Understanding C# as an Accessible Language
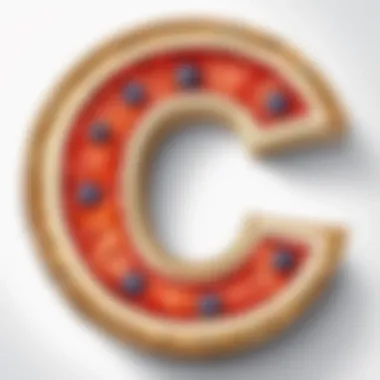
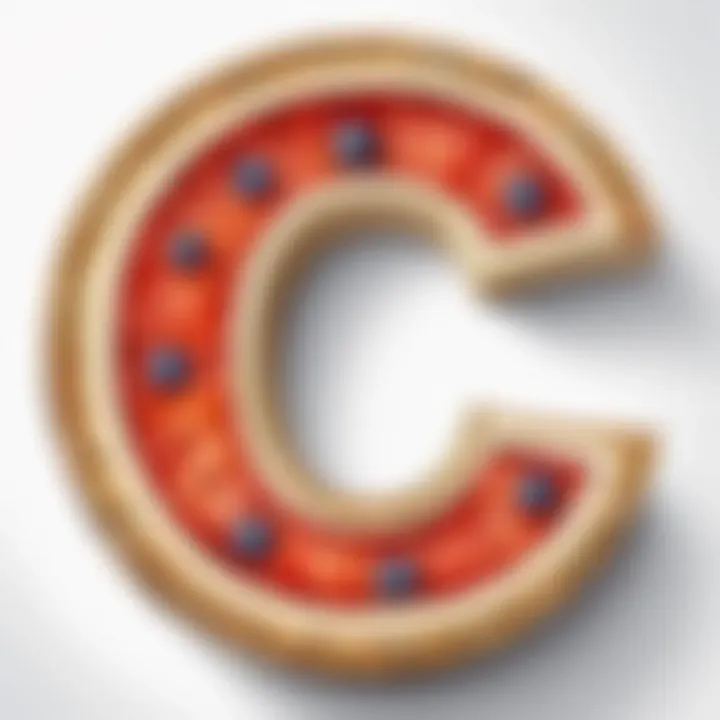
Overview of Topic
C# is a multi-paradigm programming language developed by Microsoft. It is one of the main languages used for developing applications on the .NET framework. C combines high-level programming features with strong type checking and automatic garbage collection. Its structure makes it both powerful and accessible, making it suitable for a wide range of applications from web services to game development.
In today's tech industry, C# holds a significant position due to its versatility and ease of use. It simplifies the process of coding, which can otherwise be daunting for beginners. Consequently, many educational institutes incorporate C into their curriculum. Understanding its evolution gives insight into how it has adapted to meet modern programming needs.
Fundamentals Explained
Core Principles and Theories
C# is rooted in several core programming principles, such as object-oriented programming (OOP). OOP focuses on using objects to represent data and functions. Key concepts include encapsulation, inheritance, and polymorphism. These principles enable programmers to create modular and reusable code, which is essential for managing complex applications.
Key Terminology and Definitions
Before diving deeper, it helps to understand some basic terms.
- Class: A blueprint for creating objects.
- Object: An instance of a class.
- Method: A function that is defined in a class.
- Variable: A container used to store data values.
Basic Concepts and Foundational Knowledge
C# employs a syntax that is similar to Java and C++. This similarity helps beginners transition to C from other programming languages. The basic structure of a C program includes namespaces, classes, and methods. Understanding these components is critical for writing functional code.
Practical Applications and Examples
C# has a broad spectrum of practical applications in various domains.
- Web Development: Using ASP.NET, developers can create dynamic websites. Here is a sample code snippet for a simple web server:
- Game Development: C# is widely used in game engines like Unity. It provides a rich set of libraries for graphics, sound, and control.
Advanced Topics and Latest Trends
As technology continues to evolve, C# also adapts. Recent trends include enhancements in performance, security, and cross-platform capabilities. .NET Core and the transition to MAUI (Multi-platform App UI) are examples of how developers can create applications that work on different platforms using C#. Advanced topics also explore asynchronous programming, which allows for building responsive applications that can handle multiple tasks effectively.
Tips and Resources for Further Learning
To become proficient in C#, numerous resources are available. Here are some recommendations:
- Books: “C# in Depth” by Jon Skeet and “Head First C#” by Andrew Stellman.
- Online Courses: Platforms like Coursera or Udemy offer C# programming courses.
- Tools: Visual Studio provides a robust environment for coding in C#. It includes features like IntelliSense, debugging tools, and a rich library of resources.
Furthermore, communities on sites like Reddit can provide support and additional learning opportunities. Engaging with others who are also learning can enhance understanding and retention.
C# is often recommended for beginners due to its clear syntax and ease of understanding.
Preamble to
C# is a powerful programming language that finds its place in many aspects of software development. Understanding C is pivotal for anyone venturing into the field of programming. Its design philosophy aims to be simple yet effective, making it a particularly attractive choice for beginners. C stands out with its versatility, being used in web development, desktop applications, game development, and more.
Familiarity with C# equips newcomers with essential skills useful across multiple domains. Its structure and design cater to the needs of budding programmers, allowing them to grasp crucial programming concepts efficiently.
Moreover, the language benefits from strong community support and extensive resources that ease the learning process. With a logical syntax and a focus on readability, C# ensures that learners can concentrate on logic and application rather than becoming bogged down by complicated code.
This section outlines the foundational elements necessary for understanding C# and sets the stage for delving deeper into its features and applications.
The Origin of
C# was developed by Microsoft in the early 2000s as a reaction to the demand for a modern, object-oriented language. The first version was released in 2000 alongside the .NET Framework. It aimed to combine the power of C++ with the ease of use found in languages like Visual Basic.
The core team, led by Anders Hejlsberg, sought to create a language that included strong type checking, garbage collection, and support for both object-oriented and component-oriented programming. As a result, C# evolved into a robust tool that enhances productivity without sacrificing control over the system.
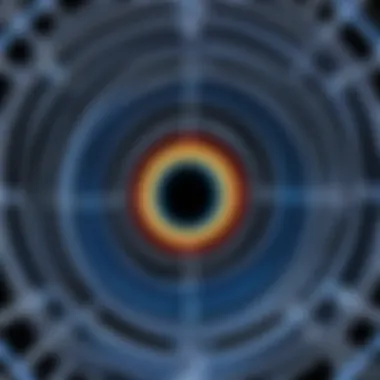
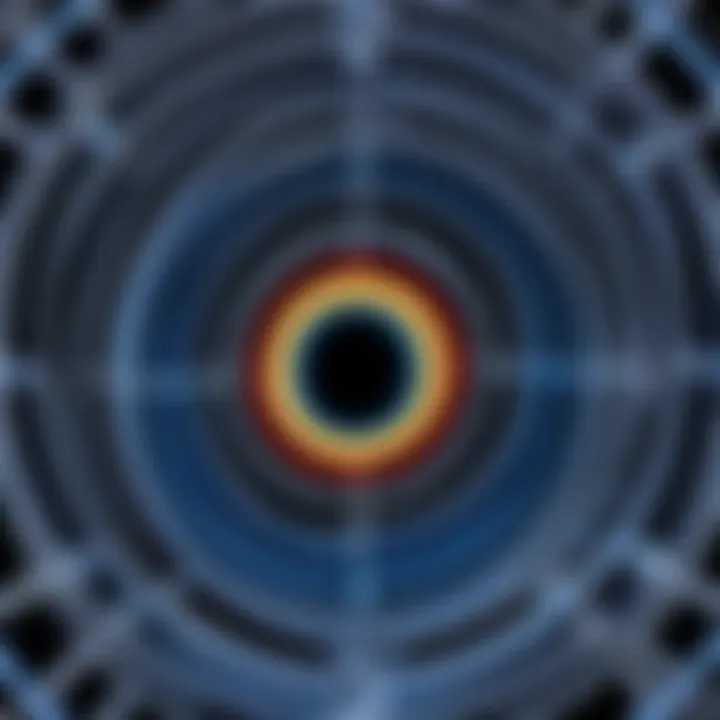
Over the years, C# has seen numerous updates, each adding new features and improving performance. The release of C 2.0 introduced generics, which allowed developers to define type-safe data structures. Subsequent versions continued to enhance its capabilities, making it a steadily evolving language suitable for modern programming needs.
Purpose and Scope of
The purpose of C# is diverse, encompassing various programming paradigms while making sure that it remains accessible to newcomers. It serves as a general-purpose language that fits well in different environments, especially with the .NET ecosystem. This adaptability means that programmers can write applications for web, desktop, and mobile platforms with a single language.
C# enables the development of efficient and secure applications, highlighting its relevance in enterprise-level programming. With built-in features that promote code reusability and maintainability, C encourages best practices that result in cleaner, more organized code.
Furthermore, its integration with Microsoft technologies makes C# a preferred language for Windows development, contributing to its widespread use across industries. In education, C is often recommended as an introductory language because it balances power and simplicity seamlessly, ensuring that beginners can transition to advanced topics progressively.
Core Principles of
C# is designed with specific principles that contribute to its usability and effectiveness, particularly for beginners. Understanding these core principles is essential to grasp how C functions and why it is frequently chosen for various development tasks. The main attributes that define C include its object-oriented nature, strong typing, and the Common Language Runtime, all of which play significant roles in shaping the experience of learning and using the language.
Object-Oriented Programming
Object-Oriented Programming (OOP) is a core principle that makes C# appealing and accessible for learners. OOP allows developers to model real-world scenarios through objects, which represent instances of classes. Each class can have its own attributes and methods, making it easier to understand and manage code structure. The benefits of learning C within the OOP paradigm include:
- Modularity: Code can be broken down into smaller, manageable parts, which promotes easier debugging and testing.
- Reusability: Once a class is created, it can be reused in different parts of an application or even in different applications, saving time and effort.
- Encapsulation: This principle hides the internal state of an object, exposing only necessary parts of it, which enhances security and reduces complexity.
- Inheritance: It allows a new class to inherit properties and methods from an existing class, enabling a natural hierarchy of classes.
These concepts minimize the learning curve and allow developers to build upon existing knowledge as they progress.
Strongly Typed Language Characteristics
C# is a strongly typed language, which means that each variable must be declared with a specific data type. This characteristic is crucial for several reasons. First, it helps prevent errors that can arise from unintended type conversions, such as treating a number as a string. This reduces run-time errors, making debugging less challenging. Second, the compiler provides immediate feedback, guiding learners to understand type constraints and their implications in programming.
The strong typing feature of C# promotes good coding practices by enforcing clarity in code. Below are some key points:
- Variables must be defined before use, which leads to better code organization.
- Type-checking guarantees that operations are valid for the data types involved, enhancing code reliability.
- Understanding data types and their interactions is fundamental for manipulating data effectively.
Common Language Runtime (CLR)
The Common Language Runtime is another defining characteristic of C#. It serves as the execution engine for .NET applications, providing a host of services that simplify the development process. Understanding CLR is crucial for beginners because it abstracts many complex details of programming. Key functions of CLR include:
- Memory Management: It handles memory allocation and garbage collection, allowing developers to focus on writing code rather than managing memory manually.
- Exception Handling: CLR provides a structured way to handle runtime errors, making programs more robust and reducing the chance of application failure.
- Interoperability: CLR allows C# applications to work seamlessly with other languages within the .NET framework, promoting flexibility and integration.
The integration of CLR in C# provides a safety net for beginners, allowing them to learn programming fundamentals without getting overwhelmed by low-level details.
Syntax and Structure
Syntax and structure form the backbone of C#. Understanding these elements is essential for anyone diving into the language. The simplicity and precision of C#'s syntax can significantly lower the barriers for beginners. It helps convey the logic of programming clearly, making it easier to learn. C# maintains a clean syntax that eliminates unnecessary complexity, which is particularly essential for newcomers.
The structure of the C# language supports enhanced readability. Each command or expression is organized systematically. This facilitates both learning and coding. Errors are easier to locate and fix. Therefore, grasping syntax and structure encourages effective programming habits early on. Below, we will discuss the fundamental aspects of C syntax in detail.
Basic Syntax Overview
C# has a straightforward syntax that resembles other C-based languages, like C++ or Java. This resemblance is beneficial for learners, especially those who may transition to C from these languages later. Each C program must contain at least one class and one method. The main method is the entry point for any C application, called . Here is a simple example:
This example outlines the essentials: a directive, class declaration, and the method. Notice how keywords are intuitive. \n In C#, case sensitivity is crucial. Variable names, method names, and even types must be used precisely as defined. Misnaming can result in errors that can frustrate beginners. Adhering to conventions, like using camel case or Pascal case, also enhances clarity in code.
Control Structures
Control structures in C# guide the flow of execution in a program. They facilitate decision-making and repetition, which are core to creating functional applications. Key control structures include conditional statements (if, switch) and loops (for, while). A concise example of a conditional statement is:
In this instance, the code evaluates a condition based on the variable. The logic is simple and clear, allowing beginners to apply it easily in various contexts. Also, loops like and enable iteration, allowing repetitive actions without redundancy. Learning these structures is vital for efficient programming and fosters logical thinking.
Data Types and Variables
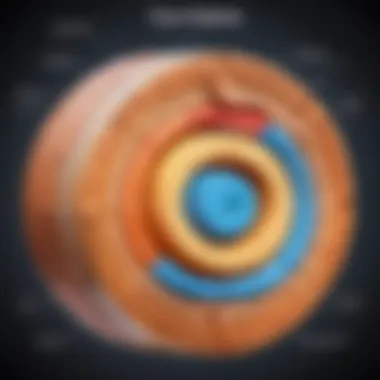
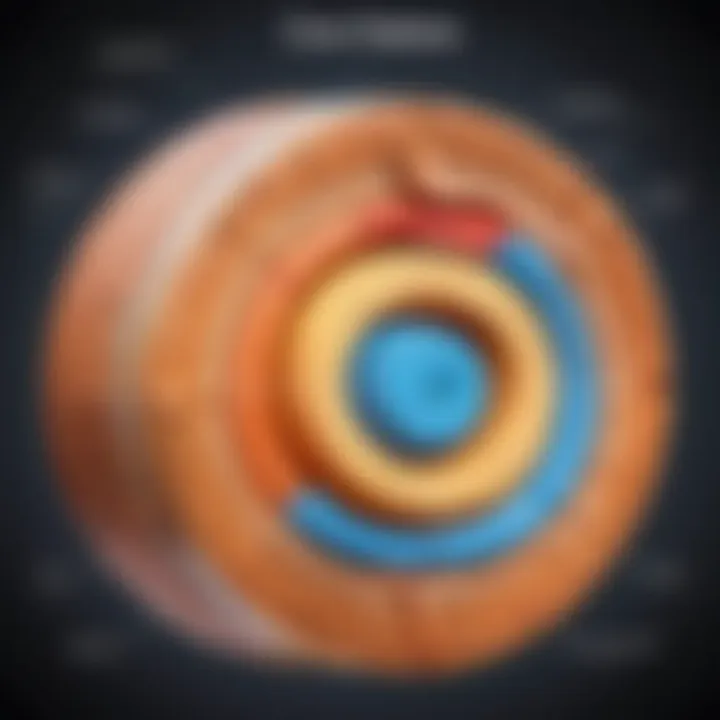
In C#, data types determine the kind of data a variable can hold. Understanding this concept is fundamental. C# supports several built-in data types, including integers, doubles, and strings. For example:
Each variable is declared with a specific type, which enhances memory management and type safety. This strongly typed nature means errors can be caught at compile time rather than runtime.
Moreover, C# utilizes type inference, which simplifies variable declaration. For instance:
Using , the compiler determines the type based on assigned value. Understanding data types and effective use of variables is central for beginners. It lays the foundation for more complex programming techniques.
Mastering C# syntax and structure will elevate your coding abilities, making it an excellent choice for those starting their programming journey.
Advantages of Learning
C# is not just another programming language; it provides a multitude of advantages that make it appealing for both beginners and seasoned developers. Understanding these advantages can give learners a clearer perspective on why investing time in mastering C is worthwhile. Its wide application, solid integration with Microsoft technologies, and the active community supporting it are key elements that contribute to its popularity.
Wide Application in Software Development
C# enjoys a wide range of applications across various domains in software development. From desktop applications to web services, C is versatile. It powers game development, particularly through the Unity platform, which is one of the most popular game engines available. Using C#, developers can create graphics-intensive games and applications with relative ease.
In addition to gaming, C# is extensively used in enterprise software development. Many businesses utilize C for building robust Windows applications, ensuring quality and performance. Moreover, with the rise of cloud technologies, C connects seamlessly with Microsoft's Azure services, enabling scalable solutions that meet modern needs. As C integrates with numerous APIs and frameworks, it positions itself as an accessible language for a myriad of project types on multiple platforms.
Integration with Microsoft Technologies
C# is ideally positioned within the Microsoft ecosystem. This integration introduces both advantages and convenience for learners. For instance, it works effortlessly with the .NET framework, allowing easy access to a wide variety of libraries and tools designed to enhance productivity.
Moreover, C# is used extensively in developing Windows applications and services, aligning perfectly with the broader Microsoft development environment, including Azure and Visual Studio. Students and new developers can easily find resources and documentation on platforms like en.wikipedia.org). The cohesive nature of Microsoft technologies simplifies the learning curve and opens doors to various career opportunities.
Active Community and Support
One of C#’s significant advantages is its vibrant and active community. This community forms a crucial resource for learners. Whether facing a coding error or seeking advice on best practices, platforms like reddit.com provide forums for discussion and troubleshooting.
Online communities, including forums, blogs, and social media groups like those found on facebook.com, foster collaboration and knowledge sharing. The collective experience can help beginners find answers quickly and encourage peer support. The prevalence of tutorials, videos, and articles makes it easier to learn at one’s own pace. This support network boosts confidence and enhances the overall learning experience.
"The strong community surrounding C# not only helps beginners overcome initial challenges but also propels them toward becoming proficient developers."
Learning Resources
In any learning process, the importance of well-structured resources cannot be understated. For individuals exploring C#, the right learning materials make a marked difference in comprehension and retention of concepts. These resources include books, online courses, community forums, and official documentation, all contributing to a thorough understanding of C# programming. Utilizing a variety of these resources helps to accommodate different learning styles and preferences, ultimately enhancing the learning experience.
Books and Online Courses
Books offer a foundational understanding of C#. Many beginners find comfort in the structured approach of written text, which often provides a chapter-by-chapter breakdown of topics. Notable titles include "C# in a Nutshell" by Joseph Albahari and "Head First C#" by Andrew Stellman and Jennifer Greene. These books not only cover syntax and semantics but also delve into practical examples, reinforcing theoretical knowledge.
Online courses provide interactive experiences. Platforms like Coursera and Udemy offer courses tailored to various skill levels, ranging from beginner to advanced. These courses often include hands-on projects that enable learners to apply their knowledge in real-time. The visual and audio elements in online courses can aid significantly in retaining information and understanding complex concepts.
Online Communities and Forums
Community engagement is essential for programming learners. Platforms such as Reddit's r/csharp and specialized forums on Stack Overflow provide spaces where novices can ask questions and seek guidance from experienced developers. These communities are invaluable for troubleshooting and acquiring tips and tricks that may not be covered in traditional learning materials.
The knowledge sharing in these spaces introduces learners to diverse perspectives and solutions. Participating in discussions can also spark motivation and help maintain enthusiasm for learning C#. Engaging with peers and experts can lead to networking opportunities, beneficial for future career prospects.
Documentation and Tutorials
Official documentation is a primary resource for understanding the intricacies of C#. Microsoft offers comprehensive documentation at docs.microsoft.com. This resource covers everything from basic principles to advanced functionalities. Tutorials often accompany documentation, providing step-by-step guides for specific tasks, such as creating applications or using libraries.
Tutorials found on platforms like YouTube or dedicated programming blogs also serve a crucial role. These resources present visual demonstrations that can simplify complex concepts. Moreover, videos offer the chance to pause and replay segments, allowing learners to absorb information at their own pace.
"The journey to learning C# is a combination of resources. Books, courses, communities, and documentation together create a tapestry of understanding, fostering competence and confidence in new programmers."
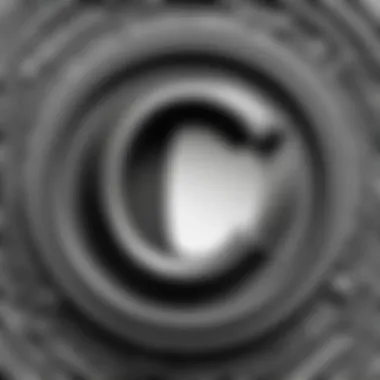
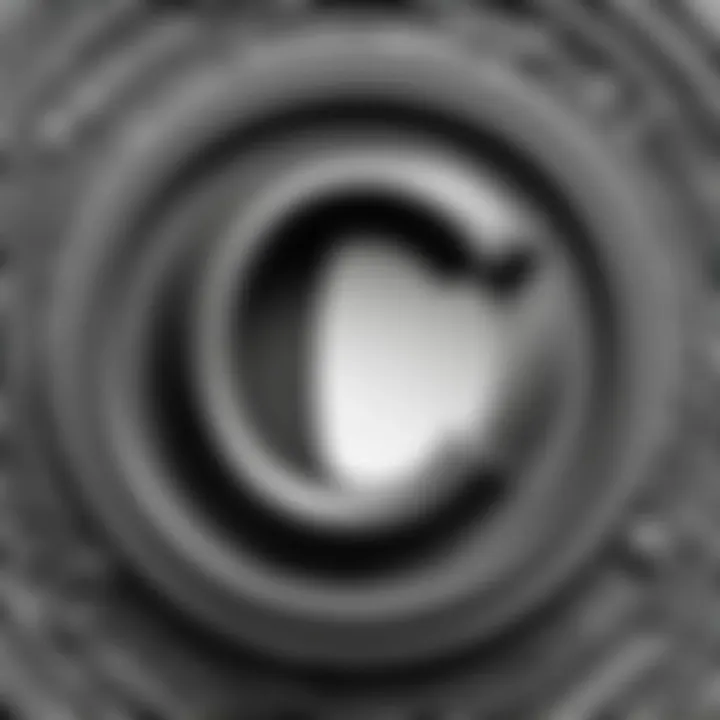
Leveraging all of these resources can greatly enhance a learner’s experience and understanding of C#. Each element complements the others, ensuring that learners do not just memorize code but develop a deep and lasting grasp of programming principles.
Career Opportunities with
C# has emerged as a key language within the software development landscape, largely due to its versatility and adaptability. In this section, we will explore various career opportunities associated with C skills. Understanding these roles offers insight into how learning C can benefit newcomers and experienced programmers alike. The following subsections will highlight specific positions and industries where C is crucial, emphasizing its relevance in today’s job market.
Positions Utilizing
Skills
C# proficiency opens the door to numerous roles in technology and development. Common positions include:
- C# Developer: Focuses on developing applications and systems using C#. They may work on web applications, desktop applications, and mobile software.
- Software Engineer: A broader title that includes designing and implementing software solutions with C#. This role often requires collaboration with other team members.
- Game Developer: Uses C# within game engines like Unity. This position is attractive as the gaming industry continues to grow.
- Web Developer: Specializes in building websites and web applications using ASP.NET, a framework for C#.
- Database Administrator: Involves managing databases that might require C# for data operations.
Each of these roles demands a solid grasp of C# principles and practice. Proficiency in this language can significantly increase one's employability.
Industries Relying on
Development
C# is widely adopted across various sectors. Key industries that rely on C development include:
- Technology: Tech companies utilize C# to create innovative software, mobile applications, and web services.
- Gaming: The gaming industry frequently employs C# within popular game engines, showcasing the language’s effectiveness for interactive applications.
- Finance: Financial institutions often develop trading systems and software tools using C#. This ensures high performance and reliability, essential for financial transactions.
- HealthCare: Many healthcare applications are built with C#, focusing on data management, patient records, and telehealth solutions.
- Education: E-learning platforms may utilize C# for their back-end systems, enhancing user experience and content delivery.
Common Challenges in Learning
Learning a programming language such as C# can be an exciting yet daunting endeavor for beginners. Even though the language has many features that simplify the learning process, certain challenges can hinder progress. Recognizing these hurdles is important, as it enables learners to prepare themselves accordingly and devise strategies to overcome them. This section will highlight the primary challenges encountered by novice programmers and provide insights into how to tackle them effectively.
Understanding Object-Oriented Concepts
Object-oriented programming (OOP) is a core pillar of C#. While OOP promotes a more organized and manageable way to code, beginners may find its concepts challenging. Fundamental concepts such as classes, objects, inheritance, and polymorphism need to be understood before effectively utilizing C#.
For instance, grasping how classes serve as blueprints for creating objects can be a significant step for new learners. If one does not understand this, the entire structure of OOP will be unclear. Beginners might also struggle with the distinction between a class and an instance of that class.
To ease this learning curve, consider the following strategies:
- Practice with Simple Examples: Start with basic class and object examples to solidify understanding. Creating simple games or applications can also help reinforce these concepts.
- Utilize Visual Aids: Diagrams and flowcharts can illustrate how classes interact. Seeing a visual representation often clarifies abstract concepts.
- Engage in Community Learning: Engaging with online communities such as Reddit can provide additional support, as fellow learners can offer valuable insight and discussions about OOP concepts.
Managing Complexity in Large Applications
As projects grow in scale, managing complexity becomes a noticeable challenge when using C#. Large applications often consist of numerous components and require intricate interconnections between them. New programmers might find themselves overwhelmed when transitioning from simple projects to more sophisticated ones.
The key to managing this complexity lies in modular design. This approach emphasizes breaking down an application into smaller, manageable modules or components. Each module should handle a specific task, making it easier to understand and implement.
Here are some effective practices to manage complexity in C#:
- Adopt a Consistent Naming Convention: By consistently naming files, classes, and methods, programmers can enhance code readability.
- Write Documentation: Documenting code as development progresses can aid in understanding the purpose and function of different parts of the application later.
- Version Control Systems: Utilizing tools such as Git can help track changes and manage various versions of the application effectively.
Epilogue
In this article, we have explored the fundamentals of C#, emphasizing its role as an accessible programming language suitable for beginners. The importance of this topic cannot be overstated. Understanding the advantages, challenges, and learning resources for C# helps to pave the way for individuals entering the programming world.
Reflecting on the Learning Journey
Learning C# can be a significant milestone in a programmer’s career. As one embarks on this journey, it is essential to acknowledge the challenges faced. Concepts such as object-oriented programming, syntax, and data structures may seem overwhelming at first. Yet, with perseverance and consistent practice, these concepts become more familiar.
As learners progress, they benefit not only from the structure of C# but also from the community surrounding it. Online forums and support networks provide valuable resources and encouragement. Each step taken is a part of a larger journey that ultimately opens doors to numerous career opportunities.
The Future of
in Programming
The future of C# remains bright within the programming landscape. Its continual evolution keeps it relevant in a technology-driven world. As more organizations adopt cloud computing and artificial intelligence, C is poised to expand. The integration of C with platforms such as Microsoft Azure further enhances its utility in developing scalable applications.
"C# not only supports creating applications but also adapts to modern development techniques."
As technology advances, so does the C# ecosystem. Emerging frameworks like .NET Core encourage greater flexibility and cross-platform development. This adaptability ensures that C will remain an essential language in software development.
In summary, the areas discussed in this article highlight the significance of C# for beginners. By reflecting on the learning process and recognizing the future trends associated with C#, individuals can prepare themselves for a fruitful career in programming.