Operators in Java: A Comprehensive Overview
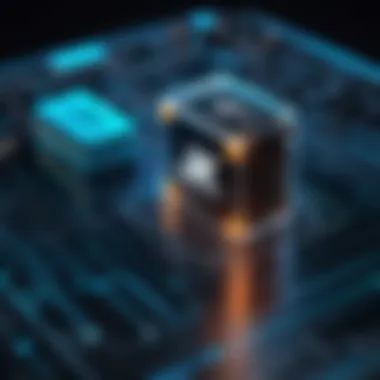
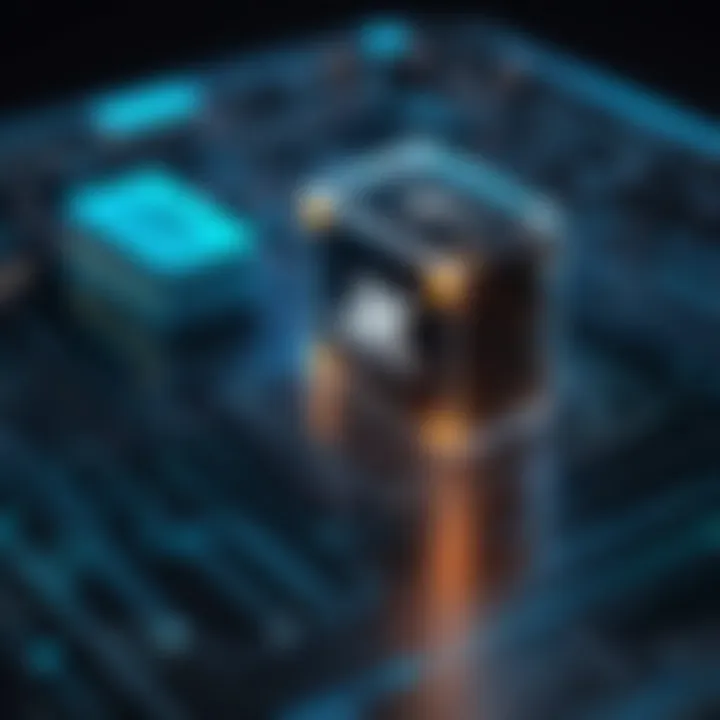
Overview of Topic
Operators play a crucial role in Java programming. They are symbols that perform operations on variables and values. Understanding operators is essential for writing effective Java code. This section will introduce the significance of operators, covering their basic types and relevance in contemporary software development.
Scope and Significance in the Tech Industry
Operators are fundamental for developers using Java, as they directly impact calculations, logic operations, and data manipulation. As Java remains an industry-standard language, proficiency with operators enhances coding efficiency and program optimization. In frameworks and applications, the misuse of operators can lead to errors and inefficient code. Therefore, a grasp of these concepts is valuable for developers in any domain.
Brief History and Evolution
Java was introduced in the mid-1990s by Sun Microsystems. Since then, it has evolved considerably. Early versions provided a basic set of operators. Over time, updates have added features and refined existing functionalities, allowing more complex operations. Today, Java has a diverse and powerful set of operators that enable a wide array of programming solutions.
Fundamentals Explained
To understand operators, one needs to grasp core principles. They can be classified into various types based on their functionality.
Core Principles and Theories Related to the Topic
Operators can be arithmetic, relational, logical, and more. Each type has specific characteristics that define how they interact with data. For example, arithmetic operators perform mathematical calculations, while relational operators compare values.
Key Terminology and Definitions
- Operator: A symbol that signifies operations performed on operands.
- Operand: The data or value on which the operator acts.
- Precedence: The order in which operations are performed in an expression.
- Associativity: The direction in which operations of the same precedence are processed.
Basic Concepts and Foundational Knowledge
A solid understanding of operators lays the groundwork for effective programming. Knowing how to use basic arithmetic operators like + (addition) and - (subtraction) is vital, as these are often the first tools used in any programming task. Understanding how deeply nested operations work is equally important.
Practical Applications and Examples
Operators are not just theoretical concepts; they have practical applications in real-world development.
Real-world Case Studies and Applications
An example of operators in use can be found in data processing applications. For instance, in a Java program that analyzes numerical data, arithmetic operators would calculate averages or totals.
Demonstrations and Hands-on Projects
Consider a simple program that calculates the area of a rectangle. Here is a basic Java snippet:
This example demonstrates the use of the multiplication operator. More complex scenarios can involve logical operators to filter data or check conditions.
Code Snippets and Implementation Guidelines
In Java, a mix of operators can be employed to create comprehensive functions. It is critical to test and validate the outcomes through various scenarios to ensure reliability and precision.
Advanced Topics and Latest Trends
Java continues to evolve, and its operators do too.
Cutting-edge Developments in the Field
With the introduction of Java 8 and later versions, new features such as Lambda expressions have influenced operator usage, particularly in functional programming context. This allows for more expressive and flexible code.
Advanced Techniques and Methodologies
Understanding how to leverage the ternary operator can significantly reduce code length and complexity in certain situations. It allows decision-making within expressions, making it a powerful tool in a programmer's arsenal.
Future Prospects and Upcoming Trends
As Java integrates more with modern technologies such as big data and cloud computing, the role of operators is likely to evolve. Staying updated with these trends is essential for any Java professional.
Tips and Resources for Further Learning
To build a strong foundation in using operators in Java, consider this:
Recommended Books, Courses, and Online Resources
- "Effective Java" by Joshua Bloch provides insight into best practices.
- Online platforms like Coursera and Udemy offer courses focused on Java programming.
Tools and Software for Practical Usage
Using Integrated Development Environments (IDEs) like IntelliJ IDEA or Eclipse can enhance your coding experience. These tools provide debugging features that can help you understand how operators function in your code.
Intro to Operators in Java
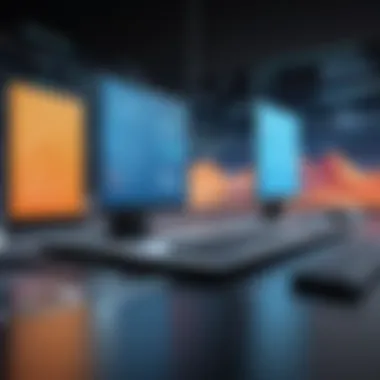
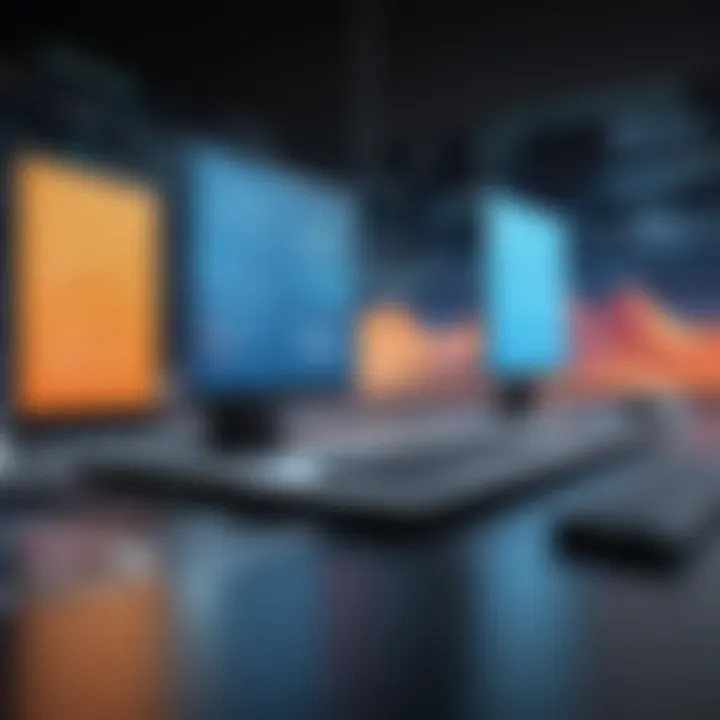
In programming, operators serve as vital components that enable the manipulation and processing of data. They perform operations on variables and values, facilitating the execution of complex expressions. Operators in Java are fundamental to the language's functionality, as they provide the means through which developers can carry out mathematical calculations, compare values, and control the flow of programs. This section aims to shed light on the importance of operators, beginning with a clear definition and subsequently discussing their significance within the realm of programming.
Defining Operators
Operators can be understood as special symbols in Java that instruct the compiler to perform specific mathematical, logical, or relational operations. Each operator has a defined function, and its correct application is essential in writing efficient code. In Java, operators can be categorized into several types, including arithmetic operators, relational operators, logical operators, bitwise operators, assignment operators, unary operators, and the ternary operator. Each type serves distinct purposes in operations involving data manipulation and evaluation. For instance, arithmetic operators like , , , and are used for basic mathematical arithmetic, whereas relational operators such as and `` compare two values and return a boolean result.
Importance of Operators in Programming
Operators play a critical role in enabling programmers to express operations and calculations succinctly. Their functionality allows for concise code, improving readability and maintainability. The significance of operators extends beyond their technical applications. Understanding how these operators work is crucial for any developer. Here are several reasons highlighting their importance in programming:
- Efficiency: Using operators allows for straightforward and effective ways to execute various operations. For example, one can quickly compute totals or differences without verbose code.
- Clarity: Proper understanding of operators enhances code clarity. Knowing how operators function helps to identify errors easily and improve the overall logic of the program.
- Logic and Decision Making: Operators are instrumental in controlling program flow through conditional statements. Logical operators such as (AND) and (OR) assist developers in defining specific pathways through code based on conditions.
Thus, operators are not merely symbols; they are the building blocks of any successful Java program, vital for arithmetic computations, logical checks, and much more.
In summary, operators are essential in the programming landscape, especially within Java. Understanding them not only aids in better coding practices but also builds a strong foundation for advanced programming concepts.
Types of Operators in Java
Operators in Java form the essential building blocks for performing various operations on data. Understanding these types of operators is crucial for any programmer looking to write efficient and clear code. Each category of operators serves a specific purpose, allowing developers to manipulate data effectively. This section will detail the main types of operators found in Java, emphasizing how they can be employed in practical scenarios.
Arithmetic Operators
Arithmetic operators are fundamental for any programming task. These operators allow you to perform basic mathematical operations like addition, subtraction, multiplication, and division. In Java, the primary arithmetic operators include:
- (Addition)
- (Subtraction)
- (Multiplication)
- (Division)
- (Modulus)
The modulus operator is often overlooked but can be quite useful when you need to determine the remainder of a division. For instance, in the case of checking if a number is even, one could use the modulus operator as follows:
This simple example illustrates how arithmetic operators can lead to more complex logic in programs.
Relational Operators
Relational operators are used for comparing two values. They provide Boolean results, thus enabling control flow in programming. The important relational operators include:
- (Equal to)
- (Not equal to)
- (Greater than)
- `` (Less than)
- (Greater than or equal to)
- (Less than or equal to)
For example, if you want to check if a value is greater than another, you can implement this as follows:
This check is common in loops and conditional statements, where flow control is essential.
Logical Operators
Logical operators are used to combine multiple boolean expressions or conditions. They include:
- (Logical AND)
- (Logical OR)
- (Logical NOT)
Logical operators are essential for creating complex logical conditions. For instance, if you want to ensure that both conditions must be true, you can use the logical AND like so:
This code snippet demonstrates how you might handle multiple conditions succinctly.
Bitwise Operators
Bitwise operators manipulate individual bits within integer values. In Java, these operators include:
- (Bitwise AND)
- (Bitwise OR)
- (Bitwise XOR)
- (Bitwise NOT)
- `` (Left shift)
- (Right shift)
Bitwise operators can be beneficial in scenarios requiring low-level data manipulation, such as hardware-related programming or optimization of memory usage. An example of a bitwise operation is:
Assignment Operators
Assignment operators are used to assign values to variables. The basic assignment operator is , but several compound operators combine assignment with other operations:
- (Addition assignment)
- (Subtraction assignment)
- (Multiplication assignment)
- (Division assignment)
- (Modulus assignment)
For example:
This concise way of modifying the value can simplify code, improving readability and maintainability.
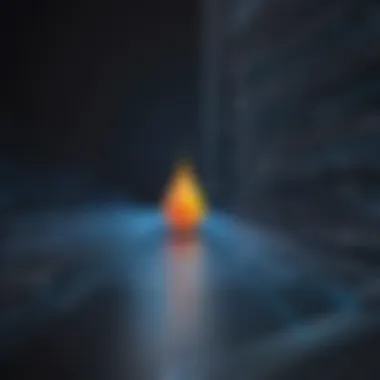
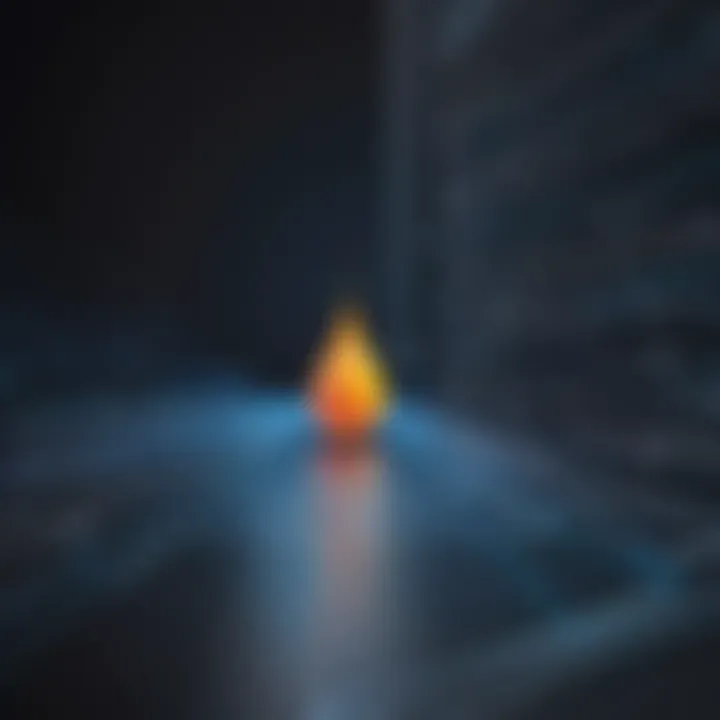
Unary Operators
Unary operators act on a single operand and include:
- (Unary plus)
- (Unary minus)
- (Increment)
- (Decrement)
For instance, incrementing a variable can be done as follows:
This operator is particularly useful in loops and counters.
Ternary Operator
The ternary operator is a unique operator in Java that simplifies conditional expressions. It is expressed as:
This operator provides a way to reduce the code size when a simple if-else statement is required. A practical example looks like this:
In this case, the maximum of and is assigned to the variable in a concise manner.
Understanding these diverse types of operators is essential for any programmer. Each type serves its purposes and enhances the power of Java, allowing for more intelligent and efficient coding practices.
Operator Precedence in Java
Operator precedence is a fundamental concept in programming, particularly in Java. Understanding this concept allows developers to write clear and effective code. Operator precedence determines the order in which different operators are evaluated in an expression. This is crucial because it affects how expressions are computed and can lead to different results if not understood correctly.
When developers execute mathematical or logical expressions, knowing which operators take priority can save time and prevent errors. It also contributes to cleaner, more maintainable code. A clear grasp of precedence helps avoid misunderstandings and bugs that arise from assumptions about the order of operations.
Understanding Precedence
Precedence rules in Java dictate that certain operators are given priority over others. For instance, multiplication and division are evaluated before addition and subtraction. This means in an expression like , the multiplication will occur first, resulting in , which equals , rather than if evaluated left to right.
A well-defined precedence table helps programmers predict the outcome of complex expressions. This knowledge is key for writing robust code that behaves as expected. A sound understanding of these rules improves logical reasoning during coding.
List of Precedence Levels
Java operators are not all equal; they have different levels of precedence. Here is a simplified list of operator precedence levels in Java:
- Postfix: ,
- Unary: , , , , ,
- Multiplicative: , ,
- Additive: ,
- Shift: ``, ,
- Relational: ``, , , ,
- Equality: ,
- Bitwise AND:
- Bitwise XOR:
- Bitwise OR:
- Logical AND:
- Logical OR:
- Ternary:
- Assignment: , , , , , etc.
The precedence of operators can greatly influence the results of your expressions. Prioritize understanding them to enhance your programming skills.
By keeping these precedence levels in mind, developers can anticipate how various operators interact and affect the evaluation of expressions. This understanding is vital for writing precise and bug-free code.
Operator Associativity
Operator associativity plays a significant role in how expressions are evaluated in Java. It determines the order in which operators of the same precedence are applied. Understanding this topic is essential for both novice and experienced programmers. A correct grasp of associativity not only helps in writing clear and predictable expressions but also aids in debugging complex code. Since many operators in Java share the same precedence, knowledge of their associativity becomes crucial. Without this understanding, one might face unexpected results in their calculations or logic operations.
Defining Associativity
Associativity defines how operators of the same precedence are grouped in the absence of parentheses. There are primarily two types of associativity in programming:
- Left-to-Right Associativity: This means that an expression is evaluated from the leftmost operator to the rightmost operator. For example, in the expression , the operation is conducted as `(a - b) - c.
- Right-to-Left Associativity: In contrast, this means the evaluation occurs from the rightmost operator to the leftmost operator. A common example is the assignment operator in Java, where is interpreted as `a = (b = c).
When forming expressions, understanding which way the associativity runs can alter the outcome significantly. Misinterpretation may lead to errors in logic that are difficult to trace.
Left-to-Right and Right-to-Left Associativity
In Java, most operators have left-to-right associativity. This includes arithmetic and relational operators. Thus, when expressions are evaluated, these operations occur sequentially from left to right. For example:
The evaluation order for this expression occurs as follows: first is computed, and then is calculated, leading to a final result of .
However, certain operators, like the assignment operator, use right-to-left associativity. Using the same assignment example:
Here, first takes the value of , and then is assigned the value of (which is ). The order of evaluation matters significantly and impacts the overall logic.
Understanding the nuances of operator associativity, especially in a language like Java, is crucial for crafting accurate and reliable code.
Practical Examples
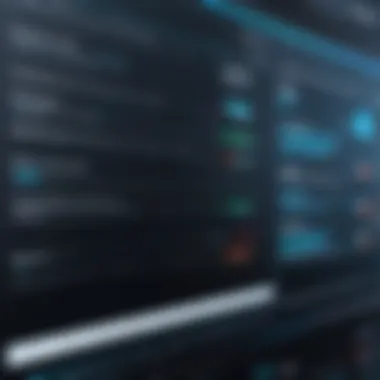
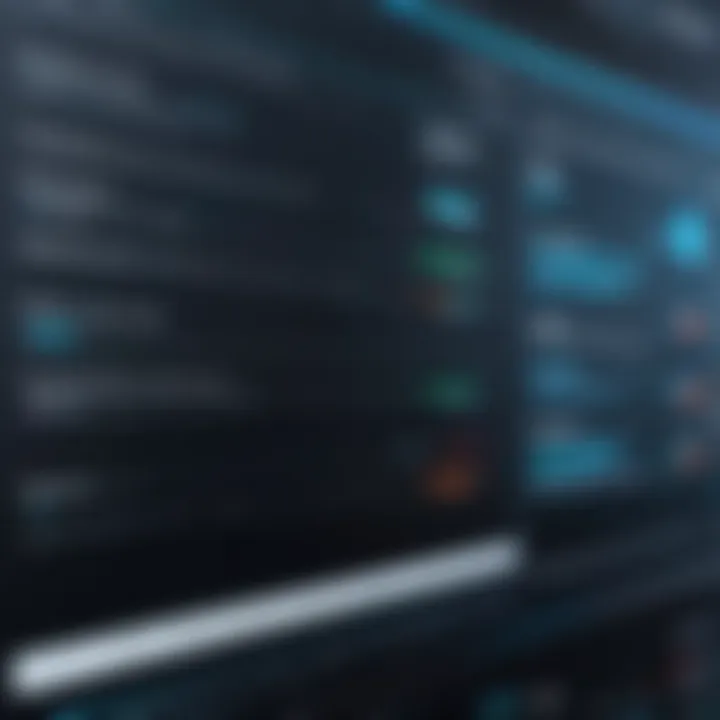
Practical examples hold a significant role in understanding operators in Java. They translate concepts into tangible instances, making it easier for readers to grasp how operators function within the programming environment. In a language like Java, where dozens of operators exist, seeing them applied in real scenarios clarifies their purpose. Moreover, practical implementations provide insight into common pitfalls that programmers encounter.
By engaging with examples, readers not only enhance their comprehension but also cultivate their problem-solving skills. Such skills are critical in programming as they enable individuals to approach coding challenges with confidence and creativity. It's also important to note that examples serve as foundational stepping stones towards mastering more complex programming tasks.
Using Arithmetic Operators
Arithmetic operators in Java are fundamental. They perform basic mathematical operations: addition, subtraction, multiplication, division, and modulus. These operators are essential for numerical calculations in any programming task. For example, if a developer needs to calculate the total of a shopping cart, they will use the addition operator to sum item prices.
Hereโs a simple code snippet demonstrating the use of arithmetic operators:
These operators are straightforward yet powerful. Understanding how to use them effectively is key for any Java programmer.
Implementing Logical Operators
Logical operators in Java primarily deal with boolean values. They evaluate expressions and return true or false. The three main logical operators are AND (), OR (), and NOT (). They are crucial in writing conditional statements and controlling the flow of programs.
For instance, a programmer may need to check whether a user input falls within a specific range. Hereโs an example of how logical operators can be applied:
This demonstrates a practical application of logical operators, essential for making decisions in code development.
Practicing with Bitwise Operators
Bitwise operators manipulate individual bits of integer data types. They allow developers to perform operations like AND (), OR (), NOT (), XOR (), left shift (``), and right shift (). Understanding bitwise operations is vital for performance optimization and low-level programming tasks.
Hereโs how a few bitwise operators can be implemented:
Bitwise operators are less commonly used but can provide powerful capabilities when needed. Practicing their usage is recommended for developers wishing to explore the depths of Java programming.
Common Mistakes with Operators
Understanding operators in Java is key for any aspiring programmer. Recognizing common mistakes can notably improve coding skills. Often, the pitfalls in the use of operators arise from misunderstandings regarding their behavior, especially when dealing with operator precedence and associativity. These concepts are not just theoretical; they have practical implications for how expressions are evaluated in Java. By addressing these common errors, readers can avoid unexpected results in their programs.
Misunderstanding Precedence
Operator precedence determines the order in which various operators are evaluated in an expression. Many beginners assume that all operations are performed from left to right. This assumption leads to errors when higher-precedence operators are involved. In Java, for example, the multiplication operator has a higher precedence than the addition operator . Therefore, in an expression like , the multiplication is performed first, resulting in , which equals , not .
To avoid this mistake, itโs essential to familiarize yourself with the precedence rules in Java. Below is an example to illustrate:
To further understand this, consider using parentheses to make expressions clearer. For instance, writing the expression as reinforces the order of operations and might prevent confusion.
Incorrect Use of Associativity
Associativity defines how operators of the same precedence are processed in expressions. Java operators can be left-to-right or right-to-left associative. A common error occurs when a programmer misuses this concept, especially with the assignment operators.
For instance, the assignment operator is right associative. In an expression like , Java evaluates it as . If not understood correctly, this can lead to unexpected behaviors. Many people mistakenly think the assignment happens from left to right, which does not yield the same results.
To illustrate:
This subtlety can lead to bugs, especially in larger codebases where operator logic may not be evident at first glance. It's vital for programmers to clarify the associativity of operators they are using to ensure correct logic in their code.
Correct understanding of operator precedence and associativity can enhance code quality significantly.
By being aware of these common mistakes, programmers can improve their proficiency in Java and produce more reliable, efficient code.
Closure
The conclusion serves as a vital part of this article on operators in Java. It encapsulates the essential insights gained throughout the discussion while reinforcing the practical utility of these concepts. Understanding operators enables programmers to craft efficient algorithms and enhance code performance. Operators are the building blocks of expressions, thus directly influencing the logic and effectiveness of programming tasks.
Summary of Key Points
In the previous sections, we have explored various categories of operators, including arithmetic, relational, logical, bitwise, and others. Each type has its unique role:
- Arithmetic Operators like , , , and facilitate basic mathematical computations.
- Relational Operators allow comparisons, essential for decision-making processes, using operators like and .
- Logical Operators such as and combine multiple boolean expressions, crucial for complex logical evaluations.
- Bitwise Operators enable manipulation at the binary level, making them useful for low-level programming tasks.
- Assignment Operators streamline variable assigning by combining with arithmetic operations.
- Unary and Ternary Operators offer shorthand solutions for common conditions and operations.
Additionally, understanding operator precedence and associativity helps prevent errors that can arise from misinterpreting the order of operations.
Further Readings
To deepen your understanding of operators and their implications in Java programming, consider exploring these resources:
- Wikipedia - Java (programming language)) for a broader view of Java's capabilities and features.
- Britannica - Java for historical context and significance in the programming landscape.
- Reddit - Programming Talk for community insights and discussions on Java and other programming languages.
- Facebook - Developer Community for networking and collaborative learning opportunities.
These references can provide practical examples and discussions, enhancing the comprehension of operators in Java.