Understanding C++ Challenges and Effective Solutions
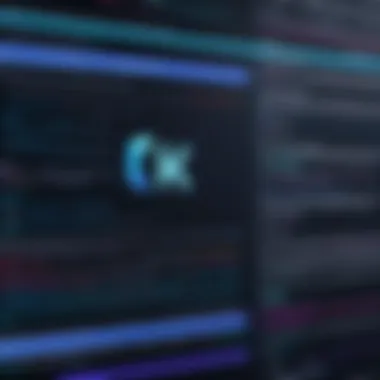
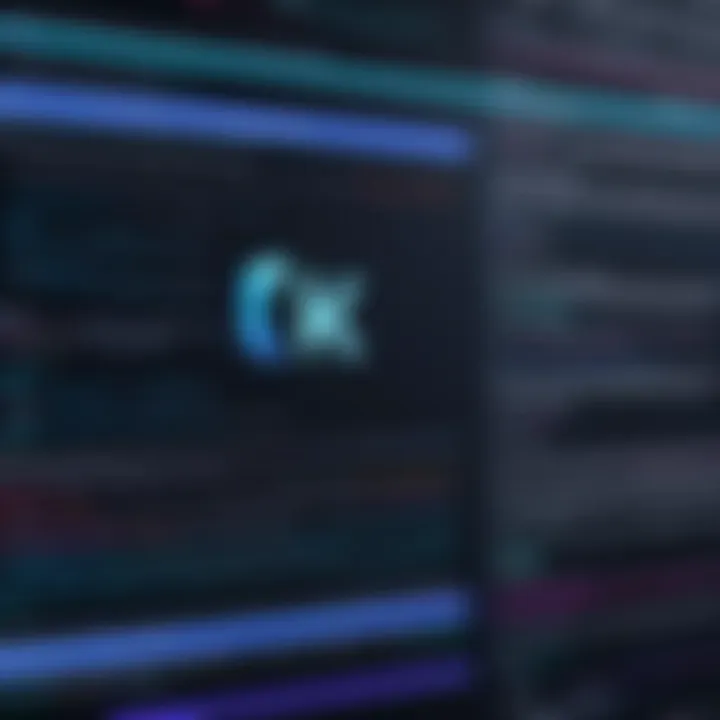
Overview of Topic
C++ is a language that has a significant impact on modern programming. Many developers face challenges while working with it, ranging from memory management issues to more complex design patterns. This article looks at common problems programmers encounter in C++ and explores different solutions. Understanding these issues is essential as they can influence the performance and reliability of software applications.
C++ has evolved since its creation in the early 1980s. Over the decades, it has become a staple in systems programming, game development, and high-performance applications. The languageβs unique features pose both advantages and challenges. Analyzing these difficulties can help enhance the skills of students and IT professionals alike.
Fundamentals Explained
This section introduces core principles related to C++ programming. Understanding memory management is crucial. Memory leaks occur when programs do not release the allocated memory, causing resource drainage and performance degradation.
Key terminology includes:
- Pointers: Variables that store memory addresses.
- Classes: Blueprints for creating objects.
- Templates: Tools for creating functions or classes that work with any data type.
Familiarity with these basic concepts provides a strong foundation for addressing more advanced issues.
Practical Applications and Examples
Real-world applications of C++ can be seen across various fields. For instance, in game development, C++ is the backbone of many game engines. Understanding these applications offers insight into C++ challenges.
Consider this code snippet that demonstrates basic class usage:
By crafting simple examples, learners gain hands-on experience which makes difficult concepts easier to grasp.
Advanced Topics and Latest Trends
As technology evolves, so does C++. New features and best practices are constantly being introduced. Examples include move semantics and smart pointers, which help manage memory more effectively. Additionally, the trend toward concurrent programming means that programmers face new challenges regarding synchronization and resource sharing.
Future prospects indicate that C++ will continue to thrive in areas requiring high performance and direct hardware manipulation.
Tips and Resources for Further Learning
For those looking to deepen their knowledge, the following resources are invaluable:
- Books:
- Online Courses:
- "The C++ Programming Language" by Bjarne Stroustrup
- "Effective C++" by Scott Meyers
- Coursera and Udemy offer C++ programming courses.
Tools like Visual Studio and JetBrains CLion streamline the coding process, allowing for more effective debugging and code management.
Through awareness of common challenges, developers can enhance their C++ skills and produce more reliable software.
Prolusion to ++ Problems
C++ programming is both powerful and complex. Understanding C++ problems is crucial for anyone wishing to master this language. Familiarity with challenges can lead to more efficient coding and problem-solving strategies. By recognizing common issues, programmers can create smoother applications and avoid pitfalls.
Significance of Understanding ++ Challenges
Recognizing the challenges in C++ is not just about overcoming errors. It allows developers to improve their coding discipline. Understanding various problems, such as memory management and debugging, can enhance the overall quality of code. Debugging can consume a significant amount of time. Knowing potential bugs and issues in advance can lead to more effective solutions and save time in the long run.
Common Misconceptions about ++
Many people think C++ is only suitable for system programming and high-performance applications. However, it has many applications, including game development and financial systems. Another misconception is that C++ is overly complex, which can discourage new learners. The truth is, it's a language that offers a lot of control, which can be incredibly empowering and beneficial for developers.
Some may believe that once they learn C++, they can write complex programs without issues. This is a common fallacy. All programming languages come with a set of challenges. Mastering C++ includes continually learning about its quirks and limitations.
In summary, understanding C++ problems equips programmers to tackle real-world challenges effectively. Misconceptions should be corrected, as they can limit oneβs approach to learning and application of the language.
"Understanding the challenges of C++ is the first step toward mastering it."
Thus, educating oneself on these challenges can ultimately result in personal growth and professional success.
Categories of ++ Problems
C++ programming is marked by its complexity and versatility. As with any sophisticated language, it has its own set of problems that can hinder development. Identifying the categories of C++ problems is essential for programmers at any skill level. This knowledge not only speeds up the debugging process but also aids in developing better coding practices. Each category presents unique challenges that, if left unresolved, can lead to significant product failures or inefficiencies.
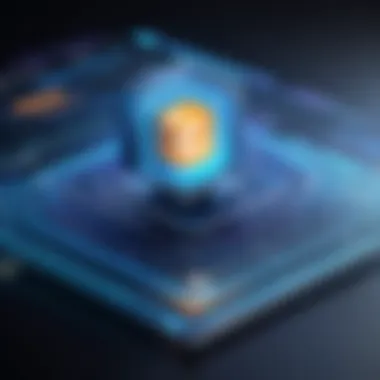
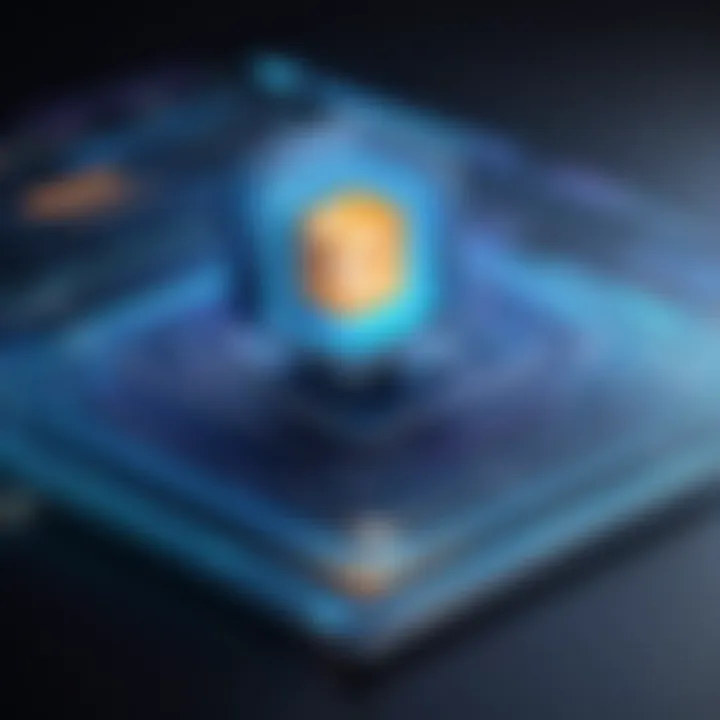
Syntax Errors
Syntax errors occur when the code violates the grammatical rules of C++. These are usually detected during compilation. The compiler provides error messages that point to the line where the issue exists. While often straightforward to fix, they can lead to frustration if not addressed promptly. Common examples include missing semicolons, mismatched parentheses, or incorrect keywords. It's crucial to carefully read and interpret compiler warnings to mitigate these issues effectively.
Logic Errors
Logic errors are somewhat insidious. They occur when the code compiles and runs, but the output is not what the developer intended. These errors stem from flawed reasoning or incorrect assumptions about how code segments interact. For instance, using an incorrect variable in a calculation may lead to unexpected results. Searching for logic errors can be challenging as they do not generate error messages. Instead, implementing comprehensive test cases and reviewing logic flow is beneficial in identifying these issues.
Runtime Errors
Runtime errors happen during program execution. Unlike syntax errors, these issues are not apparent at compile time. They may arise from operations such as dividing by zero, accessing out-of-bounds array elements, or accessing null pointers. These errors can cause program crashes or unexpected behavior in applications. Employing robust error-checking mechanisms and thorough testing can help to catch runtime errors before they cause problems in a production environment.
Memory Leaks
Memory management is crucial in C++ since it does not have automatic garbage collection. Memory leaks occur when dynamically allocated memory is not freed, leading to resource exhaustion over time. Monitoring tools can assist in detecting these leaks. Best practices such as smart pointers and RAII (Resource Acquisition Is Initialization) can help manage memory more effectively. Recognizing potential leak points is key to writing performant applications.
Debugging Issues
Debugging is an inevitable part of the development process. Various issues can arise that complicate debugging efforts, from compiler optimizations to varying environments. Programmers often face challenges in debugging multithreaded applications due to their complexity. Familiarity with debugging tools like GDB or integrated development environments (IDEs) can streamline this process. Creating a systematic approach to debugging, such as isolating problems or using breakpoints, may lead to quicker resolutions.
Understanding these categories of problems helps developers not only to avoid common pitfalls but also enables them to develop higher-quality, more reliable code.
Diagnosing ++ Problems
Diagnosing C++ problems is a crucial aspect of software development. Understanding how to effectively identify and resolve issues can significantly enhance the quality of the final product. This section explores three key methods: utilizing compiler error messages, employing debugging tools, and analyzing code structure. By mastering these techniques, programmers can save time and effort, while also reducing frustration and errors in their coding endeavors.
Utilizing Compiler Error Messages
Compiler error messages serve as the first line of defense when it comes to diagnosing problems in C++. They indicate syntax errors, type mismatches, and other issues that can prevent code from compiling. By understanding and interpreting these messages, programmers can quickly pinpoint where the problems lie.
Paying close attention to the line numbers indicated in the error messages is vital. This allows for a more straightforward approach to fixing issues. For instance, if the compiler indicates an error in line 42, start your analysis from this point instead of scanning through the entire codebase.
Common compiler errors include:
- Syntax errors, such as missing semicolons or unmatching braces.
- Type-related issues, like incorrect variable declarations.
Although compiler error messages may seem daunting at times, they are invaluable in guiding developers towards the appropriate solutions.
Employing Debugging Tools
Debugging tools are essential for diagnosing more complex issues that compiler messages may not reveal. These tools allow programmers to step through their code line by line to observe the program's behavior during execution. Two widely used debugging tools in C++ include gdb and Visual Studio Debugger.
When using these tools, you can:
- Set breakpoints to pause execution at specific lines of code.
- Evaluate the values of variables at various execution points.
- Trace through function calls to understand the flow of the program.
By employing these debugging techniques, programmers can gain insights into how their code is functioning. This can be especially useful for tracking down elusive logic errors or memory leaks that are not immediately apparent.
Analyzing Code Structure
The structure of the code plays a significant role in how easily problems can be diagnosed. Well-structured code is easier to read, maintain, and debug. Consider the following practices for optimizing code structure:
- Use meaningful variable and function names that convey their purpose clearly.
- Follow consistent formatting and indentation rules to enhance readability.
- Break down large functions into smaller, more manageable pieces.
Additionally, implementing comments throughout your code can clarify the intent behind specific sections. This not only benefits others who may read the code but also aids in your understanding when revisiting the codebase.
In summary, careful analysis of code structure can simplify the debugging process. A well-organized structure reduces the cognitive load when diagnosing issues.
"A stitch in time saves nine" β addressing problems early can prevent more complex ones from arising down the line.
Common ++ Problems and Solutions
In the landscape of C++ programming, understanding common problems and their accompanying solutions is vital. Each challenge a programmer faces can lead to significant hurdles in developing robust and efficient applications. Addressing these issues not only enhances individual coding skills but also contributes to the overall quality of software engineering practices. This section emphasizes the importance of identifying key problems, their solutions, and how one can effectively navigate through them.
Handling Array Index Out of Bounds
One prevalent error in C++ programming arises from array index out of bounds access. This occurs when a program attempts to access an index of an array that is either negative or greater than the size of the array. Such errors can lead to unpredictable behavior, program crashes, and data corruption.
To mitigate this issue, programmers should ensure proper checks before accessing an array element. For example, implementing conditions that verify if an index falls within valid limits can prevent runtime errors. Furthermore, consider utilizing standard library features like instead of raw arrays. Vectors provide bounds checking through methods like , reducing the likelihood of out-of-bounds access, thus enhancing code reliability.

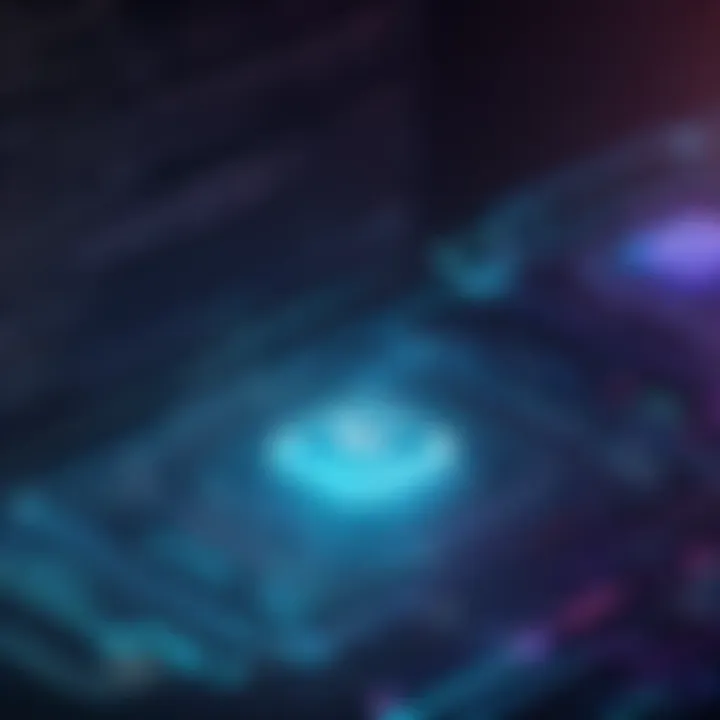
Addressing Null Pointer Dereferencing
Null pointer dereferencing represents another critical issue in C++. A null pointer points to no valid memory location, and attempting to dereference it can lead to a crash or undefined behavior. Factors contributing to this challenge often include improper initialization or failure to check pointer validity before usage.
To address this problem effectively, always initialize pointers. Use nullptr in modern C++ to denote a null pointer, making it clear when pointers are not yet assigned valid addresses. Moreover, applying checks before dereferencing guarantees that the pointer is not null. This practice significantly enhances the resilience of the application.
Managing Resource Allocation and Deallocation
Effective management of memory is a cornerstone of C++ programming. Problems with resource allocation and deallocation can lead to memory leaks or fragmentation. When dynamic memory is allocated using , a corresponding must be called to release it. Failing to do so consumes memory unnecessarily until the program terminates.
Employing smart pointers such as or can help automate resource management. These pointers manage memory automatically, thus minimizing the risk of leaks. By transferring ownership and controlling lifetimes, programmers can ensure optimal resource utilization while focusing more on logic rather than manual memory handling.
Tip: Always match every with a and each with a to uphold proper memory management practices.
In summary, by understanding these common C++ problems and implementing effective solutions, developers can cultivate better programming habits. Addressing array index limitations, managing pointers responsibly, and optimizing memory allocation are all essential components in writing efficient and stable C++ applications.
Best Practices in ++ Programming
Best practices in C++ programming are essential for ensuring code quality, maintainability, and efficiency. When programmers adhere to these practices, they can not only avoid common pitfalls but also foster a more collaborative and productive environment. Good practices enable clear communication among team members and decrease the time spent on debugging and maintenance. In a complex language such as C++, established practices become even more crucial due to its rich feature set and potential for pitfalls. Therefore, exploring these practices helps engineers strengthen their skills and advance their understanding of software development.
Writing Clean and Maintainable Code
Writing clean code is about clarity and simplicity. It should be easy to read and understand the intent behind each piece of code. Clear code reduces the risk of errors and misconceptions. When programmers return to their own code or another developer's work, they should immediately grasp what the code does and why.
Some key strategies to write maintainable code include:
- Consistent Naming Conventions: Use a uniform style for naming variables and functions. Descriptive names help convey the purpose immediately.
- Modularization: Break down large programs into smaller functions or classes. This makes testing and updates easier.
- Commenting Wisely: Include comments where necessary, but avoid redundancy. Code can often be self-explanatory; excessive comments can clutter it.
- Code Reviews: Regular reviews among peers can unveil issues and provide different perspectives.
By implementing these strategies, programmers create a foundation for code that is not only functional but also sustainable, fostering an ecosystem of continuous improvement.
Utilizing Standard Library Functions
The Standard Template Library (STL) in C++ is a robust repository of functions and data structures that can significantly enhance programming efficiency. Utilizing STL functions reduces the amount of code that developers need to write while ensuring reliability and performance.
Some benefits of using standard library functions include:
- Time Efficiency: Prebuilt functions allow developers to accomplish tasks without reinventing the wheel.
- Performance Optimization: STL functions are well-tested, meaning they often provide optimized performance compared to custom implementations.
- Code Consistency: Using standard functions promotes uniformity, allowing others to follow and understand the code more easily without needing extensive documentation.
It is advisable to thoroughly understand commonly used STL components such as vectors, lists, and maps. This knowledge allows developers to leverage them effectively rather than relying on more complex solutions.
Implementing Effective Error Handling
Error handling in C++ requires careful consideration to ensure robustness in code execution. Errors can occur due to a range of reasons from user input issues to unexpected system behavior. Effective error handling prevents program crashes and leads to a better user experience.
Key considerations for implementing error handling include:
- Exception Handling: Use , , and blocks to manage exceptions. This structured approach isolates error handling from normal code flow.
- Use of Assertions: Assertions help catch logic errors during development by validating conditions before proceeding.
- Logging Errors: Maintain logs of errors and exceptions to analyze patterns and identify problem areas in the code.
- Graceful Recovery: Plan for how your program can recover from errors. This may involve default behaviors or user prompts.
Implementing robust error handling not only prevents program failure but instills confidence among users and stakeholders, demonstrating that the application can handle unexpected scenarios gracefully.
"Effective programming is not merely about writing code; it encompasses foresight and care in managing the complexities of interactions that occur within the code."
In summary, adhering to best practices in C++ programming enhances the quality of the software being developed. From writing clean and maintainable code to effectively utilizing standard library functions and implementing error handling, these practices contribute significantly to professional development and the long-term success of programming projects.
Advanced ++ Challenges
In C++ programming, advanced challenges emerge as languages and software develop. Understanding these challenges is vital because they can significantly impact program performance, maintainability, and extensibility. As projects grow in complexity, programmers must navigate intricate concepts such as concurrency, templates, and object-oriented design. Each of these elements demands careful consideration to avoid pitfalls and achieve effective solutions. With thorough knowledge about advanced C++ challenges, developers can create robust applications that meet the demands of modern computing tasks.
Dealing with Concurrency Issues
Concurrency issues refer to problems that arise when multiple threads operate simultaneously on shared resources. This poses risks such as race conditions, deadlocks, and thread contention. Managing concurrency is crucial for performance optimization and resource management.
For example, consider a situation where multiple threads attempt to modify a shared data structure. Without proper synchronization mechanisms, the program may produce unpredictable results. To combat this, C++ provides tools like mutexes, condition variables, and atomic operations.
Here are several key strategies to deal with concurrency:
- Use Mutexes: Mutexes help in locking critical sections of code, ensuring that only one thread can access shared resources at a time.
- Avoid Deadlocks: Design systems to prevent deadlocks by establishing strict locking orders.
- Employ Lock-Free Algorithms: In certain scenarios, lock-free data structures can improve performance by reducing the overhead of locks.
By grasping these concepts, developers enhance the stability and reliability of concurrent applications.
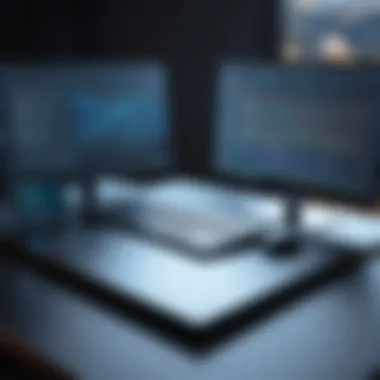
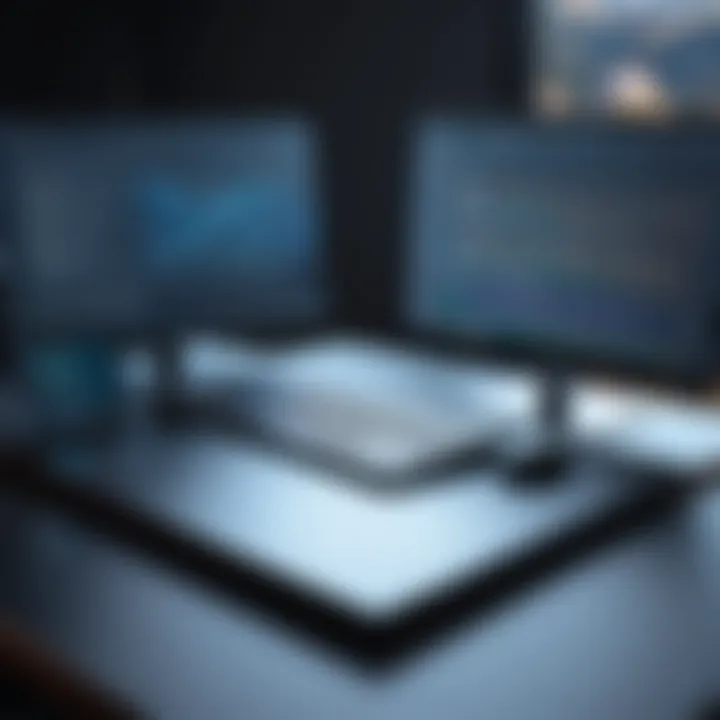
Understanding Templates and Metaprogramming
Templates in C++ allow for the creation of generic programming solutions. Metaprogramming takes it further by enabling programs to generate or manipulate code at compile time. Both techniques improve code reuse and flexibility but also introduce complexity.
Templates facilitate the development of functions and classes that can operate with different data types without rewriting code. For instance, a single template function can handle various data types like integers or floating-point numbers. This reduces code duplication and enhances maintainability.
In contrast, metaprogramming can be particularly useful for optimizing performance or enforcing constraints at compile time. However, it might also obfuscate the code, making it harder for others to read and understand.
Some considerations include:
- Use Type Traits: Type traits can determine properties of types during compilation, allowing for more efficient code generation.
- Check Template Instantiation: Ensure templates are instantiated only when necessary to minimize compile times.
- Maintain Readability: Keep template code manageable to prevent confusion, as excessive metaprogramming can make debugging cumbersome.
Ultimately, a sound understanding of templates and metaprogramming can lead to significant improvements in code quality and performance.
Navigating Object-Oriented Design Pitfalls
Object-oriented programming (OOP) in C++ provides a framework for organizing software design around objects. However, it comes with its own set of challenges. Understanding these pitfalls is crucial for fostering sound design principles.
Common issues include:
- Inheritance Misuse: Overusing inheritance can create tight coupling and introduce fragility to the design. Favor composition over inheritance when possible.
- Memory Management: Handling dynamic memory through pointers can lead to leaks if not managed correctly. Utilize smart pointers like and to mitigate these risks.
- Overcomplication: Sometimes developers create overly complex class hierarchies that complicate understanding. Keep designs simple and intuitive.
Focusing on clarity and simplicity in object-oriented design ensures that code remains manageable. This benefits not only the creator but also the maintainers in the long run.
Keep in mind that no challenge is insurmountable with the right knowledge and tools. Addressing advanced C++ challenges head-on strengthens not just your coding abilities but also your understanding of foundational programming concepts.
Resources for ++ Problems
When it comes to solving C++ problems, having access to the right resources is crucial. This section discusses valuable tools and references that can greatly aid developers at all skill levels. Utilizing these resources can help address challenges in C++ programming effectively. Moreover, they serve as a foundation for improving coding skills, troubleshooting issues, and enhancing overall understanding.
Online Community and Forums
Participating in online communities and forums can be an excellent way for C++ programmers to seek help and share knowledge. Websites like Reddit host numerous programming subreddits where learners and experienced developers engage in discussions. Platforms such as Stack Overflow provide quick answers to specific coding questions.
Benefits of these communities include immediate feedback and the possibility to connect with other programmers sharing similar challenges. Learning from real-world examples and solutions that others have encountered can greatly enhance understanding. It is important to contribute back to these communities by answering questions or sharing insights. This creates a cycle of learning that benefits everyone.
Recommended Literature and Documentation
Reading well-regarded literature and documentation is essential for mastering C++. Texts such as "The C++ Programming Language" by Bjarne Stroustrup offer foundational knowledge. Complementing this with resources like the ISO C++ standards documentation can provide clarity on language specifications.
Some other notable titles include:
- "Effective C++" by Scott Meyers
- "C++ Primer" by Stanley B. Lippman
- "The C++ Standard Library" by Nicolai M. Josuttis
Moreover, referring to online documentation from reliable sources can help clarify specific topics or functions. The official documentation from cppreference.com is incredibly valuable for understanding C++ libraries and frameworks.
Tools and Software for ++ Development
Using the right tools can greatly influence the ease and efficiency of programming in C++. IDEs like Visual Studio and Code::Blocks streamline the development process. These environments provide features such as code completion, syntax highlighting, and debugging capabilities that can save time and help avoid mistakes.
Additionally, using version control systems like Git can be indispensable for managing code changes and collaborating with others. With tools such as CMake or Make, building projects becomes more manageable.
In summary, incorporating these resources into everyday programming practice contributes significantly to overcoming challenges in C++. With a blend of community support, literature, and proper tools, developers can navigate the complexities of C++ more effectively.
Culmination
The conclusion of this article is fundamental as it synthesizes the insights gathered from various discussions on C++ problems and their solutions. Understanding these issues is not merely about recognizing the symptoms but digging deeper into their causes. This article has navigated through the landscape of common challenges in C++, from syntax errors to nuanced debugging issues, providing a framework for strategic problem-solving.
A key benefit of this understanding is the enhancement of programming proficiency, which can significantly impact both individual projects and team endeavors. By internalizing the best practices outlined, readers can improve code quality, reduce bugs, and accelerate development cycles. Additionally, being aware of advanced challenges in C++, crucial for seasoned programmers, positions them to better address concurrency problems and template metaprogramming intricacies.
Considerations for the future revolve around the increasing complexity of software systems and the evolving nature of development environments. As C++ continues to innovate, staying abreast of emerging trends and practices will be essential for programmers who aim to remain competitive in the industry. This conclusion serves not only as a summary but also as a call to action for continuous learning and adaptation in the realm of C++ programming.
Summary of Key Concepts
Throughout this article, we have explored various dimensions of C++ programming challenges. Key takeaways include:
- Error Types: Common errors such as syntax, logic, and runtime errors were examined, highlighting their impact on coding efficiency.
- Debugging Strategies: Tools like gdb and insights into error messages empower developers to troubleshoot effectively.
- Resource Management: The significance of managing memory allocations to avert memory leaks was emphasized.
- Best Practices: Techniques such as writing clean code and utilizing the standard library were discussed to promote maintainability.
This summary reaffirms the idea that a systematic approach to understanding and addressing C++ challenges promotes not only better software solutions but also fosters a deeper engagement with the language itself.
Future Trends in ++ Programming Challenges
Looking ahead, several trends are poised to shape the future landscape of C++ programming challenges. First, the rise of concurrent programming will demand a stronger emphasis on safe thread management and synchronization techniques. As systems become more distributed, programmers must adeptly handle the complexities of multi-threading, ensuring data integrity and consistency.
Moreover, the integration of templates and metaprogramming into C++ offers powerful tools for abstraction and code reuse but poses complexity challenges. Future developers will need to master these features to leverage their full potential while mitigating pitfalls associated with template instantiation and compilation times.
Finally, the community and ecosystem surrounding C++ are evolving. Resources such as online forums on platforms like Reddit and updated libraries from repositories will be essential for support. Continuous professional development and engagement with emerging technologies will keep programmers at the forefront of innovations in C++. As such, embracing a culture of learning and sharing will be crucial for overcoming future C++ challenges.