Understanding Objective JavaScript: A Deep Dive
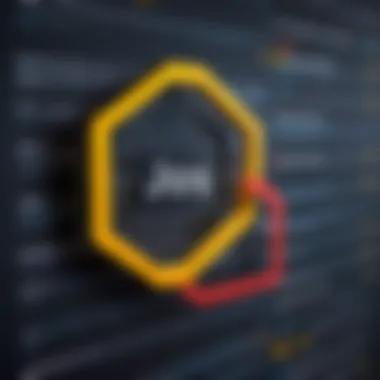
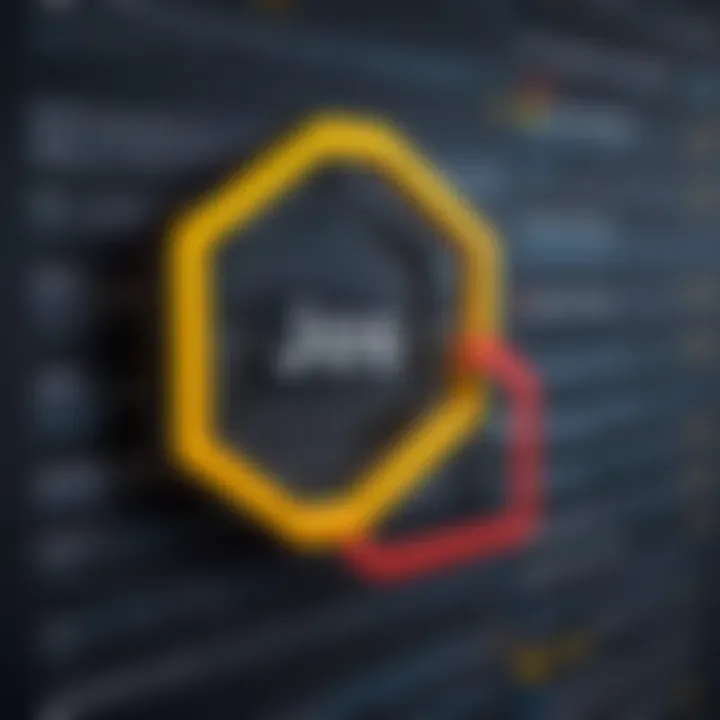
Overview of Topic
Prolusion to the main concept covered
Objective JavaScript represents a significant shift towards object-oriented programming (OOP) within the JavaScript language. Traditionally, JavaScript was viewed as a scripting language with a focus on functions rather than objects. However, with the evolution of programming paradigms and the increasing complexity of web applications, the need for a more structured approach has become vital. This section introduces the essence of Objective JavaScript and its relevance in contemporary development practices.
Scope and significance in the tech industry
In the tech industry, the implementation of OOP principles greatly enhances code maintainability and scalability. Objective JavaScript facilitates the encapsulation of data and behaviors in objects, making it easier to organize code logically. This can lead to fewer bugs and more efficient code reuse, which are significant advantages in the fast-paced world of software development where Agile methodologies can often be employed.
Brief history and evolution
JavaScript's origins date back to 1995, where it primarily played the role of enhancing interactivity on websites. As web applications grew in complexity, developers sought ways to manage this complexity. Over the years, JavaScript has incorporated various programming paradigms, with OOP being one of them. Initially, JavaScript used prototypes for object management. However, modern implementations of JavaScript 6 (ES6) introduced class syntax, further solidifying its ability to support OOP principles.
Fundamentals Explained
Core principles and theories related to the topic
- Encapsulation - Bundling data and functionality in an object.
- Inheritance - Enabling one object to acquire properties from another.
- Polymorphism - Allowing objects to be treated as instances of their parent class, facilitating method overriding.
These principles help in creating more efficient and modular code.
Key terminology and definitions
Familiarizing oneself with the terminology is essential:
- Object: A collection of properties, defined as key-value pairs.
- Prototype: An object from which other objects inherit properties.
- Class: A blueprint for creating objects, introduced in ES6.
Basic concepts and foundational knowledge
To effectively use Objective JavaScript, one must understand objects and prototypes. Objects are the backbone of JavaScript, and prototypes provide a mechanism for inheritance, which is crucial for creating complex applications. Additionally, constructors provide the means to instantiate objects based on classes with defined attributes and methods.
Practical Applications and Examples
Real-world case studies and applications
Numerous applications leverage Objective JavaScript effectively:
- Single Page Applications (SPAs): Frameworks like React utilize OOP concepts for state management and component lifecycle.
- Game Development: Many game engines use OOP to manage game entities and levels, providing clarity in code structure.
Demonstrations and hands-on projects
Consider a simple application of classes in JavaScript:
This example demonstrates inheritance through the class which extends , overriding the speak method.
Code snippets and implementation guidelines
For efficient coding practices, consider the following guidelines:
- Always use or for variable declarations to maintain scope.
- Keep your classes and methods small and focused.
- Use documentation comments to clarify usage and functionality of objects and methods.
Advanced Topics and Latest Trends
Cutting-edge developments in the field
The emergence of TypeScript has introduced static typing to JavaScript, enhancing the OOP experience. This development brings better tooling and error checking, encouraging more rigorous coding standards.
Advanced techniques and methodologies
As applications grow, advanced concepts such as design patterns become highly relevant. Patterns like the Module Pattern or Singleton Pattern can be employed to manage code efficiently within a JavaScript context.
Future prospects and upcoming trends
Moving forward, the integration of OOP within JavaScript is expected to deepen, with emerging libraries and frameworks promoting new paradigms. The shift towards serverless architectures also encourages developers to adopt modular and object-oriented techniques to manage complexity.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
For those wishing to expand their knowledge, consider the following resources:
- "You Donโt Know JS" series by Kyle Simpson.
- FreeCodeCamp offers a variety of practical coding challenges and tutorials.
- MDN Web Docs is an excellent reference for JavaScript language documentation.
Tools and software for practical usage
Utilize tools such as Visual Studio Code and Webpack for an enhanced development environment. Frameworks like Angular or Vue.js can also be instrumental in applying OOP principles in web projects.
Prelims to Objective JavaScript
Definition and Scope
Objective JavaScript encapsulates the principles of object-oriented programming. It defines "objects" which are instances of classes containing properties and methods. A property is an attribute of an object, while a method is a function associated with that object. The concept of scope refers to the visibility and accessibility of these objects and their properties in a given context, determining how and when they can be used. This paradigm empowers developers with a clear structure, facilitating code management as applications evolve.
History and Evolution
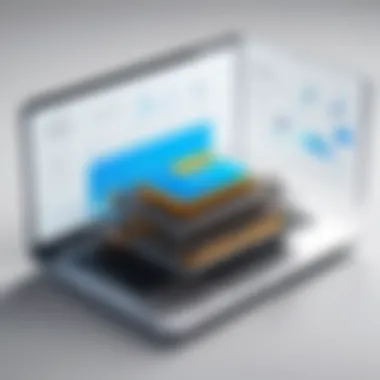
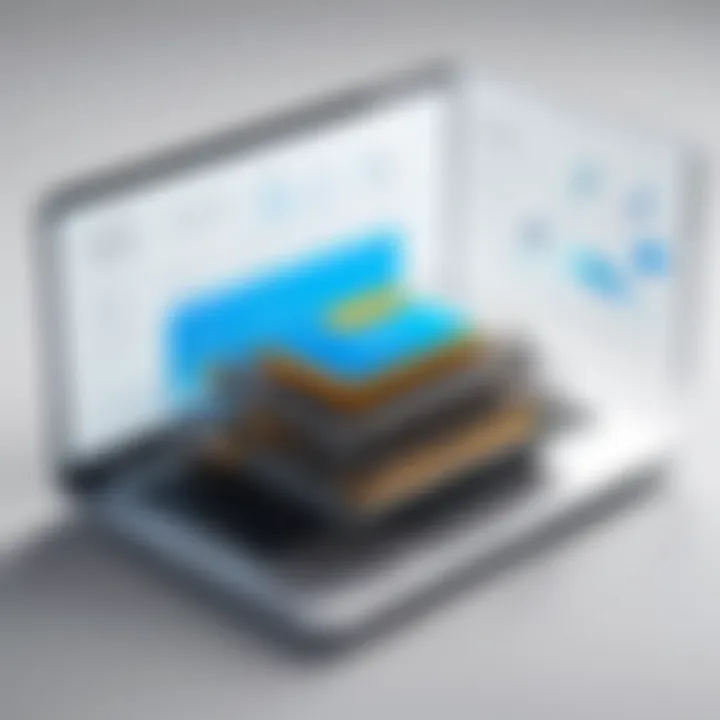
The roots of Objective JavaScript can be traced back to the inception of the JavaScript language itself, created by Brendan Eich in 1995. Initially, JavaScript was primarily procedural. As software development practices evolved and the need for more structured programming approached, concepts of object-oriented programming started to merge with JavaScript. In 2009, ECMAScript 5 was introduced, which further enhanced the support for object-oriented programming features. The introduction of prototypes and inheritance allowed JavaScript to properly utilize the OOP paradigm, pushing developers to consider design patterns and structures that were previously common in languages like Java and C++. This historical context provides a backdrop that shows how essential these extensions have become in today's web development landscape.
Core Concepts of Object-Oriented Programming
Understanding the core concepts of object-oriented programming (OOP) is essential for anyone looking to excel in JavaScript development. OOP promotes a structured approach to programming that can enhance the organization and reusability of code, making it easier to manage complex systems. By leveraging the principles inherent in OOP, developers can create applications that are scalable and maintainable. The central tenets of OOP include the use of objects and properties, methods and functions, and the concepts of encapsulation and abstraction.
Objects and Properties
In JavaScript, an object is a fundamental data structure. It can hold a collection of values as properties, and these properties can be primitive or even other objects. The significance of objects in OOP cannot be overstated; they act as blueprints for creating instances. Properties are the characteristics of these objects and define their state.
Consider that objects can reflect tangible items or abstract concepts within your application. For instance, an object representing a car may include properties like color, model, and year. Here is a simple example:
This means that understanding how to effectively create and manipulate objects is crucial for any JavaScript programmer. Moreover, the ability to define properties allows for more expressive code, leading to easier troubleshooting and maintenance.
Methods and Functions
Methods are functions associated with an object and are vital to enhancing the functionality of that object. A method can change the state of an object or interact with other parts of the application. This is where the power of encapsulation comes into play, as it allows methods to operate on an objectโs properties while keeping them safe from unintended outside interference.
For example, to define a method that changes the color of a car object, one might write:
Methods facilitate behaviors. They provide a way for the object to expose certain functionalities while keeping its internal workings hidden. This aligns well with best practices in OOP, encouraging developers to think in terms of "what" an object does rather than "how" it does it.
Encapsulation and Abstraction
Encapsulation is about bundling the data and the functions that work on that data within one unit, usually a class or an object. This protects the internal state of an object by restricting direct access to some of its components. Instead, it offers a public interface, often in the form of methods, to interact with its properties.
Abstraction, closely related to encapsulation, is the process of hiding the complexity of the implementation. It allows developers to focus on the relevant attributes of an object. This is critical in larger applications where managing complexity is essential to reducing errors and improving code clarity.
In practice, encapsulation and abstraction can work together to minimize how much the user of an object needs to know about its internal workings. This enhances security and maintains the integrity of the data.
Understanding and employing these core concepts of OOP not only improves code organization but also encourages best practices, which are essential for creating maintainable and scalable software.
By grasping the core concepts of OOP, developers empower themselves to write clearer and more efficient code. This foundation applies directly to modern JavaScript development, allowing users to take full advantage of the language's capabilities.
JavaScript Objects
JavaScript objects form the foundation of object-oriented programming in the language. They represent real-world entities and encapsulate data and behavior in a single unit. Understanding JavaScript objects is crucial for writing effective, organized, and maintainable code. Their flexible nature allows developers to create complex data structures with ease, which is essential for modern application development.
Creating Objects
Creating objects in JavaScript can be done in several ways, each catering to different use cases. One common approach is using object literals. This method provides a straightforward syntax for defining an object with properties and methods, making it easy to read and maintain.
This method illustrates how properties such as , , and can be easily defined along with methods like . Another method to create an object is using the constructor, which is more explicit and useful in instances where dynamic object creation is needed.
Each approach has its advantages and should be chosen based on the specific context of the application. Both ways emphasize the flexibility of JavaScript while enabling developers to define custom behavior.
Object Constructors
Object constructors are special functions in JavaScript designed to create multiple similar objects. They act as blueprints, enabling reuse across various instances. When a constructor function is invoked using the keyword, it creates a new object, sets the object's prototype, and binds the keyword to the new object.
Here is an example of an object constructor:
In this case, is the constructor, and is an instance created from it. Using constructors promotes code reuse and provides a clear structure for object definition.
In summary, JavaScript objects are essential to robust application design. By mastering how to create objects and leverage constructors, programmers can significantly enhance their development practices.
Prototypes and Inheritance
Prototypes and inheritance are central concepts in JavaScript, especially in the context of object-oriented programming. They provide a mechanism by which objects can share properties and methods. This promotes code reuse and organizes relationships between objects, allowing for a more structured approach to programming. Understanding these concepts is fundamental for any developer who wants to leverage JavaScript's capabilities to create efficient and maintainable code.
Understanding Prototypes
In JavaScript, every object has a prototype. The prototype is a hidden property that serves as a template from which the object can inherit properties and methods. This inheritance allows developers to avoid redundancy. Instead of defining the same method in multiple objects, one can define it on the prototype and share it across instances.
For example, if you have an object called , you can define a method in its prototype. All instances of , such as or , can use this method without needing to redefine it. This leads to cleaner and more organized code.
Prototype Chain
The prototype chain is the mechanism by which JavaScript resolves properties and methods when they are not found directly on the instance. If an object does not have the property, JavaScript looks up the prototype chain to find it. This continues up through the object's prototype and its prototypes until it reaches the root of the chain, which is .
This search mechanism is critical. It helps maintain a clear hierarchy and ensures that properties and methods can be accessed in a coherent way. However, it also demands that developers understand where properties are defined, to avoid confusion.
The prototype chain underscores the strength of JavaScript's object-oriented design, encouraging the effective organization of code through inheritance.
Inheritance in JavaScript
Inheritance in JavaScript is typically achieved using the prototype system. There are various ways to implement inheritance, which cater to different programming styles and needs.
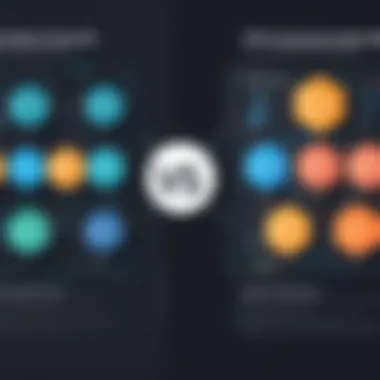
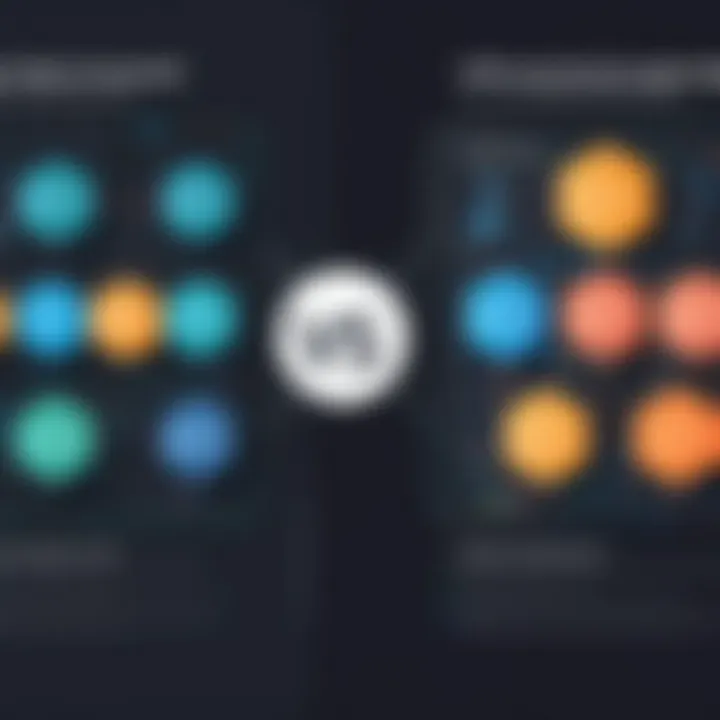
- Prototypal Inheritance: In this form, one object directly inherits from another object. This is the most straightforward method in JavaScript and aligns well with its prototype-based nature.
- Constructor Functions: This is a more traditional approach, where functions act as constructors for new objects. By using the keyword, this structure can facilitate inheritance from parent constructors.
- Class Syntax: Since ES6, JavaScript has introduced a syntax that resembles class-based inheritance. This allows developers to create classes and extends them. It provides a clearer structure and is familiar to those coming from class-oriented languages.
Effective use of inheritance leads to better-organized code and streamlined applications. However, one must tread carefully with deep inheritance chains, as they can lead to complexity and performance issues. Developers should evaluate the need for inheritance versus composition, carefully considering when to use each based on the application requirements.
Object-Oriented Design Principles
Understanding object-oriented design principles is essential for effective software development in JavaScript. These principles provide foundational guidelines for creating scalable, maintainable, and robust code. With object-oriented programming, developers can model real-world entities more accurately, which naturally leads to improved code organization and reusability.
Here are some key benefits of adhering to these principles:
- Enhanced Modularity: Code becomes easier to manage and understand when it is divided into smaller, manageable parts.
- Improved Maintainability: Systems designed with clear principles allow for easier updates and bug fixes.
- Reusability: Code can be reused across different parts of a project or even in different projects altogether.
By incorporating these principles into JavaScript programming, developers ensure that their applications are not only functional but also adaptable to change over time. This adaptability is crucial, particularly in dynamic environments like web development.
"Adopting solid design principles elevates the quality of code and extends its life cycle, aligning well with modern agile methodologies."
Single Responsibility Principle
The Single Responsibility Principle (SRP) states that a class should have one and only one reason to change. It emphasizes that each component of a program should have a single purpose. This delineation is vital in preventing unexpected outcomes caused by changes made for different reasons within the same class.
Consider the following advantages of SRP:
- Reduced Complexity: When responsibilities are well-defined, understanding the code becomes simpler for both the original developer and others.
- Easier Testing: Isolated functionality leads to straightforward testing scenarios, making it easier to identify failures.
In practice, SRP encourages JavaScript developers to think critically about class design. For instance:
In this example, the class focuses on user data, while the handles data storage. Each class has a specific responsibility.
Open/Closed Principle
The Open/Closed Principle (OCP) declares that entities like classes, modules, and functions should be open for extension but closed for modification. This principle promotes the idea of extending existing code without altering it. By doing so, developers can add new features with minimal risk of introducing bugs in already tested code.
Key benefits include:
- Safer Enhancements: New features can be integrated without affecting the core functionality.
- Long-Term Maintenance: Enhancements can be made over time, preventing code from becoming obsolete quickly.
An example in JavaScript might involve using polymorphism and inheritance to extend classes while keeping the base classes unchanged:
Here, remains unchanged while new functionality is added via the class.
Liskov Substitution Principle
The Liskov Substitution Principle (LSP) asserts that objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program. The intent is to ensure that derived classes enhance or refine the behavior of the base class, maintaining the expected functionality.
The advantages of adhering to LSP include:
- Consistent Behavior: Ensures that subclasses function correctly in place of base classes.
- Enhanced Flexibility: Developers can replace classes more freely without fear of breaking existing functionality.
For example:
In this case, a can substitute a without issues, as both share compatible behavior.
JavaScript Classes
JavaScript Classes represent a fundamental shift in how developers can structure their code in an Object-Oriented manner. Introduced in ECMAScript 2015, or ES6, they simplify the creation and management of objects, providing a clearer syntax and enhancing readability. Classes allow developers to create blueprints for objects, encapsulating properties and methods in a cohesive unit. This is particularly advantageous for larger applications, where maintaining organized and reusable code is critical for efficiency and reducing errors.
The importance of embracing JavaScript Classes cannot be overstated. They promote a modular programming approach, making it easier for developers to manage complex code bases. Additionally, the class syntax streamlines the definition of object constructors and enables inheritance, enhancing code reusability and flexibility. Essentially, JavaScript Classes enrich the development experience by reducing boilerplate code and making intentions clearer.
Class Syntax and Usage
The syntax for creating a class in JavaScript is straightforward. A class is defined using the keyword followed by the class name. The basic structure includes a constructor method, which is called when an instance of the class is created. Here's a simple example:
In this example, the class has a constructor that accepts two parameters: and . The method logs the car's details to the console.
When using classes, developers can create multiple instances easily:
This concise syntax enhances clarity and reduces cognitive load, especially for new programmers. The use of classes can significantly aid in structuring applications and is a recommended approach for modern JavaScript development.
Class Inheritance
Inheritance is a pivotal aspect of object-oriented programming, allowing one class to inherit properties and methods from another. In JavaScript, classes can extend other classes using the keyword. This promotes code reuse and establishes a hierarchical relationship between classes.
For instance, if we have a class, we can create a class that inherits from it:
In this code, the class extends , gaining its properties and methods. The keyword is used to call the constructor of the parent class, ensuring that the inherited properties are initialized correctly. Instances of will now have both the properties of and those unique to :
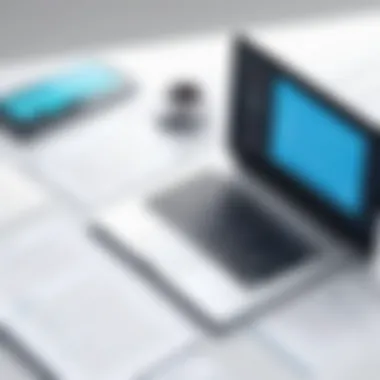
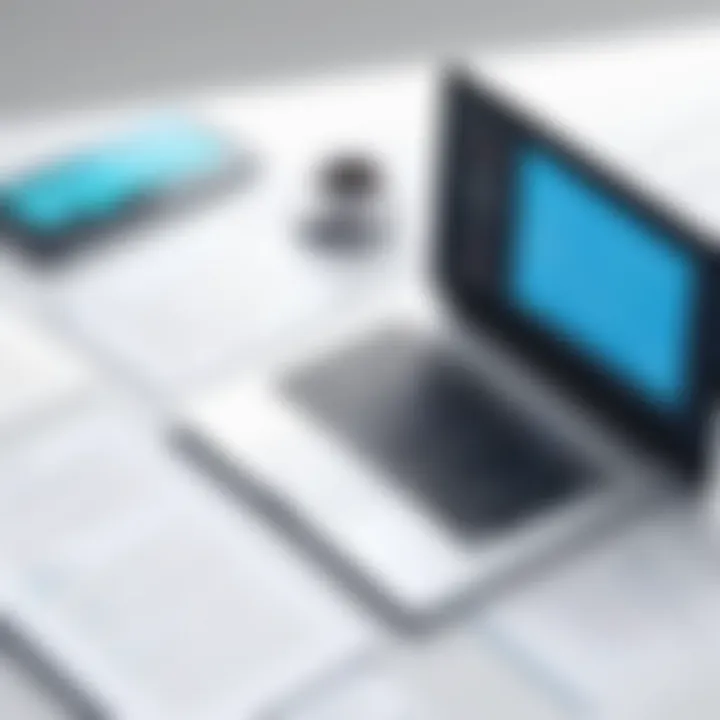
This demonstrates how inheritance allows for building upon existing functionality, fostering a more organized code structure. In summary, utilizing classes and inheritance in JavaScript provides developers precise tools to create robust applications, enhancing both productivity and code quality.
Classes in JavaScript combine a modern syntax with the core principles of object-oriented programming, facilitating cleaner and more efficient code.
Real-World Applications
Real-world applications of Objective JavaScript play a crucial role in showcasing the relevance of object-oriented programming in practical scenarios. Understanding how these principles are implemented in various frameworks and libraries helps developers grasp the full potential of JavaScript. This section focuses on the frameworks and libraries widely used in the industry today, along with significant design patterns that influence programming practices. Integrating design principles effectively allows for better code organization, reusability, and maintainability.
Frameworks and Libraries
Frameworks and libraries simplify many tasks in web development. They encapsulate complex functionality, allowing developers to focus on building applications more efficiently. Popular frameworks include React, Angular, and Vue.js, each offering unique features.
React
React is primarily focused on building user interfaces. Its component-based architecture allows developers to create reusable UI components. This approach promotes consistency and reduces redundancy in code. Reactโs virtual DOM significantly enhances performance by minimizing direct manipulation of the actual DOM, which can be slow. While React is known for its flexibility and strong community support, it comes with a learning curve due to the concepts of state management and lifecycle methods. However, its wide adoption makes it a valuable tool for developers aiming for efficient web-based applications.
Angular
Angular is a comprehensive framework for building single-page applications. It utilizes a two-way data binding process, which means that any changes in the UI are automatically reflected in the data model and vice versa. This feature simplifies the synchronization between the model and view in applications. Angular promotes the use of TypeScript, which adds static typing to the code. This can enhance code quality, but it may require some adjustment for JavaScript developers. Angular's integrated solutions for routing and state management provide a strong foundation for building robust applications.
Vue.js
Vue.js stands out for its progressive framework approach. It allows developers to incrementally adopt its features, making it an excellent choice for both small projects and large applications. Vueโs reactive data binding system is user-friendly, allowing easy integration with existing projects. It has a smaller learning footprint, which benefits new developers. However, as Vue gains popularity, the community support and the availability of resources continue to grow, making it progressively more appealing. Its simple syntax and lightweight nature make it favorable for many developers.
Design Patterns
Design patterns streamline coding practices, allowing developers to adopt established solutions for common problems.
Module Pattern
The Module Pattern serves to encapsulate code within a local scope while providing access to a public API. It helps in reducing the global namespace pollution that often occurs in JavaScript. By organizing code into modules, it promotes reusability and maintainability. The major benefit of this pattern is that it keeps related functionalities together. However, it can lead to potentially complicated code structures if not managed properly.
Observer Pattern
The Observer Pattern establishes a subscription model. This allows one object, known as the subject, to notify other objects, called observers, about changes in its state. This pattern is particularly useful in scenarios where multiple components need to react to state changes. Its main advantage lies in the loose coupling it provides between objects, enhancing flexibility. But it can also become difficult to manage, especially with numerous observers.
Singleton Pattern
The Singleton Pattern restricts the instantiation of a class to a single instance, providing a global point of access to that instance. This approach is beneficial for managing shared resources or configurations. Its key characteristic is preventing the overhead of creating multiple instances, effectively boosting performance. However, it can lead to unforeseen challenges, like difficulties in testing due to its global state.
Understanding these frameworks and design patterns equips developers with essential skills for creating scalable and maintainable applications in Objective JavaScript.
Challenges and Considerations
In any programming paradigm, including Objective JavaScript, it is vital to understand the challenges and considerations associated with its implementation. Effectively navigating these challenges can greatly improve the code's quality and maintainability. Here, we examine two significant aspects that one should be aware of when working with Objective JavaScript: common pitfalls and performance implications.
Common Pitfalls
Objective JavaScript provides many benefits, but it is not without its challenges. Developers can easily fall into traps that undermine the advantages of object-oriented design. Some common pitfalls include:
- Improper Use of Prototypes: Misunderstanding prototype inheritance can lead to unintended behavior in your objects. This might result in methods not being available as expected.
- Over-Encapsulation: While encapsulation helps in organizing code, overdoing it can lead to unnecessary complexity. This can make it harder for others to understand or for oneโs self to maintain the code in the future.
- Ignoring Context: Newcomers often struggle with the keyword, leading to unintentional binding issues. Understanding how works within different contexts is crucial for effective object manipulation.
"Prototypes can confuse even experienced developers, leading to complicated hierarchies. Understanding how to structure your prototype chain is vital."
- Redundant Code: Failing to recognize shared functionality can lead to duplication within different objects. It is crucial to use inheritance to promote code reuse effectively.
Taking time to recognize and avoid these pitfalls will create a smoother development experience and result in more dependable code.
Performance Implications
Performance remains a critical concern in any programming environment. Objective JavaScript is no exception. Itโs important to be mindful of how object-oriented principles can affect performance:
- Memory Usage: Objects consume memory. Each object instance carries its properties and methods. If too many unnecessary objects are created, this can lead to increased memory usage.
- Complexity of Hierarchies: Overly complex inheritance hierarchies can result in longer lookup times when trying to access properties or methods. Simplifying your object structure can improve performance significantly.
- Garbage Collection: JavaScript performs garbage collection to manage memory automatically. But excessive creation and destruction of objects can lead to performance degradation during garbage collection cycles.
Future of Objective JavaScript
The future of Objective JavaScript presents an intriguing landscape for developers, offering multiple pathways towards efficient coding practices and enhanced project management. The adoption of object-oriented programming (OOP) in JavaScript allows for the creation of modular and maintainable code, essential qualities in today's dynamic software environment. Understanding the future of this paradigm not only equips programmers with the necessary tools for modern web applications but also allows them to navigate the evolving JavaScript ecosystem effectively.
As the industry embraces new technologies and methodologies, the relevance of OOP principles continues to grow. By regularly adapting to these changes, developers can ensure they remain competitive and efficient. The integration of modern frameworks and libraries reinforces the significance of Objective JavaScript, as they frequently utilize OOP concepts to streamline development processes. Another key aspect is the collaborative nature of software projects that often necessitate clear structuring of codebases, ensuring that teams can work harmoniously without extensive friction.
Emerging Trends
Emerging trends in Objective JavaScript reflect both the evolution of the language itself and broader shifts within the field of web development. One notable trend is the increasing reliance on TypeScript, which offers strong typing and other features that enhance JavaScript's OOP capabilities. With TypeScript, developers can create applications that are more robust and easier to maintain, while also benefiting from improved tooling and support.
Other prominent trends include:
- Functional programming integration: Many emerging JavaScript frameworks are adopting functional programming features. This helps in designing code that is cleaner and less prone to bugs.
- Microservices architecture: As applications grow in complexity, many developers are embracing microservices instead of monolithic architectures, which align well with OOP principles.
- Serverless computing: This model simplifies the deployment of JavaScript applications and inherently encourages modular programming.
These trends are pushing the boundaries of Objective JavaScript, and they indicate a shift towards a more sophisticated programming approach, one that harmonizes various programming paradigms for most effective and efficient outcomes.
Impact of ES6 and Beyond
The introduction of ECMAScript 6 (ES6) has significantly impacted Objective JavaScript, enriching the language with features that deepen its object-oriented capabilities. With ES6, major advancements such as class syntax, arrow functions, and template literals became available, modernizing how developers can approach OOP in JavaScript.
These features simplify the creation and management of classes and objects. For example, the class keyword allows for a more structured approach to defining objects compared to previous methods. This leads to:
- Improved readability of object-oriented code.
- Enhanced implementation of inheritance through superclasses, allowing for clean and concise specialization of objects.
- The ability to leverage built-in methods and properties easily, facilitating the development of complex applications.
In addition, with the emergence of newer ECMAScript versions, ongoing improvements continue to enhance performance and efficiency. Features like async/await, destructuring, and spread operators contribute to simpler and more powerful code, fostering an overall evolution in how developers conceive and build applications.
As a whole, the impact of ES6 and beyond solidifies the standing of Objective JavaScript, making it a critical area of focus for any developer aiming to create future-ready applications.