Understanding XOR in C Programming: A Deep Dive
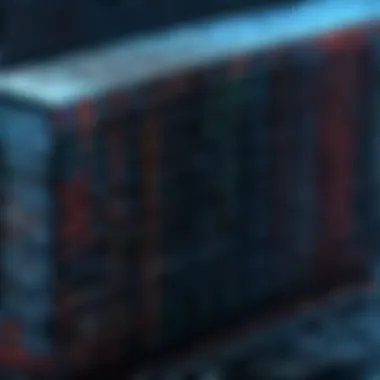
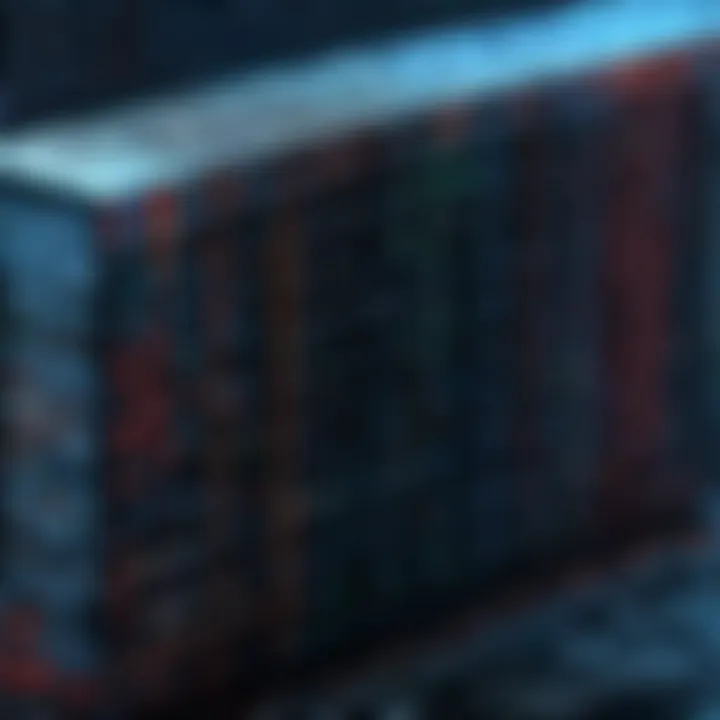
Overview of Topic
Prologue to the main concept covered
XOR, or exclusive OR, is a key operation in digital logic that plays a significant role in programming, particularly in C language. At its core, XOR is a binary operator that takes two inputs and returns true only when exactly one of the inputs is true. It’s not just a fancy term; it’s the backbone for various algorithms, encryption, and even simple tasks like toggling bits.
Scope and significance in the tech industry
The significance of XOR extends beyond mathematical curiosity. In the realm of software development, especially in systems programming, image processing, and cryptography, XOR operation is a fundamental building block. Understanding how it works can elevate a programmer’s skill, equipping them to handle complex problems more efficiently. It's often said that grasping the power of XOR is akin to wielding a secret key in the programming world.
Brief history and evolution
The concept of XOR has origins that trace back to early mathematical logic. It became prominent with the advent of computer science in the mid-20th century. Over time, as programming languages evolved, the definition and applications of XOR expanded, making it an indispensable tool in a programmer’s toolkit. The history of XOR reflects its journey from theoretical mathematics into practical, everyday use in programming.
Fundamentals Explained
Core principles and theories related to the topic
Understanding XOR requires a brief dive into binary logic. The operation works on bits, the most basic unit of data in computing, and follows these rules:
- 0 XOR 0 = 0
- 0 XOR 1 = 1
- 1 XOR 0 = 1
- 1 XOR 1 = 0
This principle of comparing two bits visually portrays how XOR can lead to results that serve diverse programming functions.
Key terminology and definitions
Before diving into more complex aspects, let’s clarify some terms.
- Bit: A binary digit, 0 or 1.
- Binary Operation: An operation that combines two elements to produce another element.
- Bitwise Operation: An operation that directly manipulates bits of binary numbers.
These terms create the framework for understanding how XOR functions and why it's essential in C programming.
Basic concepts and foundational knowledge
It’s not just about crunching numbers. XOR is often regarded as a logical operation, allowing the toggling of bits. For example, if we have two binary variables, using XOR can easily invert bits. Here’s a simple coding analogy:
This manipulation emphasizes just how powerful and versatile the XOR operation is, paving the way for complex functionalities in programming.
Practical Applications and Examples
Real-world case studies and applications
XOR finds real-world applications in various areas, including data encryption and generation of checksums. For instance, in a basic encryption scenario, XOR can be used to obscure information by combining plaintext with a key. This method already proves effective in simple applications.
Demonstrations and hands-on projects
A hands-on project could involve creating a simple encryption algorithm using XOR. Here’s a basic example:
This snippet shows how easily you can mix plaintext with a key to ensure confidentiality. Practical scenarios like this underscore the importance of mastering XOR for programmers.
Code snippets and implementation guidelines
For effective usage, it’s important to understand how to implement XOR correctly. Using in C to apply the XOR operation is straightforward. It’s crucial to ensure both operands are defined properly to avoid unexpected results or errors.
Advanced Topics and Latest Trends
Cutting-edge developments in the field
As technology progresses, XOR continues to appear in advanced applications, like error detection codes and secure communications. Its role in cryptographic algorithms keeps evolving, further cementing its importance in modern computing.
Advanced techniques and methodologies
Advanced usages of XOR may involve combining it with other mathematical functions to solve complex computational problems. Techniques like masking or role in neural networks are also areas where XOR is essential, showcasing its versatility.
Future prospects and upcoming trends
The drive for innovation in data networks and security suggests that XOR operations will continue shaping future methodologies in data science and programming languages. Keeping an eye on these trends will help developers remain competitive in their skills.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
"The C Programming Language" by Brian W. Kernighan and Dennis M. Ritchie
- Online platforms like Coursera and Udemy often feature courses on C programming and algorithms.
Tools and software for practical usage
To experiment with XOR and deepen your understanding, tools like Code::Blocks or Dev-C++ could be beneficial. Integrating these resources into learning practices will enhance programming capabilities.
By understanding XOR, you not only equip yourself with a powerful tool but also gain insights that can significantly enhance your programming acumen.
Prologue to XOR in
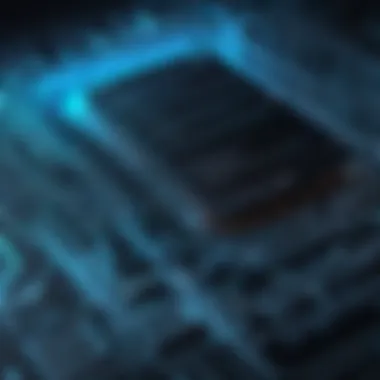
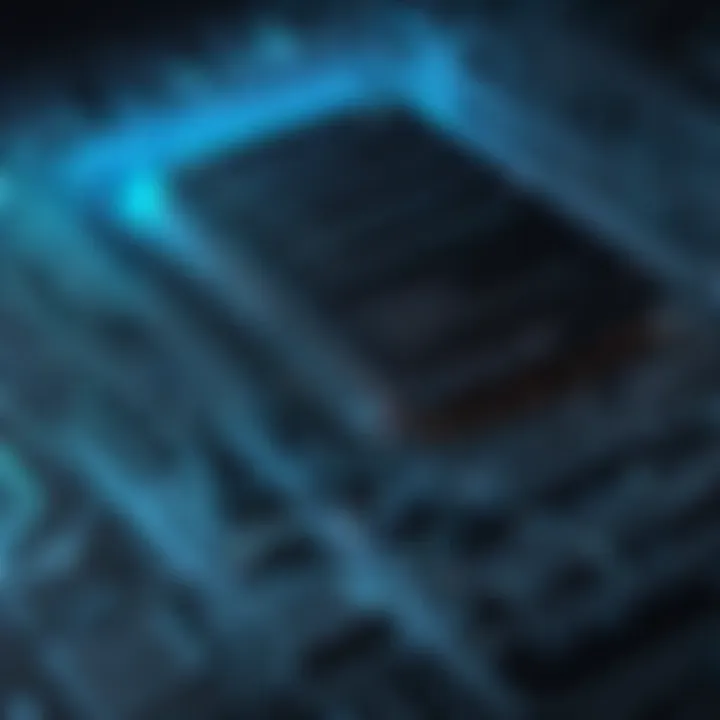
When delving into the realm of programming, each bit and byte often holds critical importance. Among various operations, XOR, or exclusive OR, creates unique effects and efficiencies that are invaluable for many coding scenarios. Understanding XOR in C is essential for manipulating binary data, optimizing performance, and implementing algorithms that require intricate bit manipulations. This article not only sheds light on the inherent functionality of XOR but also emphasizes its meaningful applications in various domains.
Defining XOR
XOR stands for "exclusive OR" and serves as a bitwise operator. It functions differently from its standard OR counterpart. Creatively, XOR compares corresponding bits between two binary numbers. The rule of thumb is straightforward: the result is true (or '1') if one, and only one, of the bits is true. If both bits are the same, either both zero or both one, the output is false (or '0'). To illustrate:
- Bit1: 0 1 0 1
- Bit2: 1 0 1 0
- Result: 1 1 1 1
This simplicity belies XOR's power, as it's routinely employed in applications from encryption to network security. These operational intricacies will be unpacked as the article progresses, giving readers an enriched perspective on how this operator shapes the coding landscape.
Historical Context
The concept of XOR isn't new. Its origins trace back to binary logic principles devised in the earlier days of computer science. The exclusive OR operation was first formalized in the mid-20th century during the development of electronic circuits and logic gates. Ideas around binary operations have evolved, but XOR retained its relevancy due to its versatility.
In simpler times, using XOR was enough to optimize space in hardware, efficiently reducing the complexity of circuit designs. As the field of computing advanced, the amplitude of XOR's application expanded. It pivoted from hardware implementations to high-level programming languages like C. Today, new generations of programmers continue to rely on XOR, driven by the need to solve complex problems efficiently.
Understanding how XOR operates in both historical and practical contexts is crucial for grasping its relevance in today’s programming tasks.
The implications of XOR extend far beyond mere theoretical exploration. As we continue with this article, we will dive into its operational mechanics, application scenarios, and how XOR can be a programmer’s best friend when properly understood.
Binary Basics
When diving into the realm of programming, especially in languages like C, understanding binary numbers and operations becomes crucial. This section serves as the bedrock for comprehending how XOR functions, as it operates at the binary level. Binary, by its very nature, is the language that computers speak; thus, any programmer stepping into the world of coding must first grasp its fundamentals. Let's break down the essential components that make binary such an important topic.
Understanding Binary Numbers
Binary numbers, unlike the decimal system we use daily, rely exclusively on two symbols: 0 and 1. This might seem simplistic, but these two digits hold profound significance in data representation. Every piece of data—be it a letter, a number, or an image—eventually translates into binary form for storage and processing within the computer’s memory.
Here's a quick overview:
- Base-2 System: The binary system is based on powers of 2. Thus, each digit's value is a power of 2, depending on its position.
- Representation of Decimal Numbers: For instance, the decimal number 5 translates into binary as 101. Each '1' and '0' in this representation corresponds to the values of the powers of 2: 1*(2^2) + 0*(2^1) + 1*(2^0).
- Critical for Understanding Operations: Having a keen understanding of binary numbers is essential not just for performing math but also for grasping higher-level concepts like bitwise operations, which will be elaborated on in the next subsection.
With this foundation, you’re now equipped to proceed into the next essential area—how we manipulate these binary digits through various operations.
Bitwise Operations Overview
Bitwise operations are integral to optimizing code performance in C, and they're heavily reliant on an understanding of binary numbers. These operations perform actions on specific bits of binary numbers in real time, allowing the programmer to carry out efficient computations. Here are a few key aspects of bitwise operations to keep in mind:
- Types of Bitwise Operators: In C, the primary bitwise operators include:
- Practical Applications: Each of these operators serves different purposes, often geared towards tasks like data manipulation, setting or clearing bits, and more. For example, XOR can be utilized in processes like swapping variables without needing a temporary variable—a nifty trick that maintains performance efficiency during manipulation.
- Performance Benefits: Using bitwise operations can drastically enhance the performance of your code. They are generally faster than arithmetic operations, and understanding how to apply them is essential for programmers looking to optimize resource use in their applications.
- AND ()
- OR ()
- NOT ()
- XOR ()
- Left Shift (``)
- Right Shift ()
Bitwise operations can make or break your coding efficiency—knowing when and how to use them is a game changer.
In summary, the binary basics set the stage for effectively implementing XOR and other bitwise operations in C. By appreciating how binary numbers function and the versatility of bitwise operations, a programmer can unlock a wealth of coding potential.
The XOR Operation Explained
The XOR operation, short for exclusive OR, is a cornerstone of bitwise manipulation in programming, particularly in C. This operation is not just a mathematical trick tucked away in textbooks; it's an integral part of how developers create efficient algorithms and optimize code for performance. When we break down the XOR function, we uncover its mechanics and how its application can enhance data handling.
In short, the importance of understanding XOR lies in its unique properties. Unlike traditional logical operators, XOR outputs true when the inputs differ. This means that it can be a powerful tool for encoding and decoding data, performing operations in a minimalistic manner, and even solving complex algorithmic challenges.
Mechanics of XOR
To grasp how XOR works, consider its function with binary digits. The XOR operation takes two bits and evaluates them based on the following rules:
- If both bits are 0, the result is 0.
- If the first bit is 0 and the second bit is 1, the result is 1.
- If the first bit is 1 and the second is 0, the result is 1.
- If both bits are 1, the result is 0.
In practical terms, when you apply XOR to binary numbers, you are effectively comparing each corresponding pair of bits. For example, if you XOR the binary numbers and , the operations unfold like this:
You can see that wherever the bits differ, the resulting bit is . Where they are the same, the output is .
Visualizing the XOR Operation
Using XOR, you can visualize its operation with colored lights:
- Light A (on) and Light B (off) makes Light C (on).
- Light A (off) and Light B (on) makes Light C (on).
- Light A (on) and Light B (on) makes Light C (off).
- Light A (off) and Light B (off) makes Light C (off).
This clarity in operation not only simplifies programming but also helps when devising algorithms that utilize the XOR operation, like in data encryption techniques.
Truth Table Analysis
Turning to the truth table, we can deepen our understanding of the XOR operation. A truth table provides a clear representation of all possible input scenarios and their corresponding outputs:
| Input A | Input B | Output (A XOR B) | | 0 | 0 | 0 | | 0 | 1 | 1 | | 1 | 0 | 1 | | 1 | 1 | 0 |
From this table, it's clear just how the XOR distinction plays a role in computational logic. The definitive outputs can be critical in algorithm design, especially when it comes to identifying unique conditions or states.
Key Insight: Understanding the truth table of XOR is crucial for recognizing how this operator can behave as a powerful tool for condition checks and branching in programming.
The XOR operation's distinct nature opens the door to various practical applications, particularly in security and error detection realms. By recognizing and applying its unique mechanics, programmers harness the potential to innovate and streamline numerous computational tasks.
Implementing XOR in
When diving into C programming, grasping how to implement XOR is pivotal. This operator is not just a fancy tool for bit manipulation; it plays a crucial role in enhancing data security and optimizing algorithms. Understanding XOR in practical terms allows programmers to harness its potential effectively, creating more efficient and secure applications.
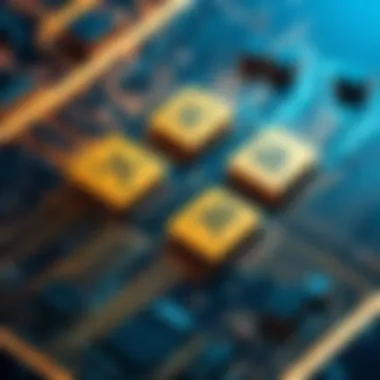
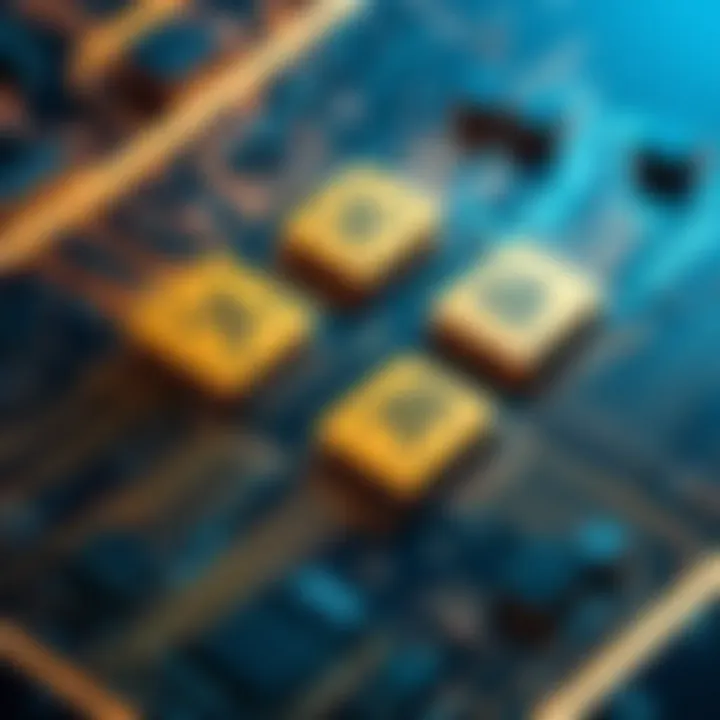
Syntax of XOR in
In C, the XOR operation is denoted by the caret symbol . To apply XOR between two int variables, you can simply use the syntax shown below:
Here, and are the two operands, and will hold the outcome after performing the XOR operation. It works by comparing corresponding bits of the operands; if they differ, the result for that bit is , and if they are the same, it is . Simple but powerful.
Basic Example Code
Let's get our hands dirty with some code to solidify our understanding. Consider the snippet below that demonstrates XOR in action:
When you run this program, you will see that results in . This example not only illustrates how XOR can be implemented but also highlights its utility in data transformation.
Common Errors and Debugging
Although using XOR can be straightforward, a few common pitfalls could trip you up. Here are some frequent mistakes programmers encounter:
- Assuming XOR behaves like normal arithmetic: Remember, it's a bitwise operation. Misunderstanding its behavior might lead you down a rabbit hole of frustration.
- Not considering the data types: When working with different types, such as mixing integers with unsigned types, unexpected results might arise. Always ensure operands are of compatible types.
- Neglecting operator precedence: In complex expressions, XOR might not execute in the desired order. Parentheses can be your best friend here, helping to clarify the sequence of operations.
When debugging, this is a good time to verify the input values and intermediary results using print statements. Also, using a debugger can shine a light on what’s happening under the hood with the binary representations of your variables.
By mastering the syntax and behavior of XOR in C, programmers can tackle a variety of challenges, from efficient data handling to improved algorithm performance.
Practical Applications of XOR
The XOR operation is not just a theoretical construct; it has significant practical implications that extend across various fields in programming, particularly in C. Understanding how XOR operates can unlock various toolsets and techniques for developers, enhancing their problem-solving skills and optimizing performance. This section delves into two critical applications of XOR: data encryption and error detection and correction.
XOR in Data Encryption
Data encryption is vital in today’s world, where security plays a paramount role. XOR’s properties make it especially valuable in constructing encryption algorithms. The core principle of XOR is that it outputs true or 1 only when the inputs differ. This characteristic is fundamental in creating a symmetric encryption method where the same key can be used to both encrypt and decrypt data.
When you take a plain text and combine it with a secret key using the XOR operation, you create cypher text that is incomprehensible without the key. Consider this simple illustration:
- Plain Text: Hello
- Key: 101010
When you XOR the binary representation of "Hello" with the key, it alters the bits in such a way that it becomes hard to decipher without the corresponding key. This method is particularly fast, leveraging bitwise operations which are directly supported by machine-level instructions, making XOR an efficient choice for encryption.
Moreover, the ease of implementation in C allows for broad usage across various software, from web applications to mobile devices. However, it’s essential to note that while XOR provides a robust and rapid means of data encryption, it should not be the sole method used in production-level scenarios. Using it in combination with other secure practices like key rotation and strong key management is crucial.
"In programming, as in life, simplicity is key; yet, simplicity in security can fall prey to vulnerabilities."
Error Detection and Correction
Another significant application of the XOR operation lies in error detection and correction. In digital communications and data storage, it’s crucial to ensure that information remains intact during transmission or retrieval. Using XOR in parity checks and checksums provides an easy yet effective solution to verify data integrity.
For instance, in parity bits, the XOR operation can determine whether the number of 1-bits in a data unit is even or odd. If we represent data with bits and perform an XOR operation across them, the results can help assess whether some bits have been flipped during transfer. If the XOR output is not what was expected, this indicates a potential error.
In more sophisticated error-correcting codes, like Hamming codes, XOR serves as a building block. These codes utilize multiple XOR operations to identify and correct errors in data blocks. An n-bit code can be formed, allowing the recovery of original data even if a small number of bits were altered.
- Benefits of Using XOR in Error Detection:
- Considerations:
- Simple to implement.
- Cost-effective in terms of resource usage.
- Works accurately even for large datasets.
- Vulnerable to certain types of errors if used independently.
- Recommended to use alongside other error detection strategies for robustness.
Comparing XOR with Other Bitwise Operators
To grasp the full significance of XOR in C programming, it is essential to juxtapose it with other bitwise operators. In C, we have several bitwise operators like AND and OR, each with its own unique behaviors and applications. Understanding how XOR stands apart from these operators not only helps in realizing its capabilities but also highlights its advantages and potential pitfalls.
XOR vs AND
The AND operator performs a fundamental function by checking whether both bits are set. For any two binary digits, it returns a one only if both bits are one. For instance, when bitwise comparing 1 and 1 using AND, the result is 1; however, any other combination yields 0. This operator is often employed in masking operations where we want to isolate certain bits in a number.
Consider the following examples:
- Example:
- Binary: 1 AND 1 = 1
- Binary: 1 AND 0 = 0
- Binary: 0 AND 1 = 0
- Binary: 0 AND 0 = 0
The AND operator can be quite handy when we need to check flags or states in a system, where multiple conditions need to be verified concurrently. In contrast, XOR shines with its distinct behave. It toggles bits but only yields true (1) when exactly one bit is set to true (1). This is particularly useful in various programming strategies where a switch or alternate condition is needed.
For clarity, here’s how XOR operates in a similar scenario:
- Example:
- Binary: 1 XOR 1 = 0
- Binary: 1 XOR 0 = 1
- Binary: 0 XOR 1 = 1
- Binary: 0 XOR 0 = 0
Key Takeaway: While both XOR and AND can be applied in masking, they serve different purposes. AND simply confirms if conditions are met entirely, whereas XOR focuses on which bits are different. Both can be potent tools depending on the task at hand.
XOR vs OR
Now, let’s delve into how XOR stacks up against the OR operator. The OR operator pushes boundaries by checking if at least one of the bits is set. It will return 1 if either bit of the compared pair is 1. This makes it incredibly useful for combining features or toggling states.
Evidence of this behavior can be seen as follows:
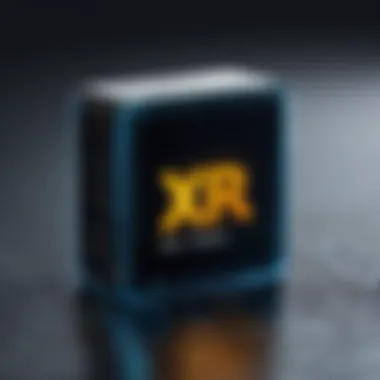
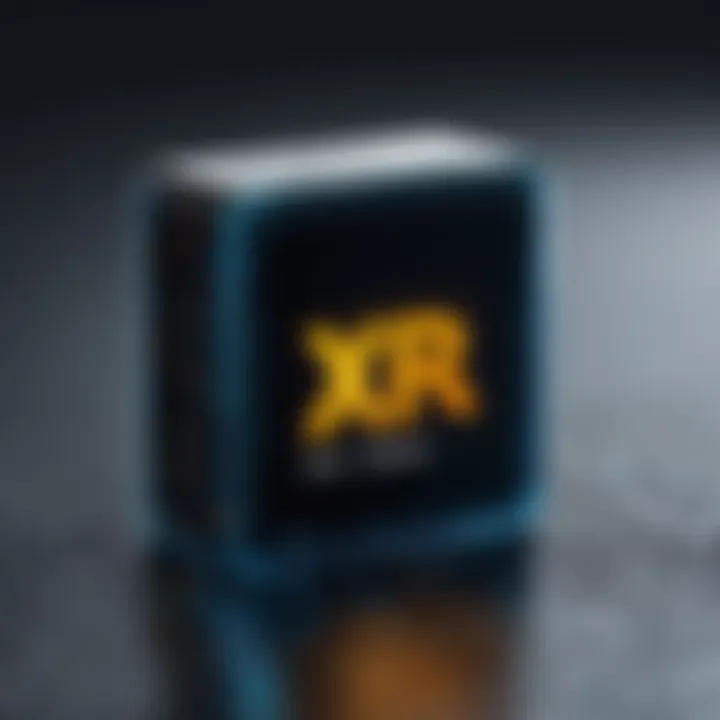
- Example:
- Binary: 1 OR 1 = 1
- Binary: 1 OR 0 = 1
- Binary: 0 OR 1 = 1
- Binary: 0 OR 0 = 0
In scenarios where you might want any of the multiple conditions to trigger a specific outcome, OR is your operator of choice. The juxtaposition with XOR becomes clear here. XOR will produce a 1 output only when one of the bits is set but not both.
- Example:
- Binary: 0 XOR 0 = 0
- Binary: 0 XOR 1 = 1
- Binary: 1 XOR 0 = 1
- Binary: 1 XOR 1 = 0
Key Takeaway: OR brings flexibility in confirming conditions, while XOR’s strength lies in discerning alternating states. Each operator has its own behavior that prescribes its usage context.
Through understanding the differences among these bitwise operators, developers can harness the right tool for the job, enhancing the efficiency and effectiveness of their code.
XOR Use Cases in Algorithms
XOR, or exclusive OR, serves as a powerful tool in the world of algorithms, often revealing solutions that can simplify complex problems. When harnessed correctly, XOR can enhance both the efficiency and effectiveness of code. With this in mind, let's explore two specific use cases that illustrate its importance and application in programming.
Swapping Variables Using XOR
Swapping two variables usually requires a temporary variable to hold one of the values. However, with XOR, you can bypass this necessity altogether. This method is particularly useful when aiming for minimal memory usage.
The principle is straightforward:
- XOR the two variables and store the result in one of the variables, say . Now holds the XOR of and .
- XOR the result with and assign it to . This operation effectively retrieves the original value of and stores it in .
- XOR the result with the new and assign it back to . Now contains the original value of .
Here is a brief example in C:
What’s especially noteworthy about this approach is that it operates in constant time, O(1), which is often a priority for developers focusing on algorithm efficiency. However, some consider using temporary variables clearer and more maintainable, especially for those new to programming.
Finding Unique Elements
Another fascinating application of the XOR operation is in discovering unique numbers within a dataset, particularly when every number appears twice except for one. The beauty of this approach lies in the properties of XOR:
- XOR-ing a number with itself gives zero: This property allows pairs of identical numbers to cancel each other out.
- XOR-ing a number with zero returns the number itself: This is crucial for ultimately retrieving the unique number.
To illustrate this, consider the following scenario: You have an array: [2, 3, 5, 3, 2]. In this array, all elements appear twice, except for . By XOR-ing all numbers together, the duplicates cancel out, leaving only . Here’s how it works in code:
This method is efficient, with a time complexity of O(n) and a space complexity of O(1), making it ideal for applications like data analysis and error detection. However, one has to be cautious when there are additional unique numbers or a more complex dataset, as the approach may need adaptation.
Thus, XOR’s flexibility and efficiency make it a valuable tool for optimizing algorithms, especially when working with bitwise operations. While some traditional methods might seem straightforward, they can often benefit from XOR’s unique properties.
Performance Considerations
When discussing XOR in C programming, one crucial aspect often overlooked is the performance considerations involved in using this operator. The efficiency of XOR operations can significantly influence the performance of algorithms, particularly those pertinent to cryptography, data analysis, and error detection. In this section, we'll delve into how XOR's efficiency and resource usage impact programming processes.
Efficiency of XOR Operations
XOR operations stand out for their speed and simplicity. The efficiency of XOR is largely attributed to how it operates at the bit level. Compared to other binary operations, such as AND and OR, XOR can be executed with fewer CPU cycles in many architectures. Here’s why this matters:
- Speed: XOR provides nearly instantaneous results because it operates directly on the binary representation of numbers, requiring minimal computational resources.
- Low Latency: In scenarios where multiple operations are sequenced, leveraging XOR minimizes delays, ensuring a quicker response time for applications.
- Single-cycle Execution: In many cases, XOR can be executed in a single CPU cycle, particularly on modern hardware, which makes it preferred in high-performance contexts like graphics computation or ciphertext manipulation.
For instance, when implementing algorithms to swap two variables, using XOR can eliminate the need for temporary storage, thus improving overall execution speed.
Here's a simple code snippet to illustrate the efficiency of using XOR for swapping variables:
This XOR operation allows developers to perform a swap without a third variable, saving both time and memory, which is particularly important in tight loops or resource-conscious applications.
Impact on Resource Usage
The impact of XOR operations on resource usage primarily hinges on memory and processing power. Since XOR is typically executed at the hardware level, it utilizes less memory overhead compared to other arithmetic operations. Here are several key points to ponder about resource usage when employing XOR:
- Memory Efficiency: XOR can effectively manipulate bits without requiring additional memory space, making it an excellent choice in embedded systems where memory is scant.
- Less Power Consumption: Given XOR's efficiency, it often results in lower power consumption during processing, which is vital for battery-operated devices.
- Reduced Complexity: The simplicity of XOR reduces the likelihood of software complexity, leading to fewer bugs and thus less debugging time, which can be a costly endeavor.
Considering these factors, programmers must assess whether the use of XOR will yield a tangible performance benefit in their specific application. In some cases, especially with shifted bit operations on larger datasets, the gains might not be as pronounced.
"Understanding the efficiency and resource implications of XOR can be the difference between a standard algorithm and a high-performance solution."
The End
The conclusion serves as a vital element in wrapping up the intricate discussions around XOR in C programming. It synthesizes the main points laid out in the article and reinforces the relevance of XOR in both theoretical and practical contexts. In today’s tech-driven world, understanding such bitwise operations is not just beneficial but essential for efficient programming.
Summarizing Key Points
XOR stands as a critical player in various programming paradigms, particularly in C. Here’s a succinct recap of its significance:
- Fundamental to Data Manipulation: XOR allows for efficient data processing and manipulation at the binary level, a must-know for anyone diving into low-level programming.
- Practical Applications: From encryption to error detection, the use cases for XOR are abundant, showing its versatility in real-world applications.
- Comparison with Other Operators: Understanding how XOR interacts with other bitwise operators enhances a programmer's toolkit, allowing for better decision-making in coding practices.
- Unique Solutions: Whether it’s swapping variables without a temporary variable or finding unique elements in arrays, XOR provides elegant solutions that often outperform traditional methods.
"In programming, understanding the how and why of operations like XOR paves the way for more efficient and innovative solutions."
Future Implications of XOR in Programming
As we move forward, the implications of XOR in programming are poised to expand, particularly with the rise of advanced programming paradigms and technologies. Here are a few anticipated directions:
- Growing Relevance in Cybersecurity: With data protection being more crucial than ever, XOR's role in developing secure encryption algorithms will remain significant. Its simplicity and effectiveness make it a staple in many security frameworks.
- Increased Use in Philosophies of Functionality: Modern programming strategies continue leaning towards functional programming, where simple, clean operations are favored. XOR fits well into this narrative, promoting efficient logic without unnecessary complexity.
- Innovation in Algorithms: As algorithm designs evolve, XOR could foster new methods of processing large data sets quicker and with lower resource usage, aligning with industry pursuits of speed and efficiency.
In summary, the discussion on XOR is more than just a programming lesson; it's a window into how fundamental concepts breathe life into modern coding practices. Embracing XOR not only enriches a programmer's skill set but also fuels advancements in tech as a whole.