Understanding JavaScript and React in Web Development
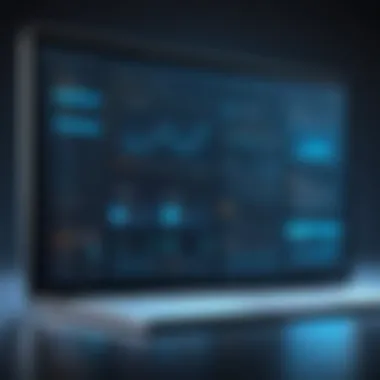
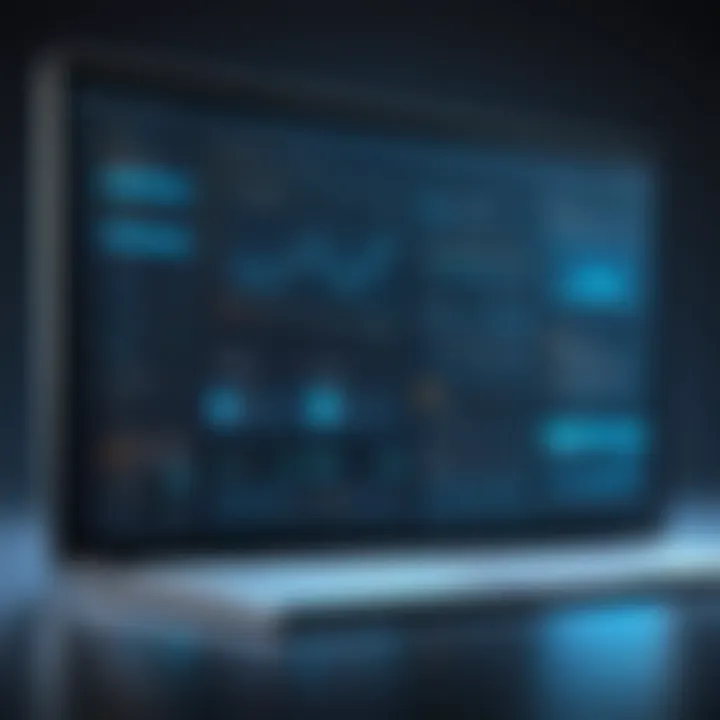
Overview of Topic
In the rapidly changing landscape of web development, understanding the relationships between JavaScript and React is essential. At its core, this conversation involves not just the tools themselves, but the architecture and design choices that shape modern applications. JavaScript, as a versatile scripting language, has become the lifeblood of dynamic and interactive web pages. React, on the other hand, serves as a powerful library enabling developers to build user interfaces that are both reactive and efficient.
Foreword to the main concept covered
The union of JavaScript and React enables developers to create engaging user experiences. This article dives deep into how these technologies intertwine. Weāll look into their basic workings, practical applications, advanced methodologies, and future directions, catering to everyone from novices wrestling with syntax to veterans refining their frameworks.
Scope and significance in the tech industry
Given that the digital landscape is continuously evolving, the importance of understanding these technologies cannot be overstated. The tech industry increasingly demands professionals who are adept in modern frameworks built on JavaScript, with React being one of the frontrunners. Companies are looking for robust, maintainable, and scalable solutions, which these tools provide. Not grasping this dynamic duo could put aspiring developers behind the curve.
Brief history and evolution
JavaScript made its debut in 1995, initially created to add simple interactivity to web pages. Over the years, it has morphed into a full-fledged programming language that powers vast sections of the web. React, developed by Facebook in 2013, marked a shift in how user interfaces could be rendered. The component-based architecture that React introduced radically changed the game, emphasizing reusability and maintainability.
Fundamentals Explained
Understanding the bedrock of JavaScript and React is paramount for anyone looking to work in modern web development.
Core principles and theories related to the topic
Key principles of both JavaScript and React include:
- Event-driven programming: This is a cornerstone for creating interactive applications.
- Component-based architecture: React takes this a step further by encouraging developers to build encapsulated components that manage their own state.
- Declarative programming: With React, rather than manipulating the DOM directly, developers describe what the UI should look like.
Key terminology and definitions
- JSX: A syntax extension for JavaScript that looks similar to HTML. JSX simplifies writing React components.
- State: A local data storage that is specific to a component.
- Props: Short for properties, these are inputs to React components, allowing data to be passed down the component tree.
Basic concepts and foundational knowledge
At its essence, JavaScript is employed to enhance HTML and CSS functionality, enabling the creation of asynchronous requests through AJAX, managing user inputs, and generally controlling web page behaviors. React builds on these JavaScript fundamentals, introducing concepts that let developers construct complex interfaces efficiently.
Practical Applications and Examples
To truly grasp how JavaScript and React work together, tangible examples are crucial.
Real-world case studies and applications
- Facebook: Uses React for its dynamic front-end, allowing for seamless updates and fast performance.
- Netflix: Leverages React to provide an engaging and responsive user interface, enhancing the viewing experience.
Demonstrations and hands-on projects
A simple hands-on project could involve creating a todo app using React. This can give developers a quick grasp of component structure and state management.
Code snippets and implementation guidelines
This snippet features a simple, functional component that allows users to add items to a todo list, illustrating key concepts of state and event handling in React.
Advanced Topics and Latest Trends
As web technologies evolve, new trends emerge, and keeping pace is vital.
Cutting-edge developments in the field
Emerging technologies like server-side rendering and static site generation with frameworks like Next.js are shaping how applications are built using React.
Advanced techniques and methodologies
- Hooks: These were introduced in React 16.8. They allow developers to use state and other React features without writing a class.
- Context API: A means for passing data through the component tree without needing to pass props at every level.
Future prospects and upcoming trends
With the advent of WebAssembly and improved performance tools, we may see a future where React-based applications will run even more efficiently, making web development more robust for developers.
Tips and Resources for Further Learning
To deepen your knowledge, consider checking out these resources:
- Books: "Eloquent JavaScript, 3rd Edition" by Marijn Haverbeke, and "Learning React" by Alex Banks.
- Online Courses: platforms like Udemy and Coursera provide excellent courses on React and JavaScript.
- Tools and software for practical usage: Using tools like Visual Studio Code for coding, or Chrome DevTools for debugging can significantly enhance productivity.
For more resources and community engagement, visiting sites like reddit.com and checking out discussions on web development can provide fresh insights.
Prologue to JavaScript
JavaScript stands as one of the foundational pillars of web development, playing a crucial role in building modern, interactive applications. Through this lens, understanding JavaScript is not just beneficialāit is essential for anyone looking to navigate the world of programming and web design effectively. This section captures the significance of JavaScript, shedding light on its evolution, core principles, and why these aspects matter in today's digital landscape.
History and Evolution
The journey of JavaScript began in 1995 when Brendan Eich developed it under Netscape Communications. Originally named Mocha, it later became known as LiveScript before settling on the name JavaScript. This initial inception was pretty humbleā a simple scripting language crafted to enhance web pages by making them dynamic. The need for such interactivity was evident; static HTML pages werenāt cutting it anymore in the fast-paced internet environment.
Over the years, JavaScript has undergone a remarkable transformation, evolving through various versions and standards set by ECMA International. For instance, the release of ECMAScript 6 (ES6) in 2015 introduced features like arrow functions, classes, and promises, making the language more robust and developer-friendly. Such enhancements have paved the way for JavaScript to dominate the web development landscape, evolving from a tool for basic interactivity into a full-fledged programming language capable of server-side programming with Node.js.
Core Principles of JavaScript
At its core, JavaScript incorporates several fundamental principles that shape how developers create applications. Primarily, it is an event-driven language. This characteristic allows developers to build applications that respond dynamically to user interactions. To illustrate, when you click a button on a web page, JavaScript can execute specific functions in response to that click, enhancing user experience.
Moreover, JavaScript follows a prototype-based inheritance model, a feature that distinguishes it from classical inheritance seen in many other programming languages. This design allows for flexible object creation, making it easy to extend and modify existing objects without requiring deep knowledge of parent-child relationships, fostering creative coding solutions.
Lastly, JavaScript is renowned for being asynchronous. This allows developers to perform multiple operations simultaneouslyāsuch as loading data from a server while allowing users to interact with the webpage. Hence, users never need to wait for data to load before conducting their tasks, which is vital in creating smooth, responsive applications.
JavaScript's evolution reflects the changing needs of web development, highlighting its adaptability and significance in creating user-responsive designs.
In summation, the significance of JavaScript in the realm of web development cannot be overstated. Not only does it power countless applications and websites, but it also continues to evolve, integrating new features that respond to developersā needs. A strong grasp of both its history and core principles sets the groundwork for mastering more advanced frameworks, such as React, and greatly enriches a developerās skill set.
Understanding React
In the vast landscape of web development, React stands out as a prominent library that simplifies the process of building user interfaces. It's not just about coding but creating a seamless experience for users. Understanding React is fundamental for anyone who wants to elevate their skills in modern web development. It makes structuring your applications easier, enhances performance, and allows for a more interactive experience.
One of the notable strengths of React is its component-driven architecture. Components are the building blocks of a React application. This means each part of your interface can be broken down into separate pieces that can be developed, tested, and reused independently. This modularity is significant as it not only boosts productivity but also promotes maintainable code.
What is React?
React is an open-source JavaScript library maintained by Facebook designed specifically for building user interfaces, particularly for single-page applications. It allows developers to create large web applications that can change data without reloading the page. Unlike traditional frameworks, React operates on the concept of a "virtual DOM," which efficiently updates the user interface. This means if a componentās state changes, only the component in question will get re-rendered, thus saving time and resources.
The elegance of React lies in its simplicity and flexibility. To illustrate, consider the process of creating a user profile interface in a social media application. Instead of constructing the entire page at once, you can build a 'Profile' component, a 'Posts' component, and a 'Friends List' component, which can be assembled together like a puzzle.
"React is not just a tool; it represents a shift in how developers approach building UI. Its component-based structure empowers developers to think in reusable units, fostering creativity and efficiency."
React's Key Features
React offers a range of features that have contributed to its widespread adoption:
- Component-Based Architecture: Each piece of the UI is a standalone component that can manage its own state. This modular approach results in easier debugging and simpler testing.
- Unidirectional Data Flow: Data flows in one direction, making the applicationās behavior more predictable and easier to trace. This flow also maintains the integrity of application state, reducing bugs.
- JSX Syntax: React introduces JSX, an extension that allows you to write HTML-like syntax directly in your JavaScript code. This makes it easier to visualize the UI structure right where the logic is defined. Here's a simple example:
- Hooks: Reactās hooks, particularly and , enable the use of state and lifecycle features in function components, enhancing the functionality without the need for class components.
- Community and Ecosystem: Being backed by Facebook, React has a robust community. This means there is a treasure trove of shared knowledge, third-party libraries, and tools, making it less daunting for beginners to start learning and becoming proficient.
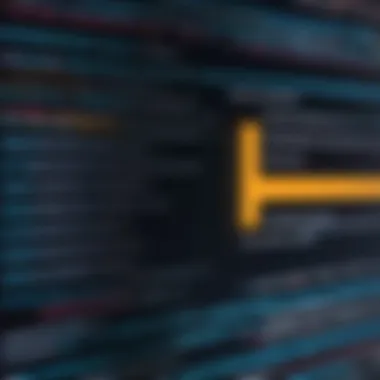
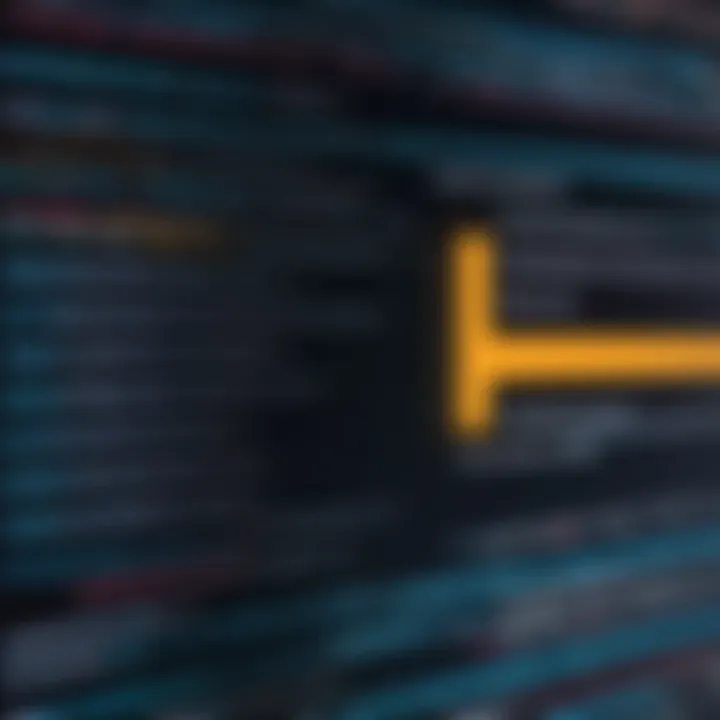
In summary, understanding React is crucial because it not only simplifies but elevates the way developers build user interfaces. The library's focus on components, predictable data flow, and performance optimization lays a strong foundation for modern web development. For anyone embarking on or progressing through their programming journey, wielding the power of React can open numerous doors in the realm of front-end development.
React Architecture
React Architecture serves as the bedrock for every application built using the React framework. Understanding its foundational elements is essential for developers who wish to create efficient and maintainable code. Not just a set of principles, this architecture embodies ideas that touch everything from component organization to performance optimization.
Component-Based Structure
The component-based structure of React is akin to building with Lego bricksāeach piece is a unit that can fit together to form something larger. This modularity gives developers the flexibility to build applications in a way that is both intuitive and scalable. Components can range from simple UI elements to complex functional units that manage their own state and render similar components recursively.
In practice, this means that:
- Reusability is key. A well-constructed component can be repurposed across various parts of your application, minimizing redundancy and maximizing coherence.
- Isolated components facilitate better testing. Each piece can be verified independently, ensuring that issues can be traced back to specific modules instead of an entire monolithic application.
- Hierarchy can simplify the UI. By crafting nested components, developers can create complex user interfaces while maintaining maintainable code. This brings clarity, where it's straightforward to discern what part of the UI corresponds to which piece of code.
For better clarity, letās consider a simple login component that could look like this in JSX:
This snippet illustrates how effortlessly one can create a functional unit without worrying about the global application state.
Virtual DOM: Understanding the Backbone
At the heart of React's performance optimization is the Virtual DOMāa concept that is often touted as a game-changer in web development. Unlike traditional approaches that directly manipulate the browser's Document Object Model, React creates a lightweight representation of the DOM in memory. This allows for faster updates and rendering.
Here's how it works:
- Initial Rendering: When a React app is first loaded, it creates a Virtual DOM that is a mirror of the real DOM.
- Change Detection: When components update, React first updates the Virtual DOM. It then performs a diffing algorithm to compare the previous Virtual DOM with the new one.
- Efficient Updates: Only the necessary parts of the actual DOM are updated, preventing the performance pitfalls associated with direct DOM manipulation.
The benefits of this approach are manifold:
- Improved Performance: By minimizing direct interactions with the DOM, applications become snappier and more responsive.
- User Experience: Smooth transitions and quick state updates lead to a better overall experience for the user.
- Resource Management: React manages resources more efficiently, which can be especially beneficial for applications with complex UI interactions or frequent updates.
"The Virtual DOM is like a safety netāit catches mistakes before any harm comes to the actual DOM."
In summary, the architecture of React empowers developers to write cleaner, maintainable code while also providing a robust mechanism for performance. This structure, combined with the component-based philosophy, creates an environment where not only creation becomes simpler but also understanding the application flow becomes clearer. As with every tool, mastery comes from practice, and knowing these architectural principles is a step in the right direction for anyone venturing into React development.
Setting Up a React Environment
Setting up a React environment is foundational for anyone venturing into the realm of modern web development. Without the proper environment, even the best ideas can struggle to come to life. This section not only highlights why a suitable environment is crucial but also walks you through the essential tools and steps needed to get off to a running start.
Choosing the Right Tools
Before diving into the nitty-gritty of coding, letās first talk about the tools. Should you use a simple text editor, or is an Integrated Development Environment (IDE) better suited for your React project? The choice you make here significantly impacts your productivity.
A well-known tool in the React community is Visual Studio Code. It's lightweight, yet packed with features that support a seamless coding experience, including syntax highlighting and debugging. Another option could be Atom, which is also flexible but might require a bit more effort to customize. The choice often boils down to personal preference, but whatever tool you select, ensure it supports JavaScript and has adequate extensions for React development.
When choosing tools, consider:
- Code Editor: Look for one that allows customization based on your needs.
- Browser: Debugging tools like Chrome Developer Tools are essential.
- Command Line Interface (CLI): A strong grasp of command line operations can enhance your workflow.
Installing Node.js and npm
Next up is Node.js and npm (Node Package Manager). It might feel like overkill for a simple React project, but having Node.js installed is a must. Why? Because React relies on Nodeās runtime to manage packages and dependencies effectively.
To get started:
- Go to Node.js official website and download the version suitable for your operating system.
- Follow the installation instructions on the website.
- Once installed, verify the installation by running the following commands in your command line:This should display the versions installed. If it does, congratulations! You've successfully set up Node.js and npm.
Npm is a powerful tool for managing libraries and dependencies that your project might need. This means you can easily install packages like React Router or Axios without wasting time sorting through files and folders.
"Setting up a proper environment saves headaches down the line!"
Creating Your First React Project
Now, the moment of truth ā creating your first React project. Youāre on the brink of building something exciting! With Node.js and npm in place, the process can be quite seamless. The standard method is to use Create React App, a boilerplate setup making your life as a developer so much easier.
To initialize your project, simply run:
Replace with whatever name you want for your project. This command does the heavy lifting by setting up all necessary directories and files according to React best practices. After completing this setup, your new React app will automatically open in your default browser.
Once you see your app running, youāve crossed a significant hurdle. From here, you can start customizing components and adding functionality, all while feeling like a true developer! This final step leads into a world of endless possibilities in React.
Setting up a React environment might seem daunting initially, but like anything, practice makes perfect. By organizing your workspace and utilizing the right tools, youāll find that creating dynamic web applications becomes second nature.
React Components
React components serve as the building blocks of any application created using the React framework. They're not just isolated snippets of code; they embody a powerful way to break down intricate user interfaces into more manageable pieces. Working with components allows developers to create UI elements that are reusable, maintainable, and scalable. With a well-structured component hierarchy, one can ensure that changes in one part of the application won't send shockwaves through the rest of the code. This modularity is quite beneficial because it enhances both the developer experience and the performance of the application.
Functional vs Class Components
When diving into React components, one encounters two primary types: functional components and class components. Each has its benefits, and understanding their distinctions is crucial.
- Functional Components
Functional components are essentially JavaScript functions that return a React element. They are generally simpler and more concise. This simplicity makes them ideal for components that mainly render UI without much state management. With the introduction of React Hooks, functional components can now manage state and handle side effects, which has further increased their utility. - Class Components
On the other hand, class components are more traditional and are built using ES6 class syntax. They come with more built-in features, such as lifecycle methods which allow actions to occur at specific points in a component's life. Itās worth noting that while versatile, class components can sometimes lead to more complex code due to their extended nature.
"Choosing between class and functional components in React often boils down to the specific needs of your application."
Given that functional components have become the go-to choice for many developers, itās essential to grasp when to use one over the other. Generally, for new projects, starting with functional components and adopting Hooks is advisable. However, understanding class components remains vital for maintaining older projects or dealing with more extensive legacy codebases.
Props and State Management
Props and state are the lifeblood of React components. Props, short for properties, serve as input for components, allowing information to flow in a one-way directionāfrom parent to child. This is crucial as it maintains the integrity and predictability of the component's data flow. For instance, if a parent component holds certain data, it can pass this data down to child components via props, ensuring that child components are only responsible for rendering accurately based on that data.
On the flip side, state refers to a component's local data that can change over time, often in response to user actions or events. While props are immutable to the child component receiving them, state can be altered, allowing for a dynamic user experience. For example, if a user interacts with a button within a component, state can dictate how that component behaves, whether showing a loading spinner or presenting new data.
When managing both props and state, one common approach is lifting state up or using Redux for global state management. This ensures the application remains organized and allows different components to synchronize when it comes to shared data.
Handling Events in React
Handling events in React is a foundational concept that shapes interactive web applications. Unlike traditional web development, where events must be dealt with in a more separated style, React unifies event management. This streamlining aids in building dynamic user interfaces. When you understand event handling, it ultimately translates into a more responsive and user-friendly application.
Event Handling Basics
At its core, event handling in React revolves around binding functions to specific events like clicks, drags, or keystrokes, which are then used to manage actions on the webpage. You will find that React provides a synthetic event system, which is similar to the native event handling. This helps you write consistent code that works across browsers. To get your feet wet, here's a simple example of how to handle a click event:
This snippet showcases a button that triggers a method when clicked. The use of arrow functions ensures the correct context, critical in class components.
Below are some advantages of utilizing event handling in React:
- Seamless Integration: Events are tied closely to the React component lifecycle, which keeps your application organized.
- Reusable Code: By creating reusable event handlers, you simplify the codebase, making it easier to maintain and scale.
- Higher Performance: React's synthetic event system optimizes performance by pooling events, which can be more efficient than handling native events.
Preventing Default Behavior
In many scenarios, you may not want the default behavior to occur when an event is triggered. React handles this gracefully through the method. This comes in handy in forms, where clicking a submit button typically reloads the page. With React, you can control the flow of your application without the risk of it behaving unexpectedly.
Hereās how you might implement it:
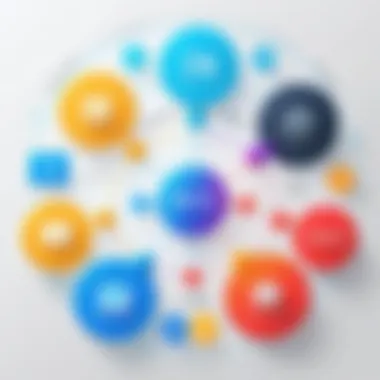
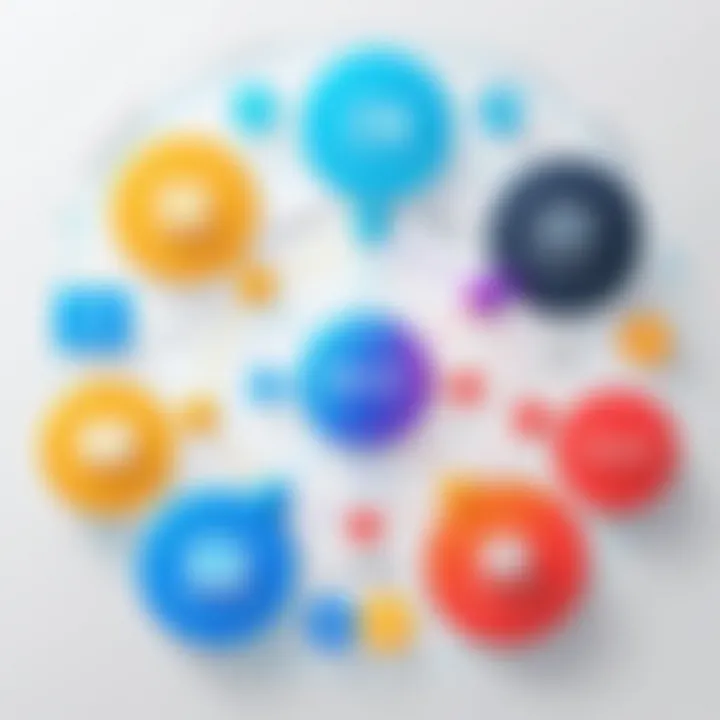
In the example above, the function uses to stop the form from submitting in the traditional way. Instead, it simply logs a message to the console.
Understanding these controls is vital as it allows you to fine-tune user interactions, leading to a smoother user experience.
Important: Always remember to bind your event handlers if you're not using arrow functions; otherwise, will be undefined!
In summary, mastering event handling provides you with the tools to create efficiently managed interactive applications in React. With a robust understanding of how to prevent default behaviors and effectively handle events, you're one step closer to writing apps that truly engage users.
State Management Solutions
In the realm of React, state management stands as a fundamental pillar, shaping how applications handle and respond to data changes. As web applications grow in complexity, the necessity for a robust state management solution becomes increasingly apparent. Effective state management enables developers to maintain the consistency and efficiency of their applications, ensuring a smooth user experience. This topic delves into the significance of state management in React, elucidating various strategies used to manage state effectively.
Understanding Local State
Local state refers to the state that is confined within a single component. Each component in React can hold its own local state using the hook or by creating a class component with . This approach is beneficial as it allows for a simple way to track and manipulate small, self-contained data. For instance, consider a form component that needs to keep track of user input on the fly. Here, you can declare a local state to manage the input values.
This approach keeps things straightforward, but it can get messy when many components need access to the same data. Managing local state works well for isolated scenarios, but as the application grows, developers often face the challenge of prop-drilling where data must pass through intermediate components unnecessarily.
Global State Management with Redux
As applications scale, managing state locally can become cumbersome, leading many developers to adopt Redux, a predictable state container for JavaScript applications. Redux pools all the application state in a central store, allowing access from anywhere in your component tree.
The core idea of Redux revolves around actions and reducers. Actions are plain objects that signal what happened, while reducers specify how the application's state changes in response to those actions. This leads to an architecture that is predictable and easier to debug. With Redux, developers can easily manage state across multiple components without having to prop-drill. Here's a simplified demonstration:
Using Redux can also streamline predictable state transitions, but itās essential to consider the overhead it may add. Learning Redux can be a steep road for some, and its boilerplate code can feel excessive, especially for smaller projects where state management is relatively simple.
Context API: An Alternative Approach
For developers seeking a middle ground between local state management and Redux, the Context API shines as a valuable alternative. Introduced in React 16, Context API allows you to create global state that can be accessed by any component, effectively avoiding the hassle of prop-drilling. It provides a way to share values (like themes or user information) without explicitly passing props through every level of the tree.
Creating a context is just a matter of defining a new context object and wrapping components within a provider, enabling child components to access the state.
Context API is lightweight and excellent for moderate complexity applications. However, it is still crucial to use it judiciously. Excessive updates to context values can lead to performance hits, thus requiring a thoughtful approach to how and when states are modified.
Routing in React Applications
Routing is the vein that carries life through a React application. At its core, routing allows the user to navigate through different views or components seamlessly without reloading the page. This ability enhances the user experience significantly, providing a smoother interaction that feels dynamic and responsive.
In modern web applications, users expect quick transitions and instant feedback. Without a robust routing system, you might find yourself in a sticky situation, losing usersā engagement while maintaining a clunky navigation process. Implementing routing effectively not only boosts usability but also organizes your applicationās structure logically.
Prelude to React Router
React Router is the go-to solution for most developers looking to implement routing within their application. This dynamic library integrates seamlessly with React, allowing for elegant navigation between different components or views. What makes it particularly beneficial are its flexibility and ease of use.
When you install React Router, you gain access to several powerful features:
- Declarative Routing: You can define routes directly in your component structure. This makes understanding the flow of your application crystal clear.
- Nested Routes: This allows creating complex layouts that can appear depending on the routing path, making the application scalable.
- Route Matching: Through pattern-matching, React Router determines which components to render based on the URL passed.
For instance, the setup of basic routing is quite simple:
This snippet shows a straightforward way to define different paths using React Router. Each component maps a URL path to a corresponding component, allowing each link to render the appropriate view.
Dynamic Routing and Navigation
Dynamic routing takes the concept of routing a step further by allowing you to adjust and craft routes at runtime. This becomes especially useful when dealing with dynamic data. For example, if you have a list of users, you can set up a route that leads to a user profile whose ID is derived from the URL.
Consider this example:
Here, signifies a route parameter that will be replaced by the actual user ID when navigating. This means your application can fetch and display personalized data based on the active route. Such flexibility not only makes your application more dynamic but also less redundant since you donāt need to create separate components for each user.
Benefits of dynamic routing include:
- Scalability: A single route pattern can manage numerous views, making it easier to handle large applications.
- User Experience: Users can bookmark or share URLs that directly link to specific content, thus enhancing the overall navigation experience.
- Adaptability: Your routing can adjust based on variables such as user roles or conditions in your app.
In summary, routing is a critical pillar in the building of React applications. An effective implementation of React Router and dynamic routing can turn an otherwise mundane experience into something engaging and responsive, keeping users glued to your application.
Building a React Application
Building a React application is not just a task; it's a pivotal step in leveraging the full potential of what modern web development has to offer. React's ability to create interactive UIs with seamless user experiences makes it a preferred choice among developers. As we dive into constructing a React application, we also unearth the importance of thoughtful project structuring and the creation of reusable components. These aspects are not merely technical feasibilities; they are the building blocks that will define the quality and performance of your application.
Structuring Your Project
When embarking on a journey to build a React application, organizing your project effectively is crucial. A well-structured project leads to cleaner code, easier maintenance, and scalability. Imagine trying to find a needle in a haystackāthatās what navigating a poorly structured project feels like.
Here are some best practices to consider when structuring your React project:
- Create Folders for Components: Organize your components within dedicated folders. Having a central location for all your reusable components can save time when updates are needed.
- Keep Your Files Small: Each component should ideally be contained within its own file. This approach fosters not only clarity but also reusability.
- Group by Features, Not Type: Instead of separating components, actions, and reducers, consider grouping related files together. This aligns with the way users interact with your app.
- Use Clear Naming Conventions: Maintain consistency in naming your files and folders. This clarity will aid both current and future developers who may read your code.
Creating Reusable Components
The concept of reusable components is at the heart of Reactās design philosophy. Simply put, when you create a component, think about its potential for reuse.
Benefits of Reusable Components
- Efficiency: Once you develop a component, you can use it in multiple locations throughout the application without rewriting code.
- Maintainability: If you need to make changes to a component, doing it in one place will reflect throughout your applicationājust like that!
- Consistency: Reusing components promotes a cohesive design. Users see the same element behaving consistently, which enhances their experience.
- Focused Development: By isolating functionalities into components, developers can focus on specific tasks without being overwhelmed by the entire application logic.
Example Component: Here's a simple example of a reusable button component:
Each time you want a button, you could just reuse this component without starting from the ground up, ensuring uniformity and saving time.
Integrating APIs with React
Integrating APIs with React is a crucial aspect of building dynamic and interactive web applications. This process allows applications to communicate with external services, fetching and manipulating data as needed. The ability to interact with APIs empowers developers to create more robust features, such as displaying live data, user-generated content, or even incorporating third-party services into their projects. This section will focus on two main topics: fetching data from a REST API and handling asynchronous operations, both of which play a significant role in the integration of APIs within React applications.
Fetching Data from a REST API
When it comes to fetching data from a REST API in React, understanding the basics of how RESTful services work is a must. These APIs expose certain endpoints through which data can be requested or submitted. Here's why this process is paramount:
- Dynamic Data: Leveraging APIs means you can pull in real-time data, like news updates or social media comments, to keep your app fresh and engaging.
- Efficiency: Instead of manually hardcoding data, fetching it from an API makes the app much easier to maintain and update.
- Separation of Concerns: By using APIs, the front end can operate independently from the back end, enabling teams to work in parallel without stepping on each other's toes.
To implement fetching data, the standard approach is using the API or libraries like Axios. Here's a basic code example using the fetch method:
This snippet shows the fundamental process ā making a GET request to the API, checking if the response is valid, and then processing the data.
Handling Asynchronous Operations
Asynchronous operations are an integral part of modern JavaScript, especially when dealing with APIs. Browsers are expected to provide a seamless user experience, and delaying a user's interaction while awaiting data can lead to problems. Hence, employing asynchronous logic is critical.
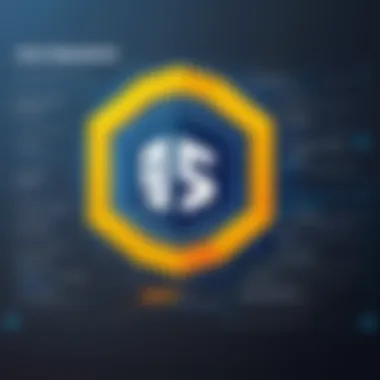
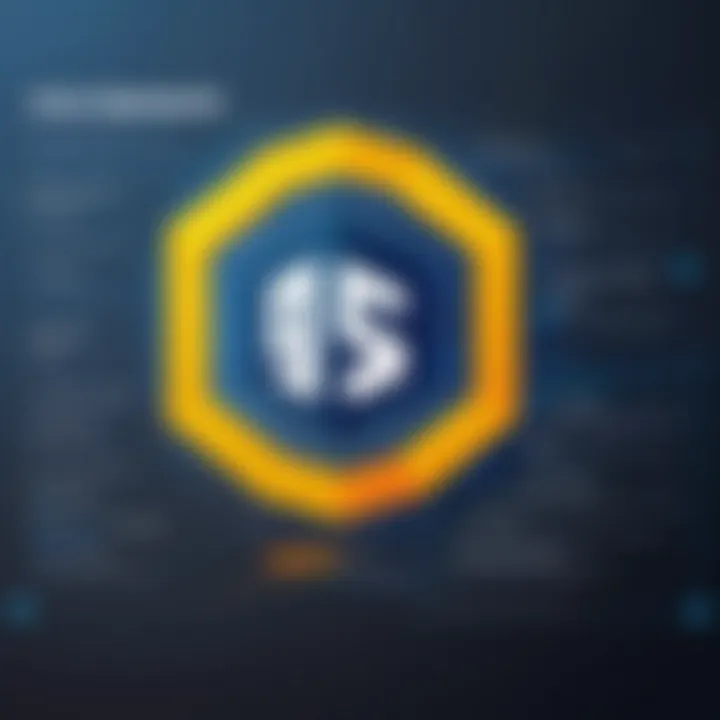
In React, one of the most common ways to handle asynchronous data fetching is through the hook along with and . This provides a straightforward way to handle side effects in functional components. Below is a succinct illustration of handling such operations:
In this snippet, the hook takes care of running the fetch operation after the component mounts. The function handles the response and updates the component state accordingly, allowing for a fluid and dynamic user experience.
Integrating APIs into React apps is not just about fetching data; it's about creating a responsive infrastructure that enhances interactivity and user satisfaction.
Integrating APIs into React applications elevates their functionality and makes them significantly more user-friendly. The ability to handle asynchronous operations ensures that the experience is smooth and snappy, allowing users to engage without frustrating delays. This knowledge is vital for anyone looking to dive deeper into React and modern web development.
Testing React Applications
Testing applications built with React is a fundamental aspect, ensuring high quality and reliability. In web development, the stakes can be high. Users expect applications that are not only feature-rich but also functional without glitches. Testing effectively helps catch issues early on, thus saving both time and effort in the long run. This section will shed light on the importance of testing in React, focusing on key elements such as the types of tests available, the benefits they bring, and what considerations need to be made.
Prolusion to Testing Libraries
Testing libraries serve as essential tools in the React ecosystem, allowing developers to systematically verify the functionality of their components. Libraries like Jest and React Testing Library are staples for many developers, providing utilities that simplify the testing process.
- Jest: This library acts as a test runner with built-in assertion capabilities, created by Facebook. It allows you to run your tests in parallel, significantly speeding up the feedback loop.
- React Testing Library: This focuses on testing components in a way that resembles how users interact with your application. Its focus on accessibility ensures that applications are user-friendly.
"Testing is not just about finding bugs; it's about building solid software."
Using these libraries, you can write unit tests, integration tests, and even end-to-end tests. This approach promotes modularity, meaning that every bit of functionality can be accounted for, leading to cleaner and more maintainable code.
Creating Unit Tests for Components
Unit testing is often the first line of defense in ensuring the integrity of your code. In the realm of React, unit tests validate individual components in isolation, allowing developers to confirm that each piece behaves as expected.
When crafting unit tests for your React components, keep these key points in mind:
- Isolation: Ensure that each test is independent. Each component should be tested with its props and state, so no external influences affect the outcome. This brings clarity to failures when they occur.
- Descriptive Tests: Use clear and descriptive test names. A helpful format is [test description] follows [expected behavior]. This helps anyone reading the tests understand what each one is checking.
- Simulating User Action: Testing isnāt just about checking if a component renders; itās also important to simulate interactions. Libraries like React Testing Library make it easy to simulate user interactions like clicks and form submissions.
Hereās a simple example to demonstrate creating a unit test for a button component:
In this snippet, the test renders the component, simulates a user click, and checks if the expected behavior follows suit. This type of systematic testing ensures components perform reliably in the real world.
Performance Optimization in React
In the fast-paced realm of web development, user experience reigns supreme. When it comes to React applications, performance optimization isnāt just a luxury; itās a necessity. This section delves into the heart of Reactās performance dynamics, offering insights into crucial aspects to fine-tune applications for speed and efficiency. The goal here is to ensure that users experience smooth, lightning-fast interactions without unnecessary loading pauses or glitches that could drive them away.
Optimizing performance isn't merely about reducing load time; it involves scrutinizing the entire lifecycle of your components. Every misstep in performance can result in delayed rendering or stuttered animations, compromising user experience. Therefore, understanding the balancing act between performance and functionality will empower developers to create robust applications that can handle high user traffic and complex operations seamlessly.
Identifying Performance Bottlenecks
Identifying performance bottlenecks is like searching for a needle in a haystack. The first step to optimization is recognizing where the slowdowns happen. Common culprits can include heavy components, unnecessary re-renders, or mismanaged state updates. Letās break it down into digestible parts:
- Component Re-renders: Components can re-render unnecessarily, often because of state or prop changes. Use tools like React DevTools to inspect each componentās render cycles and understand the causes of any excessive re-renders.
- Expensive Computations: Some tasks require significant processing power, especially if they're done every render cycle. Memoizing these calculations using or can drastically improve performance.
- Network Requests: Frequent or redundant API calls can clog up the rendering timeline. Caching strategies or use of libraries such as Axios for efficient HTTP requests can mitigate this problem.
"Finding the bottleneck is half the battle; the other half is knowing how to get past it."
It's crucial to systematically assess performance metrics by utilizing tools like Google Lighthouse or profiling techniques to understand the application's runtime behavior better.
Utilizing React's Built-In Performance Tools
React offers a plethora of built-in tools designed to help developers optimize their applications. These tools allow a closer look at the performance intricacies and facilitate targeted improvements. Some notable tools include:
- React DevTools Profiler: This tool enables developers to visualize component rendering performance and pinpoint parts of the app that are hindering speed. Through detailed graphs and statistics, it shows how often components render and how long those renders take.
- React.StrictMode: While this isnāt a performance tool per se, it can help developers identify potential problems in their application. It intentionally double-invokes certain lifecycle methods, making it easier to spot side effects that might lead to performance issues.
- React.lazy and Suspense: These features allow for the code-splitting of components. It means loading only the necessary parts of your application when theyāre required, thus reducing initial loading times and improving perceived performance.
Leveraging these tools can significantly propel your development process, providing insights that can directly inform your optimization strategy.
In summary, optimizing React applications is an ongoing process that starts with identifying performance bottlenecks and continues through meticulous attention to detail in utilizing what React has to offer. With a keen eye on performance, developers can elevate their applications to meet the demands of todayās users.
Deploying React Applications
Deploying a React application marks a crucial step in the development process. It's where your hard work transitions from local development to the online world, allowing users to interact with your application. Understanding how to effectively deploy a React app is imperative for making your project accessible and ensuring it performs well under real-world conditions.
In this section, you'll see the specific elements involved in deployment, the benefits of doing it correctly, and key considerations that will contribute to the success of your appās launch.
Preparation for Deployment
Before you roll out your React application, you'll want to do some groundwork. It's like making sure your car runs smoothly before taking it on a long road trip. Here are a few essential preparations:
- Code Clean-Up: Review your code for any unused files, components, or libraries. Streamlining your code can lead to smaller bundle sizes and improved load times.
- Environment Variables: Ensure your environment variables are correctly set. Make a note of any API keys or secrets which your app requires and configure them appropriately for the production environment.
- Build Process: Utilize React's built-in command to create an optimized version of your application. This command bundles your app into static files for production. Doing this is essential as it helps in reducing the app size and improves performance.
- Testing: Before deployment, thoroughly test your application. Make sure that all functionalities are working as expected. Testing should not be an afterthought and can save you a lot of headaches later on.
- Performance Optimization: Take advantage of tools like Lighthouse to analyze your appās performance. This can reveal opportunities to enhance speed and user experience.
Once all these preparations are in place, you can confidently move on to the deployment process.
Hosting Options for React Apps
There are numerous options available for hosting your React applications, each with its own advantages. Choosing the right hosting option depends on your specific needs, budget, and scale of your application. Here are some popular choices:
- Netlify: A favorite among developers, Netlify provides continuous deployment through Git integration. The setup is user-friendly, and it offers features like Form handling and Serverless functions.
- Vercel: Similar to Netlify, Vercel focuses on front-end frameworks and offers a robust platform for deploying React applications. It's known for its speed and offers features like automatic scaling.
- GitHub Pages: For static websites, GitHub Pages is a straightforward option. It's a great choice for personal projects or small applications that donāt require complex backend services.
- AWS Amplify: If you need more control and flexibility, AWS Amplify is an excellent option. It allows for easy integration with various AWS services and can scale well with your needs.
- Heroku: For full-stack applications that require a backend, Heroku provides a platform to deploy and manage apps. Its automated deployment process streamlines the workflow.
When deciding where to host your React app, consider factors such as cost, ease of use, scalability, and specific features that may be beneficial to your project.
"The choice of hosting can deeply impact your app's performance, security, and user experience. Always choose wisely!"
Future of JavaScript and React
The trajectory of JavaScript and React is not merely an evolution of code; it reflects the shifting paradigms of web development itself. As we look forward, several key elements emerge that indicate the role both will play in shaping responsive, more engaging user experiences.
First off, the demand for more dynamic interfaces continues to grow. Developers are recognizing the necessity of creating applications that not just function but also resonate with users on a personal level. React, with its component-based architecture, enhances this capacity significantly. By breaking down user interfaces into manageable, reusable pieces, it allows for a quicker update and a seamless user experience. This adaptability to changing demands reflects the essential nature of modern applications.
Moreover, thereās the growing integration of machine learning and artificial intelligence in web applications. JavaScript is at the forefront, adapting libraries like TensorFlow.js that enable developers to run machine learning algorithms directly within the browser. This shift is transforming how users interact with applications, resulting in a more personalized and intelligent user experience. As React continues to simplify the UI building process, developers can focus on integrating these advanced technologies without losing sight of performance and scalability.
"In the era of digital transformation, how efficiently and effectively you code can significantly influence the outcomes of user experience."
Emerging Trends in Web Development
Emerging trends often dictate the lanes in which technology advances. Among these, several stand out in their influence on JavaScript and React's development.
- JAMstack Architecture: Increasingly, developers are gravitating towards JAMstack, which emphasizes decoupling the frontend from the backend. React, with libraries like Next.js, fits seamlessly into this architecture by enabling faster, static site generation while maintaining interactivity. This approach enhances load times and search engine optimizationātwo factors essential for modern web standards.
- Server-Side Rendering (SSR): SSR has become a buzzword in modern web development for its capacity to deliver content more quickly and improve SEO. React supports SSR well through frameworks such as Next.js. This allows web applications to render pages on the server, providing users with quick access to content, positively impacting engagement metrics.
- Progressive Web Apps (PWAs): The concept of PWAs, combining the best features of web and mobile apps is gaining traction. React's flexibility in creating responsive interfaces supports the development of PWAs, making them a core component for developers wanting to enhance user experience.
The Role of JavaScript in New Technologies
JavaScript is not merely standing still; itās evolving and integrating with new tech trends that are reshaping the digital landscape.
- WebAssembly: An emerging technology, WebAssembly allows developers to run code written in multiple languages at near-native speed in the browser. With JavaScript acting as a bridge to WebAssembly, its role in performance enhancement is pivotal. As React apps need to handle ever-increasing functionality, this synergy can deliver more without sacrificing performance.
- IoT and JavaScript: The rise of the Internet of Things is spawning new challenges and opportunities. JavaScript, once confined to browsers, is now stretching its tendrils into server-side applications and beyond. Frameworks like Johnny-Five are enabling JavaScript to communicate with hardware, opening new doors for developers in various domains.
- Blockchain Integration: As blockchain technology finds broader applications beyond cryptocurrencies, JavaScript has adapted with libraries like Web3.js, allowing developers to interact with blockchains directly. React can play a vital role in creating user-friendly interfaces that engage users in these decentralized applications seamlessly.
In summary, the future of JavaScript and React is a landscape rich with opportunities. As developers embrace these upcoming trends and technologies, both JavaScript and React are uniquely positioned to lead the way, solidifying their essential roles in modern web development.
Epilogue
As we wrap up our exploration, it's clear that the understanding of JavaScript and React is not just beneficial, but essential in todayās rapidly-evolving web development landscape. This article provided an in-depth examination of the various facets of React, highlighting its importance as a powerful tool for developers aiming to create dynamic user experiences.
Importance of the End
Focusing on the conclusion, we can underline several critical elements and benefits derived from thoroughly grasping the content discussed:
- Summarization of Knowledge: The conclusion serves as a valuable touchpoint for revisiting the key concepts covered throughout this article. It condenses complex information into digestible insights, making it easier for developers, whether novice or seasoned, to recall fundamental principles.
- Contextualizing Development: Understanding how JavaScript interfaces with React positions developers to leverage both effectively. This synergy enables them to build responsive applications that meet user demands, which reflects the industry's growing expectations for speed and interactivity.
- Forward-Looking Perspective: The conclusion encourages readers to reflect on their progress and future pursuits in web development. By analyzing emerging trends and the ongoing evolution of JavaScript and React, a roadmap for continuous learning and growth becomes apparent.
"The only constant in technology is change itself."
ā Anonymous
Considerations about the Finale
- Itās important to recognize that the learning never truly ends. By embracing a mindset geared towards lifelong learning and adaptation, developers excel in their craft.
- Collaboration with the developer community through resources such as Reddit or open discussions on platforms like Facebook can enhance understanding and foster innovation in programming practices.
- The conclusion also highlights the necessity of practical application. Theory without practice can only get one so far; attempting multiple projects aids in solidifying oneās comprehension and skill set.
The journey through JavaScript and React isnāt a sprint; itās a marathon. With knowledge as the compass and practice as the path, developers are fully equipped to navigate the exciting world of web development. In closing, we aim not just to inform but to inspire a commitment to the craft that will lead to remarkable creations in the digital space.