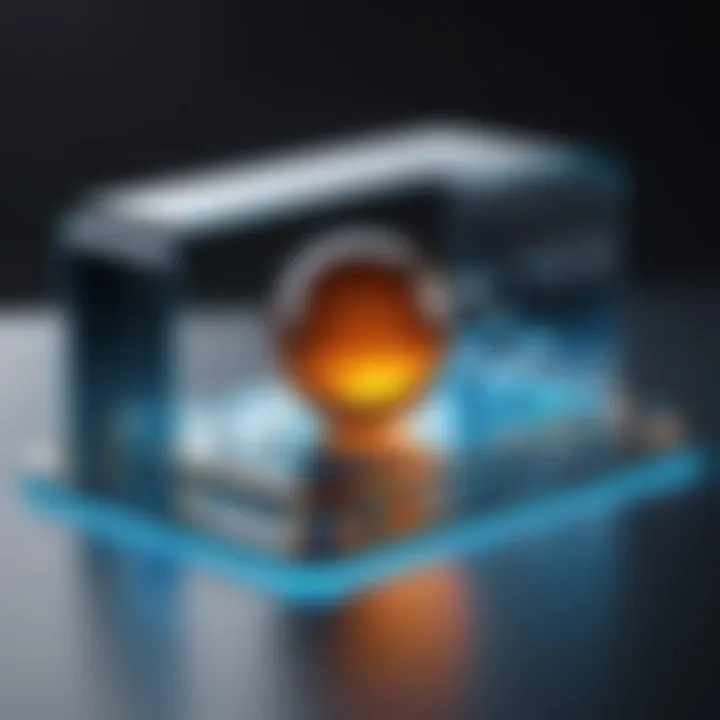
Definition of Object-Oriented Programming
At its core, Object-Oriented Programming is a programming model organized around "objects" instead of actions. An object can be a data structure that contains data, in the form of fields, often known as attributes or properties. Additionally, it may include code, in the form of procedures, often known as methods.
OOP provides a way to conceptualize and organize complex systems, allowing programmers to model real-world entities and relationships. By grouping data and the methods that operate on that data into a single entity, OOP helps in increasing the clarity of the code and improving maintainability.
Prominent features of OOP include:
- Encapsulation: This is the bundling of data with the methods that operate on it. Encapsulation restricts direct access to some of the object's components.
- Inheritance: This allows a new class to inherit properties and behavior from an existing class, enabling code reuse.
- Polymorphism: Polymorphism allows methods to do different things based on the object it is acting upon, providing flexibility in programming.
Historical Context and Evolution
The genesis of Object-Oriented Programming can be traced back to the 1960s with the development of languages like Simula. Simula introduced the concept of classes and objects, setting the stage for future OOP concepts. The 1980s saw a rise in OOP's popularity as programming languages began to adopt these principles, with languages such as Smalltalk becoming prominent.
The evolution continued into the 1990s and 2000s, leading to the mainstream adoption of OOP through languages like C++ and Java. These languages incorporated OOP principles while introducing additional features that enhanced usability. Modern programming environments heavily rely on OOP principles, showcasing its significance in achieving scalability and maintainability in software projects.
Important Note: Object-Oriented Programming is not just a theoretical concept; it has practical implications that developers utilize in everyday programming tasks.
Core Concepts of Class-Based OOP
The core concepts of class-based object-oriented programming (OOP) serve as the fundamental building blocks that inform both the design and implementation of software systems. These principles not only provide a framework for structuring code but also enhance the clarity and maintainability of programming efforts. Understanding these concepts is essential for students and IT professionals alike, as they form the necessary groundwork for more advanced programming techniques.
In this section, we will explore the essential concepts of classes, encapsulation, inheritance, and polymorphism. Each of these elements plays a critical role in shaping the way developers approach problem-solving within the object-oriented paradigm.
Classes and Objects
Classes and objects are the two most fundamental concepts in OOP. A class can be thought of as a blueprint or template that defines a set of attributes and methods applicable to a specific type of object. Objects, on the other hand, are instances of classes. When a class is defined, no memory is allocated until an object is created from the class. This distinction is crucial in understanding how data and behavior are organized in OOP.
An example would be a class called . This class may have attributes such as , , and , as well as methods like and . When you create an object from the class, say a , you instantiate all properties and methods defined in that class. This separation aids in managing relationships and complexity in larger applications, leading to a more organized codebase.
Encapsulation
Encapsulation is another core tenet of OOP that promotes data hiding and abstraction. It is the bundling of data and methods that operate on that data within a single unit, usually a class, and restricting access to some of the object’s components. This ensures that internal object state can only be modified through well-defined interfaces.
The primary benefits of encapsulation include:
- Data protection: By preventing direct access to the internal state of the object, you reduce the risk of unforeseen side effects from other parts of the program.
- Enhanced maintainability: Changes to internal implementations can be made with minimal impact on external code.
For instance, if we consider the previous class, we can make the attribute private and only allow external access through methods like or . This encapsulates the logic for speed management and ensures that it is controlled specifically through designated methods.
Inheritance
Inheritance is a mechanism that allows one class to inherit attributes and methods from another class. This promotes code reusability and establishes a relationship between classes, where the derived class (or child class) extends the functionality of a base class (or parent class).
For example, if we have a base class called , it could include methods common to all vehicles, such as and . We could then create a subclass that inherits from , adding specific characteristics such as . This means inherits all functionalities of , allowing for shared code and minimization of redundancy.
Polymorphism
Polymorphism allows for methods to do different things based on the object it is acting upon. This is typically implemented in two ways: through method overriding and method overloading.
- Method Overriding: This occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. For instance, if has a method , the class may override this method to provide a different sound.
- Method Overloading: This allows multiple methods to have the same name but different parameters within the same class. For example, a class may have a method that behaves differently when passed a versus a .
Polymorphism enhances flexibility in code by allowing the same interface to be used for different underlying forms. This reduces the complexity in handling various object types and promotes an adaptive coding environment.
Understanding these core concepts laid out in class-based OOP provides a strong foundation for developers looking to master modern software development techniques. Each component contributes to the overall success of creating scalable, maintainable, and efficient applications.
The Role of Classes in OOP
Classes serve as the blueprint of object-oriented programming (OOP), encapsulating both data and behavior. In many ways, they are fundamental to creating a coherent design in software. Understanding the role of classes can illuminate many complexities in programming and help developers structure their code more effectively.
Classes enable developers to model real-world entities in a more organized manner. Each class represents an abstraction of an entity, defining its properties, behaviors, and interaction with other objects. This object-oriented approach leads to a more intuitive way of thinking about the software architecture, allowing for better problem-solving strategies.
Defining a Class
A class is defined by a unique name and consists of attributes and methods. In programming languages such as Python, Java, and C++, defining a class involves declaring its name, specifying its properties, and defining its functions. For example, in Python, a simple class could look like this:
In this snippet, we define an class with an attribute and a method . This simple definition captures essential characteristics, illustrating how classes facilitate modular programming.
Creating Objects from Classes
Once a class is defined, you can create objects or instances of that class. Each object holds its own set of attribute values while sharing the same methods defined in the class. This feature allows multiple objects to function independently but maintain a similar structure. For example:
Here, and are instances of the class. Each instance can use the same method but operates on its own attributes.
Class Attributes and Methods
Classes can contain attributes and methods. Attributes are variables that store data related to the class, while methods are functions that define the behaviors of the objects. Using the class example, we see:
- Attributes: stores the name of the animal.
- Methods: defines a behavior that produces output based on the object's attributes.
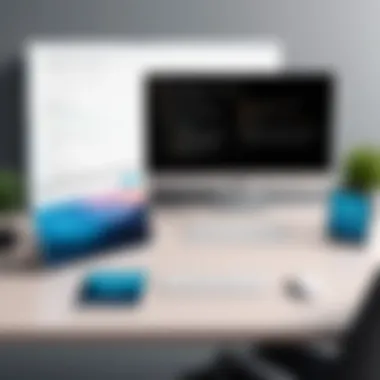
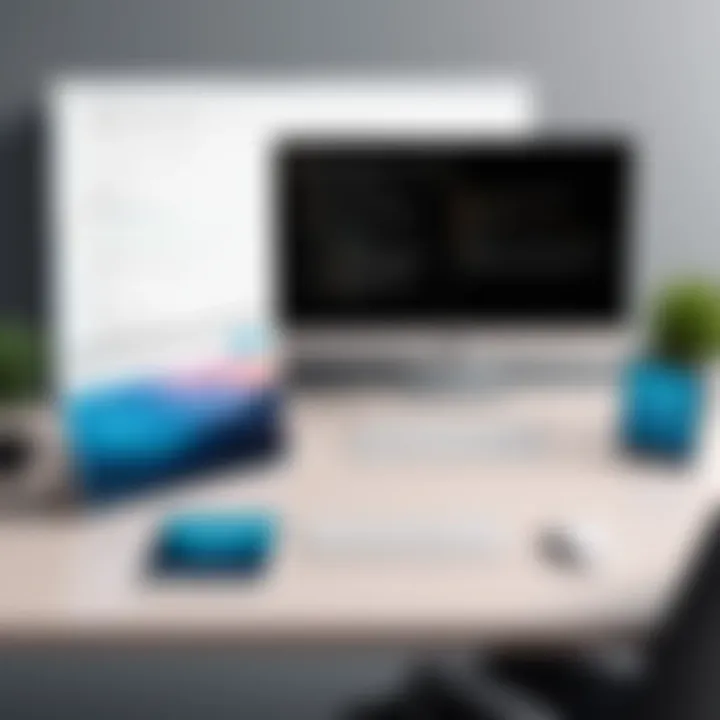
Moreover, classes can have class attributes that are shared among all instances. For instance, if all animals have a common type, it can be defined as:
This encapsulation of both data and functionality streamlines development, enhances code readability, and promotes efficiency. By leveraging classes, developers can build software that is both modular and scalable — leading to not only well-structured but also maintainable code.
"Classes compound the intelligibility of code, encapsulating data and behavior into defined roles."
Benefits of Object-Oriented Programming
Understanding the benefits of Object-Oriented Programming (OOP) is crucial for both new and seasoned developers. OOP enhances the software development process through a variety of principles and practices. These benefits not only improve code quality but also streamline the workflow. This section discusses three main advantages: modularity, reusability, and maintainability.
Modularity
Modularity is a core benefit of OOP. By breaking down complex systems into smaller, manageable sections, a program becomes easier to understand. Each part, or module, corresponds to a class that encapsulates specific functionalities.
The significance of modularity can be observed through the following points:
- Easier Management: Developers can work on different modules without impacting the entire system, which promotes parallel development.
- Simplicity: Understanding a module simplifies debugging and enhances the testing process.
- Collaboration: Teams can collaborate more effectively when working on distinct modules.
Encapsulating properties and behaviors in classes allows for clear separation of concerns. This design principle ultimately supports agile development methodologies, enabling incremental enhancements and adaptations in response to changing requirements.
Reusability
Reusability is another key advantage that OOP provides. Once a class is defined, it can be used as a blueprint to create multiple objects. This reduces redundancy and promotes efficient coding practices.
The benefits of reusability are notable:
- Time-Saving: Developers do not need to rewrite code for common functionalities, leading to faster project completion.
- Consistency: Using the same classes across different projects can lead to consistent behavior and performance.
- Cost-Effective: Fewer resources are required for development and testing, as reused components have already been vetted in previous implementations.
Reusability promotes a culture of building libraries of tested classes. This encourages developers to focus on innovation rather than reinvention of the wheel.
Maintainability
Maintainability is crucial in any software project. With OOP, maintaining software becomes simpler due to the structured approach of organizing code.
Key elements of maintainability include:
- Readability: Well-defined classes and methods improve comprehension for developers, making it easier to navigate the codebase.
- Isolated Changes: Changes can be made to one part of the software without affecting others, reducing the risk of introducing bugs.
- Easier Updates: With modular classes, updating functionality can be performed efficiently.
In summary, the benefits of modularity, reusability, and maintainability significantly enhance the development process in object-oriented programming. By implementing OOP principles, software systems become more robust and agile, thus meeting the demands of modern programming environments.
Key Design Patterns in OOP
Design patterns are essential in object-oriented programming (OOP) as they provide established solutions to common problems that arise during software development. Understanding these patterns enhances the ability to write coherent and sustainable code. By recognizing the different design patterns, programmers can improve efficiency, facilitate communication, and create a more intuitive programming environment.
In the context of class object-oriented programming, design patterns come with several benefits:
- Improved Code Structure: Design patterns serve as blueprints, promoting best practices and leading to cleaner code.
- Enhanced Communication: They provide a shared language among developers, simplifying discussions about solutions.
- Increased Flexibility: Utilizing patterns allows developers to modify existing components without significant rewrites.
- Proven Solutions: Each design pattern is built on experiences from past projects, thus serving as reliable approaches.
These benefits contribute greatly to the overall productivity of development projects and form a crucial part of mastering OOP. In the following sections, we will explore three essential design patterns: the Singleton Pattern, the Factory Method Pattern, and the Observer Pattern.
Singleton Pattern
The Singleton Pattern restricts the instantiation of a class to one single instance. This is important in scenarios where having multiple instances could lead to undesirable behavior or resource conflicts. A common use case is when managing shared resources such as database connections or configuration settings.
Key Characteristics:
- Only one instance exists.
- Global access to the instance.
- Lazy instantiation, which means the instance is created only when it is needed.
Here is an example in Python:
This pattern avoids the overhead of creating multiple instances and can help to manage state effectively.
Factory Method Pattern
The Factory Method Pattern provides an interface for creating objects in a super class but allows subclasses to alter the type of objects that will be created. This pattern is commonly used when a class cannot anticipate the class of objects it must create. Essentially, it promotes loose coupling between client classes and the classes they instantiate.
Key Characteristics:
- Delegates the responsibility of object creation to subclasses.
- Enhances code flexibility and scalability.
A simple example is as follows:
This pattern helps manage object creation processes, making it easier to manage and maintain code as projects evolve.
Observer Pattern
The Observer Pattern is a behavioral design pattern that establishes a one-to-many dependency between objects. When one object changes state, all its dependents are notified and updated automatically. This is particularly useful in scenarios where changes in one component need to reflect in others, such as in graphical user interfaces and event handling systems.
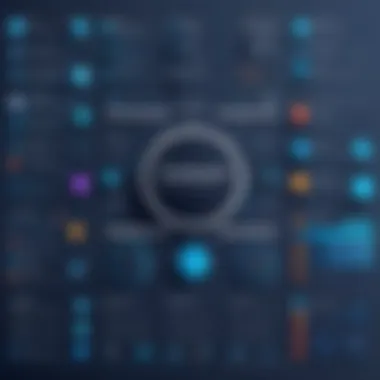
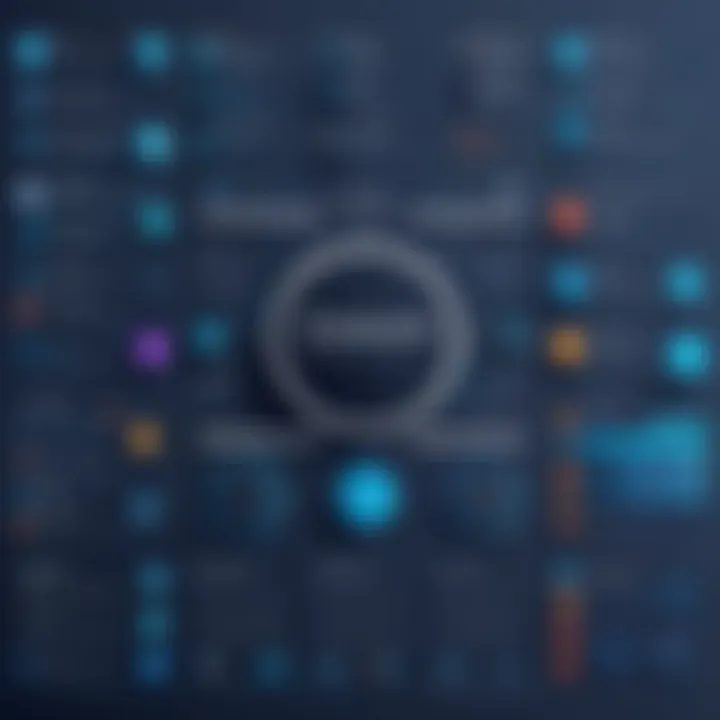
Key Characteristics:
- Defines a subscription mechanism.
- Promotes loose coupling between the subject and observer.
Here is a simplified Python example to illustrate this:
In application, it facilitates easier management of interactions among components. Developers can implement features responsively, leading to enhanced user experience.
In summary, design patterns play a vital role in class object-oriented programming. They lead to cleaner code design, improved communication, and more flexible systems, ultimately resulting in more maintainable applications. By mastering these patterns, programmers can refine their skills and approach problems with more confidence.
Challenges in Object-Oriented Programming
Object-Oriented Programming (OOP) offers several advantages, but it also presents multiple challenges that developers must address. Recognizing these challenges is essential for students, novices, and professionals alike to ensure the effective design and implementation of software systems. Understanding these obstacles is vital not only for improving one’s coding ability but also for mastering the intricacies involved in building scalable and maintainable software using object-oriented principles. This section will focus on two significant challenges: complexity in design and inheritance issues.
Complexity in Design
Designing an object-oriented system can often lead to a high level of complexity. As systems grow, the relationships between classes and objects can become convoluted. A foundational principle of OOP is that it encourages modular design, promoting the use of classes that encapsulate functionality. However, this modularity can also result in intricate interdependencies among classes. It becomes tedious to manage and update these relationships as the project advances. This complexity can lead to several issues:
- Difficulty in Onboarding: New developers joining a project may struggle to understand the connections and the overall architecture of the codebase. Without proper documentation, they may find it hard to get up to speed.
- Debugging Challenges: When systems are interdependent, tracking down bugs can be a daunting task. A change in one class may inadvertently affect others, leading to cascading failures that are difficult to trace.
- Increased Development Time: The more complex a system is, the longer it will take to develop and maintain. Teams may spend unnecessary time trying to resolve complications that arise from intricate class structures.
To mitigate complexity, developers can adopt design principles such as SOLID. These principles serve to keep classes focused on single responsibilities, thus simplifying the design process. Being aware of patterns like Dependency Injection can also help to decouple classes, promoting simpler interactions between them.
Inheritance Issues
Inheritance is one of the most crucial features of OOP. It allows for the creation of new classes based on existing ones. However, inheritance can also lead to significant issues. The primary problems relate to tight coupling and the rigidity it can impose on a design. Some of the key issues include:
- Fragile Base Class Problem: This occurs when changes in the parent class unintentionally break derived classes. If a base class is modified, all its child classes may also require updates, which can introduce bugs and require extensive testing.
- Over-Inheritance: Some developers may adopt an inheritance strategy that leads to multiple levels of inheritance. This can lead to a situation where the derived class can no longer provide the expected behavior of the base class due to accumulated complexity. Therefore, if a class hierarchy is too deep, understanding the full picture becomes problematic.
- Behavioral Conflicts: Not all inherited properties or methods fit well with subclass implementations. When a subclass overrides a method, it may not always maintain the expected behavior, which can cause runtime errors or logic flaws.
To address these issues, alternative strategies like composition over inheritance may be more appropriate. By favoring composition, developers can create more flexible and maintainable systems. This approach often leads to code that is easier to understand and modify over time, reducing the likelihood of complex, tangled hierarchies.
A careful consideration of these challenges ensures that developers can leverage the strengths of OOP while minimizing its drawbacks. Understanding these obstacles can help refine programming practices that enhance code quality and maintainability.
By acknowledging the challenges associated with object-oriented design, programmers can create more robust frameworks that are not only easier to manage but also adaptable to changes in requirements and environment.
OOP in Various Programming Languages
The study of Object-Oriented Programming (OOP) across different programming languages is vital for understanding how class-based principles are implemented and utilized in software development. This section will explore how these principles vary across languages like Python, Java, and C++. Each language has its own syntax and nuances but shares core OOP concepts such as classes, objects, encapsulation, inheritance, and polymorphism. Understanding these specifics can greatly affect how a programmer approaches problem-solving and design patterns.
The benefits of learning OOP in various programming languages include:
- Flexibility in Development: Each language may handle OOP concepts differently, which allows developers to adapt their skills and select the most suitable language for a particular project.
- Enhanced Problem Solving: Understanding multiple implementations of OOP can improve a programmer's analytical capabilities, as they learn various ways to approach a similar problem.
- Broader Job Opportunities: Proficiency in various OOP languages increases employability since many organizations seek developers with diverse programming skills.
OOP in Python
Python is an interpreted, high-level programming language known for its simplicity and readability. OOP is a key feature of Python, and its implementation is quite straightforward. In Python, everything is an object, including functions and classes. Developers can easily define classes using the keyword.
Python supports inheritance and polymorphism as well, making it versatile for a range of applications from simple scripts to complex systems. Its dynamic typing and flexibility allows for rapid prototyping. However, developers should be cautious, as dynamic typing can lead to runtime errors that are hard to debug.
Example of a simple class in Python:
OOP in Java
Java is a statically typed, object-oriented language that emphasizes portability and performance. It enforces strict OOP principles, making it an excellent choice for large-scale applications. Java requires the definition of classes and uses interfaces to implement polymorphism.
In addition to simple inheritance, Java supports multi-level inheritance but lacks multiple inheritance due to ambiguity issues. Java's and keywords provide robust frameworks for building applications that adhere to OOP principles. Exception handling is a notable feature that adds an additional layer of reliability to Java’s OOP implementation.
Java Example:
OOP in ++
C++ expands on the principles of OOP by offering both low-level and high-level features, making it powerful for performance-centric tasks. In C++, classes can hold both data and methods. C++ also allows operator overloading and includes features such as multiple inheritance, which can lead to more complex designs. However, this can introduce complications such as the diamond problem, so careful planning is essential.
C++ supports encapsulation through access specifiers—public, protected, and private—allowing finer control over class functionality. Additionally, polymorphism is achieved using virtual functions, which enable method overriding in an inheritance structure.
C++ Example:
Understanding how OOP principles manifest in various programming languages enriches the developer's toolkit, encouraging a versatile approach to problem-solving.
Ending
In this article, we have delved into various aspects of class-based object-oriented programming (OOP). Understanding OOP is crucial for any software developer, as it offers a structured approach to programming that enhances code organization and management. The importance of this topic cannot be understated, since it lays the groundwork for modern software development.
Summary of Key Points
Key points discussed include:
- Core Concepts: The foundational blocks of classes, objects, encapsulation, inheritance, and polymorphism were explained. These principles form the basis of OOP.
- Role of Classes: We looked into how classes define the blueprint for creating objects and encapsulate data and behavior.
- Benefits: The advantages of OOP, such as modularity, reusability, and maintainability, were highlighted. They contribute significantly to the efficiency and effectiveness of software development.
- Design Patterns: Common patterns like Singleton and Factory Method were introduced, demonstrating practical applications in real-world scenarios.
- Programming Languages: A brief exploration of how OOP is implemented in languages like Python, Java, and C++ showcased its versatility and widespread adoption.
Future Trends in OOP
As technology evolves, the future of OOP does as well. There are several trends to keep an eye on:
- Increased Use of Functional Programming: Many modern languages blend OOP with functional programming, which can streamline processes.
- Microservices Architecture: The shift towards microservices is pushing OOP to adapt, focusing on smaller, isolated services.
- AI and OOP: The integration of artificial intelligence into OOP is anticipated to create smarter, more adaptive systems.
- Enhanced Collaboration Tools: Advances in collaborative programming tools will likely improve how OOP is approached within teams, simplifying code management.