Unlocking CUDA Power in Python: A Step-by-Step Guide to GPU Acceleration
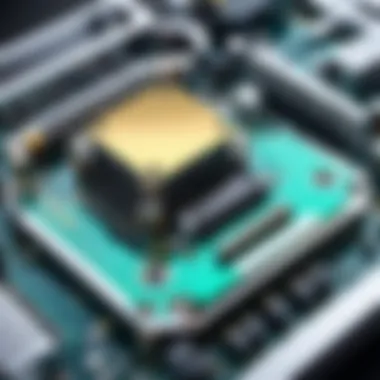
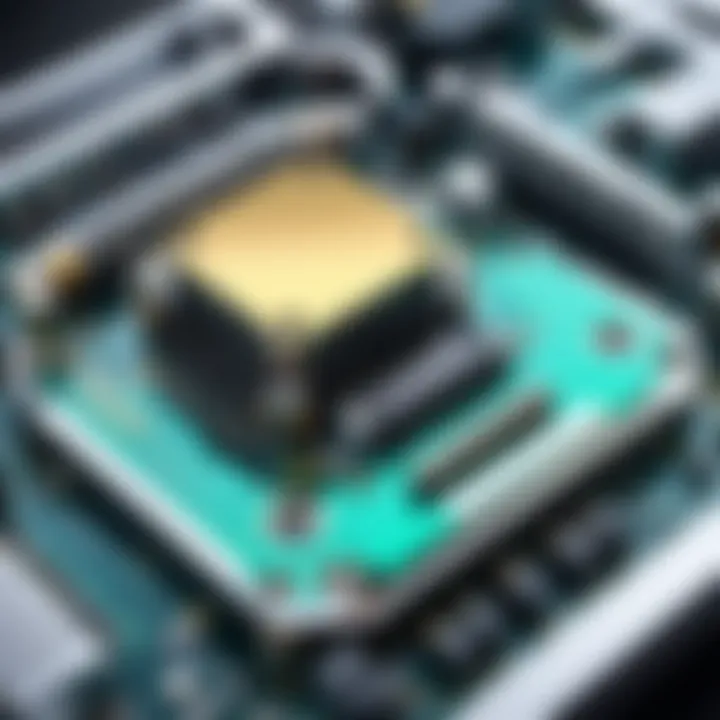
Fundamentals Explained
Delving into the realm of harnessing CUDA in Python opens doors to exceptional computational capabilities. CUDA, an abbreviation for Compute Unified Device Architecture, is a parallel computing platform developed by NVIDIA enabling programmers to utilize GPU for parallel processing tasks. This section will provide a comprehensive breakdown of the core principles and theories underlying CUDA, elucidating the fundamental concepts at play.
Core Principles and Theories
CUDA operates on the principle of exploiting the massive parallel processing power of GPUs to accelerate computations. By offloading the intense computation tasks from the CPU to the GPU cores, CUDA significantly enhances performance in tasks that can be parallelized. Understanding this fundamental concept is crucial for harnessing the true potential of CUDA in Python programming.
Key Terminology and Definitions
To navigate the world of CUDA effectively, grasping key terminology and definitions is indispensable. Terms such as threads, blocks, kernels, and grids encapsulate the building blocks of CUDA programming. Threads represent individual units of execution within a block, while blocks group threads together. Kernels are functions executed on the GPU, and grids contain multiple blocks. Familiarizing oneself with these terms is paramount for proficient CUDA programming.
Basic Concepts and Foundational Knowledge
Building a strong foundation in CUDA programming requires a grasp of basic concepts. From memory management to parallel algorithms, understanding how data is passed to GPU memory and efficiently processed through parallel computation is essential. Through mastering these basic concepts, programmers can leverage CUDA's immense power to optimize their Python applications for enhanced performance.
Introduction to CUDA and Python Integration
In this section, we delve into the crucial aspects of merging CUDA capabilities with Python, a combination that unlocks immense potential for computational power. The synergy between CUDA and Python opens up a plethora of opportunities for tech enthusiasts, beginners, and professionals to harness the power of parallel processing through GPU acceleration. Understandably, this integration is pivotal as it bridges the gap between high-performance computing and user-friendly programming, revolutionizing how tasks are handled in the realm of data science, machine learning, and scientific computing. By exploring the seamless integration of CUDA in Python workflows, individuals can elevate their coding capabilities and unlock new horizons of computational efficiency.
Understanding the Basics of CUDA
Introduction to GPU Computing
Diving deep into the realm of GPU computing provides a foundational understanding of how GPUs revolutionize parallel processing tasks in computing. GPU computing, with its emphasis on parallelism and massive data throughput capabilities, stands as a groundbreaking approach in contrast to traditional CPU-centric computing. The ability of GPUs to handle thousands of parallel threads concurrently to tackle intensive computational tasks is a defining attribute that sets GPU computing apart. This not only enhances processing speed but also offers a more cost-effective solution for computationally demanding applications within the ambit of CUDA integration.
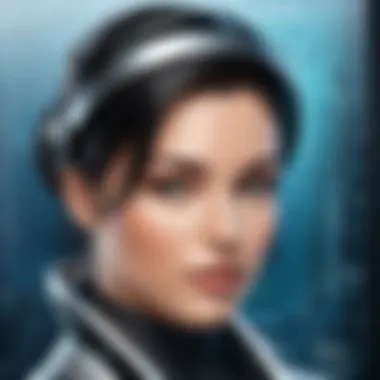
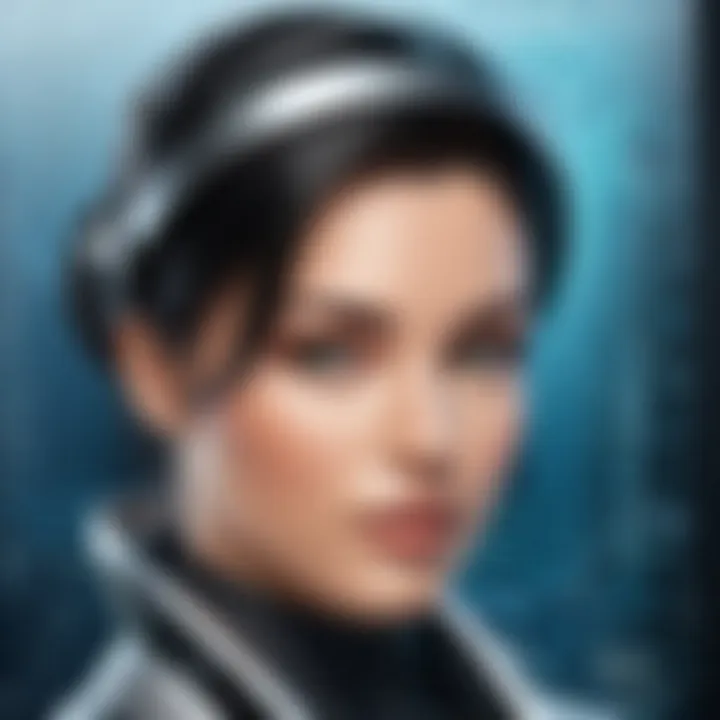
History and Evolution of CUDA Technology
Exploring the historical trajectory and evolution of CUDA technology sheds light on the milestones that have shaped the current landscape of GPU computing. Developed by NVIDIA, CUDA has a rich history of advancements that have propelled it to the forefront of parallel processing technologies. The evolution of CUDA has seen remarkable progress in optimizing algorithms for diverse GPU architectures, thereby enhancing performance and scalability. Embracing CUDA technology not only grants access to cutting-edge tools for parallel processing but also ensures compatibility with a wide array of GPU-accelerated applications, solidifying its position as a preferred choice for developers seeking efficient computational solutions.
Advantages of Utilizing CUDA for Parallel Processing
The advantages of leveraging CUDA for parallel processing are manifold, offering a significant edge in computational efficiency and performance optimization. By harnessing the parallel computing prowess of GPUs, CUDA enables the acceleration of tasks that require massive data handling and intensive computations. This translates into reduced processing times, higher throughput, and efficient utilization of computational resources. Moreover, CUDA's compatibility with popular programming languages like Python enhances its accessibility, making parallel processing capabilities more approachable for a wider audience of developers and researchers.
Setting up CUDA Environment in Python
The section on setting up the CUDA environment in Python is pivotal within the comprehensive tutorial on utilizing CUDA in Python. A crucial step in integrating CUDA with Python involves the installation of the CUDA Toolkit, which is essential for leveraging the power of GPU acceleration. Configuring environment variables for CUDA ensures smooth communication between Python and CUDA, optimizing the performance of parallel processing tasks. Setting up the CUDA environment in Python lays the foundation for harnessing the computational capabilities of GPUs effectively, enabling users to enhance their programming workflows significantly.
Installing CUDA Toolkit
Downloading and Installing CUDA Toolkit:
The process of downloading and installing the CUDA Toolkit plays a vital role in this tutorial on CUDA integration in Python. By downloading and installing the CUDA Toolkit, users gain access to a suite of tools and libraries specifically designed for GPU-accelerated computing. The seamless installation process simplifies the setup of CUDA development environments, providing programmers with a robust platform to harness parallel processing capabilities efficiently. The CUDA Toolkit stands out for its comprehensive documentation and community support, making it a popular choice among developers seeking to optimize their Python workflows using GPU acceleration. Its compatibility with a wide range of GPUs and operating systems enhances its versatility, making it a valuable asset for users engaging in CUDA programming within the Python ecosystem.
Configuring Environment Variables for CUDA:
Configuring environment variables for CUDA is a critical aspect of setting up the CUDA environment in Python. By configuring these variables, users define the paths and settings necessary for Python to interact seamlessly with the CUDA Toolkit. This configuration ensures that CUDA libraries and dependencies are accessible to Python scripts, facilitating the execution of GPU-accelerated code. The ability to customize environment variables based on hardware specifications and project requirements enhances the flexibility of CUDA integration in Python, enabling users to optimize performance based on their specific needs. While the configuration process may vary across platforms, configuring environment variables for CUDA remains a fundamental step in establishing a productive development environment for CUDA programming in Python.
Implementing CUDA Parallel Processing in Python
Implementing CUDA parallel processing in Python is a critical aspect of this comprehensive tutorial on leveraging CUDA capabilities within Python. This section focuses on harnessing the power of parallel execution using CUDA technology, which plays a pivotal role in enhancing computational performance. By utilizing CUDA for parallel processing in Python, users can significantly boost the efficiency of intensive computational tasks.
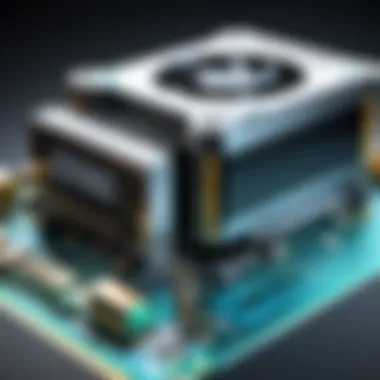
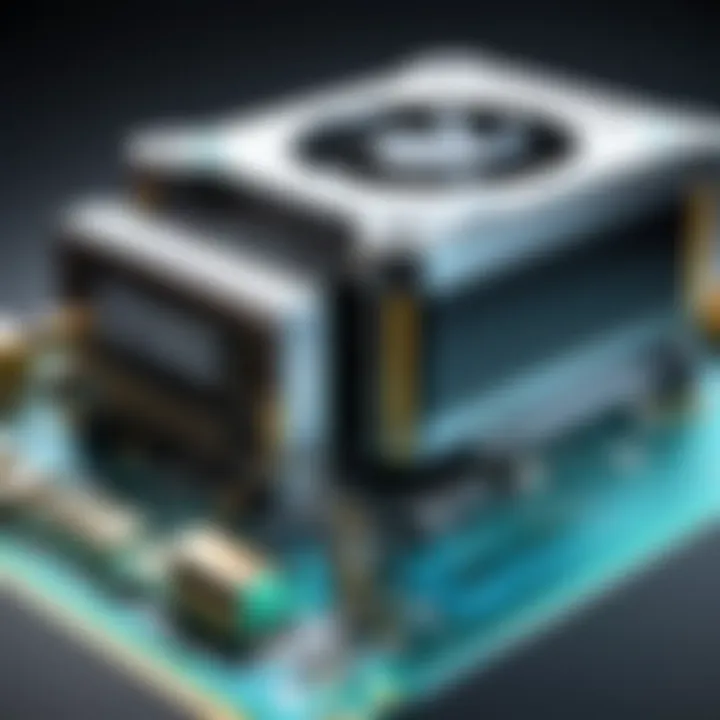
Parallel processing is of paramount importance in modern computing environments, where the demand for faster and more effective processing methods continues to grow. Implementing CUDA in Python allows for the execution of multiple tasks simultaneously, leveraging the full potential of GPUs for accelerated performance. This section explores how parallel processing with CUDA enhances the speed and efficiency of computation, making it an essential skill for anyone looking to optimize their Python workflows.
Writing CUDA Kernels in Python
Defining Kernel Functions for Parallel Execution
Writing CUDA kernels in Python involves defining kernel functions for parallel execution, a key component in optimizing computational tasks. These functions are designed to be executed in parallel by numerous threads on a GPU, allowing for efficient distribution of workload. By defining kernel functions, programmers can tailor the execution process to suit specific computational requirements, maximizing the benefits of GPU acceleration.
The unique characteristic of defining kernel functions lies in their ability to operate concurrently across multiple threads, facilitating the effective utilization of GPU resources. This approach enhances processing speed and efficiency, making it a popular choice for intensive computing tasks. Despite its advantages, programmers need to consider the intricacies of thread coordination and data dependency to ensure optimal performance.
Compiling CUDA Kernels in Python Environment
Compiling CUDA kernels in the Python environment is a crucial step in preparing code for execution on GPUs. This process involves translating the defined kernel functions into executable code that can run on CUDA-enabled devices. Compiling CUDA kernels streamlines the execution of parallel tasks, optimizing performance and resource utilization.
One significant advantage of compiling CUDA kernels in Python is the seamless integration of parallel processing capabilities into existing workflows. By compiling kernels, programmers can unlock the full potential of GPU acceleration within Python applications, enhancing computational speed and efficiency. However, care must be taken to address memory management and code optimization to avoid performance bottlenecks.
Utilizing GPU for Computational Tasks
Offloading Intensive Computations to GPU
Utilizing the GPU for offloading intensive computations is a strategic approach to improving computational performance. By transferring demanding tasks to the GPU, users can leverage its parallel processing capabilities to streamline calculations and data processing. Offloading computations to the GPU reduces the burden on the CPU, leading to faster and more efficient task execution.
The key characteristic of offloading intensive computations to the GPU lies in its ability to handle complex mathematical operations in parallel, resulting in accelerated processing speeds. This approach is particularly beneficial for tasks that involve matrix operations, simulations, and machine learning algorithms. However, developers must carefully manage data transfers between the CPU and GPU to avoid latency issues.
Optimizing Performance through Parallel Execution
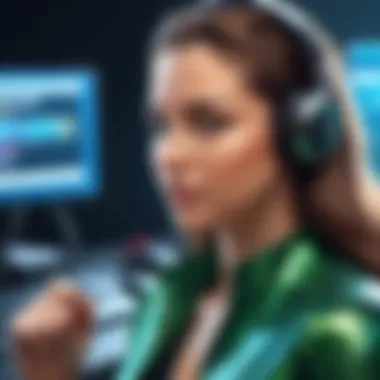
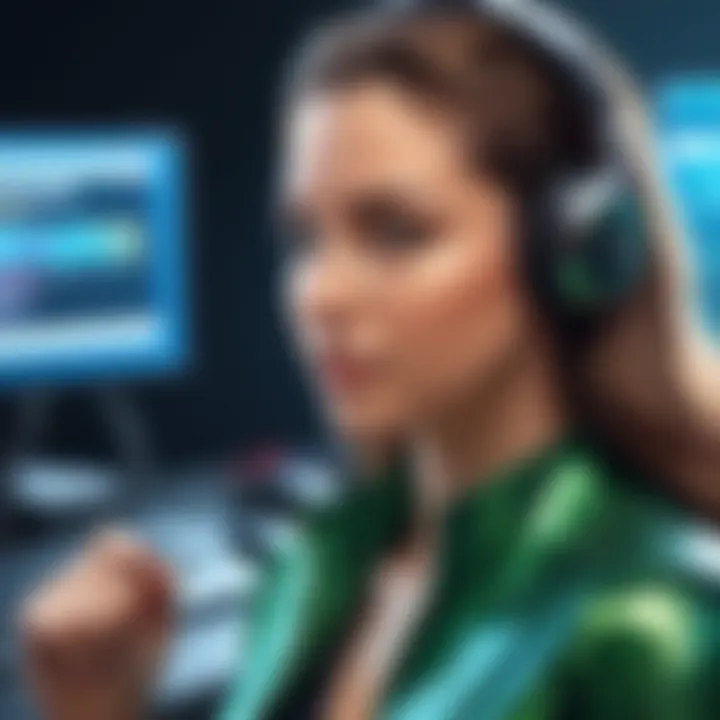
Optimizing performance through parallel execution is essential for maximizing the benefits of GPU acceleration in Python workflows. By parallelizing computational tasks, programmers can distribute the workload efficiently across GPU cores, enhancing overall system performance. This approach minimizes processing bottlenecks and improves the scalability of applications.
The key characteristic of optimizing performance through parallel execution is its ability to boost computation speed and efficiency, especially for tasks that can be parallelized across multiple threads. By leveraging the computational power of GPUs, developers can achieve significant performance gains in tasks such as data processing, image rendering, and scientific simulations. However, optimizing parallel execution requires careful consideration of thread management and synchronization to ensure optimal results.
Optimizing CUDA Performance in Python
Optimizing CUDA performance in Python is a pivotal aspect discussed in this article, focusing on how to enhance the efficiency of parallel processing tasks utilizing CUDA technology. By delving into optimizing CUDA performance, readers can extract maximum computational capabilities from GPUs, leading to a significant boost in overall program speed and memory management. This section explores various strategies such as fine-tuning parameters and analyzing performance metrics to fine-tune GPU-accelerated processes. Understanding the intricacies of CUDA performance optimization is crucial for achieving peak performance in Python-based applications, making it a key component of this comprehensive tutorial.
Profiling and Benchmarking CUDA Applications
Tools for Profiling CUDA Code
In this section, the spotlight is on the indispensable role that tools for profiling CUDA code play in enhancing the optimization process for CUDA applications. These tools offer valuable insights into the performance bottlenecks and resource utilization within CUDA-accelerated programs, guiding developers towards efficient code optimization. By analyzing key metrics and performance indicators, developers can identify areas for improvement and fine-tune their code to achieve optimal performance. This detailed analysis provided by profiling tools elevates the efficiency and effectiveness of CUDA applications, making them a crucial asset in the optimization journey.
Analyzing Performance Metrics in Python
The analysis of performance metrics in Python serves as a vital component in evaluating the effectiveness of CUDA implementations within Python environments. By scrutinizing performance metrics, developers can gauge the impact of CUDA utilization on application performance and identify areas ripe for enhancement. These metrics offer valuable insights into execution times, memory utilization, and computational efficiency, empowering developers to make informed decisions regarding optimization strategies. Leveraging performance metrics enables developers to fine-tune their CUDA implementations, ensuring maximum efficiency and performance in Python-based applications.
Fine-tuning CUDA Parameters
Adjusting Thread Blocks and Grid Dimensions
The intricate process of adjusting thread blocks and grid dimensions plays a crucial role in optimizing CUDA performance within Python applications. By finely calibrating thread block sizes and grid dimensions, developers can achieve a balance between workload distribution and resource utilization, maximizing parallel processing efficiency. This meticulous adjustment allows for the optimal utilization of GPU resources, enhancing overall program performance and computational speed. Understanding how to adjust thread blocks and grid dimensions is essential for fine-tuning CUDA applications, making it a fundamental aspect of performance optimization.
Optimizing Memory Access Patterns
Optimizing memory access patterns is a key strategy in enhancing the performance of CUDA applications in Python. By optimizing memory access patterns, developers can minimize memory latency and maximize data throughput, leading to improved computational efficiency. This optimization technique focuses on streamlining data access and minimizing redundant memory operations, resulting in faster data retrieval and processing. Understanding how to optimize memory access patterns empowers developers to boost the performance of their CUDA applications, making it a crucial aspect of performance optimization within Python environments.
Conclusion and Further Exploration
In the final section of this comprehensive tutorial on