Understanding Variables in Coding: An In-Depth Exploration
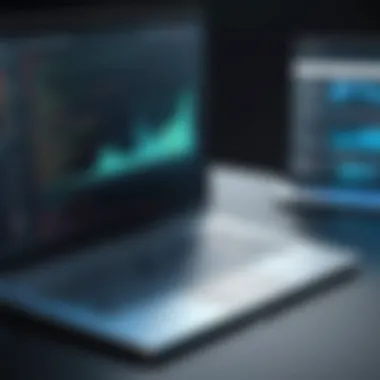
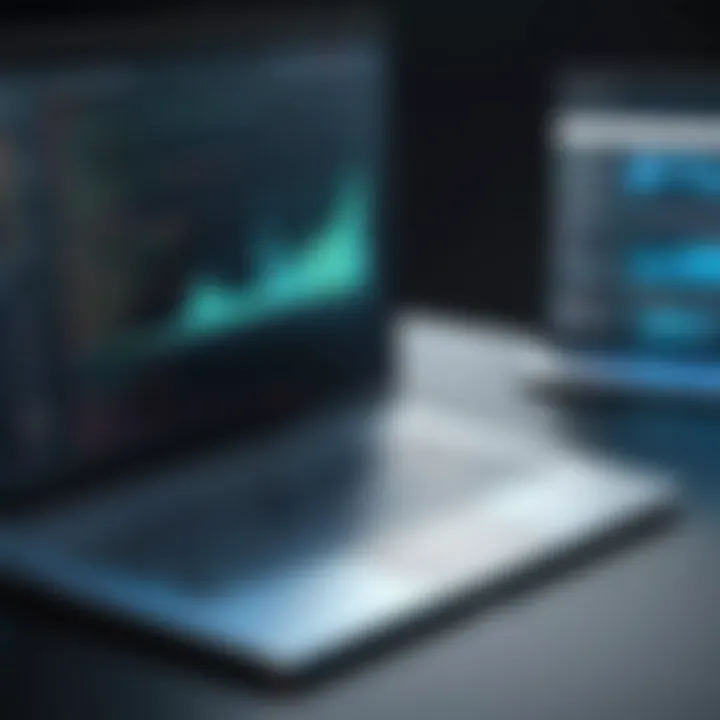
Overview of Topic
Prologue to the Main Concept Covered
Variables are fundamental components in programming that store data values. They act as symbolic names for data, allowing developers to manipulate and reference information efficiently within calculations or algorithms. Understanding variables is crucial for anyone venturing into the realm of coding.
Scope and Significance in the Tech Industry
In the tech industry, variables are the building blocks for any application. They are not only used for storing data but also for controlling program flow and managing state in software. Their correct usage impacts performance, readability, and maintainability which are critical for professional development in software projects.
Brief History and Evolution
The concept of variables dates back to the early days of computer programming. Initially, variables were simple and had limited functionality. Over time, programming languages evolved, introducing new types of variables, such as integers, floats, and strings. Each advancement aimed to provide more flexibility and power to developers, shaping modern programming practices.
Fundamentals Explained
Core Principles and Theories Related to the Topic
Every variable has a data type, which determines the kind of data it can hold. Common types include integers, floating-point numbers, strings, and booleans. Understanding these data types is critical as they influence memory allocation and performance.
Key Terminology and Definitions
- Variable: A storage location identified by a name that can hold a value.
- Data Type: The classification of data that tells the compiler or interpreter how the programmer intends to use the data.
- Scope: The context in which a variable is accessible within a program.
Basic Concepts and Foundational Knowledge
Variables can be declared in various ways across programming languages. For instance, in Python, a simple variable declaration looks like this:
This statement assigns the integer value 10 to . Understanding variable declaration is the first step to mastering their use.
Practical Applications and Examples
Real-world Case Studies and Applications
Variables are utilized in numerous applications, from web development using JavaScript to scientific computing with Python. For example, in a web application, user input data is often stored in variables, which developers can then use to interact with a database or generate dynamic content.
Demonstrations and Hands-on Projects
Consider a simple project: a calculator. Each operation can be executed by storing user inputs in variables.
Code Snippets and Implementation Guidelines
Hereโs an example of a basic addition operation in JavaScript:
This script declares two variables and , adds them, and displays the result.
Advanced Topics and Latest Trends
Cutting-edge Developments in the Field
As programming languages develop, so do the features associated with variables. For instance, newer languages introduce concepts like immutability, where variables cannot be altered once set, promoting safer code practices.
Advanced Techniques and Methodologies
Understanding closures and scope is essential for advanced variable management. These concepts help prevent variable conflicts and enhance modular programming practices.
Future Prospects and Upcoming Trends
With the rise of functional programming paradigms, many languages are changing how variables are used. JavaScript, for example, encourages the use of and to redefine variable scopes, aligning with modern coding standards.
Tips and Resources for Further Learning
Recommended Books, Courses, and Online Resources
- "Clean Code" by Robert C. Martin: Offers insights into developing maintainable code.
- Codecademy: Interactive courses on various programming languages for beginners.
- freeCodeCamp.org: A nonprofit organization providing free coding tutorials and projects.
Tools and Software for Practical Usage
- Visual Studio Code: A source code editor that supports many programming languages and is customizable through extensions.
- GitHub: A platform for version control and collaboration in coding projects, allowing developers to work on code together more effectively.
Effective variable management leads to enhanced code efficiency and maintainability, which are crucial for developing robust applications.
Foreword to Variables
In the landscape of programming, the concept of variables stands as a cornerstone. Variables serve as symbolic representations of data, allowing us to store, manipulate, and retrieve information efficiently. Understanding variables is crucial for anyone delving into programming, from students to seasoned IT professionals. This section aims to clarify the fundamental notions surrounding variables, emphasizing their significance in various coding paradigms.
Definition of Variables
A variable is essentially a named storage location in a program. It holds values that can change during the execution of the program. Each variable has a name, a data type, and a value. The name acts as an identifier, allowing programmers to reference the stored data. The data type defines the kind of data the variable can hold, which could be an integer, a string, a float, among others. For instance, in Python, you might declare a variable like this:
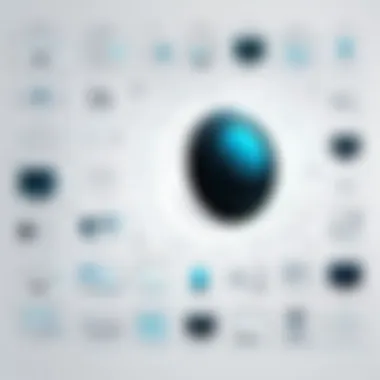
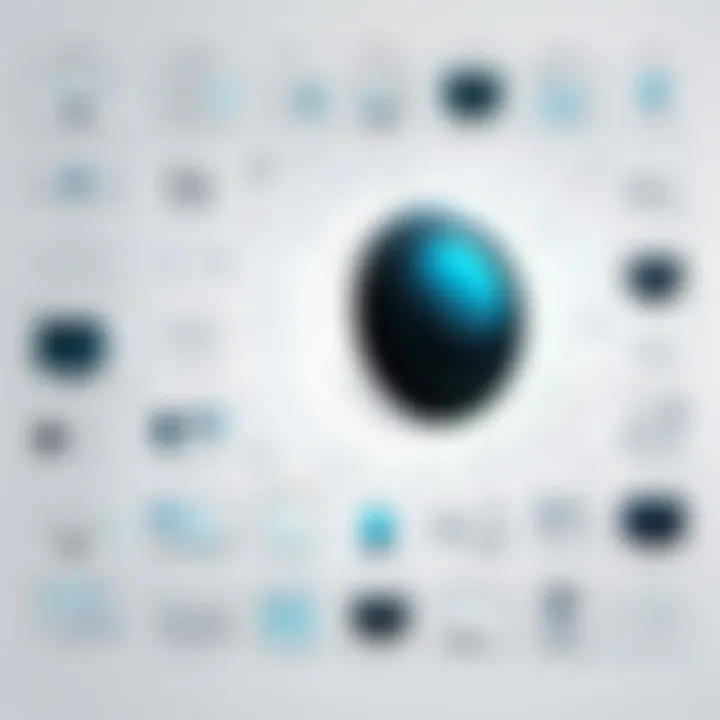
In this example, is a variable of type integer with a value of 30, and is a string variable with a value of "John". Variables provide flexibility in coding, enabling programmers to write dynamic and responsive applications.
The Role of Variables in Coding
The role of variables transcends mere storage. They facilitate the dynamic nature of programming by allowing developers to create flexible algorithms. For example:
- Variables enable data manipulation and calculations. By adjusting variable values, a program can produce different outputs.
- They enhance code readability. A well-named variable conveys its purpose clearly, aiding in understanding the code.
- Variables are essential in controlling the flow of a program. They can be used in conditions and loops to execute different code paths based on their values.
In summary, variables form an integral part of coding. They act as the building blocks of more complex data structures and algorithms. Understanding how to effectively use variables can lead to more efficient and maintainable code.
The proficient use of variables not only promotes better coding practices but also significantly improves program performance and readability.
Types of Variables
Understanding the types of variables is fundamental to coding as it shapes how data is stored, manipulated, and accessed in programs. Variables provide a framework for managing information and influence memory usage. Recognizing the types helps in implementing efficient coding strategies that enhance performance and maintainability. Each type of variable has unique properties that cater to different requirements of programming tasks.
Primitive Variables
Primitive variables are the building blocks of data in programming. They typically represent a single value and are allocated a fixed amount of memory, which makes them easy to manipulate and access. The most common primitive data types include integers, floats, booleans, and characters. Each of these types has specific characteristics that suit distinct operations. For instance, an integer can only hold whole numbers, while a float can accommodate decimal values.
Key considerations for using primitive variables include:
- Performance: They are generally faster to access since they require less memory overhead.
- Simplicity: They are straightforward to understand and implement, especially for simple calculations.
- Type Safety: Most languages enforce strict type rules on primitive types, reducing the chance of errors.
Composite Variables
Composite variables are more complex structures that can encapsulate multiple values or primitive variables. Examples include arrays, lists, and objects. Each composite type allows for organizing data in a more meaningful manner according to specific needs. They can hold various data types and relate them contextually. For example, an array can store a list of integers, while an object might store a person's attributes like name, age, and address.
Using composite variables offers several benefits:
- Data Organization: They enable better organization of related data.
- Ease of Manipulation: Functions can process entire structures instead of individual data points.
- Flexibility: They can grow dynamically, especially in languages that support array resizing.
Dynamic Variables
Dynamic variables are characterized by their ability to change in size and type during program execution. They are frequently used in situations where the amount of data is not known beforehand or when data needs to be modified frequently. Languages such as Python and JavaScript provide robust support for dynamic variable types, allowing flexibility that primitive and composite types do not offer.
With dynamic variables, programmers can leverage:
- Adaptability: The ability to grow or shrink Array or Object as needed.
- Simplified Memory Management: Many languages handle memory allocation automatically, reducing manual memory management requirements.
In many modern programming environments, the choice of variable type can significantly impact application performance and development efficiency.
Variable Naming Conventions
Variable naming conventions are essential in coding because they establish a clear method for identifying variables across the codebase. Well-defined conventions increase readability and maintainability. They help developers understand the purpose of a variable without delving into its implementation. An organized naming system can significantly reduce errors and foster teamwork, as it promotes a shared understanding of the code.
Importance of Naming Conventions
Naming conventions serve multiple purposes. First, they enhance the legibility of code, making it easier for any developer to read and understand. Clear variable names reduce time spent trying to decipher cryptic terms.
Secondly, they assist in code maintenance. When projects evolve and maintainers change, a logical naming scheme allows future developers to grasp functionality quickly. Consistency is also key; by adhering to a standard, developers can avoid confusion that arises from varying styles within the same project.
In summary, adopting naming conventions is not just a matter of preference but rather a critical aspect of effective programming. They facilitate communication among devs and increase code efficiency, which directly impacts productivity.
Common Naming Patterns
There are several established naming patterns that programmers frequently use. These patterns generally align with the language being utilized. Popular styles include:
- CamelCase: This convention capitalizes the first letter of each word except the first one. It is commonly used for variables and method names in languages like Java. For example, .
- snake_case: This style separates words with underscores, often preferred in Python. An example is .
- PascalCase: Similar to CamelCase but requires the first letter of the first word also to be capitalized. It is often used for classes in many programming languages, for instance, .
- kebab-case: Some languages, especially JavaScript for URLs, use hyphenation. For example, .
Choosing one of these patterns consistently throughout the project is crucial. It highlights professionalism and aids in seamless collaboration amongst teams.
Avoiding Common Mistakes
While establishing naming conventions, several pitfalls should be avoided:
- Ambiguous Names: Names like or provide little context. Instead, use descriptive names that clarify purpose, such as or .
- Excessive Abbreviations: While shorthand may seem efficient, it can lead to misunderstandings. Using terms like instead of detracts from clarity.
- Inconsistent Naming: Switching styles or formats often confuses both the original developer and others who work on the code later. Consistency across a project prevents potential errors.
Overall, maintaining proper naming conventions is vital for code agility and longevity. It ensures that code is not only functional but also comprehensible for all whom may engage with it later.
Scope of Variables
Understanding the scope of variables is key to mastering programming. Scope determines the visibility and lifetime of a variable within different contexts of a program. This concept is fundamental in various programming languages and directly impacts both code maintainability and efficiency.
When you declare a variable, its scope defines where it can be accessed or modified. This is crucial in large applications, where managing multiple variables across different modules can become complex. If a variable is accessible only where it is needed, the risk of unintentional changes or conflicts decreases. Adopting sound practices regarding variable scope enhances the readability and reliability of code.
Developers encounter two main types of variable scope: local and global. Both have unique characteristics and specific use cases. Let's delve into these subcategories to clarify their differences and implications for coding practices.
Local vs. Global Variables
Local variables are defined within a specific function or block of code. Their visibility is limited to that function or block, meaning they cannot be accessed outside of it. This scope is advantageous because it keeps the variables contained, reducing the risk of interference from other parts of the program. Local variables allow for temporary storage of data that does not need to persist once the execution leaves the scope where it was defined.
Example of Local Variable:
In this snippet, can only be accessed and used within . Attempting to print it outside the function would lead to an error, ensuring that the variable's influence is confined strictly to its intended scope.
Global variables, on the other hand, exist outside any function and are accessible throughout the entire program. While this allows easy access to these variables from various points in the code, it comes with risks. An unintended modification of a global variable can create bugs that are difficult to track down, as they can originate from anywhere in the codebase. Therefore, cautious management of global variables is essential, especially in bigger projects.
Example of Global Variable:
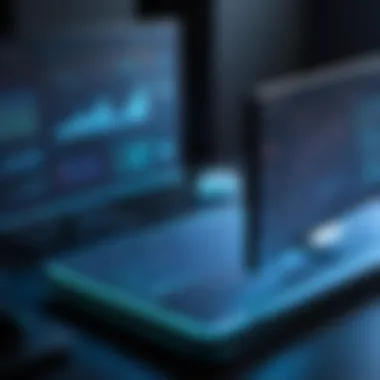
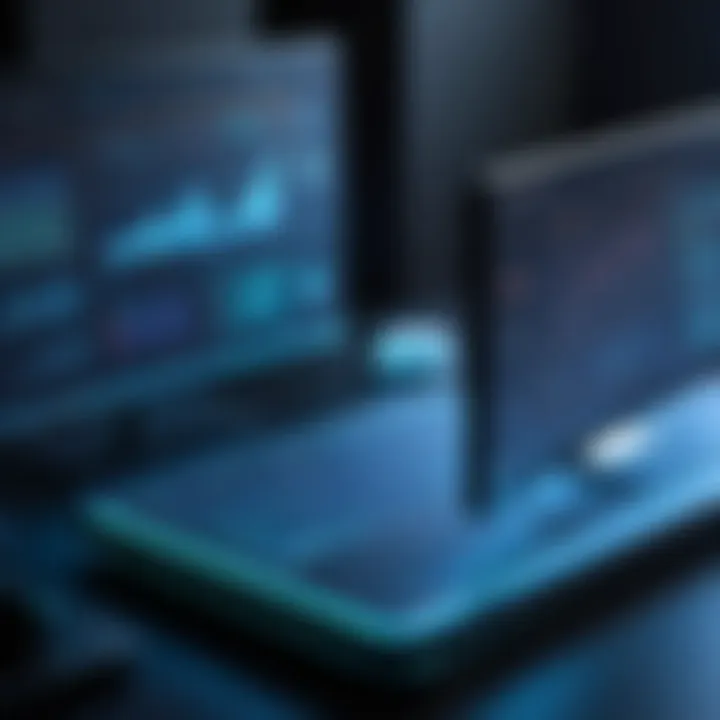
Here, can be accessed from , allowing for data sharing across different functions. However, developers should be aware of the pitfalls and aim to minimize the use of global variables unless necessary.
Static Variables
Static variables hold a special place in variable management. These are somewhat of a hybrid between local and global variables. A static variable retains its value even after the function in which it is declared has finished executing. Therefore, they are useful for maintaining state across multiple calls to a function.
In languages like C or C++, a static variable within a function holds its value between invocations, but it remains inaccessible outside that function. This characteristic is particularly useful in situations where persisting a value is crucial for computation without exposing it to broader scopes.
Using static variables judiciously can optimize memory usage and maintain control over data, preventing it from being modified outside its intended context. However, misuse can lead to confusion regarding the behavior of such variables in the broader scope of an application.
Scope defines the lifeline of a variable. Master it for lower bugs and cleaner code.
Managing variable scope effectively helps to create better-organized code, reducing complexity and improving maintainability.
Data Types and Variable Initialization
Understanding data types and variable initialization is crucial in coding. It helps programmers define the kind of data that a variable will hold, which directly influences memory allocation and operation performance. Selecting the appropriate data type for a variable ensures that the program can operate efficiently, manage resources properly, and avoid common pitfalls such as type errors or unexpected behaviors in code execution.
When initializing variables, the method used can impact overall program reliability and performance. Proper initialization prevents errors like using an uninitialized variable, which can lead to unpredictable results. It also aids in improving code readability, making it easier for developers to follow the logic behind the variable choices. By dedicating time to understand the fundamental aspects of data types and initialization, programmers set a strong foundation for writing robust and efficient code.
Common Data Types
Variables can hold different types of data, which are broadly categorized into several common data types. Each has its unique characteristics and intended uses:
- Integer: Represents whole numbers. Used in counting or indexing tasks.
- Float: Represents decimal numbers. Useful in calculations requiring precision, like financial applications.
- String: Holds sequences of characters. Ideal for textual data processing.
- Boolean: Represents truth values: or . Used in conditional statements and logic.
- Array: A collection of variables that can hold multiple values of the same type. Useful for managing lists.
- Object: Refers to complex data structures that can encapsulate properties and methods. Common in object-oriented programming.
Each data type serves a distinct purpose, making it essential for programmers to understand their usage and implications. Therefore, knowing when to use each type helps in optimizing code performance and functionality.
Initialization Techniques
Proper initialization of variables is essential for good coding practices. Different programming languages offer various techniques for initializing variables. Here are some common approaches:
- Direct Initialization: Assigning a value at the point of declaration is straightforward. For example, in Python:
- Deferred Initialization: This involves declaring a variable without immediately assigning a value. It may be assigned later in the code, as shown below:
score# Declaration only, no initialization yet score = 100# Assigned later
This results in being equal to 15. Such concise expressions help convey intent clearly and maintain readability. Understanding the nuances of various operators is crucial for anyone looking to write improved, efficient code.
Variable Types and Operations
Variable types dictate what kind of operations can be performed on them. Each type has its own rules and behaviors, which is why understanding them is invaluable. The primary variable types include integers, floats, strings, and booleans, among others. Each type interacts differently depending on the operation performed.
For instance:
- Integers can be subjected to arithmetic operations, such as addition and multiplication.
- Strings enable concatenation but cannot be added to integers directly.
- Booleans form the basis for logical operations and conditions, making them vital in control flow.
The type of a variable also influences memory management and performance. Incorrect operations, such as trying to add a string and an integer, lead to errors and bugs. Recognizing these differences leads to better programming practices.
Common Variable Pitfalls
Understanding potential pitfalls associated with variables is critical for efficient coding practices. Even small missteps can lead to bugs that are challenging to diagnose and fix. By recognizing common issues, software developers can write more resilient code and improve overall maintainability. Pitfalls can arise from improper usage or misunderstanding of how variables interact within a program. Addressing these areas leads to better coding habits, making this an essential topic for students, budding programmers, or IT professionals.
Uninitialized Variables
Variables that are declared but not initialized can lead to unexpected behavior in a program. When a variable is uninitialized, it holds a default value that is often not clear or intended. In languages like JavaScript or Python, relying on an uninitialized variable can cause it to default to or , respectively. This can lead to runtime errors or logic issues that can be difficult to trace back to the root cause.
It is important to ensure that all variables are properly initialized before they are used. For instance, in C, failing to initialize an integer variable may lead to undefined behaviors, as it could contain any garbage value from memory. Developers should always assign meaningful values at the point of declaration to avoid confusion later in the code.
Shadowing Variables
Variable shadowing occurs when a variable declared in a certain scope has the same name as a variable in a broader scope. This can lead to confusion regarding which variable is currently in use. For instance, consider the following snippet in JavaScript:
In this case, the inner shadows the outer inside the if block. While shadowing can be useful to avoid unintended changes to outer variables, it can also lead to bugs if not managed carefully. Using clear and distinct variable names can help minimize these issues, ensuring that developers understand the scope and context of their variables.
Data Type Mismatch
Data type mismatch is a common issue that can hinder code functionality. It occurs when operations are performed on incompatible data types. For example, attempting to add a string and a number in JavaScript can lead to unexpected results. Consider:
Here, JavaScript implicitly converts the types, which can lead to logic errors. It's essential to ensure that the data types used in operations match the expected types to prevent such issues. Type checking and validation become invaluable practices, especially in dynamically typed languages. By implementing robust data type checks, developers can catch these mismatches early, resulting in cleaner, more predictable code.
Variable Management Best Practices
Effective variable management is crucial in coding. Good practices not only simplify the coding process but also make maintenance much easier. When developers understand how to manage variables efficiently, they can write cleaner, more reliable code. This ultimately leads to fewer errors and improved performance. Variable management involves organizing, naming, and utilizing variables with care. Implementing these best practices also enhances collaborative efforts within development teams.

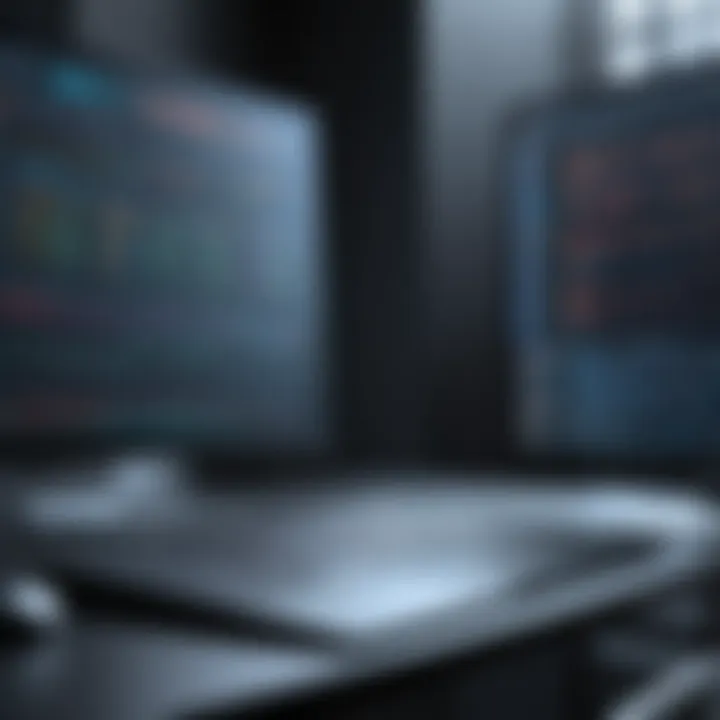
Organizing Variables
Proper organization of variables can greatly enhance the functionality of code. When variables are arranged in a logical manner, it allows for easier access and better understanding. Grouping related variables together can clarify their purpose. Furthermore, categorizing them into sections, such as constants, globals, and locally scoped variables, can reduce confusion. This organization contributes to cleaner architecture in larger projects. For programming languages like Python or JavaScript, following naming conventions helps to categorize effectively. For example:
- Constants could be in all uppercase like .
- Local variables often start with a lowercase letter such as .
Improving Readability
Readability of code is essential for both current developers and future maintainers. Variables should be named descriptively to convey their purpose. Using clear and consistent naming conventions helps prevent misunderstandings. Reducing complexity in how variables are declared and initialized also aids readability. A simple example can demonstrate this:
Instead of using a vague name like , use to clearly indicate what the variable represents. This practice helps everyone understand the code faster, reducing the time needed for onboarding new team members.
"Readable code enhances collaboration, enabling more developers to contribute effectively without extensive background knowledge."
Testing Variable Efficiency
Testing variable efficiency involves ensuring that the use of variables does not negatively impact performance. Monitoring how variables are utilized within applications can reveal inefficiencies. Tools available in many Integrated Development Environments (IDEs) can analyze this aspect, enabling developers to make necessary optimizations.
Some strategies for testing efficiency include:
- Profiling tools that track variable usage in real time.
- Benchmarking comparisons of variable performance in various situations.
- Monitoring memory usage to ensure there are no leaks.
By focusing on these aspects, developers can refine the usage of variables to enhance the overall performance of their applications.
Advanced Variable Concepts
In the realm of programming, understanding advanced variable concepts is crucial for developing efficient code. Variables can influence performance and behavior across various programming environments. Focusing on concepts such as immutability and memory management provides deeper insights into how programs run. Improved knowledge in these areas can lead to greater coding proficiency, allowing developers to make informed decisions when handling data.
Immutable vs. Mutable Variables
The distinction between immutable and mutable variables is fundamental to programming. Immutable variables cannot be altered after their initial assignment. For instance, strings in Python are immutable, meaning any change results in the creation of a new string rather than modifying the existing one. This concept ensures data integrity and creates predictable behavior in applications.
On the other hand, mutable variables can be changed. Lists in Python serve as a clear example; they allow changes to their contents without creating a new object. Both types have advantages and disadvantages.
- Benefits of Immutability:
- Benefits of Mutability:
- Thread Safety: Immutable objects are inherently thread-safe, avoiding concurrent modification errors.
- Easier Debugging: As they cannot change state, tracking issues becomes simpler.
- Flexibility: Mutability allows for dynamic changes, enhancing interactions in user interfaces or real-time applications.
- Performance: In certain cases, mutable structures can be more memory efficient as they do not create additional objects.
Understanding when to use each type is vital for optimizing code.
Variable References and Memory Management
Variable references and memory management deal with how variables point to data in memory. When a variable references another object, it can lead to complexities depending on whether that object is mutable or immutable. This detailing is crucial for understanding performance, especially in high-demand applications.
When managing memory, one must consider how data persist. Garbage collection plays a key role in automatically reclaiming memory no longer in use. It helps avoid memory leaks and enhances application efficiency. However, reliance on garbage collection can also introduce unpredictable pauses in application performance if not optimally configured.
"Understanding memory management will enhance your ability to write cleaner, more efficient code."
Efficient memory management can drastically change how applications perform. Developers must consistently analyze their use of variable references to maintain optimal performance. This analysis includes:
- Evaluating variable scope and lifecycle: understanding how long variables persist in memory.
- Monitoring reference counts in languages that do not have automatic garbage collection.
Combined, these advanced concepts provide a framework for creating more robust programming solutions. By mastering immutability, mutability, and memory management, developers can significantly enhance their coding efficiency and application performance.
Case Studies in Variable Usage
In exploring the nuances of how variables function in programming, case studies offer invaluable insights. They illustrate the practical application of theoretical concepts, shedding light on the realities that developers face in coding environments. Through detailed analysis of real-world examples, one can understand not just the mechanics, but also the implications of variable management on code efficiency and project success.
Real-World Examples
One notable real-world example comes from the development of large-scale web applications, such as those running on platforms like Facebook. Here, variables play a critical role in handling user data dynamically. For instance, JavaScript is heavily employed to manage state through variables in a single-page application. This allows for a responsive user experience, as updates can occur without requiring a full page reload. The choice of variable scopeโbe it global or localโdetermines how memory is managed and influences performance greatly.
Another example lies in data analysis platforms like Kaggle, where Python is often used. Variable data types, particularly pandas data frames, allow developers to manipulate large datasets flexibly. Here, utilizing effective naming conventions for variables enhances readability and maintainability, making collaboration easier across diverse teams. As projects scale, the initial decisions around variable management can significantly impact both speed and clarity.
"Effective variable usage is not just about coding; it significantly affects the entire software development lifecycle."
Lessons from Industry Practices
Lessons learned from industry practices underline the importance of understanding variable usage. Many organizations emphasize coding standards that advocate for clear and descriptive variable names. This practice not only benefits the individuals writing the code but also supports team members who may work on the code in the future. In environments like enterprise software development, this clarity prevents miscommunication and reduces errors.
Moreover, there is a trend toward using immutable variables where applicable. Companies aim for increased predictability in their software, reducing the chances of bugs arising from variable changes in unexpected places. This pattern of immutability is especially critical in languages like Java and Scala, where it aligns well with functional programming paradigms.
Watching how successful companies manage their variable scopes can also provide important takeaways. Creating a balance between local and global variables avoids issues like namespace collisions and inadvertent data overwrites. This careful management resonates through all development efforts, as highlighted in case studies from companies like Microsoft and Google, which demonstrate well-structured variable implementation contributes to their coding efficiency.
Culmination
In this article, we have explored the multifaceted world of variables in coding. Variables serve as the cornerstone of programming, enabling developers to store, manipulate, and manage data efficiently. Understanding the role of variables can greatly impact the effectiveness and maintainability of code.
Recap of Key Points
- Definition and Importance: Variables allow programmers to represent information in a dynamic way. Their ability to hold various data types makes them versatile tools in coding.
- Types of Variables: We examined primitive, composite, and dynamic variables, each serving its purpose in different situations.
- Naming Conventions: Adhering to clear naming conventions enhances code readability and helps prevent confusion during collaboration.
- Scope: Locating variable usage within local or global scopes is essential for managing resource visibility and memory efficiency.
- Manipulation and Management: Being aware of assignment operators and best practices in variable management assists in optimizing code performance.
- Common Pitfalls: Avoiding uninitialized and shadowed variables can prevent unnecessary errors and increase code reliability.
- Future Directions: The evolution of programming languages and paradigms will continue to influence variable usage, emphasizing the need for ongoing learning and adaptation.
Future of Variables in Programming
Looking ahead, the role of variables will remain critical as programming languages evolve. The rise of languages that prioritize safety and immutability, such as Rust, brings new considerations for variable management. Furthermore, the use of artificial intelligence in coding may introduce layers of abstraction that will redefine how we define and interact with variables.
"Variables not only hold data but also reflect the thought processes of the programmer, influencing both the logic and structure of the code."
As programming continues to advance, being conversant with variable strategies will be paramount. Developers will need to leverage their understanding of variables to enhance code efficiency and maintainability in increasingly complex environments.