Understanding the File Class in Java: A Comprehensive Guide
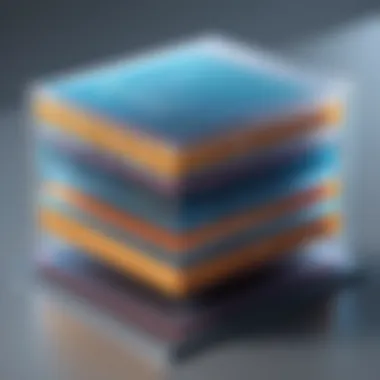
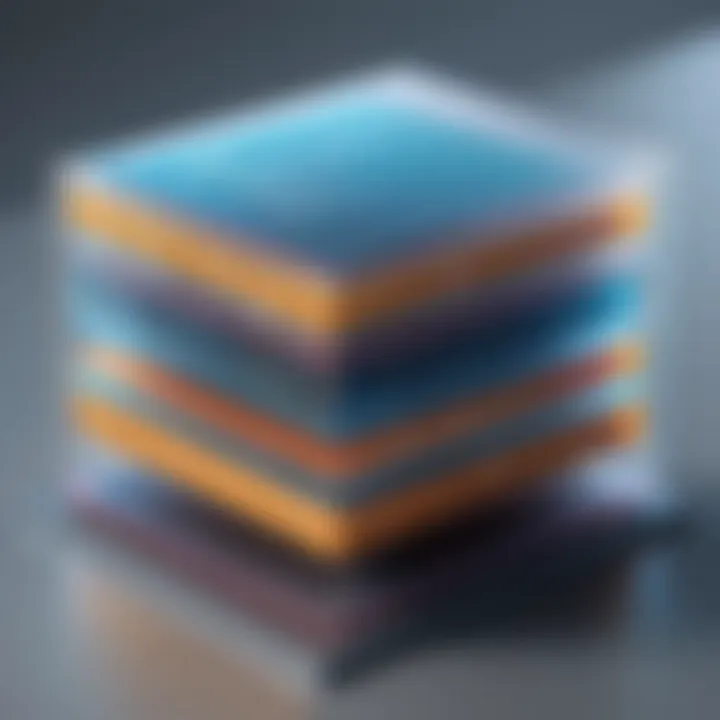
Overview of Topic
Prologue to the main concept covered
The File class in Java is an essential part of the Java Standard Library, providing a mechanism to handle file directories and file operations. It allows developers to create, delete, and alter file properties easily. Understanding this class is crucial for anyone who wants to work with files in Java. As files are integral to data storage and processing, mastering the File class will enhance one's programming capabilities.
Scope and significance in the tech industry
In today's tech landscape, file management plays a significant role in software development. Applications often require reading from and writing to files, making the File class a pivotal tool. Whether it's developing desktop applications or web applications, having a solid grasp of file handling can improve efficiency and effectiveness. Moreover, the increasing reliance on data processing and storage has made file management skills a sought-after competence in the industry.
Brief history and evolution
Initially, Java provided basic file handling features, but over time, the File class evolved to include a wider array of functionalities. Java's standard library has been updated across different versions to enhance file management support. From primitive operations to more complex manipulations, the evolution of the File class mirrors the growing complexity of applications in the field of software development.
Fundamentals Explained
Core principles and theories related to the topic
At its core, the File class serves as an abstract representation of file and directory pathnames. It encapsulates various methods that facilitate file manipulations. It is grounded in fundamental principles of Object-Oriented Programming, enabling users to interact with file systems while abstracting underlying complexities.
Key terminology and definitions
- File: An object representing a path to a file or directory.
- Path: The location of a file within the directory structure.
- Directory: A container for files, also known as a folder.
Basic concepts and foundational knowledge
To utilize the File class, one must understand its constructors and various methods. The constructors are mainly used to instantiate File objects, while methods like createNewFile(), delete(), renameTo(), and exists() perform critical file operations. These components form the backbone of file manipulation within Java.
Practical Applications and Examples
Real-world case studies and applications
Developers frequently utilize the File class in applications requiring data storage, such as text editing software or database management systems. One practical example is reading configuration files, which determine application behavior.
Demonstrations and hands-on projects
Here is a simple demonstration of creating a file and writing to it:
Code snippets and implementation guidelines
This code snippet demonstrates the creation of a new file and the writing of text into it. It showcases basic File class functionality and helps beginners grasp file operations in Java.
Advanced Topics and Latest Trends
Cutting-edge developments in the field
With advancements in technology, file management trends are shifting towards cloud storage and enhanced security. Technologies such as Java NIO (New I/O) are being integrated to improve performance and scalability for file I/O operations.
Advanced techniques and methodologies
Using streams provided by Java NIO can be more efficient for extensive file operations compared to traditional methods. Techniques involving buffering and asynchronous file access are becoming popular.
Future prospects and upcoming trends
The trend towards integrating artificial intelligence and machine learning into file management systems enhances the ability to process and analyze data stored in files effectively. Developers should stay informed about advancements to remain competitive in the industry.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
- Effective Java by Joshua Bloch - A great resource for understanding Java best practices.
- Java Programming and Problem Solving - Online courses on platforms like Coursera or Udemy can be beneficial for hands-on learning.
Tools and software for practical usage
Utilizing IDEs like IntelliJ IDEA or Eclipse can greatly assist in managing Java projects, including file manipulations. These tools often provide integrated aspects that facilitate and streamline development.
Prologue to File Class
In the realm of Java programming, the File class is pivotal. It provides a way to interact easily with the file system, allowing developers to create, delete, and manage files and directories. Understanding this class is essential for anyone engaged in Java development, particularly in areas such as data handling and system programming. The File class is not merely about opening and reading files; it encompasses a broad range of functionalities. Its role includes file path navigation, attribute retrieval, and extensive file operations, which are crucial for robust application development.
Definition of File Class
The File class in Java is part of the java.io package. Essentially, it represents a file or directory path in the file system. This representation allows developers to perform various file-related tasks. For instance, one can create instances of the File class with a specific path location, and subsequently, interrogate these instances for various file properties. Importantly, it does not read or write data on its own; rather, it serves as a conduit through which file operations are executed. The syntax is straightforward. For example:
In this line, becomes a handle to a specific file for further operations.
Importance in Java Programming
The significance of the File class in Java cannot be overstated. Here are a few key points:
- System Interaction: The File class forms a bond between Java applications and the underlying file system. This capacity allows programmers to handle resources dynamically as per application needs.
- Modularity: It encourages modular code. Separating file-handling logic into its independent component promotes cleaner and maintainable code.
- Error Handling: It provides mechanisms to check file existence and permissions, reducing runtime errors in file operations. Developers can use methods like and to ensure that operations are feasible before execution.
- Cross-Platform Compatibility: Java aims for platform independence. By using the File class, developers can write code that works seamlessly across different platforms and file systems.
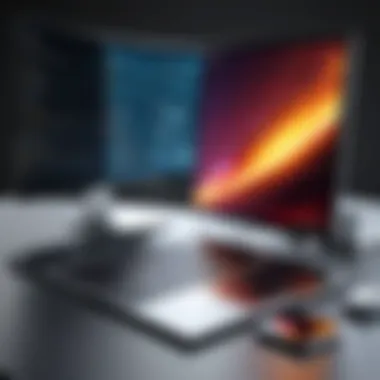
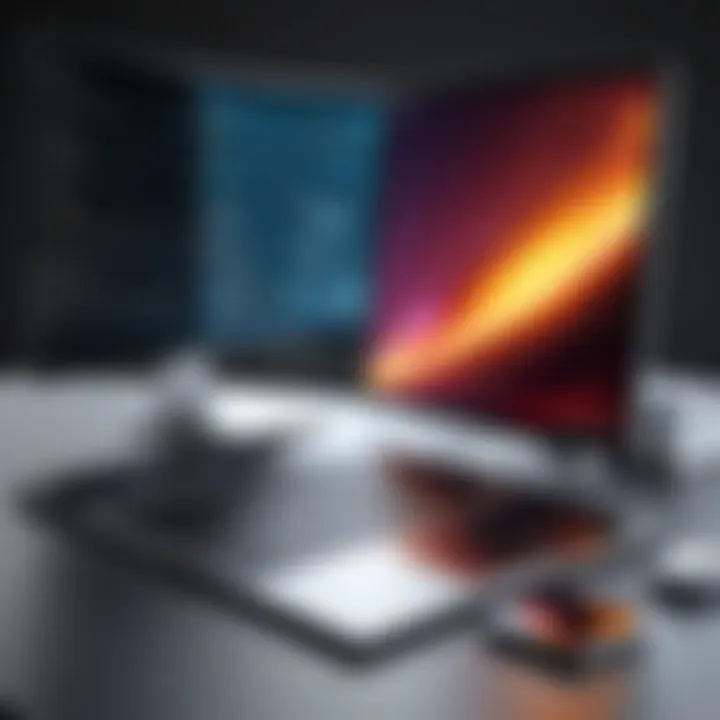
The Java File class acts as a fundamental interface between Java applications and the file system, thus essential for effective file manipulation.
In summary, understanding the File class is critical for Java programming instruction. It enables developers to manipulate files effectively while ensuring code resilience and portability.
Core Functionality
The core functionality of the File class in Java is vital to its purpose of file handling and manipulation. The File class allows developers to interact with the file system in a structured way, facilitating operations that include creating, querying, and modifying files and directories. Through its various methods, the File class enables a range of capabilities, from simple file creation to analyzing file attributes, which are essential for effective file management in Java applications. Understanding this functionality enhances a programmer's ability to write efficient and intentional code when dealing with file operations.
Creating File Instances
Using Constructors
The File class provides multiple constructors that help in creating file instances. A fundamental aspect of using constructors is its simplicity. With various constructors available, developers can create instances of files or directories by passing either a file path as a string or a URI. This flexibility contributes to easier and modular coding practices. Moreover, it allows programmers to initialize Files based on different parameters. For example, using the constructor is straightforward for most scenarios. One of the key characteristics of using constructors is that it abstracts the complexities involved in file initialization, making it a common choice for Java programmers.
However, it's worth noting that a disadvantage is that merely creating a File instance does not create the actual file until the createNewFile() method is invoked. This could lead to confusion for those new to the File class.
File Paths and Directories
File paths and directories play an essential role in file handling. In Java, paths can be absolute or relative and define the location of files within the file system. This aspect is important because choosing the correct path ensures that file operations are executed without errors. The ability to work with both absolute and relative paths provides flexibility when referencing files.
In addition, the Java File class also supports various directory operations. A significant advantage is that it allows developers to check if a file exists and whether a directory can be created, among other permissions. The interaction with directories is particularly beneficial when dealing with complex file systems, where organization becomes crucial.
However, difficulties can arise when paths are incorrect or misunderstood, leading to runtime errors that may not be immediately obvious. Careful attention must be given to how file paths are structured.
File Attributes
File Size
File size is a critical attribute when dealing with files. It gives insights into how much space a file occupies on the disk, which can be imperative for resource management and optimization in applications. The File class offers a method called that returns the size of a file in bytes. This characteristic enhances the capability of applications to monitor and manage file storage effectively.
A noteworthy feature is that a smaller file size typically means quicker read and write operations. However, a disadvantage is that for directories, the method is not applicable, which may cause confusion if one expects to retrieve sizes for all file types.
File Permissions
File permissions are essential for ensuring security and appropriate access control. The File class provides methods that allow checking and changing permissions for files. Important characteristics include read, write, and execute permissions, which ensure that only authorized users can perform particular operations on files.
Understanding and managing these permissions is a beneficial choice for applications that require file access control, such as those dealing with sensitive data. This aspect serves to protect both the integrity of the files and the privacy of users. A downside, however, is that frequently changing permissions may introduce complexity in code maintenance and user experience if not managed properly.
Last Modified Date
The last modified date is a simple yet informative attribute that reveals when a file was last altered. This information can be crucial in applications where file versions need tracking. The File class includes the method, which can help in determining the freshness of a file's content.
Highlighting the last modified date can be a beneficial practice in applications that require synchronization or backup processes. Keeping track of changes through timestamps helps developers and users manage file states effectively. However, relying too heavily on this attribute can lead to challenges in applications that involve frequent file updates, as it can be easily overlooked or misinterpreted.
File Operations
File operations are at the core of any application that interacts with data stored in files. Understanding how to read, write, and delete files is essential for both novice and experienced programmers. These operations provide the means for managing content, ensuring that applications can retrieve and manipulate data as needed. Knowing how to effectively handle files can enhance the performance of an application and prevent errors that may arise from improper file management.
Reading Files
Using FileReader
When working with text files, FileReader is a popular choice. It allows Java programs to read character files efficiently. The main feature of FileReader is its straightforward interface for reading files one character at a time or as an array of characters. This makes it particularly suitable for applications where text size may not be excessively large.
One key characteristic of FileReader is simplicity. Developers appreciate it for its ease of use, as it requires minimal coding to set up. However, FileReader has limitations in handling large files due to potential performance issues when reading character by character. In contrast, a more efficient approach would be using buffered readers in conjunction with FileReader to enhance performance.
Using BufferedReader
For more efficiency in reading files, BufferedReader comes into play. It uses an internal buffer to read data in larger chunks, reducing the number of I/O operations. This approach significantly improves the speed of file reading tasks.
The main advantage of BufferedReader is its ability to handle large files without slowing down the application. Additionally, it provides methods like that simplify reading entire lines from a file. However, it requires a FileReader or other reader as its input, which means it cannot be used independently.
Writing to Files
Using FileWriter
FileWriter is the go-to option for writing character files. It provides an easy way to create files or append to existing files. A critical feature of FileWriter is its direct method for writing characters, arrays, or strings to a file. This makes it an efficient choice for applications that need to generate or modify text files.
While FileWriter is beneficial for its straightforward nature, it lacks advanced functionalities that can be found in other writing classes. It writes data directly to the file without buffering, which can lead to performance issues for large outputs. Therefore, combining it with other classes, like BufferedWriter, can be advantageous for applications requiring substantial file writing tasks.
Using PrintWriter
PrintWriter offers more flexibility compared to FileWriter. It supports formatted output, which makes it easier to control how data is written to files. PrintWriter can handle various data types and can even print formatted strings and objects.
A unique feature of PrintWriter is its ability to automatically flush the output buffer when certain conditions are met. This minimizes the risk of losing data during unexpected terminations or errors. On the downside, its complexity may not be necessary for simple write operations, making it overkill for basic tasks.
Deleting Files
Using delete() Method
The delete() method is straightforward and powerful for removing files from the file system. Its approach is direct, allowing the programmer to specify a file path and delete the corresponding file if it exists. Understanding its usage is vital, especially for maintenance tasks in applications that deal with temporary or frequently changing files.
The major drawback with the delete() method is that it does not throw an exception if the delete operation fails, making it challenging to handle errors. It's essential to perform checks to ascertain the success of delete operations to avoid surprises.
Handling Exceptions
Exception handling is a critical aspect of any file operation. When dealing with files, numerous things can go wrong—the path might not exist, permissions may be inadequate, or other unexpected issues can arise. By employing proper exception handling mechanisms like try-catch blocks, you can ensure your application maintains stability despite errors during file operations.
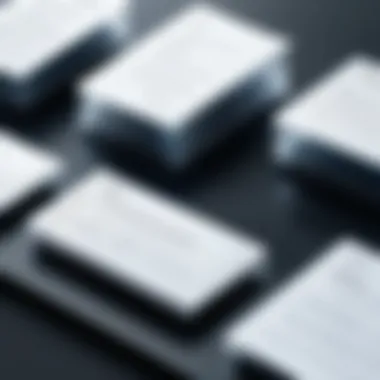
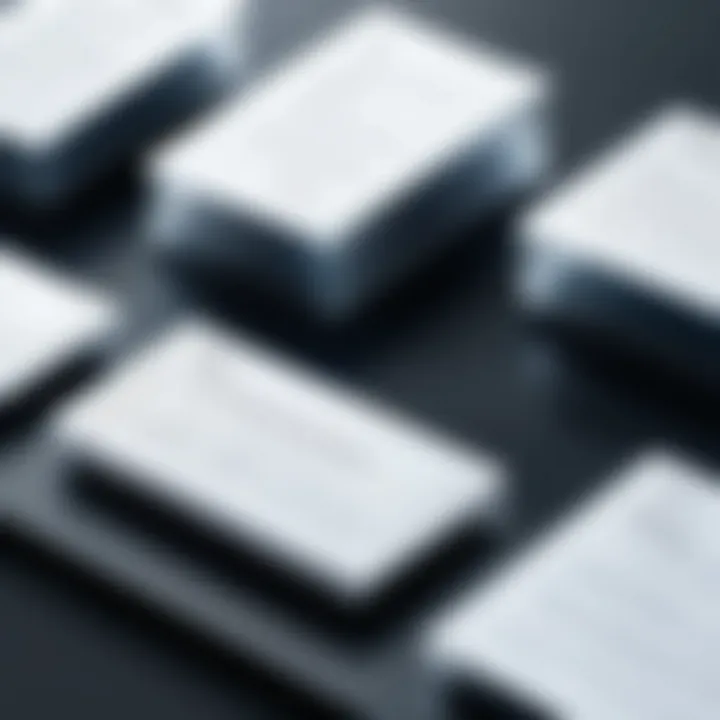
Handling exceptions allows developers to provide feedback to the user or logs for troubleshooting. This is particularly beneficial in large applications where file operations are frequent and critical to overall functionality.
File Class Methods
The methods available in the File class play a crucial role in the manipulation of files and directories in Java. This section outlines both commonly used methods and more advanced features, providing insights on how they can efficiently aid file operations in various programming scenarios. Understanding these methods can enhance programming practices by allowing developers to interact seamlessly with the file system.
Commonly Used Methods
exists()
The method is primarily used to check if a particular file or directory exists in the specified path. This feature is significant because it prevents errors that occur from trying to manipulate non-existent files. Hitting unexpected issues during runtime can derail applications, hence verifying existence is an essential step before proceeding with operations.
The key characteristic of is its simple, straightforward implementation. It returns if the file or directory exists, and otherwise. This clarity makes it a beneficial choice for many Java developers.
However, it is important to note that only confirms presence but does not assure accessibility. For instance, a file may exist but be hidden or protected from the current context. This nuanced feature can be both an advantage and a disadvantage, as it simplifies checks yet requires further validation if detailed properties are critical.
mkdir()
The method is utilized to create a new directory in the file system. Its significance stems from the necessity of organizing and structuring files effectively, which is vital in larger projects.
One of the most notable aspects of is its straightforward usage; it returns if the directory is successfully created, and returns if the directory already exists or if there are permission issues. This feedback is essential for maintaining a smooth workflow.
However, a downside is that cannot create multiple nested directories in one go. Developers encountering complex file hierarchies might find this limiting. A solution is to use , which creates all nonexistent parent directories as needed, adding a layer of flexibility to directory creation.
renameTo()
The method provides a way to rename a file or directory. Its utility is clear—renaming files can help manage file versions or correct naming conventions when needed. The success of the operation is indicated by a boolean return value.
A key characteristic of lies in its simplicity. It is straightforward, and renaming can be executed in just a few lines of code. Nevertheless, it presents some challenges: if the target name conflicts with an existing file, or if there are permission issues, the operation will fail. Also, it is important to consider that this method can be limited in scope across different file systems due to underlying system restrictions.
Advanced Methods
listFiles()
The method serves to list all files and directories within a specified directory. This capability is crucial when needing to process multiple files, such as when performing batch operations.
A significant advantage of is its return of an array of File objects, which allows for direct manipulation of each file or directory. This granularity facilitates further processing, making it a popular choice for tasks involving directory listings. However, the performance can be hindered if the directory contains a large number of files, as returning a massive list can impact memory usage and speed.
length()
The method provides the size of a file in bytes. Knowing file size can be fundamental in various contexts, such as validating uploads or checking for space constraints before file handling.
Its key characteristic is the clarity it offers; returning a long value makes it easy to understand how much data is at hand. However, it should be noted that works only on regular files. If the method is invoked on a directory, it will return 0. This limitation makes it less versatile in certain scenarios, as users must keep track of context when determining file attributes.
Error Handling and File Exceptions
Error handling is a critical aspect of any programming language, and Java is no exception. When working with files using the File class, exceptions can occur for various reasons. Understanding how to handle these exceptions is indispensable for maintaining code stability and ensuring a smooth user experience.
Java primarily utilizes exceptions to signal that something has gone wrong during file operations. If not properly handled, these exceptions can lead to application crashes or data loss. By incorporating robust error handling techniques, developers can mitigate risks associated with file manipulation and enhance the reliability of their applications.
Understanding IOException
IOException is the parent class of exceptions related to input/output operations in Java. Various subclasses extend IOException, each addressing specific scenarios. The two most common subclasses are FileNotFoundException and EOFException.
Key points to understand about IOException:
- FileNotFoundException: This occurs when an attempt to access a file fails because it doesn’t exist at the specified path.
- EOFException: This indicates that the end of the file has been reached unexpectedly during input operations.
Using IOException effectively means developers need to anticipate potential failures during file reads, writes, or processing. Properly catching and addressing these exceptions ensures that applications can respond gracefully, instead of abruptly failing.
Try-Catch Blocks
Java employs try-catch blocks to manage exceptions like IOException. This structure allows developers to specify code that may throw an exception inside the block, while the block handles the exception when it occurs.
Example of using Try-Catch in File Handling:
In the example above, if the file does not exist, the catch block for FileNotFoundException will execute, and a meaningful message will be displayed. This way, the application does not terminate unexpectedly and provides feedback on the issue. Utilizing try-catch blocks not only protects code but also improves maintainability and user experience by ensuring that file-related errors are comprehensively addressed.
Best Practices for File Handling
When working with files in Java, adhering to best practices is essential. These practices enhance code reliability, improve performance, and reduce the chances of errors. Effective file handling is crucial not only in ensuring data integrity but also in maintaining optimal application performance.
Best practices in file handling involve managing resources appropriately, following naming conventions, and ensuring proper exception handling. These elements contribute to a robust coding environment. Adhering to such practices already instills a level of discipline that can prevent headaches down the road.
Resource Management
A key aspect of file handling is resource management, particularly how input and output streams are handled. Failing to close file resources can lead to memory leaks and file corruption. This brings us to the try-with-resources statement, which simplifies resource management in Java. It ensures that each resource is closed at the end of the statement.
Using try-with-resources statement
The try-with-resources statement was introduced in Java 7 and allows for automatic resource management. This means that any resources declared in the parentheses of a try statement are automatically closed when the try block finishes, either normally or abruptly.
Key characteristics of using try-with-resources include:
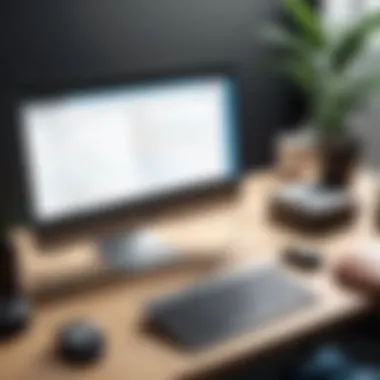
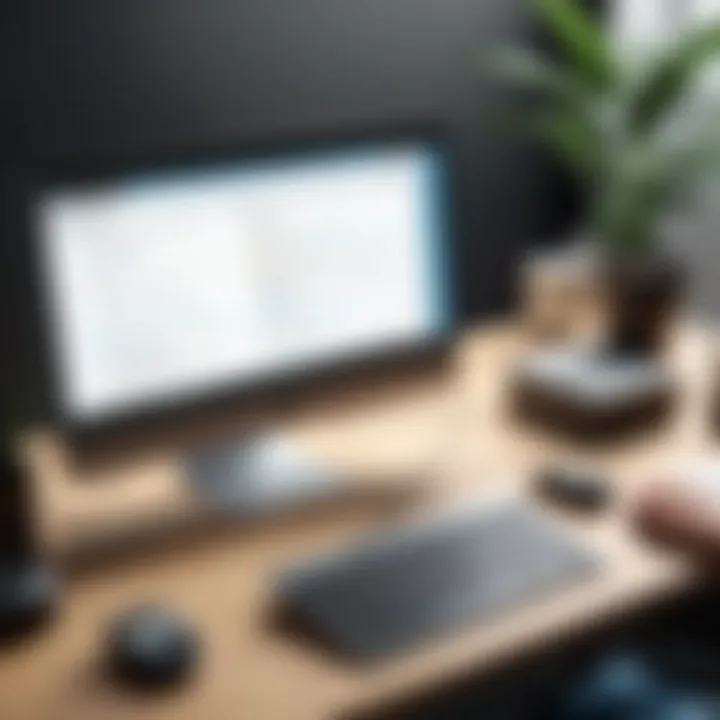
- Automatic Resource Management: Resources such as and are automatically closed. This reduces boilerplate code where developers would usually need to use a finally block to close resources manually.
- Simplified Code Structure: Using this method leads to cleaner and more readable code. It separates resource management logic from the main logic, making it easier to follow.
This approach is beneficial because it minimizes the risk of leaks, providing a more stable environment for file operations. Unique features include the ability to declare multiple resources in a single try statement, which enhances flexibility and usability. However, one must be cautious with exceptions that could be thrown during resource initialization, as they can affect execution flow.
File Naming Conventions
File naming conventions are another crucial aspect of file handling. Adopting appropriate conventions ensures consistency and can prevent confusion in file identification.
- Consistency: Use a standard format that applies uniformly across the project.
- Descriptive Names: Names should reflect the content or purpose of the file. Avoid generic terms like "data" or "output".
- Use of Underscores or CamelCase: Stick to one format, either using underscores or CamelCase, for better readability.
By establishing and following naming conventions, developers create an easier environment for collaboration and maintenance. Overall, these practices guide developers in writing cleaner, more manageable code, assisting in long-term project success.
Real-world Applications
The practical implications of leveraging the File class in Java cannot be understated. By enabling efficient file manipulation, the File class serves as a cornerstone for various applications in Java programming. Understanding its real-world applications provides both context and urgency for mastering this class. Developers encounter numerous scenarios where file creation, deletion, and management are crucial.
Data File Management
Data file management stands as one of the most significant applications of the File class. In many enterprise systems, data is stored in files, making file handling crucial for effective operations. Developers can use the File class to create directories, manage file locations, and organize datasets.
For instance, the ability to check if a file exists or if a directory is accessible can prevent unnecessary errors in data processing workflows. Here are some benefits and considerations in data file management:
- Efficiency: Automated file operations can greatly speed up data processing tasks.
- Organization: Properly managing files helps maintain clean and understandable code, crucial for long-term projects.
- Integration: Many applications interact with external data sources. The File class can facilitate data imports from CSV, XML, or JSON files.
However, developers should also be mindful of the limitations inherent in file handling, such as potential file access conflicts and permissions issues.
File Uploads and Downloads
File uploads and downloads are common requirements in modern web applications. The File class plays a vital role in facilitating these functions. It enables developers to generate file input streams and establish connections to client-side uploads or server-side downloads.
In practical terms, the File class aids in the following ways:
- User Interactions: Applications can prompt users to choose files for upload without deep technical configurations.
- Server Management: On the backend, the File class allows for the systematic storage of uploaded files, managing storage space effectively.
- Transfer Efficiency: Leveraging Java's capabilities can yield faster upload and download times as users interact with large data sets.
To implement file uploads satisfactorily, programmers need to ensure appropriate security measures are in place to protect file handling protocols, thus safeguarding data integrity and user privacy.
The File class is indispensable for managing files in Java, making it a necessary skill for any serious developer.
In summary, understanding how to apply the File class in real-world scenarios empowers developers to build more robust and efficient applications, significantly enhancing their programming repertoire.
Comparison with NIO Package
When discussing file handling in Java, it is essential to examine the comparison between the traditional File class and the New Input/Output (NIO) package. This comparison elucidates not only their fundamental differences but also the contexts in which each is most effective. The NIO package was introduced in Java 1.4 to overcome some of the limitations of the File class, making it a crucial subject in any thorough exploration of file handling in Java programming.
Prelude to NIO
The NIO package enhances Java's file handling capabilities. Unlike the original File class, NIO introduces features such as non-blocking I/O operations and the use of channels and buffers. This is particularly beneficial for applications requiring high performance, such as server-side applications or large-scale data processing. The package supports both synchronous and asynchronous operations, allowing developers more flexibility in their file handling strategies.
NIO provides the Path class, which represents file and directory pathnames as objects, and the Files class, which contains static methods that operate on files and directories. These additions allow for a more modern approach to file management that adheres to the principles of object-oriented design.
Advantages of NIO Over File Class
The advantages of NIO over the traditional File class are noteworthy:
- Non-blocking I/O: NIO supports non-blocking operations, allowing applications to continue executing while waiting for file operations to complete. This is increasingly necessary in multi-threaded applications.
- Better Performance: NIO is optimized for performance, especially with larger files. Its use of buffers allows for more efficient data manipulation compared to the byte-by-byte processing often required by File operations.
- Enhanced Capabilities: NIO offers functionality for file system monitoring through the WatchService API, which enables applications to respond to file changes without continuous polling.
- Path and Files APIs: With NIO, developers can easily work with both files and directories using the Path and Files classes. This simplifies operations such as copying, moving, and deleting files, among others.
- Improved Exception Handling: NIO introduces new exception types that provide more granular control over error management when dealing with file operations.
In summary, the NIO package provides significant improvements over the traditional File class, making it a compelling option for developers who need to optimize file handling efficiency in modern applications. Choosing between the two often depends on the specific requirements of the application, including performance needs and complexity of file management tasks.
Future of File Handling in Java
The future of file handling in Java is a topic of growing significance as technology evolves. The traditional methods of file manipulation, centered around the File class, face challenges from newer paradigms. Understanding these changes helps programmers adapt and innovate in their code. As we dive deeper, it is clear that emerging technologies and enhancements could reshape how Java developers interact with files.
Emerging Technologies
The landscape of file handling is influenced by various emerging technologies. Cloud computing and big data frameworks have prompted a shift in how files are accessed and processed. For instance, the integration of cloud services like AWS and Google Cloud makes it possible to manage large datasets without relying solely on local storage.
- Cloud Storage Solutions: Tools like Amazon S3 or Google Drive APIs facilitate remote file access and manipulation, making file management more flexible.
- Machine Learning: Algorithms now process files rapidly, analyzing contents for patterns without substantial manual interaction. This trend signals a move toward more intelligent data handling.
- Distributed Systems: Technologies like Apache Hadoop allow files to be split and processed across multiple nodes, streamlining large file working processes.
As these technologies gain traction, they usher in new functionalities and efficiencies, transforming day-to-day file handling in Java applications.
Possible Enhancements
Future advancements in Java's file handling capabilities may arise from the demand for efficiency and user experience. Here are some key enhancements to consider:
- Improved APIs: While the current File class serves its purpose, additional APIs could simplify access and modification processes. A more abstracted approach may promote usability, especially for novices in programming.
- Native Support for Different Formats: Enhancements could include native support for various file types, such as JSON, XML, and others, making file parsing and writing more straightforward.
- Integration with Java NIO: The New Input/Output package already offers benefits such as non-blocking I/O operations. A deeper integration with the existing File class could unify the file manipulation experience.
- Security Features: As files often contain sensitive data, stronger security mechanisms could be essential. Enhancements in encryption and permission management will be crucial in future iterations of file handling in Java.
Embracing future enhancements will ensure Java remains relevant in a fast-paced technological environment, empowering developers with tools that meet modern demands.
As we look ahead, it is clear that the evolution of file handling in Java hinges on the intersection of technology, user needs, and language capabilities. Regular adaptations and innovations ensure that programmers can effectively manage files while keeping pace with the ever-changing technical landscape.
The End
In the realm of Java programming, the significance of the File class cannot be overstated. This article has thoroughly explored its structure and functionalities, laying out a path to understand how to handle file operations adeptly. The File class is essentially the gateway to file manipulation, providing essential methods and constructors necessary for effective file management.
Understanding the various methods, such as , , and , empowers programmers to conduct a wide range of operations with ease. Additionally, being familiar with file attributes—like file size and permissions—enhances one's ability to manage files securely and efficiently.
Moreover, we discussed common pitfalls within file handling. Recognizing the potential errors and understanding how to utilize try-catch blocks ensures that applications can handle file-related exceptions gracefully. Adhering to best practices promotes not just efficiency, but also code maintainability. The application of resource management techniques like the try-with-resources statement ensures that resources are closed promptly, saving valuable system resources.
The discussion on real-world applications illustrated how vital the File class becomes in scenarios like data file management and file uploads. These examples help to bridge the gap between theoretical knowledge and practical application.
As technology progresses, the comparison with the NIO package highlighted the ongoing evolution in file-handling methods and potential enhancements to the File class itself. Keeping abreast of such developments is essential for programmers who wish to remain proficient in Java file management.
In summary, the File class is a cornerstone of Java programming. Its methods, functionality, and application are crucial elements for anyone embarking on the journey of file manipulation. Through the insights shared in this article, readers should now have a deeper understanding of effectively using the File class, its relevance, and its impact on their projects. Employing these insights will undoubtedly lead to better coding practices and more robust Java applications.