Mastering Coding Patterns for Improved Programming Skills
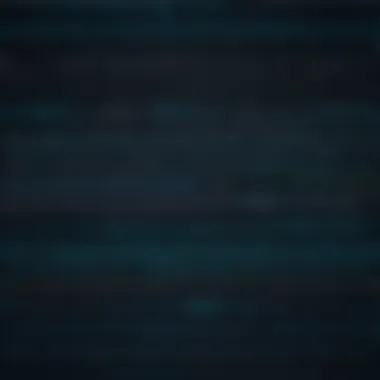
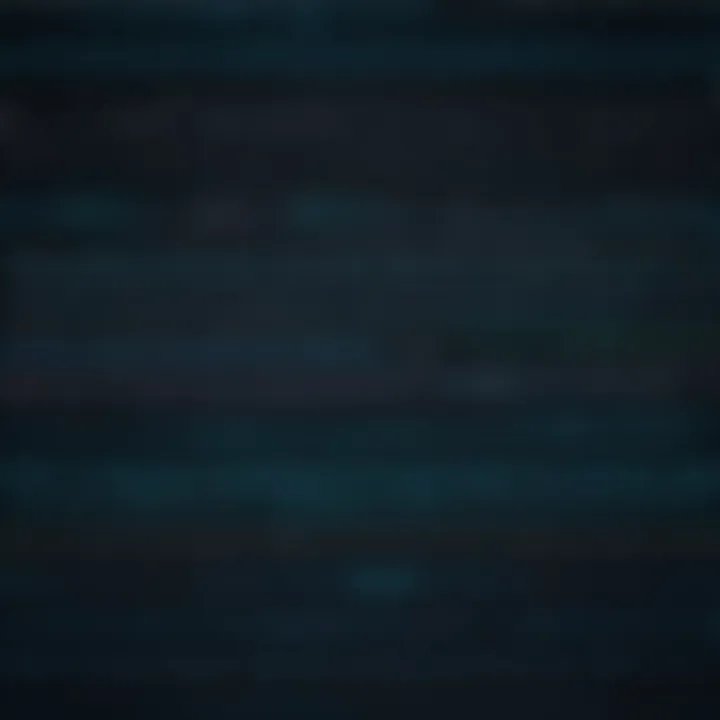
Intro
Coding patterns play a critical role in the realm of programming. They guide developers in solving common problems efficiently and provide a scaffold for clean and maintainable code. In this article, we will dive into the depths of coding patterns, exploring their importance, application, and evolution in software development.
Overview of Topic
Foreword to the main concept covered
At its core, coding patterns encapsulate best practices that have emerged over years of software development. These patterns serve as templates that developers use for solving recurrent programming challenges. Understanding and utilizing these patterns greatly enhances one's ability to write coherent and effective code.
Scope and significance in the tech industry
Figuring out coding patterns is crucial for both novice coders and seasoned professionals. In a rapidly changing tech landscape, their implementation proves fundamental. They foster efficiency and coherence, reducing both development time and effort.
Brief history and evolution
History shows that coding patterns originated from trial-and-error methods used by programmers. Their documentation reached wide recognition with the 1994 book 'Design Patterns: Elements of Reusable Object-Oriented Software' by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides, famously known as the Gang of Four. This monumental work established a solid foundation for identifying common architecture problems and their solutions in software design.
Fundamentals Explained
Core principles and theories related to the topic
Using coding patterns hinges on several core principles. These include reusability, separation of concerns, and abstraction. By understanding these principles, developers can select appropriate patterns that match their coding challenges.
Key terminology and definitions
- Design Patterns: Standard solutions to common problems in software design.
- Levels of Abstraction: Refers to visibility into a code structure, from detailed code up to broader program elements.
- Frameworks: Preceding selections that provide a foundational setup to develop specific projects.
Basic concepts and foundational knowledge
Fundamental knowledge in coding includes the grasp of various programming paradigms such as object-oriented, functional, and procedural programming. These paradigms influence how coding patterns take shape and find utilization.
Practical Applications and Examples
Real-world case studies and applications
Coding patterns are employed across various domains. For example, in web development, the Model-View-Controller (MVC) architecture methodically separates concerns, enabling better organization and scalability.
Demonstrations and hands-on projects
A simple use case involves the Singleton pattern, which restricts a class to a single object. Here’s how you might implement it in Python:
Code snippets and implementation guidelines
Using snippets like these not only speeds up coding but also maintains consistency and a clean codebase. Review and adapt it based on requirements specific to your project.
Advanced Topics and Latest Trends
Cutting-edge developments in the field
With the growth of Artificial Intelligence and Machine Learning, understanding patterns becomes even more pivotal. As algorithms get more complex, employing efficient coding patterns ensures that maintainability is not compromised.
Advanced techniques and methodologies
Some leading edge methodologies include Event-Driven Architecture and Microservices, both relying on robust patterns to manage interactions in distributed systems.
Future prospects and upcoming trends
Future innovations may introduce more advanced patterns tailored for big data and IoT. As technology evolves, it remains vital for developers to stay informed and adapt to emerging practices and patterns.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
- Design Patterns by Erich Gamma et al.
- Coursera courses on Software Design Patterns.
Tools and software for practical usage
Several integrated development environments (IDEs) like IntelliJ IDEA or Visual Studio facilitate the application of coding patterns through snippets, templates, and guidance, ensuring that common issues are proactively addressed.
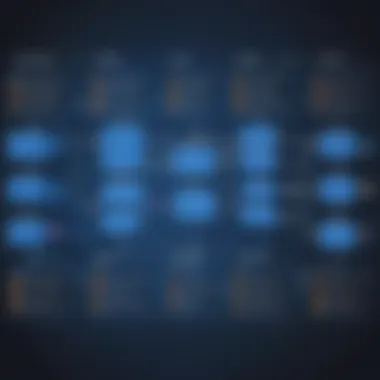
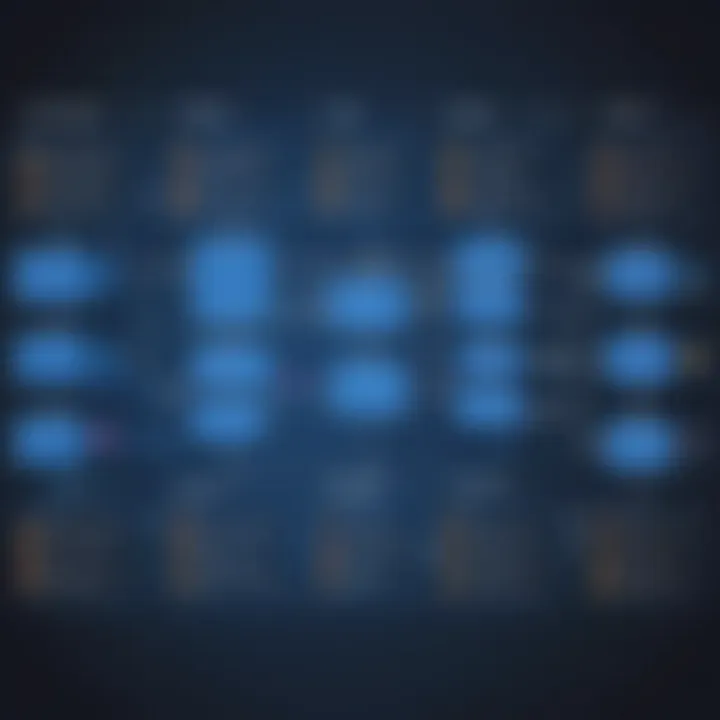
Understanding coding patterns equips individuals with a powerful approach for structuring complex solutions. Each facet of this exploration strengthens programming skills and helps in crafting reliable code across various projects.
Foreword to Coding Patterns
Coding patterns are essential framework within software development. They provide solutions to common programming issues. This section explores the importance of knowing these patterns. It showcases their practical applications and their value for both new learners and experienced professionals.
Defining Coding Patterns
Coding patterns are established solutions to recurring design problems within software. They encapsulate best practices that developers can employ. The main gain is to enhance code quality. Every coding pattern addresses specific scenarios. Therefore, recognizing these structures can speed up coding processes. There are three major types of coding patterns: creational, structural, and behavioral. Each category serves distinct functionality. For instance, creational patterns focus on object creation mechanisms. This gives more control over the types of objects being created.
Key points in defining coding patterns include:
- Patterns assist in solving design issues efficiently.
- They encapsulate best practices from extensive programming experiences.
- Understanding the purpose behind each pattern enhances problem-solving capabilities in coding.
Historical Context
The concept of coding patterns has roots in software engineering that traces back to design methodologies from the 1990s. Notably, the book Design Patterns: Elements of Reusable Object-Oriented Software authored by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides, laid out a framework for understanding these models. It looked at how developers could reuse software architectures effectively.
Patterns arose from observing success stories prominent software projects. Developers realized shared problems gave birth to reused solutions, adopting a language to convey complex concepts simply.
The historical advancements have emphasized repeated patterns in programming. In doing so, developers build upon existing knowledge rather than creating solutions from scratch. This fundamentally evolves software engineering practices into systematic, predictable approaches.
Types of Coding Patterns
Coding patterns are foundational frameworks in software design. They provide a systematic approach to solving common programming challenges. Knowing the different types of coding patterns enhances a programmer's ability to write flexible and efficient code. Notably, these categories help in organizing code structures effectively.
Creational Patterns
Creational patterns focus on the process of object creation. They abstract the instantiation process, making it easier to create generic objects in a dynamic fashion. They primarily help avoid tight coupling between the program and the created objects.
Singleton Pattern
The Singleton Pattern restricts an object to a single instance. This is crucial when one instance is sufficient for the application’s needs. Its key characteristic is its control over instance creation. Thus, it is useful in situations requiring global access and data sharing across the application. For instance, a configuration manager in an app often benefits from this pattern, reducing resource usage. However, it may introduce issues like global state, complicating unit testing.
Factory Pattern
The Factory Pattern provides a way to create objects without specifying the exact class of object that will be created. The main advantage here is the flexibility it gives developers in substituting different implementations with ease. This pattern promotes encapsulation and reduces dependencies in the code. It is especially beneficial in frameworks where various object types may be instantiated. A disadvantage might be the increase in complexity from maintaining factory methods, which can lead to a more extensive code base.
Prototype Pattern
The Prototype Pattern enables creating new objects by copying an existing object. This is helpful in scenarios requiring many similar object instances while conserving system resources. Its special feature is the ability to reduce the overhead of object creation and initialization, thus saving valuable system resources. However, implementing a prototype might lead to complications if the object-to-copy contains cyclical references.
Structural Patterns
Structural patterns ensure that when individuals combine objects, they maintain flexibility and efficiency even when changing code. They provide easy access to objects across hierarchies.
Adapter Pattern
The Adapter Pattern facilitates direct communication between two incompatible interfaces. It essentially turns the interface of a class into another interface that a client expects. A notable benefit includes fostering compatibility between systems and libraries that otherwise do not work together. However, overly elaborate adapter implementations can lead to maintainability issues over time.
Composite Pattern
Composite Pattern treats individual objects and composites uniformly. This is useful for representing hierarchical tree structures where individual and composite classes process requests indistinctively. It's particularly beneficial for graphical applications requiring the manipulation of complex structures. But this may lead to execution concerns as all components need to support a common interface, potentially leading to performance lags.
Decorator Pattern
The Decorator Pattern allows adding new functionality to existing objects dynamically. For example, decorating a window object in a graphical user interface with additional features like borders or shadows facilitates code flexibility. Its strength lies in enhancing object behavior transparently. However, managing multiple decorators can complicate the application's operations since debug information may be more distributed.
Behavioral Patterns
Behavioral patterns are critical for defining communication between objects, facilitating collaboration, screen splitting, etc. They specify how objects interact in a system.
Observer Pattern
The Observer Pattern provides a subscription mechanism to inform multiple objects about state changes. A highlight of this pattern is its ability for lazy updates, ensuring that observers only act on state changes when needed. For systems requiring dynamic updates—like event handling—the Observer Pattern is extremely beneficial. Conversely, maintaining the consistency across observers can sometimes become a challenge as the number of observers rises.
Strategy Pattern
The Strategy Pattern defines a family of algorithms and makes them interchangeable. This allows clients to vary their algorithm to suit specific needs without altering its context. The primary advantage is that the separation of concerns allows for a cleaner design. However, excessive strategy implementation can introduce complexities that may bog down understanding.
Command Pattern
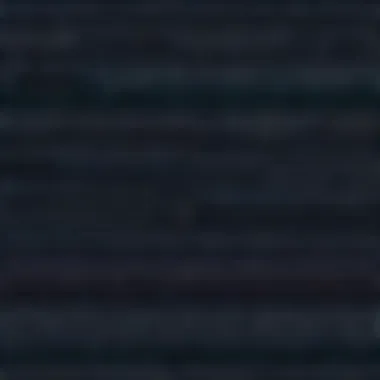
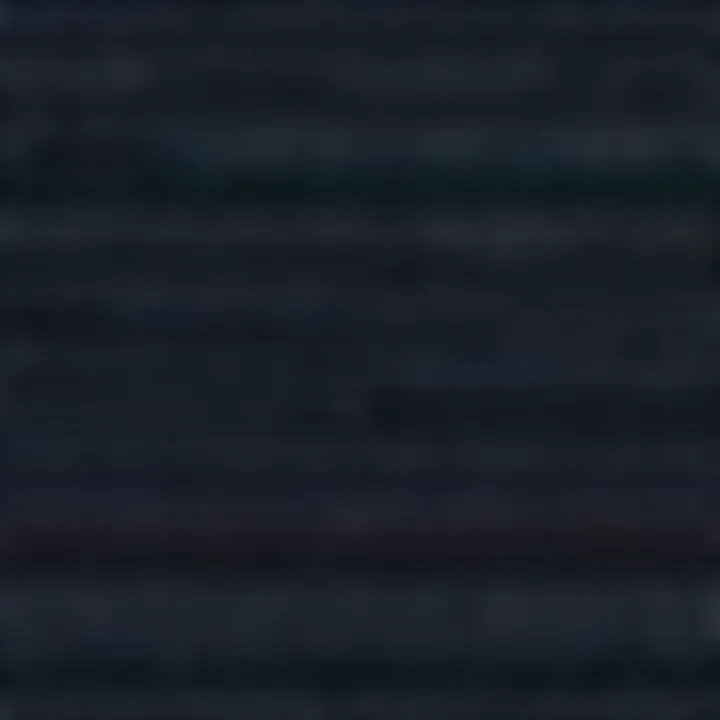
The Command Pattern encapsulates requests as objects. This enables parameterization of clients with queues, requests logging, and support for undoable operations. Its capability to manage parameterization can significantly enhance functionality in software systems. However, it may add oversights in simple implementations, potentially leading to security risks if all commands accessible remain incorrect or rogue commands.
Understanding these patterns nurtures improved programming skills and efficiency. As we venture deeper into their practical applications, the routes become more vivid for developers.
Importance of Coding Patterns
Coding patterns play a significant role in software development. They offer a structured approach to programming, solving complex problems in a standardized manner. Recognizing and employing these patterns can vastly improve various aspects of code quality. Understanding non-static methods of coding is essential. Doing this leads to better outcomes in projects, enhancing overall coding practices.
Enhancing Code Maintainability
Code maintainability is the ease with which a codebase can be modified. Incorporating coding patterns promotes clear and consistent code. Patterns often encapsulate best practices. This means that other developers can understand the intentions behind the code, making it more straightforward to manage changes or updates. For instance, implementing the Singleton Pattern can guarantee that a class has only one instance. This pattern enhances the system's organization, leading to reduced errors when adjustments need to be made. Furthermore, maintainable code typically leads to fewer bugs, making life easier for developers.
Improving Code Reusability
Another key benefit of coding patterns is their impact on code reusability. When code is structured using established patterns, components become easier to reuse. This approach to programming saves time and effort on future projects because general solutions can be adapted rather than engineered from scratch. The Factory Pattern exemplifies this as it allows for the creation of objects without exposing the instantiation logic. Resultantly, coded solutions become versatile, fostering innovation and reducing redundancy. Reusable code enhances the development process.
Facilitating Collaboration
Practical Applications of Coding Patterns
The application of coding patterns can profoundly affect the efficacy of projects. Proper use of these designs can result in cleaner, more efficient code. As a programmer or an IT professional, understanding how to implement these patterns is crucial for achieving optimal outcomes in software production.
In Software Development
Coding patterns are particularly significant in software development. They simplify complex problems by offering structured designs. For instance, using the Singleton Pattern can ensure that a class has only one instance and provide a global point of access to it. This is vital in instances where a single instance is necessary to coordinate actions.
It is also critical to apply the Factory Pattern appropriately. This pattern allows for the creation of objects without specifying the exact class of object that will be created. Thus, you can write code that is flexible and allows developers to add new types of products without modifying existing code. Such adaptability is essential in modern software development, as requirements can frequently change.
Benefits of Coding Patterns in Software Development:
- Maintainability: Development teams can easily update and maintain the code.
- Readability: Clear structure improves code comprehension.
- Collaboration: Different team members can push for solutions without duplicated efforts.
In Web Development
Web development also leverages coding patterns extensively. The Adapter Pattern is a good example, as it allows incompatible interfaces to communicate. This is especially important in web applications that integrate various APIs. By using the Adapter Pattern, developers manage changes in external APIs easily and ensure stability of the application.
Moreover, Structural Patterns like the Composite Pattern enable the development of complex user interfaces. By structuring elements hierarchically, a web developer can treat individual objects and compositions uniformly. This leads to more manageable and scalable interface designs.
Specific Aspects Benefiting from Coding Patterns in Web Development:
- Efficiency: Reduces code duplication.
- Modularity: More manageable chunks of code to test.
- Scalability: Flexibility to incorporate new features.
In Data Science
The relevance of coding patterns extends to data science as well. Here, coding patterns allow teams to innovate by making prototypes faster through the Prototype Pattern. With this, teams can create prototypes early on without creating complex variants of the final products, thereby enhancing workflow efficiency.
Another instance is using the Strategy Pattern, which allows different algorithms to be selected and implemented at run time. This is beneficial in data science when establishing models or approaches for solving problems, making the data processing versatile and adaptive to various datasets or objectives.
Advantages of Implementing Coding Patterns in Data Science:
- Adaptability: Easier when working with different data models.
- Optimization: Simpler configurations for varying approaches.
- Efficiency in Code: Less redundancy in code bases.
In sum, coding patterns provide clearer structures and significantly enhance teamwork capabilities across all fields of computer science. It is essential for both new and experienced professionals to familiarize themselves with these practical applications.
Recognizing Patterns in Coding
Recognizing patterns in coding is crucial for any programmer, whether novice or experienced. Patterns empower a programmer to identify common challenges in software development, leading to more efficient solutions. Understanding these patterns can significantly shorten development time and enhance code quality. By noticing recurring frameworks, developers can reduce errors and improve maintainability.
Pattern Recognition Techniques
Effective pattern recognition involves several techniques that help programmers distinguish different coding strategies. Here are some common methods:
- Review Classifications: Familiarizing oneself with various patterns helps in early identification. For example, knowing the differences between Singleton and Factory patterns can aid in faster decision-making.
- Implementation Analysis: Analyzing existing codebases provides insight into how similar problems are addressed. This examination prompts the discovery of effective coding patterns used by others.
- Visual Aids: Flowcharts and Diagrams are beneficial when mapping out ideas or solutions. Visualizing the structure may highlight underlying patterns quickly.
Observing one's own code over time also helps in recognizing habitual patterns one tends to employ. This self-awareness can refine a programmer's skill set further, enhancing their approach towards complex problems.
Transitioning from Theory to Practice
Transitioning from theory to practice is an essential phase in the learning curve of coding patterns. In an environment driven by technology, theoretical knowledge alone is often insuffcient, as practical application helps solidify one’s understanding and use of these patterns in real-world scenarios. By bridging this gap, programmers can align theoretical concepts with tangible examples, leading to enhanced problem-solving skills and better coding practices.
Implementing Coding Patterns in Projects
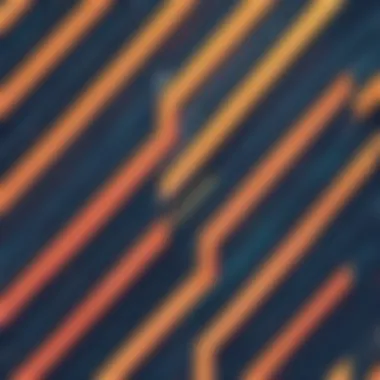
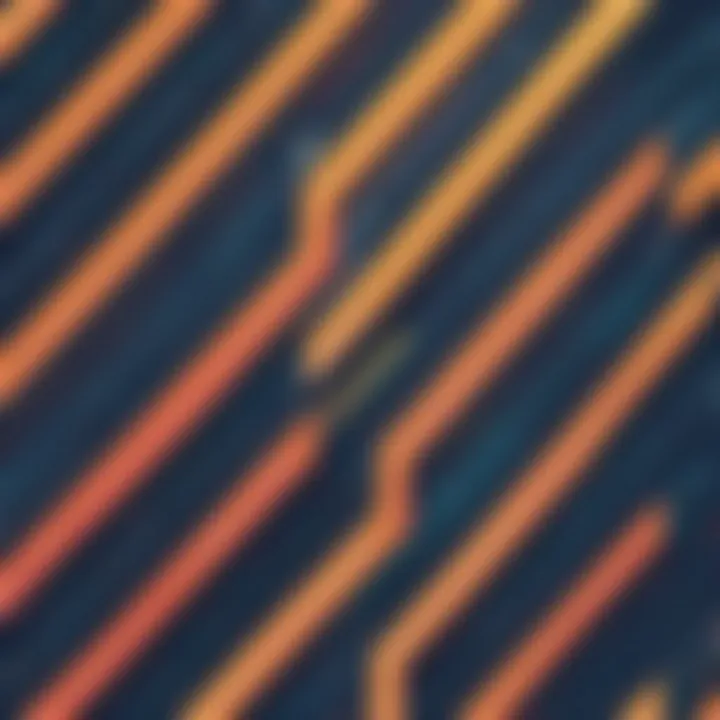
Effective implementation of coding patterns within projects requires a systematic approach. It is crucial to assess the landscape of the project and identify pain points where coding patterns can offer solutions. For starters, developers should prioritize adding patterns that increase project maintainability and adaptability. Familiarity with patterns like the Singleton or Factory can help streamline the instantiation of classes when they are critical to the system's architecture.
Consider the following steps for effective implementation:
- Assess Requirements: Before choosing a coding pattern, clarify project requirements.
- Choose Suitable Patterns: Based on project specifications, select the pattern that merges best with current task flows. For example, if managing state across multiple components, the Observer Pattern can be particularly useful.
- Integrate Gradually: When adopting several patterns, out of concerns regarding code complexity, start by integrating one pattern at a time.
- Review Sources: Utilize resources like en.wikipedia.org or other technical forums where coding conventions are discussed.
Through careful planning and realizing the context for utilizing coding patterns, the convenience gained can lead to a smoother project life cycle.
Testing and Refining Patterns
Once coding patterns are implemented, the dialogue surrounding testing and refining becomes paramount. This practice guarantees functionality, reliability, and efficiency within the project context. Testing identifies discrepancies between expected and actual achieveable outcomes, providing loading grounds for enhancement. Two distinct practices can aid in this phase:
- Unit Testing: Conduct tests specifically targeting functions utilizing coding patterns. For example, unit tests on a Factory can ensure correct object instantiation.
- Refinement Through Feedback: Continuous refinement under real user scenarios is vital. Gather insights from team members and clients post-implementation.
Regular reflection on how well patterns serve their purpose not only solidifies team dynamics but raises the intentionality of coding practices used.
Effective practical implementation is key to mastering coding patterns; it compels both understanding and flexibility for students and professionals alike.
In the not-too-distant future of programming, adopting coding patterns will differentiate efficient code from mediocre. Complexity often escalates in software due to scaling, hence learning these transitions strengthen one’s foundation in programming.
Resources for Further Study
The pursuit of knowledge in coding patterns does not conclude with this article. Continuous learning is essential in the fast-paced world of programming. Resources for further study objectively play a critical role in enriching understanding and advancing skills. These resources allow individuals, from students to seasoned programmers, to delve deeper into various aspects of coding patterns. They also facilitate exposure to differing perspectives, potentially leading to new insights and advancements in personal coding practices.
Beyond basic reading materials, incorporating diverse study formats can significantly enhance learning efficiency. Engaging with specialized books and literature offers theoretical depth. While engaging with online courses might yield practical insights through experiential learning.
Lastly, active participation in community forums works as a means for fresh ideas and solving real-time coding challenges. Personal engagement in such platforms provides opportunities to network and learn from peers. The significance of dedicated and targeted learning resources cannot be overstated. Below, we will errors not addressed during the initial learning process and enhance the knowledge in coding patterns.
Books and Literature
Books provide a wealth of knowledge and understanding, especially regarding coding patterns. The structured approach that books offer often allows readers to return to specific topics as needed. Some must-read titles include:
- Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides. It offers insights into classical design patterns.
- Head First Design Patterns by Eric Freeman and Bert Bates. This book engages with a user-friendly approach that caters to different learning styles.
- Clean Code: A Handbook of Agile Software Craftsmanship by Robert C. Martin. Here, principles describing clean coding encourage utilizing various patterns reflecting maintainable code.
Each book contains extensive examples that practically enhance the reader’s ability to recognize and employ various coding patterns effectively.
Online Courses and Workshops
Online courses and workshops provide flexible learning tailored to new trends and modern coding techniques. Platforms like Coursera, Udacity, and edX frequently update their content to address contemporary technological demands.
Conducting an online search can yield several coding pattern courses. Here’s what to consider when selecting:**
- Look for courses with practical datasets and code samples that learners can manipulate.
- Select platforms offering interactive learning segments, providing immediate engagement and feedback.
- Verify the course reviews and ratings to ensure quality content.
Popular choices include:
- Codecademy and Udemy often include workshops on design patterns in various programming languages, offering direct guidance from industry professionals.
- Pluralsight, renowned for focusing on coding video tutorials, contains an array of content on coding patterns complete with assessments.
Community Resources and Forums
In addition to structured learning resources, community engagement can greatly supplement one’s educational journey. Websites such as Reddit has several dedicated programming communities focused on coding patterns, where users share valuable insights, experiences, and solutions.
Benefits of participating in community forums are many:
- Access practical knowledge from experienced developers, who often share real-world experiences.
- Ability to discuss challenges and solutions through peer support, making resolving issues easier.
- Networking with like-minded individuals can lead to collaboration on projects or further learning opportunities.
Some important forums include Reddit (in particular the r/programming subreddit) and Facebook groups designated for coding education. Engaging in discussions there can nurture a more nuanced understanding of coding patterns.
Engagement in community resources can bridge the gap between foundational knowledge and real-world application, significantly benefiting the coder's growth journey.
Closure: Embracing Coding Patterns
Coding patterns are essential in the world of programming. They provide frameworks that support developers in making their code more meaningful. Embracing coding patterns not only enhances an individual's ability to write organized and efficient code, but it also lays a foundation for robust software architecture. This conclusion highlights the reasons why integrating these patterns into daily coding practices benefits programmers at all levels.
Summary of Key Insights
Throughout the exploration of coding patterns, some key insights emerged:
- Maintainability: Coding patterns significantly improve the maintainability of software. With established patterns, future developers can understand the existing code with less effort, making updates or modifications less problematic.
- Reusability: The design of coding patterns encourages the reuse of solutions and code. Developers can create modules that are useful across various projects, saving time and ensuring consistent quality.
- Collaboration: Patterns foster a common language among diverse teams. By using the same coding patterns, team members can collaborate seamlessly, reflecting a unified approach to coding challenges.
- Learning Curve: For beginners, familiarizing oneself with coding patterns expedites the learning process. Instead of being overwhelmed by chaotic code structures, newcomers gain immediate access to proven designs and ideas that aid in their education.
“> Using coding patterns allows us to communicate complexities effectively, thus minimizing confusion and errors in a collaborative environment.”
Future Directions in Coding Patterns
The evolution of coding patterns will align with the changes in technology and software engineering. Below are some future directions:
- Integration with AI and Machine Learning: Coding patterns will likely evolve alongside advancements in AI. Future coding patterns may optimize themselves in response to handling massive data more flexibly, signaling efficient methodologies relevant to emerging tech.
- Increased Focus on Security: As cyber threats grow, new coding patterns addressing security from the foundational level will likely emerge. Ensuring application integrity and safe user experience will become a primary concern for developers.
- Adoption of Concurrent Processing Patterns: As systems increasingly require concurrency, coding patterns targeting this necessity will develop further, assisting developers in making software more responsive and efficient.
Coding patterns play a vast role in shaping the workflows and outputs in programming. As such, continual exploration and adoption are crucial to remain relevant in an ever-evolving field.