Mastering Web Scraping with BeautifulSoup in Python
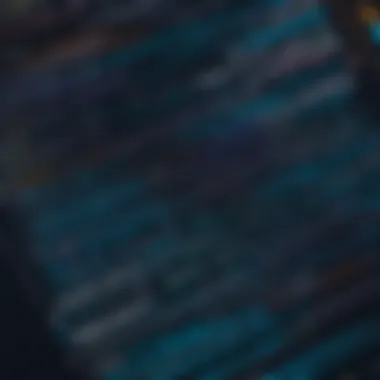
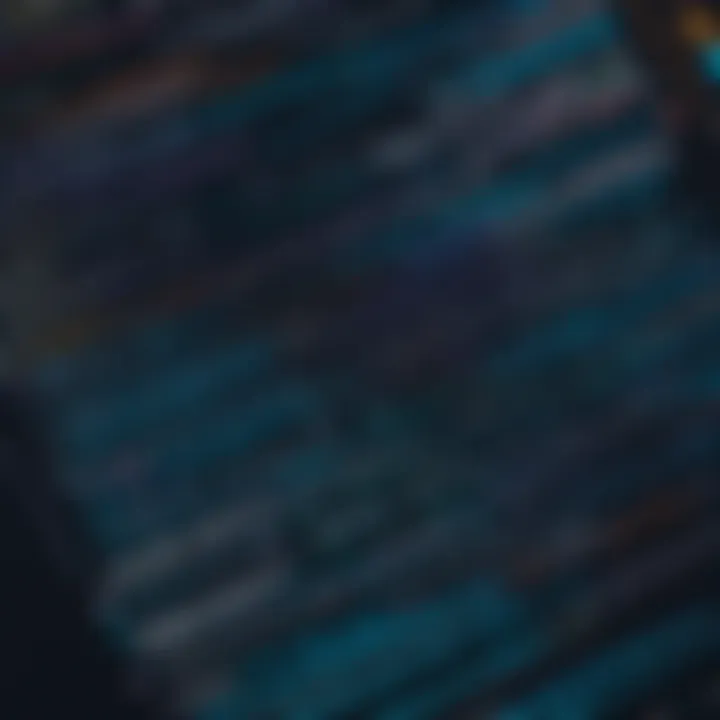
Overview of Topic
Web scraping has become a vital tool in the digital age. With mountains of data available online, extracting the right information efficiently is crucial. This is where BeautifulSoup comes into play. BeautifulSoup is a Python library that streamlines the process of web scraping by providing simple methods to navigate, search, and modify the parse tree resulting from the HTML or XML documents collected from the web.
The significance of web scraping canāt be overstated, particularly in sectors like e-commerce, data analysis, and journalism. Businesses constantly seek the latest market trends, prices, and insights about competitors. By automating this extraction of data, organizations save time and bolster their decision-making capabilities.
BeautifulSoup's inception dates back to 2004, crafted by Leonard Richardson. Over the years, it has undergone various tweaks and improvements to adapt to the swiftly changing landscape of web technologies. Its ability to work harmoniously with different parsers, like lxml and html5lib, sets it apart, making it a top choice among developers.
Fundamentals Explained
Understanding the core principles of BeautifulSoup is fundamental for anyone diving into web scraping.
Key Terminology:
- Parser: A tool that interprets the content of HTML or XML in a structured way.
- Document Object Model (DOM): The hierarchy that represents the structure of a webpage.
- Tag: An element in HTML that signifies structure (like ``, , etc.).
At its core, BeautifulSoup allows for easy navigation through the parse treeāthink of it as flipping through a book, where tags and their corresponding content form the chapters and paragraphs. The foundational knowledge hinges on knowing how to search and filter data through its methods, like and , to pinpoint specific elements within the document.
Practical Applications and Examples
Consider a scenario where an analyst needs to track the pricing of specific products across various e-commerce websites. By utilizing BeautifulSoup, they can quickly set up a script to gather this data consistently.
Hereās a simple example of how one might extract product titles from a webpage:
This code fetches product titles from a designated URL, parsing the HTML and searching for the right tags.
Real-world applications span various fields:
- Market Research: Competing prices and trends are monitored.
- Content Aggregation: News articles and blogs are indexed for easier access.
Advanced Topics and Latest Trends
As web technologies evolve, so do scraping techniques. One noteworthy trend is the incorporation of AI and machine learning in web scraping strategies. This involves using predictive algorithms to determine which data points are most relevant based on historical patterns.
Scrapers are increasingly incorporating headless browsers like Selenium to handle dynamic content that traditional libraries might miss. This blend of technologies allows for more robust data extraction, ensuring nothing slips through the cracks.
Tips and Resources for Further Learning
To deepen your understanding of BeautifulSoup and web scraping, the following resources are invaluable:
- Books: Web Scraping with Python by Ryan Mitchell.
- Online Courses: Websites like Codecademy and Coursera offer structured courses on web scraping.
- Tools and Software: Additional libraries like Requests and Scrapy can complement BeautifulSoup for a more thorough scraping experience.
For continual discussions and updates, consider joining communities on Reddit and checking official documentation on sites like Wikipedia).
"Knowledge is not power. Knowledge is only potential power. It becomes power when you take action."
Thus, with the right tools and knowledge, web scraping can be not just viable but a game-changer in various domains.
Foreword to BeautifulSoup
In the ever-evolving realm of data analysis and programming, web scraping has become a pivotal technique. Among the myriad of tools available, BeautifulSoup stands out as a versatile library that simplifies the intricate process of parsing HTML and XML documents. Understanding what BeautifulSoup offers is crucial for anyone involved in data extraction from websites, especially for those just dipping their toes into the world of Python programming.
BeautifulSoup not only facilitates the extraction of data but also makes navigating through complex HTML structures straightforward. By providing developers with a set of simple methods to access, traverse, and manipulate web content, it has earned its place as a go-to resource in the toolkit of programmers and data scientists alike. As we embark on this exploration of BeautifulSoup, we highlight its advantages, from ease of use to its ability to handle malformed markup. Such elements make it invaluable for efficient web scraping.
What is BeautifulSoup?
BeautifulSoup is a Python library that allows users to parse and navigate through HTML and XML documents. Its primary purpose is to provide tools that let you extract data from the web with minimal effort. The beauty of BeautifulSoup lies in its user-friendly design; it transforms even the messiest of web pages into a format that is much easier to work with. Think of it as a magnifying glass for your web dataāa way to zoom in on the details without getting lost in the clutter.
With its capabilities, developers can search for specific elements, modify tags, and retrieve data attributes. This library is particularly cherished for its elegance and flexibility, making it an excellent choice for both beginners and seasoned programmers.
Historical Context and Development
BeautifulSoup was first created by Leonard Richardson in the early 2000s. It has since evolved into a robust tool with widespread adoption in the programming community. Originally crafted to address the limitations of existing HTML parsers, BeautifulSoup's intent was to improve the experience of web scraping, allowing developers to retrieve information more efficiently and accurately.
Over the years, it has integrated feedback from users to enhance functionality and usability. This iterative development has led to the libraryās current version, which includes features like automatic conversion of incoming documents to Unicode and a vast range of functions to filter and search for tags easily.
The development of BeautifulSoup also coincides with the rising importance of data science and big data. As organizations began seeking more insights from web data, the libraryās ability to handle rapidly changing web formats became a game-changer.
Comparison with Other Web Scraping Libraries
When it comes to web scraping in Python, there's no shortage of options. However, BeautifulSoup holds a distinct place in the landscape. Its main competitors include libraries like Scrapy and lxml. Here's a breakdown of how BeautifulSoup compares:
- Ease of use: BeautifulSoup is often lauded for its user-friendly interface, making it an ideal starting point for individuals new to web scraping.
- Flexibility: It works well with various parsing engines, including lxml and html.parser, which allows developers to choose the best tool for their specific needs.
- Community Support: With a thriving community and extensive documentation, users can easily find help and resources, minimizing learning time.
However, it's important to note that while BeautifulSoup excels in simplicity, tools like Scrapy are more suited for extensive projects requiring advanced scraping capabilities, such as crawling multiple pages or managing complex data extraction tasks.
Setting Up Your Environment
To delve into the world of web scraping with BeautifulSoup, it's paramount to ensure your environment is properly prepared. Think of it like setting a stage for a play; if the backdrop isnāt right, the performance might falter. A well-configured environment makes your life easier when you start scraping data from websites, and helps in avoiding unnecessary headaches down the road. Here, we explore the essentials that lay the groundwork for a smooth experience while using BeautifulSoup.
Pre-requisites for Installation
Before you can even think about scraping, you ought to have a system that has Python installed. This is crucial since BeautifulSoup is a library designed to work with Python. A few other tools might come in handy:
- Python 3.x: Ensure you have the latest version. Python 2.x is slowly fading into oblivion.
- Pip: This package manager for Python helps in easily installing BeautifulSoup as well as other libraries.
- A Text Editor or IDE: While you can use Notepad, opting for an integrated development environment like PyCharm or VSCode offers features that enhance your programming experience.
Itās also good to have a fundamental understanding of Python syntax and a grip on how libraries work in general. If you analyze the requirements, they pave the road for a seamless installation processābackward compatibility is often a pain, so staying updated helps prevent that.
Installing BeautifulSoup
Installing BeautifulSoup is a piece of cake if you follow the steps closely. You can execute the installation via the command line. The breakthrough moment comes when you discover the simple command to pull in the library. Hereās how it works for a standard installation:
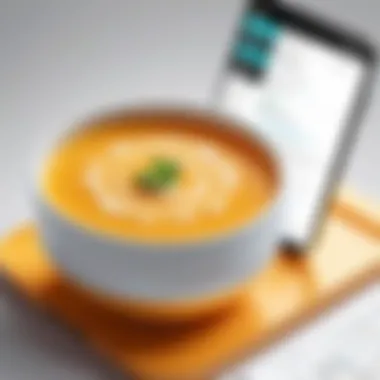
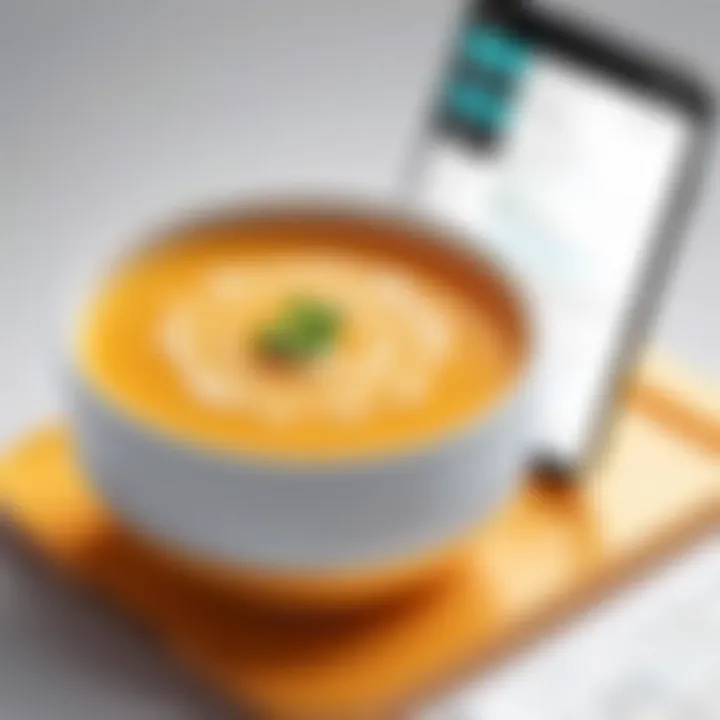
In some cases, you might want to scrape sites that render content dynamically using JavaScript. In such cases, it can be useful to install an additional package, lxml, to handle parsing:
With these commands, BeautifulSoup and the required parser are integrated into your environment. Itās that easy! Just remember, if you run into errors, take a moment to read the output. Sometimes, dependencies might require updates.
Configuring Your Python Environment
Once you have all the necessary packages in place, configuring your Python environment is a walk in the park. The environment might refer to your directory structure or the virtual environment settings for managing dependencies. Here are a few things to consider:
- Set Up Virtual Environments: Itās wise to create a virtual environment for your projects. This isolates your project dependencies and prevents any version clashes.
- Directory Structure: Organize your files effectively. A clean structure makes referencing and managing your files much easier.
- Add Your PATH: If you commonly run scripts, consider adding your Python executable to your system PATH. This makes it easier to run Python commands without typing out the full path.
- For example, your project could look like this:
Creating the right environment is crucial, both for current functionalities and potential future developments. By laying the right foundations, you set yourself up for success.
"The key to success is to start before you are ready."
In summary, setting up your environment for BeautifulSoup involves ensuring Python is properly installed, executing straightforward installation commands, and organizing your coding workspace. These are building blocks for any successful web scraping project.
Parsing HTML Documents
Parsing HTML documents stands at the heart of web scraping, forming the bridge between raw data and actionable insights. In the realm of data extraction, without an appropriate parsing mechanism, the wealth of information hidden within a website's structure would remain unexploited. This process essentially involves navigating through the often chaotic arrangement of HTML elements to extract specific data points. The importance of parsing cannot be overstated; it allows users to identify and manipulate the desired data with precision.
One key benefit of mastering HTML parsing is the ability to extract exactly what you need. When using BeautifulSoup, developers can sift through convoluted HTML layouts, unearth hidden nuggets of data, and convert them into a structured format that is easy to analyze. Practitioners should keep in mind the intricacies of different HTML structures when setting up their scripts, as variations can affect the extraction process.
Loading HTML Content
In order to begin the extraction journey, the first step is to load the HTML content either from a URL or a local file. When scraping the web, one common practice is fetching the HTML using Python's library, which simplifies the process of making HTTP requests.
Here's a brief example:
In this snippet, the method retrieves the webpage content, which is then stored in the variable. Should you opt for local files, the process similarly involves reading the file into a string format. This initial step sets the stage for the next phases of processing that follow.
Creating a BeautifulSoup Object
With the HTML content ready in hand, the next logical step is to create a BeautifulSoup object. At its core, this object serves as the primary interface through which you will interact with the scraped data. The syntax is straightforward and can be summarized as follows:
This constructs a object that contains the parsed HTML, ready for exploration and extraction. Itās important to decide on a parser, as BeautifulSoup supports multiple options, such as lxml and html5lib. Each parser comes with its own strengths, and selecting the right one depends largely on the structure of the HTML being processed.
Navigating the Parse Tree
After establishing a BeautifulSoup object, you enter the realm of the parse tree. Navigating this tree effectively allows you to find the desired elements within the HTML document, serving as the backbone of your data extraction efforts.
Finding Tags
Finding tags is perhaps one of the most fundamental aspects when dealing with BeautifulSoup. This feature allows users to specify and retrieve tags that are pertinent to their data goals. For instance, if you wanted to extract all anchor tags from a page, youād simply execute:
The beautiful part about this method is its flexibility. You can search for tags by name, class, or even custom attributes, making it a potent tool in your scraping toolbox. However, some negatives do exist, such as if the HTML structure changes, your finding mechanism might break.
Navigating Siblings and Parents
Understanding how to navigate siblings and parents further enhances the capabilities at your disposal. By leveraging methods like or , you can move fluidly through the parse tree. This becomes especially useful in instances where the required information is not directly encapsulated within a specific tag but instead is located in adjacent or relating tags.
An example could be seeking additional details related to a specific item:
This method of navigation is popular because it reflects the hierarchical nature of HTML structures, allowing developers to write more dynamic and powerful scraping scripts that can adapt to variations in HTML markup.
Searching by Attributes
The ability to search by attributes is another defining feature that enhances data extraction workflows. If tags have specific attributes like classes or ids, you can filter your queries to target these attributes specifically. For example, if you want to find a tag with a specific class, you could use:
This targeted searching not only speeds up the data retrieval process but also significantly increases the accuracy and relevance of the extracted information. Itās worth noting, however, that such queries might lead to empty results if the expected attributes are not present in the HTML, leading to potential pitfalls in your extraction logic.
Mastering the art of parsing HTML documents is ultimately about developing a keen eye for structure and understanding the idiosyncrasies of each webpage you encounter.
Data Extraction Techniques
When dealing with web scraping, data extraction techniques play a crucial role in determining how effectively one can gather usable information from various web pages. Without these techniques, the entire scraping process could become a tedious endeavor, yielding inconsistent or irrelevant data. By mastering the nuances of data extraction, users gain the ability to fetch precisely what they need, streamline their data collection processes, and ultimately make better informed decisions based on accurate information. The following sections explore key methods: extracting text from tags, retrieving attributes, and working with lists and dictionaries.
Extracting Text from Tags
Extracting text from HTML tags is often the first step in data scraping. Most webpages contain text within specific HTML elements, such as headings, paragraphs, and lists. BeautifulSoup simplifies this task. The beauty of BeautifulSoup lies in its ability to access the text content of any tag without being bogged down by the intricacies of HTML syntax.
For example, if you want to grab all the text inside paragraph tags that describe a product on an e-commerce page, you would do something like this:
This snippet illustrates how to pull text from every tag found on a webpage. The use of is particularly handy because it concatenates all the text nodes from the selected tag, resulting in clean data thatās easy to read and analyze.
Retrieving Attributes
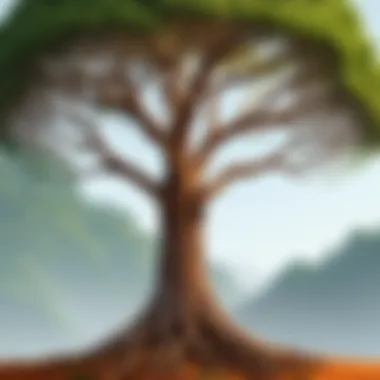
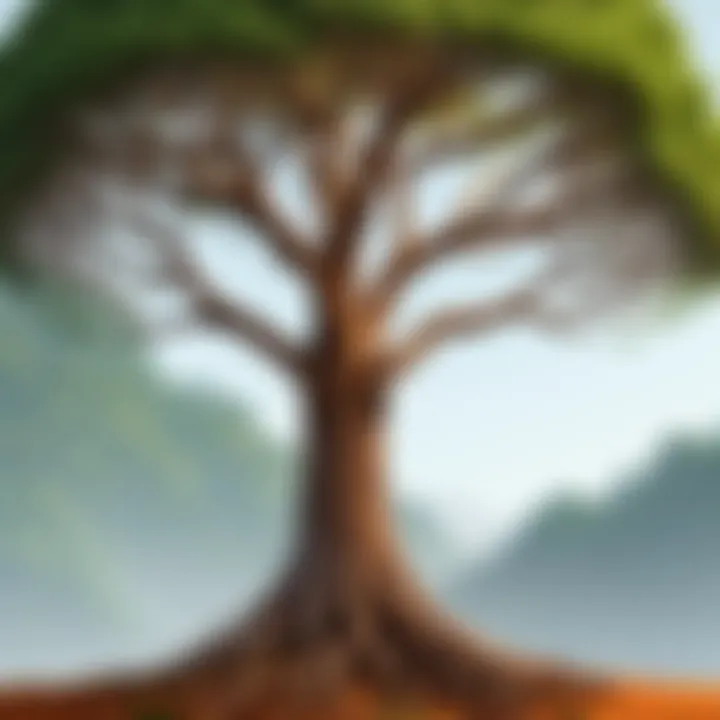
Another vital aspect of data extraction involves retrieving attributes from HTML tags. Attributes store supplementary information about the tags, like source links in image tags (), or class names that might provide insight into the styling and categorization of web elements.
To extract attributes, one can utilize BeautifulSoupās access methods. For instance, pulling an imageās source link resides within the attribute:
In the example above, locates all the image tags, and by calling , you can directly console out the URLs for each image. This capability extends beyond images; attributes can also be accessed from any HTML tag, allowing for comprehensive data harvesting from various elements.
Working with Lists and Dictionaries
When web scraping data, you might find it beneficial to organize the data into lists and dictionaries for further processing. Lists offer a straightforward way to compile sequences of similar items, while dictionaries allow for key-value pair management. This can be particularly useful when scraping product details.
Consider an example where each product scraped from an e-commerce website has a name, price, and description. To collect this information systematically, you can use a combination of lists and dictionaries:
This method builds a list of dictionaries, where each dictionary represents an individual product and its attributes. It lays a foundation for later analysis, storage, or visualization of the scraped data.
Handling Different HTML Structures
When it comes to web scraping, not all HTML is created equal. Websites vary vastly in structure, and thatās where the importance of handling different HTML structures comes into play. It can feel like navigating a labyrinth without a map, especially if the HTML is messy or non-conforming to standards. In this section, we will venture into some of the core challenges faced during web scraping and how BeautifulSoup stands as a reliable ally in overcoming these obstacles.
Parsing Invalid HTML
Let's face it, the internet is a wild place. Sometimes, the HTML you encounter is less than idealāthink missing closing tags, mismatched elements, or downright wrong nesting. Thankfully, BeautifulSoupās parser is built to manage these kinds of scenarios with relative ease.
When parsing invalid or malformed HTML, you can leverage BeautifulSoup's ability to correct structural errors while creating the soup object. For instance, you might find an HTML snippet that looks like this:
It might not pass validator.w3.org because the `` is missing a closing quote. However, the BeautifulSoup parser doesn't throw a fit over this flaw; it adapts its grasp on the coerced structure, liberating you from an exhaustive error-checking process.
To ensure efficiency, simply use the option while initializing your soup object. This way, any flaws in HTML become mere speed bumps on your road to data extraction.
Dealing with Dynamic Content
Dynamic content is the 'showbiz' aspect of web scraping. This refers to content that is generated on-the-fly by JavaScript, and letās be honest, it can make your life much more complicated. Standard HTTP requests may not capture the dynamic elements served by JavaScript.
For such instances, BeautifulSoup pairs well with requests and selenium, a tool that automates browser interactions. While BeautifulSoup will help in dissecting the soup, selenium rolls up its sleeves to fetch the rendered HTML containing content generated after page load.
For example:
- Install selenium alongside the BeautifulSoup library.
- Use selenium to interact with the web page as if you were a real user.
- Fetch the updated HTML and pass it to BeautifulSoup for further analysis.
This workflow allows you to extract data buried in dynamic content without losing your sanity over JavaScriptās tricks.
Scraping Tables and Lists
Many websites present information in tables or lists, which are often vital formats for data organization. Both structures can be a walk in the park with BeautifulSoup, provided you understand a few techniques.
When scraping tables, it's essential to identify the tag, along with its and tags which hold the content. A sample structure looks like this:
With a well-structured HTML table, data extraction becomes systematic. Iterate over the table rows to collect data and store it in a dictionary or a list for easy access later. For lists, target the , , and individual tags as your markers.
Using BeautifulSoup, you can effortlessly navigate and extract data. Using methods like , you can fish out the necessary data in no time at all.
"Navigating inconsistent HTML structures may seem daunting, but with BeautifulSoup's robust parsing capabilities, you can transform a chaotic landscape into a structured data experience."
End
Handling different HTML structures is a quintessential skill in web scraping. Whether you're parsing invalid HTML, dealing with dynamic content, or scraping tables and lists, BeautifulSoup offers the tools you need to tackle these challenges. By understanding how to manipulate the soup and embracing the possibilities of different tag structures, you set the groundwork for impressive data extraction capabilities.
Making your way through the webās varying HTML formats doesnāt have to feel like a cat-and-mouse chase. With just a small investment in understanding and practice, the gratification of having well-structured data at your fingertips is but a few lines of code away.
Application of BeautifulSoup
The importance of applying BeautifulSoup effectively in web scraping cannot be overstated. This library simplifies the process of extracting relevant data from various websites ā a task that, without the right tools and knowledge, can quickly turn into a frustrating endeavor. With its duck-to-water approach to handling HTML and XML, BeautifulSoup enables usersābe they newcomers or seasoned developersāto parse complex web structures fluidly. It's a game changer in the realm of data retrieval, making it not just a useful library but a necessary aid in todayās data-driven world.
Building a Simple Web Scraper
Creating a basic web scraper with BeautifulSoup is akin to building a sandcastle at the beach: itās straightforward once you know the right steps. First, ensure you have the relevant packages installed in your Python environment. Start with making a simple request to grab the HTML of the page you want to scrape. Hereās how you can do that:
Once you have the HTML content as a BeautifulSoup object, the next step involves using its powerful functions to navigate the parse tree. Need to extract all the headings? Use , , etc. This neat trick will help you collect the data youāre after without breaking a sweat. The beauty is in the directness of it all.
Automating Data Retrieval
In an age where routine tasks are often automated, BeautifulSoup allows you to set up scripts that perform regular data retrieval effortlessly. By utilizing scheduled jobs, you can automate the scraping process, thereby preserving your sanityāand your time. By integrating libraries such as or , you can run your scraping scripts at specified intervals. Hereās a brief example:
By crafting automated data retrieval scripts, you stay ahead of the curve, ensuring that you always have the most up-to-date data at your fingertips without the eyeball-rolling task of manually re-running your code.
Integrating with Pandas for Data Analysis
When the scraping dust settles and youāve gathered your data, the next logical step is analysis. BeautifulSoup pairs beautifully with Pandas, the data analysis powerhouse in the Python ecosystem. Once youāve extracted your data, loading it into a Pandas DataFrame makes manipulation and analysis both seamless and efficient. Hereās a small snippet to illustrate:
The integration opens the floodgates to further data processing ā visualizations, reports, and data cleaning can all become part of your workflow. By mastering the art of BeautifulSoup alongside Pandas, you position yourself to handle and analyze vast amounts of web data efficiently, bridging the gap between information and actionable insights.
Remember, knowledge without application is like a car without gas; it ain't going nowhere.
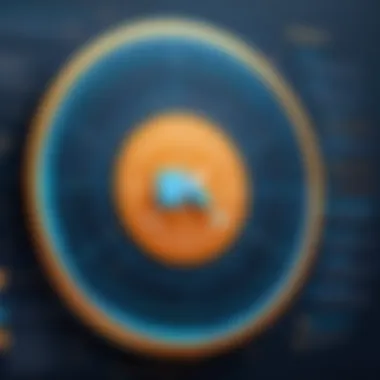
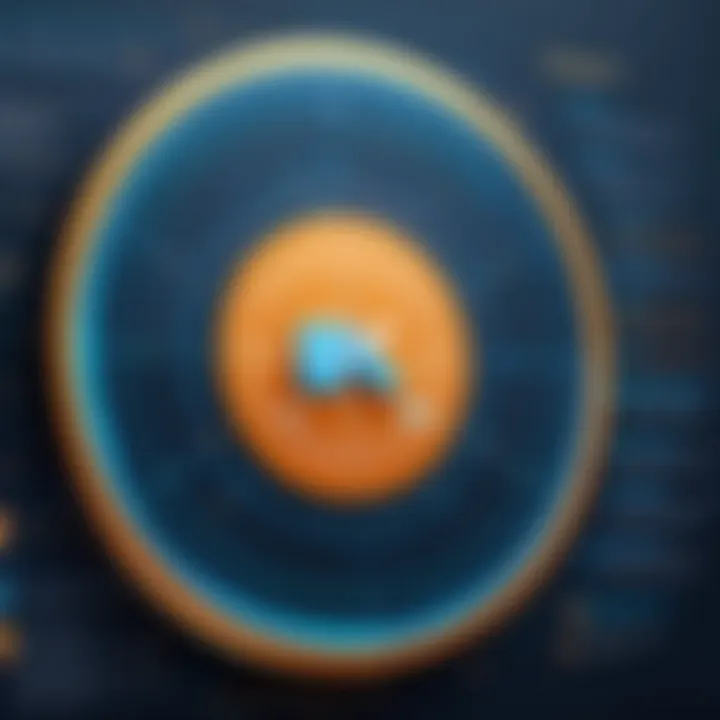
Best Practices and Ethical Considerations
When it comes to web scraping, adhering to best practices and ethical considerations is crucial. Not only does it ensure a smoother scraping experience, but it also contributes to a more responsible digital environment. As developers and data enthusiasts, understanding the nuances of web scraping behaviors can save you from legal troubles and foster respect within the web community. This section will explore the guiding principles that should govern your web scraping endeavors, ensuring that you make the most of BeautifulSoup while doing so ethically.
Understanding the Legal Aspects of Web Scraping
Web scraping exists in a gray area of legality. While the act itself might not always be illegal, the way you approach it can have significant implications. It's essential to grasp the concept of "fair use" in this context. Fair use typically allows for the limited use of copyrighted material without required permission from the rights holders.
You need to keep several factors in mind:
- Data Ownership: Be aware of the data you are accessing. Some websites explicitly prohibit scraping in their terms of service. Ignoring this could lead to severe penalties.
- Copyright Laws: Even if a webpage is publicly accessible, its contents may still be under copyright protection. Reusing or redistributing content without permission could infringe copyrights.
- Legal Precedents: Cases like the one involving LinkedIn and HiQ Labs highlight the ongoing debates about web scraping legality. HiQ argued that scraping publicly available data from LinkedIn was acceptable, but the outcome of the case can influence future regulations.
Understanding these aspects helps you navigate the legal landscape and mitigate risks associated with scraping.
Respecting Robots.txt and Rate Limiting
Websites generate their own rules signaling how their data can be accessed via a file known as "robots.txt". This file serves as a guide for web crawlers, indicating which parts of the site should not be scraped. Always check for this file before you start scraping a website to ensure compliance with their directives. Ignoring these guidelines could be not only unethical but, potentially, illegal.
Additionally, respecting rate limits is crucial. Bombarding a server with requests in a short span can overload systems and can be considered malicious intent. Implementing sleep timers between requests mimics human-like behavior, thus preventing inadvertent denial-of-service scenarios. Hereās a simple example of how you might structure your code when scraping:
Ensuring Data Accuracy and Integrity
Accuracy in your scraped data is paramount. Incorrect or misleading information can not only compromise your results but also tarnish your reputation as a data scientist or programmer. Here are steps to ensure the precision of the data you gather:
- Validate Data: Implement checks after data extraction to validate its integrity. For instance, if you're scraping prices, confirm they fall within reasonable ranges or formats.
- Update Frequency: Data can change rapidly, especially in dynamic markets. Regularly review and update your scraped datasets to ensure they remain relevant and precise.
- Error Handling: Incorporate robust error handling in your scraping scripts. This will not only assist in keeping your data clean but also help in diagnosing issues during the scraping process.
Remember: Transparency in your methods is your best ally. Disclose your scraping practices, respect data sources, and maintain a high ethical standard.
Establishing best practices and understanding ethical considerations is foundational for anyone delving into web scraping. Armed with this knowledge, practitioners can not only perform efficient scrapes but also contribute positively to the digital landscape.
Troubleshooting Common Issues
When engaging in web scraping with BeautifulSoup, it's not all smooth sailing. Often, you may encounter various bumps in the road that challenge your endeavor. Navigating these hiccups is crucial for maintaining the efficiency and effectiveness of your scraping endeavors. Understanding how to troubleshoot common issues can save you a lot of time and prevent frustration down the line.
Familiarity with potential pitfalls not only enhances your technical skills but also empowers you to tackle challenges head-on. This section delves into the common errors and exceptions you might face, how to handle connection issues, and techniques for optimizing performance. It aims to prepare you for the unexpected, ensuring your scraping projects stay on course.
Common Errors and Exceptions
In the world of BeautifulSoup, errors can crop up silently. You might find yourself scratching your head when you get an unexpected output or an outright crash. Common errors often relate to HTML parsing issues or incorrect usage of methods.
Some frequent errors include:
- AttributeError: This occurs if you attempt to access an attribute that doesnāt exist on a BeautifulSoup object. Ensure youāre calling methods on the right objects.
- IndexError: This shows up when you attempt to access an index thatās outside the bounds of a list. Often, this comes from attempting to extract data from a tag that isn āt present.
- HTTPError: If the resource isnāt available, you might see this message. This often indicates connection issues or a problem with the URL.
To mitigate these, thorough error handling using try-except blocks can be a lifesaver. For instance:
This will help you pinpoint errors quickly without stopping your entire script.
Handling Connection Issues
A key aspect of effective web scraping is managing connection issues. Sometimes, your connection to the target website may be unstable or interrupted. This is particularly true when a site imposes rate limits or blocks IPs after a certain number of requests.
A few common connection hiccups include:
- Timeout errors: Your request takes too long, either due to server slowness or network issues.
- Connection Resets: This can happen if the server forcibly closes the connection.
- 403 Forbidden Errors: This indicates that your scraper may not have the necessary permissions to access the content.
To navigate these issues, consider the following strategies:
- Implement retry mechanisms that allow your script to attempt a request again after a defined wait time. For example, using libraries like allows you to set this up easily.
- Use headers and user-agents to mimic regular browser traffic, as some sites may block parsers that look suspicious.
- Incorporate proper error handling by catching specific exceptions and acting accordingly.
Performance Optimization Techniques
Once you get past the initial troubleshooting steps, you might find your scraper slowing down. Optimizing performance becomes essential, particularly if you are scraping large volumes of data.
Here are some strategies to enhance performance:
- Use a session object from the requests library which can help keep connections alive. For example:
- Limit the depth of the parse tree navigation. Scraping overly nested elements can slow down parsing significantly.
- Optimize parsing by using specific methods in BeautifulSoup. For example, is more efficient than in specific scenarios where you expect multiple instances of an element.
- Reduce duplicate requests by caching results from previous requests or using local data files to store re-scraped data.
"By proactively addressing these common issues, you not only streamline your scraping process but also improve the reliability and speed of your data collection efforts."
Culmination
When tying together the insights gleaned from a deep dive into BeautifulSoup, the conclusion is not merely a wrap-up but rather a crucial juncture that encapsulates the significance of what has been discussed. It serves as a reminder of why mastering web scraping techniques using this library is invaluable in today's data-driven world.
Recap of Key Techniques
To reiterate the essentials, several core techniques emerged throughout the article, which should resonate with both beginners and seasoned coders alike:
- Loading HTML Content: Understanding how to load and access the HTML content you wish to scrape is foundational. Employing libraries like to obtain the webpage in question is a step many overlook, but one that sets the stage for effective scraping.
- Navigating the Parse Tree: BeautifulSoup excels at allowing users to navigate through the parse tree of an HTML document gracefully. This includes methods to find tags efficiently, and also to cleverly navigate through siblings and parents without getting tangled.
- Data Extraction Methods: Extracting relevant data points from a webpage is the crux of web scraping. Techniques like retrieving text from tags or attributes has been shown to yield results that can be formatted into lists or dictionaries, catering to further analysis or storage.
- Handling Edge Cases: Web scraping is not always straightforward, especially when dealing with complex and dynamic content. It's vital to be adaptable, employing methods to parse imperfect HTML or understanding how to manage content generated by JavaScript.
Reflecting on these techniques not only solidifies your grasp of BeautifulSoup, but also lays a robust foundation for more advanced data scraping endeavors.
Future Trends in Web Scraping
The landscape of web scraping is continually evolving. As machine learning and AI technologies proliferate, so will the implications for automated scraping. Here are some trends that may shape the future of this field:
- AI-Powered Scraping Tools: Expect to see tools that leverage AI to intelligently understand website structures, capable of predicting where data resides and how best to extract it.
- Improved Compliance Mechanisms: As legislation around data privacy grows more stringent, the integration of features that respect directives will be paramount, aiming to keep scraping within legal bounds while still yielding desired data.
- Enhanced Data Quality and Cleaning Techniques: Future tools may include advanced preprocessing steps which allow scraped data to be cleaned remotely or in real-time, ensuring whatās retrieved is accurate and easy to use.
These trends underscore the need for ongoing education and adaptability within the tech community as the methods of scraping will need to evolve alongside the internet itself.
Further Resources for Learning
For those eager to extend their knowledge beyond what has been covered, a wealth of resources exists:
- Documentation: Always a good starting point. Check out the BeautifulSoup documentation for a deep dive into its capabilities.
- Online Courses: Platforms like Coursera or Udemy host comprehensive courses tailored to web scraping, often including project-based approaches that allow for practical application.
- Community Forums: Engaging with communities on sites like Reddit or StackOverflow can provide insights and answers to specific challenges you face in your journey.
- Books: Titles such as "Web Scraping with Python" by Ryan Mitchell are fantastic for those who appreciate structured, in-depth learning.
By utilizing these resources, one can not only deepen their understanding of BeautifulSoup and web scraping but also stay up to date with the rapidly evolving data landscape.