Understanding Python's Switch Case: A Comprehensive Guide
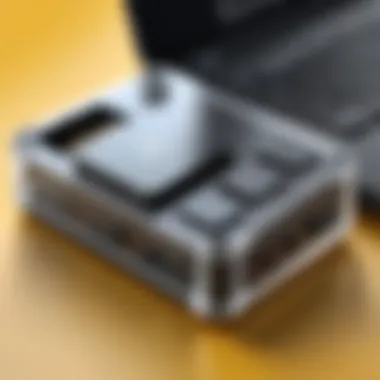
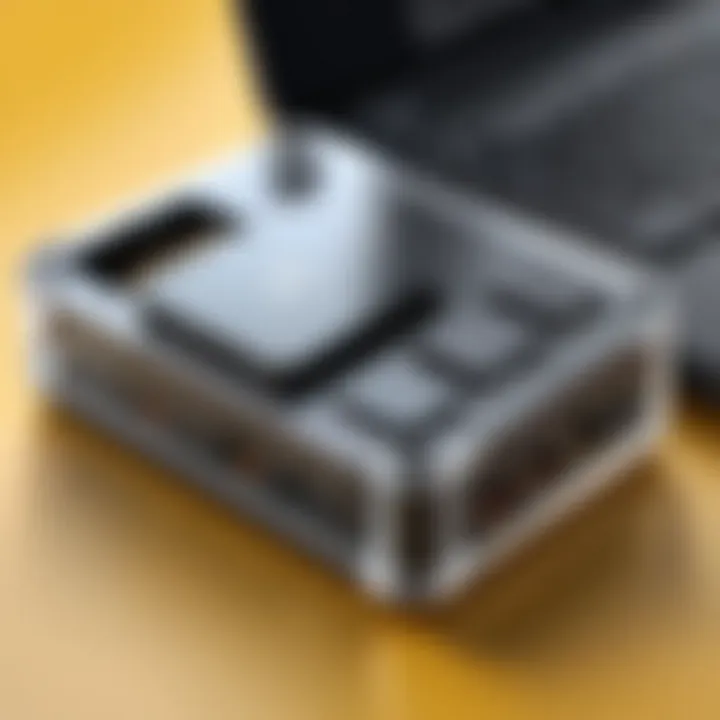
Overview of Topic
The switch case statement is a fundamental concept widely recognized in various programming languages. It offers a streamlined approach to handle multiple conditional branches more efficiently than a series of if-else statements. In Python, however, this structure is not natively available. This article examines Python's unique approach to similar problems, providing alternatives such as dictionaries and if-statements that mimic the behavior of switch cases.
The relevance of this topic lies in its utility. As software development evolves, programmers seek ways to write clearer and more maintainable code. Understanding how to implement conditional logic effectively can enhance code readability and performance. Moreover, the shift towards more functional programming styles also makes mastering these concepts crucial in today’s tech landscape.
Historically, programming languages have evolved different constructs for decision-making. C, Java, and JavaScript implement switch cases, fostering ease and quick decision-making in various applications. In contrast, Python emphasizes readability and simplicity, leading to alternative approaches that may not rely on traditional switch-case syntax.
Fundamentals Explained
To comprehend how switch cases function in other languages, it’s important to understand some core principles.
Key Terminology:
- Conditional Statements: These are structures that perform different actions based on whether a given condition is true or false.
- Dictionaries in Python: Python’s built-in data structure that stores key-value pairs and can be utilized for simulating switch statements.
- Function Objects: In Python, functions can be treated as first-class citizens allowing quick referencing when used with dictionaries.
The basic concept behind switch cases is straightforward: it evaluates an expression and executes corresponding code blocks based on the result. In simple terms, it tests a variable against a static list of values to determine the correct action to take. This is where Python’s use of dictionaries comes into play, combining keys with callable functions to achieve similar outcomes.
Practical Applications and Examples
Switch case alternatives present valuable tools for developers. Utilizing dictionaries, one can create a simple yet effective mapping toward selected functions. Here’s a basic example employing a dictionary:
In this snippet, the dictionary serves to relate user choices to specific functions. If the user inputs an invalid choice, the program returns an error message instead.
Real-world applications may include menu selections in an application, routing HTTP requests based on parameters, or handling different events in a GUI.
Advanced Topics and Latest Trends
Recent developments illustrate a growing interest in simplifying code bases and reducing complexity. Python’s evolving capabilities contribute to a more functional approach to programming. Libraries like introduced in Python 3.10 demonstrate advanced pattern matching capabilities. This feature, while not strictly a switch case, offers similar benefits for controlling the flow of code in a concise manner.
The integration of functional programming techniques into Python encourages developers to explore lambda functions and higher-order functions for even more streamlined logic management. Keeping an eye on these developments can provide important insights into upcoming trends in programming styles and best practices.
Tips and Resources for Further Learning
To deepen your understanding of switch case alternatives and Python programming techniques, consider the following resources:
- Books:
- Online Courses:
- Tools:
- Fluent Python by Luciano Ramalho
- Effective Python by Brett Slatkin
- Coursera Python Programming
- EdX Python for Everybody
- PyCharm for better code management
- Jupyter Notebook for interactive coding practice
These resources provide a solid foundation for grasping advanced programming topics. Engaging with material that challenges your thinking can significantly enhance your programming skill set.
"Understanding the principles of code structure is essential for every programmer aiming to deliver high-quality software."
In summary, mastering the equivalents of switch case statements in Python is vital for writing clean and efficient code. This article provided an exploration into their applications, implications, and encouraged further learning in this ongoing discussion.
Prologue to Switch Case Concept
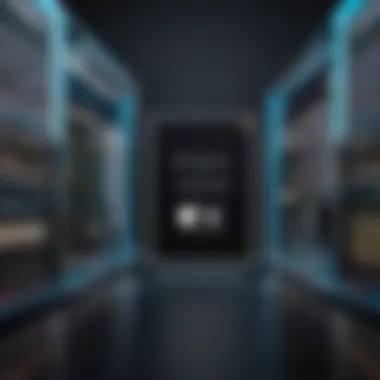
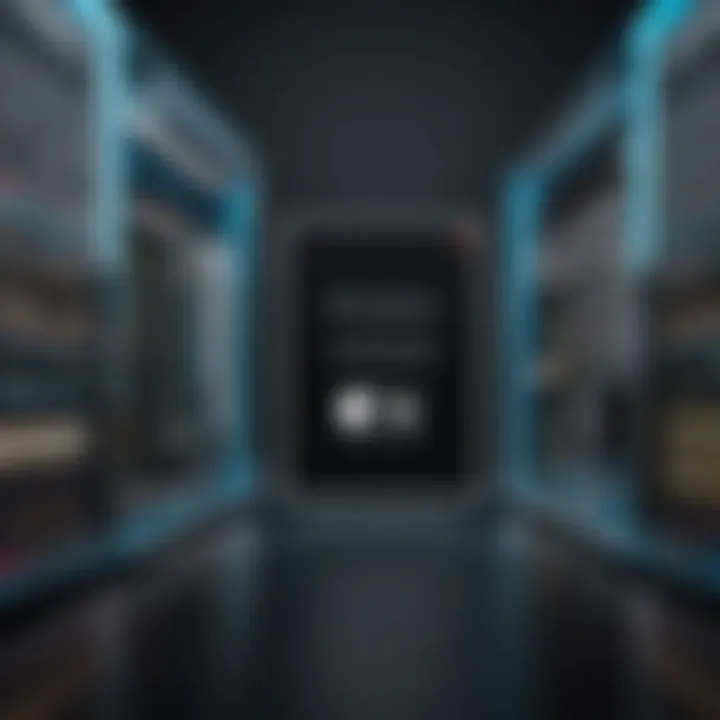
In programming, control flow remains a vital aspect, directing how a program executes its code based on conditions. The switch case concept is an essential part of this, aimed at simplifying complex conditional logic. While Python lacks this construct natively, understanding its importance unravels better coding practices. This section sheds light on the purpose of control flow, paving the way to alternatives available for Python programmers seeking clarity and efficiency in conditional statements.
The Purpose of Control Flow
Control flow is essentially the means by which a program determines the path it takes during execution. It comprises structures that allow a program to make decisions, repeat operations, and manage tasks intelligently. Control flow can make code more readable and maintainable when utilized appropriately. The switch case, in languages that support it, acts as a centralized decision point, streamlining multiple branches of execution by tying them to a single variable. This results in better organization and often enhances performance.
For programmers, especially those learning Python, grasping control flow principles is crucial. Mastering these concepts leads to improved decision-making in code design and the ability to tackle complex scenarios proficiently. Hence, exploring alternatives in Python to the switch case can provide greater flexibility and introduce unique programming styles.
Overview of Conditional Statements
Conditional statements serve as building blocks for control flow. They enable programmers to define conditions and dictate how a program responds when those conditions are met. The primary types of conditional statements in Python include if, elif, and else statements, which evaluate conditions in a sequential manner.
The key characteristics of conditional statements are:
- Mutually Exclusive Pathways: Each condition leads to a distinct block of code, allowing for clear separation of logic.
- Sequential Evaluation: Python assesses conditions in the order they are specified, halting when a condition evaluates to true.
- Familiar Syntax: Their straightforward syntax makes them accessible for beginners, aiding in early understanding of programming logic.
Though versatile, conditional statements can become cumbersome when numerous conditions arise. This highlights the need for alternatives like the switch case, which offers a cleaner approach in other languages.
By gaining insight into the switch case concept and its relation to control flow, developers can better appreciate Python's conditional nature and adapt their coding practices for efficiency and clarity.
Understanding Switch Cases in Programming
The concept of switch cases plays a significant role in programming, specifically in managing complex conditional logic. In essence, switch cases can streamline decision-making processes and improve code readability. This portion of the article will discuss the fundamentals of switch cases, their definition, and how they compare to traditional if-else statements.
In many programming languages, switch cases offer an elegant way to control program flow. They provide a structured way to execute different blocks of code based on the value of a variable. This structure makes it clearer which conditions lead to which outcomes, thus minimizing confusion and potential errors in large codebases.
Definition of Switch Case
Switch cases are a control structure primarily used to simplify multi-way branching in code. They allow a variable to be tested for equality against a list of values, known as 'case' labels. When a match is found, the associated block of code is executed.
Here’s a simple example in Python-like pseudocode:
While direct switch-case structures are absent in Python, understanding the concept lays groundwork for employing alternatives like dictionaries and if-else statements.
Comparison with If-Else Statements
The if-else statement is a core concept in programming, enabling execution of code based on conditional checks. While both if-else and switch case constructs serve similar purposes, they differ in terms of syntax and flexibility.
- Readability: Switch cases can be more readable when dealing with numerous discrete values. The structure presents a clear overview of all conditions at once.
- Execution: In switch cases, once a matching case is found, execution can continue unless interrupted by a break statement, which differs from if-else where each condition is evaluated sequentially.
- Type restrictions: Switch cases generally work with discrete values of specific types, while if-else statements can handle a broader range of expressions.
Switch Case Alternatives in Python
The absence of a traditional switch case statement in Python does not diminish the ability to execute conditional logic efficiently. Instead, Python offers several alternatives that can achieve similar functionality. Understanding these alternatives is crucial for programmers aiming to write readable and maintainable code. Each alternative has distinct benefits and considerations, which can impact performance and code clarity.
Using Dictionaries as Switch Case
Dictionaries in Python can serve as effective substitutes for switch cases. They allow a mapping of keys to functions or values, simplifying decision-making processes. For instance, if you need different behaviors based on a user input, a dictionary can directly map these inputs to specific functions without multiple if-else statements.
Here’s an example illustrating this approach:
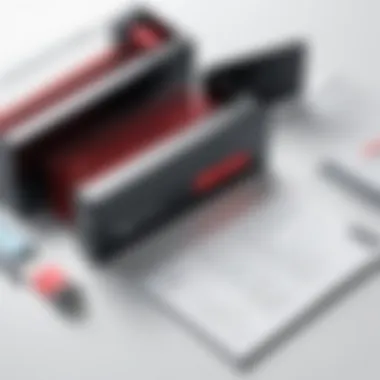
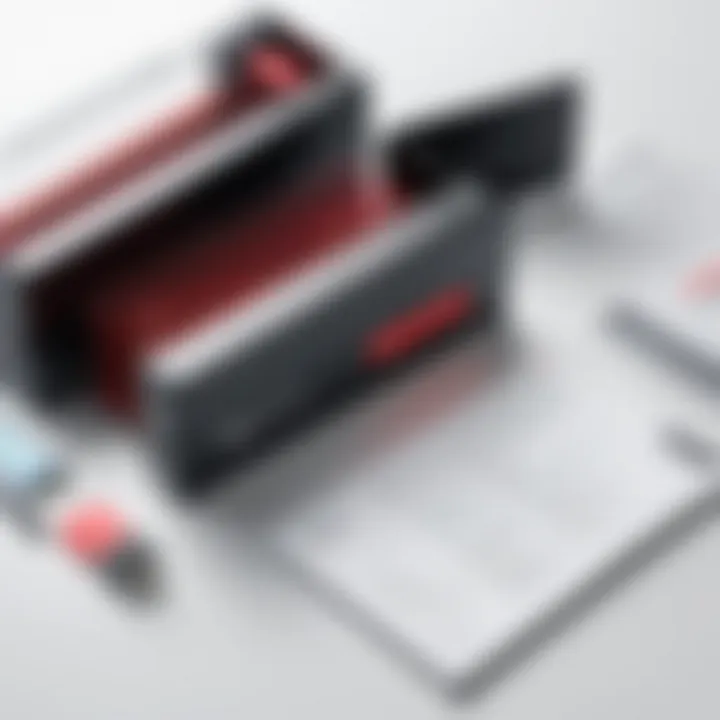
In this code, the operation selected is mapped to corresponding functions through a dictionary. This method enhances code readability by reducing complexity while maintaining efficiency. Additionally, it offers flexibility since new operations can be added easily.
Implementing If-Elif Ladder
The if-elif ladder serves as a straightforward alternative to a switch case. This technique allows for the evaluation of multiple conditions in a linear fashion. While it is the most direct solution, it may become cumbersome with a large number of conditions. The clarity diminishes as the if-elif ladder grows.
Here is a simple example:
Despite being clear in simpler scenarios, this method may lead to cumbersome code when applied to complex situations. As a best practice, use the if-elif ladder when there are only a few possible outcomes.
Employing Functions and Lambdas
Functions and lambda expressions can also effectively mimic switch case behavior. This method entails defining a set of functions for each case and calling the appropriate function based on the input. It maintains clarity and modularity.
For example:
Additionally, lambda functions provide a concise means of implementing simple operations without formally defining a function. This can streamline your code when defining small, one-off functions is needed.
Practical Implementation Examples
The section on practical implementation examples serves a crucial role in understanding how to apply the concepts of switch cases and their alternatives in Python. Through real-world scenarios, programmers can appreciate the effectiveness of various strategies, thereby improving their coding practices. By examining these implementations, one can comprehend their benefits, pitfalls, and appropriate contexts for use. This will enhance the decision-making process when faced with conditional logic tasks in Python programming.
Dictionary-Based Switch Case Example
A dictionary-based switch case is an elegant alternative in Python. Employing a Python dictionary allows mapping keys to functions or values effectively, mimicking the switch case structure found in other programming languages. Here is a simple example:
This example highlights how to create a callable that acts based on the user’s input. The functions as the core of this logic, allowing for cleaner and more organized code. Additionally, it reduces the need for multiple conditional checks, thus improving readability and performance.
If-Elif Ladder Example
Using an if-elif ladder is a conventional method to handle multiple conditions in Python. While it can be less elegant than a switch case, it remains widely understood and easy to implement. Here is an example:
The if-elif ladder provides a straightforward approach, letting developers manage various conditions flexibly. However, the more conditions added, the more cumbersome it can become. This method may be beneficial for simpler scenarios but may require reevaluation for more complex logic.
Dynamic Function Mapping
Dynamic function mapping offers an advanced method for condition handling. Here, functions are dynamically linked at runtime. This is more sophisticated and often cleaner than traditional methods. Consider this approach:
In this example, the function evaluates the input and matches it to the relevant internal functions. This method provides a concise and flexible way of handling complex conditions without cluttering the code with many conditional statements. Thus, programmers can maintain cleaner code bases, enhancing future maintainability and readability.
By understanding these implementations, Python developers can choose the appropriate strategy for their specific scenarios. Each method presents unique advantages and application contexts that can optimize the coding process.
Performance Considerations
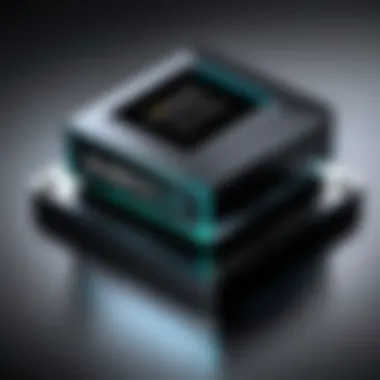
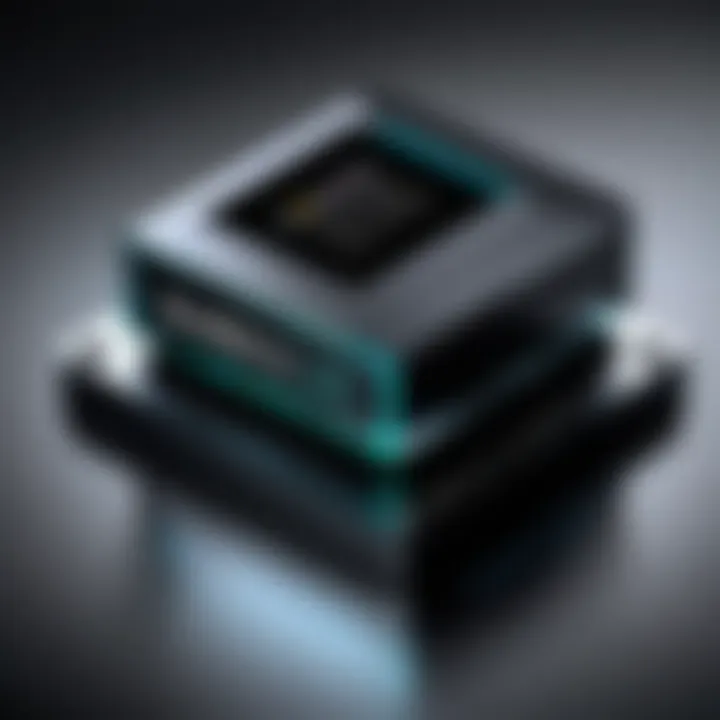
Understanding performance considerations when implementing conditional logic structures, such as switch cases, is crucial for developers. These considerations play an essential role in the efficiency and effectiveness of code. They help in optimizing control flow while maintaining code readability and maintainability. When discussing performance, two primary aspects emerge: comparative speed analysis and memory usage. Each bears significant implications on the overall performance of the application.
Comparative Speed Analysis
Speed is paramount, particularly for applications that require real-time responses. Comparing the speed of various alternatives to switch cases is necessary to gauge their efficiency. Traditional control structures like if-else statements can become cumbersome with multiple conditions. The more conditions there are, the slower the execution may become due to the sequence of evaluations.
On the other hand, when using dictionaries as a substitute for switch cases, the performance can drastically improve. Dictionary lookups in Python operate on average in constant time, making them efficient for multiple cases. This gained speed, however, comes with the consideration of how often each method is used. If an if-elif ladder is implemented in a situation with numerous conditions, the accumulated time complexity could lead to sub-optimal performance.
Some points to consider include:
- Scenarios of Use: Assess how frequently code paths are taken and whether lookup speed outweighs the cost of using more memory.
- Profile Your Code: Use Python's built-in module to identify bottlenecks in your implementation to make informed decisions about performance optimizations.
Memory Usage Insights
Memory utilization is another vital consideration when discussing performance. Different structures consume memory differently. For example, an if-elif ladder is a straightforward implementation that generally uses less memory, as it does not require creating additional mappings or objects to handle the conditions.
In contrast, when employing a dictionary for a switch-case equivalent, although lookup times are faster, it often requires more memory. This occurs because you must allocate space for every potential case, which could lead to increased memory use, especially if many conditions are present. Importantly, the size of the dictionary grows alongside the number of cases.
Key points to consider in memory usage include:
- Overhead of Structural Complexity: Factor in the memory overhead when designing complex conditional logic.
- Garbage Collection: Understand how Python's garbage collection handles memory cleaning for structures that are no longer in use, as it affects overall memory management.
Common Pitfalls and Best Practices
Overusing Symbolic Constants
In the realm of programming, symbolic constants serve as a way to provide meaningful names to values. However, their misuse can lead to confusion. The first pitfall is the tendency to overuse these constants without proper organization. When constants are sprinkled throughout the code without a defined structure, they can make understanding the logic quite difficult.
Many developers define their symbolic constants in a global scope which raises another issue: name collisions. This might arise when two constants have similar names in different modules. Moreover, if the symbolic constants are not consistently used, it can create dissonance within the code. This leads to a situation where a new developer or even the original creator of the code finds it tough to recall what a constant represents.
To mitigate these issues, it is crucial to:
- Group related constants: Bundle constants into classes or modules, making the associations clear.
- Use meaningful names: A well-named constant can significantly reduce the cognitive load needed to understand its use.
- Document constants: Providing comments or documentation about constants aids in long-term maintainability.
Maintaining Readability and Maintainability
Writing code that is not only functional but also readable is essential. Readability enhances the ease with which others can understand the code. It affects maintainability, making it simpler to alter or upgrade without fear of bugs creeping in. The act of switching cases should be managed in a way that anyone who's reading the code can follow the logic without exhaustive re-reading.
One common pitfall is creating overly complex structures. Using a dictionary to mimic a switch case can lead to deeply nested code if not executed properly. This affects readability. Consider the following practices:
- Create clear structures: Keep your conditional logic straightforward. Avoid unnecessary nesting.
- Write clear comments: Provide context where necessary; this aids other developers in understanding the complexities if your logic requires non-obvious reasoning.
- Limit scope of changes: High cohesion within functions and classes aids maintainability by isolating changes to specific parts of the code.
"Code is read much more often than it is written."
Through continuous evaluation of code readability, developers can help ensure that their creations remain sustainable in the long run. Thus, focusing on these best practices is vital for all developers aiming to improve their coding skillset.
Concluding Thoughts
At the end of any analytical piece, reflecting on conclusions is vital. In this article, the discussion around Python's switch-case concept provided significant insights into conditional logic. While Python does not have a built-in switch-case construct like some other programming languages, we explored alternatives that can achieve similar results. This realization helps developers understand that flexibility exists in coding practices regardless of the limitations imposed by a specific language.
Recap of Key Points
To summarize the core ideas discussed:
- Definition and Purpose: The switch-case concept is fundamentally about managing control flow in programming. Its structure simplifies complex branching logic into clearer cases.
- Alternatives in Python: We explored using dictionaries for mapping values to function calls and the if-elif ladder for condition handling. These constructs not only replace switch-case functionality but can also enhance code clarity in certain scenarios.
- Practical Examples: Implementing these alternatives showed how developers can replicate switch-case behavior, enabling a more organized code structure. By using dictionary-based examples and dynamic function mapping, we observed effective solutions in action.
- Performance Concerns: A comparative analysis revealed that while alternatives are functional, they may have performance implications depending on their implementation. Developers should weigh these considerations when opting for one method over another.
- Common Pitfalls: A critical reflection on overusing symbolic constants indicated the importance of keeping code maintainable and readable. Such practices can lead to confusion over time, impacting a project's longevity.
Future of Control Flow in Python
Looking ahead, the evolution of programming languages continuously shapes how developers approach control flow. Although Python's community engages actively in discussions about introducing native switch-case statements, alternative practices have emerged as practical solutions.
The possibilities that arise from utilizing dictionaries and function mapping open up a broader landscape for developers. As Python continues to gain traction in fields like web development and data analysis, understanding control flow strategies remains critical for efficient coding. This knowledge not only aids in writing elegant code but also paves the way for innovation in programming methodologies.