Understanding Python Performance: An In-Depth Exploration
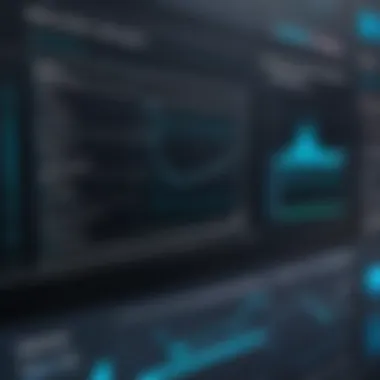
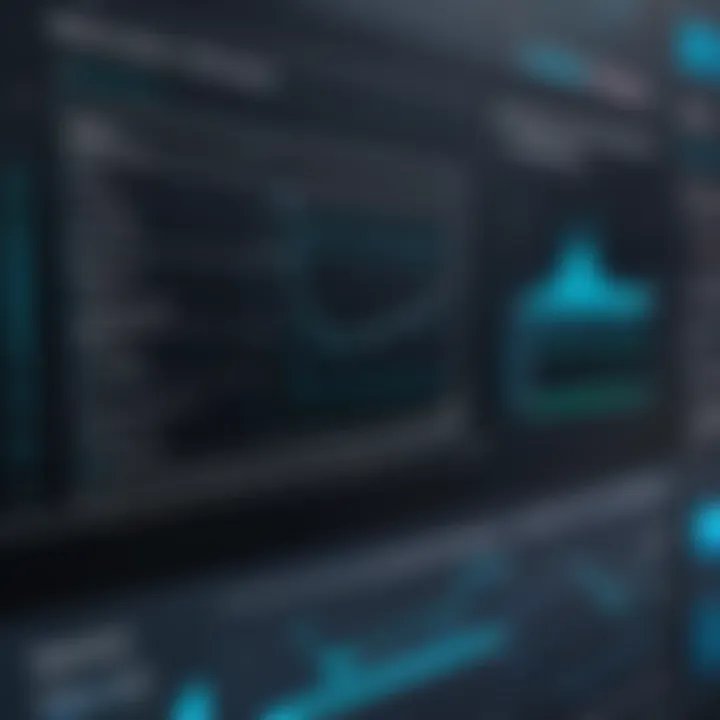
Overview of Topic
Python is a programming language that has gained immense popularity in various sectors, including web development, data analysis, artificial intelligence, and more. The efficiency and performance of Python are crucial for both hobbyists and professionals who seek to develop robust applications.
Understanding its performance is significant because it influences the choice of Python over other languages in tech industries. Performance affects system resource consumption, application speed, and overall user experience. Knowing how to optimize Python can lead to significant gains and competitive advantages in projects.
The language has evolved substantially since its inception in the late 1980s. Originally developed by Guido van Rossum, Python aimed to enhance code readability while maintaining simplicity. Over the years, updates and community contributions have broadened its capabilities, making it a versatile tool in the modern programming toolbox.
Fundamentals Explained
To grasp Python's performance, one must understand some core principles.
Core Principles:
- Interpreted Language: Python is an interpreted language, meaning that code is executed line by line. This can lead to slower execution time when compared to compiled languages.
- Dynamic Typing: The dynamic nature of Python allows flexibility, but it can lead to performance slows during runtime type checking.
Key Terminology:
- Execution Time: Time taken by Python to execute a program.
- Throughput: Number of units of output a system can produce in a given period.
Understanding these terms allows for a more informed approach to performance analysis.
Practical Applications and Examples
In real-world applications, Python’s performance can be critical. For example, web applications built with Django may require optimization for handling high traffic volumes. Here are a few approaches:
- Use of C libraries: Integrating C libraries can drastically improve execution time.
- Concurrency: Implementing asynchronous programming can lead to better resource management.
Consider this simple implementation using the module for concurrent execution:
This code snippet demonstrates how Python can utilize multiple processes to perform tasks simultaneously, enhancing efficiency.
Advanced Topics and Latest Trends
As technology evolves, so do the approaches towards optimizing Python. Recent trends focus on:
- JIT Compilation: Just-in-time compilation bridges the gap to improve execution speed. Projects like PyPy utilize this technique effectively.
- Performance Monitoring Tools: Tools such as Py-Spy and cProfile aid in profiling Python applications, identifying bottlenecks and optimizing them accordingly.
The future holds promise with advancements in Python’s own structure and performance capabilities. These advancements aim to preserve Python's usability while enhancing its performance metrics significantly.
Tips and Resources for Further Learning
For those interested in delving deeper into Python’s performance, consider the following resources:
- Books:
- Online Courses:
- Tools:
- "Fluent Python" by Luciano Ramalho
- "Python Performance Tuning" by Gabriele Lanaro
- Coursera’s "Programming in Python for Everybody"
- edX’s "Introduction to Computer Science and Programming Using Python"
- Py-Spy for profiling
- memory_profiler for analyzing memory usage
These resources provide a solid foundation for anyone eager to enhance their understanding of Python’s performance.
"Optimizing performance is not just about speed; it's about efficiency and resource management."
By comprehensively examining Python's performance, one can effectively navigate its complexities while maximizing its potential.
Prolusion to Python Performance
Python's performance is a fundamental aspect that can significantly influence not just how applications run, but also user satisfaction and resource allocation. In an era where computational efficiency is often paramount, understanding Python performance is essential for developers, particularly for those working in domains demanding high throughput and rapid response times. This section aims to dissect the elements that contribute to performance, offering insights into how they interrelate and impact real-world applications. Performance in programming encompasses aspects such as execution speed, memory consumption, and scalability. All these factors can determine the success or failure of a project.
The Importance of Performance in Programming
Performance is critical in programming for several reasons. Firstly, efficient code can lead to faster application response times. When software performs well, users are more likely to have a positive experience. This is crucial for applications that operate in competitive markets, where every millisecond can count.
Moreover, good performance can optimize resource usage. When an application runs efficiently, it consumes less memory and CPU time, which can lead to lower operational costs. For example, Python applications utilizing the cloud benefit greatly from performance optimization, as it directly relates to billing structures.
Lastly, maintainability is another element closely tied to performance. Code that is performance-conscious tends to be more structured and clear. Developers can quickly identify bottlenecks, making it easier to implement updates or scale as needed.
What Constitutes Good Performance?
Good performance can sometimes be subjective, yet certain metrics are widely accepted as indicators.
- Execution Speed: The organization of logic, choice of algorithms, and overall runtime affect how quickly a script executes. Generally, lower elapsed time indicates better performance.
- Memory Usage: Efficient use of a program’s memory plays a vital role in performance evaluation. Applications capable of running without exhausting system memory show a level of optimization that is often desirable.
- Scalability: An application's ability to maintain performance levels as load increases is crucial. This involves not just how well it performs under normal conditions, but also how it manages increased demand.
Understanding these aspects leads developers to create applications that are not just functional, but are also proficient in terms of performance. Thus, diving deeper into Python performance can yield significant advantages both in development and user engagement.
"Performance is not just about speed; it is a quality that encompasses efficiency, scalability, and maintainability."
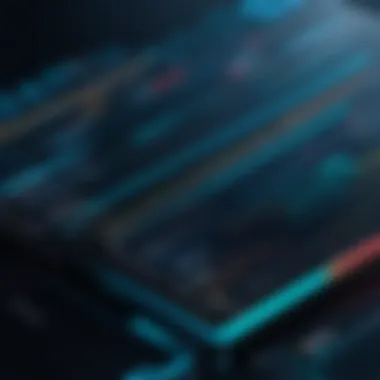
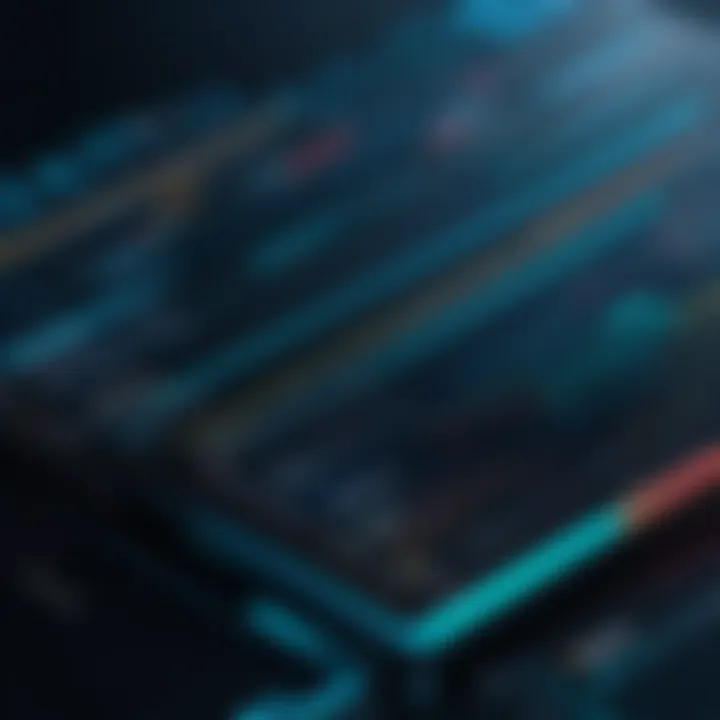
By grasping these considerations, developers can intelligently approach performance issues, applying solutions specific to the challenges they face. In the following sections, we will explore the key factors influencing performance, tools for measurement, and optimization strategies that can help enhance Python applications.
Key Factors Influencing Python Performance
Understanding the key factors that influence Python performance is crucial for anyone looking to optimize their applications. Performance is not just a matter of speed; it includes how efficiently code utilizes resources, responds to inputs, and scales as data demands grow. Recognizing these factors helps programmers make informed choices that impact usability and efficiency.
Interpreted vs. Compiled Languages
The distinction between interpreted and compiled languages is fundamental in understanding Python's performance. Python is an interpreted language. This means that, unlike compiled languages such as C or C++, Python code is executed line by line at runtime. This may lead to slower performance, as interpreted languages generally do not leverage the same optimizations available to compiled languages.
Advantages of Interpreted Languages:
- Easier debugging: Errors can be identified and corrected on-the-fly.
- Dynamic execution: Code can be run without a prior compilation step.
Disadvantages:
- Slower execution compared to compiled counterparts.
- More overhead due to interpretation, which can impact performance in computationally intense applications.
Dynamic Typing and Its Implications
Dynamic typing is one of Python's defining features. This means variables do not have a fixed type and can change at runtime. While this offers flexibility and ease of use, it can result in performance drawbacks.
Implications of Dynamic Typing:
- Overhead in type checking during execution can slow down performance.
- Potential increases in memory usage if types evolve or become complex.
Dynamic typing allows for rapid development and experimentation, yet it's essential to recognize that it can cause inefficiencies, especially in high-performance contexts. Developers must balance the need for dynamism with the implications for performance.
Memory Management in Python
Memory management is another key aspect influencing Python performance. Python uses an automatic memory management mechanism, which includes garbage collection. This means that programmers do not explicitly manage memory allocation and deallocation.
How Memory Management Affects Performance:
- Garbage collection introduces overhead. While it ensures freed memory, it can halt the execution of programs while it works, leading to delays.
- Memory allocation is done dynamically, which can lead to fragmentation and inefficiencies.
In practice, understanding the memory model in Python can guide developers to create more efficient applications. Being mindful of data structures and when they get allocated and deallocated can assist in minimizing unnecessary overhead.
"Performance optimization starts with understanding the language's core features."
By grasping the fundamental factors influencing Python's performance, developers can make strategic choices that improve the speed, efficiency, and effectiveness of their applications.
Measuring Python Performance
Measuring Python performance is a critical aspect of understanding how a Python application behaves in terms of efficiency, speed, and resource utilization. The performance of a program can have significant implications for user experience, resource consumption, and scalability. By accurately measuring performance, developers can identify bottlenecks and optimize their code, leading to better-performing applications. Effective performance measurement allows for informed decision-making when it comes to optimizing code and improving application responsiveness.
Benchmarking Techniques
Benchmarking is a systematic way to quantify the performance of code under specific conditions. It involves running a set of tests to gather data on execution time and resource usage. Certain methods for benchmarking are more effective than others, and their usage can greatly influence the reliability of the results. Common techniques include:
- Microbenchmarks: Focus on small, isolated code snippets to analyze individual components.
- End-to-End Benchmarks: Measure the performance of the entire application under realistic load conditions.
- Load Testing: Involves simulating heavy usage to evaluate performance under stress.
Properly implemented benchmarking can reveal important insights that inform performance enhancements in Python applications.
Common Performance Metrics
To measure Python performance, some specific metrics are essential to understand. These metrics provide quantitative data that help developers analyze their code efficiently. Key performance metrics include:
- Execution Time: Represents how long a piece of code takes to run.
- Throughput: Measures the number of operations or transactions completed in a given timeframe.
- Latency: Indicates the delay before a transfer of data begins following an instruction.
- Memory Usage: Tracks how much memory a program consumes during execution.
Considering these metrics helps developers to pinpoint areas for improvement, enabling them to write more efficient code.
Profiling Tools for Python
Profiling tools play a key role in measuring performance, offering insights into how well the code runs and where optimizations are necessary. Here are some noteworthy profiling tools available for Python:
cProfile
cProfile is a built-in profiler in Python that provides a detailed report of where time is being spent in a program. It captures function-level statistics, making it easier to understand which functions are the most resource-intensive. It is widely used due to its availability and ease of use.
Key characteristics of cProfile include:
- Ease of Integration: cProfile can be used as a command line utility or a module within code, making it convenient for quick assessments.
- Detailed Output: The profiler generates a comprehensive report, allowing for in-depth analysis of function calls.
Advantages of using cProfile:
- It's part of the standard library, ensuring no additional installation is needed.
- It can track time spent in calls, giving a broad view of function performance.
line_profiler
line_profiler is focused on line-by-line profiling, which allows developers to identify performance issues down to specific lines of code. This granularity enables a deeper understanding of how code execution impacts resource use.
Notable features of line_profiler include:
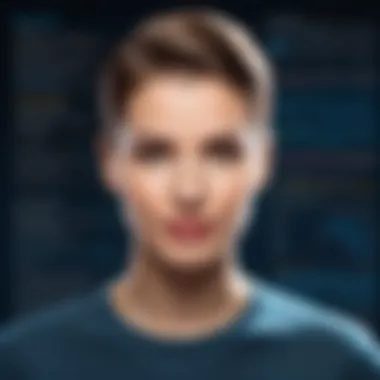
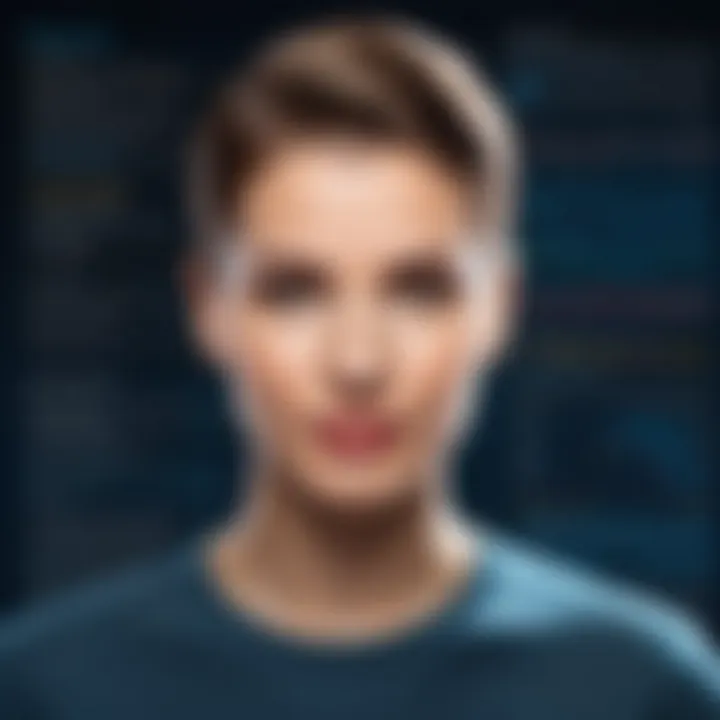
- Precision: By highlighting individual lines, it provides a clear view of performance impacts.
- Integration: It can be easily integrated into existing projects to enhance profiling capabilities.
However, line_profiler does require decorators to mark functions for profiling, which can introduce additional overhead and requires discipline when deploying.
memory_profiler
memory_profiler is tailored specifically for memory usage analysis in Python applications. Like line_profiler, it provides detailed insights but focuses on memory consumption instead of processing time.
Key aspects of memory_profiler:
- Real-Time Monitoring: It can show memory usage over time, thus allowing developers to see how memory consumption changes during execution.
- Line-by-Line Analysis: Similar to line_profiler, it can provide memory consumption for each line of code.
Advantages of memory_profiler:
- It's essential for optimizing memory-heavy applications, helping detect memory leaks or excessive usage.
- Clear visualizations make it easier for developers to interpret results.
By employing these profiling tools, Python developers can refine their code's performance, ultimately leading to better application designs and more efficient resource utilization.
Common Performance Pitfalls in Python
Understanding common performance pitfalls is critical for anyone working with Python. These pitfalls can significantly impact the efficiency and speed of applications. By identifying these areas, developers can make informed decisions to enhance their code. This section highlights some specific issues that can lead to decreased performance.
Inefficient Data Structures
Lists vs. Tuples
When comparing lists and tuples, it is essential to recognize their distinct characteristics. Lists are mutable, meaning they can be changed after creation. This allows for flexibility but may impact performance due to the overhead involved in managing the memory. On the other hand, tuples are immutable. Once created, their values cannot be altered. This immutability makes tuples slightly faster than lists, as Python can optimize their usage in memory. Therefore, using tuples over lists where possible can lead to better performance, especially in cases where the data does not need changing.
Additionally, tuples also consume less memory than lists, which is a crucial factor to consider in large-scale applications. This characteristic can lead to improved processing speed and lower memory consumption, thus enhancing overall performance.
Dictionaries vs. Sets
Dictionaries and sets serve different purposes, and understanding their unique features can guide developers in choosing the right structure for their needs. Dictionaries store key-value pairs, allowing for efficient retrieval based on the key. The main advantage here is that dictionary lookups are very fast, making them suitable for applications where quick data access is necessary.
Sets, conversely, are collections of unique elements. They do not store pairs but rather focus on the presence or absence of items. The time complexity for adding and checking for elements in a set is also very efficient. Using sets when you only need to verify existence can improve performance, especially in scenarios that deal with uniqueness or membership tests.
Excessive Function Calls
Excessive function calls can degrade performance, particularly in cases where recursive functions are involved. Each function call incurs a cost in terms of memory and computation due to the creation of a stack frame. When the number of function calls escalates, the performance can suffer considerably. In performance-critical applications, developers should consider minimizing the depth of calls and restructuring their code to avoid deep recursion or high-frequency calls to the same functions.
Global Variables and Scope Issues
Global variables can lead to performance issues, particularly when used inappropriately. Each time a global variable is accessed, Python must check if it’s defined in the global scope, which adds overhead. This can slow down performance, especially in scenarios that involve iteration or frequent access. Avoiding global variables whenever possible, and instead considering local variables or passing parameters to functions, can enhance performance and maintainability of the code. Understanding the scope and lifetime of variables plays a vital role in optimizing Python performance.
Optimizing Python Code
Optimizing Python code is crucial for improving the overall performance and efficiency of applications. In today's fast-paced digital environment, users expect quick responses and functionality without delays. Consequently, developers must focus on enhancing their code to meet these expectations while maintaining readability and functionality.
One of the primary benefits of optimizing Python code is the reduction of runtime. Well-optimized code runs faster, which can significantly improve user experience. Additionally, efficient code often leads to decreased resource consumption, which is particularly beneficial for applications running on limited hardware. It is not just about speed; optimizing code can also enhance maintainability by encouraging cleaner and more organized code structures.
When optimizing code, several considerations must be taken into account. Balancing performance improvements with code readability is often challenging. Over-optimizing for speed can result in code that is difficult to understand and maintain. Therefore, a careful approach is necessary to enhance performance without compromising the quality and clarity of the code.
Algorithm Optimization Techniques
Algorithm optimization plays a vital role in enhancing Python applications. Choosing the right algorithm can drastically affect performance. Whether it is searching, sorting, or data processing, the algorithm's complexity and efficiency can determine how quickly and effectively tasks are completed.
Common strategies include:
- Assessing Algorithm Complexity: Understanding computational complexity through Big O notation helps gauge the performance potential of an algorithm.
- Choosing Appropriate Data Structures: Utilizing data structures that facilitate quicker access and manipulation can enhance performance significantly.
- Dividing Tasks: Breaking down complex tasks into simpler, manageable components can lead to better performance through parallel execution or improved efficiency.
Using Built-In Functions and Libraries
Python's extensive standard library comes packed with built-in functions that are optimized for performance. Utilizing these built-in functionalities, such as , , and , can save time and improve code efficiency. Instead of manually implementing algorithms, relying on these functions can lead to cleaner code.
Additionally, libraries such as NumPy and pandas are designed for high-performance data manipulation. They are implemented in efficient, low-level languages like C, providing speed advantages over pure Python implementations. Thus, adopting these resources can significantly boost performance without extensive manual optimization.
Code Refactoring for Performance
Code refactoring is a systematic process of improving code without altering its external behavior. This can enhance performance markedly.
Understanding Complexity
Understanding complexity is essential to effective code refactoring. It emphasizes how different segments of the code influence performance. By analyzing where most of the time is spent, developers can target areas for optimization.
The key characteristic of understanding complexity lies in its ability to highlight “bottlenecks” - parts where the code can slow down the overall execution. It is a beneficial choice for this article because it grounds optimization efforts in quantifiable metrics, making it easier to justify changes.
Unique features of complexity understanding, like identifying best and worst-case scenarios, help developers decide which parts of their code need immediate attention. The advantages include heightened performance management and targeted optimizations, while the disadvantages might include the potential for oversight on less critical code sections, leading to imbalanced improvements.
Reducing Redundancy
Reducing redundancy is another vital aspect of optimizing Python code. It involves eliminating duplicate code, which can often lead to unnecessary slowdowns in execution. By modularizing code or using functions to encapsulate repeated logic, developers can streamline their applications.
A key characteristic of reducing redundancy is its focus on maintaining DRY (Don't Repeat Yourself) principles, which streamline code management. It is a beneficial method in this article, as it not only improves performance but also enhances the code's readability and maintainability.
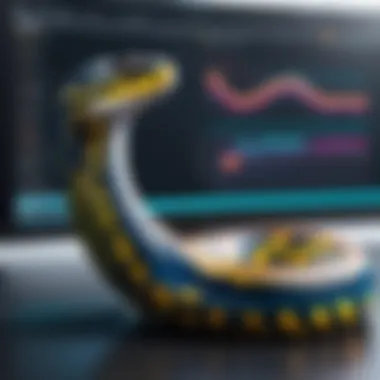
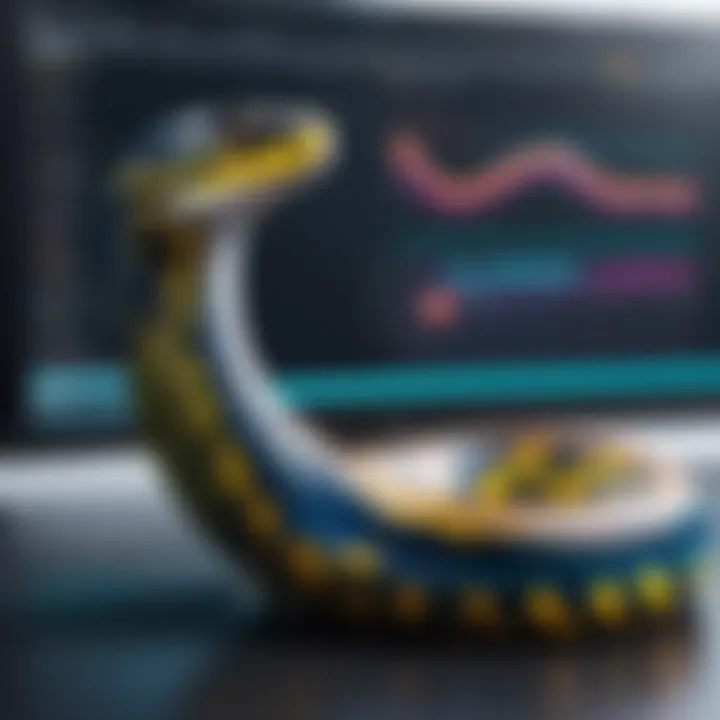
The unique feature of reducing redundancy is its direct impact on memory usage, as fewer lines of code mean reduced memory consumption. The advantages include enhanced performance and easier troubleshooting, while the disadvantages can relate to the initial time investment required to refactor existing code bases.
Concurrency and Parallelism in Python
Concurrency and parallelism are critical concepts in Python's performance landscape. They enable developers to optimize resource utilization, decrease execution time, and handle multiple tasks simultaneously. By understanding these concepts, programmers can make informed decisions on how to structure their code for maximum efficiency. In a world where applications require real-time processing and responsiveness, grasping concurrency and parallelism is essential for any Python developer aiming to enhance their applications.
Understanding Threads and Processes
In Python, threads and processes allow for concurrent execution of tasks. A thread is the smallest unit of processing that can be scheduled by an operating system. It runs within the context of a process and shares memory with other threads in the same process. Processes, on the other hand, are independent. Each process has its own memory space, and they do not share memory directly. The choice between using threads or processes depends largely on the task type.
Threads are often preferred for tasks that are I/O-bound, such as web requests or database access. Their lightweight nature allows them to manage context switches without incurring significant overhead. However, threads may be hindered by the Global Interpreter Lock (GIL), which restricts the execution of multiple threads in CPython.
Processes are more suitable for CPU-bound tasks. Because they don’t share memory, they bypass the limitations imposed by the GIL, allowing for true parallelism. Yet, this comes with increased overhead from inter-process communication. Choosing between threads and processes involves weighing these factors and understanding which fits the workload.
The Global Interpreter Lock (GIL) Explained
The Global Interpreter Lock is a mutex that protects access to Python objects, preventing multiple native threads from executing Python bytecodes simultaneously. This means that even in multi-threaded applications, only one thread can execute Python code at a time, which can become a bottleneck for CPU-bound operations. However, the GIL does not affect I/O-bound applications significantly, as the GIL is released during I/O operations.
The presence of the GIL has led to discussions in the Python community about its implications on multi-threaded performance. Some argue it limits the effectiveness of concurrent programming in Python, while others advocate for better practices and patterns to mitigate its impact. Understanding the GIL is crucial for making the right concurrent programming decisions in Python.
Strategies to Achieve Concurrency
To leverage concurrency in Python, developers can employ several strategies, each serving varying needs and use cases. Two prominent techniques are AsyncIO and using multiprocessing.
AsyncIO
AsyncIO is a library that provides asynchronous I/O capabilities. Its fundamental aspect lies in the ability to manage multiple operations without blocking the main program flow. With this model, functions can yield control while waiting for I/O operations to complete, making it efficient for handling large volumes of such tasks. This is particularly useful in applications like web servers, where handling numerous client connections is essential.
A key characteristic of AsyncIO is its event loop, which manages the execution of asynchronous tasks. This allows developers to write code that appears synchronous while still managing many concurrent tasks.
While AsyncIO offers excellent benefits in terms of performance, it requires a different mindset. The code may become harder to understand for those accustomed to traditional synchronous programming. Still, its advantages in scalability and responsiveness make AsyncIO a popular choice among Python developers.
Using Multiprocessing
Multiprocessing is another strategy that allows for parallel execution. By spawning separate processes, developers can bypass the GIL, achieving better performance for CPU-bound tasks. This approach is particularly advantageous when the computational workload is heavy and can be broken into smaller independent tasks.
A major advantage of using multiprocessing is its ability to fully utilize CPU cores. Each process runs in its own memory space, resulting in isolation that enhances robustness. However, this also leads to increased overhead due to the need for inter-process communication.
In summary, whether using AsyncIO for I/O operations or multiprocessing for CPU-bound tasks, understanding concurrency and parallelism in Python opens up opportunities for building more efficient applications. Every approach carries its pros and cons, and the choice should align with the specific performance goals of the project.
Real-World Considerations
When developing software, the practical aspects of performance cannot be ignored. Real-world considerations in Python performance encompass how code is structured, how it runs in different environments, and how it interacts with users and systems. These facets highlight the need for developers to think beyond theoretical performance metrics and focus on tangible outcomes.
Balancing Performance with Readability
A crucial aspect of writing Python code is striking a balance between performance and readability. Readability is essential for maintainability and collaboration. Python is known for its clear and concise syntax, which can be compromised in pursuit of performance. However, it is crucial to maintain an intuitive codebase. The following points illustrate this balance:
- Maintainability: Code that is easy to read is easier to maintain. Teams who work on projects benefit from clear code that explains its purpose.
- Collaboration: In environments where many developers contribute, comprehensible code reduces misunderstandings and mistakes.
- Performance Gains: Often, the pursuit of performance can lead to obfuscation. Improving performance should not come at the cost of clarity.
Effective performance optimization should consider readability as a priority. A common approach involves using descriptive variable names and breaking complex code into smaller, readable functions.
The Cost of Optimization
Optimization often incurs costs in various forms, which can be overlooked if one focuses solely on performance gains. Here are key points to consider:
- Time Investment: Implementing optimizations requires time for analysis, testing, and adjustments. This can slow initial project timelines.
- Potential Bugs: Enhancements can introduce new errors into the code. Increased complexity may lead to unintended consequences.
- Resource Consumption: In some cases, optimizations may consume more memory or processing power. The balance must be viable for target environments.
Before diving into optimizations, assessing whether performance needs improvement is essential. High performance is often desired but should be necessary based on project demands.
When to Optimize
Not every piece of code requires immediate optimization. Knowing when to focus on performance is crucial. The following guidelines can help:
- Identify Bottlenecks: Use profiling tools to detect areas of your application that slow down overall performance. Address these specific issues first.
- User Impact: If performance issues affect user experience, prioritizing optimization makes sense. Those impacts can lead to dissatisfaction and lower retention.
- Scaling Needs: As applications grow, the operational context changes. Anticipating future growth ensures that performance not only meets current needs but also scales appropriately.
In summary, real-world considerations for Python performance inform decisions regarding code optimization. A clear balance between readability and performance, understanding the costs involved, and knowing when to optimize can lead to more effective and sustainable development practices.
Future of Python Performance
In the landscape of programming languages, performance remains a critical focus area. Python, known for its readability and versatility, is continually evolving. This section examines important considerations that will shape the future of Python performance. The ongoing development in the language's infrastructure and ecosystem can significantly impact efficiency and user experience.
Trends in Python Development
The trends in Python development are influenced by several factors, including community input, new technologies, and shifting industry demands. The rise of data science has led to an increased focus on performance enhancements. Libraries like NumPy and Pandas prioritize speed and efficiency, but their potential depends significantly on the underlying Python performance. Additionally, the advent of Python 3 saw a number of optimizations that improved execution speed compared to its predecessor.
Another trend involves the increasing popularity of microservices. This architectural style demands efficient communication among services, often implemented using Python-based frameworks. The need for performance in these microservices can drive further optimizations in Python. Innovations like just-in-time compilation, seen in implementations such as PyPy, are increasingly adopted. As these trends continue, developers can expect improvements in execution times and usability.
Emerging Technologies Impacting Performance
Several emerging technologies present opportunities to boost Python's performance. Edge computing is gaining traction due to its efficiency in processing data closer to users. Python is being adapted for edge devices, allowing for faster responses and reduced latency.
Machine learning and artificial intelligence are also pushing for performance advancements. The algorithms can be computationally intensive and demand quick execution. Python libraries, such as TensorFlow and PyTorch, are optimizing their backends to leverage hardware acceleration, including Graphics Processing Units (GPUs) and Tensor Processing Units (TPUs). This shift allows Python developers to harness advanced capabilities for performance gains.
Advancements in cloud technologies stand to influence Python performance as well. The ability to deploy Python applications on scalable cloud infrastructures allows for dynamic resource allocation. Developers can exploit cloud services that enhance processing power on-demand, improving overall application responsiveness.
The future of Python performance will hinge upon both community-driven improvements and the advent of new technologies.
In summary, the trajectory of Python performance is set to be influenced by various trends and technologies. From the push for quick execution in microservices to optimizations for machine learning, the language is well-positioned to adapt and thrive in a fast-paced tech environment. Understanding these elements will be crucial for developers aiming to leverage Python effectively in their applications.